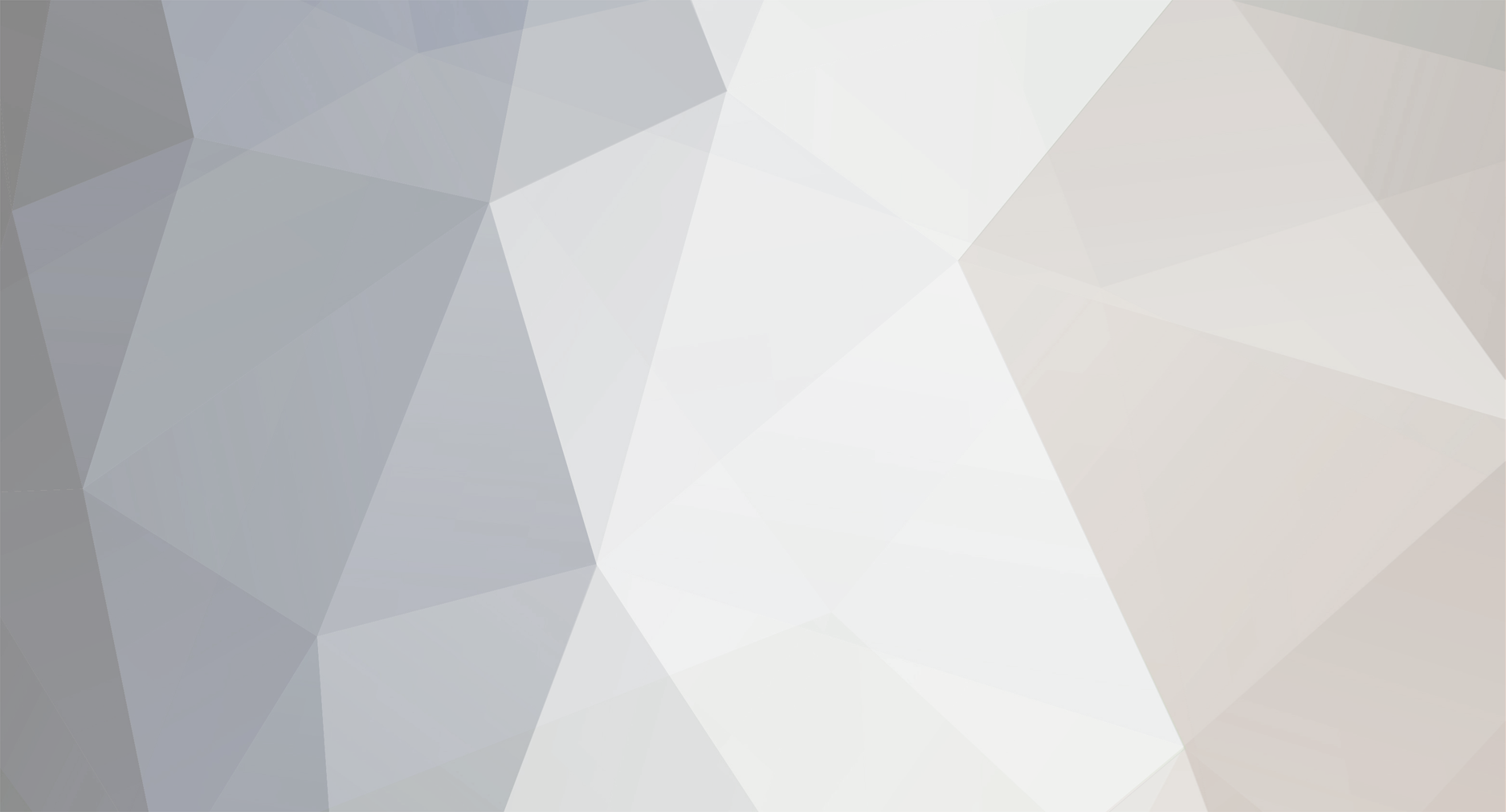
crimsonjade
Members-
Posts
10 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
crimsonjade's Achievements

Newbie (1/5)
0
Reputation
-
I have this project and so far this is what it's suppose to do: There are 4 pages. The first page asks the user to enter their username and password (these are finite and defined by the second page) and asks the user to choose a file they want to work with. The second page verifies the username and password are correct and echoes the information from the chosen file into a table and has two links underneath: one to take the user back to page 1, and the second to take the user to page 3. Page 3 calls in two functions from page 4 to loop through all the information in that same file using regex to make sure Student Numbers and email addresses are in the correct format. If it's correct, it will alert the user that all the information is legitimate, or else it will write the errors to a log file. What I'm having trouble with is on page 3 and 4. The functions aren't working because Apache says there are undefined variables, and on page 3 I can't seem to get rid of the array to string conversion notice. This is driving me nuts. Here's the code for all four pages: Page 1 <?php /* File: page1.php This file is first major assignment in the PHP level 1 course. The purpose: "To write a php application that helps an instructor set up a website for their courses." Author: name Last modified: May 04, 2007. */ echo '<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">'; echo '<html xmlns="http://www.w3.org/1999/xhtml">'; echo '<head>'; echo '<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />'; echo '<title>Comp 1920 - Assignment 1</title>'; echo '</head>'; echo '<body>'; echo '<form action = "./page2.php" method = "get" name = "as1_form">'; echo 'Please enter your username: <input type = "text" name="username"><br /><br />'; echo 'Please enter your password: <input type = "password" name="password"><br /><br />'; $directory = "./courses/"; $fileslist = scandir($directory); echo "Please Select a File:<br />"; echo '<select name="filename">'; foreach($fileslist as $filenames) { //The following code splits the string into 'filename' and 'extension'. list($name, $extension) = explode('.txt', $filenames); if (is_dir ($directory)){ //I'll think of something to put here later... } else { die ("Directory 'courses' or required files do not exist."); } if (is_dir($filenames)) { continue; } elseif (!ereg("^.*\.(txt)$", $filenames) ){ continue; } echo "<option value=\"$filenames\">$name</option><br />"; } echo "</select><br /><br />"; echo '<input type="submit" name="info_btn" value="Submit Query">'; echo '</form>'; echo '</body>'; echo '</html>'; ?