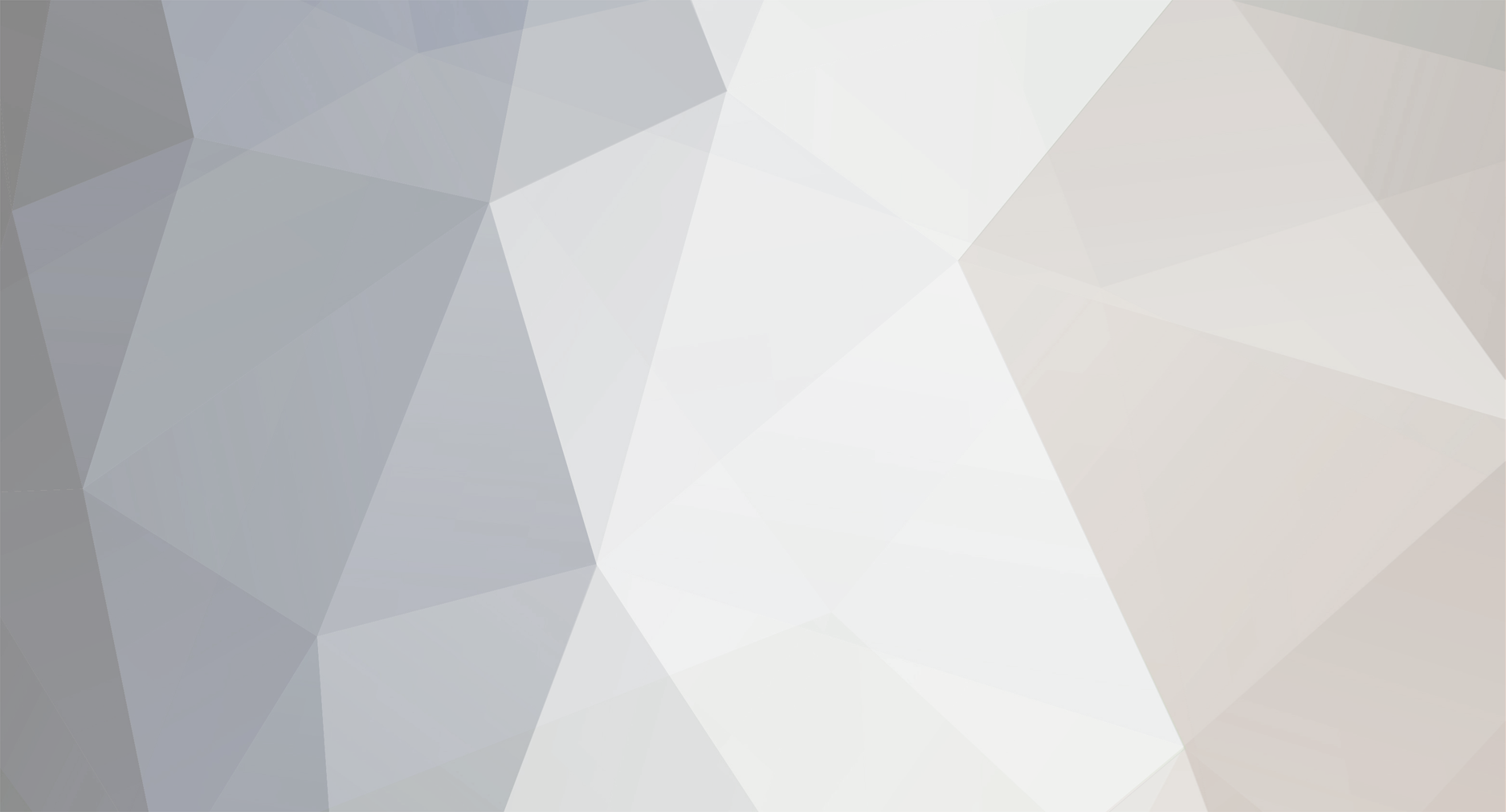
jcanker
Members-
Posts
252 -
Joined
-
Last visited
Everything posted by jcanker
-
For the record for anyone who sees this post-humously, my suspicions about scope were correct. Changing references of $output to a session variable, $_SESSION['output'] solved the problem. It's been a long night and I'm probably missing something, but I'm still not certain why scope affecting it when $output is initialized outside the function and passed to it, even when it's called recursively to get to the child elements.
