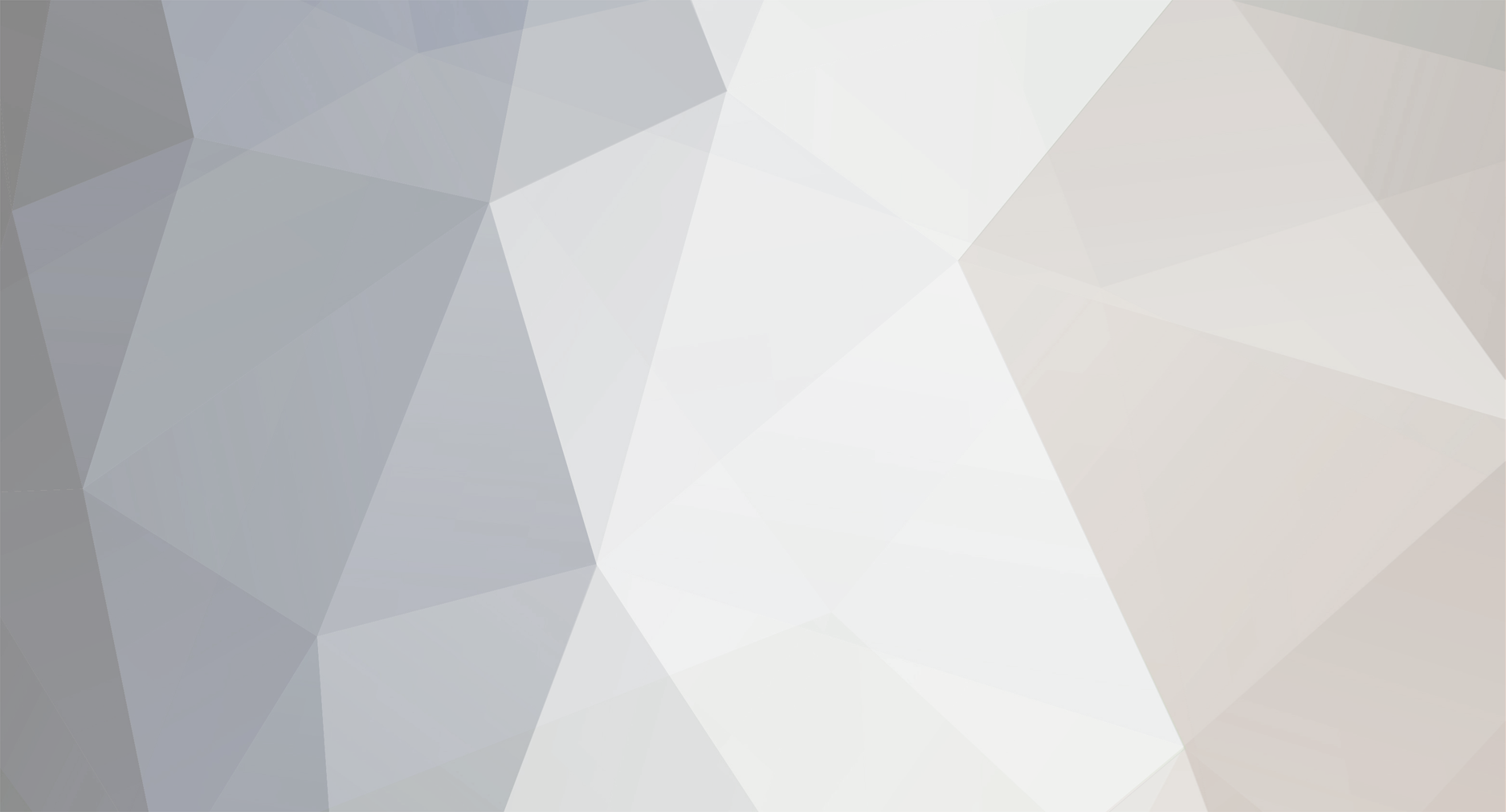
Altec
Members-
Posts
91 -
Joined
-
Last visited
Never
Everything posted by Altec
-
I'm really at a loss at to how to accurately describe what is wrong, so here is some code: <?php $query = mysql_query("SELECT * FROM categories"); if(!$query) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['CAT_DISPLAY_ERROR'] . ' - ' . mysql_error(), 'REFRESH' => '', )); $page->output(); exit; } // List our categories $page = new Page('templates/index.html'); $page->replace_tags(array( 'PAGE_TITLE' => 'Category List', 'MENU' => 'templates/menu.html', 'TITLE' => 'Category List', 'CONTENT' => 'templates/sys/cat_list.php', 'SIDEBAR' => 'templates/sidebar.html', 'FOOTER' => 'templates/footer.html', )); $page->output(); ?> My problem lies in cat_list.php: <?php while($row = mysql_fetch_array($query)) { ?> <input type="radio" name="category" value="<?php echo $row['id']; ?>" /> <?php echo $row['name']; ?><br /> <?php } ?> As you can see, I'm trying to "carry over" $query through to complete the while loop; as expected, this results in nothing. How can I "carry over" this variable? My goal is for no HTML in the PHP code and so far I haven't thought of a way to do so in this situation. For reference, my template engine: <?php class Page { var $page; function Page($template) { if(file_exists($template)) { $this->page = join('', file($template)); } else { die('Template file ' . $template . ' not found.'); } } function parse($file) { ob_start(); include($file); $buffer = ob_get_contents(); ob_end_clean(); return $buffer; } function replace_tags($tags = array()) { if(sizeof($tags) > 0) { foreach ($tags as $tag => $data) { $data = (file_exists($data)) ? $this->parse($data) : $data; $this->page = eregi_replace('{' . $tag . '}', $data, $this->page); } } else { die('No tags designated for replacement.'); } } function output() { echo $this->page; } } ?>
-
Had an extra bracket in there.
-
Hey, after finishing a few more blocks of my code I tried to run it; I'm getting an unexpected T_CASE error on line 112. If you could take a look and help me figure this out that would be great; I don't see anything amiss. <?php // Change display_errors to 0 ini_set('display_errors', 0); // Include the database, strings, and template engine files require_once('includes/database.php'); require_once('includes/strings.php'); require_once('includes/templatesys.php'); // Connect to the database if(!mysql_connect(DB_SERVER, DB_USER, DB_PASS)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['DATABASE_CONNECT_ERROR'] . ' - ' . mysql_error(), 'REFRESH' => '', )); $page->output(); exit; } // Select the database if(!mysql_select_db('phreakyo_sandbox')) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['DATABASE_SELECT_ERROR'] . ' - ' . mysql_error(), 'REFRESH' => '', )); $page->output(); exit; } // Switch