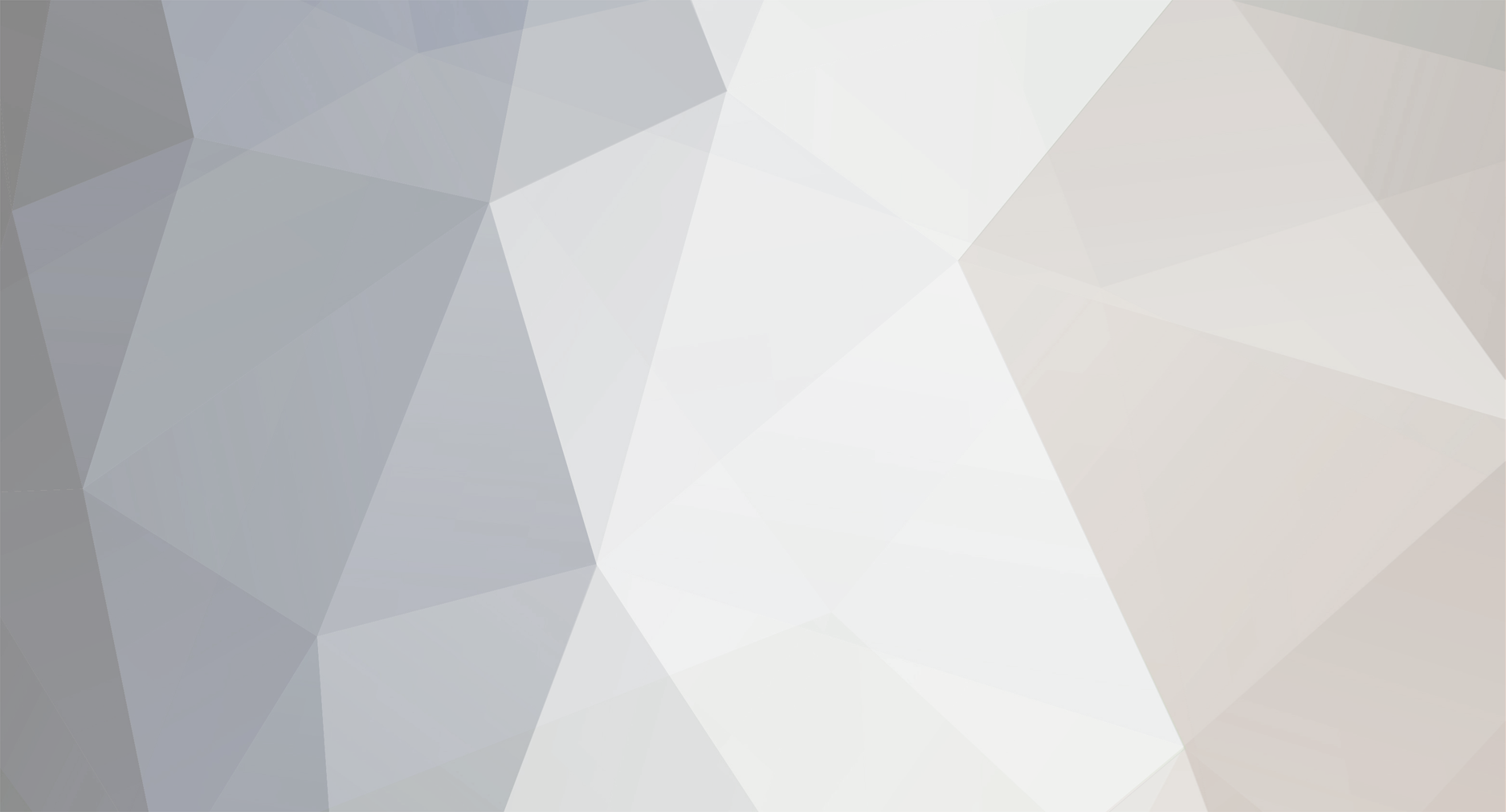
mikefrederick
-
Posts
247 -
Joined
-
Last visited
Never
Posts posted by mikefrederick
-
-
is there anyway to put & in a url and not have php thing that a GET variable in the URL has ended?