-
How Can Site Get Hacked If You Protect All Get And Post Inputs ?
jcanker replied to Eritrea's topic in Application Design
Because mysql_real_escape_string is a good step toward sanitizing input, but it doesn't go far enough. You really want to use prepared statements to protect against these: http://www.dreamincode.net/forums/blog/1735/entry-3958-why-mysql-real-escape-string-isnt-enough/ Additionally, it's not always about hacking per se as much as it is about getting information and causing chaos from there. If, for instance, variables are passed through the URL using GET, then I can see what variables are being named and see the logic behind your variable/value structure. Consider, for instance, a page that lets me view my account settings. I'm already authenticated by the login procedure. I go to my view account settings page and see the url: www.generalsite.com/view_account_settings.php?user=59293222 What happens if I start experimenting with random user numbers? Is the code set up to check that the user ID passed is actually the same as the one logged in, or was the programmer lazy and just assumed that a user wouldn't ever try to pass their own values through the URL? What if your change password page uses the same URL structure and doesn't ask for the old password? It would be real easy for a user to change account passwords. Sanitizing input won't stop any of those attempts. Only good planning and responsible coding/design will.- 7 replies
-
- php
- sql-injection
-
(and 2 more)
Tagged with:
-
I'm working on some code for my son's cub scout pack to track the boy's progress toward their rank requirements. I've successfully gotten the code to build an array from the database to show the relationships between requirements & subrequirements. As a next step, I want to step through the array and display them in a user-friendly manner. The top-level requirements (elements-numbered 1, 2, 3, etc) are added to the list fine. As it steps through the child requirements (labeled as a,b,c, etc), they seem to be added to $output as expected and appear as part of $output when echoed to the screen. However, when the foreach loop goes through another iteration, all the child-level requirements are dropped off from $output rather than have the new top-level appended to the output. My suspicion is that it has something to do with scope, but I can't understand why it says $output = 1,2,3,4,5,6,7abc then suddenly it says $output = 1,2,3,4,5,6,7,8. What am I missing? Sample echo output that I created for testing shows (I edited the output to show requirement 7a,b,&c, which is the first spot where this scenario occurs because the earlier requirements don't have children: The temporary output build at this point is: c -- Explain and agree to follow the Outdoor Code The current output at this point is: Do these 1 -- Have an adult member of your family read the Weblos Scout Parent Guide (pages 1-22) and sign here 2 -- Be an active member of your Webelos den for three months (Active) means having good attendance, payong your den dues, and working on den projects.) 3 -- Know and explain the meaning of the Webelos badge 4 -- Point out the trhee special parts of the Webelos Scout unifrm. Tell when to wear the uniform and when not to wear it. 5 -- Earn the Fitness and Citizen activity badges and one other ativity badge from a different activity group 6 -- Plan and lead a flag ceremony in your den that includes the U.S. flag. 7 -- Show that you know and understand the requirements to be a Boy Scout a -- Demonstrate the Scout sale, Scout sign, and Scout handshake. Explain when you would use them. b -- Explain the Scout Oath, Scout Law, Scout motto, and Scout slogan. c -- Explain and agree to follow the Outdoor Code %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%% We're starting a new run through the foreach loop and as we start it, output is set to: Do these 1 -- Have an adult member of your family read the Weblos Scout Parent Guide (pages 1-22) and sign here 2 -- Be an active member of your Webelos den for three months (Active) means having good attendance, payong your den dues, and working on den projects.) 