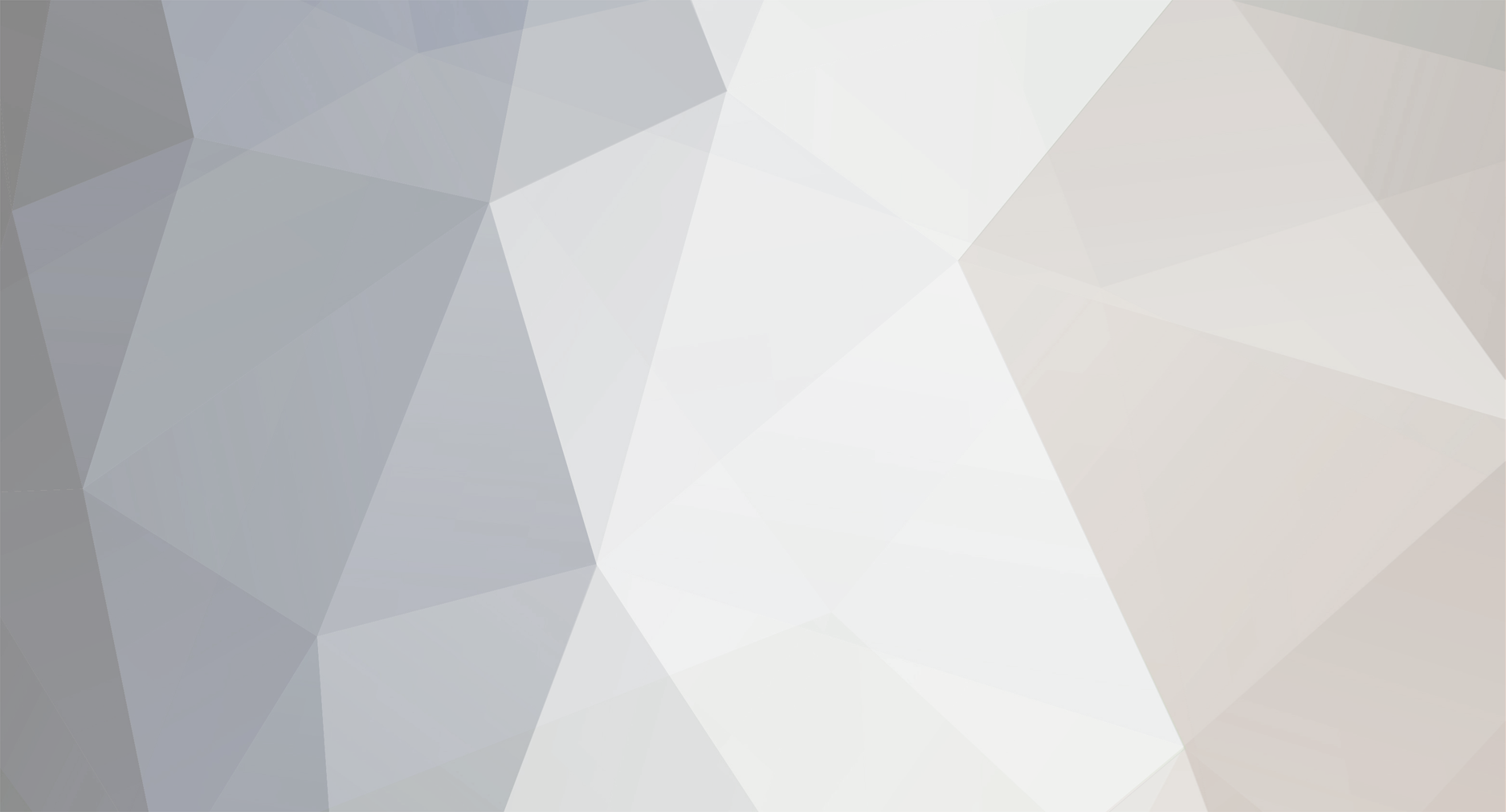
Northern Flame
Members-
Posts
816 -
Joined
-
Last visited
Never
Everything posted by Northern Flame
-
Yea I had the same problem when I tried the PHP Pagination tutorial from here, but I fixed it, heres my script, (you may need to edit some info!) <?php $localhost = 'localhost'; $user = 'username'; $password = 'password'; $database = 'db'; $mconn = mysql_connect($localhost, $user, $password); mysql_select_db($database, $mconn) or die(mysql_error()); $limit = 14; $query_count = "SELECT count(*) FROM table_name"; $result_count = mysql_query($query_count); $totalrows = mysql_num_rows($result_count); if(empty($page)){ $page = 1; } $limitvalue = $page * $limit - ($limit); $query = "SELECT * FROM table_name LIMIT $limitvalue, $limit"; $result = mysql_query($query) or die("Error: " . mysql_error()); if($page != 1){ $pageprev = $page--; echo "<a href=\"$PHP_SELF&page=$pageprev\">PREV".$limit."</a> | " ; }else{ echo ""; } $numofpages = $totalrows / $limit; for($i = 1; $i <= $numofpages; $i++){ if($i == $page){ echo('<font color=red>'.$i."</font> | "); }else{ echo("<a href=\"$PHP_SELF?page=$i\">$i</a> "); } } if(($totalrows % $limit) != 0){ if($i == $page){ echo('<font color=red>'.$i."</font> | "); }else{ echo("<a href=\"$PHP_SELF?page=$i\">$i</a> | "); } } if(($totalrows - ($limit * $page)) > 0){ $pagenext = $page++; echo("<a href=\"$PHP_SELF?page=$pagenext\">NEXT".$limit."</a><br><br>"); }else{ echo("<br><br>"); } if(mysql_num_rows($result) == 0){ echo("Nothing to Display!"); } $bgcolor = "#E0E0E0"; // light gray while($row = mysql_fetch_array($result)){ if ($bgcolor == "#E0E0E0"){ $bgcolor = "#FFFFFF"; }else{ $bgcolor = "#E0E0E0"; } echo '<a href="'.$row["file"].'">'.$row["name"].'</a><br><br>'; } if($page != 1){ $pageprev = $page--; echo("<br><br><a href=\"$PHP_SELF&page=$pageprev\">PREV".$limit."</a> | "); }else{ echo("<br><br>"); } $numofpages = $totalrows / $limit; for($i = 1; $i <= $numofpages; $i++){ if($i == $page){ echo('<font color=red>'.$i."</font> | "); }else{ echo("<a href=\"$PHP_SELF?page=$i\">$i</a> "); } } if(($totalrows % $limit) != 0){ if($i == $page){ echo('<font color=red>'.$i."</font> | "); }else{ echo("<a href=\"$PHP_SELF?page=$i\">$i</a> | "); } } if(($totalrows - ($limit * $page)) > 0){ $pagenext = $page++; echo("<a href=\"$PHP_SELF?page=$pagenext\">NEXT".$limit."</a>"); }else{ echo(""); } mysql_free_result($result); mysql_close($mconn); ?> By the way, I have my page numbers on the top and bottom of the results
