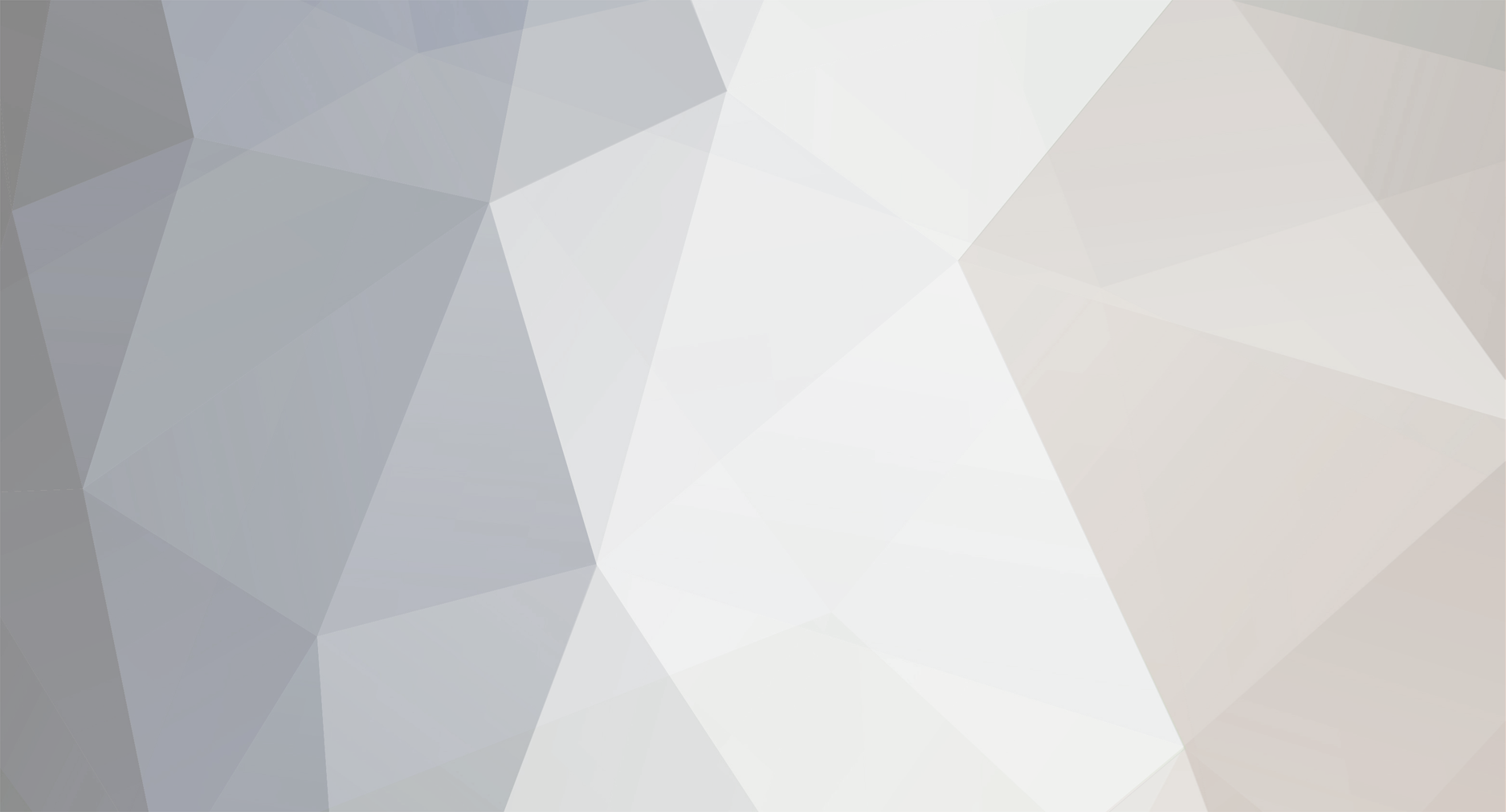
gurroa
-
Posts
87 -
Joined
-
Last visited
Never
Posts posted by gurroa
-
-
To display image you will have to create new php script that will accept pid and output only the image.
This new script vary from way you are saving image data into the dbase.
I would save images as base64_encode()d raw data.
And as long as you store imgdata into your message table don't select this field when you don't need it.
