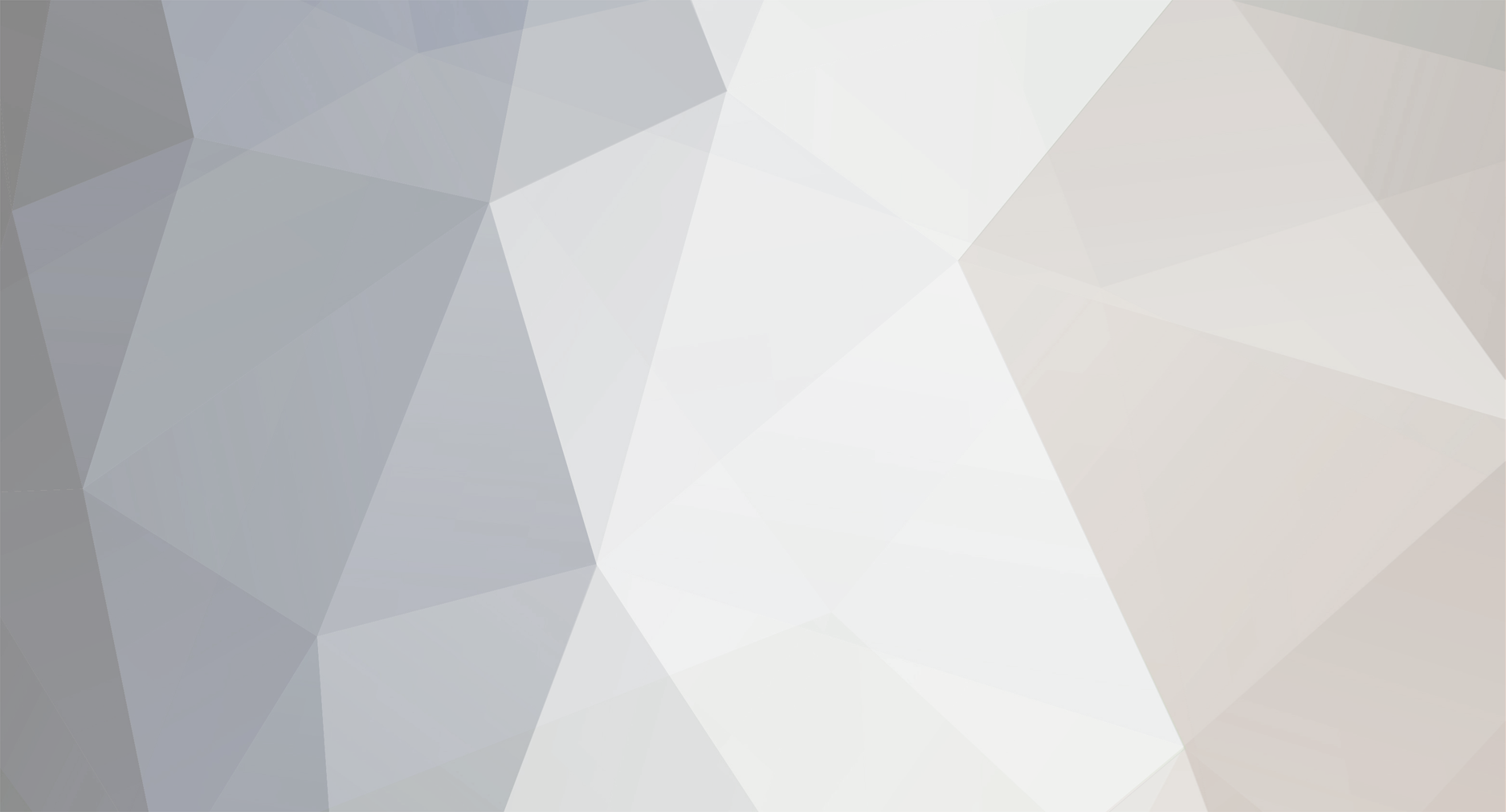
Wolphie
Members-
Posts
682 -
Joined
-
Last visited
Never
Everything posted by Wolphie
-
My web server is unix
-
Hmm, this works on my local server but not on my web server. The built-in function i mean.
