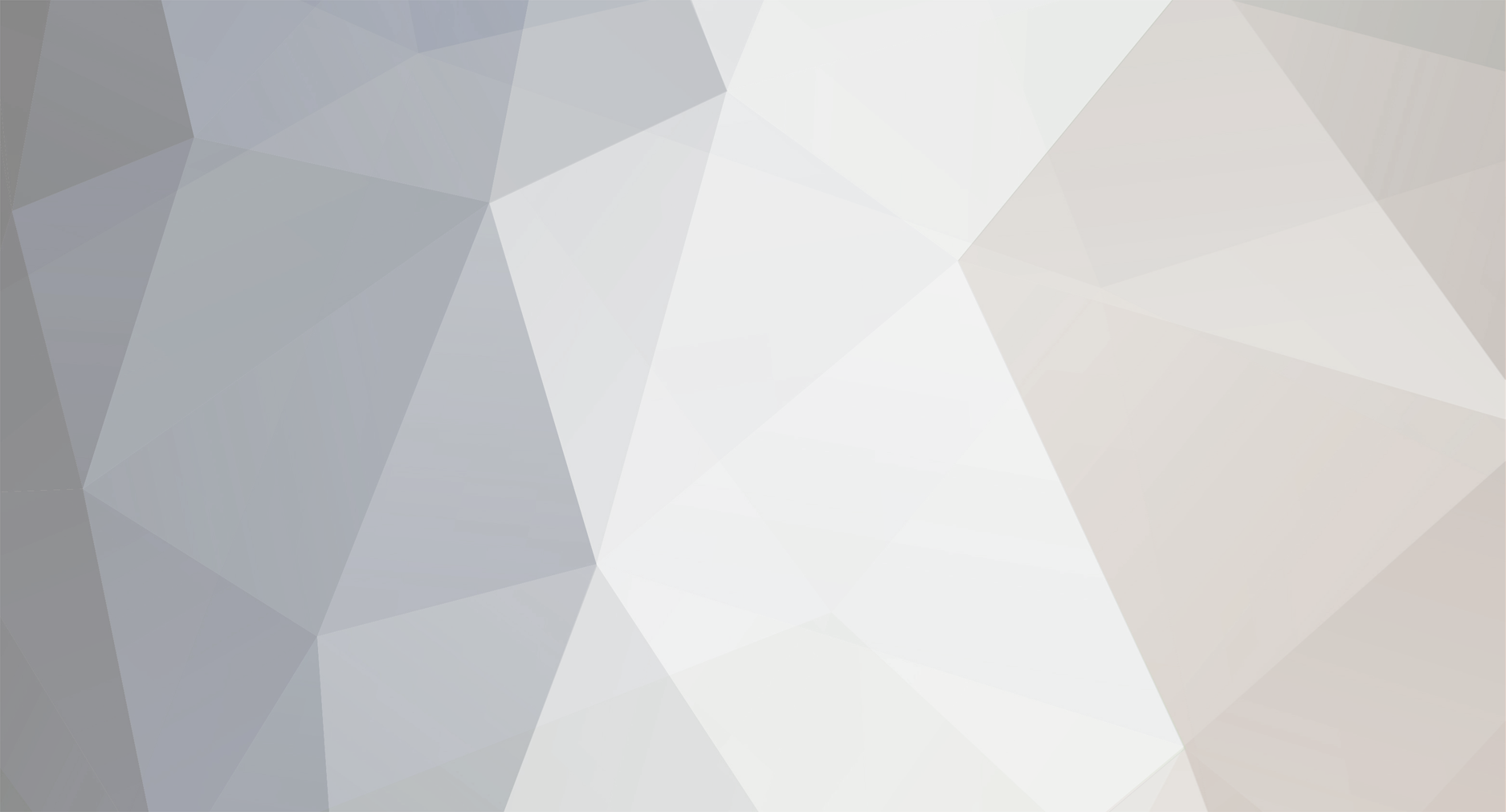
richrock
-
Posts
213 -
Joined
-
Last visited
Posts posted by richrock
-
-
I'm looking at a way to do a query where a music entry has at least one published value in another table. My mysql knowledge is ropey at best
but learning all the time anyway.