-
What is the real danger of XSS? If you can't inject PHP into the page then isn't any change you make just going to show up on your own computer?
-
minus the ) in the timestart()
-
why doesnt this work?
$getdates=mysql_query("select *, time_format(timestart),'%g:%i %a') as date_f from events order by date_f asc");
-
anyone?
-
sure, it will reverse the order but it does not make the field sensitive to am or pm.
-
i have a field in a table that contains times of the day in the format 11:00 am, 10:20 pm, etc. If I order by that field, then the pm dates will still come up before the am ones. For example, since 10:20 is less than 11 it will come before 11, but I need it to come after since it is actually later in the day. Is there anyway to do this without breaking the am/pm part into another field?
-
I fixed the repetition with the following code, now I just need the results to only show up if they contain ALL of the different words in the searches...
<?php foreach ($new as $currItem) { for($u=1; $u<=count($explodesearch); $u++) { $test.="strtoupper($explodesearch[$u]) == strtoupper($currItem) &&"; } if (strtoupper($explodesearch[0]) == strtoupper($currItem) && $test){ $i=$i+1; print "<tr><td align='center' class='daytime' width='20%'>$startsss</td><td align='left' class='daytitle' style='padding-left:10px'>";?>
-
hey I am making a search function that is currently producing correctly if you search for one word. if you search for two words, it is showing up any strings that return either word in the search, and if a string contains both words then it shows up twice. I need help fixing this....thanks for looking:
<?php $query=mysql_query("select * from whatever order by whatever"); while($sql=mysql_fetch_assoc($query)) { $explode=explode(" ",$sql['description']); $extitle=explode(" ",$sql['title']); $startss=$sql['start']; $startss=explode("-",$startss); $startss=mktime(0,0,0,$startss[1],$startss[2],$startss[0]); $startsss=date('F j, Y',$startss); $endss=$sql['end']; $endss=explode("-",$endss); $endss=mktime(0,0,0,$endss[1],$endss[2],$endss[0]); $endsss=date('F j, Y',$endss); $new=$extitle+$explode; $explodesearch=explode(" ",$search); $i==0; foreach ($new as $currItem) { for($u=0; $u<=count($explodesearch); $u++) { if (strtoupper($explodesearch[$u]) == strtoupper($currItem)){ print "<tr><td align='center' class='daytime' width='20%'>$startsss</td>"; } } } ?>
-
are you trying to insert something into the database? if so, :
mysql_query("insert into tablename (field1,field2) values ('value1','value2')");
-
use curly brackets with your if statements.
also, it might not be a great idea to use $ext = $ext[count($ext)-1];
you might consider using a variable name other than $ext.
-
$query = mysql_query("UPDATE INTO article WHERE id IN ($nupdates) ")
VALUES (NULL, '$title', '$tagline', '$content', now( ) , '$author', '$category')");
you forgot the ending bracket
-
fixed it, nevermind
-
even if i change the function so that it does not clear any rows it will still delete the last one. i cant figure this out...
-
sorry again, my mistake, it is being deleted and is not printing with print_r
-
sorry, my mistake, it turns out the last row is still there just not being printed
-
when i print_r after deletion, it shows all rows except the last one, and the one that was supposed to be deleted shows as:
[4] => Array
(
[id] =>
[title] =>
[description] =>
[visible] =>
[contact] =>
[dates] =>
[hours] =>
)
so it looks like it unsets one row and deletes the last.
-
i tried switching that line to <=10 earlier just to see if that was an issue, but it still produced the same result. i assumed this meant that the error did not lie in that line...
