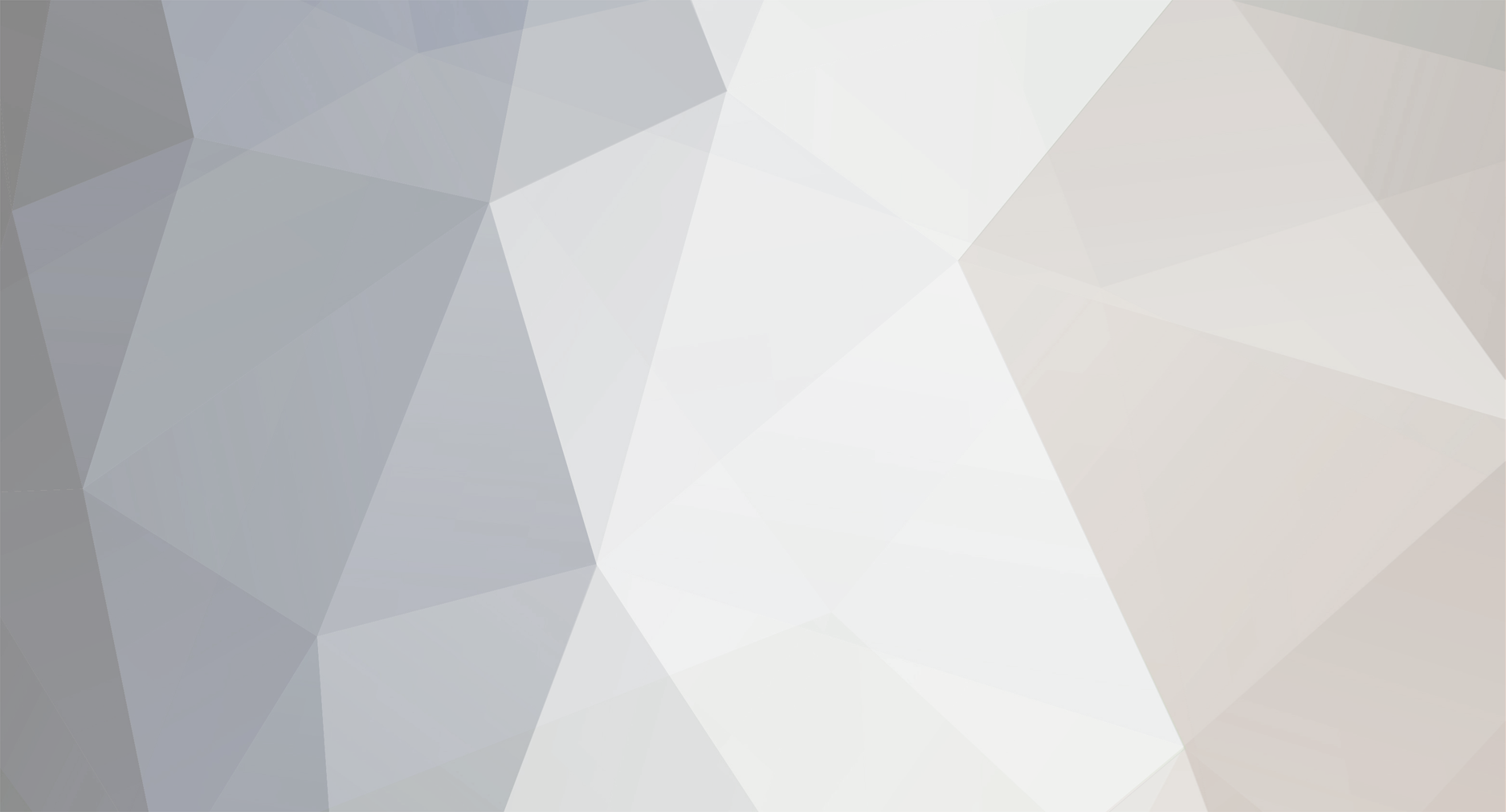
Wolphie
Members-
Posts
682 -
Joined
-
Last visited
Never
Everything posted by Wolphie
-
Please mark topic as SOLVED. Thank you.
