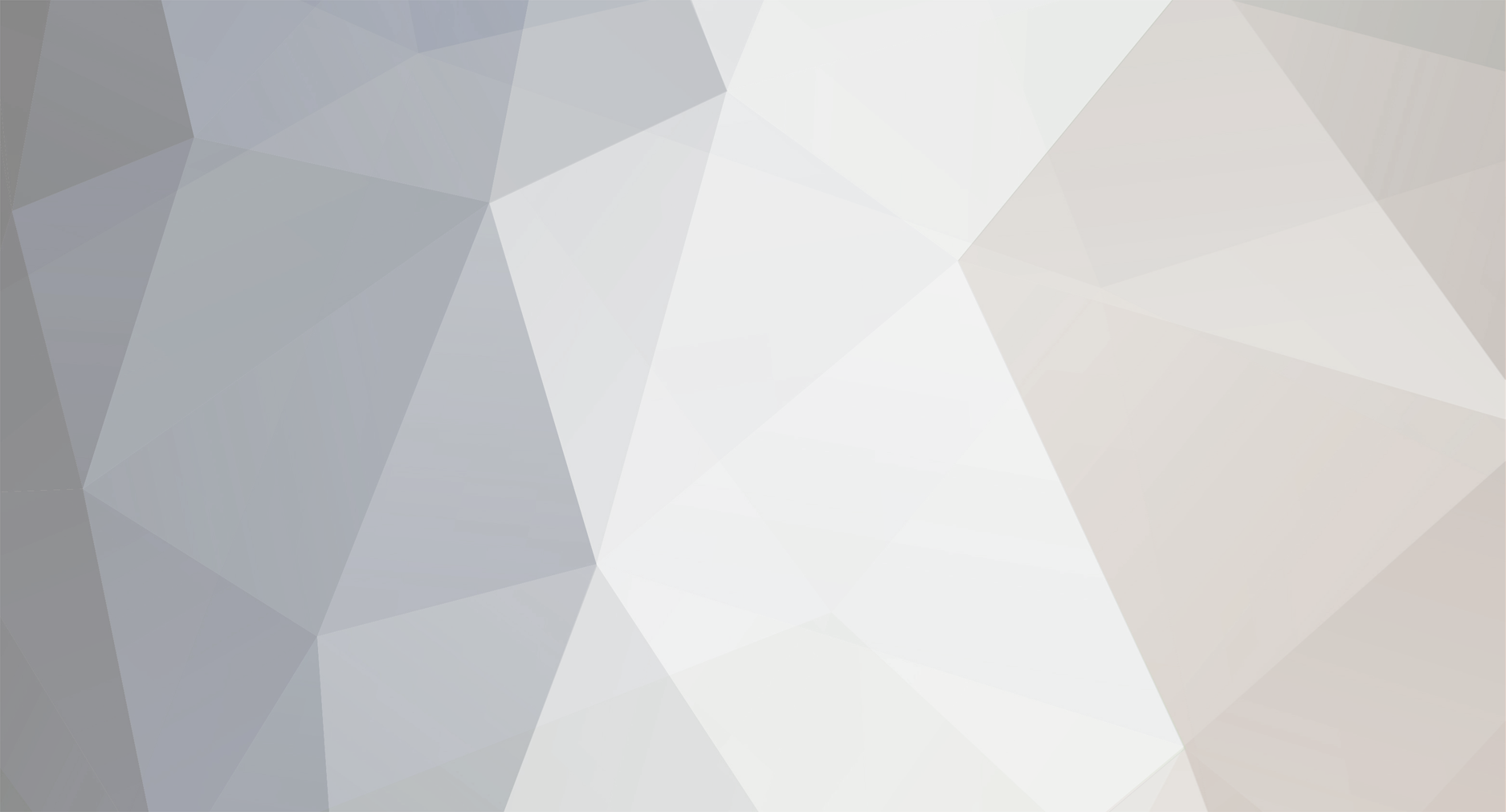
NArc0t1c
-
Posts
299 -
Joined
-
Last visited
Never
Posts posted by NArc0t1c
-
-
Ajax maybe, sending single requests and getting feedback using fsockopen.
-
In more detail:
You make an upload script using php, to upload the file to a temporary dictionary.
Once it's uploaded you can read from the using various functions, it depends on what you want to do with the text file.
