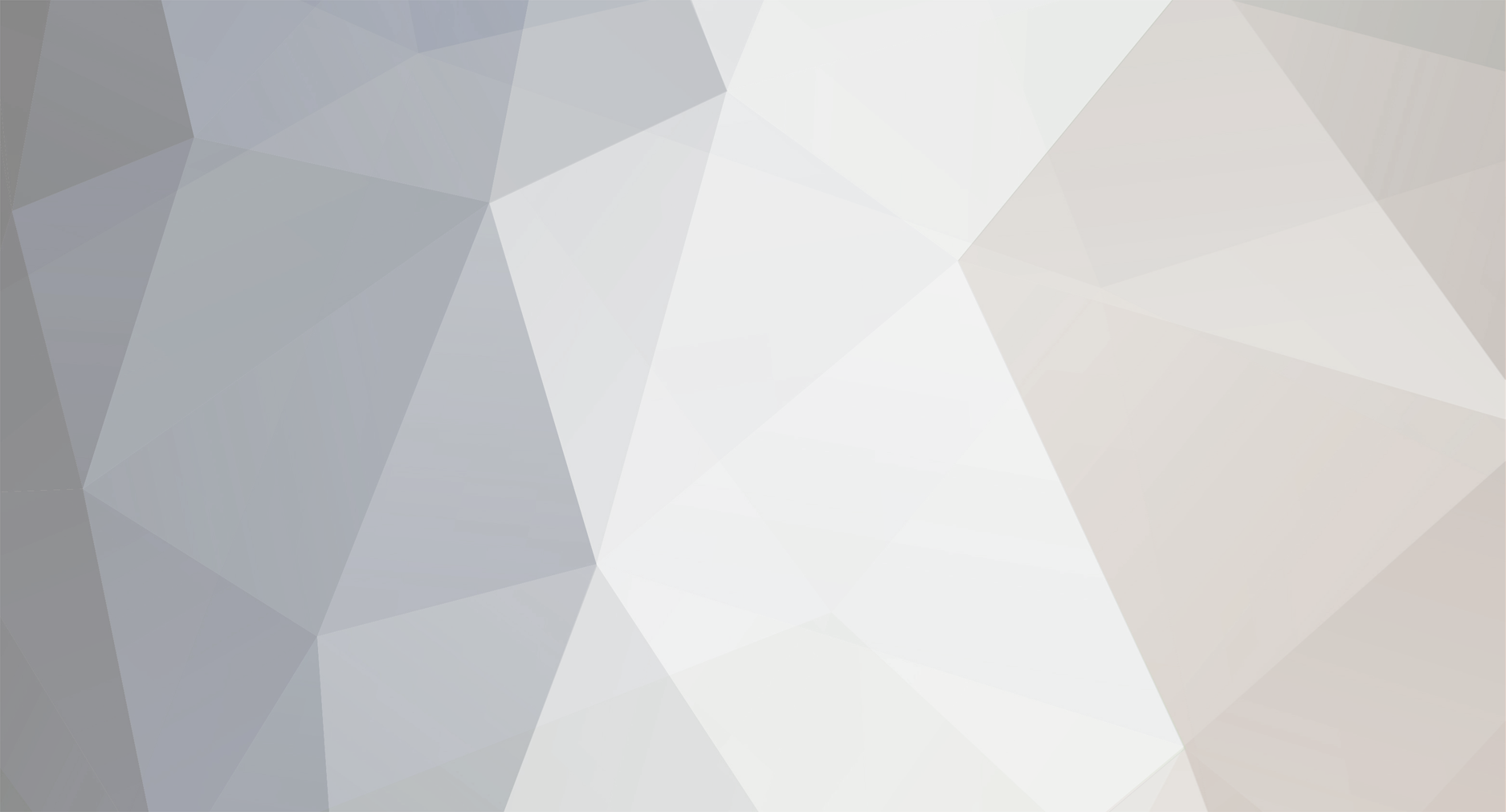
jaxdevil
Members-
Posts
268 -
Joined
-
Last visited
Everything posted by jaxdevil
-
I am generating a drop down box dynamically based on the entries in the database. I want the drop down to only show one line for each unique entry. Like if there are 8 entries that say Jacksonville I want it to just show 1 entry for Jacksonville, etc. So the output will not show any duplicates. Anyone now how I can modify this to display each entry just once? <SELECT NAME="location"> <OPTION VALUE="NONE">-----Select Product Location---- <?php $sql = "SELECT * FROM locations"; $query = mysql_query($sql); while($row = mysql_fetch_array($query)) { ?> <OPTION VALUE="<?=$row['locations']?>"><?=$row['locations']?> <?php } ?> </SELECT>
-
Database Insertion not working, maybe something with the date field?
