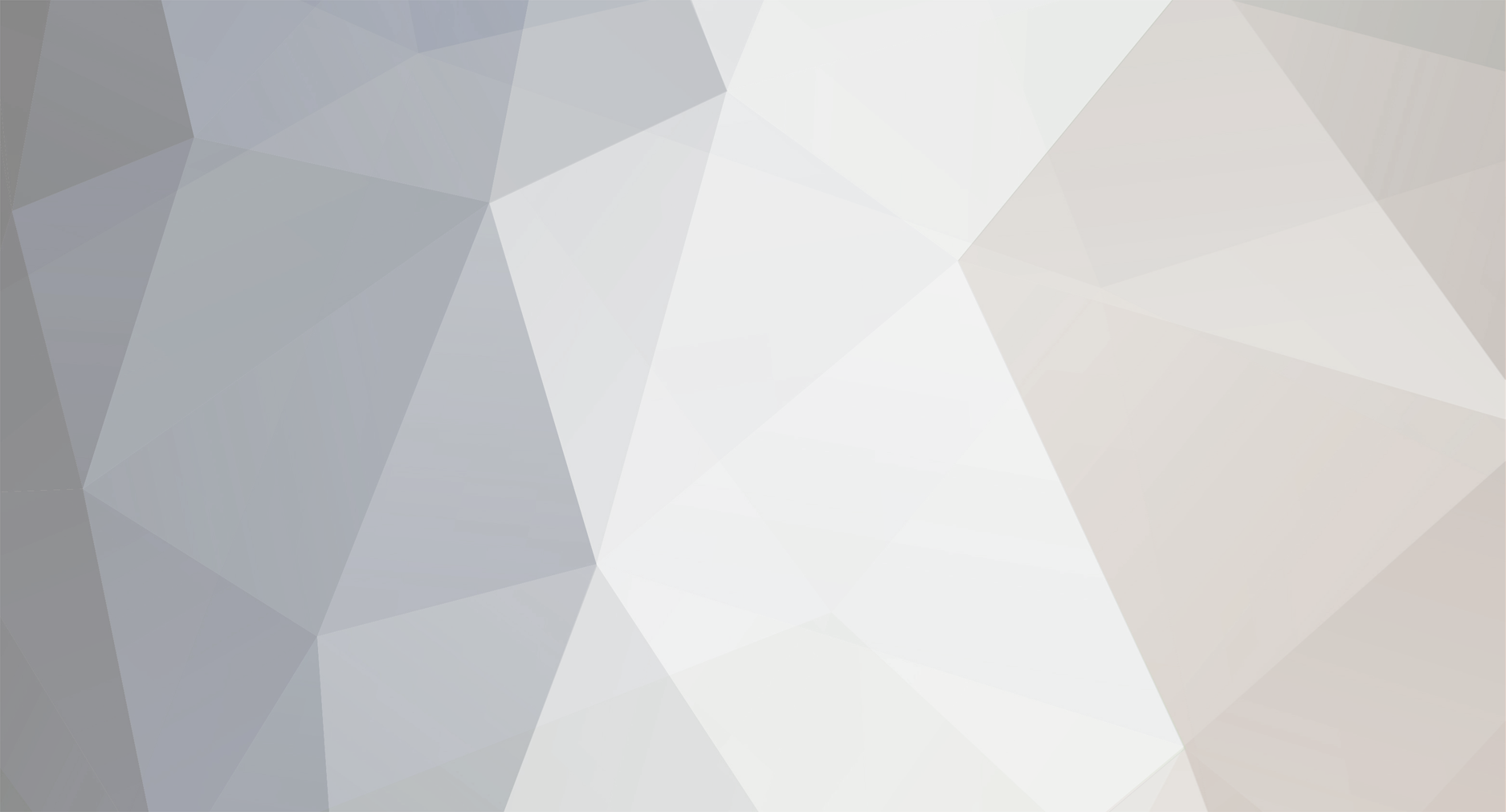
emediastudios
-
Posts
418 -
Joined
-
Last visited
Posts posted by emediastudios
-
-
I have a problem and i need it sorted asap.
I need to be able to filter the uploaded images so that they are rejected if they are a anything other than a jpeg, jpg, gif or png.
Message me with an offer, with payment amount.
This is a five minute and less job 4 someone with php skills, me i have not much, thus needing assistance.
Heres the code, the filter i have been playing with (//filter extensions) does not work, the image size filter does, as does everthing else.
<?php //This is the directory where images will be saved $path = '../images/'; //This gets all the other information from the form $name=$_POST['name']; $suburb=$_POST['suburb']; $price=$_POST['price']; $content=$_POST['content']; $content2=$_POST['content2']; $uploadFile0=($_FILES['uploadFile0']['name']); $uploadFile1=($_FILES['uploadFile1']['name']); $uploadFile2=($_FILES['uploadFile2']['name']); $uploadFile3=($_FILES['uploadFile3']['name']); $uploadFile4=($_FILES['uploadFile4']['name']); $uploadFile5=($_FILES['uploadFile5']['name']); $uploadFile6=($_FILES['uploadFile6']['name']); $uploadFile7=($_FILES['uploadFile7']['name']); $uploadFile8=($_FILES['uploadFile8']['name']); // Connects to your Database mysql_connect("localhost", "root", "***********") or die(mysql_error()) ; mysql_select_db("gcproperty") or die(mysql_error()) ; //Writes the information to the database mysql_query("INSERT INTO `employees` VALUES ('$name', '$suburb', '$price', '$content', '$content2','$uploadFile0', '$uploadFile1', '$uploadFile2', '$uploadFile3', '$uploadFile4', '$uploadFile5', '$uploadFile6', '$uploadFile7', '$uploadFile8')") ; $uploadNeed = $_POST['uploadNeed']; // start for loop for($x=0;$x<$uploadNeed;$x++){ $file_name = $_FILES['uploadFile'. $x]['name']; // strip file_name of slashes $file_name = stripslashes($file_name); $file_name = str_replace("'","",$file_name); $copy = move_uploaded_file($_FILES['uploadFile'. $x]['tmp_name'], $path . $file_name); } //filter extensions function valid_ext($file_name) { $valid = array("jpeg","jpg","jpe","png","gif"); $extension = strtolower(substr(strrchr($file_name,"uploadFile".$x),1)); if(in_array($extension, $valid)) return TRUE; else return FALSE; } //filter by size, function valid_size() { if($_FILES['uploadFile'. $x]['name']['size'] > 1048576) return FALSE; //Over one mega // check if successfully copied if($copy){ print "<meta http-equiv=\"refresh\" content=\"0;URL=property_added_successfully.php\">"; } else{ echo "$file_name The File(s) could not be uploaded!<br>The file must be under 1 meg and be of a valid extension type, (jpeg, ,jpe, jpg, png or gif!<br /> <br /> Please go back and try agian"; } // end of loop ?>
-
Thanks Orio
The image size filter works, i changed a bit of your code to suit mine and it works like a charm, thanks.
