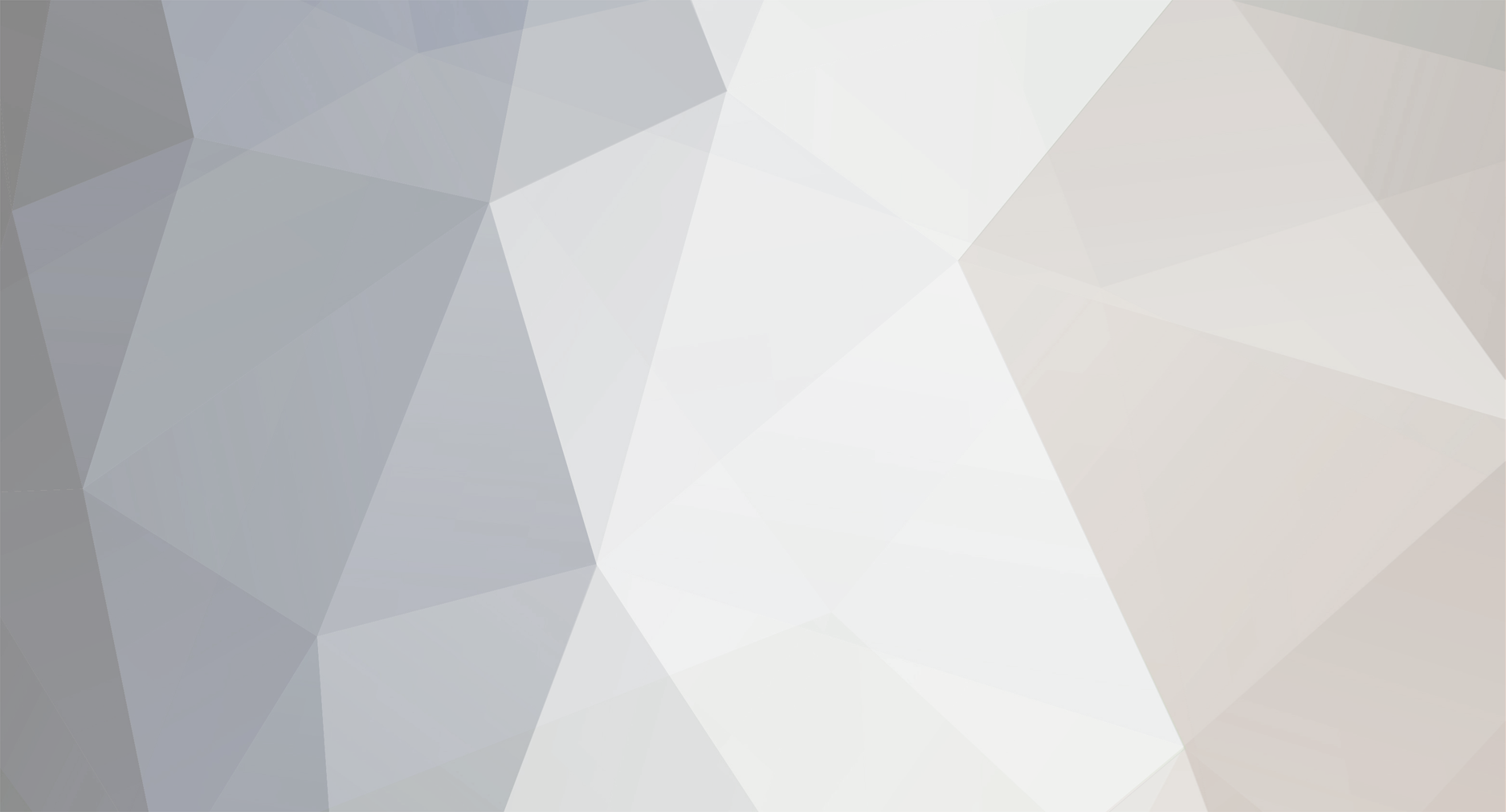
jonahpup
Members-
Posts
18 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
jonahpup's Achievements

Newbie (1/5)
0
Reputation
-
Hi there, For something simple like this, I usually use a basic 2 page submission form... something like the following... signup.php <html> <head> <title>Signup</title> </head> <body> <form action="thanks.php" method="post"> <table border="0" cellpadding="0" cellspacing="0"> <tr> <td> Name:</td> <td> <input type="text" name="name" /></td> </tr> <tr> <td> Email Address:</td> <td> <input type="text" name="email" /></td> </tr> <tr> <td colspan="2"> <input type="submit" name="Submit" value="Submit" /> | <input type="reset" name="Reset" value="Start Again" /></td> </tr> </table> </form> </body> </html> and then for error checking and email submission I use thanks.php <?php if(!isset($_POST['Submit'])) { header( 'location: http://www.domain.com/signup.php' ); //this just stops people being able to goto thanks.php // and submit blank forms } else{ //gather info from previous page $name = $_POST['name']; $email = $_POST['email']; //check that fields are not blank if(empty($name)) { echo("Name field is blank. Please enter your name"); } if(empty($email)) { echo("Email field is blank. Please enter your email"); } //check for valid email address function function CheckMail($email) { if (eregi("^[0-9a-z]([-_.]?[0-9a-z])*@[0-9a-z]([-.]?[0-9a-z])*\.[a-z]{2,4}$", $email)) { return true; } else { return false; } } if(!empty($name) && !empty($email)) { if(CheckMail($email) == false) // if email address is invalid { echo("Ut Oh... your email address is broken... please fix it!"); } if(CheckMail($email) == true) // if email address is valid { //send info via email. $sendTo = "youremail@yourdomain.com"; // your email address $subject = "new email submission"; //subject of email $message = " <html><head><title>New Email Submission</title></head> <body> <p>Name: $name</p> <p>Email Address: $email</p> </body> </html> "; $header = "FROM: <$email>\r\nContent-type: text/html; charset=ISO-8859-1\r\n"; mail($sendTo, $subject, $message, $header); echo("Thank you for your submission. You will now be added to our database"); } } } ?> I find this piece of code works really well... and because it sends emails in html you can play around with it and customise it however you want... Hope this helps...
-
Hi there... I am currently using an rss file to display news on my webpage. I would like to change it a bit so that it only displays news that is not expired. here is my rss file <?xml version = "1.0"?> <!DOCTYPE rss PUBLIC "-//Netscape Communications//DTD RSS 0.91//EN" "http://my.netscape.com/publish/formats/rss-0.91.dtd"> <rss version="0.91"> <channel> <title>My Title</title> <link></link> <description></description> <language>en-us</language> <item> <itemid>1</itemid> <title>Ground Breaking News</title> <link>http://www.google.com</link> <datePosted>22/01/2008</datePosted> <!