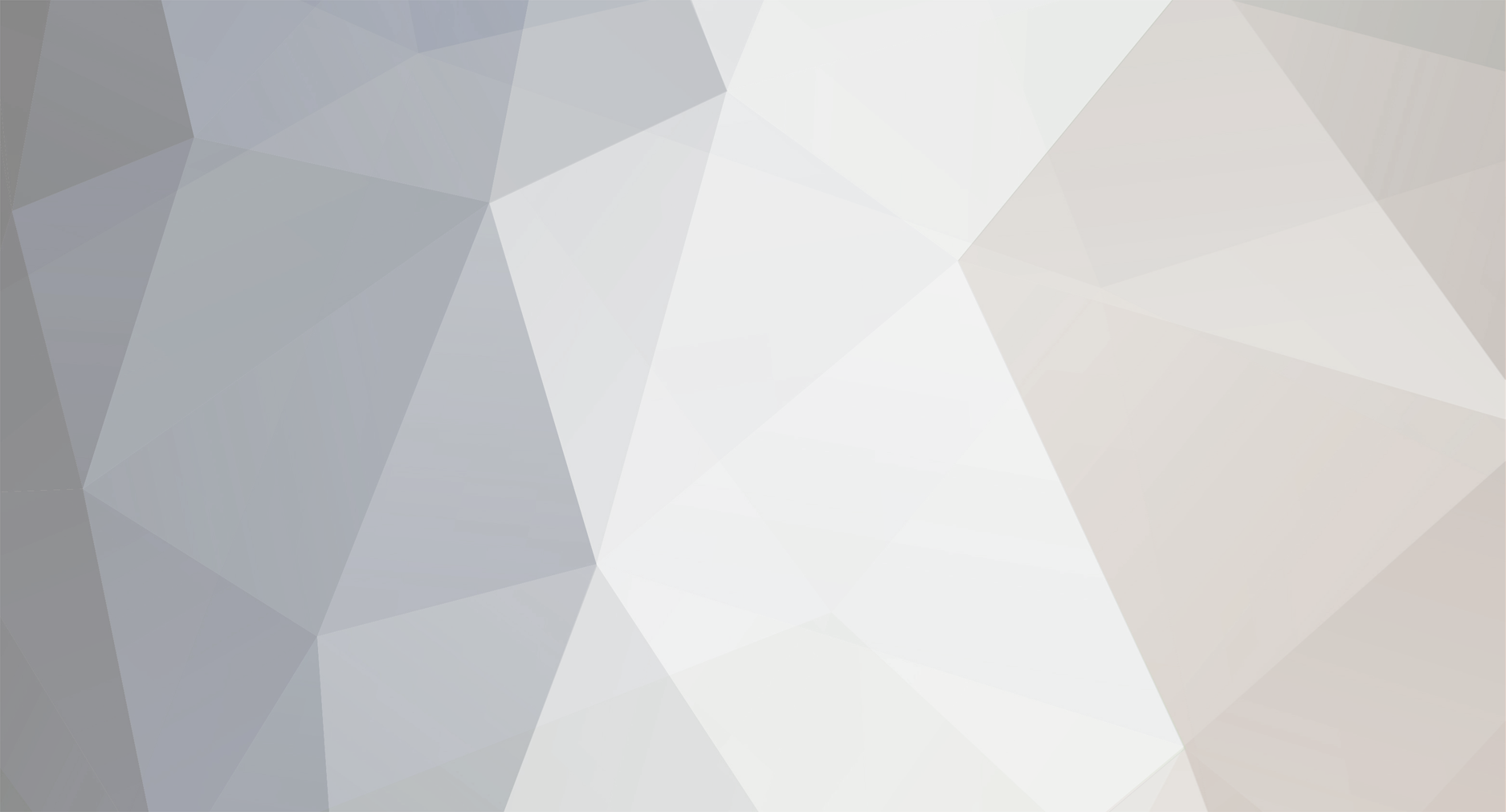
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
Well, my real name is Jermaine but most of my friends refer to me as "J". I'm an IT Manager, yes at 19 ;D
I've always been interested in technology and computing. I first started learning to program at the mere age of 11, I was intrigued at how applications were created.
I enjoy gaming, and hanging out with a few friends. I smoke and drink, unfortuantly but oh well. Been with my girlfriend near on a year now. Hoping that'll it will last, fingers crossed!
Anyway, nice to meet everybody. -
You could possibly write it to an XML format also.
