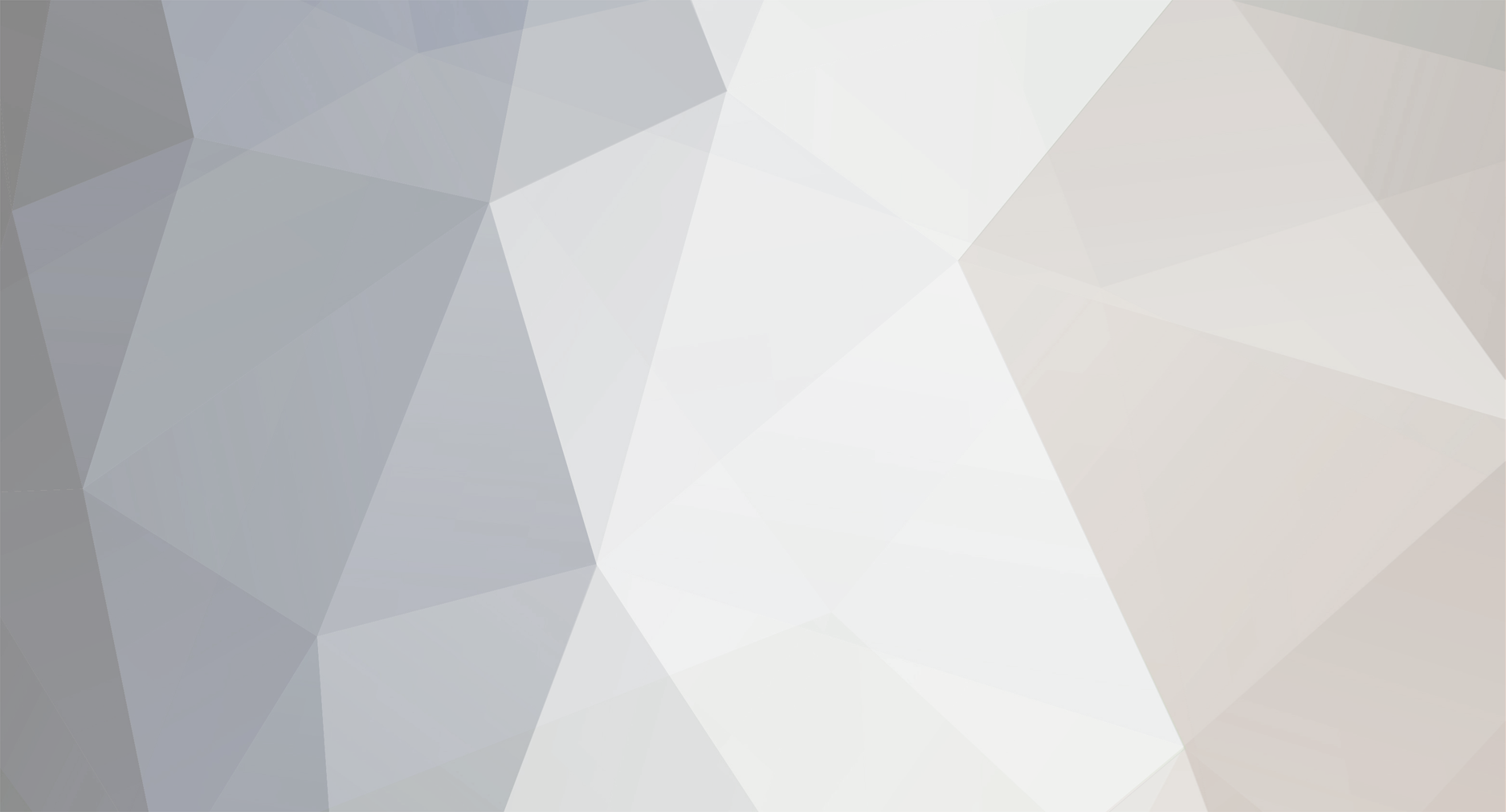
knox203
Members-
Posts
47 -
Joined
-
Last visited
Never
Everything posted by knox203
-
Hello all, I'm not sure if this is better to solve with PHP or my MSSQL query, but here's what I'm working with: I'm pulling my data out of my database with this query: SELECT SUM(O.AmountCharged) AS Amount, right('00'+convert(varchar(4),datepart(year,O.OrderDate)),2) As Year, right('00'+convert(varchar(2),datepart(month,O.OrderDate)),2) as Month FROM dbo.OrderMain O INNER JOIN dbo.Customer C ON O.CustomerID = C.CustomerID WHERE ( C.CustomerCode LIKE 'XXXXXX' ) AND ( O.OrderStatus <> 'x' ) AND ( O.OrderDate >= '04/01/08' AND O.OrderDate <= DATEADD(s,-1,DATEADD(mm, DATEDIFF(m,0,'04/01/09')+1,0)) ) GROUP BY right('00'+convert(varchar(4),datepart(year,O.OrderDate)),2), right('00'+convert(varchar(2),datepart(month,O.OrderDate)),2) ORDER BY right('00'+convert(varchar(4),datepart(year,O.OrderDate)),2), right('00'+convert(varchar(2),datepart(month,O.OrderDate)),2) This returns my data that looks like: Amount Year Month 92.40 08 04 32.34 08 05 140.82 08 06 66.08 08 07 173.58 08 08 168.95 08 10 31.89 08 11 57.68 08 12 58.03 09 03 As you can see in the month column in the results, it's missing september (09). I'd like to fill that gap inside my PHP array with the missing month and a $0 amount, so the data would look like: Array ( [0] => Array ( [Amount] => 32.34 [Month] => 05 [Year] => 08 ) [1] => Array ( [Amount] => 140.82 [Month] => 06 [Year] => 08 ) [2] => Array ( [Amount] => 66.08 [Month] => 07 [Year] => 08 ) [3] => Array ( [Amount] => 173.58 [Month] => 08 [Year] => 08 ) [4] => Array ( [Amount] => 0 [Month] => 09 [Year] => 08 ) [5] => Array ( [Amount] => 168.95 [Month] => 10 [Year] => 08 ) [6] => Array ( [Amount] => 31.89 [Month] => 11 [Year] => 08 ) [7] => Array ( [Amount] => 57.68 [Month] => 12 [Year] => 08 ) [8] => Array ( [Amount] => 58.03 [Month] => 03 [Year] => 09 ) ) These result vary greatly, sometimes an account will be missing multiple months in a row... so I've been trying to figure out a solution that will recognize this dynamically. Anybody got any ideas? Thanks!! - Adam
-