Here's what I've tried :
$q = "SELECT COUNT( a.id ) FROM #__music_artists a LEFT JOIN #__music_albums al ON a.id = al.artist_id WHERE a.surname LIKE '" . $id . "%' AND 1 IN (al.published)";
Which doesn't work. Basically my problem is is that each artist may have published and unpublished albums, so I need to check the albums table to see if there is at least one published album amongst what could be loads of unpublished albums, or the opposite.
I just need the published to return true, and let the artist do the counting for calculating a table display.
Any ideas/help on this? I looked at LEAST() but that did nothing as well.
-
I've got a script that works in pretty much everything else I've got, but Safari OSX.
The script is
<?php ini_set ("display_errors", "1"); error_reporting(E_ALL); clearstatcache(); $filename = $_GET['file']; // place this code inside a php file and call it f.e. "download.php" $path = $_SERVER['DOCUMENT_ROOT']."/"; // change the path to fit your websites document structure $fullPath = $path.$filename; if ($fd = fopen ($fullPath, "r")) { $fsize = filesize($fullPath); $path_parts = pathinfo($fullPath); $ext = strtolower($path_parts["extension"]); switch ($ext) { case "pdf": header("Content-type: application/pdf"); // add here more headers for diff. extensions header("Content-Disposition: attachment; filename=\"".$path_parts["basename"]."\""); // use 'attachment' to force a download break; default; header("Content-type: application/octet-stream"); //header("Content-type: audio/mpeg"); header("Content-Disposition: filename=\"".$path_parts["basename"]."\""); } header("Expires: 0"); header("Last-Modified: " . gmdate ("D, d M Y H:i:s", filemtime ($filepath)) . " GMT"); header("Content-length: $fsize"); header("Pragma: public"); //header("Cache-control: private"); //use this to open files directly header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Accept-Ranges: bytes"); header("Connection: close"); while(!feof($fd)) { $buffer = fread($fd, 2048); echo $buffer; } } fclose ($fd); exit; // example: place this kind of link into the document where the file download is offered: // <a href="download.php?download_file=some_file.pdf">Download here</a> ?>
The result is an url that looks like this:
The urls are correct, the mp3 file is there.
And Safari on the mac seems absolutely bent on trying to play the file instead of downloading it. PC versions of Safari work fine and download quite happily. Is this something I have any control over, or has it been set by the end-user?
I changed the header set up based on an article I found which fixed the PC Safari/Chrome issues I had.
-
Right, figured the filesize refers to actual location (as mentioned earlier), so I now get a report of
34064439
when I get the filesize of a 15mb mp3. That may explain why I'm only getting 400-1000k download file sizes. Can anyone help with filesize()?
-
Right it's now working, although I have another problem affecting the downloads - filesize($filename) fails. The error I get is:
Warning: filesize() [function.filesize]: stat failed for /bromptons_auctioneers_15/music/Sibelius/Sibelius/01 Violin Concerto.mp3 in F:\html\bromptons_auctioneers_15\components\com_music\assets\zipit.php on line 35And I have tried using clearstatcache() at the start of the script to reset anything. Still with the errors...
FWIW - the typical filesizes are around the 15-20mb mark...
-
Thanks, I'll check into those. I'm running the script via ajax, sending variables to trigger the on the download script.
-
Hi,
I've been trying to get a script to force a download of a zip archive, but it's not working... I am too dumb to figure why, although I've tried a lot of options.
// Create a zip file based on parameters. // Set up the POST data $z_title = $_POST['album']; $z_id = $_POST['id']; require_once('../../../configuration.php'); $jconfig = new JConfig(); $db_error = "Database not found. Please contact a system administrator."; $db_config = mysql_connect( $jconfig->host, $jconfig->user, $jconfig->password ) or die( $db_error ); mysql_select_db( $jconfig->db, $db_config ) or die( $db_error ); $q = "SELECT zip_file FROM jos_music_albums WHERE id = '" . $z_id . "'"; $query_execute = mysql_query($q); $result = mysql_fetch_row($query_execute); $destination = $result[0]; mysql_close($db_config); $destination_alpha = basename($destination); $destination_bravo = $_SERVER['DOCUMENT_ROOT']. "/bromptons_auctioneers_15/" . $destination; $filesize = filesize($destination); header("Pragma: public"); // required header("Expires: 0"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Cache-Control: private",false); // required for certain browsers header("Content-Type: application/zip"); header("Content-Disposition: attachment; filename=\"".basename($destination)."\";" ); header("Content-Transfer-Encoding: binary"); header("Content-Length: ".$filesize); readfile("$destination"); exit;
The actual test file is a zip archive located in http://www.sitename.com/music/artist/album.zip
It's *definitely* there
I have tried using absolute paths either from the url or from the fileserver, and relative paths don't seem to work either. The error I get (this was with the code above):
Warning: filesize() [function.filesize]: stat failed for music/Sibelius/Sibelius/Sibelius.zip in F:\html\bromptons_auctioneers_15\components\com_music\assets\zipit.php on line 25
Warning: readfile(music/Sibelius/Sibelius/Sibelius.zip) [function.readfile]: failed to open stream: No such file or directory in F:\html\bromptons_auctioneers_15\components\com_music\assets\zipit.php on line 36
When I have managed to get this to work (supposedly), I crash the browser. The memory usage leaps up - first time I noticed my browser was using 1.8gb of memory, increasing by about +/- 250mb a second. This seems to occur everytime I use absolute paths, and cannot figure why. Firebug reports nothing wrong (maybe it's failed by this point), and am at a loss.