3 -- Know and explain the meaning of the Webelos badge 4 -- Point out the trhee special parts of the Webelos Scout unifrm. Tell when to wear the uniform and when not to wear it. 5 -- Earn the Fitness and Citizen activity badges and one other ativity badge from a different activity group 6 -- Plan and lead a flag ceremony in your den that includes the U.S. flag. 7 -- Show that you know and understand the requirements to be a Boy Scout And the thisOutput is set to: 7 -- Show that you know and understand the requirements to be a Boy Scout ---/////---/////---/////---/////---/////---/////---/////---/////---/////---/////---/////---///// The temporary output build at this point is: 8 -- Faith And the relevant code is as follows: //initialize output $output = "<div>"; //step through the array and create OL/LI where appropriate //$thisArray = $testArray; function listRequirements($thisArray, $output) { echo "<br/>^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^<br/>"; echo "<br/>We're starting listRequirements and as we start it, output is set to:<br/>".$output."<br/>"; foreach($thisArray as $key => $value) { echo "<br/>%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%<br/>"; echo "<br/>We're starting a new run through the foreach loop and as we start it, output is set to:<br/>".$output."<br/>"; echo "<br/><br/>And the thisOutput is set to: ".$thisOutput."<br/>"; $thisOutput = ""; //see if this requirement has a name and include it if so if(array_key_exists("reqName", $thisArray[$key])) { $thisOutput .= "<div>".$thisArray[$key]['reqName']; $closeDiv = 1; } if(array_key_exists("reqText", $thisArray[$key])) { if(isset($closeDiv) && $closeDiv == 1) {$thisOutput .= " -- ".$thisArray[$key]['reqText'];} else{ $thisOutput .= "<div>".$thisArray[$key]['reqText']; $closeDiv = 1; } } if(isset($closeDiv) && $closeDiv == 1) {$thisOutput .= "</div>";} $closeDiv = 0; echo "<br/>---/////---/////---/////---/////---/////---/////---/////---/////---/////---/////---/////---/////<br/>"; echo "<br/>The temporary output build at this point is: <br/>".$thisOutput; $output .= $thisOutput; //echo "<br/>..............................................................<br/>"; echo "<br/>The current output at this point is: ".$output."<br/>"; //see if there are any children for this element if(array_key_exists("children", $thisArray[$key])) { echo "<br/>=================================================<br/>"; echo "<br/>We're entering the children loop, and we're passing the output: ".$output."<br/>"; $result = listRequirements($thisArray[$key]['children'], $output); } }//end foreach ($testARray as $key=> $value) //echo "<br/>After going through all the keys, the output is:<br/>".$output; // return $output; }// end function listRequirements($thisArray) $result = listRequirements($testArray, $output); For the record, the array that it's pulling from is: Array ( [1] => Array ( [reqText] => Do these [children] => Array ( [2] => Array ( [reqName] => 1 [reqText] => Have an adult member of your family read the Weblos Scout Parent Guide (pages 1-22) and sign here ) [3] => Array ( [reqName] => 2 [reqText] => Be an active member of your Webelos den for three months (Active) means having good attendance, payong your den dues, and working on den projects.) ) [4] => Array ( [reqName] => 3 [reqText] => Know and explain the meaning of the Webelos badge ) [5] => Array ( [reqName] => 4 [reqText] => Point out the trhee special parts of the Webelos Scout unifrm. Tell when to wear the uniform and when not to wear it. ) [6] => Array ( [reqName] => 5 [reqText] => Earn the Fitness and Citizen activity badges and one other ativity badge from a different activity group [children] => Array ( [7] => Array ( [reqAwardID] => 2 ) [8] => Array ( [reqAwardID] => 3 ) [9] => Array ( [awardNum] => 1 [awardGroupID] => Array ( [0] => 2 [1] => 4 [2] => 5 ) ) ) ) [10] => Array ( [reqName] => 6 [reqText] => Plan and lead a flag ceremony in your den that includes the U.S. flag. ) [11] => Array ( [reqName] => 7 [reqText] => Show that you know and understand the requirements to be a Boy Scout [children] => Array ( [12] => Array ( [reqName] => a [reqText] => Demonstrate the Scout sale, Scout sign, and Scout handshake. Explain when you would use them. ) [13] => Array ( [reqName] => b [reqText] => Explain the Scout Oath, Scout