I would do this (if I understand what you're trying to do correctly...): <?php $con = mysql_connect("localhost","root","") or die('Could not connect: ' . mysql_error()); mysql_select_db("smrpg", $con); // Get all the data from the "guestbook" table $result = mysql_query("SELECT * FROM contact ORDER BY commentdate DESC") or die(mysql_error()); echo "<h1>Sailor Moon RPG Questions/Comments - View</h1>"; echo "<table border='0'>"; // keeps getting the next row until there are no more to get while($row = mysql_fetch_array( $result )) { // Print out the contents of each row into a table echo "<tr><td width='15%'>"; echo "<b>Name:</b></td><td>"; echo $row['name']; echo "</td></tr><tr><td>"; echo "<b>E-mail:</b></td><td>"; echo $row['email']; echo "</td></tr><tr><td>"; echo "<b>Question/Comment:</b></td><td>"; echo $row['question']; echo "</td></tr><tr><td>"; echo "<b>Date & Time:</b></td><td>"; echo date("F j, Y @ g:i A T",strtotime($row['commentdate'])); echo "</td></tr>"; echo "<tr><td><a href='mailto:".$row['email']."'>Respond</a>?</td></tr>"; echo "<tr><td> </td></tr>"; } echo "</table>"; ?> It looked like you were trying to define "$email" before there was even a result array (mysql_fetch_array() was below the variable you were trying to define). Instead of creating a variable... we can just display the data straight from the result set using concatenation. Also, you don't need to use an 'if' statement during your connection string, simply using "or die()" will accomplish the same thing with less code.
-
Hey F1Fan, thanks so much for your help! I made the code compare the Timecode instead of DateTime, and it seems to be working just fine! Here's what I ended up with: <?php $drivertimes = array(); $driverstart = array(); foreach ($dates_array as $k=>$arr){ if (isset($driverstart[$arr['Driver']])){ // Check if the start exists if (!isset($drivertimes[$arr['Driver']])) $drivertimes[$arr['Driver']] = 0; $drivertimes[$arr['Driver']] += $arr['Timecode']-$driverstart[$arr['Driver']]; unset($driverstart[$arr['Driver']]); } else{ $driverstart[$arr['Driver']] = $arr['Timecode']; } } ?> Not much different than what you originally gave me... either way, it seems to be working now. Thanks again so much for all your help, I couldn't have done it without ya!! - Adam
-
DateTime is added as a key in the array (along with it's associated value)... I'm creating it with mktime() then date(). Can I just compare the Timecodes (mysql timecode) that's being created with mktime() from the query? When I print_r() the main array, it looks like SQL is sorting it correctly.