> Page 2 <?php /* File: page2.php This file is first major assignment in the PHP level 1 course. The purpose: "To write a php application that helps an instructor set up a website for their courses." Author: name Last modified: May 04, 2007. */ /* The following code determines if the username field has a value. If it doesn't, it will kill the script. */ if (!($_GET["username"])){ die ("Please enter your username."); } elseif ($_GET["username"] !="comp1920"){ die ("Invalid username, sorry."); } /* The following code determines if the password name field has a value/valid value. If it doesn't, it will kill the script. */ if (!($_GET["password"])) { die ("Please enter a password."); } elseif ($_GET["password"] != "php") { die ("Invalid password, sorry."); } /* The following script determines which file was chosen and will echo out the contents in a table for the user to review. It provides links back to page1 or onto page3, depending if they decide to use the file. */ $resultfile = $_GET["filename"]; if (!($_GET["filename"])) { die ("Please select a course file to work with."); } else { $file = file("./courses/".$resultfile); echo "You chose file: $resultfile\n<table border=\"1\">\n"; foreach ($file as $newPiece) { echo "\t<tr>\n"; $newStr = trim($newPiece); $tempArr = split(",",$newStr); foreach ($tempArr as $tempStr) { if (ereg("^[a-zA-Z0-9]*$",$tempStr)){ $finaloutput=ucfirst($tempStr); } elseif (ereg("^[a-zA-Z0-9_.*]*@*[a-z]*\.*[a-z]{2,}",$tempStr)){ $finaloutput=strtolower($tempStr); } echo "\t\t<td>".$finaloutput."</td>\n"; } echo "\t</tr>\n"; } echo "</table>\n"; echo '<br /><br />'; echo '<a href="./page1.php">Choose another course file</a> <a href="./page3.php?filename=',urlencode($resultfile),'">Work with this course file</a>'; } echo '</body>'; echo '</html>'; ?> Page 3 <?php /* File: page3.php This file is first major assignment in the PHP level 1 course. The purpose: "To write a php application that helps an instructor set up a website for their courses." Author: Irene Thanh Last modified: May 04, 2007. */ date_default_timezone_set("America/Vancouver"); $fileN = $_GET["filename"]; $resultingf = "./courses/".$fileN; $date_time = date("F j, Y (h:i:s)"); if (!($fileN)){ die ("Please specify a file."); } else { $fileorg = file($resultingf); $ContentsArr = array(); if (is_file ($resultingf)){ foreach ($fileorg as $infos) { $ContentsArr[] = $infos; } echo "<table border=\"1\">\n"; echo "\t<tr>\n"; $newArrs = split(",",$ContentsArr); //$newL = array(); foreach($newArrs as $separated) { require_once("page4.php"); isStudentNumberWellFormed($separated); isEmailAddressWellFormed($separated); if (!(ereg("^[a-zA-Z]*$",$separated))){ //This is to pass the names. } else { $newResult = $separated; } echo "\t\t<td>".