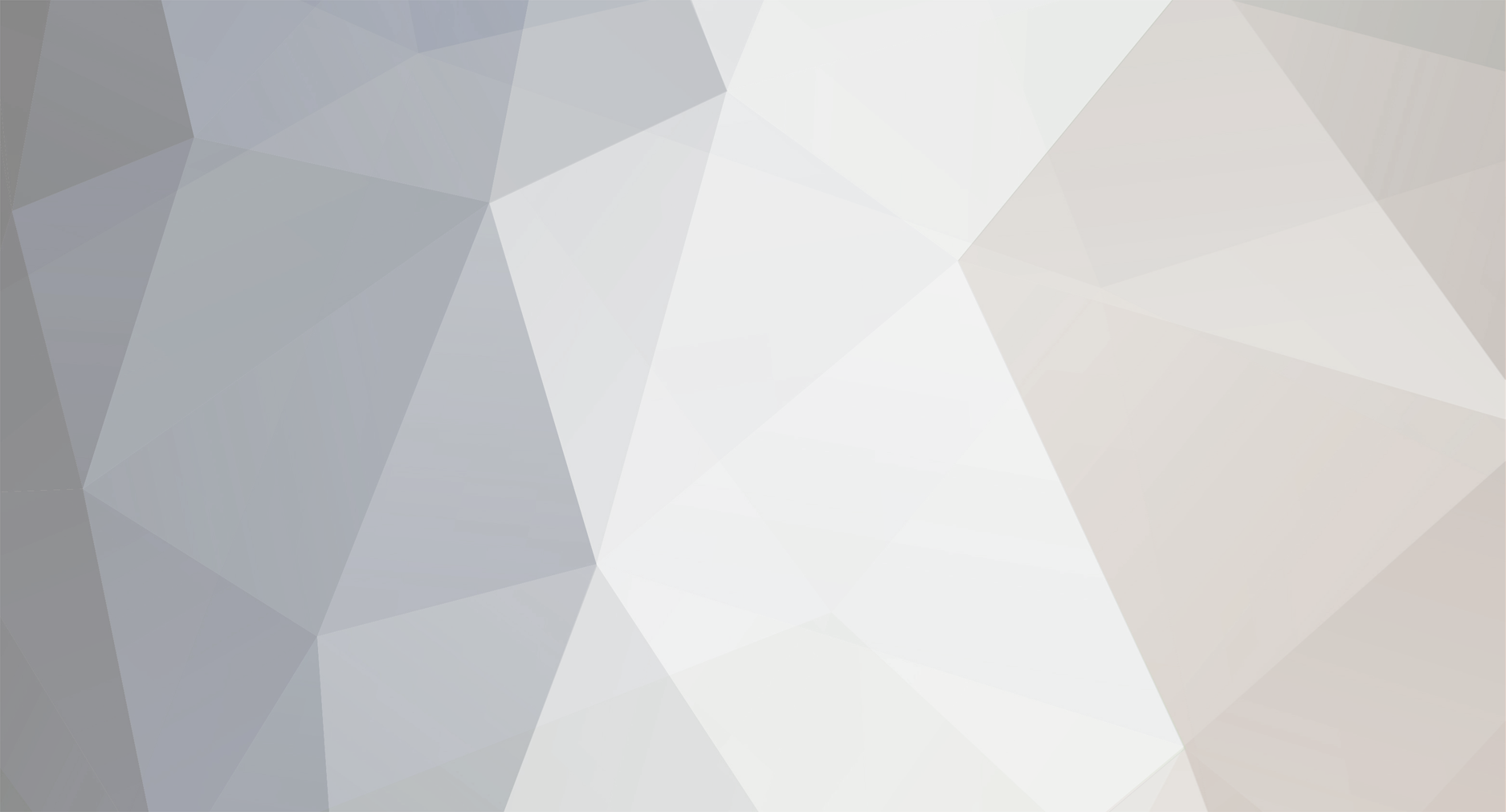
optikalefx
-
Posts
363 -
Joined
-
Last visited
Never
Posts posted by optikalefx
-
-
so using jQuery give each select box an attribute called div and make that equal to the ID of the box to hide
<select div='mydiv' onchange="myFunction(this)";>
<div id='mydiv'></div>
function myFunction(this) {
var which = $(this).attr("div");
$("#"+which).hide();
}
something like that. just be clever with attributes.
-
<body onload="alert('all')">
if it doesnt work check your error console (firefox) you probably have a syntax error stopping the code from executing.
-
if($("#door").style.top=="0px"){
does not evaluate. .style.top is a property of a DOM object. But $("#door") returns a jQuery object, not a DOM object.
Either use jquery $("#door").css("top") or use $("#door").get().style.top to evaluate.
-
Yes there is a way to easily do this
go to google and type in MDC Selection
anchorNode
anchorOffset
focusNode
focusOffset
You can use these 4 properties of the window.selection (even if there is no selection) and work it out that way. I don't remember exactly but i did this before and it worked.
Maybe also take a look at MDC Range
-
using jquery
("#idofdiv").mouseover(function() {
$(this).slideUp();
}
-
what part do you need help with?
pulling new records is as simple as pulling form the db and ordering by the date
select * from ticker order by date desc;
-
no prob dude.
I totally agree with learning without jquery first.
So Ill give you the parts and you can put them together.
I already gave you the html and css in the last post. The idea here again, is to fit 2 images on top of eachother.
The next part is creating an interval
myInterval = setInterval('dofade()',20); // dont use var myInterval
then in function dofade() {
// get a reference to the image on top using document.getElementById("idofimg");
// change its object.style.opacity -= .1;
// add some if statment to make sure it stops at 0
// when it gets to 0, use clearInterval(myInterval);
}
So when you call that interval it will fade out the first image, and since the first image is already on top of the 2nd one, the 2nd one will look like its fading in.
Of course you can make a 2nd interval, or even add more into the function, that fades the bottom in as the top one goes out. Just keep in mind the more work you make the browser do the slower and choppier it is.
Good luck man! Post back if you have any questions. If you can't get it, hit me up on youtube and ill make a video of me fixing it. youtube.com/optikalefxx
-
my first question would be, why not use jquery.
My answer would then, be you need to make a function that does what jquery fade does. Basically a setInterval timer that changes the opacity over time. The key here is that you need the parent element relative position. and the two images absolute, with z-indexes set.
<div style='position:relative'>
<img style='position:absolute;top:0px;left:0px'>
<img style='position:absolute;top:0px;left:0px'>
</div>
this way the images are on top of eachother. Now fade one out and the other will show.
-
Bump, I can't find anything on this guys.
Anyone had a similar issue?
-
So the way I looked at it, If i have a set of common data that will share common functions that the rest of the code won't use. And I have the intent of REusing this (object) then, an object should be used.
For example, I made a paypal buyer object. All the buyer information is related, and there are a few functions that pay requires so that the buyer information is correct. But no where else in the code are those functions needed. So an objected seemed the right choice.
-
Can someone explain to me why I should use OOP instead of procedure based code.
Im building a shopping cart and i created and Item object already and using it to hold data for each item. But this Object just has properties. I mean what methods could there possibly be for an item.
Why would I need a Cart object either? There will never be more than 1 instance of the cart running. So what are the advantages to putting all my functions inside of a class?
Thanks, and sorry if your offended by this, im trying to go OOP i just don't see why yet.
-
I have this overlay where it greys out the screen and there is HTML in there.
I have an input box, but in IE i can't really click it. I mean i can click something around it, and the cursor shows up, but nothing comes up in the box when i type. What quirk do i have to fix for IE that allows me to type in input boxes that float on top of the screen in a fixed position
-
So I know how to use all of the languages i listed. I know them pretty well actually. I just would like to learn some advanced techniques, like say designing a store database, or using ajax to create a site wide search. Basically out of the realm of how to use the languages but how to use them to complete complicated tasks.
lynda.com has some good stuff
-
Yea but are there any sites that give you some good guides? A lot of these advanced things require you to know a bunch of pre existing functions and tricks and techniques that i would have not else thought of.
-
I'm looking for some other resources other than forums to learn advanced website programming. Like javascript,php,mysql,air,ruby,flex,flash all that kind of stuff?
Thanks guys!!
-
Thanks! I didn't know you could set your own session folder. This is also a great way to test that I'm getting all my required information.
The php.ini file says I have to do my garbage cleaning with a cron job. Do I really have to do that or will it do that when i destroy the session?
-
Its possible, I use Powweb which has a ton of customers and I didn't pay extra for my own box, so I can only assume its shared.
-
I have session_start() at the top of every page. For some reason if I wait for more than a minute on any page, when I go to refresh the page it kicks me out. I have specific code that tests for a certain session variable, if it doesn't find it, then it kicks the user out. So why does waiting expire the session? If i just wait 30 seconds or so and refresh it works fine, but if i wait 2 or 3 minutes then when i refresh the session variable im checking for is gone.
I checked the PHPSESSID and there is no expiration on the cookie.
-
never mind, i took 5 minutes and figured it out. I was putting Quotes in front and at the end which was not correct. I'm an idiot.
-
Im beating my head against a wall here.
I am looping to form a sql string and this is the result of that
'INSERT INTO cal (company,type,corpParent,hasAflac,anotherAgent,hasHR,contact,position,decision,phone,email,fax,address,adrConf,benefits,totalEmps,fullTimeEmps,notes,whoSet,setDate,time) VALUES (\'wef\',\'wefwefwef\',\'1\',\'1\',\'1\',\'1\',\'qwefqwef\',\'qwefqwef\',\'1\',\'qwefqwef\',\'qwefqwfw\',\'qwefqwef\',\'qwefqwef\',\'1\',\'Health insurance\',\'qwefqwf\',\'qwefqw\',\'qwefqwef\',\'1\',\'1247861162\',\'1247736180\')'
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''INSERT INTO cal (company,type,corpParent,hasAflac,anotherAgent,hasHR,contact,po' at line 1
I put that EXACT sql statement into PHPmyAdmin and it works! So i have no idea what's going on. I test a SELECT to make sure the db was connected and it is. I tried with double quotes and not escaping and that that didn't work, i tried using the SET syntax and taht didn't work. WTF am I doing wrong?
Thanks!
:confused:
-
when you install a firefox extension the browser restarts. When that happens my extension pulls up a webpage that says thanks for downloading. Well that user had a session, and i want to subtract a counter from the database but i cant refer to that person unless i know who got to that page.
I solved it by setting a cookie that way when i restart just read the cookie and then get the database info that way.
-
So my entrie session uses the normal PHPSESSID cookie. I need that to last past the browser closing. How do i change the expiration of that cookie. Ive tried everything and i just get php errors or it doesnt set the cookie. Whats the right way to do it?
Thanks.
-
That line is in a php file that is included first on every page
-
This is really bothering me. I think I don't know something about sessions.
I have 5 php pages and a login php file. When you login it creates a session and stores 3 variables. first name last name and both together
part of login.php
if ($emailFound) { if ($passFound) { session_start(); $res = mysql_query("SELECT * FROM customerInfo WHERE email = '$email'") or die(mysql_error()); while($row = mysql_fetch_array( $res )) { $_SESSION['fName'] = $row['fName']; $_SESSION['lName'] = $row['lName']; $_SESSION['name'] = $_SESSION['fName'] . " " .$_SESSION['lName']; } header("Location: index.php"); } else header("Location: login.php?err=".WRONGPASS); } else header("Location: login.php?err=".EMAIL);
Then on ever page I have at the very very top included a file called session.php
at the top of every viewable page
<?PHP include('session.php');?>
contents of session.php
<?PHP if( isset( $_COOKIE['PHPSESSID'] ) ) { session_start(); $name = $_SESSION['name']; $lgn = "Welcome back ".$name."<br><a id='namez' href='http://www.mysite.com/logout.php'>Not ".$name."?</a>"; } else { $lgn = "<a id='namez' href='http://www.mysite.com/login.php'>Login</a>"; } ?>
And then later on i include a file that displays the info from the session
<?PHP include('top.php');?>
and inside of that file
<div id="namez"><?=$lgn?></div>
It works just fine for /index.php /page1.php and /page2.php
but it fails for /dir/page.php and /dir/page2.php
Even though those includes are both
<?PHP include('../session.php');?> //and <?PHP include('../top.php');?>
The issue is that with the pages that are a directory deep, the SOMETIMES work. And its not always the same ones. So i cant draw a conclusion as to why this is happening. if they were always not working, ok i can fix it. But they are sometimes working.
MOREOVER, its passing the isset for the cookie just fine on every page everytime. Its just forgetting the $_SESSION['name'] variable at times. Sometimes its there, sometimes it just says "Weclome back, Not ?"
Any ideas what Im missing in all this? Thanks
Openning web pages project
in Javascript Help
Posted
set firefox to open new windows as tabs
set a global counter var
var counter = 0;
then use javascript to setTimeout('openPage()',60000);
then the function
function openPage() {
window.open(myArray[counter]);
counter ++;
}
now just wrap this all in a for loop.
for (var i=0;i<=myArray.length;i++) {
// do the timeout here
}
You could also not have the global counter, and pass in the index of i as you loop. (probably more efficient)