Cant get the image type filter to work though.
Any ideads anyone.
Heres the full code.
<?php //This is the directory where images will be saved $path = '../images/'; //This gets all the other information from the form $name=$_POST['name']; $suburb=$_POST['suburb']; $price=$_POST['price']; $content=$_POST['content']; $content2=$_POST['content2']; $uploadFile0=($_FILES['uploadFile0']['name']); $uploadFile1=($_FILES['uploadFile1']['name']); $uploadFile2=($_FILES['uploadFile2']['name']); $uploadFile3=($_FILES['uploadFile3']['name']); $uploadFile4=($_FILES['uploadFile4']['name']); $uploadFile5=($_FILES['uploadFile5']['name']); $uploadFile6=($_FILES['uploadFile6']['name']); $uploadFile7=($_FILES['uploadFile7']['name']); $uploadFile8=($_FILES['uploadFile8']['name']); // Connects to your Database mysql_connect("localhost", "root", "5050888202") or die(mysql_error()) ; mysql_select_db("gcproperty") or die(mysql_error()) ; //Writes the information to the database mysql_query("INSERT INTO `employees` VALUES ('$name', '$suburb', '$price', '$content', '$content2','$uploadFile0', '$uploadFile1', '$uploadFile2', '$uploadFile3', '$uploadFile4', '$uploadFile5', '$uploadFile6', '$uploadFile7', '$uploadFile8')") ; $uploadNeed = $_POST['uploadNeed']; // start for loop for($x=0;$x<$uploadNeed;$x++){ $file_name = $_FILES['uploadFile'. $x]['name']; // strip file_name of slashes $file_name = stripslashes($file_name); $file_name = str_replace("'","",$file_name); $copy = move_uploaded_file($_FILES['uploadFile'. $x]['tmp_name'], $path . $file_name); } //filter extensions /////////////////////////////This Filter doesnt work///////////////////////////////////////////////////// function valid_ext($file_name) { $valid = array("jpeg","jpg","jpe","png","gif"); $extension = strtolower(substr(strrchr($file_name,"uploadFile".$x),1)); if(in_array($extension, $valid)) return TRUE; else return FALSE; } //filter by size, function valid_size() { if($_FILES['uploadFile'. $x]['name']['size'] > 1048576) return FALSE; //Over one mega else return TRUE; } // check if successfully copied if($copy){ print "<meta http-equiv=\"refresh\" content=\"0;URL=property_added_successfully.php\">"; } else{ echo "$file_name The File(s) could not be uploaded!<br>The file must be under 1 meg and be of a valid extension type, (jpeg, ,jpe, jpg, png or gif!<br /> <br /> Please go back and try agian"; } // end of loop ?>
-
I have this code, it works very well foe me except one thing.
I want to filter the the uploaded image files to be either jpeg, jpg, gif or png.
Any ideas?
Heres the code, i have a image filter in my code but it does not work.
<?php //This is the directory where images will be saved $path = '../images/'; //This gets all the other information from the form $name=$_POST['name']; $suburb=$_POST['suburb']; $price=$_POST['price']; $content=$_POST['content']; $content2=$_POST['content2']; $uploadFile0=($_FILES['uploadFile0']['name']); $uploadFile1=($_FILES['uploadFile1']['name']); $uploadFile2=($_FILES['uploadFile2']['name']); $uploadFile3=($_FILES['uploadFile3']['name']); $uploadFile4=($_FILES['uploadFile4']['name']); $uploadFile5=($_FILES['uploadFile5']['name']); $uploadFile6=($_FILES['uploadFile6']['name']); $uploadFile7=($_FILES['uploadFile7']['name']); $uploadFile8=($_FILES['uploadFile8']['name']); // Connects to your Database mysql_connect("localhost", "root", "************") or die(mysql_error()) ; mysql_select_db("gcproperty") or die(mysql_error()) ; //Writes the information to the database mysql_query("INSERT INTO `employees` VALUES ('$name', '$suburb', '$price', '$content', '$content2','$uploadFile0', '$uploadFile1', '$uploadFile2', '$uploadFile3', '$uploadFile4', '$uploadFile5', '$uploadFile6', '$uploadFile7', '$uploadFile8')") ; $uploadNeed = $_POST['uploadNeed']; // start for loop for($x=0;$x<$uploadNeed;$x++){ $file_name = $_FILES['uploadFile'. $x]['name']; // strip file_name of slashes $file_name = stripslashes($file_name); $file_name = str_replace("'","",$file_name); $copy = move_uploaded_file($_FILES['uploadFile'. $x]['tmp_name'], $path . $file_name); } //filter extensions function valid_ext($file_name) { $valid = array("jpeg","jpg","jpe","png","gif"); $extension = strtolower(substr(strrchr($file_name,"uploadFile" . $x),1)); if(in_array($extension, $valid)) return TRUE; else return FALSE; } //filter by size, function valid_size() { if($_FILES['uploadFile'. $x]['name']['size'] > 1048576) return FALSE; //Over one mega else return TRUE; } // check if successfully copied if($copy){ print "<meta http-equiv=\"refresh\" content=\"0;URL=property_added_successfully.php\">"; } else{ echo "$file_name The File(s) could not be uploaded!<br>The file must be under 1 meg and be of a valid extension type, (jpeg, ,jpe, jpg, png or gif!<br /> <br /> Please go back and try agian"; } // end of loop ?>
-
I have this code that works well for me.Just need the added function to check that "uploadFile" is a jpeg, jpg, gif or png and is no bigger than a meg.
Any help be great.
