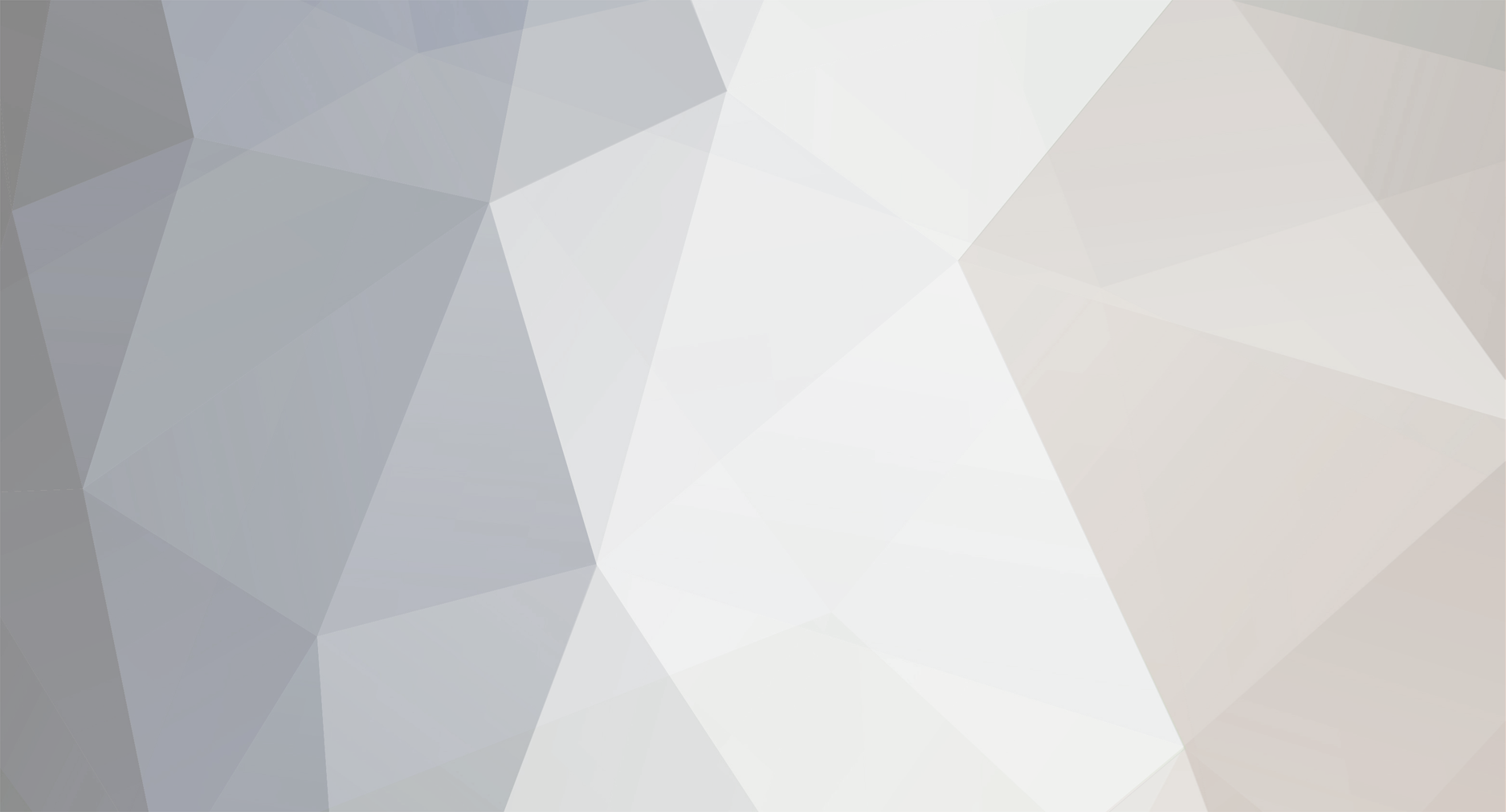
thebadbad
Members-
Posts
1,613 -
Joined
-
Last visited
Everything posted by thebadbad
-
Can't believe I couldn't figure it out. Thanks (I wanted to learn it too)
-
You're welcome And in the future, maybe consider using if, elseif, else if you want to do something if two conditions aren't met. <?php if (first condition) { //do something if first condition is met; } elseif (second condition) { //do something if first condition isn't met, but the second is; } else { //do something if none of the conditions were met; } ?> But it is always a good idea to exit your script after a header redirect.
-
Well, your last header() is run no matter what. Is that what you want? Adding an exit; very last inside the two if statements will fix it (causing the script to stop executing and thus not running your last header function).
-
When you refresh the script page, the browser (but maybe not all) WILL send the post data again. To solve your problem, you could header-redirect the user back to the form page (or just to the same script page like kopytko suggests). If the former, then you could print your 'success' message using $_GET. For example, use this instead of printing the messages: <?php header('Location: formpage.php?ok=true'); //success ?> <?php header('Location: formpage.php?ok=false'); //error ?> To display a success/error message in you form page, use: <?php if ($_GET['ok'] == 'true') { echo 'Added to database.'; } elseif ($_GET['ok'] == 'false') { echo 'Error.'; } ?>
-
I won't stop, will I? Made sure that the code I posted won't insert wrong stuff into the database when the category lines are passed: <?php $array = file('filename.txt'); if ($array) { foreach ($array as $key => $value) { if (strpos($value, "</a>") === false) {continue;} //skip the category lines $url = preg_replace("/.*href=\"(.*)\">.*/s", "$1", $value, 1); //retrieve URLs $title = preg_replace("/.*\">(.*)<\/a>.*/s", "$1", $value, 1); //retrieve titles mysql_query("INSERT INTO `table` (`url`, `title`) VALUES ('$url', '$title')"); //insert into database } } ?>
-
[SOLVED] list only entries starting on a certain letter
thebadbad replied to clown[NOR]'s topic in PHP Coding Help
Maybe this thread can help. Or this PHP Freaks guide (not as relevant). -
And, to remove the extra space added to the titles/URLs (caused by line breaks some how), add an s to the first preg_replace parameter: <?php $array = file('filename.txt'); if ($array) { foreach ($array as $key => $value) { $url = preg_replace("/.*href=\"(.*)\">.*/s", "$1", $value, 1); //retrieve URLs $title = preg_replace("/.*\">(.*)<\/a>.*/s", "$1", $value, 1); //retrieve titles mysql_query("INSERT INTO `table` (`url`, `title`) VALUES ('$url', '$title')"); //insert into database } } ?> I also added a check for the file before retrieving the strings.
-
Here's your pointer: file(). Then you could do this (read comments): <?php $array = file('filename.txt'); foreach ($array as $key => $value) { $url = preg_replace("/.*href=\"(.*)\">.*/", "$1", $value, 1); //retrieve URLs $title = preg_replace("/.*\">(.*)<\/a>.*/", "$1", $value, 1); //retrieve titles mysql_query("INSERT INTO `table` (`url`, `title`) VALUES ('$url', '$title')"); //insert into database } ?> Dunno about your last question. Didn't test the code, hope it works. EDIT: Just tested without the query part, had a small error in the last preg_replace; it's corrected now.
-
When you say your images aren't displayed, do you mean in your documents? I'm almost a 100% certain that image paths in your html tags (<img src="path_here">) won't be 'redirected'. Try double checking your paths.
-
If you're accessing files directly (like example.com/mypicture.jpg) the server won't redirect, but show you the file. At least that's what my Apache server does (and I'm using mod_rewrite as well).
-
How to prevent media link from playing when clicked
thebadbad replied to t_machine's topic in PHP Coding Help
Orio, it wasn't my intent to sound criticizing at all, the script you posted is useful and relevant I just wanted to make sure that t_machine knew about the security issues, in case he'd just copied and pasted the code. Who knows, he could be a newbie -
How to prevent media link from playing when clicked
thebadbad replied to t_machine's topic in PHP Coding Help
Orio, the script you linked to seems to have NO security concerning "../" paths and simple downloads of critical PHP files. Like the site mentions (but doesn't implement in the final script?!), you should always specify allowed download files, for example by serving allowed files via numbers (or different identifiers), and NOT file paths. Like serving the first specified allowed file when calling downloadscript.php?file=1 and so on. -
How to prevent media link from playing when clicked
thebadbad replied to t_machine's topic in PHP Coding Help
You can have a look at the notes/comments at php.net/readfile. There's lots of 'force download' scripts. -
For your first question, you can use: <?php $array = array(a => "filled", g => "also filled", h => "", c => "not empty"); //example array with one empty value foreach ($array as $key => $value) { if($value == "") {unset($array[$key]);} } ?>
-
If you have 6 input fields like Wuhtzu suggest, you should be able to use javascript (if it's possible in your application?) to disable all fields but the first one, and then enable the next field each time the previous is not empty anymore, if you get me?
-
[SOLVED] Problem serving HTML entities with javascript
thebadbad replied to thebadbad's topic in Javascript Help
Thanks mate, solved it -
Hey folks. I have a really annoying problem serving HTML entities with javascript. I have a hide/show function applied to a div, and it's triggered when I click a link. It's working great, but I also wanted the link text to change onclick, from "↓ Info" to "↑ Info" and vice versa. The problem is, that my javascript converts the ampersand to &, so that I end up with "↓ Info" and "↑ Info" in my HTML. My function looks like this: function toggle() { var link = document.getElementById("infobuttonlink"); var info = document.getElementById("info"); link.firstChild.nodeValue = (link.firstChild.nodeValue == "↓ Info") ? "↑ Info" : "↓ Info"; info.style.display = (info.style.display == "none") ? "block" : "none"; } I've tried using single quotes around the link text (in the javascript), and also using the unicode character \u0026 and the \x26 without any success. Please help
-
From my experience it's impossible to send a header with the 404 status code from within a PHP script (at least on an Apache server), because when a file is found, it's found. Likewise, you can't trick the server into not knowing about the script it's running.
-
Okay, if your URL's look like example.com/josh=birthday you could use explode() to get "josh" and "birthday": <?php $url = $_SERVER['REQUEST_URI']; // will set $url to "/josh=birthday" $removeslash = explode('/', $url); // $removeslash[1] now contains "josh=birthday" $bits = explode('=', $removeslash[1]); // $bits[0] contains "josh" // $bits[1] contains "birthday" ?>
-
If you mean example.com/index.php?josh=birthday, then $_GET['josh'] will contain "birthday". But I guess you want to use example.com/index.php?name=josh&event=birthday, where $_GET['name'] would contain "josh" and $_GET['event'] would contain "birthday".
-
[SOLVED] Thumbnail script working but still creating errors??
thebadbad replied to farkewie's topic in PHP Coding Help
Putting this in your while loop will skip the dots: if($filename == '.' || $filename == '..'){continue;} Like this i guess: while (false !== ($filename = readdir($dh))) { if($filename == '.' || $filename == '..'){continue;} $files[] = $filename; } EDIT: I had the variable names wrong at first, just fixed them. -
<?php $url = $_SERVER['REQUEST_URI']; //will set $url to "/Rates/USA/1/China/2" $bits = explode('/', $url); // $bits[1] contains "Rates" // $bits[2] contains "USA" // $bits[3] contains "1" // $bits[4] contains "China" // $bits[5] contains "2" ?>