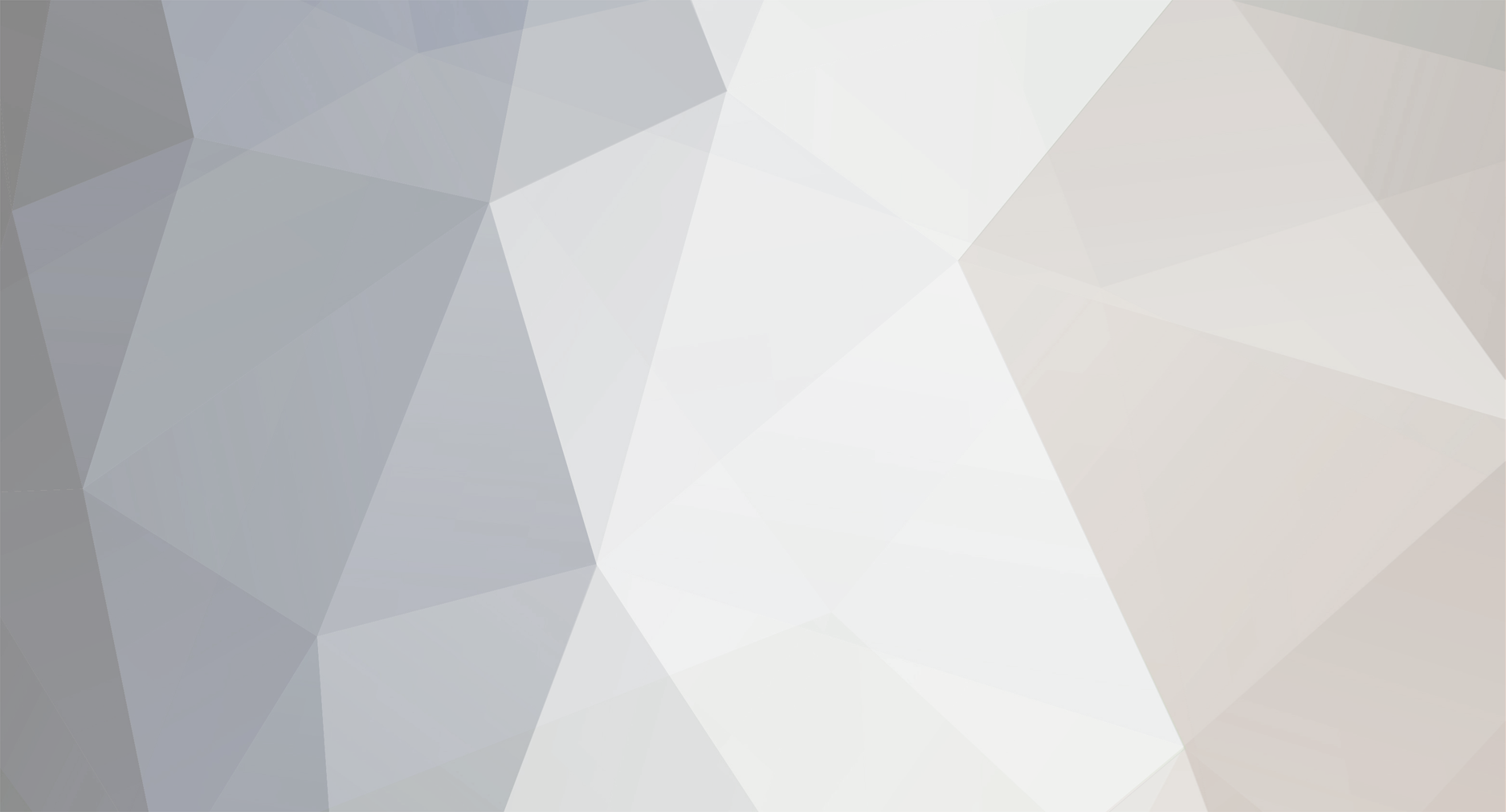
hcdarkmage
Members-
Posts
326 -
Joined
-
Last visited
Everything posted by hcdarkmage
-
I think I actually see where the problem is that you are running into. In step 4 of the tutorial it shows code for if they are registered or not and then starts the session: if (isset($_POST['register'])) { // they want to register $username = trim(strip_tags(stripslashes($_POST['username']))); if (ini_get('magic_quotes_gpc')) { $username = stripslashes($username); } } else { //they don't want to register $random = rand(1,1000); $username = 'Anon'. $random; } //end of if/else conditional</strong> $num = 0; If you notice, there is a $num = 0; just after that section. Check to see if that is in your code. That makes a big difference.
