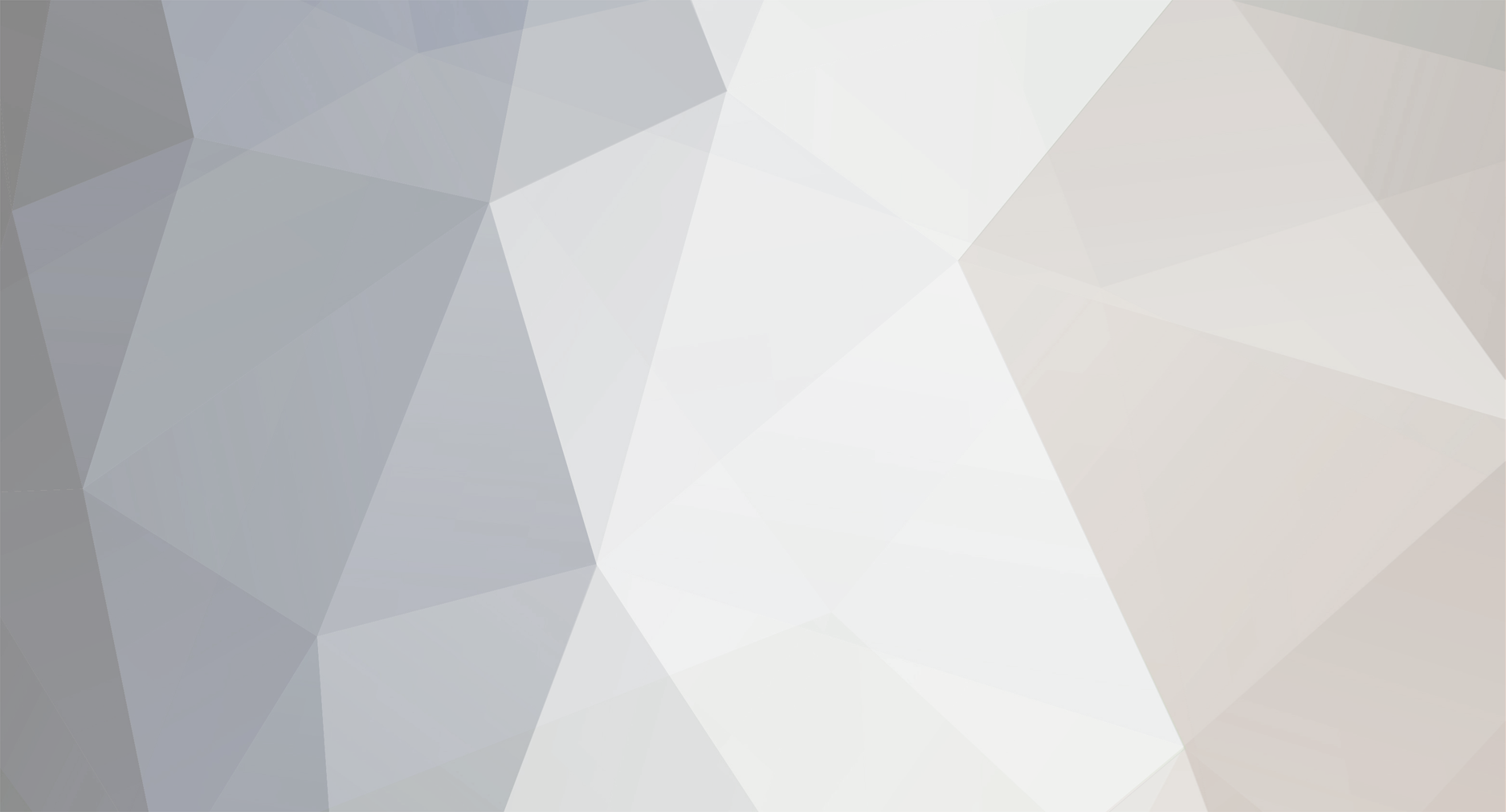
lemmin
-
Posts
1,904 -
Joined
-
Last visited
-
Days Won
2
Posts posted by lemmin
-
-
You could either use cURL (or similar) to send the correct cookie for your timezone (I see that is an option on the site), or you could combine the day headers with the time and use strtotime() with the correct time addition to create a timestamp of the correct date/time.
-
I agree with godleydesign on the structure change.
I think you are getting close!
Are you sure that your table contains that data? Try running the query as it would be in phpMyAdmin or a similar utility.
SELECT * FROM srs_course WHERE coursetitle LIKE '%accounting%'
If you get results from that, you might consider this warning from php.net:
If the last SQL statement executed by the associated PDOStatement was a SELECT statement, some databases may return the number of rows returned by that statement. However, this behaviour is not guaranteed for all databases and should not be relied on for portable applications.
http://php.net/manual/en/pdostatement.rowcount.php
See if that query works first, though
-
It sounds like you just want a generic feedback-type form. Here is some simply code that would push the input of a textarea into your database:
<form method="post"> Question:<br/> <textarea name="answer"></textarea> <input type="submit"/> </form> <?php if (isset($_POST['answer'])) //Form was submitted { mysql_connect('localhost', 'user', 'pass'); mysql_select_db('database'); mysql_query('INSERT INTO tablename VALUES ("'.$_POST['answer'].'")'); } ?>
That is very generic and obviously needs to be adjusted for your scenario. Is this the sort of form you are looking for?
-
Are you sure this is ALL pictures from an iPad? My first guess is that, since an iPad doesn't really have a permanent orientation, the pictures themselves were actually taken upside-down.
If they are ALL upside-down, you can flip anything coming from an iPad, but if it is just some, you would need some kind of orientation recognizing software to solve that problem.
-
Ok. Where will the answer go? Into a database? Emailed to someone?