function will "switch" between blocks of code based // on the value of $_GET['action']. switch($_GET['action']) { case 'post': // Check if a post was submitted; if not, display form if(!isset($_POST['submit'])) { $page = new Page('templates/index.html'); $page->replace_tags(array( 'PAGE_TITLE' => 'Post New Entry', 'MENU' => 'templates/menu.html', 'TITLE' => 'Post New Entry', 'CONTENT' => 'templates/sys/new_post.php', 'SIDEBAR' => 'templates/sidebar.html', 'FOOTER' => 'templates/footer.html', )); $page->output(); } // Otherwise enter the post into the database elseif(isset($_POST['submit'])) { $fields = array( 'month' => $_POST['month'], 'date' => $_POST['date'], 'year' => $_POST['year'], 'time' => $_POST['time'], 'title' => $_POST['title'], ); foreach($fields as $key => $value) { $value = trim($value); if(empty($value)) { $error .= 'Field ' . $key . ' empty.<br />'; } $fields[$key] = htmlspecialchar(strip_tags($value)); } if(isset($error)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['POST_FIELD_EMPTY'] . '<br /><br />' . $error, 'REFRESH' => '', )); $page->output(); exit; } extract($fields); $entry = $_POST['entry']; $timestamp = strtotime($month . " " . $date . " " . $year . " " . $time); $entry = nl2br($entry); if(!get_magic_quotes_gpc()) { $title = mysql_real_escape_string($title); $entry = mysql_real_escape_string($entry); } $query = "INSERT INTO posts (timestamp,title,entry) VALUES ('$timestamp','$title','$entry')"; if(!mysql_query($query)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['POST_INSERT_ERROR'] . ' - ' . mysql_error(), 'REFRESH' => '', )); $page->output(); } else { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['POST_INSERT_SUCCESS'], 'REFRESH' => 'templates/sys/redirect.html', )); $page->output(); } } } break; case 'category': // Check if we submitted a new category if(isset($_POST['sub_new_cat'])) { $cat_name = trim(htmlspecialchar(strip_tags($_POST['new_cat']))); if(empty($cat_name)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['CAT_SUB_ERROR'], 'REFRESH' => '', )); $page->output(); exit; } if(!get_magic_quotes_gpc()) { $cat_name = mysql_real_escape_string($cat_name); } $query = "INSERT INTO categories (name) VALUES ('$cat_name')"; if(!mysql_query($query)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['CAT_INSERT_ERROR'] . ' - ' . mysql_error(), 'REFRESH' => '', )); $page->output(); } else { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['CAT_INSERT_SUCCESS'], 'REFRESH' => 'templates/sys/redirect.html', )); $page->output(); } } // Check if we want to edit a category elseif(isset($_POST['edit_cat'])) { } // Check if we want to delete a category elseif(isset($_POST['del_cat'])) { } // Otherwise we just came here else { $query = "SELECT * FROM