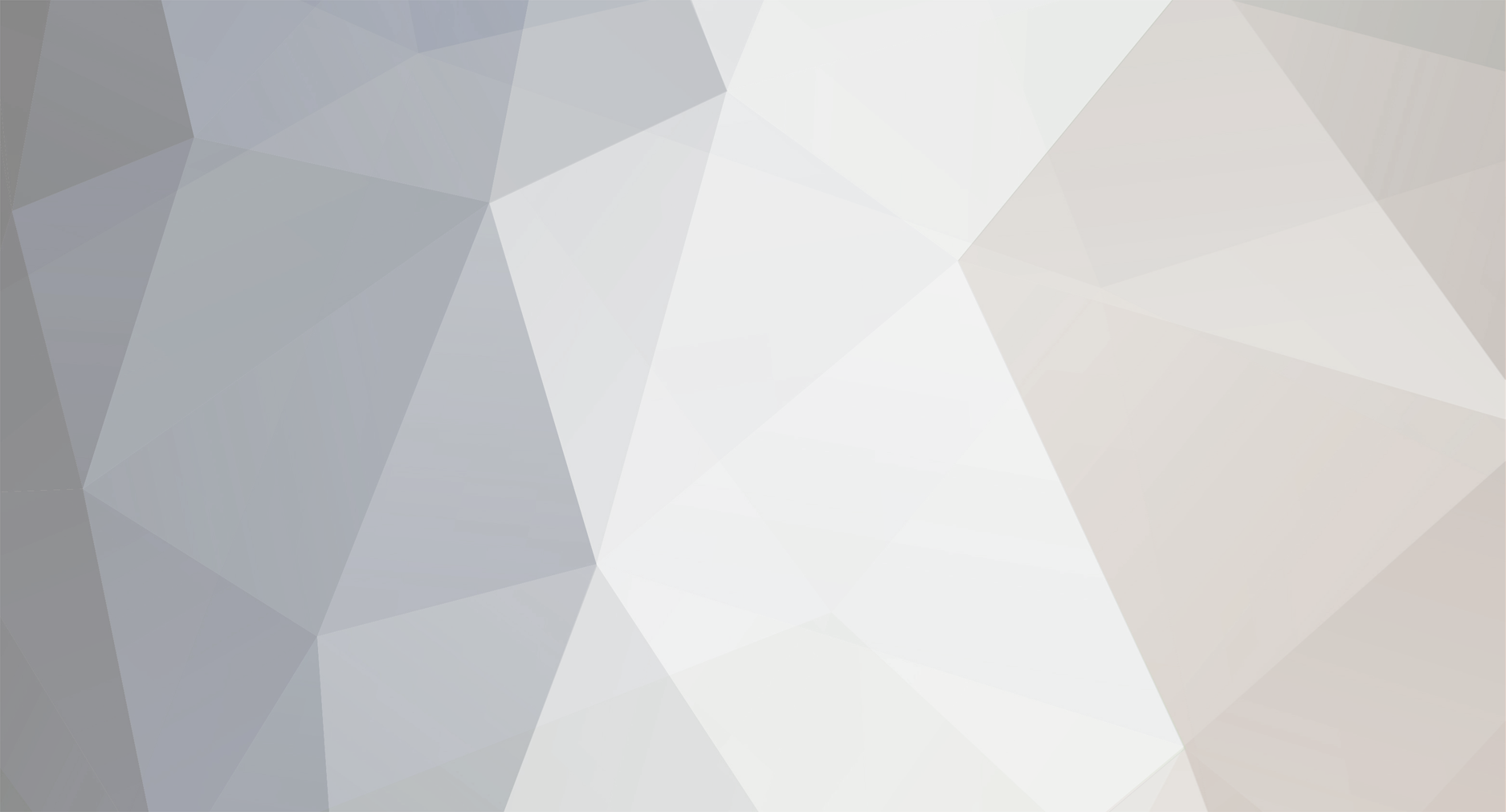
maxudaskin
-
Posts
628 -
Joined
-
Last visited
Posts posted by maxudaskin
-
-
Second, my variables $val and $q_cat aren't getting inserted into the query.