-
Oh, I read the error wrong. I read "unknown column" for some silly reason.
If you want the search term to be passed through the URL, simply change the form method to "get" and the variables from $_POST to $_GET.
I think the disconnection might be at your switch case. What variable are you switching to test for "search?"
-
I don't mean to steer you away, but this is covered in many tutorials online. You can follow this tutorial and simply adjust the filename when calling move_uploaded_file():
http://www.tizag.com/phpT/fileupload.php
move_uploaded_file():
http://us1.php.net/manual/en/function.move-uploaded-file.php
-
What kind of question? Multiple choice? Feedback text?
-
I don't see how any of this code would output that error. I think you may have forgotten a colon (
somewhere. Could you post your updated code that you are now running?
-
You aren't binding ":searchterm" to anything. Try changing these three lines:
$searchterm = '%'.$_POST['searchterm'].'%'; $sql = "SELECT * FROM srs_course WHERE coursetitle LIKE :searchterm "; $query->bindParam(':searchterm', $searchterm, PDO::PARAM_STR);
-
I've actually done efficiency testing on this and #1 is the most efficient. The difference is negligible to a fairly large number of loops, so personal choice weighs more for most situations.
Also, it is helpful to know (if you didn't) that a negative value can be passed as the length for substr():
$sql = "SELECT * FROM `navigation` ORDER BY position ASC"; $qry = mysql_query($sql); confirm_query($qry); $out = ''; while ($res = mysql_fetch_array($qry)) { $out .= "<a href=\"{$res['url']}\">" . $res['page'] . "</a> | "; } echo substr($out, 0, -3);
-
Sorry, I switched the variables on accident. This should work:
$data = file_get_contents('http://tvlistings.zap2it.com/tvlistings/ZCSGrid.do?stnNum=10179'); preg_match_all('/<a id="rowTitle\d+" class="zc-ssl-pg-title"[^>]*>([^<]+)<\/a>/im', $data, $matches); $titles = $matches[1]; print_r($titles);
-
If you are naming (or renaming) these files in PHP you can change the name based on a simple character translation:
http://us1.php.net/manual/en/function.strtr.php
$filename = strtr($filename, ' ', '_');
-
I think the geocode location type requires coordinates. Try adding this to your request
&type=geocode
https://developers.google.com/places/documentation/autocomplete#place_types
-
You can use parentheses to capture segments:
$data = file_get_contents('http://tvlistings.zap2it.com/tvlistings/ZCSGrid.do?stnNum=10179'); preg_match_all('/<a id="rowTitle\d+" class="zc-ssl-pg-title"[^>]*>([^<]+)<\/a>/im', $test, $matches); $titles = $matches[1]; print_r($titles);
-
Your URL is being encoded -- probably by the browser; since it is, in fact, a URL, there shouldn't be a problem.
I think the problem you are having is actually with your URL BEFORE it is URL-encoded. Notice the "%7D" at the end of the example you gave? That shouldn't be there. That is the encoded "}" character. Computermax2328 suggested you remove the curly braces and I think they might actually be your only problem.
Did you try his solution?
Another solution that may be more portable would be to concatenate the filename inside your link:
echo "<a href='php/website/files/".$inf."'>Link</a>";
-
The problem is in your regular expression. In your first post, you can fix the regex by simply removing the square brackets ([]) leaving the characters inside. That matches the sample input you gave in your first post, but your newest expression is completely different so I'm not sure what exactly you are trying to match.
You probably want to do something like this:
$data = file_get_contents('http://tvlistings.zap2it.com/tvlistings/ZCSGrid.do?stnNum=10179'); preg_match_all('/<a id="rowTitle\d+" class="zc-ssl-pg-title".*<\/a>/im', $data, $matches); $titles = $matches[0]; print_r($titles);
If you are NOT trying to get all the titles, which ones do you want?
-
The content div that you removed had styling for both the sidebar-right as well as that padding that you are now missing. You can add it back in by adding this line to your stylesheet (style.css):
#main-wrap{padding:50px}
-
What if the coordinates don't map a quadrilateral? What determines the corners if the shape is more of a circle?
-
You should be able to get a lot of this information in just one query. This is an example of the tables "joined." (You can avoid JOIN when every row has a related row in every table. This is much more efficient than using the JOIN clause.)
SELECT u.user_id, u.time_zone, tt.time_start, tt.time_end FROM users p, project p, project_track pt, track_time tt WHERE p.user_id = u.user_id AND pt.project_id = p.project_id AND tt.track_id = pt.track_id AND u.user_name = $username
Try running that query and looking at the output. You can probably add more criteria to the WHERE clause to narrow down your results.
-
There is still not enough information to do this, but I can give you an idea:
mysql_connect($db, $user, $pass); //Connect to db mysql_select_db('yourdb'); //Select db $r = mysql_query('SELECT * FROM yourtable'); //query for listings while ($row = mysql_fetch_assoc($r)) //loop through listings { $days = 2; //set days $seconds = 60 * 60 * 24 * $days; //calculate seconds in days if ((time()-$row['share_datecreated']) < $days) //check stamp //New listing }
This assumes that your share_datecreated field is a timestamp and that you have no criteria for which listings to grab from the database.
-
Or
if (strpos($notes, 'http:') !== false)
Since it is just checking for a string in a string.
PaulRyan's should work too.
-
More information is needed to do this.
What type of database are you using and how is it structured?
-
I remember now that I used pack() (along with my function) in the generation of the same binary data, but I can't remember exactly why I had to. I guess there was a format it couldn't do.
Anyway, pack() is definitely a better solution if it works.
Ask question
in PHP Coding Help
Posted
I agree with Jessica; the easiest (and incidentally best) solution would be to use a forum solution like Wordpress or Drupal.