-
I've used an array to define which files you want to exclude from the output, it makes adding and removing exclusion easy. This way you can also easily manipulate the output style. <?php $dir = 'path/to/dir'; // List of file names to exclude from output // NOTE: This is case-sensitive $exclude = array('somefile.php', 'somedir'); // Check to see if $dir is a valid directory if (is_dir($dir)) { $contents = scandir($dir); echo '<ul>'; foreach($contents as $file) { // This will exclude all filenames contained within the $exclude array // as well as hidden files that begin with '.' if (!in_array($file, $exclude) && substr($file, 0, 1) != '.') { echo '<li>'. $file .'</li>'; } } echo '</ul>'; } else { echo "The directory <strong>". $dir ."</strong> doesn't exist."; } ?>
-
Personally I would do something like: <?php if (isset($_POST['submit'])) { $userinputcity = $_GET['userinputcity']; if (empty($userinputcity)) { echo 'Please enter a city name.'; } else { $result = mysql_query(sprintf("SELECT prefix FROM store WHERE city = '%s'", $userinputcity)); if (mysql_num_rows($result) > 0) { echo 'Cities found for: ' . $userinputcity; while ($row = mysql_fetch_array($result)) { echo $row['prefix'] . '<br />'; } } else { echo 'No cities found.'; } } } ?> <!CITY FINDER FORM > <html> <form action="finder1.php" method="get"> <fieldset> <legend>Enter your City</legend> <input type="text" name="userinputcity" /> <input type="submit" value="Search Cities" name="submit" /> </fieldset> </form> <!POSTCODE FINDER FORM> <form action="finder1.php" method="get"> <fieldset> <legend>Enter your postcode prefix (S1,NG)</legend> <input type="text" name="userinputarea" /> <input type="submit" value="Search area's" name="submit" /> </fieldset> </form> </html>
-
XML is probably easier to support. PHP has a built-in XML parser. XML files can also be styled using CSS.
-
I would suggest only allowing certain hosts which are verified by your server/database. For example, you give the client a PIN to use, and if that PIN is verified with that host then all is good, otherwise make the application unusable.
-
I've been writing a DOCX and XML file to text converter in C. While I was doing some reading about XML conversion and parsing there was also a paragraph on RTF files. From what I can remember, parsing RTF files is extremely difficult to do. Example code of an RTF file from Wikipedia: {\rtf1\ansi{\fonttbl\f0\fswiss Helvetica;}\f0\pard This is some {\b bold} text.\par }
-
I'm not sure if I'm understanding this correctly. You've written a product, which you're making available for people to buy and then download. It's licensed so you don't really want people downloading it for free? Or you don't want people to get your customer details from the database? If you don't want people downloading it for free, then when they make a purchase, send them an e-mail with a uniquely generated key but only generate it when the customer has made the payment. Once that's been done, you can give them a URL containing the key which will allow them to download the file. Once it's been downloaded you can either remove the key so that it can't be re-used. I guess that's up to you. In terms of people not getting customer details from the database, well that all depends on how secure the website is that you're selling the product on.
-
For somebody to alter the code (unless your code has bugs and security flaws within it) they would need your FTP login details in order to physically edit the PHP code. However, if your code is prone to MySQL injections (which you should have tested against and fixed) then you shouldn't need to worry unless your remote database server also has security flaws. In terms of "secureness", it's no different than hosting a database locally.
-
I guess it really depends on how well formatted you want the array. For example: <?php $str = 'costa-del-sol-3-bedroom.html'; $details = explode('-', basename($str, '.html')); print_r($details); ?> The result would be: Array ( [0] => costa [1] => del [2] => sol [3] => 3 [4] => bedroom )
-
You will need to install a mail server on the local machine in order to send e-mails.