Code below for process php file.
<?php //This is the directory where images will be saved $path = '../images/'; //This gets all the other information from the form $name=$_POST['name']; $suburb=$_POST['suburb']; $price=$_POST['price']; $content=$_POST['content']; $content2=$_POST['content2']; $uploadFile0=($_FILES['uploadFile0']['name']); $uploadFile1=($_FILES['uploadFile1']['name']); $uploadFile2=($_FILES['uploadFile2']['name']); $uploadFile3=($_FILES['uploadFile3']['name']); $uploadFile4=($_FILES['uploadFile4']['name']); $uploadFile5=($_FILES['uploadFile5']['name']); $uploadFile6=($_FILES['uploadFile6']['name']); $uploadFile7=($_FILES['uploadFile7']['name']); $uploadFile8=($_FILES['uploadFile8']['name']); // Connects to your Database mysql_connect("localhost", "root", "password") or die(mysql_error()) ; mysql_select_db("gcproperty") or die(mysql_error()) ; //Writes the information to the database mysql_query("INSERT INTO `employees` VALUES ('$name', '$suburb', '$price', '$content', '$content2','$uploadFile0', '$uploadFile1', '$uploadFile2', '$uploadFile3', '$uploadFile4', '$uploadFile5', '$uploadFile6', '$uploadFile7', '$uploadFile8')") ; $uploadNeed = $_POST['uploadNeed']; // start for loop for($x=0;$x<$uploadNeed;$x++){ $file_name = $_FILES['uploadFile'. $x]['name']; // strip file_name of slashes $file_name = stripslashes($file_name); $file_name = str_replace("'","",$file_name); $copy = move_uploaded_file($_FILES['uploadFile'. $x]['tmp_name'], $path . $file_name); } // check if successfully copied if($copy){ print "<meta http-equiv=\"refresh\" content=\"0;URL=property_added_successfully.php\">"; } else{ echo "$file_name | could not be uploaded!<br>"; } // end of loop ?>
The image is posted from this file
Code shown below
<form action="add_test.php" method="post" enctype="multipart/form-data" onsubmit="MM_validateForm('name','','R','content','','R');return document.MM_returnValue"> <div align="left"><br /> <table width="507" border="0" align="center" bgcolor="#333333"> <tr> <td colspan="3" align="left" valign="bottom" bgcolor="#999999"><span class="style7">Add Property</span></td> </tr> <tr> <td colspan="3">Add a property to GCPRoperty Database<hr /></td> </tr> <tr> <td width="251"><div align="right">Name:</div></td> <td width="26"> </td> <td width="216"><input name="name" type="text" id="name" /></td> </tr> <tr> <td><div align="right">Suburb:</div></td> <td> </td> <td><input name = "suburb" type="text" id="suburb" /></td> </tr> <tr> <td><div align="right">Price: </div></td> <td> </td> <td><input name = "price" type="text" id="price" /></td> </tr> <tr> <td colspan=""> <p> </p></td> </tr> <tr> <td valign="top"><div align="right">Photo(s)</div></td> <td> </td> <td><p> <? // start of dynamic form $uploadNeed = $_POST['uploadNeed']; for($x=0;$x<$uploadNeed;$x++){ ?> <input name="uploadFile<? echo $x;?>" type="file" id="uploadFile<? echo $x;?>" /> <? // end of for loop } ?> <input name="uploadNeed" type="hidden" value="<? echo $uploadNeed;?>" /> </p> </td> </tr> <tr> <td><div align="right">Brief Text</div></td> <td> </td> <td><label> <textarea name="content" cols="40" rows="10" id="content"></textarea> </label></td> </tr> <tr> <td><div align="right">Full Text</div></td> <td> </td> <td><textarea name="content2" cols="40" rows="10" id="content2"></textarea></td> </tr> <tr> <td> </td> <td> </td> <td><input type="submit" value="Add" /></td> </tr> </table> <p> </p> </div> </form>
-
I used this code and it works like i wish, seems strange as to me it feel like its reversed.
<?php if (!empty($_POST['photo2'])) { echo "<a href='images/".$_POST['photo2']."' target='_blank'><img src='images/".$_POST['photo2']."' alt='image' width='150' border='0' /></a>"; } else { echo ""; } ?>
-
Seems strange but i used this code and it works,
Reversed it
<?php if (!empty($_POST['photo2'])) { echo "<a href='images/".$_POST['photo2']."' target='_blank'><img src='images/".$_POST['photo2']."' alt='image' width='150' border='0' /></a>"; } else { echo ""; } ?>
-
I want to check to see if there is a empty form varible sent.
I have this code
<?php if (isset($_POST['what do i put in here if i want to check if posted data is nothing'])) { echo "N/A"; } else { echo "<a href='images/".$_POST['photo2']."' target='_blank'><img src='images/".$_POST['photo2']."' alt='image' width='150' border='0' /></a>"; } ?>
-
What would be the prefered method in this scenero
I have a database of properties and have a show all records page.
From that page you get an option to view all details about any record.
When i choose one, how does the info from (that) record get posted to a page that displays all the info.
I used form varibles, is that the best way?
Thanks.
