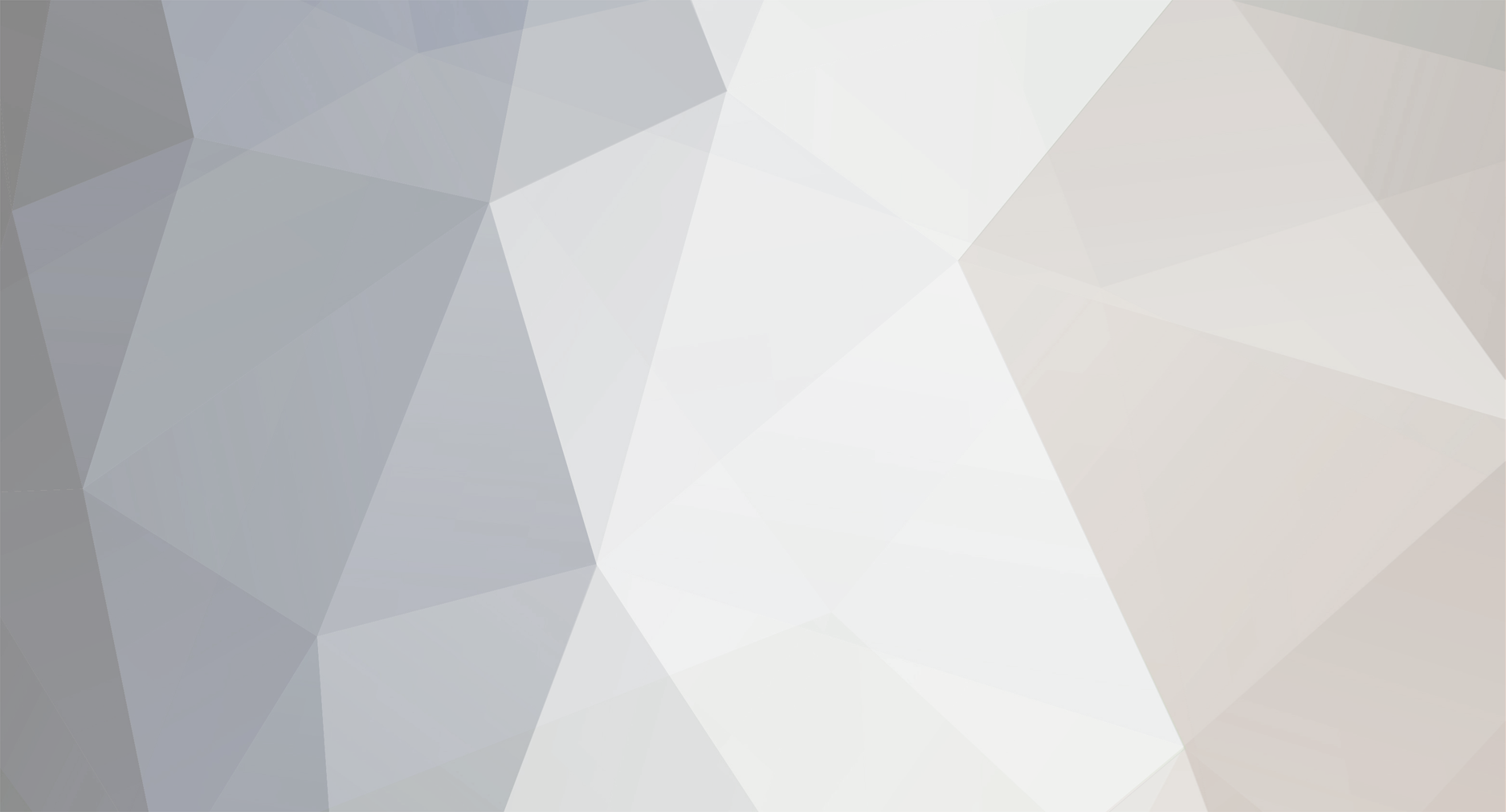
Northern Flame
-
Posts
816 -
Joined
-
Last visited
Never
Posts posted by Northern Flame
-
-
post the script that processes everything because its going to be hard to help you by only looking at the login form
***never mind for some reason it didnt let me scroll up on your code before but now i see it,
ill take a look at it right now
-
yea i probably should have mentioned that haha. well i do enjoy doing server side coding that is why i mentioned asp.net
i know i would probably have to go to school for that since i cant find any good tutorials for that online. i guess what i was
wondering was what which would benefit me more, which language is there a greater need for out in the job market. I do know
that a asp.net programmer would make a lot more than a php programmer would make, but are languages like C & C++ in greater
demand?
