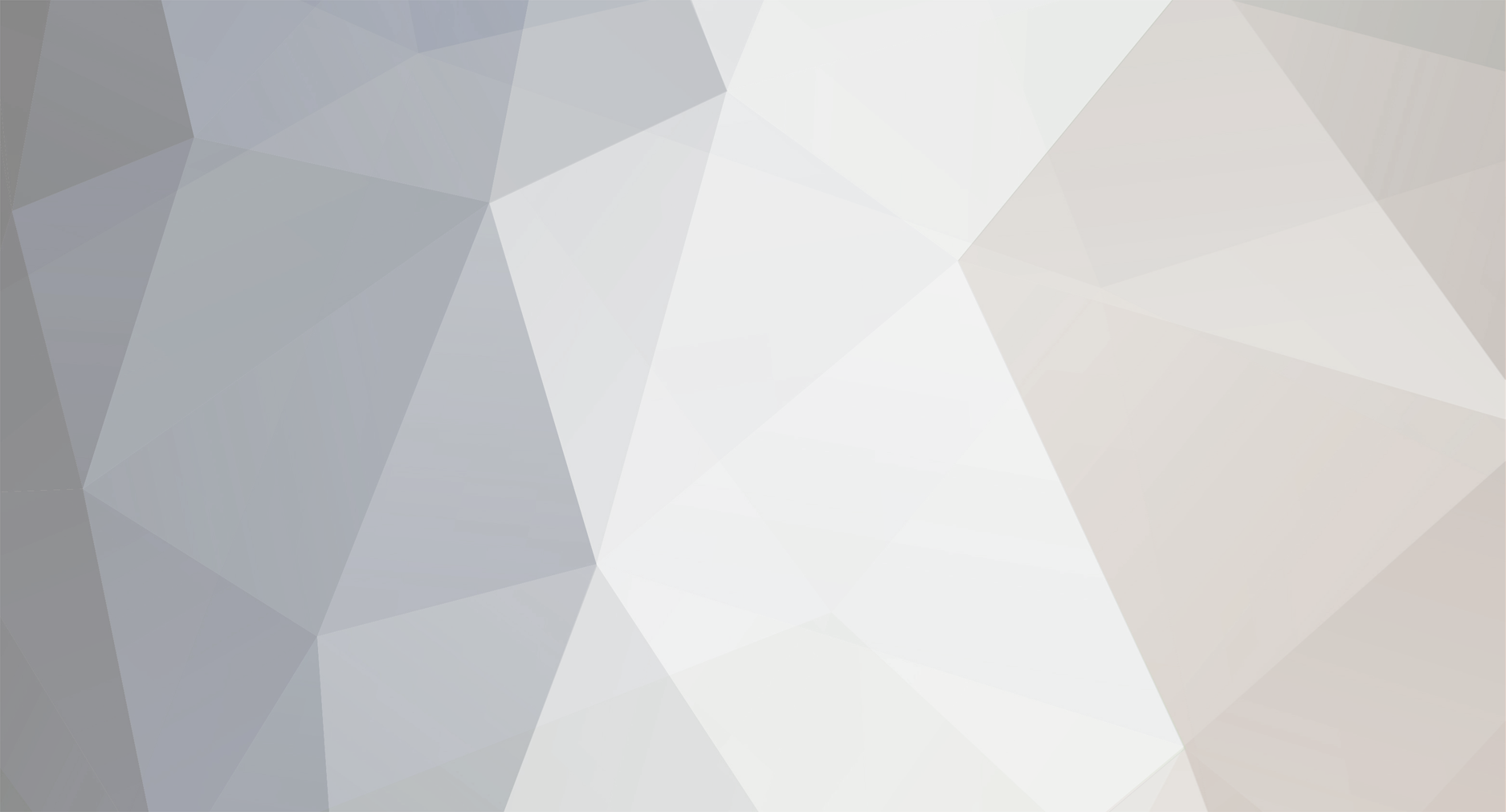
jcanker
Members-
Posts
252 -
Joined
-
Last visited
Everything posted by jcanker
-
There's not enough code here to be sure, because for starters I'm not sure why you've initialized your for loop the way you have. Why start $i at 2? what is $n? That aside, it probably doesn't have anything directly to do with the error you're seeing. Is the line $laagstegetal = $tabel[$m-1][3]; The only spot where you initalize $laagstegetal? Did you retype this code into the forum window or did you copy/paste? A cause of your issue isn't jumping out at me, but if you have retyped it rather than copy/paste, having $laagstegetal .= $tabel[$m-1][$i]; instead of: $laagstegetal = $tabel[$m-1][$i]; certainly would cause the issue you're seeing
-
Long story short: You can't use headers if you output ANYTHING first. That echo statement in the beginning is output. (And Pikachu2000 has helped me a million times on this board, it seems. Best to listen to his advice )
-
finding a value in an ASSOC using in_array()
jcanker replied to Eggzorcist's topic in PHP Coding Help
UPDATE: You can probably ignore most of the stuff I posted below. When I finished, I noticed that you're trying to find an ARRAY KEY, not value using in_array(). in_array() only looks at values, not keys. If you want to see if a key exists in an array, use array_key_exists($key,$array). I'm including the rest of what I had answered just in case (and because I spent the time to write it ) This is a function I have in an include file that greatly simplifies the task of parsing out the results of a returned MySQL query. function db_result_to_array($result) { $res_array = array(); for ($count=0; $row = @mysql_fetch_array($result); $count++) $res_array[$count] = $row; return $res_array; } Just run your query: PDO or using mysql_query($query) and whatever the returned results are (here I'll call it $result) $result = db_result_to_array($result); foreach ($result as $row) //this will parse through each row of the returned array, not each column { //this function allows you to access the returned row currently being processed by mysql column name, so you can run your in_array() here: if(in_array($mySearch, $row)){...} } That should work, although I've never used it this way. Because this is coming directly as a query from the DB, you should know which column would contain the value you're looking for. Just run an if statement on that. In the above example, the foreach would contain a line similar to: if($row['nameOfMysqlColumnThatWouldContainTheValueImLookingFor'] == $varNameThatHoldsTheValueImLookingFor){...} Seems to me that's much simpler than searching the array for a value when you know where it will be. NOTE: Also keep in mind that in_array() won't look through lower levels of a multidimentional array. You have to navigate to that level first and then search for it. You can do this in nested while or for loops with a few counters. -
PHP coding help? (using list menu selection to populate a text area)
jcanker replied to jgrant2012's topic in PHP Coding Help