jaxdevil replied to jaxdevil's topic in PHP Coding Help
Ahh yes, how did I miss that. Thank you very much! SK -
This is a very small footprint of code but it just is not working. It displays the database insertion was successful but the database is not updated. The connection settings are pointing to the correct database. I have never posted to a 'date' database field before so maybe that is the issue. I posted a screenshot of the database below so you can see that, maybe I set the date field wrong or soemthing? <?php if ( $action== "Insert" ) { mysql_connect('localhost','xxx_xxx','xxx'); mysql_select_db('xxx_xxx') or die( "An error has ocured: " .mysql_error (). ":" .mysql_errno ()); $query = "INSERT INTO events(`id`,`date`,`day`,`name`,`description`,`active`) VALUES('$id','$date','$day','$name','$description','$active')" or die( "An error has ocured: " .mysql_error (). ":" .mysql_errno ()); print "<h1>Event Insertion Successful</h1><br>database<br /><br /><em>Updated!</em><br /><br />"; } ?>
-
[SOLVED] PHP Sql UPDATE putting data into wrong columns
jaxdevil replied to jaxdevil's topic in PHP Coding Help
Thats exactly what I did, that solved it. Thanks! -
[SOLVED] PHP Sql UPDATE putting data into wrong columns
jaxdevil replied to jaxdevil's topic in PHP Coding Help
Never mind, solved it. It was a problem with the variable being sent to the script, not the script itself. Thanks anyways! SK -
Oh great, something else now. Well the function is processing, it has no more sql errors, but the data is posting incorrectly. You see the 11 fields, right? Well it is posting the $price variable into the `man` column, and posting the $list variable into the `price` column, and is posting nothing into the `list` column, or rather it is erasing the data that was in the `list` column since it is leaving nothing in that field after the updating has processed. Please look at the revised code and see if you see anything. I put quotes on everything now, just to be safe, but that didn't do it. <?php $query = "UPDATE products SET `supplier`='$supplier',`cat`='$cat',`subcat`='$subcat',`man`='$man',`mod`='$mod',`name`='$name',`list`='$list',`price`='$price',`specs`='$specs',`pdf`='$pdf',`active`='$active' WHERE `mod`='$oldmod'"; mysql_query($query) or die( "An error has ocured: " .mysql_error (). ":" .mysql_errno ()); print "<h1>Inventory Update Successful</h1><br>data<br /><br /><em>Updated!</em><br /><br />"; ?>
-
Oh great, something else now. Well the function is processing, it has no more sql errors, but the data is posting incorrectly. You see the 11 fields, right? Well it is posting the $price variable into the `man` column, and posting the $list variable into the `price` column, and is posting nothing into the `list` column, or rather it is erasing the data that was in the `list` column since it is leaving nothing in that field after the updating has processed. Please look at the revised code and see if you see anything. I put quotes on everything now, just to be safe, but that didn't do it. <?php $query = "UPDATE products SET `supplier`='$supplier',`cat`='$cat',`subcat`='$subcat',`man`='$man',`mod`='$mod',`name`='$name',`list`='$list',`price`='$price',`specs`='$specs',`pdf`='$pdf',`active`='$active' WHERE `mod`='$oldmod'"; mysql_query($query) or die( "An error has ocured: " .mysql_error (). ":" .mysql_errno ()); print "<h1>Inventory Update Successful</h1><br>data<br /><br /><em>Updated!</em><br /><br />"; ?>
-
Thank you Symmetry! That didn't work, but it put me in the right area to see where the problem is. It was the WHERE statement that was the problem, the mod='$mod' was without quotes, I made it `mod`='$mod' and it works now! YAY!! Thanks everyone for the help, I wouldn't have figured it out with out you. Thanks Symmetry!
