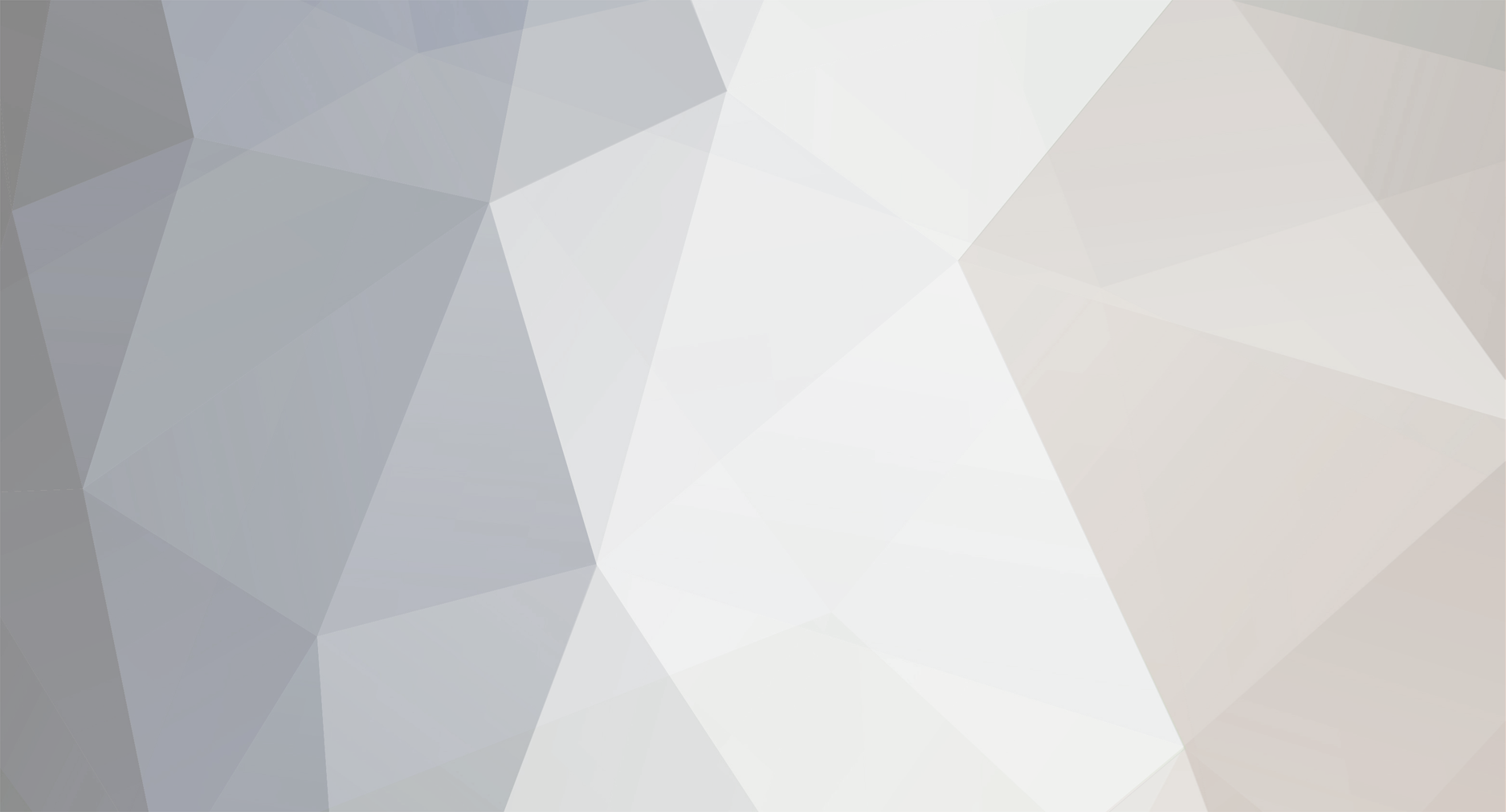
ChadNomad
Members-
Posts
46 -
Joined
-
Last visited
Never
Everything posted by ChadNomad
-
I though this was the case. As its my own code I probably don't realise the inconsistencies I'm making for everyone. Thanks. I think its reaffirmed my suspicion that its all too unnecessary. Thanks guys
-
Hi all, I am nitpicking here but I have wondered what the negatives are of assigning local variables to existing class variables are. To make more sense here is an example: <?php class myClass { private $_something; public $config = array( 'setting' => 123, ); public function __construct(array $config) { $this->config = $config; } public function go() { /* * Is this considered bad? I know an extra variable is being made * but I find it tedious constantly using $this, static, self etc */ $something = $this->_something; if($something) ... /* * Another thing I've found myself doing is typecasting arrays * to objects to save time typing. Is this bad? */ $config = (object) $config; if($config->setting === 123) ... } } I work alone mostly and have just started to become curious about all the bad habits I have. Thanks
-
Hey all, Another OOP question from me Is using constants in a class a bad idea? For example I have a config file and have this in a class: header("Location: ".LOGIN_LOCATION); Also is it OK to define $_SERVER variables in a construct? For example I have: class whatever { private $_remote_addr; private $_user_agent; function __construct() { $this->_remote_addr = $_SERVER['REMOTE_ADDR']; $this->_user_agent = $_SERVER['HTTP_USER_AGENT']; } .. or is it OK just to use $_SERVER for example in a mysql query? Thanks for all the help so far. Getting there!