-
I have not used array_multisort, so I hope I'm doing this correctly, here's the code: <?php $job_select = " SELECT Driver.DriverCode AS Driver, ManifestDriverEvent.ManifestDriverEventTypeID AS Event, DATEPART(dd, ManifestDriverEvent.StartDateTime) AS Day, DATEPART(mm, ManifestDriverEvent.StartDateTime) AS Month, DATEPART(yyyy, ManifestDriverEvent.StartDateTime) AS Year, DATEPART(hh, ManifestDriverEvent.StartDateTime) AS Hour, DATEPART(mi, ManifestDriverEvent.StartDateTime) AS Minute, DATEPART(ss, ManifestDriverEvent.StartDateTime) AS Second FROM Driver INNER JOIN ManifestDriver ON Driver.DriverID = ManifestDriver.DriverID INNER JOIN ManifestDriverEvent ON ManifestDriver.ManifestDriverID = ManifestDriverEvent.ManifestDriverID INNER JOIN DispatchGroup ON Driver.DispatchGroupID = DispatchGroup.DispatchGroupID WHERE (CONVERT(VARCHAR(25), ManifestDriverEvent.StartDateTime, 110) > CONVERT(VARCHAR(25), DATEADD(dd, - (DAY(GETDATE()) - 1), GETDATE()), 110)) AND (DATEPART(yyyy, ManifestDriverEvent.StartDateTime) = DATEPART(yyyy, GETDATE())) AND (DispatchGroup.DispatchGroup = 'Corp Driv' OR DispatchGroup.DispatchGroup = 'Truck' OR DispatchGroup.DispatchGroup = 'Corridor' OR DispatchGroup.DispatchGroup = 'PRDriv') ORDER BY Driver.DriverCode, ManifestDriverEvent.StartDateTime"; $job_query = mssql_query($job_select) or die("Didn't Work!"); $dates_array = array(); while ( $job_result = mssql_fetch_assoc($job_query) ) { $dates_array[] = array( Driver => $job_result['Driver'], Event => $job_result['Event'], Timecode => mktime($job_result['Hour'], $job_result['Minute'], $job_result['Second'], $job_result['Month'], $job_result['Day'], $job_result['Year']), DateTime => date('m-d-y H:m:s', mktime($job_result['Hour'], $job_result['Minute'], $job_result['Second'], $job_result['Month'], $job_result['Day'], $job_result['Year'])) ); } array_multisort( $dates_array[]["Driver"], SORT_DESC, SORT_NUMERIC, $dates_array[]["Timecode"], SORT_DESC, SORT_NUMERIC); ?> Here's the result: Array ( [007] => 0 [011] => -1234145363 [077] => 107996 [085] => 5353136 [088] => -1249999817 [090] => 5306472 [1031] => -1257786507 [108] => -1247141331 [112] => 10321194 [1164] => 7725662 [1334] => 21009486 [1375] => 15685186 [1378] => 10558885 [1379] => 18388873 [1385] => 18536304 [144] => 5281152 [236] => -1252494674 [313] => -1247382614 [533] => 15843645 [535] => -1252289407 [618] => 13291228 [684] => 5108410 [781] => 5119167 [8] => 0 [843] => -1249773005 [990024] => -1247152218 [990027] => -1247026223 [990047] => -1239257422 [990059] => 15681636 [990097] => 13136420 [990126] => -1260274143 [990141] => 15436738 [990155] => 18190840 [990170] => -1234202890 [990176] => 5076019 [990204] => 7851555 [990209] => 5306374 [990214] => 23716773 [990226] => -1252458675 [990232] => -1252455039 [990233] => -1254910128 [990238] => -1241881658 [990240] => -1249787325 [990242] => -1249996139 [990245] => 2872747 [990262] => -1239656943 [990264] => -1241745027 [990266] => -1252271306 [990267] => -1255151284 [990287] => -1242126584 [990289] => 7685972 [990292] => 0 [990299] => -1244599854 [990304] => 20905112 [990311] => 93666 [990312] => -1250046642 [990317] => -1247069351 [990318] => -1247335793 [990320] => 20844011 [990325] => -1252354228 [990327] => -1252498142 [990328] => -1257585014 [990329] => -1247105193 [990331] => -1260248925 [990332] => 2613548 [990333] => 39596 [990334] => 0 [994] => -1252361310 [9999] => -1249960229 [] => 0 )
-