-
I think I may see the problem. Or at least A problem: foreach ($leaders as $key => $percent) { //---changed $score to percent // Check that $counter is less than $limit. if ($counter <= $limit) { if ($key == $_SESSION['user']) { $output .= "<li><strong>$key:</strong> $percent%</li>\n"; //--changed $value to $percent } else { $output .= "<li>$key: $percent%</li>\n"; //--- again $value to $percent } if ($group) { // Use the modulus operator(%) to create new sub-list. if($counter % $group == 0) { $output .= "</ul>\n<ul class=\"leaders\">\n"; } } } $counter++; } You should have left most of this alone. It should read something like: foreach ($leaders as $key => $value) { if ($counter <= $limit) { if ($key == $_SESSION['user']) { $output .= "<li><strong>$key:</strong> $value \%</li>\n"; } else { $output .= "<li>$key: $value \%</li>\n"; } if ($group) { // Use the modulus operator(%) to create new sub-list. if($counter % $group == 0) { $output .= "</ul>\n<ul class=\"leaders\">\n"; } } } $counter++; } Because it is calling forth an array: $leaders = array(); You have already changed the score variable to percent. foreach($xml->user as $user) { $name = (string)$user->name; $percent = (string)$user->score; //---Changed $score to percent $leaders[$name] = $percent; //---again $score to percent } But, the big question here is: Where are you getting this variable $percent = (string)$user->score; //<--- this $user->score here We find where that is being set and we can find what is going on with your percentages. Find the code that is setting that variable.
-
I think you finally lost me. Just looking at the code you provided doesn't tell me anything about what you changed. Maybe if you put comments in on what you changed I could see the problem easier. Maybe that or my weekend has taken a bigger toll on me then I thought. Put comments in where you made the changes and try and show the original underneath and we will see where the problem is.
-
Actually, that comment was my fault. It had nothing to do with what was originally posted, it was me mistaking my words. As mjdamato pointed out, I was looking at the problem the wrong way and got myself confused. Ignore the comments that where put in the code. Oh, and thanks for calling me an idiot! It helps remind me that I'm human, lol.
-
I think your problem is in the answers array. <?php $questions = array('FTP','AJAX','RSS','XSS','PHP','W3C','XML','YUI','HTML','CGI','SSL','SQL','HTTP','CSS','SOAP','WAI','SSI','JSON','XSLT','WCAG'); $answers = array( array(0 =>'File Transfer Protocol','Force Through Privately','File Through Protocol','File Test Protocol'), // <--- This belongs to array "0" array(0 => 'Asynchronous JavaScript and XML','All JavaScript and XML','Alternative Java and XML','Actual JavaScript and XML'), // <--- This belongs to array "1" array(0 => 'Really Simple Syndication','Really Simple Scripting','Ready-Styled Scripting','Really Stupid Syndication'), // <--- This belongs to array "2", etc. ?> Change the zeros to correspond with the question array number. Try that, see if it helps.
-
my guess would be these lines here: if($country[0]=="brazil"){ $brazil="CHECKED";} if($country[1]=="paraguay"){ $paraguay="CHECKED";} if($country[2]=="germany"){ $germany="CHECKED";} if($country[3]=="argentina"){ $brazil="CHECKED";} // <--- Here if($country[4]=="japan"){ $brazil="CHECKED";} // <--- Here you have $brazil being checked 3 times, so it only reads 3 checks, not 4 or 5.
-
Found a neat little JS function that helps with onloads: <script type="javascript"> function addLoadEvent(func) { var oldonload = widow.onload; if (typeof window.onload != 'function'){ window.onload = func; }else{ window.onload = function(){ if (oldonload) { oldonload(); } func(); } } } </script> This code is put below the functions you want to call onload, then you do something like: if (($pageaction =="edit || $pageaction=="update") && $field_id != ""){ echo "addLoadEvent(toggledisplaymode(\"$fieldtype\")); } This pretty much makes it so that when you edit the information, it at least shows the DIVs that where hidden (see code from previous posts to see what I'm talking about). Now if I can just figure out how to put the variables that need to be edited in the proper place And if you are wondering I found the explanation for this here: http://www.webreference.com/programming/javascript/onloads/
-
Or you can do it this way: <?php $sleeves = array( "15"=>"Long Sleeve", "20"=>"Short Sleeve", ); if (!isset($_POST['submit'])) { ?> <form method="post" action="<?php echo $_SERVER['PHP_SELF'];?>"> <select name="shirt" id="shirt"> <option value="" selected="selected">--- Select Sleeve Type ---</option> <?php foreach($sleeves as $key => $value){ echo '<option value="'.$key.'">'.$value.'</option>'; } ?> </select> <input type="submit" name="submit"> </form> <?php } else { $shType = $_POST["shirt"]; echo "This should work ".$sleeves[$shType]; } ?>
-
I believe that the only way that can be done is if your drop down was populated by a database or an array, or if your value is the same as your text. If you just typed the values and options in there yourself, then it will only read the value portion of the drop down box and you lose the other information. <select id="dropdwn1" name="dropdwn1" size ="1"> <option value="0" selected="selected">Choose</option> <option value="value1">value1</option> <option value="value2">value2</option> </select> or $states = array( "AL"=>"Alabama", "AK"=>"Alaska", "AZ"=>"Arizona" ) <select name="state" id="state"> <option value="" selected="selected">--- Select State ---</option> <? foreach($states as $key => $value){ echo '<option value="'.$key.'">'.$value.'</option>'; } ?> </select> then you can get the information you wanted.