-
Thanks!
-
Does anybody know how to check e-mails using regex. E.g. i have a text box where somebody inputs an e-mail address. And if it doesn't contain certain e-mail address formats then return an error message. Characters such as "@" or "."
-
Thanks for your help guys. Much appreciated!
-
Ok so with the following code i'm trying to check the database for already used usernames and e-mail addresses. I'm not entirely sure if this is working or not, but all i'm getting back is a blank page. function checkDetails() { $username = mysql_escape_string($_POST['username']); // Retrieve username from registration form $email = mysql_escape_string($_POST['email']); // Retrieve e-mail from registration form $sql = mysql_query(sprintf("SELECT `username`, `email` FROM `users`")) or die('Error: ' . mysql_error()); while($obj = mysql_fetch_object($sql)) { if(($obj->username) == $username || ($obj->email) == $email) { ?> <table width="500" cellpadding="0" cellspacing="0" class="mainBody" align="center"> <tr> <td align="center" class="headTitle"> <u>Registration Failed!</u> </td> </tr> <tr> <td align="center"> <p> The username or e-mail address you have chosen is currently in use. Please use a different username or e-mail address. </p> </td> </tr> </table> <? } else { checkReg(); } } } checkDetails();
-
Sorry, i just got extremely confused and made a typo. It's meant to be $_FILES['file']['name']; I'm just tired, and been coding all day. Getting too much. -.-
-
That's what i'm trying to do
-
I'm pretty baffled at this. I'm not entirely sure why this isn't working: $filetype = $_POST['file']['type']; // getting the file type $file = explode('.', $filetype); // exploding the string to return just the file type $filename = rand() . '.' . $file[1]; // renaming it and then adding the file type back to save the file I'm trying to retrieve the file type seperately and then rename it.
-
Ah alright buddy, i wasn't sure if it was JUST forum software alone. (I was referring to images in general) I'm creating an image hosting service. So i was trying to make it secure as possible http://www.imgpond.phux-development.com/
-
Here is a video demonstration on how it's embedded, used and executed. http://str0ke213.tradebit.com/pub/8/57.swf
-
I've been hearing alot about PHP injections in images. I was wondering if there was a way to prevent it. e.g. reading the image and searching for specific tags such as <? etc.. Is this possible?
-
Still needing help. Sorry to be a pain. *blushes*
-
I think it could be the session variables not registering.
-
Registration Form: <?php require_once("modules/header.php"); require_once("modules/stat.php"); ?> <tr> <td class="form"> <table cellpadding="0" cellspacing="0" border="0" align="center"> <form action="modules/regv.php" method="post"> <tr><td align="right" class="formB">Banner/Image URL:</td> <td align="left" class="formB"><input type="text" class="blue" name="bannerurl" value="http://" style="font-family: Verdana;" /></td></tr> <tr><td align="right" class="formB">Website URL:</td> <td align="left" class="formB"><input type="text" name="siteurl" class="blue" value="http://" style="font-family: Verdana;" /></td></tr> <tr><td align="right" class="formB">Description:</td> <td align="left" class="formB"><input type="text" name="description" class="blue" style="font-family: Verdana;" /></td></tr> <? if(!$_SESSION['user']) { ?