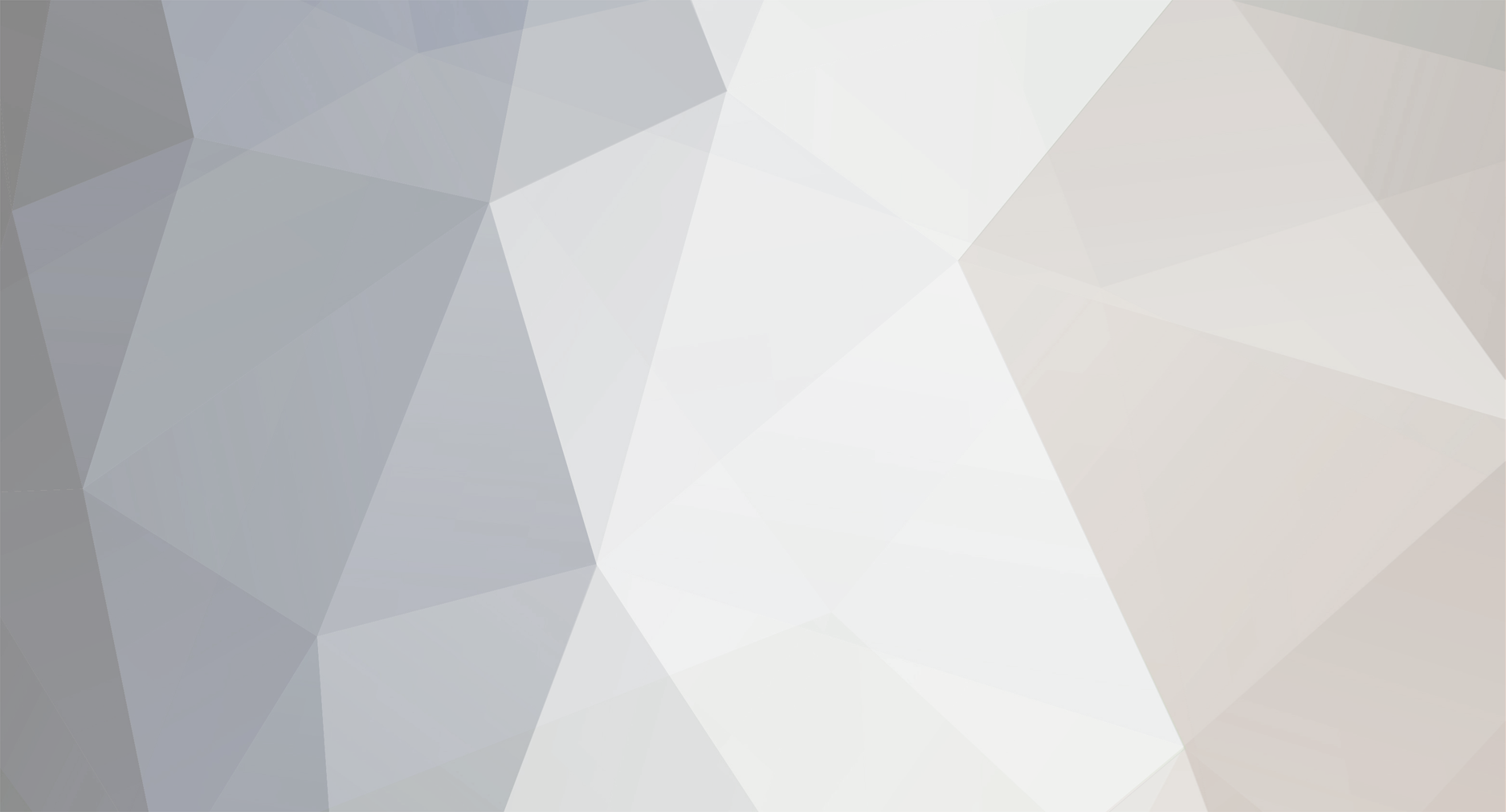
bschultz
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by bschultz
-
I can't reproduce the problem...but I've been told by several people that they are having a problem logging in to a members only section. The login page asks for a username and a password. Here's the code for the execution of that login: <?php //Start session session_start(); //Include database connection details require_once('config.php'); //Array to store validation errors $errmsg_arr = array(); //Validation error flag $errflag = false; //Connect to mysql server $link = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } //Select database $db = mysql_select_db(DB_DATABASE); if(!$db) { die("Unable to select database"); } //Function to sanitize values received from the form. Prevents SQL injection function clean($str) { $str = @trim($str); if(get_magic_quotes_gpc()) { $str = stripslashes($str); } return mysql_real_escape_string($str); } //Sanitize the POST values $login = clean($_POST['login']); $password = clean($_POST['password']); //Input Validations if($login == '') { $errmsg_arr[] = 'Login ID missing'; $errflag = true; } if($password == '') { $errmsg_arr[] = 'Password missing'; $errflag = true; } //If there are input validations, redirect back to the login form if($errflag) { $_SESSION['ERRMSG_ARR'] = $errmsg_arr; session_write_close(); header("location: login-form.php"); exit(); } //Create query $qry="SELECT * FROM members WHERE login='$login' AND passwd='".md5($password)."'"; $result=mysql_query($qry); //Check whether the query was successful or not if($result) { if(mysql_num_rows($result) == 1) { //Login Successful session_regenerate_id(); $member = mysql_fetch_assoc($result); $_SESSION['SESS_MEMBER_ID'] = $member['member_id']; $_SESSION['SESS_FIRST_NAME'] = $member['firstname']; $_SESSION['SESS_LAST_NAME'] = $member['lastname']; $_SESSION['SESS_ADDRESS'] = $member['address']; $_SESSION['SESS_CITY'] = $member['city']; $_SESSION['SESS_STATE'] = $member['state']; $_SESSION['SESS_LOGIN'] = $member['login']; $_SESSION['SESS_CAPTAIN'] = $member['captain']; $_SESSION['SESS_TEAM'] = $member['team_name']; $_SESSION['SESS_MANUAL_TEAM'] = $member['manual_team']; session_write_close(); header("location: member-index.php"); exit(); }else { //Login failed header("location: login-failed.php"); exit(); } }else { die("Query failed"); } ?> Here's the code for the "members only" section: <?php session_start(); require_once('auth.php'); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Member Index</title> <link href="loginmodule.css" rel="stylesheet" type="text/css" /> </head> <body> <h1>Welcome <?php echo $_SESSION['SESS_FIRST_NAME'];?></h1> <a href="logout.php">Logout</a> <p> <?php include "links.php"; ?> <br /> <br /> Thank you for participating in the Go100 For Health walks for 2009. Below, you will see how many miles your team has walked. <br /> blah blah blah Here's auth.php <?php //Start session session_start(); //Check whether the session variable SESS_MEMBER_ID is present or not if(!isset($_SESSION['SESS_MEMBER_ID']) || (trim($_SESSION['SESS_MEMBER_ID']) == '')) { header("location: access-denied.php"); exit(); } ?> Like I said, I can't recreate the problem, so I'm a bit at a loss as to what might be causing this. The people that are having a problem logging in are able to login once in a while...just not every time they try. Anything look out of place or wrong to anyone? Thanks!
