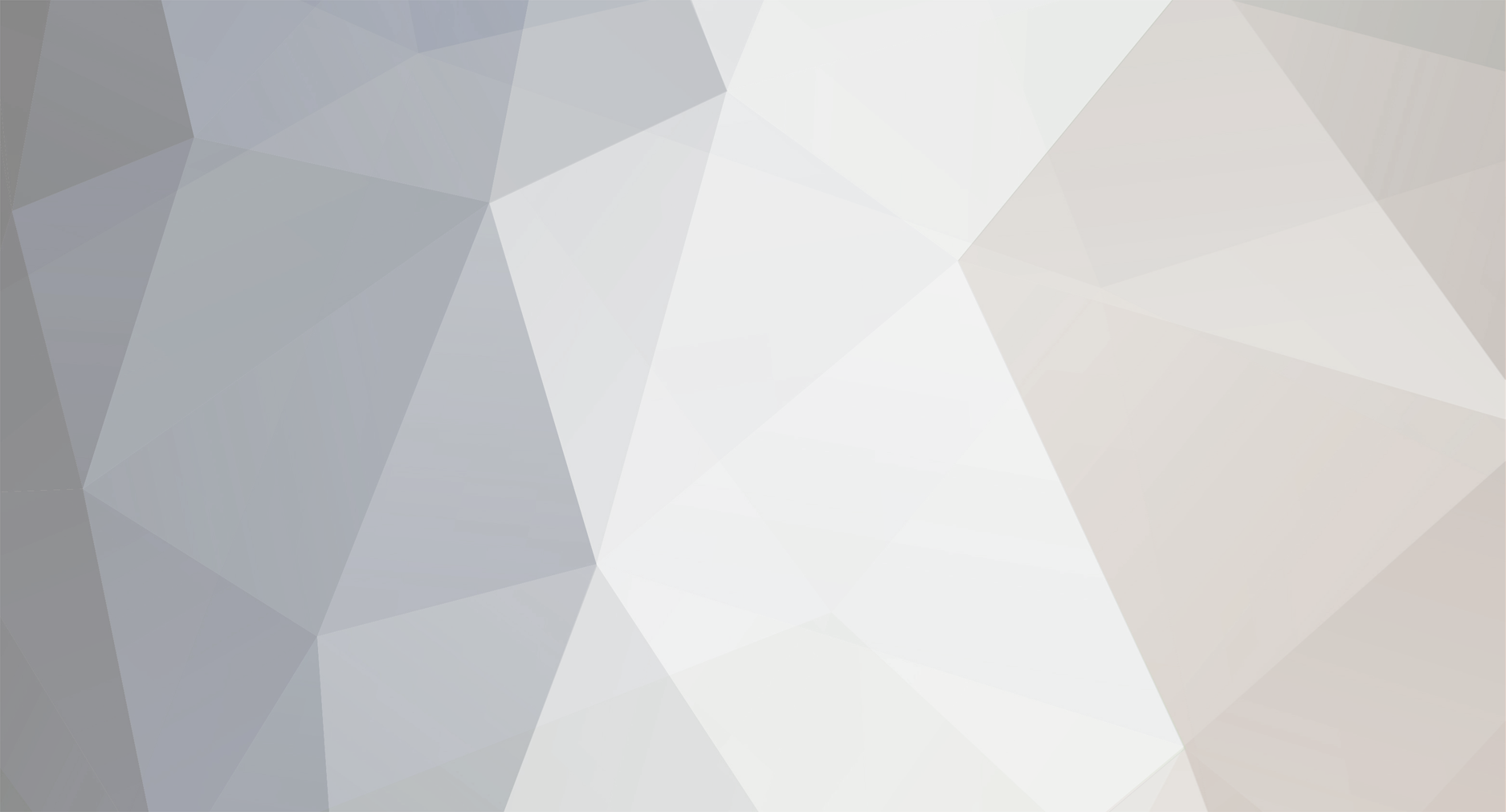
vozzek
Members-
Posts
130 -
Joined
-
Last visited
Everything posted by vozzek
-
[SOLVED] Yanking values from a text field without a form?
vozzek replied to vozzek's topic in PHP Coding Help
Yes, that's exactly it. I really want to avoid using a form if possible. -
Pefect! Thanks so much, I should've thought of that.