categories"; if(!mysql_query($query)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['CAT_DISPLAY_ERROR'] . ' - ' . mysql_error(), 'REFRESH' => '', )); $page->output(); exit; } // List our categories $page = new Page('templates/index.html'); $page->replace_tags(array( 'PAGE_TITLE' => 'Category List', 'MENU' => 'templates/menu.html', 'TITLE' => 'Category List', 'CONTENT' => 'templates/sys/cat_list.php', 'SIDEBAR' => 'templates/sidebar.html', 'FOOTER' => 'templates/footer.html', )); $page->output(); } break; default: $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => $string['NO_ACTION'], 'REFRESH' => '', )); $page->output(); break; } // Close the MySQL connection link mysql_close(); ?> I know it is a bit long; line 112 reads " case 'category': "
-
Hey guys, A couple nights ago I found an article on how to write template engine in PHP. I followed the tutorial and am using it now in my scripts. However, as my scripts get more advanced, the more I realize I need to understand EXACTLY how the script is working and how to expand upon it. Here is my code: <?php class Page { var $page; function Page($template) { if(file_exists($template)) { $this->page = join('', file($template)); } else { die('Template file ' . $template . ' not found.'); } } function parse($file) { ob_start(); include($file); $buffer = ob_get_contents(); ob_end_clean(); return $buffer; } function replace_tags($tags = array()) { if(sizeof($tags) > 0) { foreach ($tags as $tag => $data) { $data = (file_exists($data)) ? $this->parse($data) : $data; $this->page = eregi_replace('{' . $tag . '}', $data, $this->page); } } else { die('No tags designated for replacement.'); } } function output() { echo $this->page; } } ?> Very very very simple. Obviously the pages can be created by: <?php $page = new Page('templates/index.html'); $page->replace_tags(array( 'PAGE_TITLE' => 'Post New Entry', 'MENU' => 'templates/menu.html', 'TITLE' => 'Post New Entry', 'CONTENT' => 'templates/sys/form.php', 'SIDEBAR' => 'templates/sidebar.html', 'FOOTER' => 'templates/footer.html', )); $page->output(); ?> However, I have hit a small snag... Can I nest it like so: <?php $page = new Page('templates/index.html'); $page->replace_tags(array( 'PAGE_TITLE' => 'Post New Entry', 'MENU' => 'templates/menu.html', 'TITLE' => 'Post New Entry', 'CONTENT' => $content = new Page('templates/main.html'); $content->replace_tags(array( 'MAIN' => 'Hi.', )); $content->output(); , 'SIDEBAR' => 'templates/sidebar.html', 'FOOTER' => 'templates/footer.html', )); $page->output(); ?> I'm afraid everything will blow up if I try to do that. Also, the tutorial I followed is about four (4) years old; are there any apparent security flaws or updates I can make? Thanks!
-
I'm not really sure how that will help you... (Team Viewer)
-
Right; what exactly will that do for me, other than print out an array?