Haha, you're telling me! My head is running in circles trying to understand the best way to do this. Anyways, here's the output from your code, looks like were definitely getting closer, looks like there's still a couple of oddities: Array ( [007] => 0 [011] => -1234145363 [077] => 107996 [085] => 5353136 [088] => -1249999817 [090] => 5306472 [1031] => -1257786507 [108] => -1247141331 [112] => 10321194 [1164] => 7725662 [1334] => 21009486 [1375] => 15685186 [1378] => 10558885 [1379] => 18388873 [1385] => 18536304 [144] => 5281152 [236] => -1252494674 [313] => -1247382614 [533] => 15843645 [535] => -1252289407 [618] => 13291228 [684] => 5108410 [781] => 5119167 [8] => 0 [843] => -1249773005 [990024] => -1247152218 [990027] => -1247026223 [990047] => -1239257422 [990059] => 15681636 [990097] => 13136420 [990126] => -1260274143 [990141] => 15436738 [990155] => 18190840 [990170] => -1234202890 [990176] => 5076019 [990204] => 7851555 [990209] => 5306374 [990214] => 23716773 [990226] => -1252458675 [990232] => -1252455039 [990233] => -1254910128 [990238] => -1241881658 [990240] => -1249787325 [990242] => -1249996139 [990245] => 2872747 [990262] => -1239656943 [990264] => -1241745027 [990266] => -1252271306 [990267] => -1255151284 [990287] => -1242126584 [990289] => 7685972 [990292] => 0 [990299] => -1244599854 [990304] => 20905112 [990311] => 93666 [990312] => -1250046642 [990317] => -1247069351 [990318] => -1247335793 [990320] => 20844011 [990325] => -1252354228 [990327] => -1252498142 [990328] => -1257585014 [990329] => -1247105193 [990331] => -1260248925 [990332] => 2613548 [990333] => 39596 [990334] => 0 [994] => -1252361310 [9999] => -1249960229 )
-
Here's the return for $drivertimes(): Array ( [002] => 0 [007] => 0 [008] => 0 [011] => 0 [013] => 0 [077] => 0 [085] => 0 [088] => 9 [090] => 18 [1017] => 9 [1031] => 9 [1038] => 18 [108] => 252 [112] => 0 [1164] => 9 [1309] => 0 [1324] => 9 [1334] => 0 [1354] => 0 [1363] => 9 [1375] => 9 [1378] => 9 [1379] => 27 [1381] => 0 [1385] => 0 [1392] => 9 [1396] => 18 [1402] => 0 [144] => 0 [158] => 0 [236] => 0 [238] => 0 [287] => 0 [313] => 0 [533] => 0 [535] => 144 [618] => 0 [684] => 0 [744] => 9 [781] => 0 [8] => 0 [843] => 27 [932] => 9 [983] => 0 [990024] => 0 [990027] => 9 [990047] => 9 [990059] => 9 [990097] => 0 [990126] => 0 [990141] => 45 [990155] => 9 [990168] => 9 [990170] => 9 [990176] => 9 [990204] => 0 [990209] => 0 [990214] => 0 [990226] => 0 [990232] => 0 [990233] => 0 [990238] => 0 [990240] => 0 [990242] => 54 [990245] => 54 [990261] => 9 [990262] => 27 [990264] => 0 [990266] => 0 [990267] => 9 [990277] => 9 [990287] => 18 [990289] => 9 [990292] => 0 [990299] => 9 [990304] => 9 [990309] => 9 [990311] => 0 [990312] => 9 [990317] => 9 [990318] => 9 [990320] => 0 [990325] => 0 [990327] => 0 [990328] => 0 [990329] => 0 [990331] => 0 [990332] => 0 [990333] => 0 [990334] => 0 [994] => 198 [9999] => 0 ) I'm not 100% sure what I'm looking at here, would it be easier to get the total number of seconds returned by adding the differences of the Timecode, then I could just turn that into hours. I should mention that every once in a while there is a result that looks like this: [55] => Array ( [Driver] => 013 [Event] => 2 [Timecode] => 1222099751 [DateTime] => 09-22-08 09:09:11 ) [56] => Array ( [Driver] => 013 [Event] => 2 [Timecode] => 1222113483 [DateTime] => 09-22-08 12:09:03 ) [57] => Array ( [Driver] => 056 [Event] => 1 [Timecode] => 1221668478 [DateTime] => 09-17-08 09:09:18 ) [58] => Array ( [Driver] => 077 [Event] => 1 [Timecode] => 1220363863 [DateTime] => 09-02-08 06:09:43 ) [59] => Array ( [Driver] => 077 [Event] => 2 [Timecode] => 1220363875 [DateTime] => 09-02-08 06:09:55 ) Notice how driver 56 (result #57) has only returned one result, I hope this doesn't screw up what I'm trying to accomplish too much :-\ Thanks for all your help F1Fan!!