-
Hi everyone, Does a standard html text field have to be located within a form in order for me to pull values from it within php? For example: I have a text field for quantity. The user can change it. Later on in my page there's a button that launches the user to a php script on another page, and I need to know what the value of that text field was when the button was pressed. There's not a form on my page, so I'm assuming I can't use $_GET or $_POST. Is this possible? Thanks in advance.
-
I added an echo just before the mysql_query($sql). Here's what printed: DELETE FROM tbl_cart WHERE ct_session_id = 21ea32ef26924cf9e962a2b4f25f34bbUnknown column '21ea32ef26924cf9e962a2b4f25f34bb' in 'where clause'
-
Hi all, I've got some php code running an SQL delete to remove rows from my shopping cart table (tbl_cart). It works on individual cart id's, but not when I want the entire cart emptied. tbl_cart looks like this: ct_id (cart id, this is the primary key) pd_id (product id) ct_qty (quantity) ct_session_id (user's unique session id) etc... Here's the code: <?php // current session id $sid = session_id(); // $cid passed from view_cart page // if $cid=0, then empty cart if ($cid != 0) { $sql = "DELETE FROM tbl_cart WHERE ct_id = $cid"; // This works } else { $sql = "DELETE FROM tbl_cart WHERE ct_session_id = $sid"; // This doesn't work } $result = mysql_query($sql) or die(mysql_error()); ?> If I pass $cid as a whole number, it deletes that row - effectively deleting that item from the cart. But when the user presses the EMPTY CART button, I call the above code by passing $cid as zero. At this point I want all records deleted that match the current user's session id, which is $sid. The error I'm getting is: Unknown column '785fdf3a1a755e2a2189fa3028f33f22' in 'where clause' The crazy variable is of course, the session id. Thanks in advance.
-
[SOLVED] I'm doing this the hard way... hoping for some help
vozzek replied to vozzek's topic in PHP Coding Help
Barand, thanks! That saves a lot of time and coding. I wasn't sure I could make those comparisons within the SQL query. It still however, is not updating quantity. Am I doing the initialization (to null values) correctly? Because it's never getting to the 'else' portion of the if statement, even when all the parameters are the same. EDIT: Nevermind! I missed some underscores (opta should be opt_a, etc...) Thanks again Barand, for showing me the easy way. Here's the updated code: <?php // current session id $sid = session_id(); // initialize and set options $opt_a = $opt_b = $opt_c = $opt_d = $opt_e = $custom = ""; if ($option_a) $opt_a = $option_a; if ($option_b) $opt_b = $option_b; if ($option_c) $opt_c = $option_c; if ($option_d) $opt_d = $option_d; if ($option_e) $opt_e = $option_e; if ($custom_text) $custom = $custom_text; // check if the product is already // in cart table for this session // (RecordID was passed as a variable from master to detail) $sql = "SELECT * FROM tbl_cart WHERE pd_id = $RecordID AND ct_session_id = '$sid' AND ct_opt_a = '$opta' AND ct_opt_b = '$optb' AND ct_opt_c = '$optc' AND ct_opt_d = '$optd' AND ct_opt_e = '$opte' AND ct_custom = '$custom' "; $result = mysql_query($sql) or die(mysql_error()); if (mysql_num_rows($result) == 0) { // put the product in cart table $sql = "INSERT INTO tbl_cart (pd_id, ct_qty, ct_session_id, ct_date, ct_opt_a, ct_opt_b, ct_opt_c, ct_opt_d, ct_opt_e, ct_custom) VALUES ($RecordID, 1, '$sid', NOW(), '$opt_a', '$opt_b', '$opt_c', '$opt_d', '$opt_e', '$custom')"; $result = mysql_query($sql) or die(mysql_error()); } else { // update product quantity in cart table $sql = "UPDATE tbl_cart SET ct_qty = ct_qty + 1 WHERE ct_session_id = '$sid' AND pd_id = $RecordID"; $result = mysql_query($sql) or die(mysql_error()); } ?> -
Hi all, I'm populating a mySQL shopping cart database (tbl_cart), and the following code gets called whenever someone clicks the 'Add To Cart' button. Ideally I want to check the cart for duplicate items, in which case I'd UPDATE the quantity field by one instead of doing an INSERT. The problem: I have up to five non-required 'options', which could be color, size, etc... and I need to check to see if these are the same as well. If they are not, I need to do the INSERT because it would be a whole different item (large instead of small for example). But if all the options are the same (including the custom text field), I need to do the update. Right now the update works... but only if I add the same thing to the cart (with the same options) consecutively. If I add a different product number in the middle, my logic doesn't work and an insert happens where I wanted an update. Here's my code: <?php // current session id $sid = session_id(); // initialize and set options (passed from detail page) $opt_a = $opt_b = $opt_c = $opt_d = $opt_e = $custom = ""; if ($option_a) $opt_a = $option_a; if ($option_b) $opt_b = $option_b; if ($option_c) $opt_c = $option_c; if ($option_d) $opt_d = $option_d; if ($option_e) $opt_e = $option_e; if ($custom_text) $custom = $custom_text; // check if the product is already // in cart table for this session // (RecordID was passed as a variable from master to detail) $sql = "SELECT * FROM tbl_cart WHERE pd_id = $RecordID AND ct_session_id = '$sid'"; $result = mysql_query($sql) or die(mysql_error()); if (mysql_num_rows($result) == 0) { // put the product in cart table $sql = "INSERT INTO tbl_cart (pd_id, ct_qty, ct_session_id, ct_date, ct_opt_a, ct_opt_b, ct_opt_c, ct_opt_d, ct_opt_e, ct_custom) VALUES ($RecordID, 1, '$sid', NOW(), '$opt_a', '$opt_b', '$opt_c', '$opt_d', '$opt_e', '$custom')"; $result = mysql_query($sql) or die(mysql_error()); } else { // update product quantity in cart table (if not a duplicate) $dupflg = 1; if ($option_a) { if ($option_a != $row_rs_add_to_cart['ct_opt_a']) $dupflg = 0; } if ($option_b) { if ($option_b != $row_rs_add_to_cart['ct_opt_b']) $dupflg = 0; } if ($option_c) { if ($option_c != $row_rs_add_to_cart['ct_opt_c']) $dupflg = 0; } if ($option_d) { if ($option_d != $row_rs_add_to_cart['ct_opt_d']) $dupflg = 0; } if ($option_e) { if ($option_e != $row_rs_add_to_cart['ct_opt_e']) $dupflg = 0; } if ($custom) { if ($custom != $row_rs_add_to_cart['ct_custom']) $dupflg = 0; } if ($dupflg == 1) { // duplicate item, so update quantity $sql = "UPDATE tbl_cart SET ct_qty = ct_qty + 1 WHERE ct_session_id = '$sid' AND pd_id = $RecordID"; $result = mysql_query($sql) or die(mysql_error()); } if ($dupflg == 0) { $sql = "INSERT INTO tbl_cart (pd_id, ct_qty, ct_session_id, ct_date, ct_opt_a, ct_opt_b, ct_opt_c, ct_opt_d, ct_opt_e, ct_custom) VALUES ($RecordID, 1, '$sid', NOW(), '$opt_a', '$opt_b', '$opt_c', '$opt_d', '$opt_e', '$custom')"; $result = mysql_query($sql) or die(mysql_error()); } } ?> Hmmm... after re-looking at this, I'm thinking I need a WHILE loop to check the entire database instead of just the first row. That's the problem, eh? I'm unsure (php-wise) where I'd need it. Any help would be greatly appreciated, including advice on how to shorten my code. I'm sure I butchered this... but I'm just learning. Thanks in advance.
-
PHP automatically called from within javascript function?
vozzek replied to vozzek's topic in PHP Coding Help
sOcO, First off, I promise to get an Ajax book. Thanks for all the help. I took yet another Ajax tutorial and then went over your code until it made sense to me. I decided to insert your code into my site and tweak it around until I could get it to work. I think I'm close... but my database is still not being populated. I changed your naming convention of 'validateAddToCart' to 'checkAddToCart', only because I already had a validateAddToCart javascript function (to check user form input). I also thought maybe you missed the semicolon at the end of the var params = 'func=checkAddToCart' statement, but if it's not needed then I'm the one who's wrong. Here is the javascript on the detail page: <script type="text/javascript"> <!-- /** * setXMLHttpRequestObject - set the xml http request object */ function setXMLHttpRequestObject() { XMLHttpRequestObject = false; if (window.XMLHttpRequest) { XMLHttpRequestObject = new XMLHttpRequest(); } else if (window.ActiveXObject) { XMLHttpRequestObject = new ActiveXObject("Microsoft.XMLHTTP"); } } /** * httpPost - execute an HTTP POST */ function httpPost(url, params, stateChangeFunc) { if(XMLHttpRequestObject) { XMLHttpRequestObject.open("POST", url, true); XMLHttpRequestObject.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); XMLHttpRequestObject.setRequestHeader("Content-length", params.length); XMLHttpRequestObject.setRequestHeader("Connection", "close"); XMLHttpRequestObject.send(params); XMLHttpRequestObject.onreadystatechange = stateChangeFunc; } } function addToCart(val) { if(val==undefined) { if(XMLHttpRequestObject.readyState==4 && XMLHttpRequestObject.status==200) // handle http return { try { // put in your user notification here. You can figure that out yourself, use DOM or a dumb alert if lazy alert ("Successful"); } catch(err) { alert('An error occured'); } } } else // make http request { var params = 'func=checkAddToCart'; httpPost('cart_functions.php', params, checkAddToCart); } } //--> </script> As you pointed out, I also changed my <form> call to pass the '1' parameter as follows: <form ACTION="view_cart.php" method="POST" name="addtocartform" id="addtocartform" onSubmit="return validateAddToCart(); addToCart(1)"> Finally, I created a php file called cart_functions.php. Here it is: <?php /* POST: determine which function the HTTP POST request wants */ if($_POST[func]) { switch($_POST[func]) { case 'checkAddToCart': // current session id $sid = session_id(); // check if the product is already // in cart table for this session // (RecordID was passed as a variable from master to detail) $sql = "SELECT pd_id FROM tbl_cart WHERE pd_id = $RecordID AND ct_session_id = '$sid'"; //$result = dbQuery($sql); $result = mysql_query($sql) or die(mysql_error()); if (mysql_num_rows($result) == 0) { // put the product in cart table $sql = "INSERT INTO tbl_cart (pd_id, ct_qty, ct_session_id, ct_date) VALUES ($RecordID, 1, '$sid', NOW())"; $result = mysql_query($sql) or die(mysql_error()); } else { // update product quantity in cart table $sql = "UPDATE tbl_cart SET ct_qty = ct_qty + 1 WHERE ct_session_id = '$sid' AND pd_id = $RecordID"; $result = mysql_query($sql) or die(mysql_error()); } break; } return; } ?> I'm not sure if I did everything right, but it doesn't seem to be hitting the call. Do I need to include my cart_functions.php file at the top of the detail page? I didn't think that was necessary as it's being called directly during the http request. Please let me know if I missed anything. Thanks to all who read this. -
PHP automatically called from within javascript function?
vozzek replied to vozzek's topic in PHP Coding Help
I just took the Ajax tutorial on Tizag.com (love that site) but it was a bit short. If anyone has any other good Ajax resources, I'm open to suggestions. Thanks for the response everyone. -
Hi all, I've got a javascript function called addToCart() that gets called OnSubmit from my detail page. Since it's updating my SQL database (the shopping cart table) the function is 99% php... I just wrapped it up in javascript to make it easy to call from html. The problem I'm having is the function is adding the item to the shopping cart every time the page loads. Obviously I only want this to happen when and if the ADD TO CART button is clicked. Here is the <form> portion of the code that calls the addToCart script (the validateAddToCart script happening before it is working perfectly so in the interest of space I'm not going to include it). <form ACTION="view_cart.php" method="POST" name="addtocartform" id="addtocartform" onSubmit="return validateAddToCart(); addToCart()"> And now here is the javascript function addToCart, made up of the php code: <script type="text/javascript"> <!-- function addToCart() { <?php // current session id $sid = session_id(); // check if the product is already // in cart table for this session // (RecordID was passed as a variable from master to detail) $sql = "SELECT pd_id FROM tbl_cart WHERE pd_id = $RecordID AND ct_session_id = '$sid'"; //$result = dbQuery($sql); $result = mysql_query($sql) or die(mysql_error()); if (mysql_num_rows($result) == 0) { // put the product in cart table $sql = "INSERT INTO tbl_cart (pd_id, ct_qty, ct_session_id, ct_date) VALUES ($RecordID, 1, '$sid', NOW())"; $result = mysql_query($sql) or die(mysql_error()); } else { // update product quantity in cart table $sql = "UPDATE tbl_cart SET ct_qty = ct_qty + 1 WHERE ct_session_id = '$sid' AND pd_id = $RecordID"; $result = mysql_query($sql) or die(mysql_error()); } ?> } //--> </script> So I guess my question is, am I going about this the right way? I thought the php code would only get executed when the addToCart function was called, and not upon page load. Am I wrong? Thanks in advance.
-
Okay, if I can use php to call a php function, can I do something like this? <form ACTION="view_cart.php" method="POST" name="addtocartform" id="addtocartform" onSubmit="return validateAddToCart(); <?php addToCart() ?>"> And if the validate fails, will it still execute the addToCart function?
-
Ah, okay. I wasn't sure if this was possible but was hoping it was. I know exactly what you mean. Thanks.
-
Hi everyone, I'm trying to use the include function for the first time, and I can't get it to work. I'm sure it's something obvious, but could someone please tell me what's wrong? I have two files: test.php which calls cart_functions_test.php (both are in the same directory). test.php <?php include('cart_functions_test.php'); ?> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Test Page</title> </head> <body> <input name="Click Me" type="button" value="Click Me" onclick="danny()" /> </body> </html> cart_functions_test.php <?php function danny() { echo "I am here"; } ?> When I click the button, nothing happens. What am I missing? Thanks in advance. Sorry for the duplicate post... MERGED
-
Well, Mickey-Mouse strikes again. The answer lies in daylight savings time: http://kb.adobe.com/selfservice/viewContent.do?externalId=kb402776&sliceId=2 This is why it stopped working last week. Thanks for the help, you php freaks! -Danny
-
... Dreamweaver CS3 First off, yes, I know Dreamweaver is a big steaming pile of Mickey Mouse dog crap. I was new when I bought it and I'm past that. Second, I'm very new to php and my code probably sucks. So please go easy on me, and I'm certainly open to alternate and better ways to do this. The code below is all mine, meaning I wrote it from scratch and didn't use any Romper Room DW php builder to create it. Quick rundown: I'm populating a detail page from my SQL database. If an option (for example "option_a") exists, I want the dropdown list to populate with all available selections for that particular option. An example for option_a would be 'Color', and the listbox selections (a1, a2, a3...) would be something like "blue", "green", or "red". The code is attached below: <?php if (!empty($row_rs_detail['option_a'])) { ?> <label> <?php echo $row_rs_detail['option_a'].":"; ?> <select name=<?php echo $row_rs_detail['option_a']; ?> id=<?php echo $row_rs_detail['option_a']; ?>> <?php echo "<option selected='yes'>Please Select</option>"; if (!empty($row_rs_detail['option_a1'])) { echo "<option>{$row_rs_detail['option_a1']}</option>"; } if (!empty($row_rs_detail['option_a2'])) { echo "<option>{$row_rs_detail['option_a2']}</option>"; } if (!empty($row_rs_detail['option_a3'])) { echo "<option>{$row_rs_detail['option_a3']}</option>"; } ?> </select> </label> <?php } ?> DW is crashing the SECOND I close that </select> tag near the end. I was wondering if anything in my php code should be causing this crash to happen. The really strange thing is this was working for several weeks. All of a sudden, it doesn't work anymore. I wonder if there's a control character in there or something, or maybe I deleted an important character? Thanks in advance for the help.
-
Hi everyone, real quick question: I have a 'send to friend' email form working just fine from Firefox, but in Internet Explorer the link is not showing up. Here's what I've got: <?PHP $site = $_SERVER['HTTP_REFERER']; ?> My form uses "link" to store the $site variable. <input type="hidden" name="link" value="<?PHP echo $site; ?>"> It calls another .php link where it posts the message using the global function: <?PHP global $_POST; $uname = $_POST["yourname"] ; $uemail = $_POST["youremail"]; $fname = $_POST["friendsname"]; $femail = $_POST["friendsemail"]; $message = $_POST["message"]; $link = $_POST["link"]; $to = "$femail"; $subject = "$uname Wants You To Check Out This Website"; $headers = "From: $uemail\n"; $message = "Hello $fname. $uname would like you to visit $link\n $message"; if (preg_match(' /[\r\n,;\'"]/ ', $_POST['uemail'])) { exit('Invalid Email Address'); } else { mail($to,$subject,$message,$headers); } ?> The mail script works great, and on Firefox I can see and click the link. In I.E. the link is blank, as I said. Any obvious reason for this? Thanks in advance for the help.
-
Hi everyone, I'm working on an edit inventory form, and I'm pulling a field from my database that's going to be a 'Y' or 'N'. Based upon this, I want to default the 'customizable' radio button to either checked="Y" or checked="N". <tr valign="baseline"> <td align="right" valign="top" nowrap="nowrap">Customizable:</td> <td valign="baseline"><table> <tr> <td><input onclick="document.form1.character_limit.disabled = false" type="radio" name="customizable" value="Y"/> Yes</td> <?php if ($row_rs_edit_inventory['customizable']=='Y') { ?> <script> document.form1.customizable.checked='Y' </script> <?php }else{ ?> <script> document.form1.customizable.checked='N' </script> <?php } ?> </tr> <tr> <td><input onclick="document.form1.character_limit.disabled = true" type="radio" name="customizable" value="N"/> No</td> </tr> </table></td> </tr> Not sure why, but this isn't working. No matter what's in the database, the radio button is defaulting to 'Y'. Does it matter whether I place this code in the 'Y' input portion of the radio button or the 'N'? Should I put it before both inputs? Not sure. I'm also pretty sure I'm going about this the hard way. Any ideas? Thanks in advance. EDIT: Came up with something else (much simpler), but it doesn't work either: <script> if <?php echo $row_rs_edit_inventory['customizable']; ?>=='Y' document.form1.customizable.checked='Y' else document.form1.customizable.checked='N' </script> Sorry in advance is this is all newbie stuff, I'm still learning.
-
[SOLVED] Enabling a disabled textbox within a form
vozzek replied to vozzek's topic in Javascript Help
Ahhhhh, crap! Sometimes the most obvious things are the hardest to solve. You nailed it. I changed: input onclick="document.character_limit.disabled = true" to input onclick="document.form1.character_limit.disabled = true" and I am now a happy camper. Thanks fenway! -
Hi everyone, Java newbie here, so please bear with me. I really tried this 10 different ways by now, and only came here when I was absolutely stumped. I've got a form (form1) with 2 fields. One is a Y/N radio button called 'customizable'. The other is a text field called 'character_limit'. I want 'customizable' defaulted to 'N' (already have that) with the 'character_limit' field disabled. But when 'customizable' is clicked to 'Y', I want 'character_limit' to be enabled. I've tried this directly with the following code: <tr valign="baseline"> <td align="right" valign="top" nowrap="nowrap">Customizable:</td> <td valign="baseline"><table> <tr> <td><input onclick="document.character_limit.disabled = false" type="radio" name="customizable" value="Y" /> Yes</td> </tr> <tr> <td><input onclick="document.character_limit.disabled = true" type="radio" name="customizable" value="N" checked="N" /> No</td> </tr> </table></td> </tr> <tr valign="baseline"> <td nowrap="nowrap" align="right">Character Limit:</td> <td><input name="character_limit" type="text" value="" size="32" maxlength="3" disabled="disabled"/> </td> </tr> I also tried creating a function that looks like this: <script type="text/javascript"> <!-- function disable_enable(){ if (document.all || document.getElementById){ if (document.form1.customizable.value=='Y') document.form1.character_limit.disabled=false else document.form1.character_limit.disabled=true } } </script> The function looks good, but I'm not sure exactly where (or how) to place the code that calls that function. Sorry if this is a pretty elementary question, but I'm still learning. Thanks in advance for the help.