Law, Scout motto, and Scout slogan. ) [14] => Array ( [reqName] => c [reqText] => Explain and agree to follow the Outdoor Code ) ) ) [15] => Array ( [reqName] => 8 [reqText] => Faith [children] => Array ( [16] => Array ( [reqText] => After completing the rest of requirement 8, do these (a,b, and c) [children] => Array ( [17] => Array ( [reqName] => a [reqText] => Know: Tell what yo have learned about faith ) [18] => Array ( [reqName] => b [reqText] => Commit: Tell how these faith experiences help you live your duty to God. name one faith ractice you will continue to do in the future. ) [19] => Array ( [reqName] => c [reqText] => Practice: After doing these equirements, tell what you have learned about your beliefs ) ) ) [20] => Array ( [qtyCompReq] => 1 [reqText] => And do one of these (d OR e) [children] => Array ( [21] => Array ( [reqName] => d [reqText] => Earn the religios emblem of your faith [footnote] => Footnote text [footnoteMarker] => * ) [22] => Array ( [reqName] => e [qtyCompReq] => 2 [reqText] => Do two of these: [children] => Array ( [23] => Array ( [reqName] => * [reqText] => Attend the mosque, church, synagogue, temple, or other religious organization of your choice, talk with your religious leader aboutyour beliefs. Tell your family and your Webelos den leader what you learned. ) [24] => Array ( [reqName] => * [reqText] => Discuss with your family and Webelos den leader how your religious beliefs fit in with the cout Oath and Scout Law, and what character-building traits your religious beliefs hve in common with the Scout Oath and Scout Law. ) [25] => Array ( [reqName] => * [reqText] => With your religious leader, disuss and make a plan to do two things you think will help you draw nearer to God. Do these things for amonth. ) [26] => Array ( [reqName] => * [reqText] => For at least a month, pray or meditate reverently each day as taught by your family, and by your church, temple, mosque, synagogue ,or religious group. ) [27] => Array ( [reqName] => * [reqText] => Underthe direction ofyour religious leader, do an act of service for someone else. Talk about your service with your family and Webelos den leader. Tell them how it made you feel. ) [28] => Array ( [reqName] => * [reqText] => List at least two ways you blieve you have lived according to your religious beliefs ) ) ) ) ) ) ) ) ) )
-
Uh....it's not my function. It's the originally posted function with the array passed to it when it's called. The original posted question was about why he was getting an error, not how can he make the function more efficient. I'm taking some time to help people solve their error messages and to help people new to PHP become more familiar and comfortable with it. I'm not in the habit of randomly rewriting code when not asked to, and I'm certainly not in the habit of engaging in performance races and chest thumping.
-
sorry...should've paid closer attention when I looked at it the first time. You're right at that spot. Pass $numsarray in the function with $a. That works, however, you never actually add to the array because it is always length of 0 when it hits the if (count($numsarray) > 0) check, so it never goes into it to get values added to the end. It jumps right to if($match == 0), which will always be true, so the array is empty. This works, but array is empty as noted <?php $numsarray=array(); function checkChores($numsarray,$a) { $match = 0; if (count($numsarray) > 0) { foreach ($numsarray as $chorenum) { if ($chorenum == $a) {$match = 1;} } } if ($match == 0) {array_push($numsarray, $a);} } checkChores($numsarray,3);checkChores($numsarray,4);checkChores($numsarray,3); print_r($numsarray); ?> demo of result at http://betaportal.ankertechnicalservices.com/arraytest.php
-
use square brackets instead of () for the index number
-
This code specifically does what you asked way back in the beginning: Counts the number of times in each year and reports 4. (example at same url as above post) $totalCounter = 0; foreach($arr as $key0 => $val0) { $timesCount = 0; echo $key0."<br/>"; foreach($arr[$key0] as $key1 => $val1) { echo $key1."<br/>"; foreach($arr[$key0][$key1] as $key2 => $val2) { $totalCounter++; $timesCount++; } } echo "<br/>timesCount for $key0 is: $timesCount<br/>"; } echo "<br/>TotalCount is: ".$totalCounter;