The workflow process will be: Create a form with <select> elements with <options> for each exercise. The ACTION on that form will go to a php page which will grab the value of the selected OPTION when it was submitted. (Note: You CAN have the form submit to itself, e.g., the same php page. In this case, you start by looking for the _POST or _GET variables being set and processing them from the get-go, setting variables as appropriate throughout the rest of the page. This limits your total number of php pages in your site, but can get VERY messy without careful preplanning. ) That php page takes the value submitted by the form and runs the appropriate query, something like (a generic example) $submittedVariable = $_POST['exercise']; //don't forget to sanitize POST and GET, even though I leave that out for brevity here $query = "SELECT description FROM excercises WHERE exercise = '".$submittedVariable."' LIMIT 1; $result = mysql_query($query); //it's safer to use PDO, but that's another discussion entirely. This is the quick easy way to make the query if(!$result) { //use this for debugging purposes only--never reveal to users what your database structure is like. After you get it working properly replace this message with something more generic but specific enough that you know the error occurs in this spot, not at another location in your code echo "<p>Error retrieving the description. The query used was ".$query."<br/>The returned error was: ".mysql_errno().": ".mysql_error()."</p>"; } /* now process the returned query and grab the description, settting a variable with the description value. To make this quick and easy I use a function which I have in an include file: function db_result_to_array($result) { $res_array = array(); for ($count=0; $row = @mysql_fetch_array($result); $count++) $res_array[$count] = $row; return $res_array; } This function allows me to reference the specific MySQL column by column name when I process it: */ $result = db_result_to_aray($result); foreach($result as $row) { $desription = $row['description']; } /* Now, at this point you COULD give an AJAX feel to your page by setting the description in the SESSION array so the original page can access it: */ $_SESSION['description'] = $description; /* and then, if everything went smoothly, we haven't had any output to the screen, so that allows us to just redirect back to the original page. */ $referring_page = 'Location: http://'.$_SERVER['HTTP_REFERER']; //you may just want to hardcode in the page that has the original form because HTTP_REFERER is set by the browser and can't always be trusted header($referring_page); Now back on the page with the select form, at the beginning, you just look to see if $_SESSION['description'] is set. If it is, then use that text in the appropriate location in the page and then unset($_SESSION['description']); after you assign it to a variable. That way you're keeping it inside the if(isset($_SESSION['description'])) block. Once it's assigned to a variable you can use the var anywhere on the page you need to, and although you're using 2 separate PHP pages, the page just seems to refresh itself when the initial select form is submitted, showing the results and giving your page an AJAX feel without really using AJAX. I hope you can follow that...I know I ramble a bit in long explanations (and there may be a typo or two in the code, so use judgement on missing ) or ;'s etc The keyboard on my laptop isn't as responsive as it used to be and sometimes my fingers go faster than it wants to process -
PHP coding help? (using list menu selection to populate a text area)
jcanker replied to jgrant2012's topic in PHP Coding Help