-
I don't have a clue what I just did...but it's working!!!!!! <?php require_once('config.php'); $link = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } $db = mysql_select_db(DB_DATABASE); if(!$db) { die("Unable to select database"); } $qry="select *, sum(miles*(1-abs(sign(week-1)))) as week1, sum(miles*(1-abs(sign(week-2)))) as week2, sum(miles*(1-abs(sign(week-3)))) as week3, sum(miles*(1-abs(sign(week-4)))) as week4, sum(miles*(1-abs(sign(week-5)))) as week5, sum(miles*(1-abs(sign(week-6)))) as week6, sum(miles*(1-abs(sign(week-7)))) as week7, sum(miles*(1-abs(sign(week-))) as week8, sum(miles*(1-abs(sign(week-9)))) as week9, sum(miles*(1-abs(sign(week-10)))) as week10, sum(miles*(1-abs(sign(week-11)))) as week11, sum(miles*(1-abs(sign(week-12)))) as week12, sum(miles*(1-abs(sign(week-13)))) as week13, (select sum( miles ) from miles b where a.team_name=b.team_name) as total_miles, (select count(*) from members c where a.team_name=c.team_name) as num_team_members, (select sum( miles ) from miles b where a.team_name=b.team_name) / (select count(*) from members c where a.team_name=c.team_name) as avg_miles_per_team_member FROM miles a GROUP BY avg_miles_per_team_member DESC"; $result=mysql_query($qry); if( !$result ) { echo mysql_error() . '<br />'; } ?> <table width="100%" border="1" cellspacing="1" cellpadding="1"> <tr> Go 100 For Health </tr> <br /><br /> <tr> <td><div align="center">Team Name</div></td> <td><div align="center">Wk<br /> 1</div></td> <td><div align="center">Wk<br /> 2</div></td> <td><div align="center">Wk<br /> 3</div></td> <td><div align="center">Wk<br /> 4</div></td> <td><div align="center">Wk<br /> 5</div></td> <td><div align="center">Wk<br /> 6</div></td> <td><div align="center">Wk<br /> 7</div></td> <td><div align="center">Wk<br /> 8</div></td> <td><div align="center">Wk<br /> 9</div></td> <td><div align="center">Wk<br /> 10</div></td> <td><div align="center">Wk<br /> 11</div></td> <td><div align="center">Wk<br /> 12</div></td> <td><div align="center">Wk<br /> 13</div></td> <td><div align="center">Total <br />Miles</div></td> <td><div align="center">Avg Per <br />Walker</div></td> </tr> <?php while ($row = mysql_fetch_array($result)) { echo "<TR>"; echo "<TD>".$row[team_name]." </TD>"; echo "<TD><div align='center'>".$row[week1]." </div></TD>"; echo "<TD><div align='center'>".$row[week2]." </div></TD>"; echo "<TD><div align='center'>".$row[week3]." </div></TD>"; echo "<TD><div align='center'>".$row[week4]." </div></TD>"; echo "<TD><div align='center'>".$row[week5]." </div></TD>"; echo "<TD><div align='center'>".$row[week6]." </div></TD>"; echo "<TD><div align='center'>".$row[week7]." </div></TD>"; echo "<TD><div align='center'>".$row[week8]." </div></TD>"; echo "<TD><div align='center'>".$row[week9]." </div></TD>"; echo "<TD><div align='center'>".$row[week10]." </div></TD>"; echo "<TD><div align='center'>".$row[week11]." </div></TD>"; echo "<TD><div align='center'>".$row[week12]." </div></TD>"; echo "<TD><div align='center'>".$row[week13]." </div></TD>"; echo "<TD><div align='center'>".$row[total_miles]." </div></TD>"; echo "<TD><div align='center'><strong>".round($row[avg_miles_per_team_member],3)." </strong></div></TD>"; echo "</TR>"; } echo "</TABLE>"; ?> roopurt18...thank you A LOT! I've learned alot about what is possible with MYSQL and PHP...still amazes me what these can do if you know what you're doing. I'm just a lowly radio announcer who knows a little (and I stress, A LITTLE) about PHP and MYSQL. Thank you for helping me with this!
-
(@tm:=(select sum( miles ) from miles b where a.team_name=b.team_name)) as total_miles, (@ntm:=(select count(*) from members c where a.team_name=c.team_name)) as num_team_members, @tm / @ntm as avg_miles_per_team_member ...didn't produce an error...but also didn't divide out the per walker average (select sum( miles ) from miles b where a.team_name=b.team_name) as total_miles, (select count(*) from members c where a.team_name=c.team_name) as num_team_members, (select sum( miles ) from miles b where a.team_name=b.team_name) / (select count(*) from members c where a.team_name=c.team_name) as avg_miles_per_team_member did divide out the average...but put it in the total miles column of the html table...and nothing in the html average column select z.*, z.total_miles / z.num_team_members as avg_miles_per_team_member from (select *, sum(miles*(1-abs(sign(week-1)))) as week1, sum(miles*(1-abs(sign(week-2)))) as week2, sum(miles*(1-abs(sign(week-3)))) as week3, sum(miles*(1-abs(sign(week-4)))) as week4, sum(miles*(1-abs(sign(week-5)))) as week5, sum(miles*(1-abs(sign(week-6)))) as week6, sum(miles*(1-abs(sign(week-7)))) as week7, sum(miles*(1-abs(sign(week-))) as week8, sum(miles*(1-abs(sign(week-9)))) as week9, sum(miles*(1-abs(sign(week-10)))) as week10, sum(miles*(1-abs(sign(week-11)))) as week11, sum(miles*(1-abs(sign(week-12)))) as week12, sum(miles*(1-abs(sign(week-13)))) as week13, (select sum( miles ) from miles b where a.team_name=b.team_name) as total_miles, (select count(*) from members c where a.team_name=c.team_name) as num_team_members FROM miles a GROUP BY total_miles DESC ) as z did the same thing as the middle piece of code did. I have to admit...this is WAY over my head. I'm only able to copy and paste at this point. I do appreciate the help...A LOT!
-
Roopurt 18...I REALLY appreciate the help. That threw an error...no line number I follow the logic on the select (in fact, I had tried JOIN too with temp columns c and d) and that didn't work either...but what the heck is "field list"?
-
This is what I've come up with so far...and it's still not working. <?php $qry="select *, sum(miles*(1-abs(sign(week-1)))) as week1, sum(miles*(1-abs(sign(week-2)))) as week2, sum(miles*(1-abs(sign(week-3)))) as week3, sum(miles*(1-abs(sign(week-4)))) as week4, sum(miles*(1-abs(sign(week-5)))) as week5, sum(miles*(1-abs(sign(week-6)))) as week6, sum(miles*(1-abs(sign(week-7)))) as week7, sum(miles*(1-abs(sign(week-))) as week8, sum(miles*(1-abs(sign(week-9)))) as week9, sum(miles*(1-abs(sign(week-10)))) as week10, sum(miles*(1-abs(sign(week-11)))) as week11, sum(miles*(1-abs(sign(week-12)))) as week12, sum(miles*(1-abs(sign(week-13)))) as week13, (select sum( miles ) from miles b where a.team_name=b.team_name) as total_miles FROM miles a UNION (SELECT team_name,COUNT(*) FROM members WHERE miles.team_name=members.team_name) as number_walkers FROM members GROUP BY total_miles DESC"; ?>
-
Alright...I've added all the weeks into the query, and it works. Thanks again! But, I realized that I forgot a step in the query. I need to add a second table (members table) and pull a mysql_num_rows of the rows that match the team_name (members.team_name AND miles.team_name) and then divide the total miles walked per team by the total number of walkers per team. On this page (http://en.wikibooks.org/wiki/MySQL/Pivot_table) I didn't see anything to join two tables... how would I go about that? Thanks!
-
That did it...THANK YOU!
-
two errors... Unknown column 'total_miles' in 'order clause' Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource This: <?php $qry="select *, sum(miles*(1-abs(sign(week-1)))) as week1, sum(miles*(1-abs(sign(week-2)))) as week2 FROM miles GROUP BY team_name"; ?> gives me the weekly miles...but no total miles. How can I incorporate a total_miles into this?
-
WOW...is that hard to grasp! I've tried, and came up with a combination of both query's <?php $qry="select *, sum(miles*(1-abs(sign(week-1)))) as week1, sum(miles*(1-abs(sign(week-2)))) as week2, SELECT wc1.team_name AS team_name, (SELECT SUM(wc2.miles) FROM miles wc2 WHERE wc2.team_name = wc1.team_name) AS total_miles FROM miles wc1 GROUP BY wc1.team_name ORDER BY total_miles"; $result=mysql_query($qry); ?> <table width="100%" border="1" cellspacing="1" cellpadding="1"> <tr> Go 100 For Health </tr> <br> <tr> <td>Team Name</td> <td>Week 1</td> <td>Week 2</td> <td>Total Miles</td> </tr> <?php while ($row = mysql_fetch_array($result)) { echo "<TR>"; echo "<TD>".$row[team_name]." </TD>"; echo "<TD>".$row[week1]." </TD>"; echo "<TD>".$row[week2]." </TD>"; echo "<TD>".$row[total_miles]." </TD>"; echo "</TR>"; } echo "</TABLE>"; ?> This throws an error on this line: Even removing the second part of the query (Ken2K7's part) threw the same error. Any ideas? Thanks!
-
I'll give it a whirl...thanks!
-
Thanks for the help! I do, though, have a question. What is wc1 and wc2? There is only one table in the database.
-
I have been asked by the local hospital to keep track of a walking contest. Teams will walk each week, and will enter the results into a database. I need to keep track of all the miles walked. That part, I've done. Now the hospital has asked me to create a table with all the teams results for each week. Something like this: Team Name Week 1 Week 2 Week 3 Total Team 1 12 14 11 37 Team 2 10 9 11 30 Team 3 15 7 7 29 Team 4 12 9 7 28 There will be (roughly) 50 teams (with completely random team names) walking... The database is laid out like this: row_number (integer, auto increment, primary key) team_name (varchar 200) week (int,2) miles (decimal 10,2) What's the best way to write a query to spit all that info out into an html table, sorted by the team with the most total miles walked?
-
[SOLVED] select where now() between start and end
bschultz replied to bschultz's topic in MySQL Help
All is working now. I had changed the field type from date to datetime, and thought it was working...until it kept displaying the outdated entries. I deleted the table and started over (with datetime as the field types), and now it's working just fine. Thanks. -
[SOLVED] select where now() between start and end
bschultz replied to bschultz's topic in MySQL Help
Thanks for the help! The server time is correct...just checked it. Your code still displayed expired results. The one record that's displaying wrong (the only one that's expired) has this: as it's enddate value. As you can see on this page (http://www.kkbj.com/datetime.php) the time is right...and the enddate field in the db is before the current time. -
[SOLVED] select where now() between start and end
bschultz replied to bschultz's topic in MySQL Help
nope...it's sitting right next to me -
[SOLVED] select where now() between start and end
bschultz replied to bschultz's topic in MySQL Help
server version is 5.0.32 by the way... No errors, just showing events that had an enddate of a few hours ago. -
This query isn't returning what I thought it would... $sql = "SELECT * FROM content WHERE NOW() BETWEEN startdate AND enddate"; Both startdate and enddate are datetime formats in the db. What is the correct syntax for this query? Thanks.
-
That did it...thanks! It amazes me what php can do!
-
Alright...I'm dumb. I was so set on replacing WE (since that's how I currently enter the info into the db) that it never dawned on my to enter COMPANYNAMEHERE (or some other completely random thing) into the db where the company name was supposed to go. Upon looking at preg_replace and how it needs to be written, how can I turn this: echo "<span class='itemdetails2'><br /><br />".stripslashes($row[story])."</span><br /><br /></td></tr>"; into the string to be replaced?
-
right...but I don't want to replace all "We"'s with Company Name. I'd like to have this in the database: <?php if (strpos(getenv('SERVER_name'), 'domanin1.com')!==false) { echo "Company 1"; } elseif (strpos(getenv('SERVER_name'), 'domanin2.com)!==false) { echo "Company 2"; } elseif (strpos(getenv('SERVER_name'), 'domain3.com')!==false) { echo "Company 3"; }; ?> will have a float in the Memorial Day Parade. but I can't insert php code into the database...but I can't think of any other way to only replace the company name when I want to...and not every time.
-
My company has four domain names. Each of the four websites uses the some of the same info gathered from a database...and I would like to replace the "we" with the company name. Here's the example of what might be in the database: With this: Obviously, I can't put php code into the database to determine which domain they are viewing the article from...so how can I enter the info into the database (and more importantly, retrieve it), so that the company name would replace "We"? Mind you, not all entries into the database will have a company name...so I can't just replace every "WE" with the "company name" Thanks. Brian
-
I got it...$_GET[day] was in 2009-18-06 format...when it should have been 2009-06-18 format. Thanks...again!
-
int(-1)
-
Warning: cal_days_in_month() [function.cal-days-in-month]: invalid date. in daily.php on line 42 This is line 42
-
I get an "invalid day" error on this code $day = $_GET['day']; $data = "$day"; list($year, $month, $date) = explode("-", $data); $previousmonth = ($month - 1); $thisyear = date("Y"); // find out the number of days in the month $numdaysinmonth = cal_days_in_month( CAL_GREGORIAN, $previousmonth, $thisyear );