-
Here's what works to get the count of total articles (you can adapt it as necesary to get other elements...) My example wasn't working because in the jump from theoretical example to your specific array I failed to account for my example using index base, whereas your real example is associative. The code below works with associative arrays: (and here you won't need the $i or $k counters...they're just left in from the previous version and should come out. ) <?php $arr = array( "2012" => array( "January" => array( "10" => array("16:11:45"), "24" => array("12:14:16") ), "February" => array( "14" => array("11:12:43"), "17" => array("19:27:11") ) ), "2011" => array( "May" => array( "2" => array("12:13:14"), "29" => array("01:02:56") ), "August" => array( "1" => array("08:45:01"), "9" => array("23:11:24") ) ) ); $totalCounter = 0; $i =2012; foreach($arr as $key0 => $val0) { $k = 0; echo $key0."<br/>"; foreach($arr[$key0] as $key1 => $val1) { $l = 0; echo $key1."<br/>"; foreach($arr[$key0][$key1] as $key2 => $val2) { $totalCounter++; } $k++; } $i = $i-1; } echo "<br/>TotalCount is: ".$totalCounter; ?> [code=php:0] here's the script in action, counting 8 total articles: http://betaportal.ankertechnicalservices.com/arraytest.php
-
Noticed an error in my code--I forgot to put in the individual counters; it's corrected in this example Just noticed that you're trying to get, for instance, a total count of entries for the year, so you'll need to count the length of . Given the array layout you made in the last post, you can either just increase a total counter (probably easier) or set counters at each level and use that counter to access the value of specific elements. Doing something like $totalCounter = 0; $i =0; foreach($myArray as $key0 => $val0) { $k = 0; echo $key0 ,"&".$val0."<br/>"; foreach($myArray[$i] as $key1 => $val1) { $l = 0; echo $key1 ,"&".$val1."<br/>"; foreach($myArray[$i][$k] as $key2 => $val2) { $totalCounter++; } $k++; } $i++; } by the time you get done, $totalCounter should be the total number of articles
-
Think of a multidimentional array as an array inside an array key. The important part to remember here is that each KEY in your "top level" elements each contain an array instead of a single value. That means you have multiple arrays on the 2nd level. Each KEY in the 2nd level can have an array in it, so again instead of a "3rd level" which can be counted in total, each KEY in the 2nd level has an array which can be counted. ( I hope this layout works when you view it) topLevelElement0 topLevelElement1 2ndLevel Element 0 2nd Level Elem 1 2ndLevelElem 0 2ndLevelElem 1 3rdLevelElem0 3rdLevelElem1 3rdLevelElem0 3rdLevelElem1 3rdLevelElem0 3rdLevelElem1 3rdLevelElem0 3rdLevelElem1 And so on. Remember that each level can have a different number of elements than its siblings although in the example I made each element have 2 sub elements. When you nest the foreach in my example and run count(), you'll get 2 in the above example, not 2, 4, or 8 respectively. You access a subelement by accessing the parent element and stepping through it's index, just as you would a single-dimensional array. To access the keys and values in a single-dimensional array you pass the array through a foreach foreach($myArray as $key => $val) { echo $key ,"&".$val."<br/>"; } will output topLevelElement0 & array topLevelElement1 & array Now, if we nest a 2nd foreach inside the first and do the same but change the variable names so we don't conflict: foreach($myArray as $key0 => $val0) { echo $key0 ,"&".$val0."<br/>"; foreach($myArray as $key1 => $val) { echo $key1 ,"&".$val1."<br/>"; } } will output topLevelElement0 & array 2ndLevel Element 0 & array 2nd Level Elem 1 & array topLevelElement1 & array 2ndLevelElem 0 & array 2ndLevelElem 1 & array Does that help?
-
you nest foreach loops with different counters to reference the index as $myArray[$i][$k]. $i = 0; $k=0; foreach($myArray as $row) //or as $key0 => $val0 depending on what you want to do { foreach($myArray[$i] as $row1)//or $key1 => $val1 { // you can keep delving down if you need to } } just throw in your count() wherever appropriate for the subarray you need to get a count of its elements.
