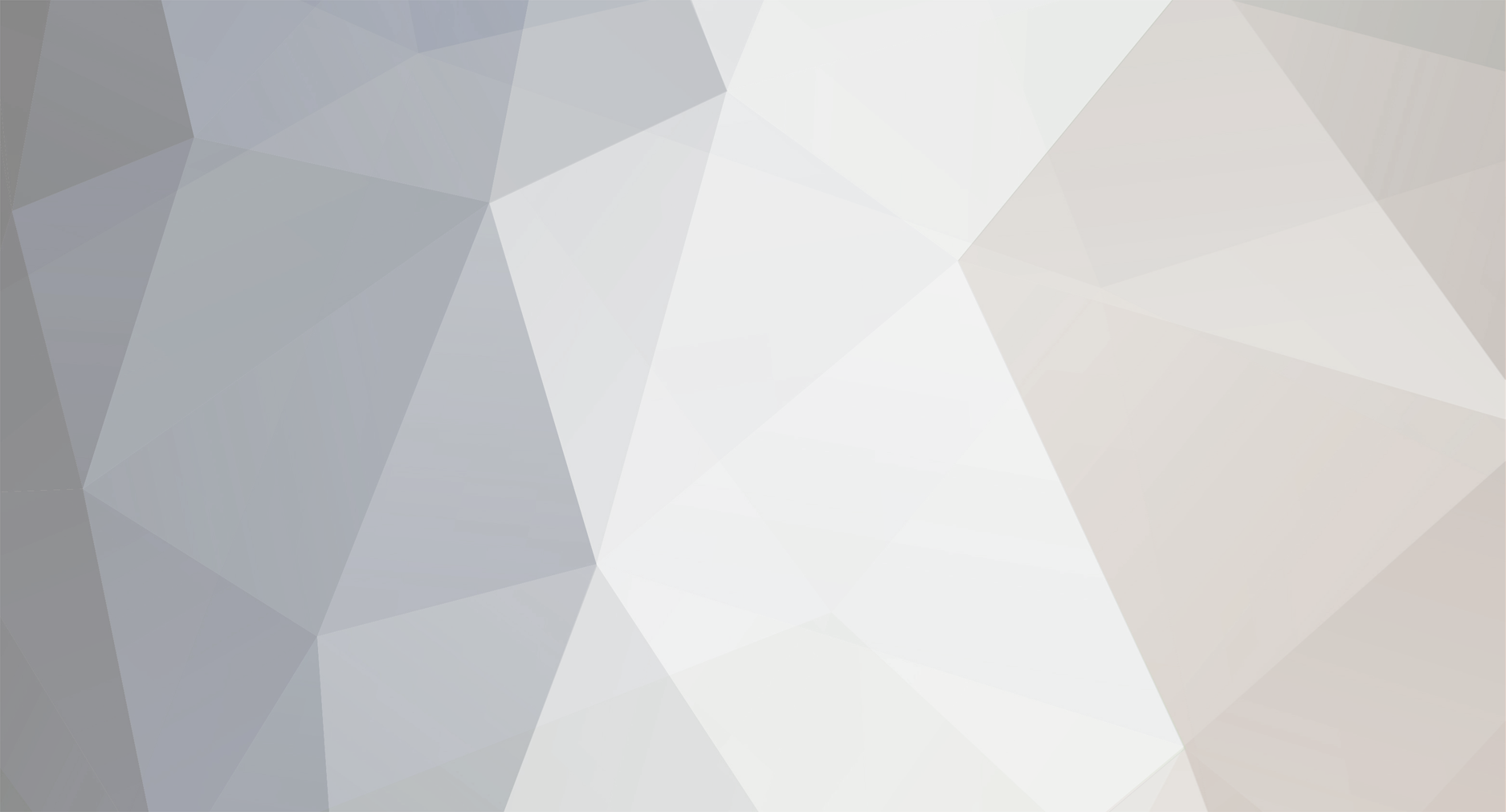
bluewaves
-
Posts
32 -
Joined
-
Last visited
Never
Posts posted by bluewaves
-
-
I have the following code that works perfectly. I would like to add an easy pagination feature that
limits the number of items, counts the number of records and makes links and pages for the next page.