$newResult."</td\n"; } echo "\t</tr>\n"; } else { die ("No such file, sorry."); } } ?> Page 4 <?php /* File: page4.php This file is first major assignment in the PHP level 1 course. The purpose: "To write a php application that helps an instructor set up a website for their courses." Author: name Last modified: May 04, 2007. */ date_default_timezone_set("America/Vancouver"); $fileN = $_GET["filename"]; $resultingf = "./courses/".$fileN; $date_time = date("F j, Y (h:i:s)"); function isStudentNumberWellFormed($studentnumber){ if (!(ereg("^[aA][0-9]{8}$",$studentnumber))){ if ($filehandle = @fopen("./courses/errors.log", "a+")){ $writetext = fwrite($filehandle, "<br />-----"); $writetext = fwrite($filehandle, "<br />$date_time."); $writetext = fwrite($filehandle, "<br />Improper student number from $fileN:"); $writetext = fwrite($filehandle, "<br />$studentnumber"); fclose($filehandle) or die ("Error closing file <br />"); } else { die("Cannot open file 'errors.log', an error has occured."); } } else { $newResult = $separated; } } function isEmailAddressWellFormed($emailaddress){ if (!(ereg("^[a-zA-Z0-9_.]*@[a-z]*\.[a-z]{2,5}$",$emailaddress))){ if ($filehandle = @fopen("./courses/errors.log", "a+")){ $writetext = fwrite($filehandle, "<br />-----"); $writetext = fwrite($filehandle, "<br />$date_time."); $writetext = fwrite($filehandle, "<br />Improper email address from $fileN:"); $writetext = fwrite($filehandle, "<br />$emailaddress"); fclose($filehandle) or die ("Error closing file <br />"); } else { die("Cannot open file 'errors.log', an error has occured."); } } else { $newResult = $separated; } } ?> The script runs fine up until page 2 where you select the link to page 3. This is what happens when I try to access page 3 (that loops through the information using regex functions to check if certain information is in the proper format, for further info, read first paragraph at top) Notice: Array to string conversion in C:\...\page3.php on line 29 Notice: Undefined variable: date_time in C:\...\page4.php on line 20 Notice: Undefined variable: fileN in C:\...\page4.php on line 21 Notice: Undefined variable: date_time in C:\...\page4.php on line 40 Notice: Undefined variable: fileN in C:\...\page4.php on line 41 <table>Array</table> Someone please help! I really can't figure out how to get page 3 to so what it's supposed to do! Link to the project description: http://php1920.redirectme.net/res_COMP1920/Assignment1.htm
-
What am I doing wrong regarding cookies?
crimsonjade replied to crimsonjade's topic in PHP Coding Help
I don't think that has anything to do with it I'm afraid..as I usually use the semicolon that way along with the html elements...*sigh* -
What am I doing wrong regarding cookies?