What I think you are envisioning really is AJAX, not php. AJAX allows you to dynamically change the page based on user interaction by passing data to a backend server (usually php), getting data in response, and then updating an element of the webpage with the returned data (or changing it based on the response, such as logging in, etc). What you're trying to do with php can be done, but it will require multiple steps. Without AJAX, you'll have to submit the form data each step in order to change the content of other elements in the form. I''ll create an example in the next post, but I'm going to click submit so you know I'm working on this ATM -
There's a couple of ways to do it---100 ways to skin a cat, right? You can always use the UNIXtime and just do the math to add: 1 minute = 60 seconds 1 hour = 60min*60sec = 3600 seconds 1 day = 24*60min*60 sec = 86400 seconds 1 Week =7*24*60*60 = 604800 seconds But the caveat to that is unless you do a little work to it first, the UNIXdate is a TIMESTAMP, so it returns the current time, so the math will be from the current time, not 00:00 of the date. The other option, and one that is becoming more popular, is to use the PHP date/time object (which also has procedural style capabilites). This is newer, so if you're running on 4.x you'll have to upgrade to 5.x. Object oriented style: <?php $date = new DateTime('2000-01-01'); $date->add(new DateInterval('P10D')); echo $date->format('Y-m-d') . "\n"; ?> Procedural style: <?php $date = date_create('2000-01-01'); date_add($date, date_interval_create_from_date_string('10 days')); echo date_format($date, 'Y-m-d'); ?> Both result in the same output PHP manual page: http://www.php.net/manual/en/datetime.add.php
-
Require allow_url_include to be enabled on the client server by way of your license agreement. Be sure to log connections in your own db and check periodically which clients are not showing up there. Don't forget to include in your license agreement that they don't have the right to alter the code. Use a require_once to a file on your webserver Inside that require_once create your check and create an obtuse $_SESSION variable to hold the logged status. For good measure, include an important function in that include which would otherwise be in the user's local php page. This will prevent them from just commenting/deleting your require_once. Between those, you won't make it locked perfectly, but you can obfusicate it enough to prevent all but the most intent users. Double checking who *should* be dialing home to your DB and who isn't would help identify abusers.
-
The easy fix is to not try to carry this function out inside an echo statement--just do the math, assign the result to a variable and put the variable in the echo statement. OR you should be able to break the echo quotes to do the math and then concat the rest of the echo: Note: When I copied this line to a code block I noticed the parenthesis are in the wrong place to boot--that *might* fix it, but I'd still do it this way to avoid mixing stuff up too much.. $("<?php echo "#".$hours_difference; ?>").val(". round(data.hours + data.minutes/60 - 0.5, 2)." ); You had a closing paren between .05, & 2.
-
What output are currently getting? Is the issue that you don't have control over the length? You're mixing up php inside of jQuery, and that's always been messier than I like to deal with. php and javascript handle round in different fashions. Javascript's Math.round doesn't let you specify how many digits you want after the decimal. In order to achieve that you have to use math inside your Math.round equation: var result=Math.round(original*10)/10 return includes one decimal place
-
You're right, I did Rushed it without proofreading. Thanks for the catch.