Any ideas/alternative code would be appreciated - codes a bit of a mess too, there's remants of stuff I've tried before too...
-
Hi,
I'm trying to extract the first paragraph from a text entry in the database, to show a list of items. I've set up some sample data like this:
<p class="team_intro"><img src="john_smith.jpg" /><span class="leadername">John Smith</span> - a great funny witty super guy. </p><p>Rest of the text blabh blablahvlvlvlvdfjerfh etc... </p>
That is the data I would retrieve in my for loop, and I can't do much about image position, as the editor (joomla) seems to place it inside the first paragraph. I can't really use ID's in the p or img tags as joomla doesn't handle that.
I have managed to extract the image using some code from another source:
$intro = $teamMembers[$i]->introtext; $intro_clean = preg_replace("/<div[^>]+\>/i", "", $intro); // Strips any divs in case they are inserted from C&P text $intro_image = preg_match("/<img[^>]+\>/i", $intro_clean, $matches); $intro_clean = preg_replace("/<img[^>]+\>/i", "", $intro);
I've tried to extract the first paragraph using:
preg_match("/<p>(.*)<\/p>/",$intro_clean,$names); $introsentence = strip_tags($names[0]); //removes anchors and other tags from the intro
but it doesn't work. How to I get the whole first paragraph? Help!?!!
-
text-align: right would not work - it's not the position of the text, but it seems you cannot make 3 columns in either a div or table fit a container and be equally spaced. I'll knock up an image to let you know what I mean, it's a bit difficult with text.
I don't do the designs, they're done by a design team, and they use a 4px grid. This means all numbers have to be divisible by 4. I just used 576 as an example, as it's the optimum width for content in html emails.
It doesn't matter on the width, but the princinple is the same. In print you can get columns correctly spaced, yet on the web you can't?
-
Although I've been doing html/css for years, I can't quite figure out how to do equal spacing 'properly'.... I typically fudge it by making the right side wider than the required border - it'll make sense below.
What I mean is : Say you have a table 600px wide, and you need to create 3 equal columns. There is a border round this table of, say, 12px, for the width available is 600-(12x2) = 576px.
Divide this by three, and you get equal column widths of 192px.
But... in the interests of good design (rather unlike our Olympic logo/mascot
) : The second and third columns would need a padding on the left of 12px too.. Thus making :
is now 180px wide (not 192)
is now 180px wide (not 192)
As you can note, cells/columns 2 & 3 are now 180px, NOT 192px. How would you work this out so that the three columns are equally spaced, and not padded on the left side, and the right side reaches the end of the 576px available? It would be easy to add padding-right to the cells, but this makes it 12px short on the last cell, and IE6 doesn't support :last-child, only :first-child
Any ideas? Bear in mind that this will have to work with end-users NOT fluent in HTML/CSS but would have basic guidance on adding styles..
-
My bad - I don't know the difference between local and remote databases
:
-
Gah! Me and my hurried typing - its simply supposed to count the total number of entries in a table, based on the left joins matching up (city is present in table, etc)
phpmyadmin tells me when I count(*) I have ~25,500 results (rounding), yet running this in joomla tells me I have #77,000, quite a discrepancy. And phpmyadmin is correct.
-
Hi,
I've got a query that's throwing up an error, really stuck why. It read right to me, but it's just not working.
Its attempting to count all fields from a table with left joins as criteria for filtering....