-
A recent code snippet. I think this may be the solution to your problem and if it isn't it's in the correct direction.
http://www.phpfreaks.com/forums/index.php?topic=155984.msg808536#new
-
Try this, you were missing an }
<?PHP $dbhost = "localhost"; $dbname = "**********"; $dbuser = "**********"; $dbpass = "*******"; mysql_connect ($dbhost,$dbuser,$dbpass)or die("Could not connect:".mysql_error()); mysql_select_db($dbname)or die(mysql_error()); $name = $_POST['name']; $email = $_POST['email']; $username = $_POST['username']; $password = md5($_POST['password']); $companyname = $_POST['companyname']; $address1 = $_POST['address1']; $address2 = $_POST['address2']; $city = $_POST['city']; $county = $_POST['county']; $postal = $_POST['postal']; $country = $_POST['country']; $phone = $_POST['phone']; $mobile = $_POST['mobile']; if(($email == NULL)&&($username == NULL)&&($password == NULL)&&($name == NULL)&&($address1 == NULL)&&($address2 == NULL)&&(city == NULL)&&($county == NULL)&&($postal == NULL)&&($country == NULL));{ echo "You must complete all fields with *"; include 'register.html'; exit(); } $checkuser = mysql_query("SELECT username FROM users Where username='$username'"); $username_exist = mysql_num_rows($checkuser); if($username_exist >0){ echo "I'm sorry but the username that you specified has already been taken. Please pick another one."; unset($username); include 'register.html'; exit(); } $query = "INSERT INTO users (name,email,username,password,companyname,address1,address2,city,county,postal,country,phone,mobile) VALUES('$name','$email','$username','$password','$companyname','$address1','$address2','$city','$county','$postal','$country','$phone','$mobile')"; mysql_query($query)or die(mysql_error()); mysql_close(); $yoursite = 'www.nurevolution.co.uk'; $webmaster = 'Johnny McCaffery'; $youremail = 'support@nurevolution.co.uk'; $subject = "You have successfully registered at $yoursite..."; $message = "Dear $name, you are now registered at our web site. To login, simply go to our web page and enter in the following details in the login form: Username: $username Password: $password Please print this information out and store it for future reference. Thanks, $webmaster"; mail($email, $subject, $message, "From: $yoursite <$youremail>\nX-Mailer:PHP/" . phpversion()); echo "you have successfully Registered."; echo "Your information has been mailed to your email address."; ?>
-
Try something similar to
<?php function validateForm() { $valid = false; foreach($_POST as $field) { if(empty($field)) { $valid = false; } else { $valid = true; } } if($valid) { echo 'Form submitted successfully.'; } else { echo 'Please fill in all fields.'; } } ?>
-
As for your code being un-secure. It is vulnerable i must say, using sprintf() with every query that requires a variable should be used. And so should mysql_real_escape_string() with every user inputted data as Thorpe stated above.
-
Woops, yes you're right Thorpe - Since i'm only selecting the data, not writing it. Thanks for the clarification.
-
Cheers dude, worked a treat. Learn something new every day!
-
I know this code is wrong, but it has the correct idea in what i want it to do.
Rather than printing 'Upload Failed' or 'Uploaded Successfully' for each file type in the array, what would be the correct way to print whether it failed or not (without using die). Am i using the wrong loop?
<?php function checkFileType($filename, $filetype) { $filetypes[0] = 'image/jpeg'; $filetypes[1] = 'image/jpg'; $filetypes[2] = 'image/gif'; $filetypes[3] = 'image/png'; $filetypes[4] = 'image/bmp'; $filetypes[5] = 'image/tif'; $filetypes[6] = 'image/tiff'; for($filetypes as $files) { if($filetype != $files) { echo 'Upload Failed'; } else { echo 'Uploaded Successfully'; } } } ?>
-
A query to confirm a login would be more secure if it was similar to
<?php session_start(); $password = $_POST['password']; $password = mysql_real_escape_string($password); $password = htmlentities($password); $password = htmlspecialchars($password); $password = MD5($password); $username = $_POST['username']; $username = mysql_real_escape_string($username); $username = htmlentities($username); $username = htmlspecialchars($username); $sql = sprintf( "SELECT * FROM `users` WHERE `username` = '%s' AND `password` = '%s' LIMIT 1", $username, $password ); $sql = mysql_query( $sql ) or die( 'Error: ' . mysql_error() ); $obj = mysql_fetch_object( $sql ); if ( $obj ) { $_SESSION['logged'] = true; echo 'Logged in successfully'; } else { $_SESSION['logged'] = false; echo 'Invalid details'; } ?>
But the answer to your question is yes, you are on the right track.[
-
Why don't you just use a boolean when the user logs in
// If logged in
$_SESSION['logged'] = true;
// If not
$_SESSION['logged'] = false;
<?php session_start(); if ( $_SESSION['logged'] ) { echo 'Logout'; } else { echo 'Login'; } ?>
-
Do you have port 80 forwarded? Or which ever port your server is running on?