-- displayed dd/mm/yyyy | Does not currently display in page--> <dateExpired>22/01/2008</dateExpired> <!-- displayed dd/mm/yyyy| Does not currently display in page --> <description>This is the description of this news article </description> </item> </channel> </rss> and here is the code I use to currently display the data on my page <?php class xItem { var $xTitle; var $xLink; var $xDescription; } // general vars $sTitle = ""; $sLink = ""; $sDescription = ""; $arItems = array(); $itemCount = 0; // ********* Start User-Defined Vars ************ // rss url goes here $uFile = "http://www.mydomain.com/3views.rss"; // descriptions (true or false) goes here $bDesc = true; // font goes here $uFont = "Verdana, Arial, Helvetica, sans-serif"; $uFontSize = "2"; // ********* End User-Defined Vars ************** function startElement($parser, $name, $attrs) { global $curTag; $curTag .= "^$name"; } function endElement($parser, $name) { global $curTag; $caret_pos = strrpos($curTag,'^'); $curTag = substr($curTag,0,$caret_pos); } function characterData($parser, $data) { global $curTag; // get the Channel information first global $sTitle, $sLink, $sDescription; $titleKey = "^RSS^CHANNEL^TITLE"; $linkKey = "^RSS^CHANNEL^LINK"; $descKey = "^RSS^CHANNEL^DESCRIPTION"; if ($curTag == $titleKey) { $sTitle = $data; } elseif ($curTag == $linkKey) { $sLink = $data; } elseif ($curTag == $descKey) { $sDescription = $data; } // now get the items global $arItems, $itemCount; $itemTitleKey = "^RSS^CHANNEL^ITEM^TITLE"; $itemLinkKey = "^RSS^CHANNEL^ITEM^LINK"; $itemDescKey = "^RSS^CHANNEL^ITEM^DESCRIPTION"; if ($curTag == $itemTitleKey) { // make new xItem $arItems[$itemCount] = new xItem(); // set new item object's properties $arItems[$itemCount]->xTitle = $data; } elseif ($curTag == $itemLinkKey) { $arItems[$itemCount]->xLink = $data; } elseif ($curTag == $itemDescKey) { $arItems[$itemCount]->xDescription = $data; // increment item counter $itemCount++; } } // main loop $xml_parser = xml_parser_create(); xml_set_element_handler($xml_parser, "startElement", "endElement"); xml_set_character_data_handler($xml_parser, "characterData"); if (!($fp = fopen($uFile,"r"))) { die ("could not open RSS for input"); } while ($data = fread($fp, 4096)) { if (!xml_parse($xml_parser, $data, feof($fp))) { die(sprintf("XML error: %s at line %d", xml_error_string(xml_get_error_code($xml_parser)), xml_get_current_line_number($xml_parser))); } } xml_parser_free($xml_parser); // write out the items <?php for ($i=0;$i<count($arItems);$i++) { $txItem = $arItems[$i]; ?> <li><a href = "<?php echo($txItem->xLink); ?>"> <?php echo($txItem->xTitle); ?></a><br /> <?php echo($txItem->xDescription); ?><br /><br /></li> <? } ?> what I am wanting to try and achieve, is to go through the rss file, and only display items that have not reached the expiry date. ie: if (todaysdate <= expirydate) { show item } but I dont know how to do that, and how to get the date information from the rss file to use it in the php... Can anyone help me?? Cheers Chris