-
For example, say I had three phrases. If Bob comes along, I want to say "Hi, Bob!" If Frank comes along, I want to say "Hi, Frank!" Perhaps a stranger comes along. I might want to just nod and give a quick hi. On my page I would have three links: one for Bob, one for Frank, and one for the stranger. <a href="say.php?person=bob">Bob</a> <a href="say.php?person=frank">Frank</a> <a href="say.php?person=stranger">Stranger</a> Notice how I am "passing" the person to the script "say.php" throught the URL. Let's start say.php: <?php $person = $_GET['person']; ?> That says "I want to define variable Person to the value of the Person variable passed in the URL." Now we just need to use either A) An IFTHEN, ELSEIF, ELSE structure or B) A switch function. Both these options will decide which phrase to say depending on the person. <?php $person = $_GET['person']; $phrases = array( 'bob' => 'Hi, Bob!', 'frank' => 'Hi, Frank!', 'stranger' => '*nods* Hi.', ); // IFTHEN, ELSEIF, ELSE if($person == $phrases['bob']) { echo $phrases['bob']; // Bob walks by } elseif($person == $phrases['frank']) { echo $phrases['frank']; // Frank walks by } else { // Must be a stranger echo $phrases['stranger']; } // Switch function switch($person) { case 'bob': echo $phrases['bob']; // Bob walks by break; case 'frank': echo $phrases['frank']; // Frank walks by break; case 'stranger': echo $phrases['stranger']; // A stranger walks by break; default: echo 'No one has walked by.'; break; } ?>
-
I'm not seeing how that will help me...
-
Thank you very much for the link about extract(). It was very helpful and is exactly what I was looking for. Also, what do you mean by "print the $_POST array first?" EDIT: Do you mean to actually define a new array with the $_POST values I want? <?php $fields = array( 'month' => $_POST['month'], 'date' => $_POST['date'], 'year' => $_POST['year'], 'time' => $_POST['time'], 'title' => $_POST['title'], ); foreach($fields as $key => $value) { $value = trim($value); if(empty($value)) { $error .= 'Field ' . $key . ' empty.'; } $fields[$key] = htmlspecialchar(strip_tags($value)); } if(isset($error)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => 'Error when processing post:<br /><br />' . $error, 'REFRESH' => '', )); $page->output(); exit; } ?>
-
I currently have this code: $month = htmlspecialchars(strip_tags($_POST['month'])); $date = htmlspecialchars(strip_tags($_POST['date'])); $year = htmlspecialchars(strip_tags($_POST['year'])); $time = htmlspecialchars(strip_tags($_POST['time'])); $title = htmlspecialchars(strip_tags($_POST['title'])); $entry = $_POST['entry']; To save a bit of space and add error detection without a massive if statement, I decided to do this: foreach($_POST as $key => $value) { $value = trim($value); if(empty($value)) { $error .= 'Field ' . $key . ' empty.<br />'; } if($key != 'entry') { $_POST[$key] = htmlspecialchars(strip_tags($value)); } } if(isset($error)) { $page = new Page('templates/sys/info.html'); $page->replace_tags(array( 'CONTENT' => 'Error when processing post:<br /><br />' . $error, 'REFRESH' => '', )); $page->output(); exit; } However, the rest of my code still uses the original variable names ($date instead of $_POST['date']). Instead of going through the rest of my code and several included files and changing all the variable names, how can I easily make a new variable with the name of $key with the value of $value? For some reason I just can't think of how to do this. Thanks.
-
Right now I'm doing: ini_set('display_errors', 0); But you're saying that on a live server is should be off by default?
-
I assume in the php.ini? Apparently my host has left that on. Is it a major security concern?
-
I really don't know how to explain my problem without posting code first, so bear with me. // Connect to the database if(!mysql_connect('localhost', DB_USER, DB_PASS)) { $page = new Page('error.html'); $page->replace_tags(array( 'TYPE_OF_ERROR' => $string['DATABASE_CONNECT_ERROR'], 'ERROR_CONTENT' => mysql_error(), )); $page->output(); exit; } All that does is attempt to connect to the MySQL database. If it fails it outputs an error page (error.html). However, it automatically outputs an error THEN outputs the error page, which results in this: How can I prevent (or "catch") the error from being displayed?
