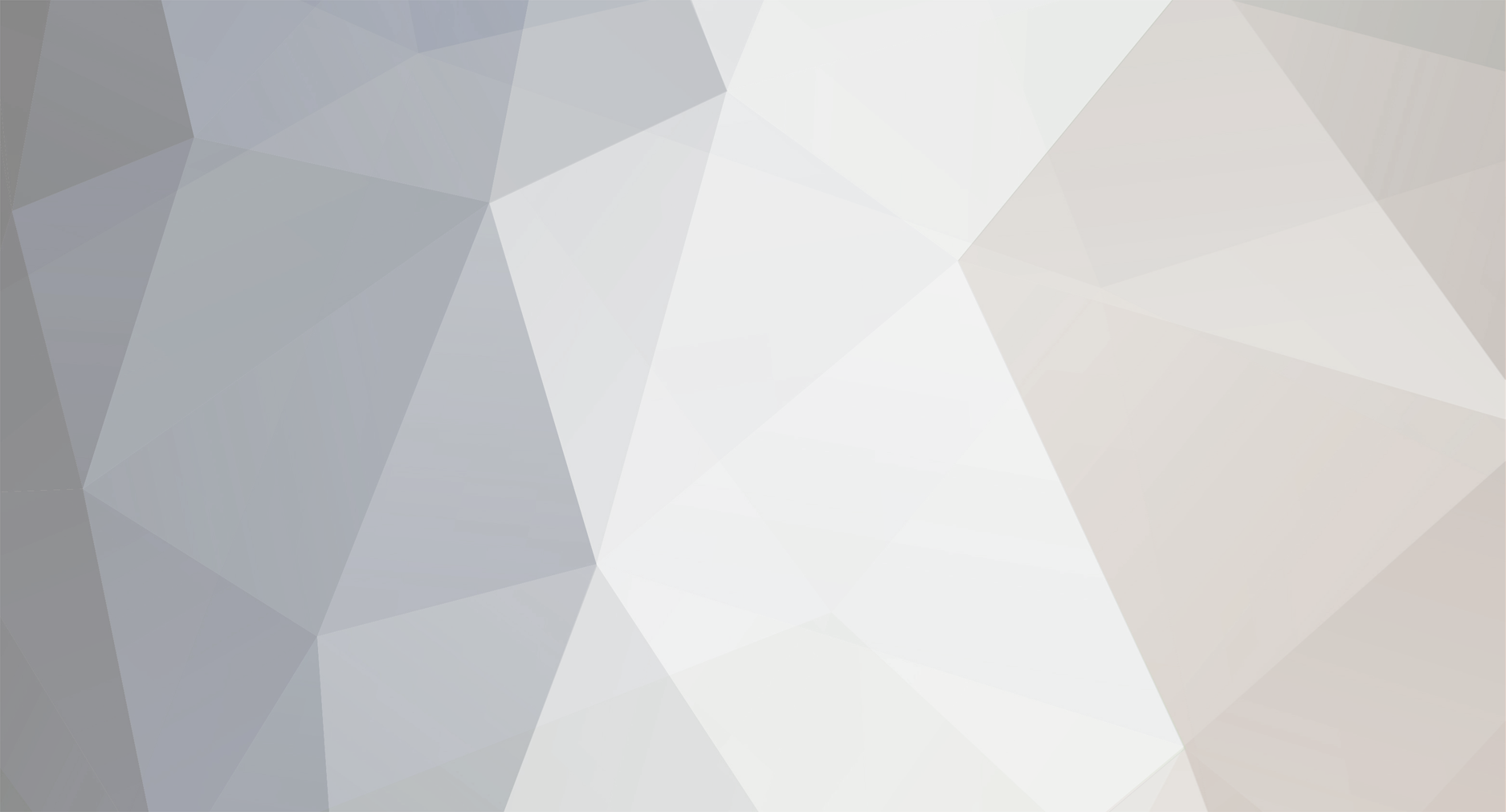
Cless
Members-
Posts
191 -
Joined
-
Last visited
Everything posted by Cless
-
If you are working on a big table though, the second one is most likely going to be faster (but still, very slightly). The first code gets extra persistent storage and loads it into memory. The second one simply does some binary manipulation with the memory without calling extra persistent storage. PHP tends to be faster than MySQL. Plus, if you have queries elsewhere that update that table/insert entries, adding more fields will of course have a performance impact. All in all, you really shouldn't be too concerned about it, unless you really have to worry about performance (an online gake/MMO/corporate website). The difference will probably be in microseconds, which are extremely small.