-
Did you solve this? This query will always result in wk == 7 because your WHERE doesn't differentiate between themselves, so the same rows are getting updated, first with 1, then with 2, then 3, etc, until 7. The problem isn't necessarily your code as much as the table structure or the way you're querying it. Seems to me that maybe this shouldn't be an UPDATE so much as an INSERT query.
-
here you make var $images but reference $image (no trailing s).
-
Off the top of my head, you can build an array. Are there 512 TEAMS or 512 Brackets (1024 teams). probably a more efficient way to store/organize the array, but this is a 1st draft pointing you in the direction I'd go $brackets512 = array(); $brackets512[0 thru 512]=array(); $bracket512[$i][0]==team1 ID $bracket512[$i][1]==team1Score this round $brackets512[$i][2]==team2 ID $brackets512[$i][3]==team2Scorethis round if($brackets512[$i][3] > $brackets512[$i][1] ==team2 moves on, else team1 moves on and gets placed in the appropriate slot in $brackets256 which follows the same array layout If you want everything to be seedings, rather than winner 1/2 plays winner 3/4, then you can store another array, $seedings where index is the seed. Just compare the index of the teams and place them in the appropriate index in $bracketsX Like I said, rough draft with some holes to fill, but that should work
-
Can you post the logout.php script? I agree with the earlier posters that 1) you need to shuffle everything so that header() comes before all output (you can store all the output in a variable like $output and just echo it at the end. That way it won't interfere with any header() calls 2) ensure that either session_destroy() is used in logout or that the appropriate $SESSION variables are unset.
-
Quite true--you'll see no argument from me there. When I was just learning PHP in the early 4.x days I was not only new to PHP but to programming period. The first book I picked up (1st Edition Welling/Thompson's PHP and MySQL Web Development) used this function throughout. Because of my neophyte understanding of programming (really more from lack of understanding) at the time, I had difficulty trying to move beyond it and use functions like mysql_fetch_array() and get the results I expected. Now I'm using PDO for all the new projects and only use mysql_fetch_array() in the older projects and only use mysql_fetch_array() when updating other features of my earliest projects more to keep everything consistent. I remember how frustrating it was to me trying to teach myself, especially at the beginning, and that function #1 made it easier to get past returned queries and worry about the rest of the program and #2 made it easier for me to visualize what was going on so that I could understand what was happening between getting the resource and assigning values from the query to variables. I offer it up to help people who were like me for the same reasons I used it back when I bought that first PHP book circa 2003.
-
I use this function and process in all of my non PDO projects: set this function in an include file somewhere: function db_result_to_array($result) { $res_array = array(); for ($count=0; $row = @mysql_fetch_array($result); $count++) $res_array[$count] = $row; return $res_array; } Then, in your main code: $query = "SELECT * FROM BlahTable WHERE foo = 'bar'"; $result = mysql_query($query); if(!$result){//put error handling here: I echo mysql_errno() and mysql_error() to the screen to tell me why I couldn't get a result back. Then I use die(); to stop the rest of the script from running until I get the query problem resolved.} $result = db_result_to_array($result); foreach($result as $row) { //now step through: each pass through the foreach loop will be one returned row from the database. The best part about this is you can reference either the index number OR the column name. Let's say the 3rd column in your database is named "foo" and the single returned row has the value of "bar" in that column. In that case: $value1 = $row[2];//index 2 is the 3rd item in a zero-based array $value2 = $row['foo'];//remember that foo is the sql column name echo "<br/>value1 is: $value1 <br/>and value 2 is: $value2</br>"; //both will include "foo" in the echo } From that point you can use whatever variable values you assigned during the processing of your returned results. This function is very simple to incorporate but it's pretty robust and helps you get what you want. To address your specific issue, you have the variable $strusername taking the value of the returned array. Whether you use the function above or some other way, you have to step through the returned results. At the point it is in your code, it's not even an array yet. I hope that makes sense. Resource #9 kicked my butt when I first started learning PHP
-
