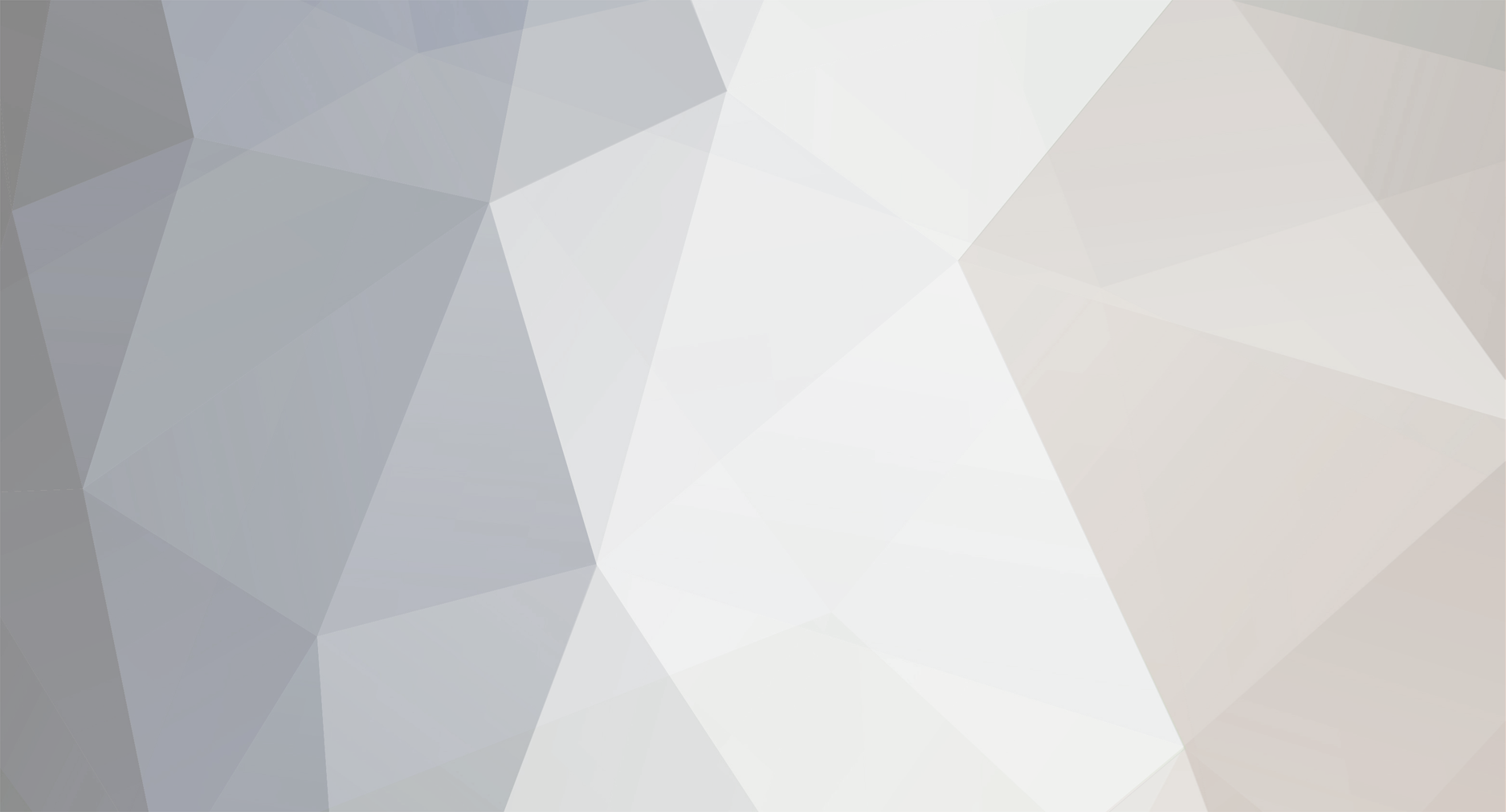
dezkit
-
Posts
1,236 -
Joined
-
Last visited
Posts posted by dezkit
-
-
$query = "SELECT * FROM $tbl_name WHERE Class='".$classname."' ORDER by Class";
-
$UserId=mysql_real_escape_string($_GET['id']);
don't forget.
-
Use a while loop to send all people an email.
Bonus: Ajax loader if your database is huge
-
remove
$process = mysql_fetch_assoc($result);
-
$data = mysql_query("SELECT DISTINCT db_Appcategory FROM application_directory") or die(mysql_error());
Not sure what you're trying to ask, but the sql above will only echo unique db_Appcategorys, no multiples.
-
<?php session_start(); $username="xxxx"; $password="xxxx"; $database="xxxx"; $host="xxxx"; $user=stripslashes(mysql_real_escape_string($_POST['user'])); $pass=stripslashes(mysql_real_escape_string($_POST['pass'])); @mysql_connect($host,$username,$password); @mysql_select_db($database) or die("Unable to select database"); $sql="SELECT username, `password` FROM users WHERE username='$user' and `password`='$pass'"; $r=mysql_query($sql); if(!$r){ $err=mysql_error(); print $err; exit(); } if(mysql_affected_rows()==0){ header("Location:user_login.htm"); print "Invalid User Name/Password. Please try again"; exit(); } else{ header("Location:memberhome.htm"); $_SESSION["user"] = $user; exit(); } ?>
memberhome.php <- note it must be php
<?php session_start(); if(!$_SESSION["user"]){ header("location: index.php"); } // all the code that the member can see echo "Welcome back," . $_SESSION["user"]; ?>
I suggest you look into this tutorial - http://phpeasystep.com/phptu/6.html
-
<?php $query1 = "SELECT * FROM `example1` LIMIT 0 , 2"; $result1 = mysql_query($query1) or die(mysql_error()); $i=1; $rows = array( ); while($row1 = mysql_fetch_array($result1)){ $rows[$i]["name"] = $row1["name"]; $rows[$i]["id"] = $row1["id"]; $i++; } $query2 = "INSERT INTO example2 (id_1,name_1,id_2,name_2) VALUES('".$rows[1]["id"]."','".$rows[1]["name"]."','".$rows[2]["id"]."','".$rows[2]["name"]."' ) "; $result2 = mysql_query($query2) or die(mysql_error()); ?>
Is this what you needed?