-
this is also an important line:
<?php function remove($pos) { unset($this->rows[$pos]); }
-
to save time, these are important lines:
function delete($id) { $this->editor->remove($this->index[$id]); $this->commit(); $this->refreshIndex(); }
$id = $jobsList[4]["id"]; print "Delete ".$id."\n"; $jobs->delete($id); print "Commit\n"; $jobs->commit(); print "\n"; print "Debug Dump After Deleting 1 Job:\n"; $jobsList = $jobs->getList(); for ($loopi=0; $loopi<sizeof($jobsList); $loopi++) { $job = $jobsList[$loopi]; if (strcmp($job["id"],"") !== 0) { print "id=".$job["id"]."; title=".$job["title"]."; description=".substr($job["description"],0,10)."... \n"; } }
-
thanks to anyone that can help ahead of time.....
What you'll see is this script adds 10 elements, and then deletes one from the middle of the list. When it deletes the one from the middle of the list, it also deletes one from the end, which shouldn't occur. You can see the script in action at:
http://scripts.loado.com/vahldebug/test.php
now for the code:
test.php:
<? require("jobs_general.php"); ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN"> <html> <head> <title>Test</title> </head> <body> <pre> <? // $id = 0; // truncate test.dsv $fh = fopen("test.dsv", 'w'); fclose($fh); // instantiate class $jobs = new GeneralJobs(); for ($loopi=0; $loopi<10; $loopi++) { print "Add "."title".$loopi."\n"; $jobs->add("title".$loopi,"description".$loopi,1,"contact".$loopi,"dates".$loopi,"hours".$loopi); } print "Commit\n"; $jobs->commit(); print "\n"; // print "Debug Dump After Adding 10 Jobs:\n"; $jobsList = $jobs->getList(); for ($loopi=0; $loopi<sizeof($jobsList); $loopi++) { $job = $jobsList[$loopi]; print "id=".$job["id"]."; title=".$job["title"]."; description=".substr($job["description"],0,10)."... \n"; //$id = $job["id"]; } print "\n"; $id = $jobsList[4]["id"]; print "Delete ".$id."\n"; $jobs->delete($id); print "Commit\n"; $jobs->commit(); print "\n"; print "Debug Dump After Deleting 1 Job:\n"; $jobsList = $jobs->getList(); for ($loopi=0; $loopi<sizeof($jobsList); $loopi++) { $job = $jobsList[$loopi]; if (strcmp($job["id"],"") != 0) { print "id=".$job["id"]."; title=".$job["title"]."; description=".substr($job["description"],0,10)."... \n"; } } print "\n"; ?> </pre> </body> </html>
jobsgeneral.php:
<?php // Includes require_once($_SERVER["DOCUMENT_ROOT"]."/vahldebug/dsveditor.php"); /** */ class GeneralJobs { var $editor; var $index; /** */ function GeneralJobs() { $this->index = array(); $this->editor = new DSVEditor(); $this->editor->setDelimiter("|"); $this->editor->setPath("test.dsv"); $this->editor->load(); $this->refreshIndex(); } /** */ function add($title,$description,$visible,$contact,$dates,$hours) { $id = $this->getUniqueId(); $row["id"] = $id; $row["title"] = $title; $row["description"] = $description; $row["visible"] = $visible; $row["contact"] = $contact; $row["dates"] = $dates; $row["hours"] = $hours; $this->editor->addRow($this->rowUnhashed($row)); $this->refreshIndex(); return $id; } /** */ function commit() { $this->editor->save(); $this->refreshIndex(); } function delete($id) { $this->editor->remove($this->index[$id]); $this->commit(); $this->refreshIndex(); } /** */ function edit($id,$title,$description,$visible,$contact,$dates,$hours) { $row = $this->rowHashed($this->editor->getRow($this->index[$id])); $row["id"] = $id; $row["title"] = $title; $row["description"] = $description; $row["visible"] = $visible; $row["contact"] = $contact; $row["dates"] = $dates; $row["hours"] = $hours; $this->editor->setRow($this->index[$id],$this->rowUnhashed($row)); $this->refreshIndex(); } /** */ function get($id) { $row = $this->editor->getRow($this->index[$id]); return $this->rowHashed($row); } /** */ function getAdminUrl() { return "/admin/jobs"; } /** */ function getList() { $list = array(); if (is_object($this->editor)) { for ($loopi=0; $loopi<sizeof($this->editor->getRows()); $loopi++) { array_push($list,$this->rowHashed($this->editor->getRow($loopi))); } } return $list; } /** */ function getUniqueId() { $randomid = 0; do { srand(time()); $randomid = (rand()%999999)+100000; } while (array_key_exists($randomid,$this->index)); return $randomid; } /** */ function refreshIndex() { $this->index = array(); if (is_object($this->editor)) { $rows = $this->editor->getRows(); for ($loopi=0; $loopi<sizeof($rows); $loopi++) { $title = $rows[$loopi][0]; if (strcmp($title,"") != 0) { $this->index[$title] = $loopi; } } } } /** */ function rowHashed($arr) { $hash = array(); $hash["id"] = $arr[0]; $hash["title"] = $arr[1]; $hash["description"] = $arr[2]; $hash["visible"] = $arr[3]; if (is_string($hash["visible"])) { $hash["visible"] = ((strcmp($hash["visible"],"true")==0) ? true: false ); } $hash["contact"] = $arr[4]; $hash["dates"] = $arr[5]; $hash["hours"] = $arr[6]; return $hash; } /** */ function rowUnhashed($hash) { $arr = array(); $arr[0] = $hash["id"]; $arr[1] = $hash["title"]; $arr[2] = $hash["description"]; $arr[3] = $hash["visible"]; $arr[4] = $hash["contact"]; $arr[5] = $hash["dates"]; $arr[6] = $hash["hours"]; return $arr; } } ?>
dvseditor.php
<? /** Delimiter Seperated Value Editor */ class DSVEditor { var $baseDir = ""; var $path = ""; var $rows; var $delimiter; /** Constructor */ function DSVEditor() { $this->init(); } /** Initialize */ function init() { $this->baseDir = $_SERVER["DOCUMENT_ROOT"]."/vahldebug"; $this->rows = array(); $this->delimiter = "&"; } function addRow($items) { $this->append($items); } /** */ function append($items) { if (is_array($items)) { for ($loopi=0; $loopi<sizeof($items); $loopi++) { if (is_string($items[$loopi])) { $items[$loopi] = urlencode($items[$loopi]); } } array_push($this->rows,$items); } else { array_push($this->rows,$items); } } /** */ function getDelimiter() { return $this->delimiter; } /** */ function getId() { $id = ""; return $id; } /** */ function getRow($position) { return $this->read($position); } /** */ function getRowCount() { return sizeof($this->rows); } /** */ function getRows() { return $this->rows; } /** */ function getRowsAsString() { $content = ""; for ($loopi=0; $loopi<sizeof($this->rows); $loopi++) { $line = ""; for ($loopj=0; $loopj<sizeof($this->rows[$loopi]); $loopj++) { if ($loopj > 0) { $line .= $this->delimiter; } $line .= $this->rows[$loopi][$loopj]; } if (strcmp($line,"") != 0) { $content .= $line."\n"; } } //foreach ($this->items as $hkey => $hval) { // $content .= $hkey."=".urlencode($hval)."\n"; //} return $content; } /** */ function getPath() { return $this->path; } /** */ function getUniquePath($subDir) { $pathFull = ""; $pathSub = ""; do { srand(time()); $randomid = (rand()%999999999999)+10000000000; $pathSub = $subDir."/".$randomid.".kvp"; $pathFull = $this->baseDir."/".$pathSub; } while (file_exists($pathFull)); touch($pathFull); return $pathSub; } /** */ function load() { $path = $this->path; $handle = @fopen($path, "r"); if ($handle) { while (!feof($handle)) { $buffer = fgets($handle, 4096); $buffer = trim($buffer); if (strcmp($buffer,"") != 0) { $items = explode($this->delimiter,$buffer); array_push($this->rows,$items); } //echo $buffer; } fclose($handle); } return $this->rows; } /** */ function read($position) { $cols = array(); for ($loopi=0; $loopi<sizeof($this->rows[$position]); $loopi++) { $cols[$loopi] = urldecode($this->rows[$position][$loopi]); //print $loopi.": ".$this->rows[$position][$loopi]."=".$cols[$loopi]."<br>\n"; } return $cols; } /** */ function readAll() { $rows = array(); for ($loopi=0; $loopi<sizeof($this->rows); $loopi++) { $rows[$loopi] = $this->read($loopi); } return $rows; } /** */ function remove($pos) { unset($this->rows[$pos]); } /** */ function save() { $path = $this->path; // Let's make sure the file exists and is writable first. //if (!file_exists($path)) { // In our example we're opening $filename in append mode. // The file pointer is at the bottom of the file hence // that's where $somecontent will go when we fwrite() it. //print $path; if (!$handle = fopen($path, 'w')) { echo "Cannot open file ($path)"; return; } $content = $this->getRowsAsString(); // Write $somecontent to our opened file. if (fwrite($handle, $content) === FALSE) { echo "Cannot write to file ($path)"; return; } //echo "Success, wrote ($somecontent) to file ($filename)"; fclose($handle); //} else { // echo "The file $path already exists"; //} } /** */ function setBasePath($basePath) { $this->baseDir = $basePath; } /** */ function setDelimiter($d) { $this->delimiter = $d; } /** */ function setRow($pos,$row) { $this->write($pos,$row); } /** */ function setRows($rows) { $this->rows = $rows; } /** */ function setPath($subPath) { $this->path = $this->baseDir."/".$subPath; } /** */ function write($position,$items) { if (is_array($items)) { for ($loopi=0; $loopi<sizeof($items); $loopi++) { $items[$loopi] = urlencode($items[$loopi]); } $this->rows[$position] = $items; } else { $this->rows[$position] = $items; } } } ?>
test.dsv:
1063198|title0|description0|1|contact0|dates0|hours0 474962|title1|description1|1|contact1|dates1|hours1 1050692|title2|description2|1|contact2|dates2|hours2 995908|title3|description3|1|contact3|dates3|hours3 792054|title5|description5|1|contact5|dates5|hours5 340774|title6|description6|1|contact6|dates6|hours6 432525|title7|description7|1|contact7|dates7|hours7 161815|title8|description8|1|contact8|dates8|hours8
-
I am making a calendar, any way to determine if a specific date is a holiday?
-
so stupid....I was writing
$eventdates[$i][$t]=date('Y-n-j', $start);
instead of:
$eventdates[$i][$t]=date('Y-j-n', $start);
-
I am using this now, changed very little from before:
<?php for($i=0; $i<=30; $i++) { $lbound=$events_r[$i]["date_lbound"]; $ubound=$events_r[$i]["date_ubound"]; $explodelower[$i]=explode(" ",$events_r[$i]["date_lbound"]); $explodeupper[$i]=explode(" ",$events_r[$i]["date_ubound"]); $start = strtotime($explodelower[$i][0]); $end = strtotime($explodeupper[$i][0]); $t=0; $newdatee=$newyear . "-".$newmonth. "-" . $date; while ($start <= $end) { $eventdates[$i][$t]=date('Y-j-n', $start); if($eventdates[$i][$t]==$newdatee) { echo 'Y'; } $t++; $start = strtotime("{$explodelower[$i][0]} +{$t} days"); } } ?>
automatic email
in PHP Coding Help
Posted
is there a way to setup an email system such that you dont have to visit a page in order for emails to be sent? for example, i have a list of events and i want users to be emailed 2 days before the event. anyway for that to happen?