-
Okay, let's try to word this differently, mostly because I am at a loss. the original script (onChange script) toggles a couple of DIVs to display so that extra information can be put in. This onChange event is in a drop down list. Now, when you go to EDIT that particular page, you can't see the extra options because there is no onChange event going on. My question is what should I do to call that function so that the extra information is shown? I have tried many things, but none of them worked and I am stumped. I even tried something like this: if (($pageaction=="edit" || $pageaction=="update") && $field_id !=""){ for($i=0; $i<count($options); $i++){ echo "<script type=\"javascript\">addOption(\"$options[$i]\")</script>"; } echo "<script type=\"javascript\">toggledisplaymode(\"$fieldtype\")</script>"; } right after the drop down list, since that is where the onChange takes effect, but it didn't work. Any other ideas?
-
Post your changed code. It may not be resetting the percent values, so it might just be running a total percentage based on how many times you do the quiz.
-
I think if you change these areas to your percent variable: $uscore = $user->addChild('score',$_SESSION['score']); // <- Here echo "<h2 id=\"score\">{$_SESSION['user']}, your final score is:</h2>\n <h3>{$_SESSION['score']}/20</h3><h4>Verdict:</h4>"; // <- Here And the same in your xml: <?xml version="1.0" encoding="UTF-8"?> <users> <user> <name>Bobby</name> <score>10</score> // <- Here </user> <user> <name>Billy</name> <score>1</score> // <- Here </user> </users> And in the leaders board: function showLeaders($file,$limit,$group = null) { $leaders = array(); // Load the xml file and place all users and associated // scores into the 'leaders' array. $xml = simplexml_load_file($file); foreach($xml->user as $user) { $name = (string)$user->name; $score = (string)$user->score; // <- Here $leaders[$name] = $score; // <- Here } // Sort the leaders array numerically, highest scorers first. arsort($leaders,SORT_NUMERIC); // Initialise our $counter variable to '1'. $counter = 1; // Start a html ordered list to hold the leaders. $output = "<ul class=\"leaders\">\n"; // Loop through the 'leaders' array and wrap each username and score // in <li> tags. If the user is the current $_SESSION['user'], wrap // the name/score in <strong> tags too. foreach ($leaders as $key => $value) { // Check that $counter is less than $limit. if ($counter <= $limit) { if ($key == $_SESSION['user']) { $output .= "<li><strong>$key:</strong> $value/20</li>\n"; // <- Here } else { $output .= "<li>$key: $value/20</li>\n"; // <- Here } Try changing those to your percentage value. i think that will take care of your problem. Oh, and take out the /20 that is there.
-
Being safe, and not wanting to offend, nowhere in my post did I say the editing was done in a text field. Here is the Edit script: echo "<td class=\"gridRow\" align=center> <a href=\"index.php?component=contactform&page=wce.field.php&pageaction=edit&field_id=$myrow[0]&sortby=$sortby&sortseq=$sortseq&start=$start\"><img src=common/image/ico_edit.gif border=0 title=\"".$lang['contactform']['editthisrecord']."\"></a> "; It basically restarts the page and pre-fills in the form areas, but it won't show the hidden areas because the selection in the drop down box is already made. Since that uses an onChange to show those hidden areas, they will not be shown when in edit mode. I had put the onBlur in the only text field there was and it didn't work there. Any other suggestions?