-
Sure Here it is
</table> <table width="645" align="center"> <tr> <td colspan="2" align="left" valign="top" bordercolor="#000000" bgcolor="#F1F1F1"><div align="left"><span class="style1"><u> <?php echo $_POST['name']; ?></u></span> </div> <div align="right"></div></td> </tr> <tr> <td width="173" align="left" valign="top" bordercolor="#000000" bgcolor="#FFFFFF"><table width="156" border="0"> <tr> <td width="150" height="403" valign="top"> <table width="150" border="0"> <tr> <td> <br /></td><?php if (isset($_POST['photo2'])) { echo "<a href='images/".$_POST['photo2']."' target='_blank'><img src='images/".$_POST['photo2']."' alt='image' width='150' border='0' /></a>"; } else { echo "N/A"; } ?> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo3']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo3']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo4']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo4']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo5']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo5']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo6']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo6']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo7']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo7']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table>
And this is the file code that sends the data
<form action="full_details.php" method="post" enctype="multipart/form-data" name="properties" target="_self" id="properties"> <table width="600" align="center" bgcolor="#F1F1F1"> <tr> <td width="437" align="left" valign="top" bordercolor="#000000" bgcolor="#FFFFFF"><span class="style1"><u><?php echo $row_Recordset1['name']; ?></u></span><span class="style3"> <label> <input name="name" type="hidden" id="name" value="<?php echo $row_Recordset1['name']; ?>" /> </label> <label></label> </span> <span class="style3"><br /> <span class="style5"><?php echo $row_Recordset1['price']; ?> <label></label> <label> <input name="price" type="hidden" id="price" value="<?php echo $row_Recordset1['price']; ?>" /> </label> <br /> <?php echo $row_Recordset1['suburb']; ?> <label></label> <label> <input name="suburb" type="hidden" id="suburb" value="<?php echo $row_Recordset1['suburb']; ?>" /> </label> <br /> </span></span><span class="style3"><?php echo $row_Recordset1['content']; ?> <label> <input name="content" type="hidden" id="content" value="<?php echo $row_Recordset1['content']; ?>" /> <input name="content2" type="hidden" id="content" value="<?php echo $row_Recordset1['content2']; ?>" /><br /> <input name="photo" type="hidden" id="photo" value="<?php echo $row_Recordset1['photo']; ?>" /> <br /> <input name="photo2" type="hidden" id="photo2" value="<?php echo $row_Recordset1['photo2']; ?>" /> <br /> </label> <label> <input name="photo3" type="hidden" id="photo3" value="<?php echo $row_Recordset1['photo3']; ?>" /> </label> <label> <input name="photo4" type="hidden" id="photo4" value="<?php echo $row_Recordset1['photo4']; ?>" /> </label> <label> <input name="photo5" type="hidden" id="photo5" value="<?php echo $row_Recordset1['photo5']; ?>" /> </label> <label> <input name="photo6" type="hidden" id="photo6" value="<?php echo $row_Recordset1['photo6']; ?>" /> </label> </span> <label> <input name="photo7" type="hidden" id="photo7" value="<?php echo $row_Recordset1['photo7']; ?>" /> <input name="photo8" type="hidden" id="photo8" value="<?php echo $row_Recordset1['photo8']; ?>" /> <input name="photo9" type="hidden" id="photo9" value="<?php echo $row_Recordset1['photo9']; ?>" /> </label></td> <td width="151"><div align="center"><a href="images/<?php echo $row_Recordset1['photo']; ?>" target="_blank"><img src="images/<?php echo $row_Recordset1['photo']; ?>" alt="image" width="150" border="0" /></a></div></td> </tr> <tr> <td colspan="2"></td> </tr> <tr> <td height="23" colspan="2" bgcolor="#666666"><a href="full_details.php" target="_self"> <label> <input type="submit" name="submit" id="submit" value="more info" /> </label> </a></td> </tr> </table> </form>
-
I am a beginner in php, could you explain in a little more detail please, sorry. But i would be so stoked to sort this out.
Thanks again
-
i used your code and i think im getting a step closer.
Your definately on the right track>
When i open the show all details page by itself i get the N/A
But if i open it from the file i post the data from it doesnt work. ???
Thyanks for your help champ.
-
Correct me if im wrong but could there be a code that checks if the posted form varible had data and display region if true,
and hide region if the posted form varible had no data.
This is my first file
<?php if ($totalRows_Recordset1 > 0) { // Show if recordset not empty ?> <form action="full_details.php" method="post" enctype="multipart/form-data" name="properties" target="_self" id="properties"> <table width="600" align="center" bgcolor="#F1F1F1"> <tr> <td width="437" align="left" valign="top" bordercolor="#000000" bgcolor="#FFFFFF"><span class="style1"><u><?php echo $row_Recordset1['name']; ?></u></span><span class="style3"> <label> <input name="name" type="hidden" id="name" value="<?php echo $row_Recordset1['name']; ?>" /> </label> <label></label> </span> <span class="style3"><br /> <span class="style5"><?php echo $row_Recordset1['price']; ?> <label></label> <label> <input name="price" type="hidden" id="price" value="<?php echo $row_Recordset1['price']; ?>" /> </label> <br /> <?php echo $row_Recordset1['suburb']; ?> <label></label> <label> <input name="suburb" type="hidden" id="suburb" value="<?php echo $row_Recordset1['suburb']; ?>" /> </label> <br /> </span></span><span class="style3"><?php echo $row_Recordset1['content']; ?> <label> <input name="content" type="hidden" id="content" value="<?php echo $row_Recordset1['content']; ?>" /> <input name="content2" type="hidden" id="content" value="<?php echo $row_Recordset1['content2']; ?>" /><br /> <input name="photo" type="hidden" id="photo" value="<?php echo $row_Recordset1['photo']; ?>" /> <br /> <input name="photo2" type="hidden" id="photo2" value="<?php echo $row_Recordset1['photo2']; ?>" /> <br /> </label> <label> <input name="photo3" type="hidden" id="photo3" value="<?php echo $row_Recordset1['photo3']; ?>" /> </label> <label> <input name="photo4" type="hidden" id="photo4" value="<?php echo $row_Recordset1['photo4']; ?>" /> </label> <label> <input name="photo5" type="hidden" id="photo5" value="<?php echo $row_Recordset1['photo5']; ?>" /> </label> <label> <input name="photo6" type="hidden" id="photo6" value="<?php echo $row_Recordset1['photo6']; ?>" /> </label> </span> <label> <input name="photo7" type="hidden" id="photo7" value="<?php echo $row_Recordset1['photo7']; ?>" /> <input name="photo8" type="hidden" id="photo8" value="<?php echo $row_Recordset1['photo8']; ?>" /> <input name="photo9" type="hidden" id="photo9" value="<?php echo $row_Recordset1['photo9']; ?>" /> </label></td> <td width="151"><div align="center"><a href="images/<?php echo $row_Recordset1['photo']; ?>" target="_blank"><img src="images/<?php echo $row_Recordset1['photo']; ?>" alt="image" width="150" border="0" /></a></div></td> </tr> <tr> <td colspan="2"></td> </tr> <tr> <td height="23" colspan="2" bgcolor="#666666"><a href="full_details.php" target="_self"> <label> <input type="submit" name="submit" id="submit" value="more info" /> </label> </a></td> </tr> </table> </form>
This is my second file that shows the full details
