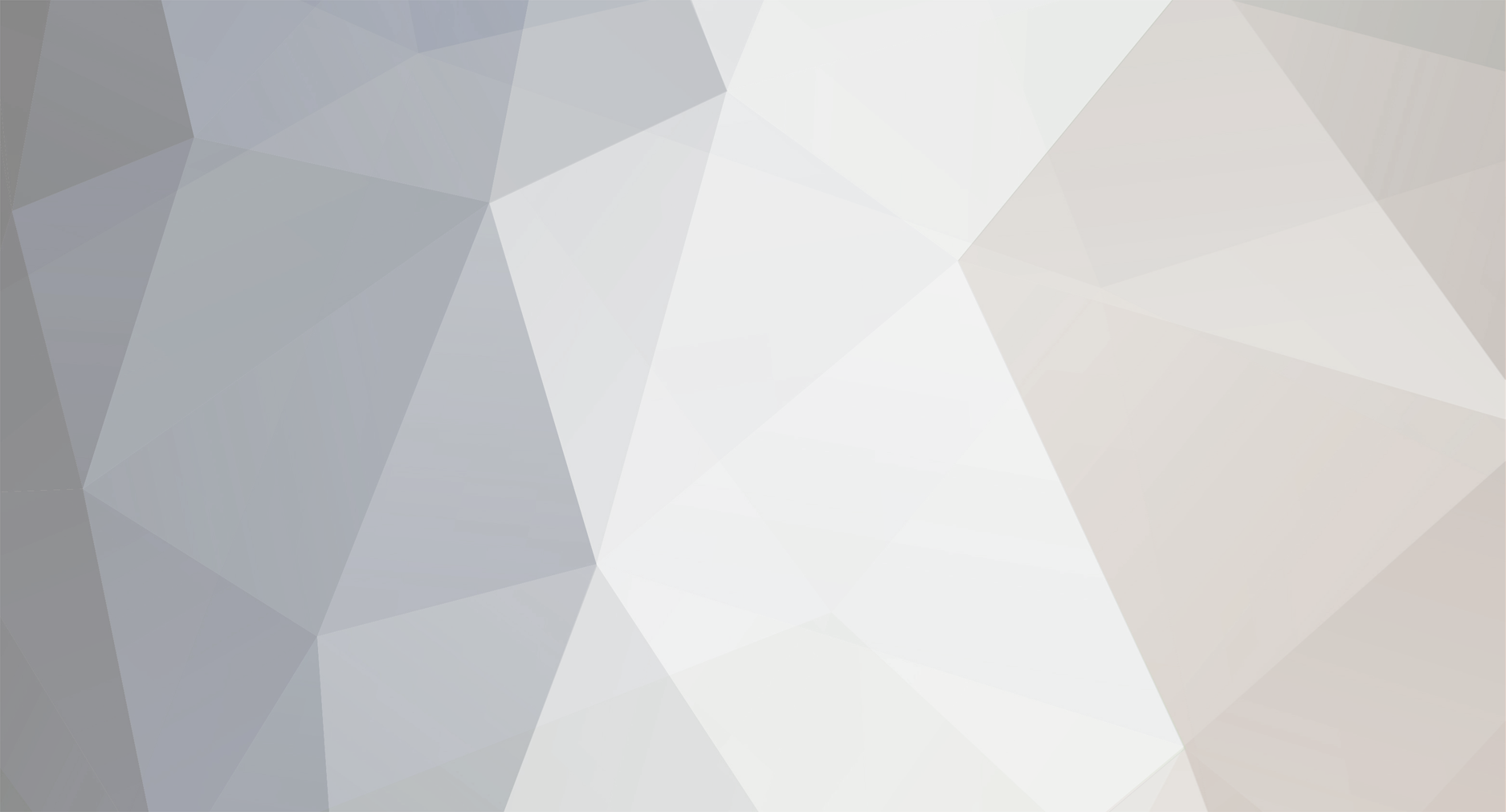
minidak03
-
Posts
63 -
Joined
-
Last visited
Never
Posts posted by minidak03
-
-
store the image path/name, not the image.
This is the best way store the image path in the database and then just store the image in a folder somewhere.