-
I thought about that too, thats actually why the quotes are off of it, if you can see I had them on everything (I always put them on everything) but since I couldn't figure it out I just tried every combination of ways to format the string trying to 'get lucky'. So $mod is actually the only one I removed the quotes on. I just put them back and its still the same error. Thanks for the idea though. SK
-
The column named mod is the one that has the value of WCCD-WCC650CX1 (the variable for that is $mod), which is why I gathered from the sql error the fault was somewhere around there. I have this code on multiple places in mutliple sites, I modified this one slightly but this portion of the code is almost exactly to the other ones and they all work, I don't know why this one is not. I mean if it is a reserved word I should be able to close it off with the `` at the beginning and end of the word but that isn't working. I appreciate your help
-
I copied the error and my code below. I do not understand what the problem is? I tried encapsulating the word mod in quotes like this `mod` but that didn't help/ Any ideas? A <?php $query = "UPDATE products SET supplier='$supplier',cat='$cat',subcat='$subcat',`man`='$man',`mod`=$mod,name='$name',list='$list',price='$price',specs='$specs',pdf='$pdf',active='$active' WHERE mod= '$mod'"; mysql_query($query) or die( "An error has ocured: " .mysql_error (). ":" .mysql_errno ()); print "<h1>Inventory Update Successful</h1><br>data<br /><br /><em>Updated!</em><br /><br />"; ?>
-
I really wish I could actually get help here, most of the time lately I have to keep figuring these out for myself. Here is the fix: <?php $path1= "./usedimages/$id/".$HTTP_POST_FILES['ufile']['name'][0]; copy($HTTP_POST_FILES['ufile']['tmp_name'][0], $path1); chmod("$path1", 0755); $orig_filename = "$path1"; $new_filename = "./usedimages/$id/$id.jpg"; $status = rename($orig_filename, $new_filename) or exit("Could not add the item"); echo "Item added successfully!"; ?> <?php function createThumbs( $pathToImages, $pathToThumbs, $thumbWidth ) { // open the directory $dir = opendir( $pathToImages ); // loop through it, looking for any/all JPG files: while (false !== ($fname = readdir( $dir ))) { // parse path for the extension $info = pathinfo($pathToImages . $fname); // continue only if this is a JPEG image if ( strtolower($info['extension']) == 'jpg' ) { // load image and get image size $img = imagecreatefromjpeg( "{$pathToImages}{$fname}" ); $width = imagesx( $img ); $height = imagesy( $img ); // calculate thumbnail size $new_width = $thumbWidth; $new_height = floor( $height * ( $thumbWidth / $width ) ); // create a new temporary image $tmp_img = imagecreatetruecolor( $new_width, $new_height ); // copy and resize old image into new image imagecopyresized( $tmp_img, $img, 0, 0, 0, 0, $new_width, $new_height, $width, $height ); // save thumbnail into a file imagejpeg( $tmp_img,"{$pathToThumbs}{$fname}") or die ("Could not create image from JPEG"); } } // close the directory closedir( $dir ); } // call createThumb function and pass to it as parameters the path // to the directory that contains images, the path to the directory // in which thumbnails will be placed and the thumbnail's width. // We are assuming that the path will be a relative path working // both in the filesystem, and through the web for links createThumbs("./usedimages/$id/","./usedimages/$id/thumbs/","150"); ?>
-