$q_cat = ""; if ($key == "1") { $q_cat = "4";} elseif ($key == "2") { $q_cat = "1";} elseif ($key == "3") { $q_cat = "11";} elseif ($key == "4") { $q_cat = "2";} elseif ($key == "5") { $q_cat = "10";} elseif ($key == "6") { $q_cat = "9";}
First off, you provide no default for $q_cat. I assume that the issue lies with $key.
You can also make the logic sexier by using a switch statement.
switch($key) { case 1: $q_cat = 4; break; // Without this statement, the page would continue checking the other cases, albeit, they would all get evaluated as false case 2: $q_cat = 1; break; case 3: $q_cat = 11; break; case 4: $q_cat = 2; break; case 5: $q_cat = 10; break; case 6: $q_cat = 9; break; default: // If the key is not 1-6, have a fallout. You can log the error, or do whatever you want. $q_cat = -1; }
You do not need to enclose the number in quotes. PHP automatically converts variable types. Keep it clean.
As far as $val, if $key is messed up, it is as likely that $var will be too.
Enter this before the foreach and copy the results so we can see.
print_r($results_done);
-
I know that this will be a stretch, as it would likely require a major rewrite of your script, but I'd suggest having two tables with a many to many relationship.
Your example seemed very... flat... so, here's a more in-depth example.
An company/employee database.
Table: Company
Columns: id, name, address, ect.
Description: The list of companies
Table Employee
Columns: id, name, sin, ect.
Description: The list of employees
Table: Company_has_Employee
Columns: id, Company_id, Employee_id
Description: The link between companies and employees
The reasoning behind this is that each company has many employees. Just because an employee works at one company, does not mean that they do not work at another. Having the third table, you have one row per relationship.
Company
-------
1 | Company Inc. | 123 Anywhere
2 | Incorp Ltd. | 456 Somewhere
Employee
--------
1 | Jon | 123456
2 | Max | 654321
3 | Laura | 532673
4 | Hank | 639256
Company_has_Employee
------------------
1 | 1 | 1 // Company Inc has Jon
2 | 1 | 2 // Company Inc has Max
3 | 2 | 3 // Incorp Ltd has Laura
4 | 1 | 4 // Company Inc has Hank
4 | 2 | 4 // Incorp Ltd has Hank
You can see that Jon and Max are employees of Company Inc, Laura is an employee of Incorp Ltd, and Hank is an employee of both.
-
-
I have little idea what you are trying to accomplish, but reloading a page multiple times to add selections to an array seems very ineffective. Try Javascript.
-
Why are you using a function to echo a thank you button?
<div style="text-align:center;"> <input type="button" value="Thank You!" /> </div>
-
-
<?php echo 'starting regex test<BR>'; $data = "c799 1 000ffe-fcc811 1 000ffe-fcc86f 1 000ffe-fcc898 1 000ffe-fcc92d 1 000ffe-fcc934 1 000ffe-fcc965 1 000ffe-fcc96b"; $pattern="/([a-f0-9]){6}-([a-f0-9]){6}/"; echo 'Looking for ' . $pattern . ' in ' . $data; if (preg_match_all($pattern,$data,$matches, PREG_SET_ORDER)) { echo '<pre>'; print_r($matches); echo '</pre>'; } else { echo "No, the mac address is wrong"; }
I have removed the $ and the i at the end, as well as removing the extra a-f in the second match.
If you run it, you will start to see what PHP is doing.
Array ( [0] => Array ( [0] => 000ffe-fcc811 [1] => e [2] => 1 ) [1] => Array ( [0] => 000ffe-fcc86f [1] => e [2] => f ) [2] => Array ( [0] => 000ffe-fcc898 [1] => e [2] => 8 ) [3] => Array ( [0] => 000ffe-fcc92d [1] => e [2] => d ) [4] => Array ( [0] => 000ffe-fcc934 [1] => e [2] => 4 ) [5] => Array ( [0] => 000ffe-fcc965 [1] => e [2] => 5 ) [6] => Array ( [0] => 000ffe-fcc96b [1] => e [2] => b ) )
$matches is an array of arrays. Each sub-array has three values, the entire matched string, the last matched character in the first bracket, and the last matched character in the last bracket. If you were to remove the brackets, it would only return the matched string.