-
Hi there, I am wanting to use the existing code I have for displaying an RSS feed, however, I want to only display the last 3 items that were added to the feed on a particular page. I have it working so that it will display every item in the rss file at present, but dont know how to only show the last 3 items... ie: itemid=4, itemid=5 and itemid=6. here is my RSS xml code: <?xml version = "1.0"?> <!DOCTYPE rss PUBLIC "-//Netscape Communications//DTD RSS 0.91//EN" "http://my.netscape.com/publish/formats/rss-0.91.dtd"> <rss version="0.91"> <channel> <title>MANZ Torque Newsletters</title> <link>http://www.manz.org.nz</link> <description></description> <language>en-us</language> <item> <itemid>1</itemid> <title>March 2007</title> <link>http://www.domain.com/documents/March07.pdf</link> <description>Description... </description> </item> <item> <itemid>2</itemid> <title>June 2007</title> <link>http://www.domain.com/documents/June07.pdf</link> <description>Description... </description> </item> <item> <itemid>3</itemid> <title>September 2007</title> <link>http://www.domain.com/documents/September07.pdf</link> <description>This is the description for September 2007. </description> </item> <item> <itemid>4</itemid> <title>October 2007</title> <link>http://www.domain.com/documents/September07.pdf</link> <description>This is the description for October 2007. </description> </item> <item> <itemid>5</itemid> <title>November 2007</title> <link>http://www.domain.com/documents/September07.pdf</link> <description>This is the description for November 2007. </description> </item> <item> <itemid>6</itemid> <title>December 2007</title> <link>http://www.domain.com/documents/September07.pdf</link> <description>This is the description for December 2007. </description> </item> </channel> </rss> The code I am using to parse and display these items is: <?php class xItem { var $xTitle; var $xLink; var $xDescription; } // general vars $sTitle = ""; $sLink = ""; $sDescription = ""; $arItems = array(); $itemCount = 0; // ********* Start User-Defined Vars ************ // rss url goes here $uFile = "http://www.domain.com/newsletters.rss"; // descriptions (true or false) goes here $bDesc = true; // font goes here $uFont = "Verdana, Arial, Helvetica, sans-serif"; $uFontSize = "2"; // ********* End User-Defined Vars ************** function startElement($parser, $name, $attrs) { global $curTag; $curTag .