-
No, your form has to be processed by php. Which php page processes this form is determined by the ACTION attribute of your web form. User clicks submit, and the form data is submitted to the php page designated in the ACTION attribute. That php page gets the $_POST or $_GET data (depending on which method you set in the form) as an array. From that point it's easy to access it. In your code you named the input block "name" so it will now be in the $_POST under the key name. We access it by: $_POST['name'] $myvariable = $_POST['name'] So, if all you have to do is get the value entered by the user in that form and use that to complete the URL you will send them to, it's as simple as <?php $url = "http://ww.mywebsite.com/wp-content/uploads/".$_POST['name'].".jpg"; header($url); That will take them to the page to process the form when they click submit and then redirect them to the specified jpg file, which will cause the save/download prompt. Consider here that at that point you've stranded your user 2 pages away from any legitimate content of you page since you sent them directly to a file. If they click the back button, the first back button click will revert to the redirect page, sending them back to the jpg, etc. You should always redirect to another page, not a file. Of course, I have to issue the standard warning that you should NEVER trust user input (do a google search for Bobby Tables) so you should at least sanitize the $_POST data before you access what they put in the form. I'm not sure what this project is, but it sounds awfully quirky to have them be directed to a .jpg file based on what they type in a form. How will they know the filename exists? It may be inconsequental to your question, per se, and I may be wrong (wouldn't be the first time) but I have to caution that this question stinks to high heaven of trying to do something that php wasn't intended for and/or using the wrong workflow to achieve the end result you want.
-
It's been a while since I've actually done this in PHP. (It's actually easier to do with AJAX, but if you're just learning PHP then AJAX will make your head spin ) I'm assuing you're having php build the web form dynamically based on what is in the shopping cart and that the form elements aren't hard coded? You can always pass the Itemnum as the VALUE of the checkbox. If the box is checked, remove that item from their list. It still goes back to rethinking how your arrays work. Don't forget that $_GET and $_POST are arrays themselves, so assuming you've taken the proper precautions such as sanitizing your data (or even better, used prepared statements!), you can work directly with POST or GET data without recasting into your own new array.
-
Psycho's right (and that's not the first time I've typed that sentence ) That section of the code is far too convoluted and you don't do any error checking, so you dont' know what's what. Make LIBERAL use of print_r or echo throughout your code. It's real easy to comment out when you're done and delete them when everything's tested as working. Without that feedback, you can't see why it thinks your count is higher than 5 (which is another issue--in your description you said 2 messages a day, but your code says 5.) And that whole section which Psycho pointed out is too much code--and slower code at that. The PHP manual points out that mysql_result is slower that the corresponding mysql_fetch_array and related functions. That whole section is as simple as $query = "SELECT * FROM `CysticAirwaves` WHERE `FromUserID` = $auth->id AND date = `CURDATE()`"; $result = mysql_query($query); if(!$result){echo "<br/><span style="color:red">Whoops! We couldn't run the query ".$query."! The SQL error is: ".mysql_errorno()." : ".mysql_error()."</span>;} else{ $post_count = mysql_num_rows($result); echo "<br/>TEST RESULT: post_count is: $post_count"; } But your code would be fine if you just ran it once instead of 2x. Add in some error checking and troubleshooting feedback. That will help you narrow down what's going on.
-
You can't do this directly in PHP, but you can do this in AJAX, which would get the value of the input element named "name" (with an id "name" to be more specific...) Now that I think about this, tho, you CAN do this in PHP using header on the php page that is processing the form after user clicks submit. Make the form action go to the page to process the form, then redirect from there header('Location: http://www.example.com/'); Just alter that so that your URL is properly formed instead of the example.com. One word of warning can't can't be said strongly enough--header() will NOT WORK if you output anything before you call it. You can process all you want, create variables, assign values, etc, but you cannot output anything before you call header()
-
Psycho's right--If I understand what you're trying to do with this array you're tackling it from the completely wrong angle. You're trying to do too much here with one array--it product desc, etc should be in another array, but if you want to pass it along in this array for easier parsing later on, that's fine. Think in terms of each item as the head element, and what you have currently as top-level elements should be sub elements of the rest. [0]->array [itemID]->ID of the item being ordered [desc]->Description of the item [price]->Price of this item ...etc for each item [1]->array [itemID]->ID of the item being ordered [desc]->Description of the item [price]->Price of this item ...etc for each item [2]->array {...} and so on. A user wants the 2nd item deleted? No problem unset($Myitems[1])
-
$_GET is an array-that's why you can access values by key name inside square brackets. foreach($_GET as $key => $value) {...} [\code] Although I should point out that at the beginning of this thread you were using $_POST, not $_GET
-
Never mind... I didn't change the name of $row so...... Changing it to $rowID makes it work like a charm Maybe I should go take a break from this project for a little while...