-
I would do this another way like so
$string = 'Test image <a href="http://domain.com"><img src="http://domain.com/images/test.jpg" alt="test"></a> is a test.'; $clean = strip_tags($string); echo $clean;
I know your trying to hack it out with Regex or learning but sometimes its easier not to do it with Regex.
-
Well my username was created for a number of reasons, the first reason is because I used to own a small Mazda B2200 truck which I lowered to the ground then I sold it for a new 2003 Dodge Dakota (New Back then) and I planned to lower the dodge just like I pimped out and lowered the mazda.
So my name came from that mini for low truck and dak which stands for dakota and 03 because its a 2003.
So minidak03 really stands for mini dakota 2003. -
if($row_hour_count['hour_count'] > $row_rankd['hours']) { $rank1 = $row_rankd['title']; } do( elseif($row_hour_count['hour_count'] > $row_rank['hours']) { $rank1 = $row_rank['title']; } } while ($row_rank = mysql_fetch_assoc($rank));
Look at where your do statement is it should be inside of your else if or completely outside of your if statement that is what is causing your error.
-
The best structure is the one you can remember unless you want added security, for instance I usually always place my images in a folder called images which makes sense right however if you don't place an index file in this folder as well on some servers someone can type in mypage.com/images/ and see a full indexable list of all your sites images.
They can also hot-link to them but there are php tactics which you can use to get around that. For videos its not suggested you place them in a videos folder this folder should be much more complex like 55sdaf65as and a sub-folder in that folder called anything like 54sad564sad5f.
The reason I do this with videos is because when people want to hot-link to a video it really does a number on your bandwidth however if you have a flash player to play these videos you can code flash so that its pretty much impossible for the visitor to guess your document structure.
For secured and protected files you can always place them in a folder called secure or protected but the true security comes into place in your actual code to restrict access to these files.
Your document structure can be whatever makes sense to you but you also really need to think about security in your code to restrict access to your document structure.
-
lol nevermind I must have mis-read your post its because your encoding is us-ASCII this can usually be changed in the program you are using under file settings.
Not exactly sure which program your using though but the meta tag won't change that information.
if your using non-ASCII characters like Japanese then you would be using different file settings.
-
If your data in the text file is seperate on each line you can do this
$lines = file('textfile.txt'); echo '<select>'; foreach($lines as $line) { echo '<option>'.$line.'</option>'; } echo '</select>';
-
Try using your title tags inside of your <head> tags this usually fixes that.
<title>This is a title</title>
EDIT: So now your code will look like this
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <meta http-equiv="content-type" content="text/html; charset=windows-1250"> <meta name="generator" content="PSPad editor, www.pspad.com"> <title>This is a title</title> </head> <body> test </body> </html>
-
http://php.net/manual/en/reference.pcre.pattern.modifiers.php
A good resource for RegEx: http://www.regular-expressions.info/
Thanks for posting some links I was too lazy to grab them its going on 3 am here so my explanations are a little broad haha.
-
Yes you have all of that right and as for your last question of what would it return well 9 times out of 10 it wouldn't return anything at all because its a NULL value it would be like saying
$value = '';
Now in the past (Haven't seen this happen in decades maybe it was an old time bug that got worked out) that when using SQL (Note I didn't say MySql But SQL) the output would actually be the text NULL, however with MySql it doesn't return anything and there is no error to worry about because its just a blank field.
Maybe that difference is still around I don't know its been ages since I used SQL over MySql but if your wondering the difference there is but just think of MySql which is mainly used in Linux (PHP) based applications where SQL Windows (ASP) is mainly used for things like ASP.NET or windows applications.
I might get some flack for saying that but thats just how I see the main difference between SQL and MySql even though there are a lot of differences.
-
Ok preg_replace uses something called Regex in case you couldn't find any reference material on it, Regex was basically created by the suckers who used to code in Binary (Not Really lol) I just said that because most of the time it makes no sense but you get used to it after banging your head against the walls for a few years.
I understand that (.*) represents a value which could be a combination of any characters. And that for the $pattern part of the function, you used the / / to declare what you want to find. In this case its <a href=\"(.*)\">(.*)<\/a> but then what is with the /i after it? And as for what you want it to be replaced with, what does \\2 (\\1) mean? I also know the \ is used to escape characters such as the " or the / which would screw with the function and produce errors.To answer some of your questions lets start with the above block. First off the /i after the entire thing is like saying the string is not case sensitive so it compares case insensitive or case does not matter when searching. There are a few and I still have to re-look them up when using them and I've been doing it for awhile so don't feel bad if you can't remember all Regex because you probably never will thats what reference material was created for.