-
Yes, that's exactly right, although there are cases where "event 3" and "event 4" pop up, I haven't been able to determine which stands for what (The data is from a closed source application and they're not giving me much info into this) that's why I'm sorting by datatime, to try to avoid comparing by the Event code (even though it seems it would be much easier to do it that way).
-
Driver 1 Result 1 vs Driver 1 Result 2, Driver 1 Result 3 vs Driver 1 Result 4. Sub Total 1 plus Sub Total 2 to give total time worked, then the same thing for the next driver (all drivers are returned in the array with the results sorted by driver, then by datetime). Again, drivers open and close their manifests multiple times per day, so there are multiple results per driver per day.
-
Discomatt, I'm getting these values from MSSQL, here's the code: <?php $job_select = " SELECT Driver.DriverCode AS Driver, ManifestDriverEvent.ManifestDriverEventTypeID AS Event, DATEPART(dd, ManifestDriverEvent.StartDateTime) AS Day, DATEPART(mm, ManifestDriverEvent.StartDateTime) AS Month, DATEPART(yyyy, ManifestDriverEvent.StartDateTime) AS Year, DATEPART(hh, ManifestDriverEvent.StartDateTime) AS Hour, DATEPART(mi, ManifestDriverEvent.StartDateTime) AS Minute, DATEPART(ss, ManifestDriverEvent.StartDateTime) AS Second FROM dbo.Driver INNER JOIN dbo.ManifestDriver ON Driver.DriverID = ManifestDriver.DriverID INNER JOIN dbo.ManifestDriverEvent ON ManifestDriver.ManifestDriverID = ManifestDriverEvent.ManifestDriverID WHERE (CONVERT(VARCHAR(25), ManifestDriverEvent.StartDateTime, 110) > CONVERT(VARCHAR(25), DATEADD(dd, - (DAY(GETDATE()) - 1), GETDATE()), 110)) AND (DATEPART(yyyy, ManifestDriverEvent.StartDateTime) = DATEPART(yyyy, GETDATE())) ORDER BY Driver.DriverCode, ManifestDriverEvent.StartDateTime"; $job_query = mssql_query($job_select) or die("Didn't Work!"); $dates_array = array(); while ( $job_result = mssql_fetch_assoc($job_query) ) { $dates_array[] = array( Driver => $job_result['Driver'], Event => $job_result['Event'], Timecode => mktime($job_result['Hour'], $job_result['Minute'], $job_result['Second'], $job_result['Month'], $job_result['Day'], $job_result['Year']), DateTime => date('m-d-y H:m:s', mktime($job_result['Hour'], $job_result['Minute'], $job_result['Second'], $job_result['Month'], $job_result['Day'], $job_result['Year'])) ); } ?> Allow me to add on to what I previously explained, I want to compare the time difference between the first two results for a driver, then do the same for the next two results and so on, then combine the total time differences for each driver to give the total time worked. I need to do it this way because we have drivers that open a manifest that we dispatch jobs to. The drivers frequently close their manifests when they're not working, this can happen multiple times in a day. It doesn't matter to the drivers how long they work, since they are paid on pure commission depending on how many packages they deliver, but we need to know the total time worked for these guys for various reasons for our dispatchers. Thanks for your help guys! - Adam
-
Thanks for the response, F1Fan. I'm not sure if array_multisort is going to do what I need it to do. Ultimately what I need to do is calculate the time difference of the first two results in the array for a driver, then the next two so on and so forth. I then need to group those results by the corresponding driver to give me a total time worked for each driver. Does that make sense?