-
How can I add various checkboxes to my Form Fillout page ?
ChadNomad replied to WilliamGrant's topic in PHP Coding Help
I think* you would need a unique name for each checkbox. If a checkbox is ticked/tiked it posts as "on". So... if($_POST['checkbox_name'] == "on") { // It's checked } -
Hi. I've been learning OOP and watched a about a "secure" OOP PHP login script. It was pretty good, and helped me to understand some OOP approaches, but I couldn't help thinking some of it was wrong. I'm not sure so feedback appreciated! The class starts of something like this: <?php class Login { private $_id; private $_username; private $_password; private $_passmd5; private $_errors; private $_access; private $_login; private $_token; public function __construct() { $this->_errors = array(); $this->_login = isset($_POST['login'])? 1 : 0; $this->_access = 0; $this->_token = $_POST['token']; $this->_id = 0; $this->_username = ($this->_login)? $this->filter($_POST['username']) : $_SESSION['username']; $this->_password = ($this->_login)? $this->filter($_POST['password']) : ''; $this->_passmd5 = ($this->_login)? md5($this->_password) : $_SESSION['password']; } Isn't "hard" setting variables, like the POST vars in the contruct bad? Shouldn't they be passed through elsewhere? I've learnt that OOP needs to be reusable and manageable as it's primarily the point of using OOP in the first place. I might be wrong but I noticed straight away that the above class doesn't seem reusable (in the true sense). Hopefully i'm getting the hang of it... Thanks
-
Hi. I want my code to be more efficient and logical and was wondering if anyone could point me in a good direction? For example i concatenate a lot of stuffs as well as escaping a lot. Example; $content = "<p>something</p>".$content; mysql_query("UPDATE the_table SET content='".$content."' WHERE id='".$r['id']."'") or die (mysql_error()); I cant think of any others right now but i'm sure i have lots of bad practices. Thanks
-
Hi all, Is it possible to use an already open socket? Sorry I'm finding it difficult to describe this. I have created a persistent connection to a service with this: $connection = pfsockopen($servier, $port, $errno, $errstr) or exit($errno . " " . $errstr); Using a while look like while (!feof($connection)) I can use fputs to send through the socket. My question... Can I find and use an already open socket from another file completely unrelated so I can send custom commands "on the fly" so to speak? Everything I've tried has landed me with supplied argument is not a valid stream resource so far... Any help appreciated. Thanks
-
Thanks for the help. That did it (I'm not any good with regular expressions). I ended up with this: $content = preg_replace('/\[img\](.*?)\[\/img\]/is', '', $r['body']); As it's the only tag I don't want parsed. Thanks
-
Sorry I have googled. I don't want to parse BB code, I want to remove everything within certain tags. So: [img=image] is completely removed. The tag, the content imbetween too. I appreciate the reply though.
-
I am getting news from a column that's in BB Code. I want snippets as "news" but I'm having some problems. For example some code that comes out is [img=http://linktoimage.com] which I want to remove. Basically I would like to remove the tags and the text inside! Help appreciated, thanks.
-
I'm writing a class (or trying) to make forms quicker to produce. Heres an example function: function input($type, $class, $value, $newline) { if (!empty($type)) { $html .= "type=".$type.self::space; } if (!empty($class)) { $html .= "class=".$class.self::space; } if (!empty($value)) { $html .= "value=".$value.self::space; } if (!empty($newline)) { $html .= self::lineBreak; } $this->output = '<input '.$html.' />'.self::newLine; echo $this->output; } What would be a better approach to all the if statements or is that perfectly fine? Thanks
-
You really do need to show more code... If you want to hide the image use CSS. For example: <img src="<?php echo $row_motos['img2']; ?>" width="250" height="187" border="0" style="visibility:hidden;" />
-
Can't you just replace the spaces with %20 with str_replace? <?php $url = "www.site.com/uploads/this is a test.doc"; // www.site.com/uploads/this%20is%20a%20test.doc echo str_replace(" ", "%20", $url); ?>
-
The reason your getting the "X" image with Internet Explorer is because the image doesn't exist (or your linking to it wrong). That's just the way the browser behaves. Are you sure you aren't trying to display an image depending on whether it's set in your array? You could do something like: if (isset($row_motos['img1'])) { // print the HTML for img1 } else { // print the HTML for img2 } ??