-
Yay for simple, simple mistakes I make! <?php if(isset($_POST['flag'])) { $id = (int)$_POST['comment']; $entry_id = (int)$_POST['entry']; require_once('recaptchalib.php'); $privatekey = "rawr"; $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if (!$resp->is_valid) { die ("The reCAPTCHA wasn't entered correctly. Go back and try it again." . "(reCAPTCHA said: " . $resp->error . ")"); } mysql_connect ('localhost', 'rawr', 'rawr') ; mysql_select_db ('rawr'); $timestamp = strtotime("now"); $result = mysql_query("UPDATE comments SET status='flagged', flagged_date='$timestamp' WHERE id='$id' AND entry='$entry_id'") or print ("There was an error flagging the comment.".mysql_error()); if ($result != false) { print "<p>Thank you. Comment ID: $id under Entry: $entry_id has been flagged. It has been added to the moderation queue and will be handled shortly.</p>"; } } else { mysql_connect ('localhost', 'rawr', 'rawr') ; mysql_select_db ('rawr'); if (!isset($_GET['id']) || !is_numeric($_GET['id']) || !isset($_GET['entry']) || !is_numeric($_GET['entry'])) { die("Invalid ID specified."); } $id = (int)$_GET['id']; $entry_id = (int)$_GET['entry']; $sql2 = "SELECT status FROM comments WHERE id='$id' AND entry='$entry_id' LIMIT 1"; if (mysql_query($sql2 == 'public')) { $sql = "SELECT * FROM comments WHERE id='$id' AND entry='$entry_id' LIMIT 1"; $result = mysql_query($sql) or die("Can't select comment from table comments.<br />" . $sql . "<br />" . mysql_error()); while($row = mysql_fetch_array($result)) { $id = stripslashes($row['id']); $entry = stripslashes($row['entry']); $name = stripslashes($row['name']); $url = stripslashes($row['url']); $comment = stripslashes($row['comment']); $timestamp = date("l, F d, Y", $row['timestamp']); ?> <p>Below is the comment you have chosen to flag. This feature is to be used ONLY to flag the following type(s) of comments: spam, advertising messages, and problematic (harassment, fighting, or rude) comments.</p> <hr /> <?php echo $name; ?> | URL: <?php echo $url; ?> <blockquote><p><?php echo $comment; ?></p></blockquote> Entry ID: <?php echo $entry; ?> | Comment ID: <?php echo $id; ?> | Date: <?php echo $timestamp; ?> <hr /> <br /> <p>Please solve the following CAPTCHA (to prevent misuse of this feature) and click "Flag This Comment."</p> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <p> <?php require_once('recaptchalib.php'); $publickey = "rawr"; echo recaptcha_get_html($publickey); ?><br /><br /> </p> <p> <input type="hidden" name="comment" id="comment" value="<?php echo $id; ?>" /> <input type="hidden" name="entry" id="entry" value="<?php echo $entry_id; ?>" /> <input type="submit" name="flag" id="flag" tabindex="2" value="Flag This Comment" /> </p> </form> <?php } } else { echo '<p>The comment you have chosen to flag has already been flagged. It will be moderated shortly.</p>'; } } ?> The above code is my script to flag a comment. It worked perfectly before I added these lines: $sql2 = "SELECT status FROM comments WHERE id='$id' AND entry='$entry_id' LIMIT 1"; if (mysql_query($sql2 == 'public')) { // ........ } I was hoping that would check if the comment has not been flagged before. However, every single public comment I try to flag I can't because this says it is already flagged. I see no problem with the code... Anyone? mod edit: code tags added.