SELECT COUNT(i.*) AS value FROM jos_yearbook_instruments i LEFT JOIN jos_yearbook_country co ON i.country_id = co.id LEFT JOIN jos_yearbook_city ci ON i.city_id = ci.id LEFT JOIN jos_yearbook_circa c ON i.circa_id = c.id LEFT JOIN jos_yearbook_makers m ON m.id = i.maker_id LEFT JOIN jos_yearbook_auctioneers a ON a.id = i.auctioneer_id
Can anyone help? I tried i.id but that gave me an incorrect total... ?
-
Well, it's a case of just redirecting to the nearest usable page, as the whole site has been rebuilt, etc.
So in the old site it would have had:
product.html?prod_id=3
We're not going to bother with linking to the extact product again (there are 30,000 items, a lot of headaches could ensue in testing) so we'll go to the nearest main catalogue page, eg:
product/catalogue.html
Without the appended query string...
Hope this makes sense...
-
Oops, I may have mis-worded my question -
I need to remove the ?page=something, redirecting to somthing.html instead?
Sorry if I'm a little dull, I'm only 24hrs into htaccess (other than redirecting domains
)
-
excellent! I can work it from there, I think
One other query - I have a number of fixed pages using ?page=something on the url - I've tried doing simple redirects, but these are still adding the appended ?page=something on the url, instead of going to site.com/something.html??
-
I'm in the process of updating a website, but we also have to modify htaccess to do 301 redirects on the existing links, for reasons not strictly needed.
90% of this has gone well, but:
I have thousands of dynamic SEF urls similar to this -
www.example.com/product/2234/producttitle.html
Which would need to be redirected, to something like:
www.example.com/catalogue/1009/producttitle.html
- I would guess I need to send this to a script to process, but how do I refer to a php script to run this query through? The 2234 is the product ID in the database, and 1009 is the Catalogue ID (shows how many need to be done
)
Any pointers greatly appreciated. .htaccess is not my strong point, in fact only really learnt stuff today!
EDIT = OR......
change to a non-sef url like this:
index.php?option=com_items&id=2234&lang=en&task=viewitem
where I can put the 2234 from the url at the top into the url above....
-
deanlearners snippet did it for me - I'll keep that on snipplr now
Thanks
-
Hi all,
I've got a string, extracted from a database of stored filenames. I can remove the .jpg part, and I need to also remove the last part - a couple of examples:
21_amati-andrea_cremona-circa1580-rtc - need to remove '-rtc'
33_amati-antonius-and-hieronymus_cremona-circa1620-b - need to remove '-b'
35_amati-antonius-and-hieronymus_cremona-circa1620-rs - need to remove '-rs'
These are view codes on the image for a page being used to show different views of an instrument. I just need to dynamically extract this part to make a SEF url.
Anyone know how to remove the end part including the '-'? I'm sure I'd done it before, but cannot figure out whne or where
I've checked the PHP pages, and looked through w3c schools pages but nothings registering with my brain cells...
-
Hi,
I'm doing some validation on a form, and just need to fine-tune one part of it - the dates are entered numerically, eg 01-02-10, and want to ensure that a number is used for the date. So I did this:
if (practitioner_month_referred == null || practitioner_month_referred == "" || practitioner_month_referred == "<?php echo $monval; ?>" || isNaN(practitioner_month_referred)) { if (focused == false ) { document.getElementById('practitioner_month_referred').focus(); focused = true; } if(isNaN(practitioner_month_referred)) { numvalid = false; } else { numvalid = true; } document.getElementById('practitioner_month_referred').style.color="<?php echo $formcol_3; ?>"; document.getElementById('practitioner_month_referred').style.fontWeight="bold"; valid = false; }
But, it seems that if I type in 01 as a month in this example, is tells me that this is not a number (by the big red error message from numvalid being switched to false.
Is the isNaN right? Or is there some other way of checking that it is in fact a number, not letters or anything else?
-
Never mind - figured that the return valid line should have been uncommented - works fine now.
-
Okay, I've been scratching my head on this for a while, but can't see why: When I send this form, the Referring Dentist name should highlight with a red background colour, however, it only does it momentarily, before reverting to white - anyone see why?