crimsonjade replied to crimsonjade's topic in PHP Coding Help
Actually, I think we sorta fixed that... Now I don't know how to actually call the setcookie function... This is what the script's suppose to do. The first page sees if you've set a cookie before and if not it'll say that you're a first timer, and you must enter your first and last names. Choice of colour is optional (it should affect the bg color later). Second script verifies that a first and last name has been entered, then sees if a colour has been chosen as a background colour. If it has been chosen, then it'll change the background to that colour and echo out "welcome back [fullname]." or something of the like. code for page 1: <?php echo '<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">'; echo '<html xmlns="http://www.w3.org/1999/xhtml">'; echo '<head>'; echo '<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />'; echo '<title>Comp 1920 - Lab 17</title>'; echo '</head>'; echo '<body>'; if (!(isset($_COOKIE["firstname"]))) { echo '<form action = "./ithanhL17-2.php" method = "post" name = "L17_form">'; echo "Welcome! This seems to be your first visit! <br /><br />"; echo 'Please enter your first name: <input type = "text" name="firstn"><br /><br />'; echo 'Please enter your last name: <input type = "text" name="lastn"><br /><br />'; echo 'Red <input type="radio" name="colour" value"red" checked="checked"><br /><br />'; echo 'Blue <input type="radio" name="colour" value"blue"><br /><br />'; echo 'Yellow <input type="radio" name="colour" value"yellow"><br /><br />'; echo 'Green <input type="radio" name="colour" value"green"><br /><br />'; echo '<input type="submit" name="submit_btn" value="Submit">'; echo '</form>'; } else { echo isset($_COOKIE["firstname"]); //echo $_COOKIE["first"]; } echo '</body>'; echo '</html>'; ?> code for page 2: <?php /* The following code determines if the username field has a value. If it doesn't, it will kill the script. */ if (!($_POST["firstn"])){ die ("Please press Back and enter your first name."); } /* The following code determines if the password name field has a value/valid value. If it doesn't, it will kill the script. */ if (!($_POST["lastn"])) { die ("Please press Back and enter your last name."); } $firstname = $_POST["firstn"]; $lastname = $_POST["lastn"]; $color = $_POST["colour"]; if (!(isset($_COOKIE["firstname"]))) { setcookie("firstname",$_POST["firstn"],(time()+(24*60*60)),"","",0); echo "The cookie has been set."; } else { if($_POST["colour"] != "red" ||$_POST["colour"] != "green"|| $_POST["colour"] != "blue" || $_POST["colour"] != "yellow"){ echo "Hello $firstname $lastname"; } else{ echo"<body bgcolor=\"$color\">Hello $firstname $lastname"; } } ?> So when I type in my information in page 1, get it sent to page 2, the "cookie has been set" shows up. So then I refresh and all I get is "1" So...how would I call the setcookie function properly in page 1? -
Here's my first page: <?php echo '<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">'; echo '<html xmlns="http://www.w3.org/1999/xhtml">'; echo '<head>'; echo '<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />'; echo '<title>Comp 1920 - Lab 17</title>'; echo '</head>'; echo '<body>'; if(!($_COOKIE["firstname"])){ echo '<form action = "./page2.php" method = "post" name = "L17_form">'; echo "Welcome! This seems to be your first visit! <br /><br />"; echo 'Please enter your first name: <input type = "text" name="firstn"><br /><br />'; echo 'Please enter your last name: <input type = "text" name="lastn"><br /><br />'; echo 'Red <input type="radio" name="colour" value"red" checked="off"><br /><br />'; echo 'Blue <input type="radio" name="colour" value"blue" checked="off"><br /><br />'; echo 'Yellow <input type="radio" name="colour" value"yellow" checked="off"><br /><br />'; echo 'Green <input type="radio" name="colour" value"green" checked="off"><br /><br />'; echo '<input type="submit" name="submit_btn" value="Submit">'; echo '</form>'; } else { echo $_COOKIE["firstname"]; } echo '</body>'; echo '</html>'; ?> And here's my second page: <?php /* The following code determines if the username field has a value. If it doesn't, it will kill the script. */ if (!($_POST["firstn"])){ die ("Please press Back and enter your first name."); } /* The following code determines if the password name field has a value/valid value. If it doesn't, it will kill the script. */ if (!($_POST["lastn"])) { die ("Please press Back and enter your last name."); } $firstname = $_POST["firstn"]; $lastname = $_POST["lastn"]; $color = $_POST["colour"]; if(!($_COOKIE["firstname"])) { setcookie("firstname",$_POST["first"],(time()+(24*60*60)),"","",0); echo "The cookie has been set."; } else { if($_POST["colour"] != "red" ||$_POST["colour"] != "green"|| $_POST["colour"] != "blue" || $_POST["colour"] != "yellow"){ echo "Hello $firstname $lastname"; } else{ echo"<body bgcolor=\"$color\">Hello $firstname $lastname"; } } ?> So...When I visit the first page, I get this error message: " Notice: Undefined index: firstname in [filename and path] on line 24" The line number'll be different as I took out some comments required for the assignment (ie, name, etc). So...I'm not sure what I'm doing wrong here... Any help please?