-
Here is what I have so far: <?php switch ($_GET['page']) { case 'process': function db_connect() { DEFINE('SQL_USER','blah'); DEFINE('SQL_PASS','blah'); DEFINE('SQL_HOST','localhost'); DEFINE('SQL_DB','blah'); mysql_connect(SQL_HOST, SQL_USER, SQL_PASS); mysql_select_db(SQL_DB); } db_connect(); $name = mysql_real_escape_string($_POST['name']); $desc = mysql_real_escape_string($_POST['desc']); $album = (int)$_POST['album']; $alt = mysql_real_escape_string($_POST['alt']); if(isset($_GET['filename'])) { $filename = $_GET['filename']; } else { $filename = basename($_SESSION['photo_filename']); } if(empty($album)) { die('You must choose an existing album.'); } $query = mysql_query("INSERT INTO photos (name, description, album, alt, filename) VALUES ('$name', '$desc', '$album', '$alt', '$filename'"); if($query == true) { echo '<p>Photo "'.$filename.'" was successfully added to the database. Photo is now available for public viewing.</p>'; } mysql_close(); break; case 'editbyname': if(isset($_POST['filename'])) { $filename = $_POST['filename']; ?> <p>Use the form below to edit the photo's details.</p> <form method="post" action="edit_photo.php?page=process&filename=<?php echo $filename; ?>"> <table border="0"> <tr><td>Name:</td><td><input type="text" id="name" name="name" size="50" maxlength="255" /></td></tr> <tr><td>Description:</td><td><input type="text" id="desc" name="desc" size="50" /></td></tr> <tr><td>Album:</td><td><select id="album" name="album"> <?php function db_connect() { DEFINE('SQL_USER','blah'); DEFINE('SQL_PASS','blah'); DEFINE('SQL_HOST','localhost'); DEFINE('SQL_DB','blah'); mysql_connect(SQL_HOST, SQL_USER, SQL_PASS); mysql_select_db(SQL_DB); } db_connect(); $query = mysql_query("SELECT * FROM albums"); while(list($id, $name) = mysql_fetch_row($query)) { ?> <option value="<?php echo $id; ?>"><?php echo $name; ?></option> <?php } mysql_close(); ?> </select></td></tr> <tr><td>Alt:</td><td><input type="text" id="alt" name="alt" size="50" maxlength="255" /></td></tr> <tr><td> </td><td><input type="submit" id="submit" name="submit" value="Add Photo" /></td></tr> </table> </form> <?php } break; default: if(isset($_SESSION['photo_filename'])) { ?> <p>You have just uploaded the following photo: <?php echo $_SESSION['photo_filename']; ?></p> <p>Use the form below to edit the photo's details.</p> <form method="post" action="edit_photo.php?page=process"> <table border="0"> <tr><td>Name:</td><td><input type="text" id="name" name="name" size="50" maxlength="255" /></td></tr> <tr><td>Description:</td><td><input type="text" id="desc" name="desc" size="50" /></td></tr> <tr><td>Album:</td><td><select id="album" name="album"> <?php function db_connect() { DEFINE('SQL_USER','blah'); DEFINE('SQL_PASS','blah'); DEFINE('SQL_HOST','localhost'); DEFINE('SQL_DB','blah'); mysql_connect(SQL_HOST, SQL_USER, SQL_PASS); mysql_select_db(SQL_DB); } db_connect(); $query = mysql_query("SELECT * FROM albums"); while(list($id, $name) = mysql_fetch_row($query)) { ?> <option value="<?php echo $id; ?>"><?php echo $name; ?></option> <?php } mysql_close(); ?> </select></td></tr> <tr><td>Alt:</td><td><input type="text" id="alt" name="alt" size="50" maxlength="255" /></td></tr> <tr><td> </td><td><input type="submit" id="submit" name="submit" value="Add Photo" /></td></tr> </table> </form> <?php } else { ?> <p>Type in the filename of the photo you want to edit (not added to the database yet) <strong>or</strong> type in a photo id.</p> <form method="post" action="edit_photo.php?page=editbyname"> Filename: <input type="text" id="filename" name="filename" size="50" value="example.jpg" /> <input type="submit" value="Edit" /> </form> <?php } break; } ?> When you go to edit a photo the script does nothing. There is no output although here should be. I can't find anything.