PHP code with JS
<html> <head> <script type="text/javascript"> function formValidator(){ var valid = true; var message = ""; // Make quick references to our fields var practitioner_name = document.getElementById('practitioner_name').value; var focused = false; if (practitioner_name == null || practitioner_name == "" || practitioner_name == "Name*") { //if (focused == false ) {document.getElementById('practitioner_name').focus(); focused = true;} document.getElementById('practitioner_name').style.backgroundColor="#ff0000"; alert('pause'); valid = false; } if (valid == false) { document.getElementById('error_in_form').style.display='block'; } else { document.getElementById('vstr').value = 'ax8F5zEeS'; alert('sending form'); //document.getElementById('referral').submit(); } //return valid; } </script> <link href="template.css" rel="stylesheet" type="text/css" /> </head> <body> <?php $separate = "<span style='display: inline-block; width: 100%; border-bottom: 1px solid silver; padding-top:12px; margin-bottom:12px;'><!-- underline --></span>"; ?> <div id="referral_form"> <?php echo $separate; ?> <div id="error_in_form" style="display:none;">Please fill in the highlighted fields.</div> <table cellpadding="0" cellspacing="0" border="0" width="100%"> <tr> <td width="458" style="padding-right:16px;"> <form action="referral.php" method="post" name="referral"> <table cellpadding="0" cellspacing="0" border="0" width="100%"> <tr> <td colspan="2"><h3>Referring Dentist Details</h3></td> </tr> <tr> <td><input type="text" name="practitioner_name" id="practitioner_name" value="Name*" onfocus="if(this.value == 'Name*'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Name*';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Name*') { this.value=''; this.style.color = '#a7b325';}" onkeyup="if(this.value == 'Name*') { setSelectionRange(0,0)}" /></td> <td>Date <input type="text" name="practitioner_day_referred" value="DD" size="2" onfocus="if(this.value == 'DD'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'DD';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'DD') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'DD') { setSelectionRange(0,0)}" /> <input type="text" name="practitioner_month_referred" value="MM" size="2" onfocus="if(this.value == 'MM'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'MM';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'MM') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'MM') { setSelectionRange(0,0)}" /> <input type="text" name="practitioner_year_referred" value="YY" size="2" onfocus="if(this.value == 'YY'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'YY';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'YY') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'YY') { setSelectionRange(0,0)}" /> </td> </tr> <tr> <td colspan="2" class="contact-cell"> <textarea name="practice_address" value="Practice Address*" onfocus="if(this.value == 'Practice Address*'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Practice Address*';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Practice Address*') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Practice Address*') { setSelectionRange(0,0)}" >Practice Address*</textarea> </td> </tr> <tr> <td><input type="text" name="practice_postcode" value="Postcode*" onfocus="if(this.value == 'Postcode*'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Postcode*';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Postcode*') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Postcode*') { setSelectionRange(0,0)}" /></td> <td><input type="text" name="practice_email" value="Email" onfocus="if(this.value == 'Email'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Email';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Email') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Email') { setSelectionRange(0,0)}" /></td> </tr> <tr> <td><input type="text" name="practice_phone" value="Telephone*" onfocus="if(this.value == 'Telephone*'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Telephone*';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Telephone*') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Telephone*') { setSelectionRange(0,0)}" /></td> <td><input type="text" name="practice_mobile" value="Mobile" onfocus="if(this.value == 'Mobile'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Mobile';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Mobile') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Mobile') { setSelectionRange(0,0)}" /></td> </tr> <tr> <td colspan="2"> <?php echo $separate; ?