> <tr><td align="right" class="formB">Desired Username:</td> <td align="left" class="formB"><input type="text" name="username" class="blue" maxlength="25" style="font-family: Verdana;" /></td></tr> <tr><td align="right" class="formB">Desired Password:</td> <td align="left" class="formB"><input type="password" name="password" class="blue" maxlength="25" style="font-family: Verdana;" /></td></tr> <tr><td align="right" class="formB">Email Address:</td> <td align="left" class="formB"><input type="text" name="email" class="blue" style="font-family: Verdana;" /></td></tr> <tr><td align="right" class="formB">PayPal Email Address:</td> <td align="left" class="formB"><input type="text" name="paypalemail" class="blue" style="font-family: Verdana;" /></td></tr> <? } else { ?> <tr><td align="right" class="formB"><br /><br />Username:</td> <td align="left" class="formB"><br /><br /><?php echo '<span class="formA">' . $_SESSION['user'] . '</span>'; ?></td></tr> <tr><td align="right" class="formB">Email Address:</td> <td align="left" class="formB"><?php echo '<span class="formA">' . $_SESSION['email'] . '</span>'; ?></td></tr> <tr><td align="right" class="formB">PayPal Email Address:</td> <td align="left" class="formB"><?php echo '<span class="formA">' . $_SESSION['paypalemail'] . '</span>'; ?></td></tr> <? } ?> <tr><td><br /><br /> </td><td align="center" class="formB"><input type="submit" value="Continue" style="font-family: Verdana;" /> <input type="reset" value="Reset" style="font-family: Verdana;" /></td></tr> </table> </td> </tr> <? require_once("modules/footer.php"); ?> Registration PHP: <?php session_start(Header("Location: pay.php")); $host = "localhost"; $user = ""; $pass = ""; $db = ""; $con = mysql_connect($host, $user, $pass); if (!$con) { die("Could not connect: " . mysql_error()); } mysql_select_db($db, $con); $bannerurl = $_POST['bannerurl']; $websiteurl = $_POST['siteurl']; $description = $_POST['description']; $username = $_POST['username']; $password = MD5($_POST['password']); $email = $_POST['email']; $paypalemail = $_POST['paypalemail']; $sql = mysql_query(sprintf("INSERT INTO `paddle` ( bannerurl, description, siteurl, username, password, email, paypalemail ) VALUES ( '%s', '%s', '%s', '%s', '%s', '%s', '%s' )", mysql_real_escape_string($bannerurl), mysql_real_escape_string($websiteurl), mysql_real_escape_string($description), mysql_real_escape_string($username), mysql_real_escape_string($password), mysql_real_escape_string($email), mysql_real_escape_string($paypalemail) )); if($obj = mysql_fetch_object($sql)) { $_SESSION['logged'] = true; $_SESSION['user'] = $_POST['username']; $_SESSION['website'] = $obj -> siteurl; $_SESSION['description'] = $obj -> description; $_SESSION['email'] = $obj -> email; $_SESSION['paypalemail'] = $obj -> paypalemail; $_SESSION['banner'] = $obj -> bannerurl; } else { $_SESSION['logged'] = false; } ?> Login Form: <?php require_once("modules/header.php"); require_once("modules/stat.php"); ?> <tr> <td class="form" align="center"> <? if(!$_SESSION['user']) { ?> <table cellpadding="0" cellspacing="0" border="0" align="center"> <form action="modules/logv.php" method="post"> <tr><td align="right" class="formB">Username:</td> <td align="left" class="formB"><input type="text" class="blue" name="username" style="font-family: Verdana;" /></td></tr> <tr><td align="right" class="formB">Password:</td> <td align="left" class="formB"><input type="password" class="blue" name="password" style="font-family: Verdana;" /></td></tr> <tr><td><br /><br /> </td><td align="center" class="formB"><input type="submit" value="Login" style="font-family: Verdana;" /> </form> </table> <? } else { ?> You are already logged in. <? } ?> </td> </tr> <? require_once("modules/footer.php"); ?> Login PHP: <?php session_start(Header("Location: usercp.php")); $host = "localhost"; $user = ""; $pass = ""; $db = ""; $con = mysql_connect($host, $user, $pass); if (!