-
Grr. Okay, this script is supposed to add a photo's description to the database. (I'm making a gallery). When I click submit, I get a 404 error and nothing is added. <?php switch ($_GET['page']) { case 'process': if(isset($_GET['submit'])) { function db_connect() { DEFINE('SQL_USER','blah'); DEFINE('SQL_PASS','blah'); DEFINE('SQL_HOST','localhost'); DEFINE('SQL_DB','blah'); mysql_connect(SQL_HOST, SQL_USER, SQL_PASS); mysql_select_db(SQL_DB); } db_connect(); $name = mysql_real_escape_string($_POST['name']); $desc = mysql_real_escape_string($_POST['desc']); $album = (int)$_POST['album']; $alt = mysql_real_escape_string($_POST['alt']); $filename = $_SESSION['photo_filename']; if(empty($album)) { die('You must choose an existing album.'); } $query = mysql_query("INSERT INTO photos (name, description, album, alt, filename) VALUES ($name, $desc, $album, $alt, $filename"); if($query == true) { echo '<p>Photo "'.$filename.'" was successfully added to the database. Photo is now available for public viewing.</p>'; } mysql_close(); } break; default: if(isset($_SESSION['photo_filename'])) { ?> <p>You have just uploaded the following photo: <?php echo $_SESSION['photo_filename']; ?></p> <p>Use the form below to edit the photo's details.</p> <form method="post" action="edit_photo?page=process"> <table border="0"> <tr><td>Name:</td><td><input type="text" id="name" name="name" size="100" maxlength="255" /></td></tr> <tr><td>Description:</td><td><input type="text" id="desc" name="desc" size="100" /></td></tr> <tr><td>Album:</td><td><select id="album" name="album"> <?php function db_connect() { DEFINE('SQL_USER','blah'); DEFINE('SQL_PASS','blah'); DEFINE('SQL_HOST','localhost'); DEFINE('SQL_DB','blah'); mysql_connect(SQL_HOST, SQL_USER, SQL_PASS); mysql_select_db(SQL_DB); } db_connect(); $query = mysql_query("SELECT * FROM albums"); while(list($id, $name) = mysql_fetch_row($query)) { ?> <option value="<?php echo $id; ?>"><?php echo $name; ?></option> <?php } mysql_close(); ?> </select></td></tr> <tr><td>Alt:</td><td><input type="text" id="alt" name="alt" size="100" maxlength="255" /></td></tr> <tr><td> </td><td><input type="submit" id="submit" name="submit" value="Add Photo" /></td></tr> </table> </form> <?php } break; } ?> I know the code is redundant; at least the database function. Can anyone see what is wrong? Thanks.
-
Thanks for all the help. Here is what I have so far: <?php switch ($_GET['page']) { case 'process': if((!empty($_FILES['uploadedfile'])) && ($_FILES['uploadedfile']['error'] == 0)) { $filename = basename($_FILES['uploadedfile']['name']); $path = pathinfo($_SERVER['SCRIPT_FILENAME']); $serv_path = str_replace('admin', 'photos', $path['dirname']); $ext = substr($filename, strrpos($filename, '.') + 1); if (($ext == 'jpg') && ($_FILES['uploadedfile']['type'] == 'image/jpeg') && ($_FILES['uploadedfile']['size'] < 2097152)) { $newname = $serv_path.'/'.$filename; if (!file_exists($newname)) { if ((move_uploaded_file($_FILES['uploadedfile']['tmp_name'],$newname))) { echo 'The photo has been saved as: '.$newname; $_SESSION['photo_filename'] = $filename; } else { echo 'Error: A problem occurred during file upload.'; } } else { echo 'Error: File '.$_FILES["uploadedfile"]["name"].' already exists.'; } } else { echo 'Error: Only .JPG images under 2 megabytes are accepted for upload.'; } } else { echo 'Error: No file uploaded.'; } break; default: ?> <p>Use this form to upload a photo.</p> <form enctype="multipart/form-data" action="add_new_photo.php?page=process" method="POST"> <input type="hidden" name="MAX_FILE_SIZE" value="2097152" /> Choose a photo to upload: <input name="uploadedfile" type="file" /><br /> <input type="submit" value="Upload Photo" /> </form> <?php break; } ?> It works like a charm. However, I'd like to follow up on phpSensei's idea, but with a small twist. I want the file to be uploaded but with a different filename. Here is what I tried: <?php switch ($_GET['page']) { case 'process': function rand_string($len, $chars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789') { $string = ''; for ($i = 0; $i < $len; $i++) { $pos = rand(0, strlen($chars)-1); $string .= $chars{$pos}; } return $string; } if((!empty($_FILES['uploadedfile'])) && ($_FILES['uploadedfile']['error'] == 0)) { $filename = rand_string(10).'.jpg'; $path = pathinfo($_SERVER['SCRIPT_FILENAME']); $serv_path = str_replace('admin', 'photos', $path['dirname']); $ext = substr($filename, strrpos($filename, '.') + 1); if (($ext == 'jpg') && ($_FILES['uploadedfile']['type'] == 'image/jpeg') && ($_FILES['uploadedfile']['size'] < 2097152)) { $newname = $serv_path.'/'.$filename; if (!file_exists($newname)) { if ((move_uploaded_file($_FILES['uploadedfile']['tmp_name'],$newname))) { echo 'The photo has been saved as: '.$newname; $_SESSION['photo_filename'] = $filename; } else { echo 'Error: A problem occurred during file upload.'; } } else { echo 'Error: File '.$_FILES["uploadedfile"]["name"].' already exists.'; } } else { echo 'Error: Only .JPG images under 2 megabytes are accepted for upload.'; } } else { echo 'Error: No file uploaded.'; } break; default: ?> <p>Use this form to upload a photo.</p> <form enctype="multipart/form-data" action="add_new_photo.php?page=process" method="POST"> <input type="hidden" name="MAX_FILE_SIZE" value="2097152" /> Choose a photo to upload: <input name="uploadedfile" type="file" /><br /> <input type="submit" value="Upload Photo" /> </form> <?php break; } ?> However, the name doesn't change when the same image is uploaded. Also, there is a fundamental flaw in naming the file right there, if you know what I mean.