-
I don't see anything special about that website address, so yes... It can indeed be recreated in PHP, HTML, CSS and JavaScript.
-
Ruzzas is correct, we will not write the code for you. However, to point you in the right direction you should look at cron jobs and php mail.
-
Do you mean that $fullname = $username . $domain; isn't working? If so could you please post the HTML code for the form, and the relevant part of the PHP script please. I have a feeling that the select field isn't correctly constructed.
-
Check to see if the data has been inserted into the database and echo a thank you message, then send an e-mail using the mail() function. E.g. <?php // MYSQL CONNECTION CODE $email = trim($_POST['email']); // This is the users e-mail address $subject = trim($_POST['subject']); $message = trim($_POST['message']); if (isset($_POST['submit'])) { $sql = mysql_query(sprintf("INSERT INTO contact ( email, subject, message ) VALUES ( '%s', '%s', '%s' )", mysql_real_escape_string($email), mysql_real_escape_string($subject), mysql_real_escape_string($message)) ); if ($sql) { echo 'Thank you for contacting us.'; // Header information $from = 'From: '. $email ."\r\n" . 'Reply-To: '. $email; mail('admin@domain.com', $subject, $message, $headers); } else { echo 'Sorry your request could not be completed.'; } } ?> That's very basic and it should be improved upon and secured properly with form validation.
-
It should be noted that when using inline variables which contain arrays you must use curly braces around it. E.g. echo "Hi my name is {$user['name']}";
-
Well assuming you have a posts table in your database, you should be storing the ID of the user that makes a post.. E.g. post_id, user_id, post_title, post_body etc... Then you just do a SELECT COUNT(*) FROM posts_table WHERE user_id = [user_id]
-
I also failed to mention that you don't need to open a new MySQL connection twice in the same script unless you use the function mysql_close() on a MySQL handler. I also noticed you haven't included a password in the mysql_connect() function, unless you just left this out to post your code here?
-
The line $num=mysql_numrows($result); should be $num = mysql_num_rows($result); This code $result=mysql_query($check); $num=mysql_numrows($result); $i=0; while ($i < $num) { $username=mysql_result($result,$i,"username"); $bnaam=mysql_result($result,$i,"Bedrijfsnaam"); $kvk=mysql_result($result,$i,"Kvknr"); $tel=mysql_result($result,$i,"Telefoonnummer"); $email=mysql_result($result,$i,"Emailadres"); $contpers=mysql_result($result,$i,"Contactpersoon"); $adres=mysql_result($result,$i,"Adres"); $jaar=mysql_result($result,$i,"Jaar_van_oprichting"); $pcode=mysql_result($result,$i,"Postcode"); $plaats=mysql_result($result,$i,"Plaats"); $i++; } should also be within the if(isset($_COOKIE['ID_my_site'])) statement, otherwise $check may not be declared and you will get a T_UNDEFINED_VARIABLE error.