-
Hello all, I'm running results of a query into a 2 level array, and am now in need of finding the time difference, of all the results per user. an example of the array: Array ( [1] => Array ( [Driver] => 002 [Event] => 1 [Timecode] => 1220546013 [DateTime] => 09-04-08 09:09:33 ) [2] => Array ( [Driver] => 002 [Event] => 2 [Timecode] => 1220549134 [DateTime] => 09-04-08 10:09:34 ) [3] => Array ( [Driver] => 002 [Event] => 1 [Timecode] => 1220894334 [DateTime] => 09-08-08 10:09:54 ) [4] => Array ( [Driver] => 002 [Event] => 2 [Timecode] => 1220984242 [DateTime] => 09-09-08 11:09:22 ) ) I'm at a loss on how to code this correctly, if anyone could point me into the right direction, it would be greatly appreciated!! My current idea is to put every other result into a separate array (the sql query is sorting the results by time) then find the difference between $array1[0]["timecode"] and $array2[0]["timecode"] so on and so forth, but on top of that, am at a loss on how to group all the users when all is said and done. I've got a feeling this could be done much much more efficiently, someone please prove me right
-
Thanks for your advice everyone. I'll see what I can come up with. (PREG looks like it's the way to go)
-
You know, I'm not too sure, I'm new to regex. I need to group "John M." and "John Middle" as one group (first name) and "Doe" as last name. The names are in all sorts of different formats, so I'll include the rest of the code to hopefully give you a feel for what I'm working with. <?php $odbc = odbc_connect('sms','','') or die(odbc_errormsg()); $strsql= 'SELECT * FROM cardmstr.DBF'; $query = odbc_exec($odbc, $strsql) or die (odbc_errormsg()); while($row = odbc_fetch_array($query)) { // LastName, FirstName if ( ereg("^([A-Za-z]+)(,) ([A-Za-z]+) (J(unio)?r(.)?|S(enio)?r(.)?)?", $row['item_desc'], $new) ) { echo '<b>'.$new[3]." ".$new[1]." ".$new[4].'</b><br />'."\n"; } // FirstName (2nd FirstName) LastName - Emp# elseif ( ereg("^([A-Za-z]+( *)?([A-Za-z]+)?) ([A-Za-z]+)( *)?- (C?[0-9]+)", $row['item_desc'], $new) ) { echo '<b>'.$new[1]." ".$new[4].'</b><br />'."\n"; } // Emp# - FirstName LastName elseif ( ereg("^(C?[0-9]+) (-)? ?([A-Za-z.?]+) ([A-Za-z]+)", $row['item_desc'], $new) ) { echo '<b>'.$new[3]." ".$new[4].'</b><br />'."\n"; } // "Truck #0-9" - Vehicle Type elseif ( ereg("^Truck (#[1-9]+) - ([A-Za-z]+)", $row['item_desc'], $new) ) { echo '<b>'.$row['item_desc'].'</b><br />'."\n"; } // FirstName LastName elseif ( ereg("^([A-Za-z]+ ?[A-Za-z]?.?) ([A-Za-z]+)", $row['item_desc'], $new) ) { echo '<b>'.$new[1]." ".$new[2].'</b><br />'."\n"; } elseif ( trim($row['item_desc']) ) { echo '<b>'.$row['item_desc'].'</b><br />'."\n"; } } odbc_close($odbc); ?> Thanks!
-
Hey effigy, I'm trying to break the names into pieces.