Otherwise imgdata is retrieved from your db too.
SELECT pid, usrname_email, userID, userstatus, full_message FROM $table_name ORDER BY userstatus ASC
<?php //viewimage.php Header("Pragma: no-cache"); Header("Cache-Control: no-cache"); Header("Expires: ".GMDate("D, d M Y H:i:s")." GMT"); if (isset($_GET['pid']) && is_int($_GET['pid'])) { mysql_connect(.... // connection $res = mysql_query("select imgdata from message where pid = ".$_GET['pid']); if ($res) { $row = mysql_fetch_assoc($res); // This is only if you are storing jpeg images Header("Content-type: image/jpeg"); Header("Content-Disposition: inline; filename=img".$_GET['pid'].".jpeg"); echo base64_decode($row['imgdata']); } } ?>
To put such image into your messages script
while($row = mysql_fetch_array($result)) { $display_block .= " <tr><td>---------------------------------------- <font color=blue><p>User/Email:".$row['usrname_email']." ".$row['userID']."</font> <img src=\"viewimage.php?pid=".$row['pid']."\" align=left ...> ----------------------------------------<p> User Status:$row['userstatus']<p>Date:$row[date]<p>Full Message:$row['full_message'] </td> </tr>"; }
-
Perhaps try copy/paste into new file and save again.
I've copied your code to a local file and it was compiled correctly.
-
I now have the following but get the error:
"Notice: Undefined variable: savecontent in /home/fhlinux165/s/swfro/user/htdocs/edityear.php"
Yes it is the same as with $save_file variable.
Read that manual page http://www.php.net/register_globals.
-
See source of the page that is returned. I think the whole code is displayed. (part that you can't see is in tag and hidden)
Check wheter php is installed properly.
Check wheter your file has acceptable extension (.php, .php5 etc.)
-
Your linux php configuration has registry_globals set to false.
$save_file is stored in associative array $_POST['save_file'] instead of being global.
-
If you can use javascript then save body.innerHTML
var origpage = document.body.innerHTML; function Revert() { document.body.innerHTML = origpage; }
-
Sorry to miss all the others...
$storedata = mysql_query('INSERT INTO liam_database(Username, Password, Email,Favourite Sport,IP,date); VALUES ( "'.addslashes($user).'", "'.md5($pass).'", "'.addslashes($email).'", "'.addslashes($sport).'", "'.addslashes($IP).'", "'.addslashes($date).'")') or die(mysql_error());
Missing , in values set and ) at the end.
-
Try your sql command without ; sign.
See http://dev.mysql.com/doc/.
INSERT INTO liam_database(Username, Password, Email, Favourite_Sport, IP, date) VALUES (....)
-
Try adding
<?php ini_set('max_execution_time', 300); // this should gave you more time ob_start(); // this should speed up outputing data
-
You should mention...
$year = trim($_POST['year']); $datefrom = ''; if (!empty($year)) { $ardate = array($year); if (strpos($year, '.') !== false) $ardate = Explode('.', $year); else if (strpos($year, '-') !== false) $ardate = Explode('-', $year); $c = count($ardate); if ($c == 3) { $datefrom = sprintf("%04d-%02d-%02d", trim($ardate[2]), trim($ardate[1]), trim($ardate[0]) ); $dateto = $datefrom; } elseif ($c == 2) { $datefrom = sprintf('%04d-%02d-01', trim($ardate[1]), trim($ardate[0]) ); $dateto = Date("Y-m-d", strtotime("+1 month -1 day", strtotime(sprintf('%04d-%02d-01', trim($ardate[1]), trim($ardate[0])) )) ); } elseif (is_integer($year)) { $datefrom = sprintf('%04d-01-01', $year); $dateto = sprintf('%04d-12-31', $year); } } if (!empty($datefrom)) { $sql = "SELECT * FROM Temper WHERE Date >= '{$datefrom}' and Date <= '{$dateto}'"; .... }
-
Simple don't do it.
Use session, dbase or plain file.
If user can see it but should not change it, use md5 check method
$url = 'viewarticle.php?id='.$id.'&check='.md5(md5('my'.$id.'test'.$id));
and test it on the other side.