-
Nope, not between. Anything you want to run on everything should go before or after the if/elseif strings, not between them. Good luck
-
I'm assuming this is javascript at the beginning? You're missing the type="text/javascript" attribute inside that script tag. Not sure this will make a difference, but it can't hurt to have the browser know how to interpret the code Where exactly is the problem occurring? Just on the echo at the end? The problem doesn't seem to be on the PHP side but the javascript side since the amtdue and balance are calculated based on what is typed in for amount paid. All that happens before PHP gets hold of anything.
-
You are running other statements after the preceding if but before you use elseif. elseif must come immediately after the close of the preceding if: if(condition1) { echo "foo"; }//end of if elseif(condition2) { echo "bar"; } You have something like: if(condition1) { echo "foo"; }//end of if echo "I have some stuff here after if but before elseif--I can't use elseif after this! elseif(condition2) { echo "bar"; }
-
I'm writing PHP for my son's cub scout pack. It populates the MySQL db users table from data contained in a csv file. So far, it puts the scout's info in just fine...until I try to run another query as a nested query to retrieve the auto-generated userID for the scout that was just placed in the DB. The result of this if I don't include the second query--the one trying to get the new userID for that scout, it iterates through the whole file as expected and puts all the scouts in the users table. The second I uncomment the 2nd query and try to get it to run, it only puts the 1st scout in the DB, properly retrieves the newly generated userID for him, and then stops. I need to have this userID for the scout to properly associate that scout with the parents later on and in other sections of this project. the db_connect() function just creates the PDO object and contains the username, etc for the connection. That part is working fine so I'm not posting that function here. I've tried renaming the connection, query, etc to add "ID" to the end to ensure I wasn't trampling on var names, but that hasn't made a difference //include necessary files: include_once("../../sys/php/includes/data_fns.php"); //start session session_start(); $conn = db_connect(); $bufferConn = $conn->setAttribute(PDO::MYSQL_ATTR_USE_BUFFERED_QUERY, true); //////////////////////////////////////// // Read through the .csv file and populate the users table /* Uses the model from the php documentation on fgetcsv: * $row = 1; if (($handle = fopen("test.csv", "r")) !== FALSE) { while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { $num = count($data); echo "<p> $num fields in line $row: <br /></p>\n"; $row++; for ($c=0; $c < $num; $c++) { echo $data[$c] . "<br />\n"; } } fclose($handle); } */ //////////////////////////////////////// $row = 0; //set the row counter $table1 = "<table id='csvTable'>"; //start the table HTML markup if(($handle= fopen("../../sys/source/pack238.csv", "r"))!== FALSE) //open the csv file { while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) //fgetcsv reads through the csv file line by line { //ignore the first row which has the header data--we don't want that in the database if($row==0){$row++; continue;} $num = count($data); //get the scout's info, check to see if he's already in the db, then put it in if not--the scout should NOT already be in db when we run this for the first time, but later versions will only add new scouts. For now, just alert us if they're already in and halt //TODO Figure out why this still runs if the table name doesn't exist!! $query = "SELECT * FROM users WHERE fName=:firstName && lName=:lastName"; $stmt = $conn->prepare($query); $stmt->execute(array(':firstName'=>$data[1], ':lastName'=>$data[0])); $dbCount=0; while ($stmt->fetch()){$dbCount++;} if($dbCount >0) //we found this scout--he shouldn't be in the DB. Halt the process and check what's going on { echo "<br/>The scout, $data[1] $data[0] was found in the database already. We cannot continue. <b/>Please check this duplicate data and try running this setup again"; die(); } else //he's not in the DB....put him in there { //get the Den unit as a number, dropping "Den" $den = substr($data[10],4); //get birthday as something to be used as PHP date object if($data[7]!