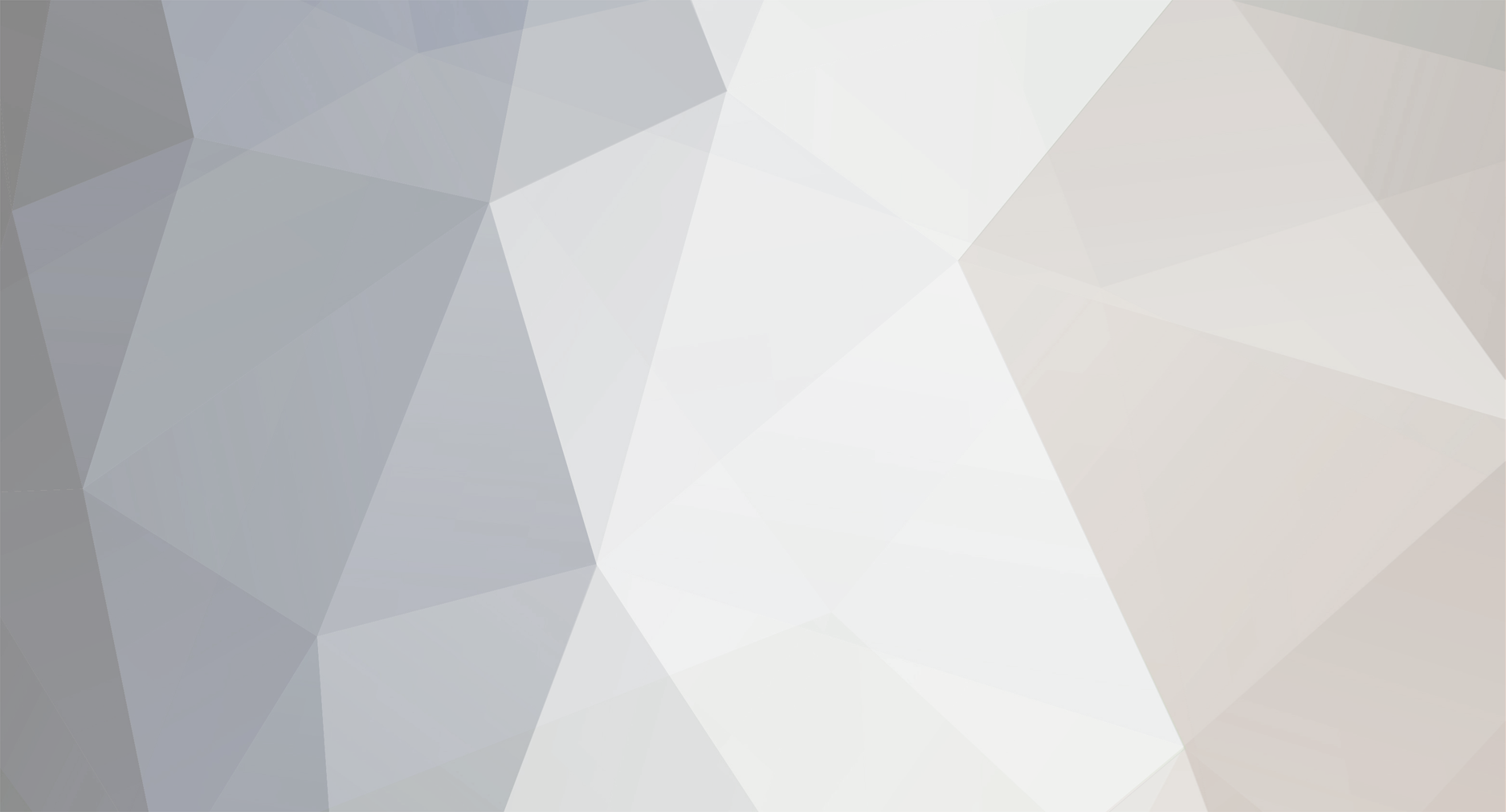
dennismonsewicz
Members-
Posts
1,136 -
Joined
-
Last visited
Everything posted by dennismonsewicz
-
Well I am trying to write a script that takes a string and splits it after a certain amount of characters but if the split occurs in a word then do not split until the next whitespace.