<?php echo 'starting regex test<BR>'; $data = "c799 1 000ffe-fcc811 1 000ffe-fcc86f 1 000ffe-fcc898 1 000ffe-fcc92d 1 000ffe-fcc934 1 000ffe-fcc965 1 000ffe-fcc96b"; $pattern="/[a-f0-9]{6}-[a-f0-9]{6}/"; echo 'Looking for ' . $pattern . ' in ' . $data; if (preg_match_all($pattern,$data,$matches, PREG_SET_ORDER)) { echo '<pre>'; print_r($matches); echo '</pre>'; } else { echo "No, the mac address is wrong"; }
Array ( [0] => Array ( [0] => 000ffe-fcc811 ) [1] => Array ( [0] => 000ffe-fcc86f ) [2] => Array ( [0] => 000ffe-fcc898 ) [3] => Array ( [0] => 000ffe-fcc92d ) [4] => Array ( [0] => 000ffe-fcc934 ) [5] => Array ( [0] => 000ffe-fcc965 ) [6] => Array ( [0] => 000ffe-fcc96b ) )
-
Is there any reason why if you're looking for xxxxxx-xxxxxx, with x being 0-9 or a-f, why you would do '/([a-f0-9]){6}-([a-fa-f0-9]){6}$/i'?
Specifically, ([a-fa-f0-9]).
Don't you want /([a-f0-9]){6}-([a-f0-9]){6}$/i?
-
I'm in a rush to go, but I'll try to point you in the right direction. I haven't read your code yet.
You need a database for the users, groups, posts, and user_sees_posts, user_has_groups. Something like that.
For every user, there can be multiple groups. For every group, there can be many users. Many to many.
For every group, there's many posts. One to many.
For every post, there are many users that can see it. For every user, there are many posts visible. Many to many.
User:
id, name, email, password (ect.)
groups:
id, name, creator (ect.)
user_has_groups:
id, user_id, groups_id
The id in this table will be used for updating or deleting rows.
user_sees_posts:
id, user_id, posts_id
If user with id 1 is part of group 5 and can see posts 32 and 45, both associated with group 5, you would have:
user:
id=1, name=blahblahblah
groups:
id=5, name...
user_has_groups:
id=1, user_id=1, groups_id=5
user_sees_posts
id=1, user_id=1, posts_id=32
id=2, user_id=1, posts_id=45
-
One to One Relationship:
Essentially, one table that is split up. Each row is associated to exactly one row of the other table.
One to Many Relationship:
Table 1: Employees
Description: A list of all employees registered with the website.
Relation to Table 2: Many employees to one company
Table 2: Companies
Description: A list of all of the companies
Relation to Table 1: One company to many employees
id | name | companies_id
------------------------------------
1 | Max | 1
2 | John | 1
3 | Sarah | 2
4 | David | 1
id | name
---------------------
1 | Company Inc.
2 | Incorp Ltd.
Therefore, Max, John, and David, all work for Company Inc.
Sarah works for Incorp. Ltd.
Many to Many Relationship:
Table 1: Employee
Description: A list of all employees registered with the website.
Relation to Table 2: Many employees to many companies
Table 2: Companies
Description: A list of all of the companies
Relation to Table 1: Many companies to many employees
Table 3: Employee_has_Companies
Description: Every row is a one to one relationship between an employee and a company.
Employee
id | name
---------------------
1 | Max
2 | John
3 | Sarah
4 | David
Companies
id | name
---------------------
1 | Company Inc.
2 | Incorp Ltd.
Employee_has_Companies
Employee_id | Comanpies_id
--------------------------
1 | 1
2 | 1
3 | 2
4 | 1
4 | 2
Same as before, except that David works for both Company Inc. and Incorp Ltd.
-
Post the relevant code that you have, and some data about the relevant database tables.
Table Relationships. It's an advanced concept, but you'll benefit learning it early.