<form action="show2.php" method="get" enctype="application/x-www-form-urlencoded" name="fav" target="_self" id="fav"> <br /> <table width="640" border="0" align="center"> <tr> <td width="101"> </td> <td width="41"> </td> <td width="350"> </td> <td width="40"><div align="left"><script type="text/javascript" src="favlink.js"> </script> </div></td> <td width="40"><div align="left"><a href="javascript:window.print()"><img src="images/print.png" alt="print this page" border="0" /></a> </div></td> <td width="42"><div align="left"><a href="sendpage.php" target="_self"><img src="images/send.png" alt="send to friend" width="16" height="16" border="0" /></a></div></td> </tr> </table> <table width="645" align="center"> <tr> <td colspan="2" align="left" valign="top" bordercolor="#000000" bgcolor="#F1F1F1"><div align="left"><span class="style1"><u> <?php echo $_POST['name']; ?></u></span> </div> <div align="right"></div></td> </tr> <tr> <td width="173" align="left" valign="top" bordercolor="#000000" bgcolor="#FFFFFF"><table width="156" border="0"> <tr> <td width="150" height="403" valign="top"> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo2']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo2']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo3']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo3']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo4']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo4']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo5']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo5']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo6']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo6']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo7']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo7']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo8']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo8']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td> <a href="images/<?php echo $_POST['photo9']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo9']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> </td> </tr> </table> </td> <td width="460" valign="top"><table width="200" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo']; ?>" alt="image" width="450" border="0" /></a></td> </tr> </table> <br /> <table width="459" border="0"> <tr> <td bgcolor="#F1F1F1"><span class="style5"><?php echo $_POST['price']; ?></span></td> </tr> <tr> <td><span class="style5"><?php echo $_POST['suburb']; ?></span></td> </tr> <tr> <td height="270" valign="top"><span class="style3"><?php echo $_POST['content']; ?><br /> <hr width="300" /> </span><span class="style3"><?php echo $_POST['content2']; ?></span></td> </tr> </table> <div align="right"></div></td> </tr> <tr> <td colspan="2" align="left" valign="top" bordercolor="#000000" bgcolor="#F1F1F1"> </td> </tr> <tr> <td colspan="2"></td> </tr> </table> </form>
Hope this helps
Thanks for your help, thought this would be a simple fix but have been on it for a week and i am tearing my hair out over it.
-
Thanks pocobueno1388.
The code you have is what i need im sure.
I'm new to php and have been teaching myself from google searches and from these forums 4 around 6 months now.
sorry if i sound stupid or you find it hard to understand what im trying to do, i am having difficulty explaining and i cant blame you
Will try your code and get back to you.
Just one more question, what would i put in (put condition here).
I try to explain what i want to do.
I have a page that shows all records. That page displays some information on every record, but hides the bulk of the records info in hidden fields.
I then made form varibles in dreamweaver, named to match the fields in my database.
when the user presses the view more button it posts all the form varibles onto my show all details page.
My show all details page has (9) dynamic image fields that are populated from the form varibles from the previous file.
Problem is not all my properties have (9) images and i wish to hide that region if there is no data posted to that dynamic image field.
Does this make sense?
-
I need an alteration to my code to show region only if the form varible from the previous file is not empty.
I have a list of properties in a table called employees, database name gcproperty.
Some properties dont have full records in the fields (photo1, photo2, etc..........to photo9. and are empty.
On the show all details page some images are blank as there is no record in the database or where drawn from the form varibles browse page that where also empty.
Does this make sense? ??? ???
here is the code for image 2, the rest are the same
table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo2']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo2']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table>
-
Had an idea.
I think the code i require is something along the lines - If posted form varible (photo6) is empty dont show region.
But in a fancy php way.
-
I am a newbe and experimenting.
Its hard for me to explain.
I have a file that repeats region, (browse properties) the data is drawn fron the employees table of my gcproperty database and shows all records.
I have hidden fields in the form that i have named to match the fields in my table
code below
<?php require_once('Connections/gcproperty.php'); ?> <?php if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? "'" . doubleval($theValue) . "'" : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } mysql_select_db($database_gcproperty, $gcproperty); $query_Recordset1 = "SELECT * FROM employees"; $Recordset1 = mysql_query($query_Recordset1, $gcproperty) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); $totalRows_Recordset1 = mysql_num_rows($Recordset1); ?><!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>results</title> <style type="text/css"> <!-- .style1 { font-size: 18px; font-weight: bold; color: #666666; } .style3 {color: #666666} .style5 {font-size: 12px} .style6 {color: #FF0000} --> </style> </head> <body><br /> <?php if ($totalRows_Recordset1 == 0) { // Show if recordset empty ?> <table width="600" border="0" align="center"> <tr> <td><div align="center" class="style6">There are no current properties to display</div></td> </tr> </table> <?php } // Show if recordset empty ?> There are currently <span class="style6"><?php echo $totalRows_Recordset1 ?></span> properties listed in the GCProperty database<br /> <?php do { ?><?php if ($totalRows_Recordset1 > 0) { // Show if recordset not empty ?> <form action="full_details.php" method="post" enctype="multipart/form-data" name="properties" target="_self" id="properties"> <table width="600" align="center" bgcolor="#F1F1F1"> <tr> <td width="437" align="left" valign="top" bordercolor="#000000" bgcolor="#FFFFFF"><span class="style1"><u><?php echo $row_Recordset1['name']; ?></u></span><span class="style3"> <label> <input name="name" type="hidden" id="name" value="<?php echo $row_Recordset1['name']; ?>" /> </label> <label></label> </span> <span class="style3"><br /> <span class="style5"><?php echo $row_Recordset1['price']; ?> <label></label> <label> <input name="price" type="hidden" id="price" value="<?php echo $row_Recordset1['price']; ?>" /> </label> <br /> <?php echo $row_Recordset1['suburb']; ?> <label></label> <label> <input name="suburb" type="hidden" id="suburb" value="<?php echo $row_Recordset1['suburb']; ?>" /> </label> <br /> </span></span><span class="style3"><?php echo $row_Recordset1['content']; ?> <label> <input name="content" type="hidden" id="content" value="<?php echo $row_Recordset1['content']; ?>" /> <input name="content2" type="hidden" id="content" value="<?php echo $row_Recordset1['content2']; ?>" /><br /> <input name="photo" type="hidden" id="photo" value="<?php echo $row_Recordset1['photo']; ?>" /> <br /> <input name="photo2" type="hidden" id="photo2" value="<?php echo $row_Recordset1['photo2']; ?>" /> <br /> </label> <label> <input name="photo3" type="hidden" id="photo3" value="<?php echo $row_Recordset1['photo3']; ?>" /> </label> <label> <input name="photo4" type="hidden" id="photo4" value="<?php echo $row_Recordset1['photo4']; ?>" /> </label> <label> <input name="photo5" type="hidden" id="photo5" value="<?php echo $row_Recordset1['photo5']; ?>" /> </label> <label> <input name="photo6" type="hidden" id="photo6" value="<?php echo $row_Recordset1['photo6']; ?>" /> </label> </span> <label> <input name="photo7" type="hidden" id="photo7" value="<?php echo $row_Recordset1['photo7']; ?>" /> <input name="photo8" type="hidden" id="photo8" value="<?php echo $row_Recordset1['photo8']; ?>" /> <input name="photo9" type="hidden" id="photo9" value="<?php echo $row_Recordset1['photo9']; ?>" /> </label></td> <td width="151"><div align="center"><a href="images/<?php echo $row_Recordset1['photo']; ?>" target="_blank"><img src="images/<?php echo $row_Recordset1['photo']; ?>" alt="image" width="150" border="0" /></a></div></td> </tr> <tr> <td colspan="2"></td> </tr> <tr> <td height="23" colspan="2" bgcolor="#666666"><a href="full_details.php" target="_self"> <label> <input type="submit" name="submit" id="submit" value="more info" /> </label> </a></td> </tr> </table> </form> <?php } // Show if recordset not empty ?> <?php } while ($row_Recordset1 = mysql_fetch_assoc($Recordset1)); ?></body> </html> <?php mysql_free_result($Recordset1); ?>
When you click the more info button it posts the form varibles into the full_details.php file which looks like this
<?php require_once('Connections/gcproperty.php'); ?> <?php if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? "'" . doubleval($theValue) . "'" : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } mysql_select_db($database_gcproperty, $gcproperty); $query_Recordset1 = "SELECT * FROM employees"; $Recordset1 = mysql_query($query_Recordset1, $gcproperty) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); $totalRows_Recordset1 = mysql_num_rows($Recordset1); ?><!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>GCProperty - <?php echo $row_Recordset1['name']; ?></title> <style type="text/css"> <!-- .style1 { font-size: 18px; font-weight: bold; color: #666666; } .style3 {color: #666666} .style5 {font-size: 12px} .style6 {color: #FF0000} --> </style> </head> <body> <form action="show2.php" method="get" enctype="application/x-www-form-urlencoded" name="fav" target="_self" id="fav"> <br /> <table width="640" border="0" align="center"> <tr> <td width="101"> </td> <td width="41"> </td> <td width="350"> </td> <td width="40"><div align="left"><script type="text/javascript" src="favlink.js"> </script> </div></td> <td width="40"><div align="left"><a href="javascript:window.print()"><img src="images/print.png" alt="print this page" border="0" /></a> </div></td> <td width="42"><div align="left"><a href="sendpage.php" target="_self"><img src="images/send.png" alt="send to friend" width="16" height="16" border="0" /></a></div></td> </tr> </table> <table width="645" align="center"> <tr> <td colspan="2" align="left" valign="top" bordercolor="#000000" bgcolor="#F1F1F1"><div align="left"><span class="style1"><u> <?php echo $_POST['name']; ?></u></span> </div> <div align="right"></div></td> </tr> <tr> <td width="173" align="left" valign="top" bordercolor="#000000" bgcolor="#FFFFFF"><table width="156" border="0"> <tr> <td width="150" height="403" valign="top"> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo2']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo2']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo3']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo3']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo4']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo4']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo5']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo5']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo6']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo6']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo7']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo7']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo8']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo8']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> <br /> <table width="150" border="0"> <tr> <td> <a href="images/<?php echo $_POST['photo9']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo9']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table> </td> </tr> </table> </td> <td width="460" valign="top"><table width="200" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo']; ?>" alt="image" width="450" border="0" /></a></td> </tr> </table> <br /> <table width="459" border="0"> <tr> <td bgcolor="#F1F1F1"><span class="style5"><?php echo $_POST['price']; ?></span></td> </tr> <tr> <td><span class="style5"><?php echo $_POST['suburb']; ?></span></td> </tr> <tr> <td height="270" valign="top"><span class="style3"><?php echo $_POST['content']; ?><br /> <hr width="300" class="style5" /> </span><span class="style3"><?php echo $_POST['content2']; ?></span></td> </tr> </table> <div align="right"></div></td> </tr> <tr> <td colspan="2" align="left" valign="top" bordercolor="#000000" bgcolor="#F1F1F1"> </td> </tr> <tr> <td colspan="2"></td> </tr> </table> </form> </body> </html> <?php mysql_free_result($Recordset1); ?>
So i guess it doesnt retrive the info from the database but rather the form varibles that where populated from the database in the previous file.
I did it this way as i had no idea on how to get the current record to display in my full_details file and it worked..
??? I,m confusing myself now.........
-
I have a form that allows my client to upload up to (9) images to a directory and records the image names in a sql database.
The details go into a table called employees and my database name is gcpropery.
This works fine.
Problem is, that if my client only has 5 images, the other fields (photo6, photo7...etc..) get no input and are empty.
My show details page shows all the images and details that are drawn from form varibles that are in my browse records page.
It has 9 dynamic image fields that show the images, if there is no record in any of these fields i get a blank box with no image, this makes sense.
how do i not show that region if the field is empty in that record?
This is the code for the image fields
<table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo4']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo4']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table>
How do i alter this to only show this image if (photo4) exsists and the field is not empty?
I was told that if i altered it to
<?php if (!empty($db_var)){ ?> <a href="images/<?php echo $_POST['photo4']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo4']; ?>" alt="image" width="150" border="0" /> <?php } ?>
But it doesnt show the image at all, even if there is a record.
-
The show details page that the tabe is on is populated by form varible and not from a database binding.
If this makes a difference.
Im a newbe to this and am still learning.
Any help will be appriciated
Thanks in advanced
-
This is my table that draws from a sql database.
<table width="150" border="0"> <tr> <td><a href="images/<?php echo $_POST['photo2']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo2']; ?>" alt="image" width="150" border="0" /></a><br /></td> </tr> </table>
How do i edit this to only show this region if the file exsists in db - gcproperty, table - employees, field - photo3
thanks for any help
-
The table within the gcproperty database is called employees.
If thats any help.
Thanks again
-
i did as you mentioned and it now refuses to show the image wether there is a record or not.
This is what i did
<a href="images/<?php echo $_POST['photo3']; ?>" target="_blank"></a><a href="images/<?php echo $_POST['photo2']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo2']; ?>" alt="image" width="150" border="0" /></a>
Changed to
<?php if (!empty($db_var)){ ?> <a href="images/<?php echo $_POST['photo3']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo3']; ?>" alt="image" width="150" border="0" /></a> <?php } ?>
-
I want to be able to show region if recordset, column, is not empty.
This is the code in my php file.
<a href="images/<?php echo $_POST['photo3']; ?>" target="_blank"><img src="images/<?php echo $_POST['photo3']; ?>" alt="image" width="150" border="0" />
How would i alter this code if i wanted to only show this image (region) it if there was a record in database (gcproperty) column (photo3)
Thanks a heap
-
Sure i guess, the form is in 3 stages, (uploadForm1.php), (property_add_process_form.php) and (add_test.php)
The uploadForm1.php is included in property_add.php but that shouldnt matter should it?
This is the first form
<html> <head> <title># of Files to Upload</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> </head> <body> <form name="form1" method="post" action="property_add_process_form.php"> <p>Enter the amount of images you have for your new property below. Max = 9.</p> <p> <input name="uploadNeed" type="text" id="uploadNeed" maxlength="1"> </p> <p> <input type="submit" name="Submit" value="Submit"> </p> </form> </body> </html>
This opens property_add_process_form.php.
The code in this page is
<?php require_once('../Connections/gcproperty.php'); ?> <?php if (!isset($_SESSION)) { session_start(); } $MM_authorizedUsers = ""; $MM_donotCheckaccess = "true"; // *** Restrict Access To Page: Grant or deny access to this page function isAuthorized($strUsers, $strGroups, $UserName, $UserGroup) { // For security, start by assuming the visitor is NOT authorized. $isValid = False; // When a visitor has logged into this site, the Session variable MM_Username set equal to their username. // Therefore, we know that a user is NOT logged in if that Session variable is blank. if (!empty($UserName)) { // Besides being logged in, you may restrict access to only certain users based on an ID established when they login. // Parse the strings into arrays. $arrUsers = Explode(",", $strUsers); $arrGroups = Explode(",", $strGroups); if (in_array($UserName, $arrUsers)) { $isValid = true; } // Or, you may restrict access to only certain users based on their username. if (in_array($UserGroup, $arrGroups)) { $isValid = true; } if (($strUsers == "") && true) { $isValid = true; } } return $isValid; } $MM_restrictGoTo = "restricted.php"; if (!((isset($_SESSION['MM_Username'])) && (isAuthorized("",$MM_authorizedUsers, $_SESSION['MM_Username'], $_SESSION['MM_UserGroup'])))) { $MM_qsChar = "?"; $MM_referrer = $_SERVER['PHP_SELF']; if (strpos($MM_restrictGoTo, "?")) $MM_qsChar = "&"; if (isset($QUERY_STRING) && strlen($QUERY_STRING) > 0) $MM_referrer .= "?" . $QUERY_STRING; $MM_restrictGoTo = $MM_restrictGoTo. $MM_qsChar . "accesscheck=" . urlencode($MM_referrer); header("Location: ". $MM_restrictGoTo); exit; } ?> <?php if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? "'" . doubleval($theValue) . "'" : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } mysql_select_db($database_gcproperty, $gcproperty); $query_Recordset1 = "SELECT * FROM employees"; $Recordset1 = mysql_query($query_Recordset1, $gcproperty) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); $totalRows_Recordset1 = mysql_num_rows($Recordset1); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Administration Panel</title> <style type="text/css"> <!-- .style1 {color: #000000} body { background-color: #000000; } .style2 { color: #FFFFFF; font-size: 10px; } --> </style> <link href="../css/admin.css" rel="stylesheet" type="text/css" /> <style type="text/css"> <!-- .style4 {font-size: 16px} --> </style> <link href="../css/main.css" rel="stylesheet" type="text/css" /> <style type="text/css"> <!-- .style7 {font-size: 18px} .style8 {font-size: 10px} --> </style> <script type="text/javascript"> <!-- function MM_validateForm() { //v4.0 if (document.getElementById){ var i,p,q,nm,test,num,min,max,errors='',args=MM_validateForm.arguments; for (i=0; i<(args.length-2); i+=3) { test=args[i+2]; val=document.getElementById(args[i]); if (val) { nm=val.name; if ((val=val.value)!="") { if (test.indexOf('isEmail')!=-1) { p=val.indexOf('@'); if (p<1 || p==(val.length-1)) errors+='- '+nm+' must contain an e-mail address.\n'; } else if (test!='R') { num = parseFloat(val); if (isNaN(val)) errors+='- '+nm+' must contain a number.\n'; if (test.indexOf('inRange') != -1) { p=test.indexOf(':'); min=test.substring(8,p); max=test.substring(p+1); if (num<min || max<num) errors+='- '+nm+' must contain a number between '+min+' and '+max+'.\n'; } } } else if (test.charAt(0) == 'R') errors += '- '+nm+' is required.