doubledee, I've incorporated some of the security measures you're thinking about in one of my sites in the past, including elements like storing it in a SESSION and comparing it to ensure that it hasn't changed between sessions. I've learned, however, that this doesn't really provide the level of security you want in a reliable fashion. For example, using my example of checking for a changing IP during the same session--it completely destoyed mobile access to the site (which was bad considering it was the trouble ticket system I wrote for my PC repair business and I had difficulties using it in the field with my phone!). A phone moving from one "cell" to another can get reassigned an IP addess in no time flat, so just travelling down the Interstate can cause this check to flag the action and declare a "fail" Additionally,while I can see your point about ensuring that someone from one IP checking one person's profile a large number of times would be cause for concern, there are a few things to think about here: This check won't stop someone from using anonymizer (sp?) services. One person can look like 20 unlogged people throughout the day because they constantly have a different address. Additionally, have a chat with your lawyer. One thing I've learned over time is that when you do something you aren't required to do, even when (maybe especially when), when the proverbial poop hits the fans, the suing attorneys will not say,"Well, at least you tried." Instead, they'll say, "You intentionally set up a system so that you could flag occurances like that man stalking a single profile. Yet he stalked that profile for three days before he killed my client's daugher and you did nothing about it, although you clearly had the ability to do something." It sucks, and it's a shame, and I personally think it weakens society, but when you try to do take those extra non-required-by-law steps, it actually puts more of a legal burden on you, not less. Also, based on the sound of this site, you should keep a sharp eye /ear out for recent developments on the individual state levels about social networking sites (especially dating sites) and state requirements about forcing registered sex offenders to announce themselves within the site and requiring the site owners to do background checks on its members. Seems to me that if you're designing a site that would cause you pause enough to check if someone is stalking another member's profile, you might be affected by some of these laws that are currently being kicked around committees in different state houses. If you want to track IP, go into it knowing that there are very simple ways to defeat it and that any information you glean from it has to be taken with a grain of salt. It can give you a general idea, but never anything with actionable specific detail. All of that to say my $.02 is: No, don't make it fail if you can't get that info.
-
Ahh.....so it wasn't really adding the values, they were just all output to the screen in succession so it seemed like it I always bracket my echo statements with <br/> to make it easier to delineate the information it's trying to tell me Looking at the returns, at least you know it's sticking with the lowest compared value like you intended
-
How are the image screenshots being uploaded? What type of files are the others being downloaded? If you prepend the word "IMAGE" or something similar, you'd be able to easily discern which ones are the image files. Alternatively you could just look at the last 3 chars of the filename and look for JPG, GIF, PNG, etc. Alternatively you can look for the period for the file extension and grab everything after that to also catch the random jpeg extension. It's fairly simple to scan a directory and list the files in there and display them. I did something similar almost 10 years ago when I first started working with PHP. What is it you're trying to do that the scripts you've looked at aren't showing you how to accomplish? It's just a matter of: Get a list of the files in the directory foreach and build your links as you go (OL/UL maybe? Table? What's more appropriate to the overall site design?)
-
The main question you have to answer first is, do you want to require that your user has cookies turned on? (Since they're logging in, I would guess so since tossing login credentials around via URL is bad...) If so, storing in the SESSION is quick and easy and you can access it from any page without having to throw around GET vars in your URL. Via URL you're passing it around to a bunch of pages that don't need to know about it other than to pass it along to the next step. With SESSION it's there when you need to use it, stays out of the way when you don't. This scenario is the type of thing SESSION is used for
-
Jason Lengsdorf' Apress book, Pro PHP and jQuery has a whole section on this matter that's outstanding. http://www.apress.com/9781430228479/
-
try thowing in an echo statement in that loop so you can see the value of each one as it passes through and how it affects $laagstegetal. Is the value being appended to it? Prepended? Is every value being attached or just some of them? A quick echo will help determine that an narrow it down
-
assuming you have your date already formated 'Y-m-d' in a variable (here it will be $date) $newDate = date_add($date, date_interval_create_from_date_string('1 day')); From there you have the new date to reformat, output, do what you please There are lots of examples on ways to use date_add at the php manual page for it: http://www.php.net/manual/en/function.date-add.php