-
Hello all, I'm trying to separate names in a list. I'm having a hard time telling the code to do the same thing if the name format is either "John M. Doe" or "John Middle Doe". Here's the code: <?php $odbc = odbc_connect('sms','','') or die(odbc_errormsg()); $strsql= 'SELECT * FROM cardmstr.DBF'; $query = odbc_exec($odbc, $strsql) or die (odbc_errormsg()); while($row = odbc_fetch_array($query)) { if ( ereg("^([A-Za-z]+ ?[A-Za-z]?.?) ([A-Za-z]+)", $row['item_desc'], $new) ) { echo '<b>'.$new[1]." ".$new[2].'</b><br />'."\n"; } } odbc_close($odbc); ?> Results returned with this code looks like: "John M. Doe" "John Middle" I tried <?php ereg("^([A-Za-z]+ ?([A-Za-z]|[A-Za-z]+)?.?) ([A-Za-z]+)", $row['item_desc'], $new) ?> but fails all together. Any sort of help would be great, thanks! - Adam
-
Hello all! I have a little block of code I wrote to open a .sql file, replace a word in the sql file, then by using output buffering, create a table in a MySQL database. I keep getting a MySQL error returned saying "You have an error in your SQL syntax". Can anyone tell me if something looks blatantly wrong?? Thanks! <?php ob_start(); $file = "temp/test.sql"; $file_open = fopen($file, 'r+') or die("Can't Open File!!"); do { $line = fgets($file_open, filesize($file)); $line = str_replace("UPDATE", "UPDATE_DATE", $line); print $line; } while (!feof($file_open)); fclose($file_open); $create_select = ob_get_contents(); ob_clean(); $host = "sql1"; $username = "test"; $password = "test"; $db_name = "test"; mysql_connect("$host", "$username", "$password") or die("cannot connect"); mysql_select_db("$db_name") or die("cannot select DB"); mysql_query($create_select) or die(mysql_error()); ?>
-
Thanks, worked like a charm!
-
Can anyone tell me why I can't download pdf's from a MySQL Blob field when it works just fine in Firefox and others? <?php require_once("conduit/ic_commission.php"); $invoice_num = $_GET['invoice_num']; $query = "SELECT invoice_num, invoice, invoice_name FROM $databasename.invoice where invoice_num=$invoice_num"; $result = mysql_query($query); $data = mysql_result($result,0,"invoice"); $name = mysql_result($result,0,"invoice_name"); header("Cache-Control: must-revalidate"); header("Content-type: application/pdf"); header("Content-Disposition: attachment; filename=$name"); header("Content-Length: ".filesize($data)); header("Pragma: no-cache"); header("Content-transfer-encoding: binary"); echo $data; ?> Muchas gracias!
-
Hey Lemmin, thanks. That seems to work fine... although, do you think the issue is caused by the System (or PHP) interpreting the first day of the week as Sunday and not Monday? If so, do you know of any way to override that and set Monday as the first day?
-
Hey everyone, I have some code that converts monday before last's date into the week of the year... I want to then convert it back later on to populate a drop down list. Here's the code: <?php $start_date = date('Y-m-d', strtotime("last monday -1 week")); // 08-04-2008 $week_num = date('W', strtotime($start_date)); // 32 ?> So far that works with no issues, it returns with week number 32 (08-04-08) which is correct since Monday is the first day of the week. Although, later on when I try to convert it back to a date with this code, I get "08-11-08" as the result (week #33): <?php echo "<!-- ".date('m-d-Y', strtotime(date('Y')."-01-00 +$week_num weeks"))." -->"; ?> Anyone have any ideas on what could be going wrong? Thanks! - Adam
-
[SOLVED] Creating an array from values of a function
knox203 replied to knox203's topic in PHP Coding Help
Ahh, that did it! I knew I wasn't too far off =) Thanks, Barand! - Adam -
Hey all, I have a simple function I made with some conditional statements, I'm trying to call this function into an array during a do-while loop. When I print the array, I get the amount of keys that should be in it, but no values. Can anyone point out what I might be doing wrong?? Thanks! <?php function driver_restriction($jobcount, $basecount, $no) { if ( !$no ) { if ( $jobcount >= '10' || $basecount >= '120' ) { $grandtotal = "1"; } else { $grandtotal = "2"; } } if ( $no ) { $grandtotal = "2"; } echo $grandtotal; } ?> Code for the do-while: <?php $count = " SELECT date, count(date) as job_count, sum(base) as base_count FROM $databasename.$databasetable WHERE driver = '$username' AND left(dts, 15) = '$dts_select' AND pay_period = '".$_POST['pay_period']."' GROUP BY date"; $count_query = mysql_query($count) or die (mysql_error()); $count_result = mysql_fetch_assoc($count_query); $count_array = array(); do { $daily_job_count = $count_result['job_count']; $daily_base_count = $count_result['base_count']; $count_array[] = driver_restriction("$daily_job_count", "$daily_base_count", "$no_result"); } while ($count_result = mysql_fetch_assoc($count_query)); ?>
-
I see, how do you want the sample data... in a .sql file? You could also just hack something together if you'd like... I could probably end up building on it and making it more efficient. Whichever is easiest for you man!
-
<Unnecessary post...>