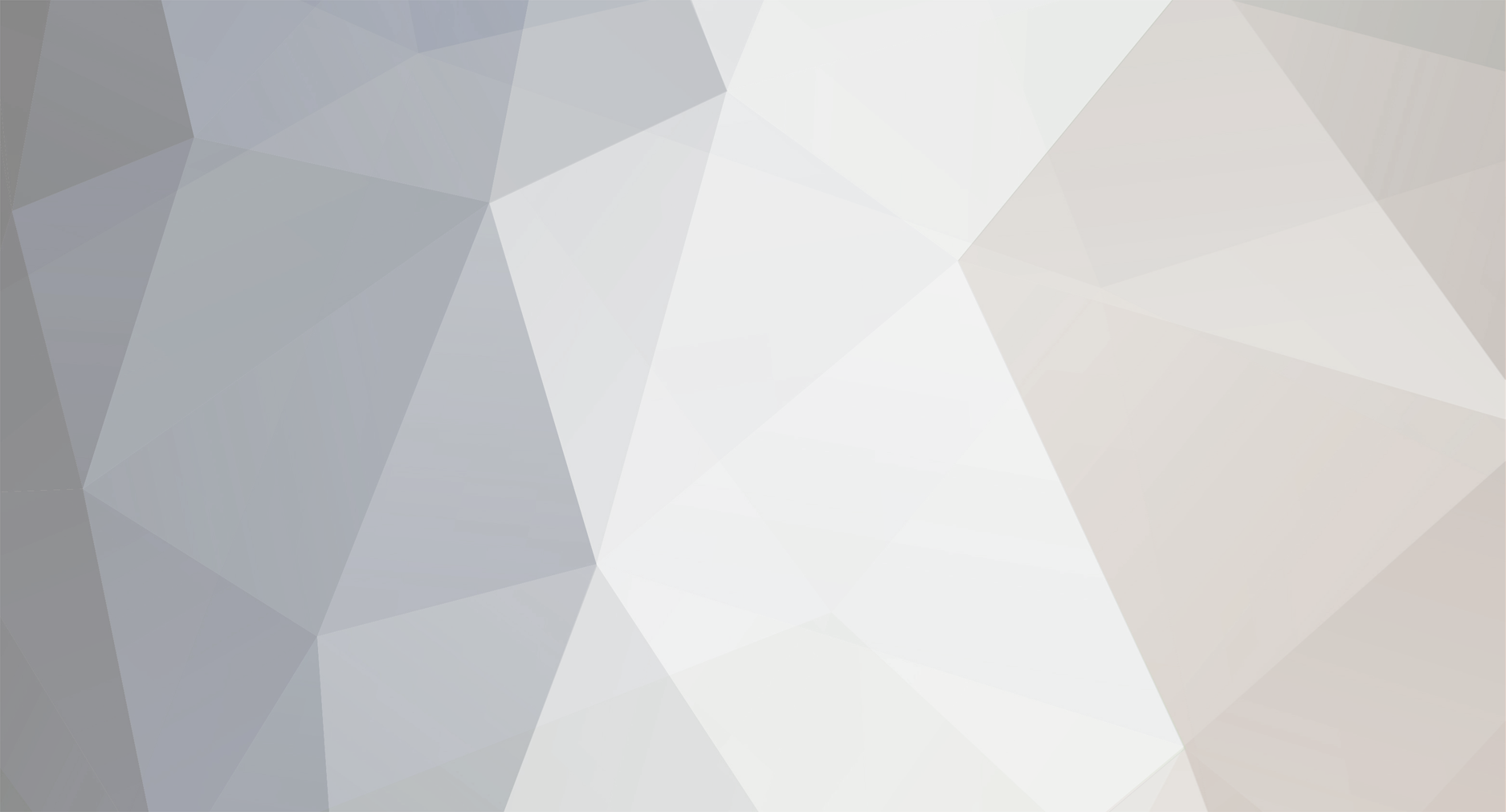
immanuelx2
-
Posts
96 -
Joined
-
Last visited
Never
Posts posted by immanuelx2
-
-
Hey guys, wondering if someone could point me to a sample preg_replace() function that searches for an URL of any kind and encloses it in tags
The one I found so far is
<?php $text = preg_replace('@(https?://([-\w\.]+)+(:\d+)?(/([\w/_\.]*(\?\S+)?)?)?)@', '<a href="$1">$1</a>', $text);
but for some reason, if the URL contains a dash "-" it thinks the URL has ended.. ???