-
oh and by the way, you formatted your form at the very end wrong, you put <method='POST' id='frm'> when it should be <form method='POST' id='frm'>
-
I found your problem, try this: <?php include('Connect.php'); $userID = $_COOKIE['UserID']; $submit = $_POST['submit']; $pokemonname = $_POST['pokemon_name']; $attack = $_POST['pokemon_attack']; $defence = $_POST['pokemon_defence']; $pokemonimage = $_POST['pokemon_image']; $pokemonimage2 = $_POST['pokemon_icon']; $gender = $_POST['pokemon_gender']; $result="INSERT INTO news_comments (user_id,pokemon_name,pokemon_attack, pokemon_defence, pokemon_image, pokemon_gender, pokemon_icon) values ('$userID','$pokemonname','$attack','$defence','$pokemonimage','$gender','$pokemonimage2')"; if (!isset($_COOKIE['UserID'])) { echo "Sorry, you are not logged in."; include('bottom.php'); exit; } if(!mysql_num_rows(mysql_query($result) == 0)) { echo "Sorry, you already have your starter"; include('bottom.php'); exit; mysql_num_rows(mysql_query($result)or die(mysql_error())); if ($submit) { if($pokemonname=="Turtwig"){ $pokemonname="Turtwig"; } elseif($pokemonname=="Piplup"){ $pokemonname="Piplup"; } elseif($pokemonname=="Chimchar"){ $pokemonname="Chimchar"; } else{ $pokemonname="Turtwig"; } } if($gender=="Male"){ $gender="Male"; } elseif($gender=="Female"){ $gender="Female"; } else{ $gender="Male"; } if($pokemonname=="Turtwig"){ $pokemonimage="http://i9.tinypic.com/6sapvo1.png"; } elseif($pokemonname=="Piplup"){ $pokemonimage="http://i13.tinypic.com/6jzrsdv.png"; } elseif($pokemonname=="Chimchar"){ $pokemonimage="http://i10.tinypic.com/6p98rch.png"; } else{ $pokemonimage="http://i9.tinypic.com/6sapvo1.png"; } if($pokemonname=="Turtwig"){ $pokemonimage2="http://i12.tinypic.com/81i2tkl.png"; } elseif($pokemonname=="Piplup"){ $pokemonimage2="http://i4.tinypic.com/6uepa2h.png"; } elseif($pokemonname=="Chimchar"){ $pokemonimage2="http://i15.tinypic.com/717m0oy.png"; } else{ $pokemonimage2="http://i12.tinypic.com/81i2tkl.png"; } if($pokemonname=="Turtwig"){ $attack="15"; } elseif($pokemonname=="Piplup"){ $attack="20"; } elseif($pokemonname=="Chimchar"){ $attack="18"; } else{ $attack="15"; } if($pokemonname=="Turtwig"){ $defence="20"; } elseif($pokemon_name=="Piplup"){ $defence="15"; } elseif($pokemon_name=="Chimchar"){ $defence="17"; } else{ $defence="20"; } echo "You have obtained your starter. It is $pokemonname."; } else{ echo "<html> <method='POST' id='frm'> <input type='hidden' name='submit' value='1'><br> <b>Choose your pokemon</b><br /> <select name='pokemon_name'> <option value='Turtwig'>Turtwig</option> <option value='Piplup'>Piplup</option> <option value='Chimchar'>Chimchar</option> </select> <b> Choose it's gender</b> <br /> <select name='pokemon_gender'> <option value='Male'>Male</option> <option value='Female'>Female</option> </select> <input type='submit' name='submit' value='Get starter'> </form> </html>"; } include('bottom.php'); exit; ?> By the way, I suggest you use a switch() instead of all those elseif statements
-
sometimes when you format your script by indenting you can easily tell where you need to close brackets, example: <?php // this is only an example, not a solution to your problem! $variable = $_POST['data']; if(isset($variable)){ function testFunction($variable){ switch($variable){ case 'test': echo 'test'; break; case 'testing': echo 'testing'; break; default: echo 'empty'; break; } } } ?> Now you see how each bracket lined up so that you can easily tell where the command starts and ends? indenting really makes your script easier to read!
-
I have never worked with anything like what you are doing, but I can tell you this what you need to input, I just dont know where to input it. insert "\n" when you want a line break and as far as the spacing you can just have a variable with the spacing and insert that before text, example: <?php $level_1 = ' '; $level_2 = ' '; // echo $level_1 and then insert the text and then put \n; // echo $level_2 and then insert the text and then put \n; ?>
-
in your explode() function you are telling it to look for /r when it should be looking for \n
-
can we see your PHP script?