\n'; } } if (errors) alert('The following error(s) occurred:\n'+errors); document.MM_returnValue = (errors == ''); } } //--> </script> </head> <body> <div id="container"><table width="780" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td colspan="7"><img src="../images/admin-header.jpg" alt="GC Propery" width="780" height="223" /></td> </tr> <tr> <td width="149" height="24" background="../images/navbar.jpg" class="name"><div align="center" class="admin"><a href="edit_privacy.php" target="_self">Terms / Privacy</a></div></td> <td width="112" background="../images/navbar.jpg" class="name"><div align="center" class="admin"> <a href="property_add.php" target="_self">Property</a></div></td> <td width="113" background="../images/navbar.jpg" class="name"><div align="center" class="admin"><a href="news.php" target="_self">News</a></div></td> <td width="111" background="../images/navbar.jpg" class="name"><div align="center" class="admin"><a href="email.php" target="_self">email</a></div></td> <td width="113" background="../images/navbar.jpg" class="name"><div align="center" class="admin"> <a href="links.php" target="_self">Links</a></div></td> <td width="101" background="../images/navbar.jpg" class="name"><div align="center" class="admin"><a href="main.php" target="_self">Home</a></div></td> <td width="81" background="../images/navbar.jpg" class="name"><div align="center" class="admin"><a href="logout.php" target="_self" class="admin">Logout</a></div></td> </tr> <tr> <td height="2" colspan="7" background="../images/bar.jpg" class="admin"> </td> </tr> <tr> <td colspan="7" class="admin"><div align="left"> <blockquote> <p class="style2"><br /> <u><span class="style4">Edit GCProperty.</span></u><br /> <br /> <a href="property_delete.php" target="_self"><span class="admin">Click here to Delete Property</span></a></p> </blockquote> </div></td> </tr> <tr> <td colspan="7" class="admin"><div align="center"><br /> </div></td> </tr> <tr> <td colspan="7" class="admin"><form action="add_test.php" method="post" enctype="multipart/form-data" onsubmit="MM_validateForm('name','','R','content','','R');return document.MM_returnValue"> <div align="left"><br /> <table width="507" border="0" align="center" bgcolor="#333333"> <tr> <td colspan="3" align="left" valign="bottom" bgcolor="#999999"><span class="style7">Add Property</span></td> </tr> <tr> <td colspan="3">Add a property to GCPRoperty Database<hr /></td> </tr> <tr> <td width="251"><div align="right">Name:</div></td> <td width="26"> </td> <td width="216"><input name="name" type="text" id="name" /></td> </tr> <tr> <td><div align="right">Suburb:</div></td> <td> </td> <td><input name = "suburb" type="text" id="suburb" /></td> </tr> <tr> <td><div align="right">Price: </div></td> <td> </td> <td><input name = "price" type="text" id="price" /></td> </tr> <tr> <td colspan="3"> <p> </p></td> </tr> <tr> <td valign="top"><div align="right">Photo(s)</div></td> <td> </td> <td><p> <? // start of dynamic form $uploadNeed = $_POST['uploadNeed']; for($x=0;$x<$uploadNeed;$x++){ ?> <input name="uploadFile<? echo $x;?>" type="file" id="uploadFile<? echo $x;?>" /> <? // end of for loop } ?> <input name="uploadNeed" type="hidden" value="<? echo $uploadNeed;?>" /> </p> </td> </tr> <tr> <td><div align="right">Text</div></td> <td> </td> <td><label> <textarea name="content" cols="40" rows="10" id="content"></textarea> </label></td> </tr> <tr> <td> </td> <td> </td> <td><input type="submit" value="Add" /></td> </tr> </table> <p> </p> </div> </form> <br /><br /></td> </tr> <tr> <td colspan="5"> </td> <td> </td> <td> </td> </tr> </table> <div align="center"><br /> <br /> </div> </body> </html> <?php mysql_free_result($Recordset1); ?>
and finally the property_add_test.php we have been working on.
<?php //This is the directory where images will be saved $path = '../images/'; //This gets all the other information from the form $name=$_POST['name']; $suburb=$_POST['suburb']; $price=$_POST['price']; $content=$_POST['content']; $uploadFile0=($_FILES['uploadFile0']['name']); $uploadFile1=($_FILES['uploadFile1']['name']); $uploadFile2=($_FILES['uploadFile2']['name']); $uploadFile3=($_FILES['uploadFile3']['name']); $uploadFile4=($_FILES['uploadFile4']['name']); $uploadFile5=($_FILES['uploadFile5']['name']); $uploadFile6=($_FILES['uploadFile6']['name']); $uploadFile7=($_FILES['uploadFile7']['name']); $uploadFile8=($_FILES['uploadFile8']['name']); // Connects to your Database mysql_connect("localhost", "root", "5050888202") or die(mysql_error()) ; mysql_select_db("gcproperty") or die(mysql_error()) ; // Check the extension is valid if (preg_match('/\.(jpg|jpeg|gif|png)$/i', $_FILES['uploadFile'. $x]['name'])){ //Writes the information to the database mysql_query("INSERT INTO `employees` VALUES ('$name', '$suburb', '$price', '$content', '$uploadFile0', '$uploadFile1', '$uploadFile2', '$uploadFile3', '$uploadFile4', '$uploadFile5', '$uploadFile6', '$uploadFile7', '$uploadFile8')") ; $uploadNeed = $_POST['uploadNeed']; // start for loop for($x=0;$x<$uploadNeed;$x++){ $file_name = $_FILES['uploadFile'. $x]['name']; // strip file_name of slashes $file_name = stripslashes($file_name); $file_name = str_replace("'","",$file_name); $copy = move_uploaded_file($_FILES['uploadFile'. $x]['tmp_name'], $path . $file_name); } } else { // Add your error code here echo "Sorry, file must be of type .jpg .jpeg .gif or .png\n"; } // check if successfully copied if($copy){ print "<meta http-equiv=\"refresh\" content=\"0;URL=property_added_successfully.php\">"; } else{ echo "$file_name | could not be uploaded!<br>"; }// end of loop ?>
Huggie, where are you from?
I want to pay you for the work you have done for me!
You have been of extream assistance, thanks a ton.
I will have php work in the future and may (most likely) need some help, paid work of course!
If you are interested i would like to be able to contact you when i need some help now and then.
I am not being paid much for this site but id feel better if i gave you something.
-
I added it as you had it, i cant upload any file now, i get the error
Sorry, file must be of type .jpg .jpeg .gif or .png | could not be uploaded! ???
Thanks again huggie
DELETE IMAGE(3) FROM DIRECTORY
in PHP Coding Help
Posted
I use the code that dreamweaver produces to delete the record from my sql database.
Code below
This deletes the record but not the images from the directory, i have to add code to do that and thats where im stuck.
The field in which the file name is displayed is here
Can i use this reference to delete the image from the ../images dir
Thanks 4 any help