-
Is there anyway to check if the next character in a string is a whitespace?
-
I am trying to use the mysql command LOAD DATA INFILE and then spit out the results from the DB and I get nothing. No errors or anything. <?php mysql_connect('localhost', 'username', 'password')or die(mysql_error()); mysql_select_db('db_name')or die(mysql_error()); $insert = mysql_query("LOAD DATA LOCAL INFILE 'myfile.csv' INTO TABLE mytbl FIELDS TERMINATED BY ',' LINES TERMINATED BY '\n' (`make`, `model`, `deductible`, `published`)") or die(mysql_error()); if($insert) { $query = mysql_query("SELECT * FROM mytbl"); while($row = mysql_fetch_object($query)) { $results .= $row; } echo '<pre>'; print_r($results); echo '</pre>'; } else { echo 'NOPE!'; } ?>
-
breaking string apart question
dennismonsewicz replied to dennismonsewicz's topic in PHP Coding Help
Awesome! Thanks! I will take a look at these examples! -
breaking string apart question
dennismonsewicz replied to dennismonsewicz's topic in PHP Coding Help
Ok so I got it working based on the latest solution... Basically I have a writable PDF that I am creating based on user generated information from the web. The PDF has a two part question and I am taking the user input from a textarea that I am then breaking apart and placing on the PDF in two separate parts. But my dilemma is what if the last part of the first section contains a word that breaks in half (IE: description, between, because, etc.) and then the word continues on the second line. I am wondering if I could split the last word on the first line and place a dash in the word to let others reading it know that the word continues on the second line... Make sense? Sorry if that is confusing... its hard to place what I need help on to words lol -
breaking string apart question
dennismonsewicz replied to dennismonsewicz's topic in PHP Coding Help
THANKS!!!! This works like a charm! -
I have a string I want to break apart at a certain limit and then set the two parts into different variables... I know I can use the explode functionality but am confused as to how to break the string apart... any suggestions?
-
Nevermind, figured it out! $array = array(); foreach($_POST as $k => $v) { $array[$k] = $v; } unset($array['submit']); echo '<pre>'; print_r($array); echo '</pre>';
-
I have a web form and upon submission I append all the $_POST values to an array but it is capturing the submit $_POST value... Is there anyway to strip that out of the array? Here is my code so far: $array = array(); foreach($_POST as $k => $v) { unset($_POST[array_search("submit", $_POST)]); $array[$k] = $v; } echo '<pre>'; print_r($array); echo '</pre>'; When I look at my results I am still seeing the submit post in the array
-
I found this http://josmykutty.blogspot.com/2008/02/php-function-remoteaddr-is-not-working.html Hope it helps
-
I am trying to create a jpeg image and its not saving the image to my server... // Create a 100*30 image $im = imagecreate(100, 30); // White background and blue text $bg = imagecolorallocate($im, 255, 255, 255); $textcolor = imagecolorallocate($im, 0, 0, 255); $ranstr = 'Hello World'; // Write the string at the top left imagestring($im, 5, 0, 0, $ranstr, $textcolor); if(imagejpeg($im, 'images/test.jpg') === TRUE) { echo 'SUCCESS!'; } else { echo 'FAIL!'; } The images folder is set to 777, any ideas?
-
Found what I was looking for: http://nadeausoftware.com/articles/2007/09/php_tip_how_strip_html_tags_web_page
-
Is there anyway to limit what comes back in a cURL call? Instead of returning the whole page, only returning part of it?
-
Awesome that worked! but I have a weird problem happening... $encArray = array( "claimID" => $_GET['barcode'], "dnumber" => $_GET['dnumber'] ); $text = ''; foreach($encArray as $k => $v) { $text .= escapeshellarg("$k=$v" . '\\n'); } echo $text; the expected out put is claimID=12345\ndnumber=235342434 but I am getting: claimID=213243131\n''dnumber=2563398716\n' There is a double quote between the first var and the second... is there anyway to get rid of that?
-
I always seem to get confused when I start working with an array where I want to append the results to the end of a string vs resetting the variable in a foreach statement. $encodeArray = array( 'claimID' => $_GET['barcode'], 'deviceNumber' => $_GET['deviceNumber'] ); foreach($encodedText as $k => $v) { $text = escapeshellarg($k . "=" . $v . '\n'); } $text = ''; I want each key/value pair to show up in one string but all together... sorry if that is confusing. Also, how do I echo out a literal \n instead of the line break actually occurring?
-
I am having to develop an AJAX request application in Mootools 1.1 because of our legacy systems and am having some problems with my code <script type="text/javascript" src="/media/system/js/mootools.js"></script> <script type="text/javascript"> window.addEvent('domready', function() { $('click').addEvent('click', function(e) { e = new Event(e).stop(); var input = $('test').value; var url = "http://www.myurl.com/test.php?mdn="+input; var ajax = new Ajax(url, { method: 'get', update: $('log') }).request(); }); }); </script> <input type="text" name="test" id="test" /> <p> <a href="#" id="click">Click Me!</a> </p> <p id="log"></p> When I run this code and click the link nothing happens... no error message, nothing... any ideas why? I tried searching for some mootools 1.1 demos/docs and found demos111.mootools.com, and followed their tutorial line for line but cannot get my code to run...
-
Anyone? I went back to another solution but for future knowledge how could I accomplish this with my code?
-
I wrote a quick function to generate form fields for me and am stuck on generating checkboxes. Code: function fieldGenerator($title, $fieldName, $type="text", $opts="") { switch($type) { case "submit": $input = '<input type="' . $type . '" name="' . $fieldName . '" value="' . ucwords($fieldName) . '" />'; break; case "checkbox": $input = '<input type="checkbox" value="text" />'; foreach($opts as $name => $value) { //$input .= '<input type="checkbox" value="' . $value . '" name="phoneproblem" />'; } break; case "text": default: $input = '<span>' . ucwords($title) . '</span>'; $input .= '<input type="' . $type . '" name="' . $fieldName . '" id="' . $fieldName . '" />'; break; } return $input; } $ckOptsArray = array( "Lost" => "lost", "Stolen" => "stolen", "Damaged (Dropped, Liquid Damage, Broken, etc)" => "damaged", "Malfunctioning" => "malfunctioning" ); $inputArray = array( "Claim ID Number" => "barcode", "Wireless Device Number" => "deviceNumber", "First Name" => "firstName", "Last Name" => "lastName", "Daytime Phone Number" => "dayNumber", "Evening Phone Number" => "eveningNumber", "E-Mail Address" => "email", "Home Address" => "homeAddy", "City" => "city", "State" => "state", "Zip Code" => "zip", "Device Manufacturer" => "manufacturer", "Model" => "model", "checkbox" => "checkbox", "submit" => "submit" ); //print_r(getimagesize("images/image.jpg")); ?> <form action="generate.php" method="post" id="barcode"> <?php foreach($inputArray as $title => $name) { if($name != "submit") { echo fieldGenerator($title, $name, "text"); } elseif($name == "checkbox") { echo 'Hello!'; echo fieldGenerator($title, $name, "checkbox", $ckOptsArray); } else { echo fieldGenerator($title, $name, "submit"); } } ?> </form> The code above does not print out any checkboxes or the word "hello" in the if else statement. Instead a regular textbox is printed.. any suggestions?
-
simplexml_load_string problems
dennismonsewicz replied to dennismonsewicz's topic in PHP Coding Help
Sorry... my question would be more along the lines of what could cause such an error? -
I am getting the following error in my code: Premature end of file. [simplexml_load_string] My code posts to this page and returns results from a parsed XML string... I have never seen this error before... any ideas?
-
HAHA I could, but I wouldn't even know where to begin to code the algorithm for that kind of thing
-
I already have a 1D barcode PHP generator script... seems like if you want 2D you have to pay someone for their script. And I meant Data Matrix, not Dot Matrix lol
-
Is there anyway to produce a 2-D Dot Matrix barcode in PHP?
-
Thanks! that actually makes a lot of since. I appreciate your help!
-
I am trying to understand telling a function to return true or false and was wondering if the following code is right. function helloWorld($param) { if(isset($param)) { return true; } else { return false; } } if(helloWorld('test') != false) { echo 'it worked!'; } else { echo 'Nope!'; }