-
pear system_daemon runs on debian but wont start on rhel
Cless replied to jmurch's topic in PHP Coding Help
Please be more detailed. Any answers you might get are generally only as detailed as the question asked. -
Not to mention you shouldn't be using the same ID for different elements.
-
PHP/CSS issue - Won't load divs images when echoed through PHP
Cless replied to dragon2309's topic in CSS Help
Try pinning down the error by incrementally deleting parts of the PHP code. I don't see anything particularly wrong with it. Also, try putting ob_start() at the top of the PHP code. -
PHP/CSS issue - Won't load divs images when echoed through PHP
Cless replied to dragon2309's topic in CSS Help
If the problem lies with the PHP as you say, you should post the PHP. Otherwise, properly diagnosing the problem will be a tedious task. -
What am I doing wrong? This looks to be so simple.
Cless replied to fizzzgigg's topic in PHP Coding Help
$conid = $_GET['conid']; $result = mysql_query("SELECT * FROM `content` WHERE `cid`='$conid' LIMIT 1"); $row = mysql_fetch_array( $result ); if(empty($row['content'])) { echo "No files submitted."; } Edit: I see you're trying to change the value of $row. I'm pretty sure it only takes the first character because $row is an array. -
Well, I spent over an hour trying to figure this out, and I think I've finally figured it out. <?php if(!empty($_GET['delid']) && $_SESSION['login'] == "true" && !empty($_GET['article'])) { if($_SESSION['level'] == "rootuser" || $_SESSION['level'] == "admin" || $_SESSION['level'] == "moderator") { $comments['article'] = $_GET['article'] . ".xml"; $doc = new DOMDocument(); $doc->load("../../xml/" . $comments['article'] . ".comments"); $searchNodes = $doc->getElementsByTagName("comment"); $domElemsToRemove = array(); foreach($searchNodes as $searchNode) { $check = preg_replace("/%20/", "", $_GET['delid']); $valueID = $searchNode->getElementsByTagName("id")->item(0)->nodeValue; if($valueID == $check) { $domElemsToRemove[] = $searchNode; } } foreach( $domElemsToRemove as $domElement ){ $domElement->parentNode->removeChild($domElement); $deleted = "deleted"; } if($deleted == "deleted") { $doc->save("../../xml/" . $comments['article'] . ".comments"); header("Location: /error.php?title=Comment%20Deleted&msg=Comment%20Deleted"); } else { header("Location: /error.php?title=Error&msg=ID%20Does%20Not%20Exist!"); } } else { header("Location: /error.php?title=Error!&msg=You%20do%20not%20have%20Permission%20to%20delete%20Comments!"); } } ?> First of all: if($valueID == $check) $valueID was not a string, and thus could not be compared to one, so: $valueID = $searchNode->getElementsByTagName("id")->item(0)->nodeValue; nodeValue had to be added in order to get the text value of the ID and compare it to $check. Secondly, to remove the child successfully, I had to put it in an array then later use foreach() to delete it. Make sure that the XML file has proper file permissions to be modified and such.
-
So there's no error messages? The only thing wrong is that it is not properly deleting the element and writing it to the file?
-
Pretty sure that kind of error is in relation to removeChild. Hence, you're attempting to delete an element that does not exist, or so it seems. When does this happen? Also, is there any more to the error code? Such as line numbers, etc.