="") { $bdayO = date_create($data[7]); $now = date_create("now"); $interval = date_diff($bdayO, $now); $age = $interval->format('%y years'); $bday = $bdayO->format('Y-m-d'); } else{ $bdayO = NULL; $bday = NULL; $age = NULL; } echo "<br/> Test message: The scout $data[1] $data[0], Den:$den would be put into the database! His bday is:$bday. AGE IS: $age"; $query = "insert into users (gen,fName,lName, nName,memberOf,bday) VALUES ('M',:firstName,:lastName,:nickName,:den,:bday)"; $stmt= $conn->prepare($query); $stmt->execute(array(':firstName'=>$data[1], ':lastName'=>$data[0], ':nickName'=>$data[3], ':den'=>$den, ':bday'=>$bday)); //get the new userID for this scout now that he's in the db $connID = db_connect(); $queryID = "SELECT userID from users WHERE fName=:firstName && lName=:lastName"; $stmtID = $connID->prepare($queryID); $stmtID->execute(array(':firstName'=>$data[1], ':lastName'=>$data[0])); while ($row = $stmtID->fetch()) { $scoutUserID = $row[0]; echo "<br/>This scout's userID is: $scoutUserID"; } }//end else //get the father's info, check to see if he's already in the db, then put it in if not //if he is in the db, get this scout's userID number and add it to the father's children array $query = "SELECT * FROM users WHERE fName=:firstName && lName=:lastName"; $stmt = $conn->prepare($query); $stmt->execute(array(':firstName'=>$data[18], ':lastName'=>$data[19])); $dbCount=0; while ($stmt->fetch()){$dbCount++;} if($dbCount >0) //This father is already in the DB...we need to add this scout to his children array { echo "<br/>For testing purposes, this father is already in the DB...let's add this scout, ".$data[1]." to his children array"; //get the userID of the current scout } else //he's not in the DB....put him in there {} //get the mother's info, check to see if he's already in the db, then put it in if not //if she is in the db, get this scout's userID number and add it ot the mother's children array }// end while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) }//end if(($handle= fopen("../../sys/source/pack238.csv", "r"))!== FALSE)
-
I have an associative array that is serialized and stored in a MySQL DB. The array is a duty roster for my son's Cub Scout camping trip (adult volunteers for specific jobs). When a user clicks a spot on the jQuery/client side, it sends it to my php page via ajax, which should pull up the array from the DB, unserialize it, replace the value "Available" with the person's name for the date/time/job that they clicked on. For ease of processing, I need to reference the value to replace by numerical indexes rather than the associative key string-type name, but when I try it, it just says Undefined offset. I thought I could access key/values using the numerical index in square brackets, just as I could if it were a non-associative array. What gives? (You can see near the end where I tried to access the array by index; this now-commented out line just tacked it on at the end, like it was recognizing the digits as strings instead of integers. I've tried to cast the values as integers before putting them in brackets, but same effect. // get the duty roster array from the database $query = "select dutyroster from ".$eventsTable." where eventID = 1"; $result = mysql_query($query); if(!$result) { } else { //put the returned result into a usable array $returnedRows = mysql_fetch_array($result,MYSQL_BOTH); $unserializedArray = unserialize($returnedRows[0]); } //get the returned values for ID, email, and fillerValue $ID = "0-0-2-2";//$_POST['ID']; $email = "[email protected]";//$_POST['email']; $fillerValue = "Dude (test)";//$_POST['fillerValue']; // parse $ID to break out the array index & set them as variables $explodedArray = explode("-",$ID); $iDate = (int)$explodedArray[0]; $iTimeblock = (int)$explodedArray[1]; $iJob = (int)$explodedArray[2]; $iSlot = (int)$explodedArray[3]; echo "<br/> the values are: idate: ".$iDate." and iTimeblock: ".$iTimeblock." and iJob: ".$iJob." and iSlot: ".$iSlot."</br>"; echo "<BR/> the random selected value to display is: ".$unserializedArray[0]; //$unserializedArray[$iDate][$iTimeblock][$iJob][$iSlot] = $fillerValue; print_r($unserializedArray);
-
Simple XML just outputing values of inner-most elements
jcanker replied to jcanker's topic in PHP Coding Help
OK, nevermind, I guess.... The echo statements and the HR threw a "only one top level error" message when I followed the link I posted... But even if I click refresh on the page I had been using, it still doesn't show properly....same browser, different tabs...hrm.... Anywhoo.... Is there a more recursive way to step through the levels of the array and process it? -