Thanks in advance
-
<?php $value= 'This is my "article"'; function input($value) { if (get_magic_quotes_gpc()){ $value = stripslashes($value);} if (!is_numeric($value)){ $value = "'" . mysql_real_escape_string($value) . "'";} return $value; } echo $value; ?>
gives me:
'This is my "article"'
a) you are not even using the function
b) you are not using it in a query
-
How do you know - "it only inputs the contents until the first double quote is reached"
It's likely you have a problem when you output it onto a web page. What does a "view source" of the output look like?
Say the following is true:
<?php $_POST['articletitle'] = 'This is my "article"';
After the insert, if I look in the MySQL table, I only get 'This is my '
-
Hey guys.
I have a piece of code that puts the contents of a textbox into a mysql database:
<?php mysql_query("INSERT INTO articles VALUES(NULL, ".input($_POST['articlecategory']).", ".input($_POST['articletitle']).", NULL, ".input($_POST['articletext']).", ".input($_SESSION['user_id']).", UNIX_TIMESTAMP(), '0')")
And here is the input function
<?php function input($value) { if (get_magic_quotes_gpc()) $value = stripslashes($value); if (!is_numeric($value)) $value = "'" . mysql_real_escape_string($value) . "'"; return $value; }
Now the problem is, if there is a double quote inside the $_POST['articletitle'] variable, it only inputs the contents until the first double quote is reached. However, there are no problems with single quotes.
Now the weird thing is, that only has a problem with textbox contents. The $_POST['articletext'] can have single or double quotes with no problems!
Any help is greatly appreciated!
-
Thanks for the link.
I am very new to REGEX especially with MySQL. Could you show me an example query I could derive from?
-
Hey guys.
I am building a PHP/MySQL search feature that is quite simple. A user inputs keyword(s) and the MySQL finds the terms in a body of text.
However, if that body of text contains an URL (bbcode [ur=http://www.blahblahblah.com]Blah[/url]) it will actually search through the URL and return a part of that. Is there some way I can get the MySQL to ignore anything inside [] brackets?
Thanks in advance.
-
Here's what i've got:
<?php function comment_output($comment) { $bbcode = array ( '#\[/quote\]\n#i' => '[/quote]', '@\[quote=(.*?)\](.*?)\[/quote\]@si' => '<div class="quote"><h1>\\1 said:</h1>\\2</div>' ); $comment = preg_replace(array_keys($bbcode), array_values($bbcode), $comment); $comment = nl2br($comment); return $comment; }
Here's what it's giving me:
-
'#\[/quote\]\n#i'=>'[/quote]'
still aint working
-
Well I suppose we could exchange str_replace for a version of your preg_replace like so:
Example:
$article['short'] = 'Some text... Car Some more text... [Car] Even more text.. finally, Car and [Car]!'; $pattern = '#(^|\])[^[]+#'; $_GET['search'] = 'some'; // Obviously, you won't use this line, as you have your $_GET value.. I just use this as a test function replace($a){ return preg_replace('#\b('.$_GET['search'].')\b#i', '<span style="background:#ffffab">\1</span>', $a[0]); } $article_short = preg_replace_callback($pattern, 'replace', $article['short']); echo $article_short;
This worked
Thanks a lot for helping with this complex problem. However, now I realize that it still return articles if a match is found in the URLS... so I have to figure out a way for MySQL to recognize where the URLS are and not include them in the LIKE '%search%' statement
-
Any idea why this isn't working? Different but similar problem..