-
I've actually tested it now and it works okay but improved error handling is required for the SQL queries and connection handler. <?php if (isset($_POST['submit'])) { ///// BETTER ERROR HANDLING IS REQUIRED ///// $conn = mysql_connect('localhost', 'dbname', 'dbpass'); if ($conn) { mysql_select_db('dbname'); } // This defines a directory separator, i.e. "/" define('DS', DIRECTORY_SEPARATOR); // The directory you're uploading to (if in the same directory as this script) $target = dirname(__FILE__) . DS .'uploads'; // Maximum file size for file upload (currently 1MB) $max_file_size = round(1024 * 1024); if ((isset($_FILES['uploaded_file']) && ($_FILES['uploaded_file']['error']) == 0)) { // The name of the file you're uploading $filename = $_FILES['uploaded_file']['name']; $filetype = $_FILES['uploaded_file']['type']; $filesize = $_FILES['uploaded_file']['size']; $tempfile = $_FILES['uploaded_file']['tmp_name']; // File extension $extension = substr($filename, strrpos($filename, '.') + 1); // Only JPEG images are accepted if ($extension == 'jpg' && $filetype == 'image/jpeg') { if ($filesize < $max_file_size) { if (!file_exists($target . DS . $filename)) { if (move_uploaded_file($tempfile, $target . DS . $filename)) { // Insert the data into the database $sql = "INSERT INTO images ( name, type, size, extension, path ) VALUES ( '%s', '%s', '%s', '%s', '%s' )"; $result = mysql_query(sprintf($sql, $filename, $filetype, $filesize, $extension, $target . DS . $filename), $conn); if ($result) { echo 'You have successfully uploaded the file.'; } else { // You should never use this kind of error handling in production, this is just for testing die (mysql_error()); } } else { echo 'Error: A problem has occurred during the file upload.'; } } else { echo 'Error: The file <strong>'. $filename .'</strong> already exists.'; } } else { echo 'The file you have exceeded the upload limit.'; } } else { echo 'Error: Invalid file.'; } } else { echo 'Error: No file selected.'; } } ?> <html> <body> <form name="upload" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>" enctype="multipart/form-data"> <input type="hidden" name="MAX_FILE_SIZE" value="<?php echo $max_file_size; ?>" /> <input type="file" name="uploaded_file" /> <input type="submit" name="submit" value="Upload" /> </form> </body> </html>
-
It's pretty straight forward to do, I haven't tested this so it might not work straight out of the box. <?php if (isset($_POST['submit'])) { // This defines a directory separator, i.e. "/" define('DS', DIRECTORY_SEPARATOR); // The directory you're uploading to (if in the same directory as this script) $target = dirname(__FILE__) . DS .'uploads'; if ((!empty($_FILES['uploaded_file']) && ($_FILES['uploaded_file']['error']) == 0)) { // Secure the input somewhat foreach ($_FILES['uploaded_file'] as $key => $val) { $_FILES['uploaded_file'][$key] = mysql_real_escape_string($val); } // The name of the file you're uploading $filename = $_FILES['uploaded_file']['name']; $filetype = $_FILES['uploaped_file']['type']; $filesize = $_FILES['uploaded_file']['size']; $tempfile = $_FILES['uploaded_file']['tmp_name']; // File extension $extension = substr($filename, strrpos($filename, '.') + 1); // Only JPEG images are accepted if ($ext == 'jpg' && $filetype == 'image/jpeg') { // Only files below 1MB if ($filesize < 1024) { if (!file_exists($target . DS . $filename)) { if (move_uploaded_file($tempfile, $target . DS . $filename)) { // Insert the data into the database $sql = "INSERT INTO images ( name, type, size, extension, path ) VALUES ( '%s', '%s', '%s', '%s', '%s' )"; $result = mysql_query(sprintf($sql, $filename, $filetype, $filesize, $extension, $target . DS . $filename)); echo 'You have successfully uploaded the file.'; } else { echo 'Error: A problem has occurred during the file upload.'; } } else { echo 'Error: The file <strong>'. $filename .'</strong> already exists.'; } } else { echo 'The file you have uploaded exceeded the upload limit.'; } } else { echo 'Error: Invalid file.'; } } } ?> <html> <body> <form name="upload" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>" enctype="multipart/form-data"> <input type="hidden" name="MAX_FILE_SIZE" value="1024" /> <input type="file" name="uploaded_file" /> <input type="submit" name="submit" value="Upload" /> </form> </body> </html>
-
<input name="usr_email" id="name" class="required" value="" title="username" tabindex="4" type="text"> There's your problem. The name attribute is "usr_email". "user_name" doesn't exist in the POST array.
-
Post the HTML form code.
-
How do I escape the backslash after using mysql_real_escape_string?
Wolphie replied to Lee's topic in PHP Coding Help
echo stripslashes($row['body'] You're missing a closing bracket ) -
Sorry, I wasn't paying complete attention to your code. I noticed the error straight away and thought I'd post without knowing the exact conditions.
-
No, the correct way of doing this would be: if ($cpage != 'product') { // code }