-
i used 2 be really active on this website but about a year ago i joined the army,
lately i have been thinking about my future after the army and thought about going
to college and studying some programming languages, but my only problem is that
im not sure which language would help me out the most career wise. I am already
proficient in HTML, CSS, Javascript, and PHP/AJAX. Im thinking about studying
languages like C & C++ or maybe even asp.net or pearl. any ideas as to which would
benefit me the most?
-
oh yea good point,
i totally forgot about the time function in SQL,
sorry i havnt worked with PHP in about a year so im still trying to refresh my mind
-
<?php
echo date("m/d/y") . ' ' . date("h:i a");
?>
-
you can give this a try:
$result = mysql_query("SELECT SUM(`views`) FROM `video` WHERE `username` = '$id'") or die(mysql_error());
if that doesnt work,
display the exact setup of your table
-
what number is exactly in the column views
-
I know how to make search scripts in PHP,
but can someone help me with making a script that will search for certain
database entries that are either 5 10 or 15 miles from a zip code?
Im guessing im going to have to have a huge list of zip codes in a database.
hopefully i can find something on google with a huge list of zip codes, but
if i end up getting a big list of zip codes, how can i search the locations in my
database that are a certain distance from those zip codes? or do I even need a
database full of zip codes? is there an easier way?
if someone can help me out with this or show me a tutorial on this I would greatly
appreciate it!
-
How can I make the following function return false:
<?php
function equalValues($one, $two){
if($one == $two){
return true;
} else{
return false;
}
}
if(equalValues("test", "TEST")){
echo "same";
} else{
echo "different";
}
?>
-
wow thanks,
that whole while statement in the middle of the sql
really helped! I would have never thought of that!
thanks!
-
well
$sqlr = "SELECT userid FROM jos_session";
will grab all the ids in the table jos_session
are you sure thats what you want?
-
I am developing a social networking site and i am finishing up the user profiles.
I am currently working on the friends list of the user, but I am having trouble
finding a way to have the friends table and on grabbing the data. Currently the
table is like this:
id - the id of the user
friends - list of ids of users on friends list, ex: 1,34,65,32,11
sent - list of ids of users that current user has sent friend requests to
pending - list of ids of users that have requested this user as a friend
the problem I am having is with grabbing the information of each friend on
the friends list when displaying the current users friends. The page will have
pagination, it will only display about 20 users per page.
I am wondering if I should do something like this:
<?php $q = mysql_query("SELECT `friends` FROM `friends` WHERE `id` = '$id'")or die(mysql_error()); $f = mysql_fetch_object($q); $array = explode(",", $f->friends); foreach($array as $key => $fid){ $query = mysql_query("SELECT `display`, `img`, `username` FROM `users` WHERE `id` = '$fid'"; $object = mysql_fetch_object($query); echo ''; // here i echo the users info }
now, is this the best way to do this?
run 20 queries in the foreach() loop or is there a better way?
Please help,
ive also considered rearranging my table but I cant find anything that will really work out better
than this solution.
-
you can just move that chunk of code to the top of where the main html is echoed.
if you need more help show us more code so we can see where you will need to move
that code to.
-
-
I am using the function htmlentities() to protect my website from people
trying to post html on my website, and also I added the ENT_QUOTES
option to the function so that it converts double quotes to " and
converts single quotes ' so is there a need for my to also use
mysql_real_escape_string? I guess what Im asking is if mysql_real_escape_string()
protects against more than single and double quotes.