= "^$name"; } function endElement($parser, $name) { global $curTag; $caret_pos = strrpos($curTag,'^'); $curTag = substr($curTag,0,$caret_pos); } function characterData($parser, $data) { global $curTag; // get the Channel information first global $sTitle, $sLink, $sDescription; $titleKey = "^RSS^CHANNEL^TITLE"; $linkKey = "^RSS^CHANNEL^LINK"; $descKey = "^RSS^CHANNEL^DESCRIPTION"; if ($curTag == $titleKey) { $sTitle = $data; } elseif ($curTag == $linkKey) { $sLink = $data; } elseif ($curTag == $descKey) { $sDescription = $data; } // now get the items global $arItems, $itemCount; $itemTitleKey = "^RSS^CHANNEL^ITEM^TITLE"; $itemLinkKey = "^RSS^CHANNEL^ITEM^LINK"; $itemDescKey = "^RSS^CHANNEL^ITEM^DESCRIPTION"; if ($curTag == $itemTitleKey) { // make new xItem $arItems[$itemCount] = new xItem(); // set new item object's properties $arItems[$itemCount]->xTitle = $data; } elseif ($curTag == $itemLinkKey) { $arItems[$itemCount]->xLink = $data; } elseif ($curTag == $itemDescKey) { $arItems[$itemCount]->xDescription = $data; // increment item counter $itemCount++; } } // main loop $xml_parser = xml_parser_create(); xml_set_element_handler($xml_parser, "startElement", "endElement"); xml_set_character_data_handler($xml_parser, "characterData"); if (!($fp = fopen($uFile,"r"))) { die ("could not open RSS for input"); } while ($data = fread($fp, 4096)) { if (!xml_parse($xml_parser, $data, feof($fp))) { die(sprintf("XML error: %s at line %d", xml_error_string(xml_get_error_code($xml_parser)), xml_get_current_line_number($xml_parser))); } } xml_parser_free($xml_parser); // write out the items ?> <table style="width: 80%; text-align: left;" align="center"> <?php for ($i=0;$i<count($arItems);$i++) { $txItem = $arItems[$i]; ?> <tr> <td><span style="font-size: large; font-weight: bold"><a href = "<?php echo($txItem->xLink); ?>"> <?php echo($txItem->xTitle); ?></a></span><br /> <?php echo($txItem->xDescription); ?><br /> </td> </tr> <?php } ?> </table> What do I need to alter, to tell it to only show the last 3 posts?? ie: if there are 25 posts, it will only show itemid=23, itemid=24, itemid=25... if there are 9 posts it will show itemid=7, itemid=8, itemid=9 etc... so as posts are added to the feed and the itemid increases it still only shows the last 3?? Can anyone help?? Thanks in advance... Chris
-
[SOLVED] Displaying XML List in php page
jonahpup replied to jonahpup's topic in Other Programming Languages
Hey... cool... thanks for that... the only problem I have is my hosting server doesnt support php5 and therefore doesn't support SimpleXMLElement... is there any alternative way of doing this in php4?? -
Hi there... I have the following xml list <publications> <pub> <date>November 2007</date> <location>/pubs/nov07.pdf</location> </pub> <pub> <date>October 2007</date> <location>/pubs/oct07.pdf</location> </pub> <pub> <date>September 2007</date> <location>/pubs/sep07.pdf</location> </pub> <pub> <date>August 2007</date> <location>/pubs/aug07.pdf</location> </pub> </publications> What I am wanting to do is display only the current month, and the previous month, as a hyperlink on my page. I have no idea how to use xml in php. Can someone help me please??