-
First . . . your math function is a little off. 4 out of 20 is only 20% not 40%. You may try redoing your math function to something like this: $scorePer = $right / $total; $percent = $scorePer * 100; For some reason your math function is working funny. I don't see how it got 40 percent. As for doing it percent wise, try something like this: if($_SESSION['percent'] <= 25) echo "<p id=\"verdict\"><span>S</span>everely <span>H</span>indered <span>I</span>n the <span>T</span>est!</p>\n"; if(($_SESSION['percent'] > 5) && ($_SESSION['percent'] <= 50)) echo "<p id=\"verdict\"><span>C</span>ould <span>R</span>ead <span>A</span>nd <span>P</span>ractice more.</p>\n"; if(($_SESSION['percent'] > 50) && ($_SESSION['percent'] <= 75)) echo "<p id=\"verdict\"><span>A</span>cronyms a<span>R</span>e <span>S</span>o <span>E</span>asy!</p>\n"; if($_SESSION['percent'] > 75) echo "<p id=\"verdict\"><span>S</span>uper <span>A</span>cronym <span>S</span>pecialist</p>"; echo "<p id=\"compare\"><a href=\"results.php\">See how you compare! <img src=\"images/arrow.png\" /></a></p>"; } Basically, change $score to $percent and see if that works. Hope that helps.
-
Ok. I put the onBlur in a couple of areas, hoping to get the right place. First place I put it was on the link that sends it into edit mode . . . no results. There was no value set at that time for it. Second place was in the if ($pageaction=="edit" && $field_id != "") section, because it should define the $fieldtype variable for the JS function to use . . . still no results. Am I just putting it in the wrong place, or is my JavaScript-Fu that bad?
-
That is a good question. My bets would be somewhere AFTER the score is tallied. Other then that, without seeing the whole thing, I wouldn't know where you'd put it.
-
the simple answer would be to take the number they got right and divide it by the total number of questions. $rightAnswer = $session['correct']; // Or whatever the code is for this. $totalQuestions = $numberOfQuestions; // Same here. $perc = $rightAnswer/$totalQuestions; echo "You got ".$perc."% of the questions correct.";
-
Alright, so I got my onChange script to do what it is supposed in the dropdown, but how can I get it to work when they need to EDIT the options? <tr> <td valign=top width=28%><? echo $lang['contactform']['fieldtype'] ?></td> <td> <select name="fieldtype" id="fieldtype" onChange="toggledisplaymode(this.options[this.selectedIndex].value)"> <option value="textbox" <? echo $fieldtype=="textbox"?"selected":"" ?>><? echo $lang['contactform']['textbox'] ?></option> <option value="drop" <? echo $fieldtype=="drop"?"selected":"" ?>><? echo $lang['contactform']['dropdownselection'] ?></option> <option value="checkbox" <? echo $fieldtype=="checkbox"?"selected":"" ?>><? echo $lang['contactform']['checkbox'] ?></option> <option value="radio" <? echo $fieldtype=="radio"?"selected":"" ?>><? echo $lang['contactform']['radiobutton'] ?></option> <option value="textarea" <? echo $fieldtype=="textarea"?"selected":"" ?>><? echo $lang['contactform']['textarea'] ?></option> </select> </td> </tr> <tr> <td valign=top><? echo $lang['contactform']['fieldwidth'] ?></td> <td><input type=textbox name=fieldwidth style="width:60px" value="<? echo $fieldwidth?$fieldwidth:"200" ?>"> px</td> </tr> <tr id="tbdataHeight" style="display:none"> <td valign=top><? echo $lang['contactform']['fieldheight'] ?></td> <td><input type=textbox name=fieldheight style="width:60px" value="<? echo $fieldheight?$fieldheight:"50" ?>"> px</td> </tr> <tr id=tbrowOption style="display:none"> <td valign=top><? echo $lang['contactform']['fieldoptions'] ?></td> <td> <table border=0 cellpadding=0 cellspacing=0 width=98%> <tr> <td><input name=optionvalue style="width:160px"> <input type=button value="<? echo $lang['contactform']['add'] ?>" onclick="addOption(document.thisform.optionvalue.value); document.thisform.optionvalue.focus();"> <input type=button value="<? echo $lang['contactform']['del'] ?>" onclick="deleteOption()"></td> </tr> <tr> <td><select size=5 name=options style="width:230px" onchange="populateItem(this.options[selectedIndex].index)"></select></td> </tr> </table> </td> </tr> So when they make the selection to edit, it will automatically select the field, so the onChange event is basically canceled. I STILL need to show the hidden fields (as it is) so they can edit their contact, but I can't use the onChange. Any ideas on how I can call the function when they do an edit?e Oh, the function code is: function toggledisplaymode(selectType){ var tbrowOption = document.getElementById('tbrowOption'); var tbdataHeight = document.getElementById('tbdataHeight'); if (selectType=="drop" || selectType=="checkbox" || selectType=="radio"){ tbrowOption.style.display = "table-row"; tbdataHeight.style.display = "none"; }else if(selectType=="textarea"){ tbrowOption.style.display = "none"; tbdataHeight.style.display = "table-row"; }else{ tbrowOption.style.display = "none"; tbdataHeight.style.display = "none"; } }
-
When working properly it hides 2 options that won't be needed when you select a drop down box, radio buttons, or check boxes,. but shows options for what text could be put with those options. Just tested the code, and it works to a degree. At least the hidden field is shown. Now I need to get it to hide the others. EDIT Actually, apparently that did the trick. Thank you very much.