-
Have you heard of AJAX?
Basically, it's Javascript that calls a php file, and uses the output.
You would have the javascript call the ajax function when text is typed into the textbar, and when @ is found in the text.
Isolate the text directly adjacent to the @, send it to the function. PHP would then search the database for similar results, and return them. Javascript would update the screen with the suggestions.
-
You'll want to use a many to many relationship with a database.
That aside, we cannot tell you anything else without more information.
-
-
Math works the same way here as it does on paper...technically in this case you don't even need to wrap anything in parens (though I usually do for better readability)...order of operations will have the division happen first...
-
I want to return a result based on a session status and want to know how many minutes '10000' would be.
if($_SESSION['first'] < (time() - 10000))
Many thanks.
166 minutes, 40 seconds.
I would do a quick google search before posting next time.
-
Even if you could, that would present major security issues.
-
An IP does not necessarily mean a computer. There can be many computers to an IP. What if the user is using a public computer (many responses, few IPs).
Register the survey with an email. This allows you a reply-to address, as well as a way to weed out multiple survey submits. What ever system you use, unless you require a fingerprint scan, there is always the risk of multiple submissions.
-
You literally have to tell PHP what you want to remove. Are you removing " " or ? One is an HTML entity, the other is a literal character.
Check out html_entity_decode()
You could also use preg_replace.
<?php $str = 'html text with entities.'; $find = '/\&.{2,6};/'; $replace = ''; echo preg_replace($find, $replace, $str);
That should remove any entity.
-
Try making a function to insert the data into the database.
function insertData($gender, $data) {
$sql = 'UPDATE table_name SET sizes=\'' . $data['sizes'] . '\', colors=\'' . $data['colors'] . '\' WHERE gender=\'' . $gender . '\'';
mysql_query($sql);
}
$data = Array(
'mens' => Array(
'colors' => 'red, green, blue',
'sizes' => '1,3,5,6,7,8,9')),
'womens' => Array(
'colors' => 'red, green, blue',
'sizes' => '1,3,5,6,7,8,9')),
);
You can have the colours and sizes in a subarray. Use implode to put them in the database, comma seperated. Use explode to take them from the database and put them into a sub array.
-
-
With
preg_replace ()
you can use a Regular Expression to capture and replace all of the template variables in one call, skipping the loop entirely. Something which you can do with "str_replace ()" as well, coincidentally, seeing as it supports arrays as the first two arguments. Latter one just requires a bit more work, to get the square brackets added to the keys.In any case, example of a
preg_replace ()
to insert the template data:return preg_replace ('/\[([\w\d_-]+)\]/e', '$this->values[$1]', $this->output);
Whether this or the existing method is better, is something you'll have to decide.
IIRC, PCRE regex allows you to replace values of any variable in your template. Let's say you use {{var_name}} to signify variables, you can be almost certain that it won't come up randomly in a sentence. You can replace anything that has not been defined with [var_name not defined], or whatnot.
-
<?php $currency = '$'; $string = 'This item costs $29.97. You may pay cash or credit.'; $find = '/\\' . $currency . '[0-9]*[.]?[0-9]{0,2}/'; preg_match($find, $string, $matches); echo 'Searching for "' . $find . '" in "' . $string . '" <br />'; print_r($matches);
-
Hey,
I would like to be able to select a variable using another variable. I have tried the code displayed but it does not work. Any ideas?
$gender = "mens"; $test_mens_test = "test"; echo $test_{$gender}_test;
Thanks
ChristianF has already mentioned the stupidity of using variable name variables, but if you're going to do it, you might as well do it right.
You have set the name to $test_{$gender}_test;
Try this:
$var_name = 'test_' . $gender . '_test';
echo $$var_name;
php, ajax help
in Javascript Help
Posted
Correct me if I'm wrong, but what I think you're trying to do is have a loading image while AJAX is fetching the next page.
If you post your AJAX javascript code, I can show you exactly what to do, but in the mean time, in the function that fetches the PHP page data, change the element innerHTML to <img src="path/to/loading_image.gif" height="100 width="100" alt="Loading..." />.