-
Actually, ended up doing something like this: $resultfile = $_GET["filename"]; if (!($_GET["filename"])) { die ("Please select a course file to work with."); } else { $file = file("./courses/".$resultfile); foreach ($file as $newPiece) { $newStr = trim($newPiece); $tempArr = split(",",$newStr); foreach ($tempArr as $tempStr) { echo "\t\t<td>".$tempStr."</td>\n"; } echo "\t</tr>\n"; } echo "</table>\n"; }
-
!!! I never thought of exploding it! Hmm, I'll try that out, thanks! Edit: I tried it...and all that comes out is "Array"...not my information at all :'( P.S. I also have to use split() as it's mandatory in the assignment *sigh*
-
[SOLVED] Hiding files within a directory
crimsonjade replied to crimsonjade's topic in PHP Coding Help
My goodness...that's...that's so much simpler...*frowns* unfortunately I'm only supposed to use that which we've learned about in class since this is what the assignment's testing *sigh* But thank you! Definitely will keep that in mind in the future! -
I'm working on a school project that requires me to echo out information in a table. How it's supposed to work is this: The first php script asks the user to input the proper username and password, and select the course file they want to work with from a selection box (drop down). The second php script receives the information and checks to see if the username and password are correct and if they are, it will proceed to echo out the information contained in the selected file in a table. The format of information within a file is like this: studentnumber,lastname,firstname,coursename,email So they're delimited by the comma. Each file contains a varying amount of lines. I have to split the information into a table with each piece of information in their own cell in the appropriate row. It's required that I use file();, trim();, and split(); however, when I try to use trim or split, I get this notice of an array to string conversion and I don't know how to go around it. $resultfile = $_GET["filename"]; if (!($_GET["filename"])) { die ("Please select a course file to work with."); } else { $file = file("./courses/".$resultfile); foreach ($file as $newPiece) { $newStr = trim($newPiece); $newLine = split(",",$newStr); } } I read that array_walk loops through an array and can perform functions on each element of the array, but I'm not quite sure how to go about it. So...how would you go about it for either foreach or array_walk? Any help would be much appreciated! Thanks in advance ^^"
-
[SOLVED] Hiding files within a directory
crimsonjade replied to crimsonjade's topic in PHP Coding Help
Actually, I ended up having to do this (as I had tried your suggestion...but then my select box came out blank...): foreach($fileslist as $filenames) { list($name, $extension) = explode('.txt', $filenames); if (is_dir ($directory)){ //I'll think of something to put here later... } else { die ("Directory 'courses' or required files do not exist."); } if (is_dir($filenames)) { continue; } elseif (!ereg("^.*\.(txt)$", $filenames)){ continue; } echo "<option value=\"$filenames\">$name</option><br />"; } But thanks anyway -
Hi, I'm so sorry if this may have been asked before...but I've been searching and can't seem to find the right topic. I'm looking for a PHP code that will hide (not unset) any filename within a directory that doesn't end in a particular extension (i.e. ".txt") from showing up in an array using foreach. In this case, I am only showing txt files in an array displayed in an HTML selection form element. I'm a total newbie at PHP (taking my first PHP course ever) and am entirely stuck on this. Any help would be most appreciated! Thanks in advance! P.S. If this has been posted before (and I have a feeling it has) maybe a link or a keyword hint would suffice. Thanks again ^^ ~**Crimsonjade**~