$con) { die("Could not connect: " . mysql_error()); } mysql_select_db($db, $con); $username = $_POST['username']; $password = MD5($_POST['password']); $sql = mysql_query("SELECT * FROM `paddle` WHERE username = '$username' AND password = '$password' LIMIT 1"); if($obj = mysql_fetch_object($sql)) { $_SESSION['logged'] = true; $_SESSION['user'] = $_POST['username']; $_SESSION['website'] = $obj -> siteurl; $_SESSION['description'] = $obj -> description; $_SESSION['email'] = $obj -> email; $_SESSION['paypalemail'] = $obj -> paypalemail; $_SESSION['banner'] = $obj -> bannerurl; } else { $_SESSION['logged'] = false; } ?> UserCP: <?php require_once("modules/header.php"); require_once("modules/stat.php"); ?> <tr> <td align="center" class="regular"> <? if(!$_SESSION['logged']) { ?> You are not logged in. [<a href="login.php">Login</a>] <? } else { ?> <u>User Control Panel</u> <br /><br /> <a href="details.php">Change Details</a> <br /> <a href="purchase.php">New Advertisement</a> <? $sql = mysql_query("SELECT * FROM `paddle` WHERE username = '" . $_SESSION['user'] . "'") or die('Error: ' . mysql_error()); if($obj = mysql_fetch_object($sql)) { ?> <table width="100%" cellpadding="0" cellspacing="0" border="0" align="center" class="tusercp"> <tr> <td>Status</td> <td>Website</td> <td>Featured</td> <td>PayPal Email</td> <td>Description</td> </tr> <tr> <td><? echo $obj -> status; ?></td> <td><? echo $obj -> siteurl; ?></td> <td><? echo $obj -> featured; ?></td> <td><? echo $obj -> paypalemail; ?></td> <td><? echo $obj -> description; ?></td> </tr> </table> <? } ?> <a href="logout.php">Logout</a> <? } ?> </td> </tr> <? require_once('modules/footer.php'); ?>
-
Well, i've probably been looking at TOO much code to identify the 'exact' problem myself. But the problem is within the membership system. It's not logging the users correctly. (Registration & Login)
-
Hey, i'm currently working on an advertisement project. This requires paypal payment methods etc... and a membership system. Most of these i have alreayd implemented myself. But i'm currently having trouble on the membership system. Since this project would be too large to post the code for, i was wondering if anybody would be kind enough to email me or send me a private message and/or add me to MSN so i could send them the folder. Also, if you have already looked at this project, suggestions to improve it would be highly appreciated.
-
Alright, well to be honest i'm pretty familiar and experienced in PHP programming. But i don't believe my method of coding is efficient enough to build large open source and public applications. Such as i'm writing a CMPS but my method of coding isn't efficient. Does anybody have any reccomendations on methods? Also examples could help. Something a bit like codeigniter but without changing the entire structure and framework. I just want something like how they program vBulletin. They have all the php scripts and then the style/design pages. Examples would be greatly appreciated.
-
Yeah sorry, i need to clean it up a bit. The reason why the head is included in a loop is because i need to retrieve the head tags from a database. The loop is also terminated in <?php echo $row['title]; } ?> E.g. Someone edits the websites information(Title, keywords etc..) And they'll be displayed on each page. As for the code that is being run, that is the code for the whole page.
-
Ok, sorry. You can witness it for yourself. http://81.132.229.36/dev/dave/register.php Just put your info in and hit register, then you'll see what i mean.