And then delete the file using unlink or something similar, keeping the text file will result in loss of client security.
-
133 times?
And error messages could be avoidable(ever heard of the at(@) sign).
I just cannot think of any examples on how I could use that for an application that would be better than a while loop(maybe there isn't).
But it is "unique", and not wrong.
-
Short and simple - No.
Edit:
It may be you're javascripting, try removing some.
-
It's slower the way you did it - guess you could say it's wrong.
Actually a clever way, most people would not have thought of it.
It's another method of doing it, it's not "Wrong".
-
So basically everyone Likes what the client does ?
-
Try contacting the webmaster of that website.
-
Ooh..
<?php $Number = rand(1,5); If ($exp =< 100){ if ($Number < 4){ Include "You Loose.php"; } else { Include "You Win.php"; } else { if ($Number < 2){ Include "You Loose.php"; } else { Include "You Win.php"; } } ?>
Edit: Sorry, was afk, only saw the otehrs now.
-
Try inserting an row in the table that hold the active coloum.
And then add a update query to every page(include a single file to do it) to update it to current time.
And then you could use this to see if they are active:
<?php $Limit = 3600; //A hour; $LastTime = mysql_query("SELECT last_time FROM table WHERE user='$user'); $Fetch = mysql_fetch_row($LastTime); if ($Fetch['last_time'] >= $Limit){ echo 'User is not active.'; } else { echo 'User Is active.'; } ?>
-
Try the ext variable as the haystack.
if(in_array($ext_list,$ext)){ echo '<li><a href="'.$image.'"><img src="'.$image.'" width="25"> '.$image.'</a></li>\n'; }
--Edit
Also try:
<?php $ext_list = array ('jpg', 'JPG', 'gif', 'GIF', 'png', 'PNG', 'JPEG', 'jpeg'); $list_ignore = array ('.','..'); if($handle = opendir('.')){ echo "Directories:<ul>"; while($dir = readdir($handle)) { if (is_dir($dir) && !in_array($dir,$list_ignore)) { echo '<li><a href="'.$dir.'"> '.$dir.'</a></li>'; } } echo "</ul>Images:<ul>"; while($image = readdir($handle)) { $ext = strstr($image, '.'); if(in_array($ext,'.'.$ext_list)){ echo '<li><a href="'.$image.'"><img src="'.$image.'" width="25"> '.$image.'</a></li>\n'; } } } ?>
-
um, what kind of categories?
-
Try:
$array = array('.gif','.jpg','.jpeg','.png'); if($ext == $array){ ...
-
Easy, Someone play's it in w3 and takes a screenshot.
-
heh, keep trying.
You need server power here..
-
Try:
<?php $rnum = 10001; //remember that these are incremented, so start out 1 less... $lup = 20000; $ldown = 0; $lwest = 0; $least = 10001; for($rnum = 10001;$rnum<20001;$rnum++) { if($rnum >= 20001) { die("Completed, you may now exit to the room.php page"); } $lup++; $ldown++; $lwest++; $least++; if($rnum == "10101") { $lnorth = 10001; } elseif($rnum >= 10102) { $lnorth++; } elseif($rnum <= 19900) { $lsouth++; } else{ $lsouth = ""; } //since nobody seems to get this, it sets all of these rooms blank either on the left link or right link... and then on the page that displays this, I use an if statement to echo a link back if it's filled, and then just the words "move left" or "move right" if blanks... if($rnum == "10100" || $rnum == "10200" || $rnum == "10300" || $rnum == "10400" || $rnum == "10500" || $rnum == "10600" || $rnum == "10700" || $rnum == "10800" || $rnum == "10900" || $rnum == "101000" || $rnum == "11100" || $rnum == "11200" || $rnum == "11300" || $rnum == "11400" || $rnum == "11500" || $rnum == "11600" || $rnum == "11700" || $rnum == "11800" || $rnum == "11900" || $rnum == "12000" || $rnum == "12100" || $rnum == "12200" || $rnum == "12300" || $rnum == "12400" || $rnum == "12500" || $rnum == "12600" || $rnum == "12700" || $rnum == "12800" || $rnum == "12900" || $rnum == "13000" || $rnum == "13100" || $rnum == "13200" || $rnum == "13300" || $rnum == "13400" || $rnum == "13500" || $rnum == "13600" || $rnum == "13700" || $rnum == "13800" || $rnum == "13900" || $rnum == "14000" || $rnum == "14100" || $rnum == "14200" || $rnum == "14300" || $rnum == "14400" || $rnum == "14500" || $rnum == "14600" || $rnum == "14700" || $rnum == "14800" || $rnum == "14900" || $rnum == "15000" || $rnum == "15100" || $rnum == "15200" || $rnum == "15300" || $rnum == "15400" || $rnum == "15500" || $rnum == "15600" || $rnum == "15700" || $rnum == "15800" || $rnum == "15900" || $rnum == "16000" || $rnum == "16100" || $rnum == "16200" || $rnum == "16300" || $rnum == "16400" || $rnum == "16500" || $rnum == "16600" || $rnum == "16700" || $rnum == "16800" || $rnum == "16900" || $rnum == "17000" || $rnum == "17100" || $rnum == "17200" || $rnum == "17300" || $rnum == "17400" || $rnum == "17500" || $rnum == "17600" || $rnum == "17700" || $rnum == "17800" || $rnum == "17900" || $rnum == "18000" || $rnum == "18100" || $rnum == "18200" || $rnum == "18300" || $rnum == "18400" || $rnum == "18500" || $rnum == "18600" || $rnum == "18700" || $rnum == "18800" || $rnum == "18900" || $rnum == "19000" || $rnum == "19100" || $rnum == "19200" || $rnum == "19300" || $rnum == "19400" || $rnum == "19500" || $rnum == "19600" || $rnum == "19700" || $rnum == "19800" || $rnum == "19900" || $rnum == "20000") { $tempvar = $least; $least = ""; } if($rnum == "10001" || $rnum == "10101" || $rnum == "10201" || $rnum == "10301" || $rnum == "10401" || $rnum == "10501" || $rnum == "10601" || $rnum == "10701" || $rnum == "10801" || $rnum == "10901" || $rnum == "11001" || $rnum == "11101" || $rnum == "11201" || $rnum == "11301" || $rnum == "11401" || $rnum == "11501" || $rnum == "11601" || $rnum == "11701" || $rnum == "11801" || $rnum == "11901" || $rnum == "12001" || $rnum == "12101" || $rnum == "12201" || $rnum == "12301" || $rnum == "12401" || $rnum == "12501" || $rnum == "21601" || $rnum == "12701" || $rnum == "12801" || $rnum == "12901" || $rnum == "13001" || $rnum == "13101" || $rnum == "13201" || $rnum == "13301" || $rnum == "13401" || $rnum == "13501" || $rnum == "13601" || $rnum == "13701" || $rnum == "13801" || $rnum == "13901" || $rnum == "14001" || $rnum == "14101" || $rnum == "14201" || $rnum == "14301" || $rnum == "14401" || $rnum == "14501" || $rnum == "14601" || $rnum == "14701" || $rnum == "14801" || $rnum == "14901" || $rnum == "15001" || $rnum == "15101" || $rnum == "15201" || $rnum == "15301" || $rnum == "15401" || $rnum == "15501" || $rnum == "15601" || $rnum == "15701" || $rnum == "15801" || $rnum == "15901" || $rnum == "16001" || $rnum == "16101" || $rnum == "16201" || $rnum == "16301" || $rnum == "16401" || $rnum == "16501" || $rnum == "16601" || $rnum == "16701" || $rnum == "16801" || $rnum == "16901" || $rnum == "17001" || $rnum == "17101" || $rnum == "17201" || $rnum == "17301" || $rnum == "17401" || $rnum == "17501" || $rnum == "17601" || $rnum == "17701" || $rnum == "17801" || $rnum == "17901" || $rnum == "18001" || $rnum == "18101" || $rnum == "18201" || $rnum == "18301" || $rnum == "18401" || $rnum == "18501" || $rnum == "18601" || $rnum == "18701" || $rnum == "18801" || $rnum == "18901" || $rnum == "19001" || $rnum == "19101" || $rnum == "19201" || $rnum == "19301" || $rnum == "19401" || $rnum == "19501" || $rnum == "19601" || $rnum == "19701" || $rnum == "19801" || $rnum == "19901") { $tempvar2 = $lwest; $lwest = ""; } $permis = "0"; mysql_query("INSERT INTO `rooms` (rnum, lup, ldown, lwest, least, lnorth, lsouth, rcontents, rname, permis) VALUES ('$rnum','$lup','$ldown','$lwest','$least','$lnorth','$lsouth','$rcontents','$rname','permis');") or die(mysql_error()); if(!$least) { $least = $tempvar; } if(!$west) { $lwest = $tempvar2; } } ?>
-
Why not search all three tables for the user?
