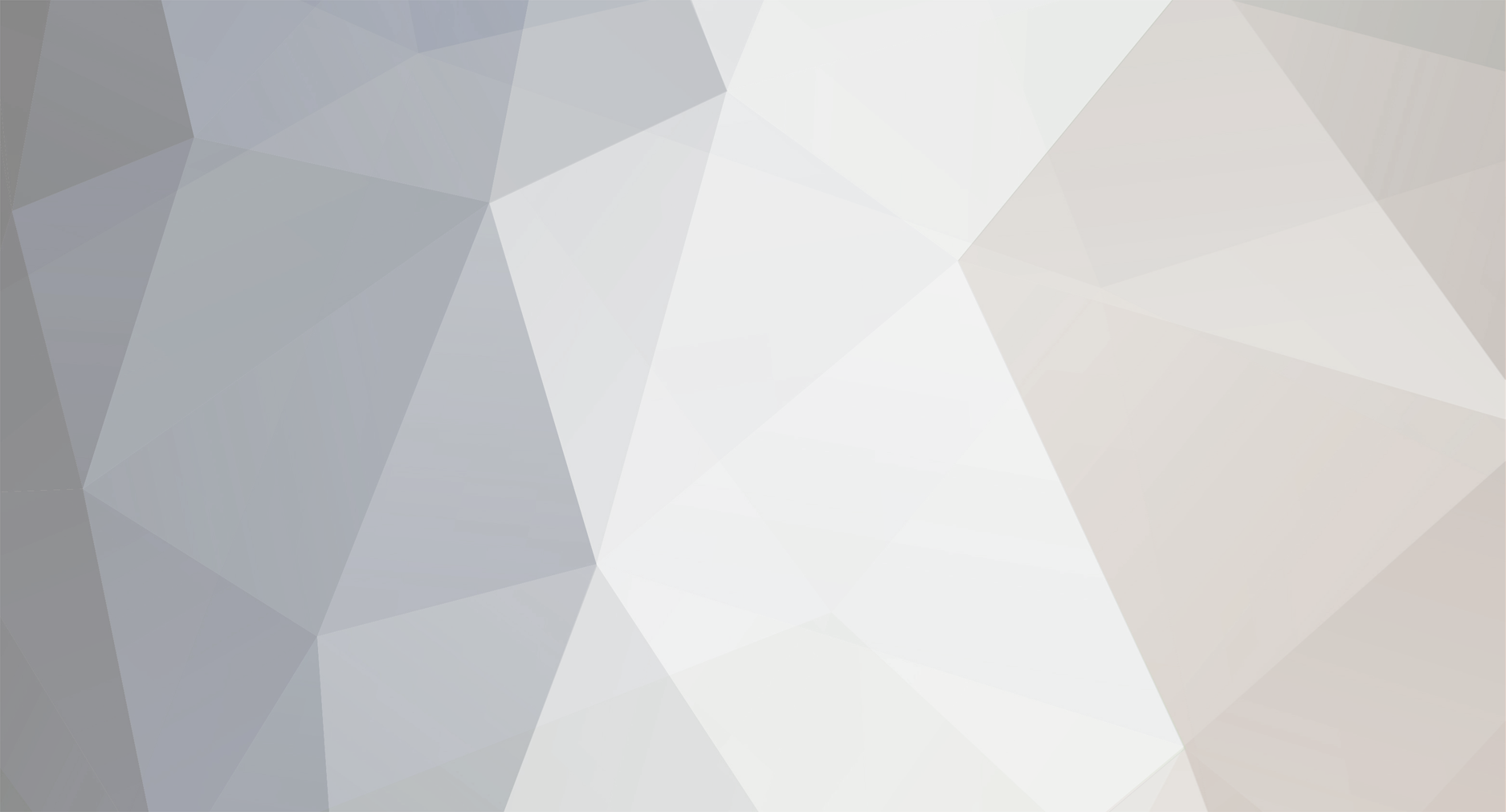
hinchcliffe
Members-
Posts
19 -
Joined
-
Last visited
Never
Everything posted by hinchcliffe
-
Hello, I'm currently grabbing data from an XML feed & with this data I'm updating product information in our database. We have over 2500+ products in our database. I'm trying to run a script that will go through and update every products price from the xml feed. My issue is that the xml feed only allows you to pull 50 products at once. So this is where I'm stuck. Right now I have a for each statement that limits to grabbing 50 products. <?php $i = 0; foreach ($products as &$value) { //product update code goes in here if (++$it == 50) break; } ?> Is there anyway I can continue where I left off and pull the next 50 products to update? I'm kind of lost.
-
Foreach Value's into a variable??? Need help.
hinchcliffe replied to hinchcliffe's topic in Applications
Thank you, this worked like a charm. -
Hello, I'm trying to update more than one products at once using Magento's API. I found the multiCall function and decided to give it a try. My code looks like this, but it doesn't seem to be working can someone guide me where I've gone wrong? <?php foreach ($xml->PartList->Sku as $PNAResponse) { $partNumber = $PNAResponse->PartNumber; $Storeprice = $PNAResponse->Price; // Update product price $calls[] = array('product.update', array($partNumber, array('price'=> $Storeprice))); } // Send the multi call $proxy->multiCall($sessionId, $calls); ?> Any help on this would be awesome. The API Wiki can be found here: http://www.magentocommerce.com/wiki/doc/webservices-api/introduction
-
Hello, Is there a way I can echo an for each variable outside the for each statement? <?php foreach ($products as &$value) { $partnumber = '<PartNumber>'.$value["sku"].'</PartNumber>'; echo $partnumber; } ?> I need $partnumber to be echoed in another piece of php code with out using the for each to grab each value. How can this be done?
-
Hello, Is there a way I can echo an for each variable outside the for each statement? <?php foreach ($products as &$value) { $partnumber = '<PartNumber>'.$value["sku"].'</PartNumber>'; echo $partnumber; } ?> I need $partnumber to be echoed in another piece of php code with out using the for each to grab each value. How can this be done? *EDIT* - Crap I posted in the wrong forum, still if you can help this would be nice.
-
Hi, I'm new to learning XML web services and I've been looking around on the web for a decent tutorial. So far I've failed to find one. Does anyone know any good resources for learning XML web services / Any prerequisite needed before starting? I've read through w3schools tutorials but they only show ASP.NET examples. Can you do SOAP XML Web Services through php? Thanks, Justin
-
Show Specials between certain times / days of the week.
hinchcliffe replied to hinchcliffe's topic in PHP Coding Help
Cool Thanks, Roopurt. Here's the code I have now that works. <?php $day = (date("D")); $time = (date("H") + 2); // Actuall Specials // Test Code if( $time >= 9 && $time <= 15 ) { // Mon if ($day == 'Mon'){ if ($time >= 0 && $time <= 11 ) { $special = 'Monday Breakfast Special'; }} if ($day == 'Mon'){ if ($time >= 11 && $time <= 16 ) { $special = 'Monday Lunch Special'; }} if ($day == 'Mon'){ if ($time >= 16 && $time <= 24 ) { $special = 'Monday Dinner Special'; }} // Tue if ($day == 'Tue'){ if ($time >= 0 && $time <= 11 ) { $special = 'Tuesday Breakfast Special'; }} if ($day == 'Tue'){ if ($time >= 11 && $time <= 16 ) { $special = 'Tuesday Lunch Special'; }} if ($day == 'Tue'){ if ($time >= 16 && $time <= 24 ) { $special = 'Tuesday Dinner Special'; }} // Wed if ($day == 'Wed'){ if ($time >= 0 && $time <= 11 ) { $special = 'Wednesday Breakfast Special'; }} if ($day == 'Wed'){ if ($time >= 11 && $time <= 16 ) { $special = 'Wednesday Lunch Special'; }} if ($day == 'Wed'){ if ($time >= 16 && $time <= 24 ) { $special = 'Wednesday Dinner Special'; }} // Thu if ($day == 'Thu'){ if ($time >= 0 && $time <= 11 ) { $special = 'Thursday Breakfast Special'; }} if ($day == 'Thu'){ if ($time >= 11 && $time <= 16 ) { $special = 'Thursday Lunch Special'; }} if ($day == 'Thu'){ if ($time >= 16 && $time <= 24 ) { $special = 'Thursday Dinner Special'; }} // Fri if ($day == 'Fri'){ if ($time >= 0 && $time <= 11 ) { $special = 'Friday Breakfast Special'; }} if ($day == 'Fri'){ if ($time >= 11 && $time <= 16 ) { $special = 'Friday Lunch Special'; }} if ($day == 'Fri'){ if ($time >= 16 && $time <= 24 ) { $special = 'Friday Dinner Special'; }} // Sat if ($day == 'Sat'){ if ($time >= 0 && $time <= 11 ) { $special = 'Saturday Breakfast Special'; }} if ($day == 'Sat'){ if ($time >= 11 && $time <= 16 ) { $special = 'Saturday Lunch Special'; }} if ($day == 'Sat'){ if ($time >= 16 && $time <= 24 ) { $special = 'Saturday Dinner Special'; }} // Sun if ($day == 'Sun'){ if ($time >= 0 && $time <= 11 ) { $special = 'Sunday Breakfast Special'; }} if ($day == 'Sun'){ if ($time >= 11 && $time <= 16 ) { $special = 'Sunday Lunch Special'; }} if ($day == 'Sun'){ if ($time >= 16 && $time <= 24 ) { $special = 'Sunday Dinner Special'; }} echo $day; echo ('<br /><br />'); echo $time; echo ('<br /><br />'); echo $special; ?> I also had to change up the $day = to $day == Thanks for the help. -
Show Specials between certain times / days of the week.
hinchcliffe replied to hinchcliffe's topic in PHP Coding Help
Ok, So what's happening is... It's showing the very last day & not filtering the day if statement. So right now it's supposed to be showing Thursday, Breakfast Special It's showing Sunday, Breakfast Special. Oh it's also not echo'ing the day*. Know what's wrong? -
Hello, I'm kind of a noob at php. I'm trying to write some code that shows what a restaurant special is between certain times of the week and it switches depending on the day of the week as well. Each day of the week will have different specials. There's a breakfast special between 6am-11am, then there's a lunch special between 11am-4pm, then there's a dinner special from 4pm - 11pm. Anyways I've been struggling with some code, could someone take a look at it and help me out? Thanks! <?php $day = (date("D")); $time = (date("H") + 2); // Actuall Specials // Mon if ($day = 'Mon' && $time == (11 > $time && $time > 0)) { $special = 'Monday Breakfast Special'; } if ($day = 'Mon' && $time == (16 > $time && $time > 11)) { $special = 'Monday Lunch Special'; } if ($day = 'Mon' && $time == (24 > $time && $time >= 16)) { $special = 'Monday Dinner Special'; } // Tue if ($day = 'Tue' && $time == (11 > $time && $time > 0)) { $special = 'Tuesday Breakfast Special'; } if ($day = 'Tue' && $time == (16 > $time && $time > 11)) { $special = 'Tuesday Lunch Special'; } if ($day = 'Tue' && $time == (24 > $time && $time >= 16)) { $special = 'Tuesday Dinner Special'; } // Wed if ($day = 'Wed' && $time == (11 > $time && $time > 0)) { $special = 'Wednesday Breakfast Special'; } if ($day = 'Wed' && $time == (16 > $time && $time > 11)) { $special = 'Wednesday Lunch Special'; } if ($day = 'Wed' && $time == (24 > $time && $time >= 16)) { $special = 'Wednesday Dinner Special'; } // Thu if ($day = 'Thu' && $time == (11 > $time && $time > 0)) { $special = 'Thursday Breakfast Special'; } if ($day = 'Thu' && $time == (16 > $time && $time > 11)) { $special = 'Thursday Lunch Special'; } if ($day = 'Thu' && $time == (24 > $time && $time >= 16)) { $special = 'Thursday Dinner Special'; } // Fri if ($day = 'Fri' && $time == (11 > $time && $time > 0)) { $special = 'Friday Breakfast Special'; } if ($day = 'Fri' && $time == (16 > $time && $time > 11)) { $special = 'Friday Lunch Special'; } if ($day = 'Fri' && $time == (24 > $time && $time >= 16)) { $special = 'Friday Dinner Special'; } // Sat if ($day = 'Sat' && $time == (11 > $time && $time > 0)) { $special = 'Saturday Breakfast Special'; } if ($day = 'Sat' && $time == (16 > $time && $time > 11)) { $special = 'Saturday Lunch Special'; } if ($day = 'Sat' && $time == (24 > $time && $time >= 16)) { $special = 'Saturday Dinner Special'; } // Sun if ($day = 'Sun' && $time == (11 > $time && $time > 0)) { $special = 'Sunday Breakfast Special'; } if ($day = 'Sun' && $time == (16 > $time && $time > 11)) { $special = 'Sunday Lunch Special'; } if ($day = 'Sun' && $time == (24 > $time && $time >= 16)) { $special = 'Sunday Dinner Special'; } echo $day; echo ('<br /><br />'); echo $time; echo ('<br /><br />'); echo $special; ?> Any help teaching me would be very appreciated.
-
weird still not updating, there are no errors in the code though. hmm Maybe take a look at more of my code. <?php // if the submit button is clicked, do this. if($_POST['update']) { $table = $_POST['table']; $returnurl1 = "find_articles.php"; // this is the redirect url after succes $returnurl2 = "edit_articles.php?id=".$_GET['idr']."&mode=modify"; // this is the redirect url after success if($_GET['mode']=='add') { $insert = "INSERT INTO articles (title, body) VALUES ('".$_POST['title']."', '".$_POST['body']."')"; mysql_query($insert); // redirect after 2 seconds echo "<div><center>Success! Article Added!</center></div>"; echo "<META HTTP-EQUIV=\"Refresh\" CONTENT=\"2; URL=".$returnurl1."\">"; } //if mod eis not add, then the mode is modify else { $update = "UPDATE articles SET title = '".$_POST['title']."', body = '".$_POST['body']."' WHERE id ='".$_GET['idr']."'"; $check = mysql_query($update); echo "<div><center>Success! Job Saved!</center></div>"; echo "<META HTTP-EQUIV=\"Refresh\" CONTENT=\"2; URL=\"index.php\">"; } } ?>
-
Hey guys can you take a look over my code my update query isn't working. Ty $update = "UPDATE articles SET title = '".$_POST['title']."', body = '".$_POST['body']."' WHERE id ='".$_GET['idr']."'"; $check = mysql_query($update); echo "<div><center>Success! Job Saved!</center></div>"; echo "<META HTTP-EQUIV=\"Refresh\" CONTENT=\"2; URL=\"index.php\">";
-
[SOLVED] Quick help - Select all check boxes
hinchcliffe replied to hinchcliffe's topic in Javascript Help
thanks so much -
[SOLVED] Quick help - Select all check boxes
hinchcliffe replied to hinchcliffe's topic in Javascript Help
Sorry, I'm very bad with javascript, How would I implement that with my code? Thanks for helping - Justin. -
Hey guys, Ok I've got help / solved the first bit of php from before. ---> http://www.phpfreaks.com/forums/index.php/topic,164936.0.html Now I need the actual mailing part of this to work. Can someone take a look over my code and guide me? Site: www.bbold.com/mail.php <?php // Connect to DataBase include 'includes/dbconnect.php'; // Query database $query = "SELECT * FROM mail"; $rs = mysql_query($query); echo 'Subscription List <br /><br />'; echo "<form name=\"myform\" action=\"" . $_SERVER['PHP_SELF'] . "\" method=\"post\">" . "<br />"; while($row = mysql_fetch_array($rs)) { echo "<input type=\"checkbox\" name=\"list[]\" value=\"" .$row['email']. "\" >" . " <a href=\"mailto:" . $row['email'] . "\">" . $row['email'] . "</a><br />"; } // If summit button clicked do this!! if($_POST['submit']) { //send the email $content = "put content here"; //start mail script $to = foreach($_POST['list'] AS $email){ $email . "; ";} $subject = "Thank you for registering at Morowat Global"; $message = $content; $headers = "From: jordan@morowatglobal.com\r\n"; $headers .= "Reply-To: jordan@morowatglobal.com\r\n"; $headers .= "Return-Path: jordan@morowatglobal.com\r\n"; $headers .= "'From: ".$from."'\n"; $headers .= 'MIME-Version: 1.0' . "\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\n"; if ( mail($to,$subject,$message,$headers) ) } ?> <br /> <input type=button value="Check All" onClick="this.value=check(this.form.list[])"> <br/> Send an e-mail:<br /> <textarea name="message" rows="10" cols="70"></textarea><br/><br /> <input type="submit" name="submit" value="Send"> Thanks
-
Hi, there I have a script that I need to change up a bit. So what I want it to do is select all boxes at once when the select button is clicked. I have a code that will do that but I now need to change my name from name="list" to name="list[]" and it's messing up the rest of the script. Can some one tell me the right code so I can use name="list[]"? Thanks. Site: www.bbold.com/strataman/mail.php Here is my code... <SCRIPT LANGUAGE="JavaScript"> <!-- Begin var checkflag = "false"; function check(field) { if (checkflag == "false") { for (i = 0; i < field.length; i++) { field[i].checked = true;} checkflag = "true"; return "Uncheck All"; } else { for (i = 0; i < field.length; i++) { field[i].checked = false; } checkflag = "false"; return "Check All"; } } // End --> </script> <?php // Connect to DataBase include 'includes/dbconnect.php'; // Query database $query = "SELECT * FROM mail"; $rs = mysql_query($query); echo 'Subscription List <br /><br />'; echo "<form name=\"myform\" action=\"" . $_SERVER['PHP_SELF'] . "\" method=\"post\">" . "<br />"; while($row = mysql_fetch_array($rs)) { echo "<input type=\"checkbox\" name=\"list[]\" value=\"" .$row['email']. "\" >" . " <a href=\"mailto:" . $row['email'] . "\">" . $row['email'] . "</a><br />"; } // If summit button clicked do this!! if($_POST['submit']) { foreach($_POST['list'] AS $email){ echo $email . "; " ; } } ?> <br /> <input type=button value="Check All" onClick="this.value=check(this.form.list)"> Thanks
-
Checkbutton e-mail list, with check all.
hinchcliffe replied to hinchcliffe's topic in PHP Coding Help
Sorry, I'm not very good at javascript could you explain it better? Do you want me to change this section? echo "<form name=\"myform\" action=\"" . $_SERVER['PHP_SELF'] . "\" method=\"post\">" . "<br />"; -
Checkbutton e-mail list, with check all.
hinchcliffe replied to hinchcliffe's topic in PHP Coding Help
Oh ok, Thanks, that works good, Now I just have one more problem. Since you changed the last name, my javascript for the check all isn't working. I'll post the code... <SCRIPT LANGUAGE="JavaScript"> <!-- Begin function checkAll(field) { for (i = 0; i < field.length; i++) field[i].checked = true ; } function uncheckAll(field) { for (i = 0; i < field.length; i++) field[i].checked = false ; } // End --> </script> <?php // Connect to DataBase include 'includes/dbconnect.php'; // Query database $query = "SELECT * FROM mail"; $rs = mysql_query($query); echo 'Subscription List <br /><br />'; echo "<form name=\"myform\" action=\"" . $_SERVER['PHP_SELF'] . "\" method=\"post\">" . "<br />"; while($row = mysql_fetch_array($rs)) { echo "<input type=\"checkbox\" name=\"list[]\" value=\"" .$row['email']. "\" >" . " <a href=\"mailto:" . $row['email'] . "\">" . $row['email'] . "</a><br />"; } // If summit button clicked do this!! if($_POST['submit']) { foreach($_POST['list'] AS $email){ echo $email; } } ?> <br /> <input type="button" name="CheckAll" value="Check All" onClick="checkAll(document.myform.list)"> <input type="button" name="UnCheckAll" value="Uncheck All" onClick="uncheckAll(document.myform.list)"> <br/> Send an e-mail:<br /> <textarea name="message" rows="10" cols="100"></textarea><br/><br /> <input type="submit" name="submit" value="Send"> I think it's just this part thats messing it up <input type="button" name="CheckAll" value="Check All" onClick="checkAll(document.myform.list)"> <input type="button" name="UnCheckAll" value="Uncheck All" onClick="uncheckAll(document.myform.list)"> I already tried changing the (document.myform.list) to (document.myform.list[]). Any other suggestions? Thanks for your help so far. -
Checkbutton e-mail list, with check all.
hinchcliffe replied to hinchcliffe's topic in PHP Coding Help
Warning: Invalid argument supplied for foreach() in /home/bbold07/public_html/strataman/admin.php on line 50 if($_POST['submit']) { foreach($_POST['list'] AS $email){ echo $email . "<br />\n"; } } ?? -
Hi Guys, I was wondering if you could give me a bit of help. I have a e-mail list that I would like to be able to select witch user is going to be e-mailed, also it would have the check all / uncheck all buttons. I have an none working example of what I need to code here: http://bbold.com/strataman/admin.php Here is what I got so far. <!-- Includes Header start --> <?php include('includes/header.php'); ?> <!-- Includes Header Ends / Content Starts --> <h1>E-mail Subscribers:</h1><br /> <div id="listings"> <center><h3>Mailing List</h3></center> <SCRIPT LANGUAGE="JavaScript"> <!-- Begin function checkAll(field) { for (i = 0; i < field.length; i++) field[i].checked = true ; } function uncheckAll(field) { for (i = 0; i < field.length; i++) field[i].checked = false ; } // End --> </script> <?php // Connect to DataBase include 'includes/dbconnect.php'; // Query database $query = "SELECT * FROM mail"; $rs = mysql_query($query); echo 'Subscription List <br /><br />'; echo "<form name=\"myform\" action=\"" . $_SERVER['PHP_SELF'] . "\" method=\"post\">" . "<br />"; while($row = mysql_fetch_array($rs)) { echo "<input type=\"checkbox\" name=\"list\" value=\"" .$row['email']. "\" >" . " <a href=\"mailto:" . $row['email'] . "\">" . $row['email'] . "</a><br />"; } // If summit button clicked do this!! if($_POST['submit']) { echo $_POST['list']; } ?> <br /> <input type="button" name="CheckAll" value="Check All" onClick="checkAll(document.myform.list)"> <input type="button" name="UnCheckAll" value="Uncheck All" onClick="uncheckAll(document.myform.list)"> <br/> Send an e-mail:<br /> <textarea name="message" rows="10" cols="100"></textarea><br/><br /> <input type="submit" name="submit" value="Send"> <br /><br /> Attach a file:<br /> <input type="file" name="file"> </form> </div> <!-- Includes Footer Starts --> <?php include('includes/footer.php'); ?> <!-- Includes Footer Ends -->