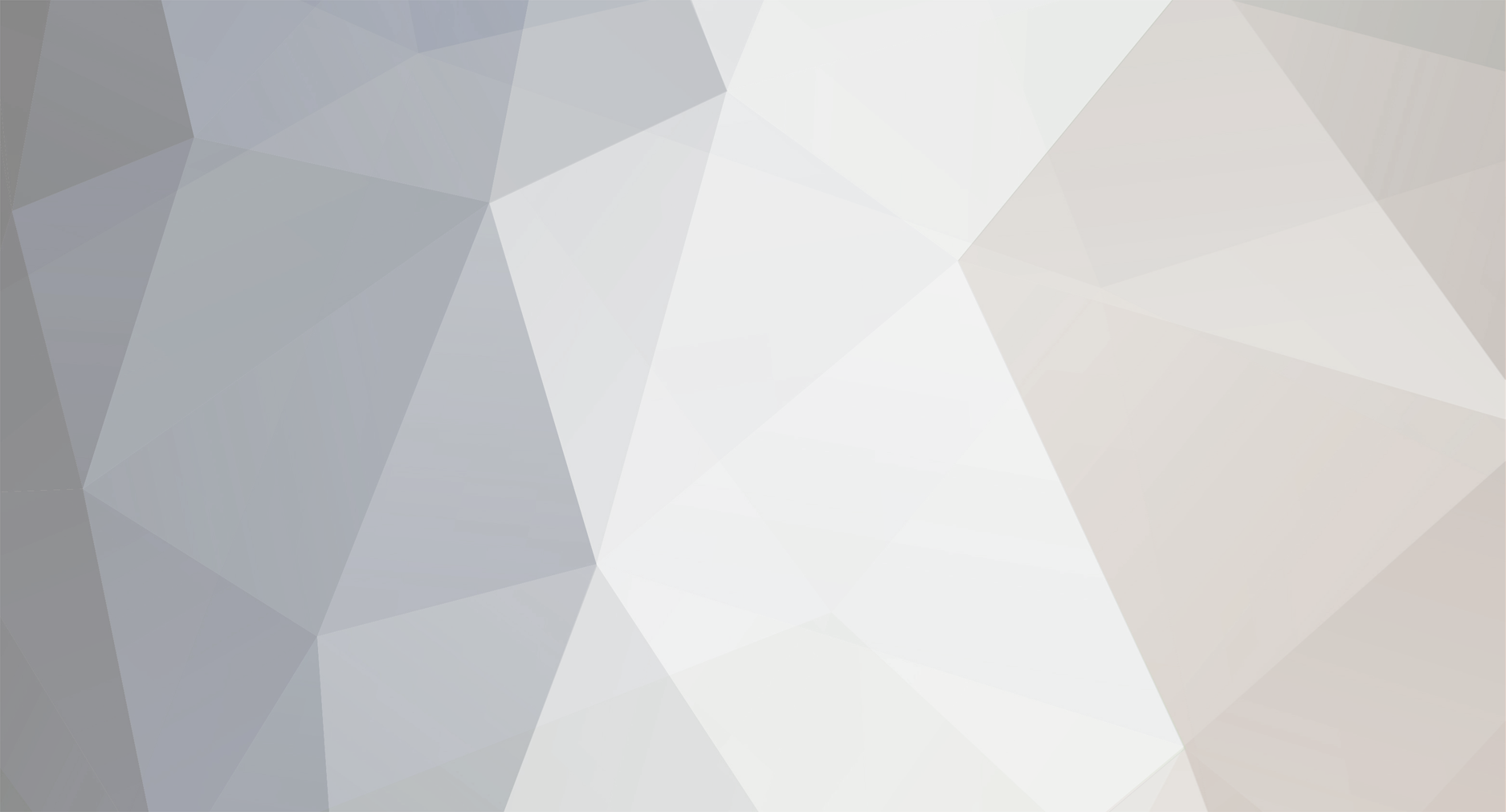
uramagget
-
Posts
66 -
Joined
-
Last visited
Never
Posts posted by uramagget
-
-
Yes; I have been meaning to fix that. Apologies:
SELECT * FROM ndsg_brawl.tourneys_brackets WHERE tourney = 'brawlan'
-
Bump, I think? I wish I can edit my post to fix out issues I have left within it, though. For some reason I cannot edit it.