As for what does \\2 (\\1) mean? Well this means that we need to ignore the / before the 2 and ignore the / before the 1 because when you declare Regex you declare everything between two / and every other slash needs to be escaped as well as other things like single or double quotes depending on what your using.
Also things like the period, the * and the ? should all be escaped if you are searching for it directly because you can think of those as types of commands where (.*) gets everything, ect... now you can begin to group items with [] and () for instance using () says combine . and * which means grab everything and using [] is like a conditional statement so if we had [0-9] that means only grab numbers between 1 and 9.
$string = "The cat ran up <a href="http://www.google.ca">Google's</a> dead oak tree."; $clean = preg_replace('/<a\shref=\"(.*)\"/'); $output = clean;
Ok in the above example the output would be <a href="http://www.google.ca" after which you would clean up the rest by removing the <a href=" and " like this
$replace = array('<a href="' => '', '"' => ''); $img[$b] = strtr("$subject", $replace);
Now lets examine the actual Regex shall we which looks like this <a\shref=\"(.*)\" Ok the first part says match the string <a href=" however there are no spaces in Regex so we replace a single space with \s which tells Regex that this is a space (Again there are lots of these like \w but you need to look at reference materials to understand them all) anyway the next part is this (.*) which says match everything between the two double quotes which we also escape by placing the / before them.
I'll stop there and this little explanation may or may not have helped you and I've refered to reference materials a lot in this description but a simple search on Google for Regex, Regex Tutorials, or PHP Regex will bring up a lot more then I can ever offer on the subject.
Just remember that you don't have to learn it all because it really is a complicated mess but eventually you'll remember a lot of common things and you'll be able to do almost anything with Regex.
-
Hang on one second when the data is being taken out of the database you may have to use stripslashes() function as well that may be your issue as well because the source may become <p/> or something like that.
-
Try this it should be all you need because using $var = htmlentities($var); will make all your HTML render as text and not HTML and there is no reason why that would be a security concern unless they are inserting JavaScript into the page but thats a whole nother story
function clean($var) { $var = strip_tags($var, '<b>,<p>'); $var = mysql_real_escape_string($var); return $var; }
EDIT: Also note that you will get an error if mysql_real_escape_string($var); is used before the database connection is made.
-
I also want to say that if you want the allowed HTML to render as in making something bold then you don't even need this line
$var = htmlentities($var);
-
Based upon your last post your issue is with this line
$var = htmlentities($var);
That will not make your tags work, that will disable them and just display them as standard TEXT and not HTML elements so when the page gets rendered it will look like this
<b>
and not like this BOLD
-
would $_POST work or is it just $_GET?
thanks
When dealing with the URL you can only use $_GET
Think of form data with method="POST" that is when you use the $_POST var but you always use $_GET when dealing with urls like mysite.com?action=true
-
I'll write it out for you if your just protecting user insertion into the database then just do this
<?php function clean($var) { $var = mysql_real_escape_string($var); return $var; } ?>
however if your not putting it into the database and just want to display PHP Code on a PHP page without the Code being fired but displayed instead then do this
<?php function clean($var) { $var = htmlentities($var); return $var; } ?>
If you want to first strip out the required HTML tags and then protect the info for insertion into the database then do this
<?php function clean($var) { $var = strip_tags($var, '<b>,<p>'); $var = mysql_real_escape_string($var); return $var; } ?>
I hope that helps a bit more.
-
i changed the paths to:
$fileName = 'imap.zip';
$createZip->addDirectory('Daouk');
I uploaded the php page to the directory where the zip file exists.
Both the zip file and Daouk directory exist now.
I run the code online, the zip file disappeared and Daouk directory is empty
I think your misunderstanding how it all works.
The class creates a new ZIP file (One that isn't there at first) and the line
$createZip->addDirectory('Daouk');
Adds a new directory inside of the created zip file.