-
What does this output? print_r($row_motos);
-
[SOLVED] Using other class objects inside another class
ChadNomad replied to ChadNomad's topic in PHP Coding Help
Just what I was looking for, thanks! -
Use the time() and date() functions and store it in a column. <?php $nextWeek = time() + (7 * 24 * 60 * 60); // 7 days; 24 hours; 60 mins; 60secs echo 'Now: '. date('Y-m-d') ."\n"; echo 'Next Week: '. date('Y-m-d', $nextWeek) ."\n"; // or using strtotime(): echo 'Next Week: '. date('Y-m-d', strtotime('+1 week')) ."\n"; ?> http://uk.php.net/time Edit: Use the time function to create a timestamp like 1211287800 and the date function to format the string.
-
Still getting to grips with my transition to OOP. I have a simple database class like this: <?php // Database constants define("DB_SERVER", "localhost"); define("DB_USER", "root"); define("DB_PASS", ""); define("DB_NAME", "my_db"); class MySQL { function connect() { mysql_connect(DB_SERVER, DB_USER, DB_PASS) or die (mysql_error()); mysql_select_db(DB_NAME) or die (mysql_error()); } function query($sql) { $mysql_query = mysql_query($sql) or die (mysql_error()); return $mysql_query; } function fetchrow($sql) { $mysql_rows = mysql_fetch_row($sql) or die (mysql_error()); return $mysql_rows; } function fetcharray($sql) { $mysql_array = mysql_fetch_array($sql) or die (mysql_error()); return $mysql_array; } function fetchnum($sql) { $mysql_num = mysql_num_rows($sql) or die (mysql_error()); return $mysql_num; } } // Instantiate the class for procedural use $db = new MySQL(); // Connect to the database $db->connect(); ?> Now I'm using another class I need to use the db object. The only way I've figured this out is to instantiate it again within the class, like this: // Headers class. Used to find out age information class SystemInformation { // Header information function head_content() { $db = new MySQL(); $this->head_information_query = $db->fetch_array("SELECT * FROM system WHERE active='1'"); Is there a way to make the $db object global (not sure if global is the right word) so it can be used throughout the entire SystemInformation class?
-
Im new to OOP so my terminologies may be wrong. Is it pointless to use procedural scripting in OOP methods/functions? I'm trying to move over to OOP to make my code easier to update but I noticed it's basically the same... For example: class SystemInformation { // URI Variables var $page_id; var $reference_id; // Page Information function page_information() { $page_id = $_GET['id']; $reference_id = $_GET['ref']; // Category Page (i.e. central, development) if (isset($page_id) && !isset($reference_id)) { $category_information_query = mysql_query("SELECT * FROM categories WHERE category='".$page_id."'") or die (mysql_error()); $category_information = mysql_fetch_array($category_information_query); } // Specific Page (i.e. article, review) if (isset($page_id) && isset($reference_id)) { $section_information_query = mysql_query("SELECT * FROM indexation WHERE id='".$reference_id."'") or die (mysql_error()); $section_information = mysql_fetch_array($section_information_query); $category_information_query = mysql_query("SELECT * FROM categories WHERE category='".$section_information[category]."'") or die (mysql_error()); $category_information = mysql_fetch_array($category_information_query); } Is that completely pointless? I mean I can use classes for some good effect, such as a mysql wrapper, but should this style of scripting not be used?
-
I've been using this to highlight PHP code: $newcontent = preg_replace('/\[command\](.*?)\[\/command\]/ie', 'highlight_string(\'$1\', TRUE)', nl2br($page_information[content])); The only problem is it doesn't work on content that has multiple lines. Confused... Also, another question, how would I wrap a html div block around the highlight_string function? Any help appreciated.
-
I have some text stored in a database like this: I can't thing of a logical way to get the code and use the highlight_string function.... Confused! Help appreciated, thanks!
-
Just curious. If, for example, 5 people connected to a web page creating an image on the fly at the same time would the results be the same? Would the script just not execute to finish processing each image on at a time?
-
Determine whether user successfully downloaded the file
ChadNomad replied to lovesmith's topic in PHP Coding Help
Wouldn't giving an expiry date (such as 1 hour per mb) be a better way to achieve something like this? -
[SOLVED] Add/Subtract depending on positive/negative number?
ChadNomad replied to ChadNomad's topic in PHP Coding Help
Yeah I just noticed that! Thanks