I'm working on a way to let parents in my son's cub scout pack volunteer for specific spots in a duty roster via an AJAX page and MySQL db. The duty roster is saved as an array in the db. My intent is to pull out the array, parse it into XML to send back to the jQuery which asked for it. The end result right now is that it just displays a bunch of "available" strings in a row, which is the default value for a job slot which hasn't been filled yet. The xml should look something like: <data> <DAY val="DAYVALUE"> <TIMEBLOCK val ="TIMEVALUE"> <JOB title="titlevalue"> <slot num="x">AVAILABLE or NAME</slot> <slot num="x">AVAILABLE or NAME</slot> {...} </JOB> </timeblock> </DAY> <data> Where the attribute changes to reflect the different days for the campout, and the TIMEBLOCK val attribute changes for the different timeblocks which have job slots (Breakfast, Lunch, etc). Here is the snippet of code. The page starts with Header('Content-type: text/xml'); before making the call to the db (I've left that section out for security). The part where it gets retrieves and traverses the array are working as expected. It's where the XML gets built that everything goes wonky. (But if someone can show me a more recursive, efficient way of getting the same effect, I'm all ears) The output of this page shows up at:http://www.pack238.net/campingregistration/retrievedutyroster.php You'll see at the bottom where it just says "available available available available available available available available...." The code is: //start the xml $xml = new SimpleXMLElement('<data></data>'); // get the duty roster array from the database $query = "select dutyroster from ".$eventsTable." where eventID = 1"; $result = mysql_query($query); if(!$result) { } else{ //put the returned result into a usable array $returnedRows = mysql_fetch_array($result); $day = unserialize($returnedRows[0]); foreach($day as $key => $value) //this gives us each day { echo "<Br/> Key is: $key && the value is $value"; $day1 = $xml->addChild('DAY'); $dayValue = $day1->addAttribute('val',$key); //Here, we know we're at the top level, so parse out the sub arrays foreach($value as $key1 => $value1)//this gives us each time block { $timeblock = ""; $timeblockValue = ""; $timeblock = $day1->addChild('TIMEBLOCK'); $timeblockValue = $timeblock->addAttribute('val',$key1); echo "<br/> Let's see the time blocks for each day: $key1 $value1"; //check to see if there's a JOBS sub array yet again or if it's a standalone if(is_array($value1)) { foreach($value1 as $key2 => $value2) { $job = ""; $title = ""; $job = $timeblock->addChild('JOB'); // $title = $timeblock->addAttribute('title',$key2); //this is the line throwing the attribute error echo "<br/> We're in a sub array, and the values are: $key2 $value2"; if(is_array($value2)) { foreach($value2 as $key3 => $value3) { //for each slot: $slot = $job ->addChild('slot',$value3); $slotnum = $slot ->addAttribute('num',$key3); echo "<br/> The jobs are: $key3 $value3"; } } else //we need to account for individual jobs that don't have a subarray { $slot = $job ->addChild('slot',$value3); $slotnum = $slot -> addAttribute('num','0'); } } } } // echo "<br/><br/>The array returned is: ".$usableArray."<br/>"; // $usableArray = unserialize($usableArray); // print_r($usableArray); }//end of the main foreach //we got the duty roster array....now just parse out the days, time periods, and jobs then create the xml $dom = dom_import_simplexml($xml)->ownerDocument; $dom->formatOutput = true; echo $dom->saveXML(); echo "<hr/>"; //print_r($xml); }//end of else to if(!result)
-
That would be a more elegant solution, but I need the host headers to be configured for IIS to send the user to the right defined website in the first place. This is sitting on an IIS server that is hosting multiple sites beyond this one, so I can't just have all http requests sent to one directory for PHP to get the HTTP_HOST. The issue isn't getting the host URL into PHP so much as it is creating, on-the-fly (e.g. minimal interaction from me), the ability for a cub scout troop to tell the system, "We have a registered domain, we want to point it to the dynamically created template site that uses the DB backend, and we'll point the DNS A record here. The domain is "www.mypack333.net" Unless I'm missing something, when a user types www.mypack333.net and the registrar's DNS server points it my way, IIS won't know what to do with it unless one of the configured websites has a host header for that domain, right?