-
Anything aside from the widgets?
-
I may have asked this before, although i'm not certain.
My primary concern regarding paypal is, what's the easiest, most efficient way to create an automized billing system.
For example, somebody purchases something on my website, they then click the button to then be directed to the paypal gateway - After that, they then send the money.
How could i possibly know if the money is sent automatically? Does paypal redirect users to a certain page on your website or what?
-
I agree, i assumed he was getting header errors.
-
Using PHP is pretty easy.
<?php $get = $_GET['do']; switch($get) { default: $form = '<form method="post" action="?do=sendmail">'; $form .= 'Email To: <input type="text" name="sendto" /><br />'; $form .= 'Your Email: <input type="text" name="email" /><br />'; $form .= 'Subject: <input type="text" name="subject" /><br />'; $form .= 'Message: <textarea name="message"></textarea><br />'; $form .= '<input type="submit" value="Send" />'; $form .= '<input type="hidden" name="form_check" value="1" />'; $form .= '</form>'; echo $form; break; case 'sendmail': if ( array_key_exists($_POST, 'form_check') ) { $sendto = $_POST['sendto']; $from= $_POST['email']; $subject = $_POST['subject']; $message = $_POST['message']; $message = nl2br($message); // Every new line is a break mail($sendto, $subject, $message, $from); echo 'Your mail has been successfully sent. Thank you for using our mail service.'; } else { echo 'Your mail failed to be sent, Please go back and try again.'; } break; } ?>
This is a simple, and reasonably un-secure script, although not much damage could be done through an email.
Please bear in mind, i rarely test my code that i write on here. So please let me know if you encounter any issues.
-
You should just use a <meta> tag - If that's what i think you mean.
Example:
<?php echo '<meta http-equiv="refresh" content="5;directory/file.php">'; echo 'You will now be re-directed in 5 seconds. <br />'; echo 'If you are not re-directed, please <a href="directory/file.php">Click Here</a>.'; ?>
-
If i'm correct you want to be able to add, change, display and update code in the database?
If so it's not too difficult.
<?php $host = 'localhost'; $user = 'root'; $pass = ''; $dbname = 'dbname'; $con = mysql_connect($host, $user, $pass); if($con) { mysql_select_db($dbname); } else { echo 'Error: ' . mysql_error(); } switch($_GET['do']) { default: $sql = sprintf("SELECT `content` FROM `main_page` LIMIT 1"); $sql = mysql_query($sql) or die('Error: ' . mysql_error()); $obj = mysql_fetch_object($sql); echo '<form action="?do=update" method="post">'; echo '<textarea name="content">' . $obj->content . '</textarea>'; echo '<input type="submit" value="Submit" />'; echo '</form>'; break; case 'update': $content = $_POST['content']; $content = mysql_real_escape_string($content); $sql = sprintf("UPDATE `main_page` SET `content` = '%s'", $message); $sql = mysql_query($sql) or die('Error: ' . mysql_error()); echo 'Updated Successfully.'; break; } ?>
I think thats what you're looking for, although i haven't tested it myself to make sure it works. But i'm confident.
-
Try
<?php if ( $num > 0 ) { echo 'Number is above 0'; } else if ( $num == 1 ) { echo 'Number is equal to 1'; } else { echo 'We do not currently know the status of the number.'; } ?>
-
One of your main errors is most likely here:
<strong>Grant #<? $_POST['gnum']; ?></strong> </td>
You are only opening the PHP tag using the short method, you aren't actually printing the results.
Although short hand methods of programming aren't usually reccomended, it shouldn't make too much difference.
Methods:
<? echo $_POST['gnum']; ?> // Short hand opening statement
<?=$_POST['gnum'];?> // Short hand opening and echo'ing the results
<?php echo $_POST['gnum']; ?> // Long hand opening and echo'ing the results, reccomended
-
Would anybody be able to provide an example on how to remove the text area part of the file field leaving only the browse button?
-
This is more of an HTML question but also relates to PHP slightly.
Could anybody provide an example of how to remove the text field from a file input type, leaving only the browse button.
Also, in doing this - Would this effect a PHP upload in any way?
-
Why shouldn't i store them in the database itself? It makes no difference. Storing in a directory, the issue there is that directories have file limits.
-
Is is possible to convert images in PHP? ImageMagick i assume.
Total newbie question (-> and =>)
in PHP Coding Help
Posted
-> is used with classes, example below
The ouput of the above would be "My name is Wolphie"
=> is used to assign keys in array, example below
The above output would be:
Wolphie
2