> </td> </tr> <tr> <td colspan="2"> <h3>Patients Details</h3> </td> </tr> <tr> <td><input type="text" name="patient_fname" value="First Name*" onfocus="if(this.value == 'First Name*'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'First Name*';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'First Name*') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'First Name*') { setSelectionRange(0,0)}" /></td> <td><input type="text" name="patient_sname" value="Surname*" onfocus="if(this.value == 'Surname*'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Surname*';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Surname*') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Surname*') { setSelectionRange(0,0); }" /></td> </tr> <tr> <td><input type="text" name="patient_title" value="Patient Title" onfocus="if(this.value == 'Patient Title'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Patient Title';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Patient Title') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Patient Title') { setSelectionRange(0,0); }" /></td> <td>Date <input type="text" name="patient_day" value="DD" size="2" onfocus="if(this.value == 'DD'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'DD';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'DD') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'DD') { setSelectionRange(0,0); }" /> <input type="text" name="patient_month" value="MM" size="2" onfocus="if(this.value == 'MM'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'MM';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'MM') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'MM') { setSelectionRange(0,0); }" /> <input type="text" name="patient_year" value="YY" size="2" onfocus="if(this.value == 'YY'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'YY';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'YY') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'YY') { setSelectionRange(0,0); }" /> </td> </tr> <tr> <td colspan="2" class="contact-cell"> <textarea name="patient_address" onfocus="if(this.value == 'Contact Address'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Contact Address';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Contact Address') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Contact Address') { setSelectionRange(0,0); }" >Contact Address</textarea> </td> </tr> <tr> <td><input type="text" name="patient_postcode" value="Postcode" onfocus="if(this.value == 'Postcode'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Postcode';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Postcode') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Postcode') { setSelectionRange(0,0); }" /></td> <td><input type="text" name="patient_email" value="Email" onfocus="if(this.value == 'Email'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Email';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Email') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Email') { setSelectionRange(0,0); }" /></td> </tr> <tr> <td><input type="text" name="patient_phone" value="Telephone" onfocus="if(this.value == 'Telephone'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Telephone';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Telephone') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Telephone') { setSelectionRange(0,0); }" /></td> <td><input type="text" name="patient_mobile" value="Mobile" onfocus="if(this.value == 'Mobile'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Mobile';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Mobile') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Mobile') { setSelectionRange(0,0); }" /></td> </tr> <tr> <td colspan="2"> <?php echo $separate; ?> </td> </tr> <tr> <td colspan="2"> <h3>Reason for referral</h3> </td> </tr> <tr> <td colspan="2" class="contact-cell"> <textarea name="referral_reason" value="Reason for referral" onfocus="if(this.value == 'Reason for referral'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Reason for referral';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Reason for referral') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Reason for referral') { setSelectionRange(0,0); }" >Reason for referral</textarea> </td> </tr> <tr> <td colspan="2"> <table cellpadding="0" cellspacing="0" border="0"> <tr> <td> Investigate and treat Yes <input type="radio" name="investigate" /> No <input type="radio" name="investigate" /> </td> <td> Opinion only Yes <input type="radio" name="opinion" /> No <input type="radio" name="opinion" /> </td> </tr> </table> </td> </tr> <tr> <td colspan="2" class="contact-cell"> <textarea name="medical_details" onfocus="if(this.value == 'Relevant medical details'){ this.style.color = '#b3b3b3';} setSelectionRange(0,0);" onblur="if(this.value == ''){ this.value = 'Relevant medical details';} this.style.color = '#a7b325';" onkeypress="if(this.value == 'Relevant medical details') { this.value=''; this.style.color = '#a7b325'; }" onkeyup="if(this.value == 'Relevant medical details') { setSelectionRange(0,0); }" >Relevant medical details</textarea> </td> </tr> <tr> <td colspan="2">Patient anxious Yes <input type="radio" name="anxious" /> No <input type="radio" name="anxious" /></td> </tr> <tr> <td colspan="2"><button name="checkmail" value="confirm" onclick="formValidator();" >Submit</button></td> </tr> </table> <input type="hidden" name="vstr" id="vstr" value="" /> </form> </td> <td><h3>If you would like to refer one of your patients for specialist treatments, please fill in the form below. If you would like to discuss your patient's needs further, please call 0121 236 7630</h3></td> </tr> </table> </div> </body> </html>