-
huh? what is my script not doing right for you?
- It takes two random rows
- while its looping through the rows it's...
-- inserting the data to another table
-- deleting the data in the original table
-
IN REPLY TO:
Pull two rows from same table that have ID and Name.
Create a row in different table with both ids and names from first two.
Delete the original two rows
Make sense?
<?php $query1 = "SELECT * FROM example1 LIMIT 0, 2"; $result1 = mysql_query($query1) or die(mysql_error()); while($row1 = mysql_fetch_array($result1)){ $query2 = "INSERT INTO example2 (id, name) VALUES('".$row1["id"]."', '".$row1["name"]."' ) "; $result2 = mysql_query($query2) or die(mysql_error()); $query3 = "DELETE FROM example1 WHERE id='".$row1["id"]."'"; $result3 = mysql_query($query3) or die(mysql_error()); } ?>
Is this what you wanted?
-
no problem
-
Yeah!! I thought you had included that thing lol, I thought it was a problem on php's side, but then I re read the xml.<?php $forecast = simplexml_load_file('http://www.google.com/ig/api?weather=New%20York'); foreach ($forecast->weather->forecast_conditions as $weather) { echo $weather->day_of_week["data"]; } ?>
lol
You are awesome! Thanks
So I'm guessing anytime it has an encapsulating tag of its own it needs to be included in that foreach?
-
<?php $forecast = simplexml_load_file('http://www.google.com/ig/api?weather=New%20York'); foreach ($forecast->weather->forecast_conditions as $weather) { echo $weather->day_of_week["data"]; } ?>
lol
-
try foreaching
echo $weather->day_of_week["data"];
if doesnt work:
echo $weather["day_of_week"]["data"];
-
you should also use trim() incase there is an accidental space in the beginning
-
Wait are you trying to get two different rows?
Sorry, I'm confused at what your doings.
-
if($timenow1 == $timenow5 && $timenow2 >= $timenow6){ $update1 = mysql_query("UPDATE leagues SET status='0' WHERE id='$id'"); $update2 = mysql_query("SELECT * FROM leagueplayers WHERE league='$id'"); while ($player1 = mysql_fetch_array($update1) && $player2 = mysql_fetch_array($update2)) { echo $player1[id]; echo"-"; echo $player2[id]; } }
try this
-
Post code, error occurs because copy() is empty
-
I'm not sure but I think another solution can be a sql join, is that correct?
-
What are you trying to say? You want to get all the data from another sql database to your own? You may want to export the sql in the export tab in the other database.
-
Umm, you can use simpleXML instead, easier.
-
$next_date = strtotime($i++." day", strtotime($start_date));
should work
-
Well if you are not using an admin system you can use this ->
using array:
$blockIPs = "192.168.1.1,127.0.0.1"; $blockIPs = explode(",",$blockIPs); foreach($blockIPs as $IP){ if($_SERVER['REMOTE_ADDR'] == trim($IP)){ header("location: you-are-banned.html"); } }
using database:
$result = mysql_query("SELECT * FROM ip_ban_list") or die(mysql_error()); while($row = mysql_fetch_array( $result )) { if($_SERVER['REMOTE_ADDR'] == $row["ip"]){ header("location: you-are-banned.html"); } }
oh and btw-CV, I just totally got suprised when I saw a quote of mine in your signature, haha!
-
-
<?php if($logged == "yes"){ // number 3, notice how i closed the php tag, to the right-> ?> <div class="urbangreymenu"> <h3 class="headerbar">Pilot Center</h3> <ul> <li><a href='ATR42.php'><b>ATR42 Schedules</a></li></b> <li><a href='skedatr.php'><b>ATR42F Schedules</a></li></b> <li><a href='b737.php'><b>B737 Schedules</a></li></b> <li><a href='a310.php'><b>A310 Schedules</a></li></b> <li><a href='b767.php'><b>A300 Schedules</a></li></b> <li><a href='b777.php'><b>B777 Schedules</a></li></b> <li><a href='b747.php'><b>B747 Schedules</a></li></b> <li><a href='logbook.php'><b>My logbook</a></li></b> <li><a href='download.php'><b>Download Section</a></li></b> <li><a href='help.php'><b>Pilot's Manual</a></li></b> <li><a href='rankings.php'><b>Rankings</a></li></b> <li><a href='pilotroster.php'><b>Pilot hours</a></li></b> <li><a href='flights.php?map=karachi' target='_blank'><b>Flight Movements</a></li></b> </ul> </div> <?php // <- here also }?>
There were a couple problems-
1. You forgot to close <ul>
2. Dont use php short tags, they are the bad.
3. When echo'ing outside of php tags, you have to close them
Convert raw text data into a mysql insert statement
in PHP Coding Help
Posted
well first you can explode() them by comma, then explode() them by =