-
If you don't want to wait for reply..
http://www.php.net/manual/en/index.php
$prod = array(); // creates new empty array reset($arProd); // set array position to the first element while(list(,$row) = each($arProd)) { // this will take element from $arProd array on current position and push position to the next element $prod[] = $row['Name']; // add value from the $row array under the key 'Name' to the $prod array } echo Implode(", ", $prod); // print out all values in the $prod array split by ', ' string
You can't use your while statement cause it will be an infinitive loop
To print out data as you've mentioned use my previous construct with this output
if (count($arProductsByItem) > 0) { reset($arProductsByItem); while(list($Item,$arProd) = each($arProductsByItem)) { echo 'Item: '.$Item.'<br />'; reset($arProd); while(list(,$row) = each($arProd)) { echo 'product'.$i.' '.$row['Name'].' : '.$row['PriceRetail'].'<br />'; ++$i; } echo '<br />'; } }
-
<?php $arProductsByItem = array(); if ($numProduct > 0 ) { $i = 0; while ($row = dbFetchAssoc($result)) { $arProductsByItem[$row['ItemName']] = $row; } } // example of later output if (count($arProductsByItem) > 0) { reset($arProductsByItem); while(list($Item,$arProd) = each($arProductsByItem)) { echo 'Item: '.$Item.' - Products: '; $prod = array(); reset($arProd); while(list(,$row) = each($arProd)) { $prod[] = $row['Name']; } echo Implode(", ", $prod); } } ?>
-
I meant second and others rows for bleach
-
Your multi_file table consist at least of fields
mult_id
synopsis
file_picture
file_name
file_description
file_link
first row for bleach is
mult_id => '1', synopsis => 'Ichigo Kurosaki ... SEE anime', file_picture => 'http://www.mu-anime.com/bleach.jpg',
file_name => 'Bleach Episode 1', file_description => 'The day I became a shinigami', file_link => 'http://www.megaupload.com/?d=IASUWFNF'
second and others has only filled: mult_id, file_name, file_description, file_link
You should change your dbase. My opinion is to split your table into two
multi_file - mult_id, synopsis, file_picture
sub_files - mult_id, file_picture, file_name, file_description, file_link
-
And have you put the link to every row?
It seems that only first entry has the file_picture field and synopsis field filled.
-
Make sure you're not missing file_picture values in the db.
while($myarray = mysql_fetch_array($sql)){ // Build your formatted results here. echo $myarray['synopsis']."<br /><br />"; if (!empty($myarray['file_picture'])) { echo "<img src='" .$myarray['file_picture']. "' align=\"right\" alt=\"\" />"; } echo "<font color=\"#3399FF\">Episode:</font>" . " " .$myarray['file_name']."<br />"; echo "<font color=\"#3399FF\">Title:</font>" . " " .$myarray['file_description']."<br />"; echo "<font color=\"#3399FF\">Link:</font>" ." ". "<a href=\"".$myarray['file_link']. "\" target=\"_blank\">Click Here To Download</a>"; }
-
$sql = "SELECT * FROM Temper WHERE Date >= '{$_POST['year']}-01-01' and Date <= '{$_POST['year']}-12-31'"
-
This construct
echo (isset($SireRow) ? $SireRow['RegName'] : '');
is the same as
if (isset($SireRow)) echo $SireRow['RegName']; else echo '';
php function isset() returns true/false if the variable is set or not.
-
include('phpthumb.functions.php'); include('phpthumb.bmp.php'); $jpg = imagecreatefromjpeg('test.jpg'); $phpbmp = new phpthumb_bmp(); file_put_contents('test.bmp', $phpbmp->GD2BMPstring($jpg));
-
won't do TIFF and it does say BMP but its using the GD library so i think thats only WBMP
See http://phpthumb.sourceforge.net/index.php?source=phpthumb.bmp.php.
-
-
It's because you don't resend your ID value
Try http://cristi.prosportequipment.ro/prosportnew/produse.php?id=1&page=2 and you will see second page.
Also you should add some tests to prevent sql hacks and never shows query to the user.
-
http://www.w3.org/TR/CSS21/text.html#propdef-text-decoration
...The color(s) required for the text decoration must be derived from the 'color' property value of the element on which 'text-decoration' is set. The color of decorations must remain the same even if descendant elements have different 'color' values.
[SOLVED] Pop up box
in Javascript Help
Posted
use