-
I can't see what the problem is but theres a continuious loop when i try to valdiate the data. <?php require('includes/config.php'); $sql = mysql_query("SELECT * FROM `site_options`"); while($row = mysql_fetch_array($sql)) { ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <meta name="description" content="<?php echo $row['description']; ?>" /> <meta name="keywords" content="<?php echo $row['keywords']; ?>" /> <link rel="stylesheet" href="styles/style.css" type="text/css" /> <script type="text/javascript" src="js/jquery.js"></script> <title><? echo $row['title']; } ?> - Register</title> </head> <body> <? require('includes/config.php'); require('includes/header.php'); ?> <td width="200"> <table cellpadding="0" cellspacing="0" border="0" width="200"> <? require("includes/config.php"); $sql = mysql_query("SELECT * FROM `left` ORDER BY `id` ASC"); while($row = mysql_fetch_array($sql)) { echo $row['head']; echo $row['title']; echo '</td></tr>'; echo $row['body']; echo $row['content']; echo '</td></tr>'; } ?> </table> </td> <? $var = $_GET['do']; switch($var) { default: ?> <td width="700"> <table width="720" cellpadding="0" cellspacing="0" border="0" align="center"> <tr> <td width="500" class="cmodhead"> Register </td> </tr> <tr> <td width="500" class="cmodbody"> <table width="500" cellpadding="0" cellspacing="0" border="0" class="create"> <form action="?do=register" method="post"> <tr><td> Username: </td></tr> <tr><td> <input type="text" size="25" maxlength="100" name="username" style="font-family: Tahoma; font-size: 11px;" value="Username" onClick="this.value='';" /> </td></tr> <tr><td><br /> Password: </td></tr> <tr><td> <input type="password" maxlength="100" size="25" name="password" style="font-family: Tahoma; font-size: 11px;" value="password" onClick= "this.value='';" /> </td></tr> <tr><td><br /> Email Address: </td></tr> <tr><td> <input type="text" size="25" maxlength="100" name="email" style="font-family: Tahoma; font-size: 11px;" value="Yourname@email.com" onClick="this.value='';" /> </td></tr> <tr><td> <input type="submit" value="Register" style="font-family: Tahoma; font-size: 11px;" /> </td></tr> </form> </table> </td> </tr> </table> <? break; case "register": @include("register.php"); ?> <td width="700"> <table width="720" cellpadding="0" cellspacing="0" border="0" align="center"> <tr> <td width="500" class="cmodhead"> Register </td> </tr> <tr> <td width="500" class="cmodbody"> <table width="500" cellpadding="0" cellspacing="0" border="0" class="create"> <tr><td align="center"> <? $flag = false; foreach($_POST as $field) { if($field == "") { $flag = false; } else { $flag = true; } } if($flag == false) { die('Please fill in all of the fields.'); } else { Header("Location: login.php"); } ?> </td> </tr> </table> </td> </tr> </table> <? $username = $_POST['username']; $password = MD5($_POST['password']); $email = $_POST['email']; $sql = mysql_query(sprintf("INSERT INTO `user` ( username, password, email ) VALUES ( '%s', '%s', '%s' )", mysql_real_escape_string($username), mysql_real_escape_string($password), mysql_real_escape_string($email) )) or die('Error: ' . mysql_error()); break; } require('includes/footer.php'); mysql_close($con); ?> </body> </html>
-
The most basic of what you mean: <?php //Open the directory $dir = dir("dir_name"); //List files in the directory while (($file = $dir -> read()) !== false) { echo "Filename: " . $file . "<br />"; } $dir -> close(); ?> Output will be: filename: . filename: .. filename: google.gif filename: pulse.exe filename: example.pdf filename: images.zip Etc..
-
Yes i understand how to make the hyper link. But how would i go about coding the server side? E.g. If someone clicked delete on Module 1(With ID 1) It would direct to that URL and that specific module will be deleted.
-
Ok, so i'm creating a miniature CMS. At the moment i'm working on the creation/deletion and editing of modules. I've got the creation working. But i'm struggling on the deletion and editing. This is what i was thinking: [Deletion] 1. (ID) Module 1 [Delete] (Link: modules.php?do=delete?name=Module%201&id=1) [Editing] 1. (ID) Module 1 [Edit] (Link: modules.php?do=edit?name=Module%201&id=1) How would i make it so the URL was that?