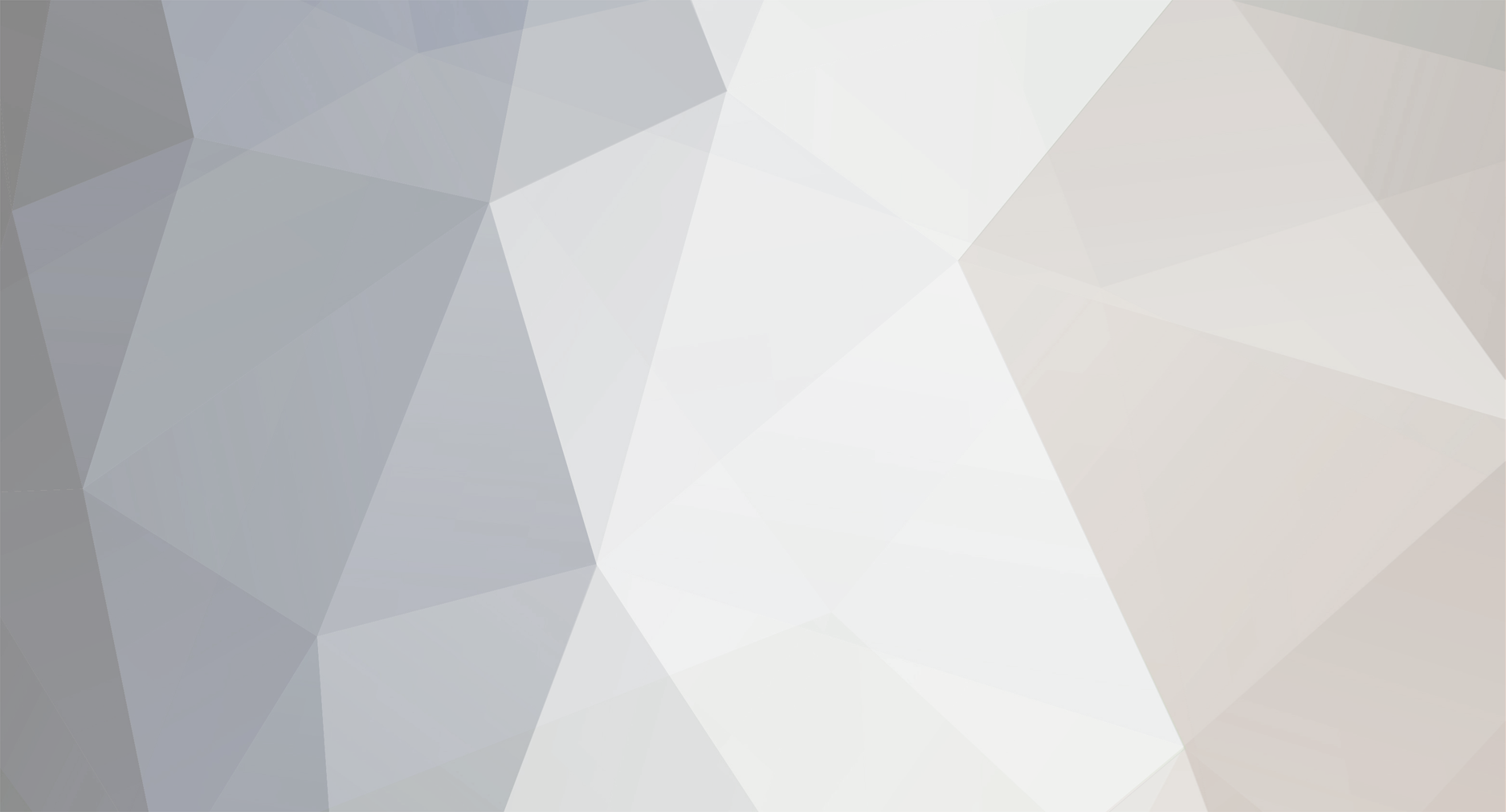
ngreenwood6
Members-
Posts
1,356 -
Joined
-
Last visited
Everything posted by ngreenwood6
-
i have 4 files: index.php <?php include("includes/variables.php"); ?> <html> <head> <script language="javascript" type="text/javascript" src="includes/login.js"></script> <link href="includes/style.css" rel="stylesheet" type="text/css" /> <title><?php echo $sitename; ?></title> </head> <body> <center> <h1>Login Here!</h1> <form name="login_form"> <table id="login_table"> <tr> <td>Username</td> <td>:</td> <td id="username"><input name="username" type="text"></td> <td id="username_empty"></td> </tr> <tr> <td>Password</td> <td>:</td> <td id="password"><input name="password" type="password"></td> <td id="password_empty"></td> </tr> <tr> <td></td> <td></td> <td><input type="button" name="submit_login" value="Log In" onClick="CheckLogin();"></td> </tr> </table> </form> </center> </body> </html> check_user.php <?php //include the variables include("includes/variables.php"); //define the variables from the form $username = strtolower($_POST['username']); $password = $_POST['password']; //connect to the database $connect = mysql_connect($host, $db_user, $db_pass) or die("could not connect"); //select the database $select_db = mysql_select_db($db) or die("could not select database"); //query for the database $query = "SELECT * FROM $table WHERE username='$username'"; //get the results $results = mysql_query($query); //number the rows $num_rows = mysql_num_rows($results); //put it into an array $row = mysql_fetch_array($results); //give the username and password from the database a variable $row_username = $row['username']; $row_password = $row['password']; //set the initial process value $process = TRUE; //if the username is blank if($username == "") { echo 1; $process = FALSE; } //if the password is blank if($password == "") { echo 2; $process = FALSE; } //if the username is not in the database if($num_rows < 1) { echo 3; $process = FALSE; } //if the password is not correct if ($password != $row_password) { echo 4; $process = FALSE; } //if username is in database if($usernamme == $row_username) { echo 5; } //if the password is correct if($password == $row_password) { echo 6; } ?> login.js function DoCallback(data) { // branch for native XMLHttpRequest object if (window.XMLHttpRequest) { req = new XMLHttpRequest(); req.onreadystatechange = processReqChange; req.open('POST', url, true); req.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); req.send(data); // branch for IE/Windows ActiveX version } else if (window.ActiveXObject) { req = new ActiveXObject('Microsoft.XMLHTTP') if (req) { req.onreadystatechange = processReqChange; req.open('POST', url, true); req.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); req.send(data); } } } function DoCallback2(data) { // branch for native XMLHttpRequest object if (window.XMLHttpRequest) { req = new XMLHttpRequest(); req.onreadystatechange = processReqChange; req.open('POST', url2, true); req.