-
The only reason I have the MAX_FILE_UPLOAD size as a hidden form field is because most browsers will catch it. It is by no means an attempt at setting a limit; PHP has an upload limit which is two megabytes. If you didn't notice, the limit (2097152 bytes) is coded into the script itself. @toplay: Basically I just have to change all instances of $_FILES["uploaded_file"] to $_FILES["uploadedfile"], yes?
-
I'm currently writing a gallery with PHP and MySQL and I'm having a little trouble with uploading the photo. Here is what I have right now: <?php switch ($_GET['page']) { case 'process': $_FILES["uploaded_file"] = $_POST["uploadedfile"]; if((!empty($_FILES["uploaded_file"])) && ($_FILES['uploaded_file']['error'] == 0)) { $filename = basename($_FILES['uploaded_file']['name']); $ext = substr($filename, strrpos($filename, '.') + 1); if (($ext == "jpg") && ($_FILES["uploaded_file"]["type"] == "image/jpeg") && ($_FILES["uploaded_file"]["size"] < 2097152)) { $newname = '/home/phreakyo/public_html/playground/gallery/photos/'.$filename; if (!file_exists($newname)) { if ((move_uploaded_file($_FILES['uploaded_file']['tmp_name'],$newname))) { echo 'The photo has been saved as: '.$newname; $_SESSION['photo_filename'] = $filename; } else { echo 'Error: A problem occurred during file upload.'; } } else { echo 'Error: File '.$_FILES["uploaded_file"]["name"].' already exists.'; } } else { echo 'Error: Only .JPG images under 2 megabytes are accepted for upload.'; } } else { echo 'Error: No file uploaded.'; } break; default: ?> <p>Use this form the upload a photo. All uploaded photos are saved to "/photos/."</p> <form enctype="multipart/form-data" action="add_new_photo.php?page=process" method="POST"> <input type="hidden" name="MAX_FILE_SIZE" value="2097152" /> Choose a photo to upload: <input name="uploadedfile" type="file" /><br /> <input type="submit" value="Upload Photo" /> </form> <?php break; } ?> Every time I upload a photo I get "Error: No file uploaded" which means that the file doesn't get uploaded. This is my first attempt at uploading files with PHP, so I'm sure the answer is pretty obvious. Anyone?
-
I have the following code: $query1 = mysql_query("SELECT `ID` FROM `members` WHERE username = '{$username}' LIMIT 1;"); if ($query1) { $memid = $query1; // E-mail message $subject = 'Activation required for Ballistic Designs'; $message = 'Dear '.$username.',\n'; $message .= 'Thank you for registering at Ballistic Designs. Before we can activate your account one last step must be taken to complete your registration.\n\nPlease note - you must complete this last step to become a registered member. You will only need to visit this url once to activate your account.\n\nTo activate your account, please visit this URL:'; $message .= 'http://www.phreakyourgeek.com/activate.php?id='.$memid.'&conf='.$confcode; mail($email,$subject,$message); header('Location: index.php?msg=true'); } First off, I don't know if I'm getting the member's ID correctly; normally I'd do $_SESSION['member_ID'], but this is the registration script so I haven't set the session yet. Furthermore, the e-mail comes as: In the case above, user "Rawr" has the ID 3 in the database, which means that I'm not getting the member ID correctly. Can anyone help?