I'm basically trying to ignore the first \n (newline) after the
tag<?php $bbcode = array ( '@\[/quote\]\\n@si' => '[/quote]', // this one doesn't work '@\[quote=(.*?)\](.*?)\[/quote\]@si' => '<div class="quote"><h1>\\1 said:</h1>\\2</div>' // this one works fine ); $comment = preg_replace(array_keys($bbcode), array_values($bbcode), $comment);
-
Well, those 'extra steps' takes what preg finds (outside the [] characters), then to the replace function, uses str_replace to replace any $variable found. A regular preg_replace alone would not do all that.
All you need to do is change a) the $variable that you want to see replaced, and b) $str to the string you want to check.
Hmm. Thanks for the reply... Here is my delimma though
I am trying to get a search highlighted fields script going, and right now I have
$article_short = preg_replace("/\b(".$_GET['search'].")\b/i", '<span style="background:#ffffab">\1</span>', $article['short']);
that goes through and encloses all instances of $_GET['search'] with that span tag. However, the problem is that if there is a with the variable present, it will replace the URL with span tags and mess it up.
So how can I do that? Your example used str_replace which I don't think will work for my situation.
-
Great, thanks!
Is there a way to do it with just a preg_replace function or does it require those extra steps?
-
Hey all.
I've been trying to replace all occurrences of $var that are NOT found between sets of brackets [], but can't figure out how.
Can anyone point me in the right direction?
Much thanks in advance!
These forums are very helpful
-
bump. Again, I am trying to get the search term to not parse inside URLS
Any help?
-
hey all.
I currently have a preg_replace function to highlight search terms with the following:
<?php $article_text = preg_replace("/\b(".$_GET['search'].")\b/i", '<span style="background:#ffffab">\1</span>', $article['text']);
However, the problem is that if a URL using bbcode (ex. Link) contains the search keyword, it will wrap <span> around it and mess up the link. Is there any way I can add to the regexp where "search term" is NOT between "" ?
-
not sure it's working
-
Hey all. I am trying to implement a comment system for my website, which makes use of nl2br().
My question is how to limit the number of consecutive \n so that users cannot spam enter and enter a long blank comment.
I would preferably like to replace all consecutive \n with just "" (nothing) after the first two ( \n\n )
Regex Noob here, so please explain it for a dummy
Thanks in advance..
-
anyone?
-
bump
-
You can use \s
And m modifier for multiple lines.
Could you elaborate? Sorry I'm a newb when it comes to regexp... Could you maybe give me an example of what you mean?
-
Hey all. I have been trying to figure this out for literally several hours and my head is starting to hurt. I come to you experts as a last resort. I am by no means a regexp expert, in fact the format below is something I snagged off the internet, so it may be wrong...
So far I have this for my bbcode parsing:
<?php $bbcode = array( '@\[url=(.*?)\](.*?)\[/url\]@si' => '<a href="\\1" target="_blank">\\2</a>', '@\[quote\](.*?)\[/quote\]@si' => '<i>"\\1"</i>', '@\[ul\](.*?)\[/ul\]@si' => '<ul>\\1</ul>' , '@\[li\](.*?)\[/li\]@si' => '<li>\\1</li>' ); return preg_replace(array_keys($bbcode), array_values($bbcode), $string); ?>
However, when it comes to the "ul" and "li" tags... I want to omit any newline ("\n") characters before or after those elements, because I have a nl2br() statement that parses right at the end to output the HTML, and since <li> tags are naturally list-type elements, they don't need extra <br /> around them.
Just like in these forums:
[list] [*]item 1 [*]item 2 [/list]
between items 1 and 2, and the two list tags, no additional line breaks are added, even though there are newlines created when that code is written. So my question is, how can I remove the newlines at those specific points only, so that my nl2br() can parse the rest of the newlines in my string as breaks?
Thanks in advance for any help
-
Small graphical update. what do you guys think?
-
Thanks guys. So basically, IE is incompetent to handle image submit buttons?
-
Thanks for the reply. I'm still a little bit confused, how can I fix it? Right now the post variables are just the form element names like $_POST['zip'] etc..
[SOLVED] Enclose URL in tags?
in Regex Help
Posted
Thank you so very much, worked beautifully!!