And the css just incase (I've looked through this but the inputs are not set with any colors anyway)
/*----------------------------------------------------------------------------- [client] Screen Stylesheet version: 1.0 date: 01/10/09 author: richard@newwave-design.co.uk email: richard@newwave-design.co.uk website: http://www.newwave-design.co.uk version history: blank stylesheet created. -----------------------------------------------------------------------------*/ /* This is the global reset to allow fresh styling for each build. -----------------------------------------------------------------------------*/ html, body, div, span, applet, object, iframe, h1, h2, h3, h4, h5, h6, p, blockquote, pre, a, abbr, acronym, address, big, cite, code, del, dfn, em, font, img, ins, kbd, q, s, samp, small, strike, strong, sub, sup, tt, var, b, u, i, center, dl, dt, dd, ol, ul, li, fieldset, form, label, legend, table, caption, tbody, tfoot, thead, tr, th, td, .contentpaneopen, .contentheading { margin: 0; padding: 0; border: 0; outline: 0; font-size: 100%; outline:none; vertical-align:top; line-height:16px; } html, body { height:100%; } body { font-size: 11px; font-family: Arial, sans-serif; color: #808080; text-align:center; } #mainblock { position:relative; min-height:100%; } #wrapper { /* padding-bottom:156px; */ padding-bottom:166px; } #footer-wrapper { position:absolute; bottom: 0; } #wrapper { font-size: 11px; text-align:left; } /* HTML Tags -----------------------------------------------------------------------------*/ h1, .componentheading { font-size: 46px; color: #bfcc2a; line-height:46px; } h2 { font-size: 20px; } h3 { font-size: 18px; } #content-right h3 { font-size: 18px; color: #000; } h4 { font-size: 16px; } #content-right h4 { font-size: 14px; color: #000; } h5 { font-size: 14px; } h6 { font-size: 12px; } p { font-size: 11px; padding-bottom:6px; } a, a:visited { text-decoration:none; color:red; /* makes it pretty obvious to change */ } a:hover { color:aqua; /* makes it pretty obvious to change */ } ul, ol { padding-left:16px; } li { } dt { color:#000ff0; } dd { color: #ff0000; } legend { font-weight:bold; } input, textarea, select, optgroup { font-size:11px; font-family: Arial, sans-serif; } blockquote { font-family: "Times New Roman", serif; font-size: 16px; font-style: italic; color: #bfcc2a; padding-left:16px; padding-right: 16px; } /* Default Attributes -----------------------------------------------------------------------------*/ .clear { clear:both; } .right { float: right; } .left { float: left; } /* Header -----------------------------------------------------------------------------*/ #banner-wrapper { float:left; width:100%; background-image: url('../images/homepage-banner-background.png'); background-position: top center; background-repeat: repeat-x; } #banner { width:980px; margin-left:auto; margin-right:auto; height:403px; padding-top:16px; } /* Navigation -----------------------------------------------------------------------------*/ #header-wrapper { float:left; width:100%; background-color:#151515; } #header { width:948px; margin-left:auto; margin-right:auto; background-color:#151515; height:80px; } #logo { float:left; width:300px; height:80px; } #menu { float:left; width:648px; height:80px; } #mainmenu ul, #mainmenu li { float:left; display:inline; padding-left:0px; padding-right:24px; font-size: 16px; padding-top:16px; } #mainmenu a, #mainmenu a:visited { color: #fff; } #mainmenu #current, #mainmenu .active a, #mainmenu a:hover { color: #d7e62f !important; } #submenu ul, #submenu li { float:left; display: inline; padding-left:0px; } #submenu li a, #submenu li a:visited { float:left; padding-top: 6px; padding-bottom: 7px; padding-right:12px; padding-left:12px; border-top:4px solid #333; color: #666; } #submenu #active_menu, #submenu li a:hover { border-top:4px solid #87a41e; background-image: url('../images/active-submenu.png'); background-position: top center; background-repeat: no-repeat; color:#000; } #submenu-wrapper { float:left; width:100%; background-image: url('../images/submenu-bar.png'); background-repeat: repeat-x; background-position: bottom left; background-color: #333; } #submenu { width:948px; margin-left:auto; margin-right:auto; background-color:#333; } #submenu li { } /* Footer -----------------------------------------------------------------------------*/ #footer-wrapper { width:100%; background-color:#333; background-image: url('../images/footer-bar.png'); background-position: top center; background-repeat: repeat-x; text-align:left; padding-top: 7px; float:left; } #footer-main { width:948px; margin-left:auto; margin-right:auto; background-image: url('../images/footer-bar.png'); background-position: top center; background-repeat: repeat-x; margin-top: 7px; /*height: 216px;*/ } #footer-modules { width:948px; margin-left:auto; margin-right:auto; background-color:#333; height:182px; } #footer-modules table { margin-top:24px; } #footer-sub-wrapper { float:left; width:100%; background-color:#1a1a1a; color: #666; } #footer-sub { width:948px; background-color:#1a1a1a; margin-left:auto; margin-right:auto; padding-top:4px; height:22px; } #footer-sub a, #footer-sub a:visited { color: #666; } #footer-sub a:hover { color:#ccc; } #footer-sub-left { float:left; width:474px; height:22px; } #footer-sub-right { float:left; width:474px; height:22px; text-align: right; } /* Template q -----------------------------------------------------------------------------*/ #left-column { } #content-wrapper { float:left; width:100%; height:100% !important; } #content { width:948px; margin-left:auto; margin-right:auto; height:100%; padding-top:24px; } #content-left { float:left; padding-top:16px; padding-bottom:16px; } #content-left-narrow { padding-top:16px; padding-bottom:16px; padding-right:16px; float:left; height:100%; } #content-right { float:right; width: 236px; } #content-right div.moduletable { padding-left:28px; padding-top:16px; padding-bottom:16px; border-bottom:1px solid silver; } /* Homepage Modules -----------------------------------------------------------------------------*/ #homepage-module-main { width:948px; float:left; background-color:#bea; padding-left:16px; padding-right:16px; height:30px; } #homepage-sub-modules { float:left; width: 948px; padding-left:16px; padding-right:16px; height:30px; } /* Forms -----------------------------------------------------------------------------*/ input.text { padding: 3px; border: 1px solid #999999; } /* Tables -----------------------------------------------------------------------------*/ table { border-spacing: 0; border-collapse: collapse; } td { text-align: left; font-weight: normal; } table.homepage-table { margin-bottom:32px; } table.homepage-table td { padding-top:28px; padding-left:22px; padding-right: 22px; } /* Components CSS -----------------------------------------------------------------------------*/ #component-contact { display: inline-block; width: 674px !important; } .contact-cell { padding-bottom: 8px; } input, .contact-cell input, .contact-cell textarea { border: 1px solid silver; padding:6px; color: #a7b325; } .contact-cell input { width:206px; } .contact-cell textarea { width:456px; height:126px; } /* Referral Form -----------------------------------------------------------------------------*/ #referral_form { width:690px; }
NOTE - I have only done the first field, once I get that right I can do the rest
-
Excellent - did the trick!
Was part way there with putting the author id first. Thanks
-
Hi,
Got a wierd prob with a query string, and here it is:
SELECT bi.id, bi.author_id, bi.firstname, bi.secondname, bi.thirdname, bi.fourthname, bi.fifthname, bi.surname, bi.dates, bi.biography, bi.created, au.name AS author, au.id AS auth_id FROM jos_yearbook_biographies bi LEFT JOIN jos_yearbook_authors au ON bi.author_id = au.id WHERE bi.author_id = '1' AND bi.surname LIKE 'cuy%' OR bi.firstname LIKE 'cuy%' OR bi.secondname LIKE 'cuy%' OR bi.thirdname LIKE 'cuy%' OR bi.fourthname LIKE 'cuy%' OR bi.fifthname LIKE 'cuy%' OR bi.biography LIKE '%cuy%'
The problem is with the author_id - it seems to ignore it completely, and shows a result regardless. In the DB I've got two items, one with author_id 1, and the other with 2.
It shows the item with an author_id of two every time - I've checked the code, and it's sending 1 as seen in the statement.
I've also tried it using this statement:
SELECT bi.*, au.name AS author, au.id AS auth_id FROM #__yearbook_biographies bi LEFT JOIN #__yearbook_authors au ON bi.author_id = au.id WHERE bi.surname LIKE 'cuy%' OR bi.firstname LIKE 'cuy%' OR bi.secondname LIKE 'cuy%' OR bi.thirdname LIKE 'cuy%' OR bi.fourthname LIKE 'cuy%' OR bi.fifthname LIKE 'cuy%' OR bi.biography LIKE '%cuy%' AND bi.author_id = '1'
(I know one has jos_ and the other has #__ - I was testing the variant in phpmyadmin)
Any ideas why I always get items with author_id of '2'??? Puzzled.
-
As mentioned in my OP - "I have also used a global reset to zero off the extra paddings/margins that each browser decides is necessary, so I am working from the ground up with any elements on the page. " I have used the global reset for a number of sites, but it makes no difference.
Further research shows that even Google's buttons render in differing sizes depending on browser. Argh! I'll try the size thing, but I think I'm running out of options.
Display image preview - file input incorrect...
in Javascript Help
Posted
Hi,
Having a little difficulty with some JS to preview a local image:
And this is run with an input type="file" :
Which *should* display here:
Problem is, the JS fires, but the input tag strips the file location from :
C:/Documents and Settings/<user>/My Pictures/image.jpg
to
image.jpg
Which results in a broken image link because there is no image.jpg... Any ideas how/why? I've tried 3 different scripts and it seems that they all do the same.