-
alright thanks
-
thanks ill give that a try
2. Why do you use static methods (i.e. lyrics::search()) in this sense? They look like they use instance-level data... You might want to go over the OOP Section and look at methods once more.
I didnt know it made a big difference. I thought lyrics::search() and $echo->search() did the same thing?
the reason I use this method, lyrics::search(), is because a lot of the times the variables inside my classes
are established and used inside the functions of the class and I never have to call them, so I hardly ever
do stuff like:
$echo = new lyrics;
the only reason i did it in this case is because I needed to echo out the html that is generated in
the functions.
but for future reference, is there something wrong with doing it that way?
-
that looks correct,
but can we see more code?
-
put the array in a variable,
$array = array(/* your array here*/);
then change:
$strQueryString = "filetype=tab&cateogry=ALL&manufacturer=ALL&invtype=both&columndata=Array&input=SUBMIT"
to:
$strQueryString = "filetype=tab&cateogry=ALL&manufacturer=ALL&invtype=both&columndata=$array&input=SUBMIT"
-
I have a variable that is generated in the functions in my class,
but for some reason, I can't pass that variable in my new function
that is made outside of the class. Here's pretty much what I'm doing:
<?php include('./../_CLASSES/lyrics/view.php'); $echo = new lyrics; if(!isset($_GET['lyrics'])){ lyrics::search(); } else{ lyrics::grabInfo(mysql_real_escape_string($_GET['lyrics'])); lyrics::getThisEdit(); lyrics::filterLyrics(); lyrics::makeHTMLfriendly(); lyrics::generateHTML(); } function pageContent($html = $echo->html){ echo $html; } include('./../templates/'. $config['template'] .'/template.php'); ?>
I also tried:
<?php include('./../_CLASSES/lyrics/view.php'); $echo = new lyrics; if(!isset($_GET['lyrics'])){ lyrics::search(); } else{ lyrics::grabInfo(mysql_real_escape_string($_GET['lyrics'])); lyrics::getThisEdit(); lyrics::filterLyrics(); lyrics::makeHTMLfriendly(); lyrics::generateHTML(); } function pageContent($html = $echo){ echo $html->html; } include('./../templates/'. $config['template'] .'/template.php'); ?>
**Difference:
1)
function pageContent($html = $echo->html){
echo $html;
}
2)
function pageContent($html = $echo){
echo $html->html;
}
heres the error I receive both times:
Parse error: parse error, unexpected T_VARIABLE in /path/to/website.com/lyrics/view.php on line 17
heres line 17:
function pageContent($html = $echo->html){
can anyone please help?
-
I am developing a website and I have a directory, _CLASSES,
and I want to be able to include files from that directory and all its
sub-directories, but I dont want anyone to be able to view them.
can anyone show me a tutorial on .htaccess that deals with things
similar to this or can someone help me out by showing me how to do this?
thanks
Edit:
I dont want it to require a password to view the files in it,
but maybe show an error page when someone tries to access
the files in that directory or in any of its sub-directories.
-
the {} let you add in variables inside there to echo out with the HTML
example:
<?php $variable = "test"; echo <<<HTML <div> <p>This is a {$variable}</p> </div> HTML; ?>
and _TOTAL_NUMBERS must have been defined somewhere
example:
<?php define("_TOTAL_NUMBERS", 28); echo <<<HTML <div> <p>Your Total Number Is: {_TOTAL_NUMBERS}</p> </div> HTML; ?>
the define() function sets things just like variables do except its a constant
instead of a variable. read this:
-
what error do you get?
-
can you also show logged_in.php
[SOLVED] Login form not working
in PHP Coding Help
Posted
heres one problem i found,
$_SESSION['loggedin'] is not even set yet so its going to default to NULL and later on in the code it is set to 1,
that is why it gives you an error message first and then lets you log in....try this: