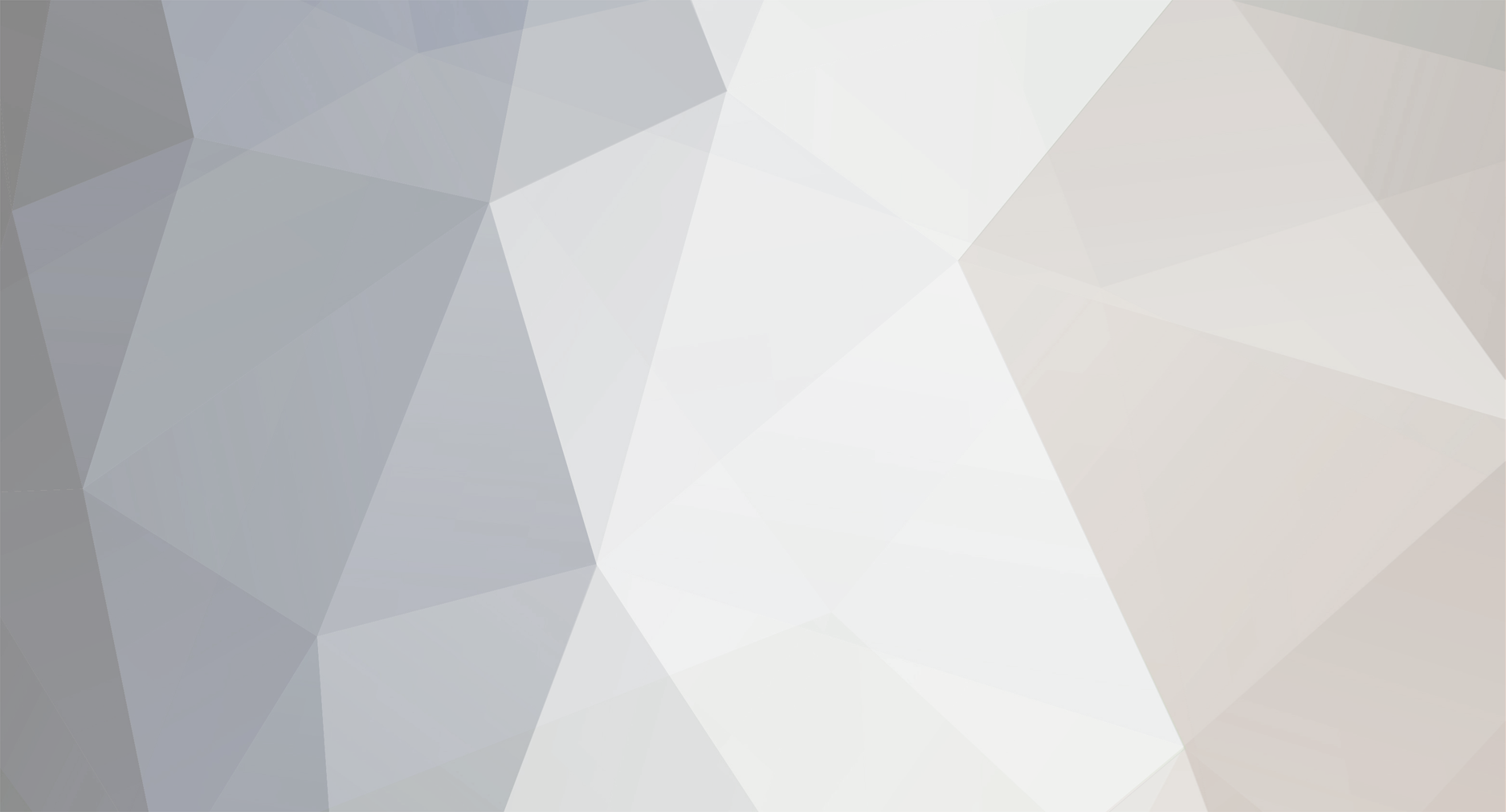
samoht
-
Posts
421 -
Joined
-
Last visited
Posts posted by samoht
-
-
ajax.functions.php
function wpsc_add_to_cart() { global $wpdb, $wpsc_cart, $wpsc_theme_path; /// default values $default_parameters['variation_values'] = null; $default_parameters['quantity'] = 1; $default_parameters['provided_price'] = null; $default_parameters['comment'] =null; $default_parameters['time_requested']= null; $default_parameters['custom_message'] = null; $default_parameters['file_data'] = null; $default_parameters['is_customisable'] = false; $default_parameters['meta'] = null;
This is the function that actually adds items to the cart and what you'll need to use. However, you will need to come up with a way to call this function properly - and it looks as though they are calling it from an ajax script. I assume that you still want a "Add to Cart" button so that the user will see your page with all the ingredients and the quantities for a certain recipe and from that page simply hit the "Add to Cart"?