I am trying to rename my files as they are uploaded. here is my upload and conversion code now. I just want to modify it to make the filename the same as the variable $id. Any ideas? <?php $path1= "./usedimages/$id/".$HTTP_POST_FILES['ufile']['name'][0]; copy($HTTP_POST_FILES['ufile']['tmp_name'][0], $path1); ?> <?php function createThumbs( $pathToImages, $pathToThumbs, $thumbWidth ) { // open the directory $dir = opendir( $pathToImages ); // loop through it, looking for any/all JPG files: while (false !== ($fname = readdir( $dir ))) { // parse path for the extension $info = pathinfo($pathToImages . $fname); // continue only if this is a JPEG image if ( strtolower($info['extension']) == 'jpg' ) { // load image and get image size $img = imagecreatefromjpeg( "{$pathToImages}{$fname}" ); $width = imagesx( $img ); $height = imagesy( $img ); // calculate thumbnail size $new_width = $thumbWidth; $new_height = floor( $height * ( $thumbWidth / $width ) ); // create a new temporary image $tmp_img = imagecreatetruecolor( $new_width, $new_height ); // copy and resize old image into new image imagecopyresized( $tmp_img, $img, 0, 0, 0, 0, $new_width, $new_height, $width, $height ); // save thumbnail into a file imagejpeg( $tmp_img,"{$pathToThumbs}{$fname}") or die ("Could not create image from JPEG"); } } // close the directory closedir( $dir ); } // call createThumb function and pass to it as parameters the path // to the directory that contains images, the path to the directory // in which thumbnails will be placed and the thumbnail's width. // We are assuming that the path will be a relative path working // both in the filesystem, and through the web for links createThumbs("./usedimages/$id/","./usedimages/$id/thumbs/","150"); ?>
-
[SOLVED] Dropping first symbol or character from a variable return
jaxdevil replied to jaxdevil's topic in PHP Coding Help
Thanks. That is definitely more elegant than what I came up with in the interim... <?php $string = $rate; $new_string = preg_replace("/[^a-zA-Z0-9s]/", "", $string); $newstring = substr($new_string, 0, -2); echo $newstring ?> -
Thanks!
-
Below is my code. based on my servers time (which appears to be off by like 20 minutes) I set the following to say 'work time' when it is between 8:30AM and 5:00PM, and say 'not work time' when it is not those times. Any idea why it is displaying "work timenot work time" (both if statements)? <? if (date("Hi")>0410 and date("Hi")<1240); { ?>work time<? } ?> <? if (date("Hi")>1239 and date("Hi")<2400 or (date("Hi")>0000 and date("Hi")<0411)); { ?>not work time<? } ?>
-
Well, there is my code below. I use the same code (with modification of the gooey HTML center) on another site, changing the sql connection settings of course, the results come up, it paginates, but it only shows 1 single result per page. I can't figure it out. Anyone see what I am doing wrong? <?php $result = mysql_query("SELECT count(*) FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%'"); $num_records = mysql_result($result,0,0); // Set maximum number of rows and columns $max_num_rows = 3; $max_num_columns = 1; $per_page = $max_num_columns * $max_num_rows; // Work out how many pages there are $total_pages = ceil($num_records / $per_page); // Get the current page number if (isset($_GET['page'])) $page = $_GET['page']; else $page = 1; // Work out the limit offset $start = ($page - 1) * $per_page; // Select the results we want including limit and offset $brand = mysql_query("SELECT `brand` FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%' ORDER BY `ID` ASC LIMIT $start, $per_page"); $modelo = mysql_query("SELECT `model` FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%' ORDER BY `ID` ASC LIMIT $start, $per_page"); $dtitle = mysql_query("SELECT `dtitle` FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%' ORDER BY `ID` ASC LIMIT $start, $per_page"); $ad_descrip = mysql_query("SELECT `ad_descrip` FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%' ORDER BY `ID` ASC LIMIT $start, $per_page"); $price2 = mysql_query("SELECT `price2` FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%' ORDER BY `ID` ASC LIMIT $start, $per_page"); $model = mysql_query("SELECT `model` FROM `equip` WHERE drop1 LIKE '%$searcht%' OR drop2 LIKE '%$searcht%' OR dtitle LIKE '%$searcht%' ORDER BY `ID` ASC LIMIT $start, $per_page"); $num_columns = ceil(mysql_num_rows($result)/$max_num_rows); $num_rows = ceil(mysql_num_rows($result)/$num_columns); echo "<center><table>\n"; for ($r = 0; $r < $max_num_rows; $r++){ echo "<tr align=\"center\">\n"; for ($c = 0; $c < $max_num_columns; $c++){ // 1 $x = $r * $max_num_columns + $c; if ($x < mysql_num_rows($result)){ // $y = mysql_result($result, $x, 0); // Commented out so I could show your example $brand = mysql_result($brand, $x, 0); $modelo = mysql_result($modelo, $x, 0); $dtitle = mysql_result($dtitle, $x, 0); $ad_descrip = mysql_result($ad_descrip, $x, 0); $price2 = mysql_result($price2, $x, 0); $model = mysql_result($model, $x, 0); $unformated_price = $price2; $price = number_format($unformated_price, 2, '.', ','); $y = <<<HTML <table> <tr> <td width="400"> <div style="padding-left:80px; padding-right:70px; "> <font face="Tahoma" color="000000" size="2"> <b>Brand:</b> {$brand} <br> </font> <font face="Tahoma" color="000000" size="2"> <b>Model:</b> {$model} <br> </font> <font face="Tahoma" color="000000" size="2"> <b>Description:</b> {$dtitle} <br> </font> <font face="Tahoma" color="000000" size="1"> {$ad_descrip} <br> </font> <font face="Tahoma" color="000000" size="2"> <b>Price:</b> {$price2} <br> </font> </div> </td> <td width="100"> <img src="inventory/tn_{$model}.jpg" border="0"> </td> </tr> </table> <hr> HTML; } else { $y = '<table align="center" border="0" cellpadding="0" cellspacing="0" width="400"> <tr> <td width="16" align="center"><center></center></td> </tr> </table>'; } echo "<td>"; echo "$y"; echo "</td>"; } echo "</tr>\n"; } // Echo page numbers echo "</table></center>\n"; ?> <? // Echo the results for ($i=1;$i <= $total_pages;$i++) { if ($i == $page) echo " $i "; else echo " <a href=\"?gs_cat=$gs_cat&page=$i\">$i</a> ";} ?>
-
I just retried it on a PHP page and it works.. AWESOME! I must have typed something wrong before. Thank you! ;D SK
-
Generate thumbnails of images after uploading
jaxdevil replied to jaxdevil's topic in PHP Coding Help
Thanks, I think I can work with that no prob Thank you! SK -
Generate thumbnails of images after uploading
jaxdevil replied to jaxdevil's topic in PHP Coding Help
Yes, thats exactly what i am trying to do. it doesn't necessarily 'have' to be in another directory, I can even use it appended with a tn_ or something on the filename in the same directory. Any idea? Thanks a ton, SK -
Damn, that works perfect, except I need it to display the HTML output of a PHP page. I tried that on an HTML page and that is exactly what I am trying to do, but I need it for a page that gets dynamically created in PHP, then I want to view the HTML output of that page. What I am trying to do is format a page with all the data for a person, which the PHP will draw from a SQL database, and then the person can copy and paste the html data from there to elsewhere. Any ideas? That was perfect if it worked on PHP pages.
-
Anyone know a way that I can display the html code output data, other than doing the right click and 'View Source'? SK