<?php
include('../myconnection.inc');
$database = "mydatabasename";
$cxn = mysql_connect($host,$user,$password)
or die ("couldn't connect to server");
mysql_select_db($database);
$query = "SELECT * FROM baghaus ORDER BY Price";
$result = mysql_query($query)
or die ("Couldn't execute query.");
define ("NUMCOLS", 3);
$res = mysql_query("SELECT Thumbnail, Link, Name, Price FROM baghaus WHERE custom3='Marc Jacobs' ORDER BY Price");
$count = 0;
echo "<center><TABLE border=0 cellpadding='10'>";
while (list($Thumbnail, $Link, $Name, $Price) = mysql_fetch_row($res))
{
if ($count % NUMCOLS == 0) echo "<TR>\n"; # new row
echo "<TD width='150' valign='top'><a href='$Link'><img src='$Thumbnail' border='0'><br>$Name</a><br>\$$Price</TD>\n";
$count++;
if ($count % NUMCOLS == 0) echo "</TR>\n"; # end row
}
# end row if not already ended
if ($count % NUMCOLS != 0) {
while ($count++ % NUMCOLS) echo "<td> </td>";
echo "</TR>\n";
}
echo "</TABLE>";
?>
I have this script that works for counting and pagination...but I need to merge the two and don't know how:
<?php
include('../myconnection.inc');
$database = "voipvide_checks";
$cxn = mysql_connect($host,$user,$password)
or die ("couldn't connect to server");
mysql_select_db($database);
// Determine how many records there are:
if (isset($_GET['np'])) {
$num_pages = (int) $_GET['np'];
} else {
// Find out how many records there are.
$q = "SELECT COUNT(*) FROM checks";
// Get the number.
$r = mysql_query($q);
list($num_records) = mysql_fetch_array($r, MYSQL_NUM);
mysql_free_result($r);
// Calculate the number of pages:
if ($num_records > $display_number) {
$num_pages = ceil ($num_records/$display_number);
} else {
$num_pages = 1;
}
}
// Determine where in the database to start returning results:
if (isset($_GET['s'])) {
$start = (int) $_GET['s'];
} else {
$start = 0;
}
// Define the query:
$q = "SELECT * FROM checks WHERE SubCategory='Check' ORDER BY Name ASC LIMIT $start, $display_number";
// Run the query:
$r = mysql_query ($q);
// Display all of the records:
while ($row = @mysql_fetch_array ($r, MYSQL_ASSOC)) {
echo "<table width='380' cellpadding='10'><tr><td width='400'><a href='{$row['Link']}'>
<font face='Arial' size='2'><img border='0' src='{$row['Image']}'><P>{$row['Name']}</a><P>
{$row['Description']}</font></td>
<td> <br /></td></tr></table>\n";
}
// Clean up:
mysql_close($cxn);
// Make the links to other pages, if necessary:
if ($num_pages > 1) {
echo '<hr width="50%" align="left" />';
// Determine what page the script is on:
$current_page = ($start/$display_number) + 1;
// If it's not the first page, make a Previous button:
if ($current_page != 1) {
echo '<a href="browse_checks.php?s=' . ($start - $display_number) . '&np=' . $num_pages . '"> Previous</a>';
}
// Make all the numbered pages:
for ($i = 1; $i <= $num_pages; $i++) {
// Don't link the current page:
if ($i != $current_page) {
echo '<a href="browse_checks.php?s=' . (($display_number * ($i - 1))) . '&np=' . $num_pages . '">' . $i . '</a> ';
} else {
echo $i . ' ';
}
}
// If it's not the last page, make a Next button:
if ($current_page != $num_pages) {
echo '<a href="browse_checks.php?s=' . ($start + $display_number) . '&np=' . $num_pages . '"> Next</a> ';
}
}
?>
</body>
</html>
-
Thank you...that worked like a charm.
-
Anyone care to give me some tips on my new website:
I am self taught, so I hope you are kind.
The top of my html should have something in it, but I've
tried some of the standard suggested coding and it messes
up my graphics. Any suggestions on what should go before
the <html>?
Thanks...
-
Will that allow the flow of data to be displayed:
Box 1 Box 2 Box 3
All of Box 1 Attributes All of Box 2 Attributes All of Box 3 Attributes
and continue on until the end of the records?
-
I have this code that displays results in a single entry column fashion:
// Display all of the records:
while ($row = @mysql_fetch_array ($r, MYSQL_ASSOC)) {
echo "<center><table width='380' cellpadding='10'><tr><td width='400'><a href='{$row['Link']}'>
<font face='Arial' size='2'><img border='0' src='{$row['Thumbnail']}'><P>{$row['Name']}</a><br> \${$row['Price']}</a>
</font></td>
</tr>
</table></center>\n";
}
I'd like to display products in a 3 column fashion. I've read some of the posts here, but can't get my script to work to do it.
-
(($display_number * ($i - 1)))
I'd like to get the display numbers to read something like
1,2,3,4...24 Next ....something so that the whole string of numbers isn't showing.
How do you do that?
Thanks.
SQL Query that selects one word from a column many words
in Microsoft SQL - MSSQL
Posted
What is the format for selecting one word from
a Name Column?
Example:
Column=Name
Name=Sterling Silver Three Pronged Fork
I want to pick products that say "fork" in the name.