Here is a breakdown of how it all works
$fileName = 'imap.zip';
$createZip->get_files_from_folder('Daouk',$fileName);
What the above does is takes all the files from the folder called Daouk on your webserver and puts it into the zip file which will be created (imap.zip).
If you don't want to place all the files inside a directory but instead only include certain files you can do this
$createZip->addFile($data,$directory_name);
What this does is takes $data and puts it inside of a directory in the ZIP file.
$createZip->addDirectory($directory_name);
Creates a new directory inside of the zip file
And
$createZip->forceDownload($archiveName);
This opens a download window so the user can download the file.
So lets say I want to create a zip file called test.zip and I wanted to place a folder in this zip file called FOLDER and in that folder I wanted to place a file called FILE.php then my code would look like this
$fileName = 'imap.zip';
$createZip->addDirectory('FOLDER');
$createZip->addFile('FILE.php','FOLDER');
$createZip->forceDownload($fileName);
I hope this helps you better understand the code flow a little more.
-
I've done this with zip files but it might be slightly different with images but you can give it a shot.
<?php $url = "URL_TO_IMAGE"; $ext = substr(strrchr($url, '.'), 1); $imgData = file_get_contents($url); $myFile = "image_name.".$ext; $fh = fopen($myFile, 'w') or die("can't open file"); fwrite($fh, $imgData); fclose($fh); ?>
-
Your function does some strange things for instance why are you using
$var = mysql_real_escape_string($var);
That should only be used to insert data into a database if you are just cleaning out HTML just use the following
$var = strip_tags($var, '<b>,<p>');
However your getting things like < because of the line
$var = htmlentities($var);
Also your string
"text text textp>some text here</p>
Should not return everything between the paragraph tags because its improperly formatted
-
Don't try to use absolute urls use things like '../directory' or put it in the same folder where the zip is going to be.
I think there was an issue with this before.
-
Here you go
<?php $string = "Hello And welcome To ^#phpfreaks# the best php forum and the ^#yahoo# is the best email and my ^#facebook# is the best site"; $pattern = '/#(.*?)#/'; preg_match_all($pattern,$string,$data,PREG_SET_ORDER); $a = 0; $count = count($data); while($a < $count) { echo $data[$a][1].'<br />'; $a++; } ?>
-
Hello Everyone,
I'm not new to freelancing but I'm newer to the PHP Freaks forums and even though I'm willing to help out for free in the other sections of the forums I would also like to take the time to advertise my services here.
Here are some of my qualifications:
- 5 Years of PHP
- 5 Years of MySQL
- 7 Years of (X)HTML
- 5 Years of CSS
- 3 Years of Wordpress Development
- 3 Years of JavaScript
- I've also built 3 Facebook applications
- I also have 3 years experience with ASP.NET (1.0 & 2.0) but I no longer contract out work using ASP
- In total I've been programming for just over 8 years
Here are a couple of websites which I have created 100% by myself using PHP.
I've got a solid reputation on another forum called WickedFire, my username there is Aequitas and you can see some 100% positive feedback on my iTrader notifications, I can prove that its me if you send me a private message there in response to this one and I will respond to it.
My prices are very competitive compared to the industry standard, if you are interested in my services I can give you a quote at no charge and am very good with deadlines.
You can contact me by PM or email (minidak03@gmail.com)
Here are a list of things which I am more then capable of handling and is along the lines of what I would like to do for the members here.
* WordPress Plugins
* Converting CSS Templates To Wordpress Themes
* Integrating PayPal In To A Website
* Building Website Scrapers
* Coding A PSD Design or Template Into A Fully Functional Website
* PHP Security (Spam Filtering, Bot Restrictions, PayPal Transaction Security)
* On-Site SEO (Search Engine Optimization) - This includes making SEO friendly URLs and full consultation on how to rank in Google.
This is not a full list of what I can do and am very flexable and responsible when it comes to working with my customers.
Thank You.
Adam Losier.
minidak03@gmail.com
-
Ahh yes a program that adds funny things to your code a good program to use is PSPad its free and I've build some pretty amazing websites with it.
[SOLVED] if's elfif's and else statement help
in PHP Coding Help
Posted
This might not work sorry I didn't test this but I think i see an issue with your SQL statement.
Should be
No need for the single quotes around $type after SELECT because it will be searching for the wrong name unless your name also incldues the single quotes around it.