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); req.send(data); // branch for IE/Windows ActiveX version } else if (window.ActiveXObject) { req = new ActiveXObject('Microsoft.XMLHTTP') if (req) { req.onreadystatechange = processReqChange; req.open('POST', url2, true); req.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); req.send(data); } } } function processReqChange() { // only if req shows 'loaded' if (req.readyState == 4) { // only if 'OK' if (req.status == 200) { eval(what); } else { alert('There was a problem retrieving the XML data: ' + req.responseText); } } } var url = "check_user.php"; var url2 = "process_login.php"; var what = "LoginStatus(req.responseText)"; function CheckLogin() { var username = document.login_form.username.value; var password = document.login_form.password.value; DoCallback("username="+username+"&password="+password); } function LoginStatus(Status) { var username = document.login_form.username.value; var password = document.login_form.username.value; if(Status == 12356){ document.getElementById("username_empty").innerHTML="Please enter a username!"; document.getElementById("password_empty").innerHTML="Please enter a password!"; } if(Status == 2356){ document.getElementById("username_empty").innerHTML="That username is not in the database!"; document.getElementById("password_empty").innerHTML="Please enter a password!"; } if(Status == 24){ document.getElementById("username_empty").innerHTML=""; document.getElementById("password_empty").innerHTML="Please enter a password!"; } if(Status == 4){ document.getElementById("username_empty").innerHTML=""; document.getElementById("password_empty").innerHTML="That password is not correct!"; } if(Status == 345){ document.getElementById("username_empty").innerHTML="That username is not in the database!"; document.getElementById("password_empty").innerHTML="That password is incorrect!"; } if(Status == 6){ document.getElementById("username_empty").innerHTML=""; document.getElementById("password_empty").innerHTML=""; DoCallback2("username="+username+"&password="+password); } } and process_login.php <?php //include the variables include("includes/variables.php"); //get the username from the form $username = strtolower($_POST['username']); if($username != "") { header("Location: test.php"); } ?> This is actually a lot simpler than it seems. All it does is check the variables and if they are not correct it displays the error without reloading the page. Then if everything is correct it is supposed to process the login(process_login.php) and go to test.php. The problem is that it is not going to test.php which means that it is not processing the page how i want it to. My question is how do I get the ajax to stop and just allow the form to be processed hence taking me to test.php when the username and password are correct. Sorry for the long post but I figured I would show incase I was missing or had something incorrect. Any help is appreciated.
-
I am in need of some help with error handling. I have my main page: <?php include("includes/variables.php"); ?> <html> <head> <link href="includes/style.css" rel="stylesheet" type="text/css" /> <title><?php echo $sitename; ?></title> </head> <body> <center> <h1>Login Here!</h1> <form name="login_form" method="post" action="check_user.php"> <table id="login_table"> <tr> <td>Username</td> <td>:</td> <td><input name="username" type="text"></td> <td id="username_empty"></td> </tr> <tr> <td>Password</td> <td>:</td> <td><input name="password" type="password"></td> <td id="password_empty"></td> </tr> <tr> <td></td> <td></td> <td><input type="submit" name="submit_login" value="Log In"></td> </tr> </table> </form> </center> </body> </html> Then I have the check_user.php: <?php //include the variables include("includes/variables.php"); //define the variables from the form $username = strtolower($_POST['username']); $password = $_POST['password']; //connect to the database $connect = mysql_connect($host, $db_user, $db_pass) or die("could not connect"); //select the database $select_db = mysql_select_db($db) or die("could not select database"); //query for the database $query = "SELECT * FROM $table WHERE username='$username'"; //get the results $results = mysql_query($query); //number the rows $num_rows = mysql_num_rows($results); //put it into an array $row = mysql_fetch_array($results); //give the username and password from the database a variable $row_username = $row['username']; $row_password = $row['password']; //if the username is blank if($username == "") { echo "Please enter a username!"; } //if the password is blank else if($password == "") { echo "Please enter a password!"; } //if the username is not in the database else if($num_rows < 1) { echo "That username is not in the database!"; } //if the password is not correct else if ($password != $row_password) { echo "The password you entered does not match!"; } else { echo "You are good!"; } ?> At this point it checks that the username and password are not empty and that they are in the database and match the database. If everything is good it says "You are good!". When the user enters something incorrect it displays the error on a separate page. I want it to display the error on the same page without submitting the form. I know you can do it with ajax but am lost. Can someone please help?
-
I am not that dumb I used the database name. I was just using database for the example so you would know that i put the database name in there. Anyways i got it working.
-
It is giving me this error now: Error: No database selected with query SELECT * FROM users WHERE username='test' I even tried changing the: $select_db = mysql_select_db($db); to: mysql_select_db("database"); Any ideas?
-
does anyone know what the actual error means?
-
That looks ok but what does the javascript page look like. If we cant see what the page is doing we cant help you.
-
yeah it is. It looks like: $table = "table"; I also tried just putting the table name in there.
-
I have the following page: <?php //include the variables include("includes/variables.php"); //define the variable from the form $username = strtolower($_POST['username']); //connect to the database $connect = mysql_connect($host,$user,$pass) or die("could not connect"); //select the database $select_db = mysql_select_db($db); //query for the database $query = "SELECT * FROM $table WHERE username = '$username'"; //get the results $results = mysql_query($query); //number the rows $num_rows = mysql_num_rows($results); if ($num_rows == 1) { //put it into an array $row = mysql_fetch_array($results); } //give the username a variable $row_username = $row['username']; //if the username is not in the database if($num_rows < 1) { echo "That username is not in the database!"; } ?> Whenever I submit the form I get this error: Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in C:\wamp\www\check_user.php on line 21 Any ideas why?
-
Can you post some code for us to look at?
-
Ok it is best if you use the code tags to put the code into it makes it much easier to read. It is the # sign when you are posting. you can edit your post and add the tags before and after your code.
-
How to add +6 hour time offset in this script..
ngreenwood6 replied to Kibit's topic in Javascript Help
You could try something like this: var hours=new Date(hour); var currenthours = date+6; You will have to make different variables for the hour, mins and seconds. then when you want to display it you would display it like: var time = hours+":"+mins+":"+secs; hope this helps -
If you need some help I would suggest that you post the code that you are having problems with and also the problem that you are having. Just saying that it doesnt work and giving us a link doesnt do any good. Post the code and tell us what it isnt doing that it is supposed to.
-
I have a membership to another forum that allows you to thank someone for a post. I think that would be very nice to be able to thank someone for their help. Underneath their name you could show how many thanks they have been given and how many times today they have been thanked.
-
no problem. I dont know if you saw the link or not because I accidently put it into the code poriton but you can set the timezone in the date function. Check out www.php.net/date for more information.
-
Make the time your own time. For example if they are 3 hours behind you: <?php $hour = date(g); $minutes = date(i); $seconds = date(s); $my_hour = $hour + 3; echo "$my_hour:$minutes:$seconds"; ?> If that doesnt work for you you can check out [url]www.php.net/date[/url]. I believe there is a way to set the timezone.
-
I have 3 files: index.php <?php include("includes/variables.php"); ?> <html> <head> <script type="text/javascript" src="includes/login.js"></script> <link href="includes/style.css" rel="stylesheet" type="text/css" /> <script type="text/javascript" src="includes/check_username.js"></script> <title><?php echo $sitename; ?></title> </head> <body> <center> <h1>Login Here!</h1> <form name="login_form"> <table id="login_table"> <tr> <td>Username</td> <td>:</td> <td><input name="username" type="text"></td> <td id="username_empty"></td> </tr> <tr> <td>Password</td> <td>:</td> <td><input name="password" type="password"></td> <td id="password_empty"></td> </tr> <tr> <td></td> <td></td> <td><input type="button" name="submit_login" value="Log In" onClick="check_user(this.value);"></td> </tr> </table> </form> </center> </body> </html> login.js //check that a username has been put into the form function check_user(username, password) { //set value for form to be ready to submit var form_ready = true; //get username from form var username = document.login_form.username.value; //get password from form var password = document.login_form.password.value; //if nothing is set for username if(username == "") { //set the error for no username var no_username = "Please enter a username!"; //set the form to not be ready for submission form_ready = false; //If nothing is set show the error document.getElementById("username_empty").innerHTML=no_username; } else { //if something is there remove the error document.getElementById("username_empty").innerHTML=""; } //if nothing is set for password if(password == "") { //set the error for no password var no_password = "Please enter a password!"; //set the form to not be ready for submission form_ready = false; //if nothing is set for password show the error document.getElementById("password_empty").innerHTML=no_password; } else { //if something is set remove the error document.getElementById("password_empty").innerHTML=""; } if (form_ready == true) { alert("The form is ready"); } } and check_user.php <?php //include the variables include("includes/variables.php"); //get the variables from the form $username = $_POST['username']; $password = $_POST['password']; //connect to the database $connect = mysql_connect($host,$db_user,$db_pass); //select the database $select_db = mysql_select_db($db); //query the database $query = "SELECT * FROM $table WHERE username = '$username'"; //get the results $results = mysql_query($query); ?> What this does at this point is check to make sure that the user puts in a username and if not it tells them to enter a username. The check_user.php is not in use yet. What I want to be able to do is check the username and password before the form is submitted. If the username does not exist or the password is incorrect I want to be able to display those errors. At this point if they enter a username and password it alert's them that everything is good. The problem is that I am having a time trying to figure out how to pass the variables to the check_user.php form and how to display the error if it isn't there. Any help is appreciated.
-
Thank you for the response. That is very helpful and I will have to try and implement that into my code. Again thank you for your help it is appreciated.
-
Thanks for the help xtopolist never thought about doing something like that. Sorry I am a noob and I have a couple of books I have been reading and they dont really show real world experiences lol. I will use what you have shown me. I am not very good at arrays at this point though. Could you give me an example of how you would create an array for the errors?
-
Another reason is that after I check that the user enters a username and password I want to check in the database that the username exists. If it doesn't exist I want it to say username doesnt exist. Then if the username and password arent blank and the username is in the database, log the user in. If I do the commands separately like you said to. It will check each value independently. so I would have to create another if statement that logged the user in. The only problem is that because they are not together it checks those values and then it will still log the user in as long as the new statement that I create for the user to be logged in is true. I hope that makes sense to someone.
-
Ok that is how I originally had it but my question now is what about telling it to do something after all the variables are true. For example I want it to check the username then the password (if either are wrong display error) then if all of them pass do this. If I do it the way that you have it I will have to create a whole new if and it will not go through the username and password the get to the last statement(if that makes any sense). That is why I did it with the else if's. Does anyone know how I could accomplish this?
-
I am trying to set up some error handling with ajax so that it will be better for the user in the fact that they wont have to reload the page. I have 2 pages index.php: <?php include("includes/variables.php"); ?> <html> <head> <script type="text/javascript" src="includes/login.js"></script> <link href="includes/style.css" rel="stylesheet" type="text/css" /> <title><?php echo $sitename; ?></title> </head> <body> <center> <h1>Login Here!</h1> <form name="login_form"> <table id="login_table"> <tr> <td>Username</td> <td>:</td> <td><input name="username" type="text"></td> <td id="username_empty"></td> </tr> <tr> <td>Password</td> <td>:</td> <td><input name="password" type="password"></td> <td id="password_empty"></td> </tr> <tr> <td></td> <td></td> <td><input type="button" name="submit_login" value="Log In" onclick="check_user(this.value);"></td> </tr> </table> </form> </center> </body> </html> login.js: //check that a username has been put into the form function check_user(username, password) { //get username from form var username = document.login_form.username.value; //get password from form var password = document.login_form.password.value; if(username == "") { //set the error for no username var no_username = "Please enter a username!"; //If nothing is set show the error document.getElementById("username_empty").innerHTML=no_username; } else if(username != "") { //if something is there remove the error document.getElementById("username_empty").innerHTML=null; } else if(password == "") { //set the error for no password var no_password = "Please enter a password!"; //if nothing is set for password show the error document.getElementById("password_empty").innerHTML=no_password; } else if(password != "") { //if something is set remove the error document.getElementById("password_empty").innerHTML=null; } Now I know some php but I am trying to incorporate the ajax just so that it does it on the fly instead of having to load a new page everytime. It is more for learning experience. Anyways, the problem is that it displays the username error but it is not displaying the password error. The other problem is that when viewing it in firefox it shows the username error when nothing is set and when something is set it goes away, but in internet explorer when something is set it displays the words null. Any help on any of these issues is appreciated.
-
Thanks that is exactly what I was looking for. Nightslyr and F1Fan you guys are great. I dont understand why you cant just find simple tutorials with this information in it. In my opinion some of the people making tutorials assume that you have a good knowledge of php or ajax for this matter and use complex codes to show a tutorial. It would be nice if there was some tutorials out there that just showed basic commands and even explained each part of it. Maybe I will come up with something like that. Anyways thanks to the both of you and if you guys are ever in need of anything please let me know and I will be more than willing to help. EDIT: Oh yeah I meant to tell you too F1Fan that I am not using it for the log in I was just using that as a simple example lol.
-
Say I had this function function submitUser(str) { var url="get.php"; url=url+"?username="+str; url=url+"&sid="+Math.random(); xmlHttp.onreadystatechange=stateChanged; xmlHttp.open("GET",url,true) xmlHttp.send(null) then i had this php page <?php //database variables $host = "localhost"; $user = "user"; $pass = "pass"; $db = "database"; //get from the form $username = $_GET['username']; //connect to database mysql_connect($localhost,$user,$pass); //select database mysql_select_db($db); //query $query = "INSERT INTO table (username) VALUES ('$username')"; //insert the results $result = mysql_query($query); ?> That would take what the user inputs into the form and submit it into the database but like you said it is passed throught the url which is fine for this example but if I added the password it would not be good. How would I do the post for this example in the function and then how would the php page change? Another question I have is how do you pass more than one variable (for example adding the password the the function so that I can call this onclick of the submit button)?
-
I know how to do a php post. That is why I am in the AJAX forum. I am looking for how to submit the request to the php page in AJAX. Also would the php page then use post or get?
-
Ok thank you for the response. Can you show me an example of how to use the post then because I can use the get but I am not quite sure how to use the post. I am in the process of reading a book and it shows good examples of the get but not the post which is weird because all of the php books show the post and a little get. Anyways looked on google for a good example but they want to make everything complex. Any help is appreciated once again F1Fan!!