-
I sheepishly admit I don't know what to put within the <script> tag. I'm guessing I need to define an onclick function or nothing happens? I'm extremely new to java (having just started messing with php), so forgive me if I sound like a total noob.
-
Great advice haaglin, thanks. I've just only started learning javascript, and it looks like I should probably finish the tutorial(s) I'm taking before moving too far forward. One thing: Do I need a semicolon after the quotes in the input function? Because it's still not working. I added <script type="text/javascript"> <!-- //--> </script> to the <head> portion of the page.
-
Hi everyone, Maybe I'm going about this the wrong way (or the more complicated way...) but I'm trying to use PHP to conditionally disable a field within a form. It's not working out for me, and I don't know why. Here's the scenario: I have a field called "customizable" which is a Y or N radio box (defaulting to N). The next field is "character_limit". If "customizable" is set to N, I want this field grayed out (disabled?) But if someone checks the "Y" box for "customizable", I want this field to be activated. Below is the code I tried, showing both fields. (Note that both fields are contained within the same form): <tr valign="baseline"> <td align="right" valign="top" nowrap="nowrap">Customizable:</td> <td valign="baseline"><table> <tr> <td><input type="radio" name="customizable" value="Y" <?php if (!(strcmp("Y","Y"))) {echo "CHECKED";} ?> /> Yes</td> </tr> <tr> <td><input type="radio" name="customizable" value="N" checked="N" <?php if (!(strcmp("Y","N"))) {echo "CHECKED";} ?> /> No</td> </tr> </table></td> </tr> <tr valign="baseline"> <td nowrap="nowrap" align="right">Character Limit:</td> <td><input name="character_limit" type="text" value="" size="32" maxlength="3" <?php if ($_GET['customizable']="N") { ?> <?php echo "disabled=\"disabled\""; } ?> /></td> </tr> What am I doing wrong, and is there an easier way to do this? Thanks in advance.
-
Thanks Liam. I tried what you said and was able to see the image in a different file, so I figured Gmail must be blocking the image using the traditional <img> html tag. However... my cousin sends me emails (through Gmail) all the time, and the logo of his mortgage company is always embedded at the bottom of the mail. So there must be a way to do it. When I click on the element properties of the image in one of his emails it says: http://mail.google.com/mail/?attid=0.1&disp=emb&view=att&th=1155d0ab78e4c1b4 The disp=emb and view=att must have something to do with allowing me to see his image, but truth be told it's all greek to me...
-
Hi all, Quick question: I've got a php script calling some java code that executes a "send to friend" email from the url of my website to a chosen email address. Works great. Last thing I need is to maybe embed a small company logo within the body of the email, so when they open it up they can see it. I've tried different things with the code below (do I maybe have to echo it?), but can't get more than a link to show up: <?PHP global $_POST; $uname = $_POST["yourname"] ; $uemail = $_POST["youremail"]; $fname = $_POST["friendsname"]; $femail = $_POST["friendsemail"]; $message = $_POST["message"]; $link = $_POST["link"]; $to = "$femail"; $subject = "$uname Wants You To Check Out This Website"; $headers = "From: $uemail\n"; $logo = "<img src='http://www.mywebsite.com/images/small_logo.jpg'></img>"; $message = "Hello $fname. $uname wants you to visit $link\n $logo\n $message"; if (preg_match(' /[\r\n,;\'"]/ ', $_POST['uemail'])) { exit('Invalid Email Address'); } else { mail($to,$subject,$message,$headers); } ?> The red code is the stuff I added to try and get the image to show up. Any ideas? Thanks in advance.
-
Ah, I should probably smack myself in the forehead for not trying concatenation. Thanks! Someone else just pointed this out to me also. However, it still doesn't pass the 'id' value. I think your syntax is 100% correct but unfortunately since this is the posting form, the 'id' value hasn't actually been defined yet (at the time) if that makes any sense. It's a self-incrementing element of the database, so I guess maybe it happens after the posting? I think I'm trying to do the database call before the element is populated... dunno. Still confused.