-
$players = $mysql->select( "ndsg_brawl.tourneys_brackets", "*", "WHERE tourney = '{$title}'" ); if ($players) { while ($pairings = @mysql_fetch_assoc($players)) { echo ' <h2>Round '.$pairings['round'].'</h2> <table class="list"> <thead> <tr> <td>User #1</td> <td>User #2</td> <td>Round</td> </tr> </thead><tbody>'; $username1 = $mysql->select( "ndsg_vbulletin.vb_user", "username", "WHERE userid = '{$pairings['userid']}'" ); $username2 = $mysql->select( "ndsg_vbulletin.vb_user", "username", "WHERE userid = '{$pairings['userid2']}'" ); $username1 = @mysql_fetch_assoc($username1); $username2 = @mysql_fetch_assoc($username2); echo '<tr> <td>'.$username1['username'].'</td> <td>'.$username2['username'].'</td> <td>'.$pairings['round'].'</td> </tr>'; echo '</tbody></table>'; } } else { echo '<p>There are no standings up yet.</p>'; }
I am not sure how to have it only display 1 table for each round. I tried using GROUP BY `round`, but it only displays 1 pairing instead of all. :/
-
Thanks very much. I changed the count() to a sum() for the 2nd one and it works splendidly. Again, thanks.
-
I've tried searching through the documentation of MySQL but the right words on how this is done exactly is just is not successful.. Basically here is my schema:
Table:
sprites
--id
--title
--sheet_file
--game
--console
--amount
--author
I want to do two things, though. For one, I want to have a MySQL query count the total amount in the Database. I have tried:
SELECT amount FROM `sprites` AS COUNT
Although I don't think that it displays the total 'amount' added...
Next is the table displaying the submitters. I want to be able to display the submitters, though I want it to display the respectful amount of sprites that user has submitted, while ordering by the greatest amount first.
Thanking in advance, and sorry if it sounded a bit..odd.
-
Hi, I'm trying to set-up a tournament, though I want the system to be automated. I'm not sure how to approach this, since I thought the process would be confusing. I want to setup random pairing, but how can that be done and then stored in a row? Plus, I'm not sure how I would display the pairings correctly for [x] round. Can anybody help out this confused user?
My basic layout for a bracket is: *removed by request of op*
If that is required to help out..
-
Oh! Thanks man!
-
My auto_increment value is no longer incrementing after it reaches 127. I'm not sure what's happening exactly, and after Googling documentation or anything related to it, no results. =/; I don't know what to provide exactly, although when I attempt altering the auto_increment in 'Operations' to 128, it says it successfully incremented it although it does NOT as changes revert.
In addition when I insert it returns a message regarding a truncated value in the field 'id'.
-
In addition to blackcell's code, attempt using switch() before you create a plethora of messy if statements. if statements still run even if one of the if's conditions are matched.
-
Seems to be that there was a syntax error in my post. I have changed it.
-
I'm having so many headaches building a function for selecting data from a Database easier in mySQL, so I tried a simple function out:
function select($table, $fields, $options = null, $loop = false) { if (!defined('MYSQL_NOCONNECT')) { if (isset($table) && isset($fields)) { $sql = "SELECT ".$this->escape($fields)." FROM ".$this->escape($table)." ".$options.""; $result = mysql_query($sql); if ($result) { if (mysql_num_rows($result) > 0) { return $result; } else { return false; } } } else { echo "Wrong number of parameters supplied for select(). Try again, buddy boy."; exit; } } else { return null; } }
Usage
if (is_numeric($_GET['view'])) { $view = $_GET['view']; $sub = $mysql->select("submissions", "*", "WHERE sub_id=".$mysql->escape($view).""); if ($sub) { while ($subview = mysql_fetch_array($sub)) { //Define Variables for template $sub_id = $view; $sub_name = $subview['sub_title']; $sub_author = $subview['sub_author']; $sub_description = $subview['sub_description']; //Load Template $tpl->load('submission/submission_view'); } } else { echo "The requested submission was not found. Are you sure it exists?"; } } else { echo 'Numerical values only.'; }
But then, all it returns is:
Resource id #15
I'd really appreciate if anybody could help me out with this headache..
-
Ah, I see. Thank you very much. It seems that the $detect_numeric return value was causing this. Thanks again. ^^;
EDIT: Uh, what happened to the [sOLVED] button? o_O
-
Escape Function
/* Escapes illegal characters within a variable */ function escape($value,$allow_wildcards = true, $detect_numeric = true) { $return_value = $value; if (get_magic_quotes_gpc()) { if(ini_get('magic_quotes_sybase')) { $return_value = str_replace("''", "'", $return_value); } else { $return_value = stripslashes($return_value); } } //Escape wildcards for SQL injection protection on LIKE, GRANT, and REVOKE commands. if (!$allow_wildcards) { $return_value = str_replace('%','\%',$return_value); $return_value = str_replace('_','\_',$return_value); } // Quote if $value is a string and detection enabled. if ($detect_numeric) { if (!is_numeric($return_value)) { return "'" . mysql_real_escape_string($return_value) . "'"; } } //Finally, return the end result with the addition of mysql string escaping. return mysql_real_escape_string($return_value); }
MySQL Query:
if (empty($_POST['nick']) or $_POST['nick'] == " ") { $nick = "Anonymous"; } else { $nick = escape($_POST['nick']); } $msg = escape($_POST['msg']); $ip = $_SERVER['REMOTE_ADDR']; $date = date('M j, y'); //SQL $sql = "INSERT INTO msgs (`nick`, `message`, `date`, `ip`) VALUES('$nick', '$msg', '$date', '$ip');";
Error Returned:
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'nick'', ''nick'', 'Apr 5, 08', '66.98.84.184')' at line 1
It seems like it's adding a single-quote to a single-quote, thus contradicting the escape function itself. =/; Can anybody help out?
-
Well, I have run into a problem, but not with with the provided code. It works great, though the problem is, my comic has a built-in comics system. I would like to, for example, grab the comments that apply to the newest comic. How would I go to doing this?
-
I don't know if it was erased by posting, though shouldn't it be:
include "connectdb.php";
-
Well theres a few ways but to do it simply....
Select COUNT(*) FROM database WHERE username_column = $username_variable
In addition to that
$sql = "SELECT COUNT(*) FROM database WHERE username_column = $username_variable"; $result = mysql_query($sql); if (mysql_num_rows($result) > 0) { //username taken } else { //proceed }
-
Ah, silly me. Thanks graham and wildteen!
-
Hi, can anybody assist me on a mySQL based issue I have? I have a comics system that I wrote just now, and well, the only thing I need to complete it is to display the newest comic first. For example, the way I usually look for comments is by passing a mySQL query that locates the comic using $_GET. Though, I have seen many people first land without the $_GET being set. So, is there a mySQL query out there that selects the newest comic? I imagine it would select the comic with the highest ID, no?
-
Ah, I get it. Thank you very much, GingerRobot.
-
Thank you Gingerbot, though I'd like to know one thing though. Do I replace the total with something? Also, my comments are located in another table, for the sake of organization. How would I add something from another table?
-
I want to make a top 5 for a little mods script I downloaded, but in order to be an overall part of the top 5, a mod has to be high in each:
# of Downloadeds
# of Installs Marked
# of Views
# of Comments
Though the Math and performing the SQL query is just complicated. I do not know if there is further information to provide, but if there is, please tell me.
-
Nevermind, it seems I have fixed this on my own somehow by modifying a mySQL table. Thanks anyway!
-
foreach ($where_array as $key => $value) { if ($row[$table."_".$key]!=$value) { $included=false; } }
Basically, my problem is that is shows a warning about an invalid argument supplied. I have no problem dealing with warnings, but since this will be a public script, well.. yes.. I require assistance, please.
-
I'm fetching this directly from the myBB database. I haven't created any of the tables, I just used SQL to fetch data from it with PHP.
I don't get your question much though.
[SOLVED] Organizing results
in MySQL Help
Posted
Of course:
Database: ndsg_brawl
Table: tourneys_brackets
-> userid
-> userid2
-> round
-> winner
I believe that it all though, unless, you need me to post the query for the user IDs (to grab usernames from the forum database). Anything else you need?