-
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
lol damn I left for like 5 minutes to eat and you guys are just about ready to kill each other! -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
what is the id of your first comment? 1 or 0 if its 0 then that might be why its not showing it.... not sure though -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
try this: <?php include('Connect.php'); include('top.php'); $result = mysql_query("SELECT id_comment FROM news_comments ORDER BY id_comment DESC"); echo "<table border='1' bgcolor='lightgrey'> <tr> <th>Hi,<br /> It's Xyphon. I have just made the news page, I hope you like it! Please, leave comments here!</th> </tr></table>"; echo "<table border='1' width='500' bgcolor='lightgrey'>"; echo "<tr> <td> <a href='postcomment.php'>Post Comment</a></td>"; echo "<td> <a href='viewcomments.php'>View Comments</a></td></tr></table>"; if(!$row = mysql_fetch_array($result)){ echo "There are no current comments. Layout issues may accure."; } else { while($row = mysql_fetch_array($result)){ echo "<table border='1' width='500' height='20' bgcolor='lightgrey'>"; echo "<br /><br />"; echo "<tr>"; echo "<td><center><b>Username: </b><br />" . $row['username'] . "</center></td>"; echo "<td><center><b>ID: </b><br />" . $row['user_id'] . "</center></td></tr></table>"; echo "<table border='1' width='500' height='20' bgcolor='lightgrey'><tr><td><b>Comment:</b>"; echo "<td>" . $row['comment'] . "</td>"; echo "</tr></table>"; } } include('bottom.php'); ?> -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
what exactly are your results? can you post your script so that I can see how you are doing it? -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
Oh wait, are you using mysql_fetch_array() or are you just using mysql_query() you need to add a while() loop with the mysql_fetch_array() function -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
oh, well if he has an ID column that auto increases so that each ID is unique and the newest post gets the newest ID, wont that work the same? -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
try ASC like phpSensei suggested -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
Doesn't DESC give you the last one first? http://www.w3schools.com/php/php_mysql_order_by.asp#table8 -
The MySQL help froum is inactive, so can I post this here?
Northern Flame replied to Xyphon's topic in PHP Coding Help
mysql_query("SELECT * FROM comments ORDER BY id DESC"); you can change the ORDER BY thingy but all you really need is the DESC to make it go backwards -
try this: <?php // CONNECT TO YOUR DATABASE! // You need to change the table name to your table name! $query = mysql_query("SELECT * FROM users"); while($row = mysql_fetch_array($query)){ // edit the column names below $add = $row['column1'] + $row['column2'] + $row['column3']; $result = $add / 3; $user = $row['user']; mysql_query("UPDATE users SET average = '$result' WHERE user = '$user'"); } $x = 0; $select = mysql_query("SELECT * FROM users ORDER BY average DESC"); while($var = mysql_fetch_array($select)){ $person = $var['user']; mysql_query("UPDATE users SET rank = '$x' WHERE user = '$person'"); $x++; } ?> Be sure to edit all the information above to fit your MySQL Database Columns!
-
I have some post data that comes in from a text area and when the user types in symbol such as ' it returns it as \'. I know this is necessary for my script to do this to protect it from damaging my script, but is there a way to edit that out? Is there a certain way that I need to do this for my post data or can the function str_replace() find all the \ out of my post data and delete those?
-
I'm pretty sure this needs to be done with AJAX (if you intend to use PHP) but I am not exactly sure how, sorry, but try this: http://www.google.com/search?hl=en&safe=off&q=file+upload+status+tutorial+PHP&btnG=Search
-
oh i was talking about putting in a switch statement to the first half of your script
-
when he says to use a switch, he means this http://www.tizag.com/phpT/switch.php
-
[SOLVED] value="<?=$_POST['mail']?> shows in email
Northern Flame replied to j634's topic in PHP Coding Help
try this: <?php if($_GET['f']=='forgot') { ?> <form method="post" action="users.php?f=forgot" style="margin:0px"> <input type="hidden" name="a" value="forgot"> <?php $t=explode(' ', microtime()); $uniqCode=uniqid($t[1]); session_register('uniqCode'); print ('<input type="hidden" name="uniqId" value="'.$uniqCode.'">'); ?> <table align="center" border="0" cellspacing="5"> <tr><td style="text-align:center; height:21px; font-weight:bold" colspan="2">Enter your e-mail:</td></tr> <tr><td width="50">E-mail: </td><td width="180"><input type="text" name="mail" value="<?php echo $_POST['mail']; ?>"></td></tr> <tr><td style="text-align:center"; colspan="2"><input type="button" value=" SUBMIT " onClick="this.form.submit();this.disabled=true"></td></tr> </table> -
what exactly do you plan to use the number for? and how will you get this number (post data, pre-defined, etc) there are ways to do this, but it depends on certain information
-
you also need to store the text you want displayed in the link, then you simply just connect to your database and run this: <?php /* CONNECT TO DATABASE! */ $query = mysql_query("SELECT * FROM table"); while($row = mysql_fetch_array($query)){ echo '<a href="'.$row['url'].'">'.$row['text'].'</a><br>'."\n"; } ?>