-
Ok //This is test.php the page I initially goto. If I am logged in, I am shown the page... if not I am shown a message saying I must log in <?php $username = $_COOKIE['loggedin']; if (!isset($_COOKIE['loggedin'])) die("You are not logged in, <a href=login.php>click here</a> to login."); echo "You are logged in $username"; ?> <html> <head> </head> <body> <h2>This is a test page.</h2> <p>If you see this text, you are currently logged in.</p> <p><a href="logout.php">Click here to log out</a></p> </body> </html> // This is login.php - the page users are sent to to enter their login details. // Once username and password has been checked and is correct, I want the user to be able to go back to the page they came from... in this case test.php <?php session_start(); $_SESSION['referer'] = $_SERVER['HTTP_REFERER']; $ref = $_SESSION['referer']; ob_start(); include("config.php"); $pass = md5($_POST['password']); // connect to the mysql server $link = mysql_connect($server, $db_user, $db_pass) or die ("Could not connect to mysql because ".mysql_error()); // select the database mysql_select_db($database) or die ("Could not select database because ".mysql_error()); $match = "select id from $table where username = '".$_POST['username']."' and password = '$pass';"; $qry = mysql_query($match) or die ("Could not match data because ".mysql_error()); $num_rows = mysql_num_rows($qry); if ($num_rows <= 0) { echo "Sorry, there is no username or password with: <strong>".$_POST['username']."</strong><br>"; echo "<a href=login.html>Try again</a>"; exit; } else { setcookie("loggedin", "".$_POST['username']."", time()+(3600)); setcookie("username", "".$_POST['username']."", time()+(3600)); echo "Welcome: <strong>".$_POST['username']."</strong><br>"; echo "Return to the <a href=$ref>previous</a> page."; } ob_end_flush(); ?>
-
thanks caesar... same thing is still happening tho... basically what it is doing is: I goto newpage.php I am told that I need to login... so I click the "login" anchor which takes me to login.php I then enter my username and password, and click Login... I am then being taken back to login.php instead of newpage.php I need it so that I am taken back to newpage.php (or whatever page I have come from)...
-
you need to use mysql_select_db($db) or die ("Could not select database because ".mysql_error()); or similar to tell the server which database to look at.
-
ok... sorry dont mean to sound like retard, but im pretty new to php... how do I go about doing that???