-
I'm relatively new to Java programming, to a certain extent. More specifically, new to programming applets. I used to do Java programming somewhat about three years ago, but not too regularly. Anyway, I had attempted to create an image and display it to the screen outside of the paint() function. It is in a manually created method called "update_text". m_letter1= getImage(getCodeBase(), ut_character); m_graphics1.drawImage(m_letter1, 21, 21, this); This executes at the end of the update_text method. However, I get a NullExceptionError when it runs, and therefore the image is not displayed. Here (before the above code is executed), apparently m_letter1 and m_graphics1 are null. ut_character is not. After executing the above code, only m_graphics1 is null. Outside of the update_text method, I defined the graphics and image variables (m_letter1 and m_graphics1): private Image m_letter1; private Graphics m_graphics1; This is the error I get: Exception in thread "Thread-5" java.lang.NullPointerException at base.Main.update_text(Main.java:113) at base.Main.run(Main.java:156) at java.lang.Thread.run(Thread.java:619) Main.java:113: m_graphics1.drawImage(m_letter1, 21, 21, this); Main.java.156: update_text(); Anyway, I was wondering if there was a way to fix this. I can't necessarily use the paint method, I'm pretty sure, since I don't want all of this displayed exactly at run time. Any help would be much appreciated.
-
I use PHP and MySQL on almost every page of my website. I also use AJAX excessively. Anyway, while users are browsing upon my website, sometimes they get this error: Can't connect to local MySQL server through socket '/var/lib/mysql/mysql.sock' Does this mean there are too many connections? At one time I usually have 500~600 users online. This error doesn't happen too often but it can be annoying. Does anybody know why this occurs?
-
So is that just another way of saying that mysql_num_rows() is best? =P
-
For a query such as: SELECT count(*) FROM table LIMIT 5 What would be the best way to count the rows found? I'm aware you can use: mysql_num_rows (though I don't think you can use count() with this), mysql_result, and mysql_fetch_row, depending on the circumstance. I've heard mysql_result($rows, 0) is the most efficient, however, if there are no rows found, it will give an error. So what do you recommend?
-
There. Apparently cron wasn't the answer. I just ran my query (but I used set_time_limit(0) and turned my site offline) and it ran perfectly, surprisingly. Didn't even lag.
-
Thank you. I will try it out and post my results later on.
-
Yeah, that's what I was planning. However, in the cPanel, I'm not exactly sure what to type in as the command to run.
-
Would you know what command I would use to call the file? I can use cPanel's cron feature to do it, but I haven't use cron much, so I'm not quite sure what command to use.
-
Yeah, would cron be good for doing that? Or even, the use of JavaScript setInterval and AJAX.
-
Maybe. Would cron jobs be sufficient for something like this?
-
That's not what I'm actually trying to do (was a basic example). There are some MySQL checks during the loop, and some other inserts in that loop.
-
Well, it's not an exact copy: it needs to select the data, convert it (divide some fields by 2, etc.) then insert the converted data into a different database. For example: <?php $result= mysql_query("SELECT * FROM table"); while($rows= mysql_fetch_array($result)) { $number1= $rows['number1']; $number2= $rows['number2']; $number3= $rows['number3']; $number1= $number1 / 2; $number2= $number2 + 5; $number3= $number3 * 10; $result2= mysql_query("SELECT * FROM table2 WHERE number='$number1' LIMIT 1"); $rows2= mysql_fetch_array($result2); if(empty($rows2)) { $number1= $number1 + 5; } //change database here mysql_query("INSERT INTO table_thats_in_the_other_database (number1, number2, number3) VALUES ('$number1', '$number2', '$number3')"); } ?> So, basically, I have to loop through a table with about 2.7 million entries, do some conversions, check for certain thing using MySQL, then insert the final results in the new database.
-
This is probably going to sound odd, but anyway: I need to loop through almost 3 million+ entries in an MySQL database. Obviously using the while statement for this is not ideal. What would be the best way to do this? Cron jobs? Or is there some sort of premade script that would allow for me to do efficiently do this? What I have to do is loop through these entries, change some things, then essentially duplicate the entry to a different database on my server (not an exact copy: it converts many things before doing this), and do some other checks. For the record, I have a 3.0 GHz quad server, 8 GB of ram.
-
Thanks!
-
I need to iterate through a directory, which only contains .txt files. Except, I need to use PHP, preferably. What I want to do is loop through the contents of all files in a directory: open the file, use the str_replace function on the file, save it, then move onto the next file. Is there a way to do this with PHP?