I think we'll have to create a custom function that is triggered by your "Add to Cart" and that will loop through each product to call the function wpsc_add_to_cart()
-
I'm not sure about this, but it may work to put each product_id in a hidden input like this:
foreach($products as $key => $value) { $product = $wpdb->get_row("SELECT meta.product_id as pid, list.name AS name, list.price AS price FROM ".WPSC_TABLE_PRODUCTMETA." AS meta INNER JOIN ".WPSC_TABLE_PRODUCT_LIST." AS list ON ( list.id = meta.product_id ) WHERE `meta_key` IN ( 'sku' ) AND `meta_value` IN ( '{$key}' ) ORDER BY list.id DESC"); echo $value .' x '. $product->name .' @ '. $product->price .'<br />'; echo '<input type="hidden" name="product_id[]" value="' .$product->pid. '">'; }
However, It looks like the wpsc_add_to_cart_button function only processes one product id at a time.
(I don't think you want wpsc_add_to_cart_button() anyway since this just shows the add to cart button)
The best thing I can think to do is find the function they use to process the form - then we can use that code to populate the cart with multiple products.
-
Why is your textarea an array value?
try
<td><textarea name="text">Today is saturday, no work today.</textarea></td>
-
you set the value to an array here:
<select name="bizCatSelect[]" size="4" multiple>
-
not sure I understand your issue. Are you sending the values of a form using a submit button?
Maybe you could post some code as an example?
-
can you assign a 'child' to be the parent of another 'child'?
if so then this function should still work. I think we would need to see the code that calls this function
-
Opps yes I am sorry you have an object not an array so the code should look like:
<?php if (is_page()) { } else { if(!empty($wpdb->prefix)) { $wp_table_prefix = $wpdb->prefix; } else if(!empty($table_prefix)) { $wp_table_prefix = $table_prefix; } // Define the database table names define('WPSC_TABLE_PRODUCT_LIST', "{$wp_table_prefix}wpsc_product_list"); define('WPSC_TABLE_PRODUCTMETA', "{$wp_table_prefix}wpsc_productmeta"); $cf="Linked Products"; $sku = get_post_meta($post->ID, $cf, true); $array = explode(",",$sku); $products = array_count_values($array); foreach($products as $key => $value) { $product = $wpdb->get_row("SELECT meta.product_id, list.name AS name, list.price AS price FROM ".WPSC_TABLE_PRODUCTMETA." AS meta INNER JOIN ".WPSC_TABLE_PRODUCT_LIST." AS list ON ( list.id = meta.product_id ) WHERE `meta_key` IN ( 'sku' ) AND `meta_value` IN ( '{$key}' ) ORDER BY list.id DESC"); echo $value .'x'. $product->name .'@'. $product->price .'<br />'; } }
-
to make sure the sql works put it directly into your phpmyadmin and replace the table names and the $key value if the sql returns an error let me know what it is
BTW, there is no extra table to check - it is just that the sku's are stored on the productmeta table and those are the values that you know. You want the product name and price which is stored on the product_list table and what links them is the product_id. So using INNER JOIN we grab the product_id where the sku is what we have and then pull the name and price from the other table.
-
Sorry but here is the correct query
$product = $wpdb->get_row("SELECT meta.product_id, list.name AS name, list.price AS price FROM ".WPSC_TABLE_PRODUCTMETA." AS meta INNER JOIN ".WPSC_TABLE_PRODUCT_LIST." AS list ON ( list.id = meta.product_id ) WHERE `meta_key` IN ( 'sku' ) AND `meta_value` IN ( '{$key}' ) ORDER BY list.id DESC");
I was having problems with the `
hate those things
-
OK,
You can try this for now:
<?php if (is_page()) { } else { if(!empty($wpdb->prefix)) { $wp_table_prefix = $wpdb->prefix; } else if(!empty($table_prefix)) { $wp_table_prefix = $table_prefix; } // Define the database table names define('WPSC_TABLE_PRODUCT_LIST', "{$wp_table_prefix}wpsc_product_list"); define('WPSC_TABLE_PRODUCTMETA', "{$wp_table_prefix}wpsc_productmeta"); $cf="Linked Products"; $sku = get_post_meta($post->ID, $cf, true); $array = explode(",",$sku); $products = array_count_values($array); foreach($products as $key => $value) { $product = $wpdb->get_row(" SELECT `meta.product_id`, list.name as name, list.price as price FROM `".WPSC_TABLE_PRODUCTMETA." as meta` INNER JOIN `".WPSC_TABLE_PRODUCT_LIST." as list` ON (list.id = meta.product_id) WHERE `meta_key` IN ( 'sku' ) AND `meta_value` IN ( '{$key}' ) ORDER BY `id` DESC "); echo $value .'x'. $product['name'] .'@'. $product['price'] .'<br />'; } }
This is not the best solution - but it should work.
if you have trouble with the query - try putting it all on one line like:
$product = $wpdb->get_row("SELECT `meta.product_id`, `list.name` as name, `list.price` as price FROM `".WPSC_TABLE_PRODUCTMETA."` as meta INNER JOIN `".WPSC_TABLE_PRODUCT_LIST."` as list ON (list.id = meta.product_id) WHERE `meta_key` IN ( 'sku' ) AND `meta_value` IN ( '{$key}' ) ORDER BY `id` DESC ");
-
Yes, I think am starting to understand.
Are you saying that you are manually adding the sku's that you need for a recipe as a custom field "Ref" in your post - and then looking for a way to query the db for the product_id's associated with the sku's?
-
OK,
is the FRE0001 the 'sku' in the productsmeta table? (because I could not find a product code )
I noticed their code looks like:
$product_id = $wpdb->get_var("SELECT `product_id` FROM `".WPSC_TABLE_PRODUCTMETA."` WHERE `meta_key` IN ( 'url_name' ) AND `meta_value` IN ( '{$wp_query->query_vars['product_url_name']}' ) ORDER BY `id` DESC LIMIT 1"); $full_product_name = $wpdb->get_var("SELECT `name` FROM `".WPSC_TABLE_PRODUCT_LIST."` WHERE `id`='{$product_id}' LIMIT 1");
When they have to retrieve the product name and the values they have are in the productmeta table.
I'll take a look further and see what I come up with. If I can recreate the situation your in it would be helpful.
Are you writing this code on a custom template for your theme?? (can you chow me the whole page?)
-
each entry has it's own unique values that are not arrays.
entry 1 =
Mark Name = entry 1's mark name
Shortname = entry 1's short name
...
etc
entry 2 =
Mark Name = entry 2's mark name
Shortname = entry 2's short name
...
etc
and so on.
that means that you should have an array of entries and each entry will have an array of data - but the Mark name will NOT be an array. it is still unique per entry.
Also, you should not do the for loop the way that you show. We can use a foreach loop to loop through each entry if we need.
You mentioned a SQL code. What do you need from the DB??
-
you should consider using javascript instead of marquee too
-
yes, but you need to define $id and you need to provide an excape from the function otherwise you'll be in an infinite loop.
-
don't confuse js and php
client side and server side need to stay on their sides
Anyway, your form is not collecting multiple shortNames so you should not try to store them in an array.
php will process the $_POST array NOT javascript.
-
firstly, try adding value="" to your text inputs
<div id="" style="display: block;"> <input value="Remove Mark" onclick="this.parentNode.parentNode.removeChild(this.parentNode);" type="button"><br> Mark Name:<sup>*</sup> <input name="markname[]" type="text" value=""> Short Name:<sup>*</sup> <input name="shortname[]" size="3" type="text" value=""><br><br> Description: <input name="description[]" type="text" value=""><br><br> Longitude:<sup>*</sup> <input name="longitude[]" size="8" type="text" value=""> Latitude:<sup>*</sup> <input name="latitude[]" size="8" type="text" value=""><br> <hr width="75%" align="center"> </div>
Second - These don't look like arrays to me. You are just collecting one bit of data. Now if you had checkboxes - yes, store that in array. If you for some odd reason want mutliple text data for every entry then you can use name="somevalue[]" but you need it on more than one input for it to be an array
-
so what page is the form on?
3.php ??
-
Wow, I can't believe I didn't see that you were missing that.
use mjdamato's suggestion to print out the $_POST array and see if all your content is there
-
Yes!
It is not doing anything anyway. I suppose you could use extract() to get your mysql results into php var's but I wouldn't do that if you return more than one row from the db.
-
what thorpe is getting at is that you don't automatically have the query results as php variables. You need to set them as such or use $row['shortName'] etc instead of $shortName
But this :
$fieldarray = array('characterName','shortName','height','weight','hometown'); foreach ($fieldarray as $fieldlabel) { if (isset($row[$fieldlabel])) { $fieldlabel = $row[$fieldlabel]; } }
makes no sense
-
I'm not sure. I need more of the code from the sending page to be able to tell.
But at this point if $_POST['addclub'] is blank - then nothing will happen
-
What does the url look like on the action page?
-
try adding a value="" to your text input
Trouble with array
in PHP Coding Help
Posted
OK,
I am thinking through a way to do this, but I wondered if it wouldn't be better to actually have certain recipes be products themselves?
That not only would make the coding much simpler but it would also look nicer on your site. all of the component products and their quantities would then just go into the description field for the recipe and the recipe would have a single cost etc.
What do you think?