-
Hi, I have a login script that is working great, except for one little bit... Once the user is logged in, I want to give them the option of returning to the page they came from... However, when the user is logged in, and they click on the return to previous page link, they are taken back to the login page they just filled in... how do I get around this?? Here is my code! <?php ob_start(); include("config.php"); $pass = md5($_POST['password']); // connect to the mysql server $link = mysql_connect($server, $db_user, $db_pass) or die ("Could not connect to mysql because ".mysql_error()); // select the database mysql_select_db($database) or die ("Could not select database because ".mysql_error()); $match = "select id from $table where username = '".$_POST['username']."' and password = '$pass';"; // Gather referrer info $ref = $_SERVER['HTTP_REFERER']; $qry = mysql_query($match) or die ("Could not match data because ".mysql_error()); $num_rows = mysql_num_rows($qry); if ($num_rows <= 0) { echo "Sorry, there is no username or password with: <strong>".$_POST['username']."</strong><br>"; echo "<a href=login.html>Try again</a>"; exit; } else { setcookie("loggedin", "".$_POST['username']."", time()+(3600)); setcookie("username", "".$_POST['username']."", time()+(3600)); echo "Welcome: <strong>".$_POST['username']."</strong><br>"; echo "Return to the <a href=$ref>previous</a> page."; } ob_end_flush(); ?>
-
hey guys, I am about to pull my hair out... im still pretty new to php, and I am looking for a GOOD php form to html email script... I have tried about 8 different scripts, including one I paid for, and I just cannot seem to get one that works properly... the issues I am having is either im getting errors when submitting the form, form submission is successful I get directed to the thankyou page and then never receive an email, or I will get one that I cant change the from name and address when it appears in outlook... Does anyone know where I can get a GOOOD script from that is going to allow me to send an email from my page, that will actually arrive, and in HTML??? Chris
-
<?php // Connects to your Database mysql_connect("localhost", "username", "password") or die(mysql_error()); mysql_select_db("database") or die(mysql_error()); //checks cookies to make sure they are logged in if(isset($_COOKIE['ID_my_site'])) { $username = $_COOKIE['ID_my_site']; $pass = $_COOKIE['Key_my_site']; $check = mysql_query("SELECT * FROM users WHERE username = '$username'")or die(mysql_error()); while($info = mysql_fetch_array( $check )) { //if the cookie has the wrong password, they are taken to the login page if ($pass != $info['password']) { header("Location: login.php"); } //otherwise they are shown the admin area else { ?> <html> <head> <link rel="stylesheet" href="styles/main.css" type="text/css" /> </head> <body> <div id="main"> <h1>Page 2</h1> <div style="border: 1px dashed black; padding: 1.5em"> <h3>You are now logged in and can view content on Page 2</h3> <a href="logout.php">Logout</a><br /> </div> </div> </body> </html> <? } } } else //if the cookie does not exist, they are taken to the login screen { header("Location: login.php"); } ?> Here is the code!
-
I tried that... but it will then redirect to index2.php when logged in, no matter what page they have come from... I need it so that whatever page a user comes from, once the user is successfully logged in, I want them to be returned to the page they came from... ???
-
*looks embarrassed and confused* How?? sorry I am new to php and get messed up with the syntax...
-
Ok... after getting some really good help on here yesterday, I decided I would ask for a bit more help... I have a 3 page (index.php, login.php, and logout.php) user login script which connects to my user database to check username and password for user logging in. if you have a look at www.350finale.co.nz/test and use the username: test and password: test you will see how it all works... What I want to know is, if I directly type in www.350finale.co.nz/test/page2.php it will redirect me to the login page (unless I am already logged in), which is what I want, however, once I have logged in, I want it to take me back to page2.php not index.php like it currently is. How do I do this??? I will include the code for my index.php page, and login.php (logout.php is as I want it). index.php <?php // Connects to your Database mysql_connect("localhost", "username", "password") or die(mysql_error()); mysql_select_db("database") or die(mysql_error()); //checks cookies to make sure they are logged in if(isset($_COOKIE['ID_my_site'])) { $username = $_COOKIE['ID_my_site']; $pass = $_COOKIE['Key_my_site']; $check = mysql_query("SELECT * FROM users WHERE username = '$username'")or die(mysql_error()); while($info = mysql_fetch_array( $check )) { //if the cookie has the wrong password, they are taken to the login page if ($pass != $info['password']) { header("Location: login.php"); } //otherwise they are shown the admin area else { ?