-
*bump*
-
Ok, it has been a while, but I am having a problem with some JavaScript. I can't locate the head and body tags, because the script is all template based. I have a function that is supposed to show some extra options when a selection is made from the drop down list, but it isn't working properly. Hopefully we can come to a solution together. This is the code that has the drop down and the function call: <tr><td valign=top width=28%><? echo $lang['contactform']['fieldtext'] ?></td> <td colspan=3><input type=text name=fieldtext id=fieldtext style="width:265px" value="<? echo $fieldtext ?>"> *</td></tr> <tr><td valign=top width=28%><? echo $lang['contactform']['fieldtype'] ?></td> <td colspan=3> <select name="fieldtype" id="fieldtype" onChange="toggledisplaymode(this.options[this.selectedIndex].value)"> <option value="textbox" <? echo $fieldtype=="textbox"?"selected":"" ?>><? echo $lang['contactform']['textbox'] ?></option> <option value="drop" <? echo $fieldtype=="drop"?"selected":"" ?>><? echo $lang['contactform']['dropdownselection'] ?></option> <option value="checkbox" <? echo $fieldtype=="checkbox"?"selected":"" ?>><? echo $lang['contactform']['checkbox'] ?></option> <option value="radio" <? echo $fieldtype=="radio"?"selected":"" ?>><? echo $lang['contactform']['radiobutton'] ?></option> <option value="textarea" <? echo $fieldtype=="textarea"?"selected":"" ?>><? echo $lang['contactform']['textarea'] ?></option> </select></td></tr> <tr><td valign=top><? echo $lang['contactform']['fieldwidth'] ?></td><td><input type=textbox name=fieldwidth style="width:60px" value="<? echo $fieldwidth?$fieldwidth:"200" ?>"> px</td> <td valign=top id=tbdataHeight1 style="display:none"><? echo $lang['contactform']['fieldheight'] ?></td><td id=tbdataHeight2 style="display:none"><input type=textbox name=fieldheight style="width:60px" value="<? echo $fieldheight?$fieldheight:"50" ?>"> px</td></tr> <tr><td colspan=3> <table id=tbrowOption style="display:none" border=0 cellpadding=0 cellspacing=0 width=98%> <tr><td > blah blah text </td></tr> </table> </td></tr> And this is the function code: <script language=javascript> function toggledisplaymode(selectType){ if (selectType=="drop"){ tbrowOption.style.display = "block"; tbdataHeight1.style.display = "none"; tbdataHeight2.style.display = "none"; }else if(selectType=="textarea"){ tbrowOption.style.display = "none"; tbdataHeight1.style.display = "block"; tbdataHeight2.style.display = "block"; }else{ tbrowOption.style.display = "none"; tbdataHeight1.style.display = "none"; tbdataHeight2.style.display = "none"; } } </script> There are other options for the if, but for now I am just trying to get it to work with the one. And I am sorry for the messy code, I didn't write it, just trying to fix it. Any help would be appreciated.
-
Submitting form data to paypal and saving it to a DB???
hcdarkmage replied to craigtolputt's topic in PHP Coding Help
When I was creating APIs for working with PayPal, I never had to pay any fees. I have never had that problem. I know that a fee is taken when you GET money through PayPal, like a paycheck, but I never had to pay a fee to use the API. -
Submitting form data to paypal and saving it to a DB???
hcdarkmage replied to craigtolputt's topic in PHP Coding Help
You can get the API information from https://www.x.com/docs/DOC-1374. These should help you create the Paypal API for submitting the information you need. They used to have documentation to go with it, but I don't remember where to find it. -
You can create a timer script that would log someone out after so much time of inactivity (requires Javascript) or you can create a script that will do a session_destroy() if they navigate away from the site.
-
put an id tag in the text areas and then you could use a little javascript to hide and show the fields.