I think a join would do.
-
Someone could easily make a script to post to b.php.
Why not just check the referrer?
if ($_SERVER['HTTP_REFERER'] !== $_SERVER['HTTP_HOST']){ echo 'Please do not use proxies.'; }
I would also suggest that you encrypt sessions, because they could upload a php script to view/edit sessions(I had that happen to me before), but that's if you want strict security(and also get stronger/anonymous passwords).
-
What do you mean by speed?
Try using less variables.
<?php $connection = mysql_connect("localhost", "1", "1"); mysql_select_db("1", $connection); $result = mysql_query("SELECT isbn, description, title, author FROM books"); // WHERE catid <> 'TBP' while($myrow = mysql_fetch_array($result)) { echo '<a href="show_book.php?isbn=' . $myrow['isbn'] . '">' . $myrow['isbn'].' '.$myrow['description'].' '.$myrow['title'].' '.$myrow['author'] . '</a><br /> } ?>
Maybe that will make it about a few micro seconds faster.
This is a little script so really..
-
$size = filesize('archive.zip');
Result in bytes.
-
heh, Sorry didn't notice the variables(might seem weird because there is a mysql query).
-
afr short for Afrikaans.
lmga directly translated: laugh my ass off(laugh out loud).
Will fix the wikipedia link.
rawky1976 try this:
<?php $user = $_GET['userid']; include 'config.php'; include 'opendb.php'; $query = "select * from users WHERE user_id LIKE '$user'"; $result = mysql_db_query("servicemgmt", $query . mysql_error()); $r = mysql_fetch_array($result); $firstname = $r['user_fname']; $lastname = $r['user_lname']; $ext = $r['user_ext']; $bleep = $r['user_bleep']; $exttel = $r['user_extno']; $email = $r['user_email']; echo '<table style="width: 80%">'; echo '<tr><td>First Name</td><td><input type="text" value="$firstname" name="firstname" id="firstname" style="width: 160px "/></td><td>Last Name</td><td> <input type="text" value="$lastname" name="lastname" id="lastname" style="width: 160px" /></td></tr>'; echo '<tr><td> </td><td> </td><td> </td><td> </td></tr>'; echo '<tr><td>Extension</td><td> <input type="text" value="$ext" name="ext" id="ext" style="width: 160px" /></td><td>Bleep No.</td><td> <input type="text" value="$bleep" name="bleep" id="bleep" style="width: 160px"/></td></tr>'; echo '<tr><td> </td><td> </td><td> </td><td> </td></tr>'; echo '<tr><td>External Tel.</td><td><input type="text" value="$exttel" name="exttel" id="exttel" style="width: 160px"/></td><td> </td><td> </td></tr>'; echo '<tr><td> </td><td> </td><td> </td><td> </td></tr>'; echo '<tr><td>Email</td><td><input type="text" value="$email" name="email" id="email" style="width: 200px" /></td><td> </td><td> </td></tr>'; echo '<tr><td> </td><td> </td><td> </td><td> </td></tr>'; echo '<tr><td><input name="updateuser" type="submit" id="updateuser" value="Update User Details" /></td><td> </td><td> </td><td><input name="reset1" type="reset" value="Clear Form" /></td></tr>'; echo '</table>'; ?>
-
@akitchin - lmga
i have no idea what lmga means. all i could find was "Location Managers Guild of America" and "Louisiana Meat Goat Association."
afr for lol.
-
@akitchin - lmga
Try echo statements with single quotes.
It should give an php error actually.
-
see... php 5.1.2.0 was compiled WITHOUT one important C header file that would make it possible for php to be used with ajax asa per request platform
Either you recompile php with 5.1.2 and the cuproq.c and cuproq.h files,
or you find another wya of doing it, without ajax or cgi...
What?
<?php echo '<script language="Javascript" type="text/javascript"> Ajax here.. </script>'; ?>
Is there a simpler way to this (below-mysql query) ?
in PHP Coding Help
Posted
For what you wanted to do, it's more efficiant to use a while loop.