> <html> <head> <link rel="stylesheet" href="styles/main.css" type="text/css" /> </head> <body> <div id="main"> <h1>Welcome</h1> <div style="border: 1px dashed black; padding: 1.5em"> <h3>You are now logged in</h3> <p>Click on a link below!</p> <a href="logout.php">Logout</a><br /><br /> <a href="page2.php">Next Page</a> </div> </div> </body> </html> <? } } } else //if the cookie does not exist, they are taken to the login screen { header("Location: login.php"); } ?> login.php <?php // Connects to your Database mysql_connect("localhost", "username", "password") or die(mysql_error()); mysql_select_db("database") or die(mysql_error()); //Checks if there is a login cookie if(isset($_COOKIE['ID_my_site'])) //if there is, it logs you in and directes you to the members page { $username = $_COOKIE['ID_my_site']; $pass = $_COOKIE['Key_my_site']; $check = mysql_query("SELECT * FROM users WHERE username = '$username'")or die(mysql_error()); while($info = mysql_fetch_array( $check )) { if ($pass != $info['password']) { } else { header("Location: index.php"); } } } //if the login form is submitted if (isset($_POST['submit'])) { // if form has been submitted // makes sure they filled it in if(!$_POST['username'] | !$_POST['pass']) { die('You did not fill in a required field.'); } // checks it against the database if (!get_magic_quotes_gpc()) { $_POST['email'] = addslashes($_POST['email']); } $check = mysql_query("SELECT * FROM users WHERE username = '".$_POST['username']."'")or die(mysql_error()); //Gives error if user dosen't exist $check2 = mysql_num_rows($check); if ($check2 == 0) { die('That user does not exist in our database. <a href=add.php>Click Here to Register</a>'); } while($info = mysql_fetch_array( $check )) { $_POST['pass'] = stripslashes($_POST['pass']); $info['password'] = stripslashes($info['password']); $_POST['pass'] = md5($_POST['pass']); //gives error if the password is wrong if ($_POST['pass'] != $info['password']) { ?> <html> <head> <link rel="stylesheet" href="styles/main.css" type="text/css" /> </head> <body> <div id="main"> <div style="border: 1px dashed black; padding: 1.5em"> <? die('Incorrect password, click the BACK button and please try again.'); } else { // if login is ok then we add a cookie $_POST['username'] = stripslashes($_POST['username']); $hour = time() + 3600; setcookie(ID_my_site, $_POST['username'], $hour); setcookie(Key_my_site, $_POST['pass'], $hour); //then redirect them to the members area header("Location: index.php"); } } } else { // if they are not logged in ?> <html> <head> <link rel="stylesheet" href="styles/main.css" type="text/css" /> </head> <body> <div id="main"> <div style="border: 1px dashed black; padding: 1.5em"> <form action="<?php echo $_SERVER['PHP_SELF']?>" method="post"> <table border="0"> <tr><td colspan=2><h1>Login</h1></td></tr> <tr><td>Username:</td><td> <input type="text" name="username" maxlength="40"> </td></tr> <tr><td>Password:</td><td> <input type="password" name="pass" maxlength="50"> </td></tr> <tr><td colspan="2" align="right"> <input type="submit" name="submit" value="Login"> </td></tr> </table> </form> <?php } ?> </div> </div> </body> </html> page2.php <?php // Connects to your Database mysql_connect("localhost", "username", "password") or die(mysql_error()); mysql_select_db("database") or die(mysql_error()); //checks cookies to make sure they are logged in if(isset($_COOKIE['ID_my_site'])) { $username = $_COOKIE['ID_my_site']; $pass = $_COOKIE['Key_my_site']; $check = mysql_query("SELECT * FROM users WHERE username = '$username'")or die(mysql_error()); while($info = mysql_fetch_array( $check )) { //if the cookie has the wrong password, they are taken to the login page if ($pass != $info['password']) { header("Location: login.php"); } //otherwise they are shown the admin area else { ?> <html> <head> <link rel="stylesheet" href="styles/main.css" type="text/css" /> </head> <body> <div id="main"> <h1>Page 2</h1> <div style="border: 1px dashed black; padding: 1.5em"> <h3>You are now logged in and can view content on Page 2</h3> <a href="logout.php">Logout</a><br /> </div> </div> </body> </html> <? } } } else //if the cookie does not exist, they are taken to the login screen { header("Location: login.php"); } ?> If anyone can help me, that would be awesome!