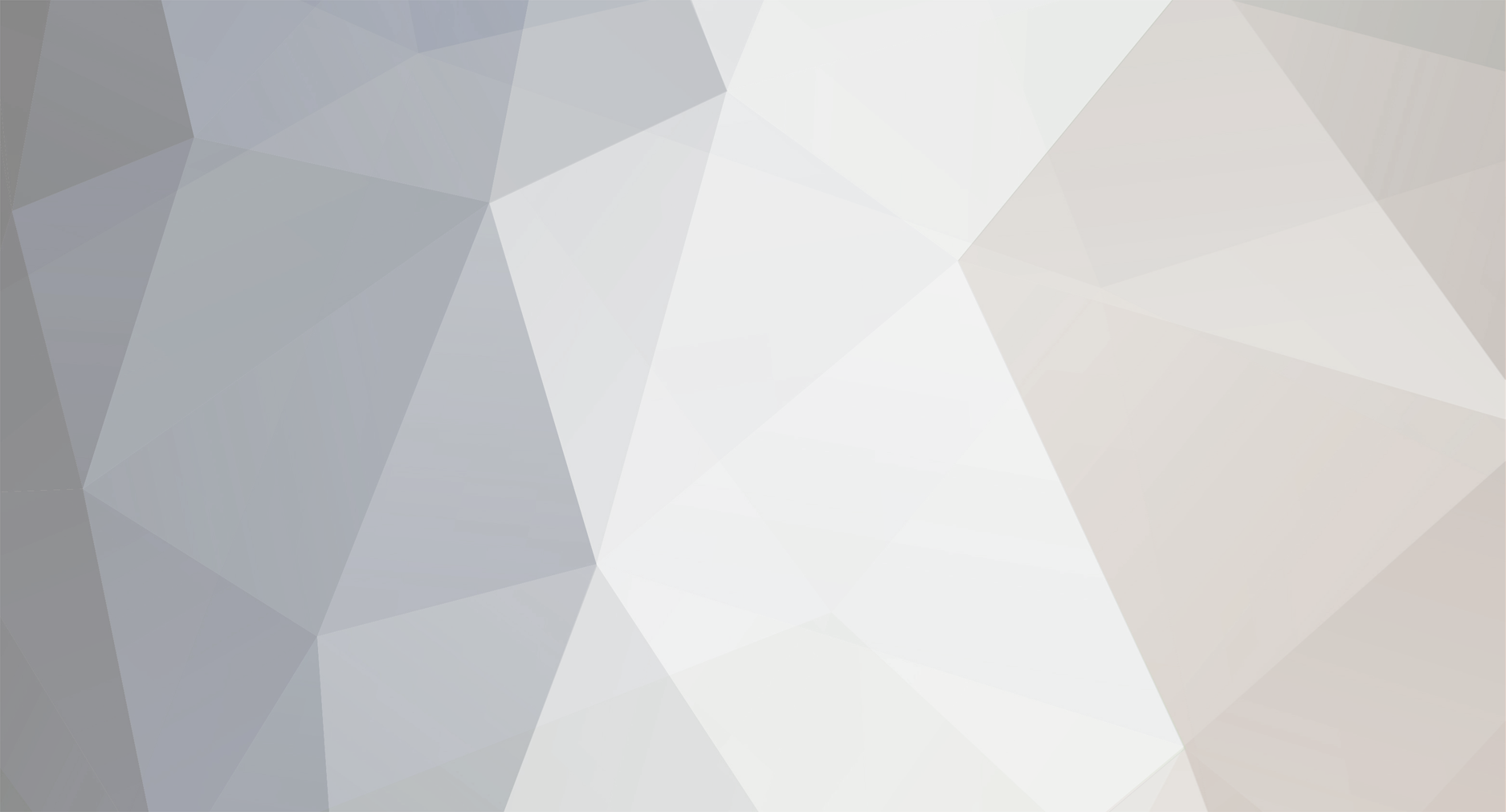
sjslovechild
New Members-
Posts
7 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
sjslovechild's Achievements

Newbie (1/5)
0
Reputation
-
Ok here is what I'm trying to do. There is a link in a email that is sent out to the people who use this app. When they click on the email link they are brought to this site which is password protected. So they have to enter their username and password. What I want to below script to do is to log them in while checking to see if the $id variable is set. If it is, then the script is to take them to the page in the email link. If not take them to the submit job page. <?php $id=$_POST["id"]; $cmd = $_POST['cmd']; $connection = mysql_connect("host", "user", "pass"); mysql_select_db("database", $connection) or die(mysql_error()); switch($cmd) { case "login": $u = $_POST['username']; $p = $_POST['password']; $query = "SELECT * FROM login WHERE username='$u' AND password='$p'"; $result = mysql_query($query); $row = mysql_fetch_array($result); if (isset($id))($row){ session_start(); $_SESSION['user_id'] = $row[0]; $_SESSION['residentname'] = $row[1]; $_SESSION['unit_num'] = $row[2]; setcookie("TestCookie", time()+3600); /* expire in 1 hour */ $resite = "submitjob.php?do=viewone&id=$id"; header("Location:$resite"); exit(); } else if ($row){ session_start(); $_SESSION['user_id'] = $row[0]; $_SESSION['residentname'] = $row[1]; $_SESSION['unit_num'] = $row[2]; setcookie("TestCookie", time()+3600); /* expire in 1 hour */ $resite = "submitjob.php"; header("Location:$resite"); exit(); } else { echo "Sorry the app didn't find a match."; } break; } ?>
-
I have a very tricky php problem here. At least for me. On a page that is editing a job entry. I have a database generated group of checkboxes some of which have been checked when the job was entered. So the script has to do two things generate the complete list of checkboxes and check the ones that have already been selected in the job request. The below script is what I've done trying to figure it out. Description of what I'm trying to do: I'm generating the the boxes with a call to my database for the list of checkboxes. Then I'm building an array with all the possible items that could be checked to compare against. Ok here is where I think my first problem is. I need to make a second query to another table "worklog" in the same database. This is where the list of checked items are stored. What is the best way to do this? A second query seems wrong "or at least not efficient" <div class="text">Job Type (<a class="editlist" href="javascript:editlist('listedit.php?edit=typelist',700,400);">edit list</a>):</div> <div class="field"> <?PHP $connection=mysql_connect ("localhost", "user", "password") or die ("I cannot connect to the database."); $db=mysql_select_db ("database", $connection) or die (mysql_error()); $query = "SELECT type FROM typelist ORDER BY type ASC"; $sql_result = mysql_query($query, $connection) or die (mysql_error()); $i=1; echo '<table valign="top"><tr><td>'; while ($row = mysql_fetch_array($sql_result)) { $type = $row["type"]; if ($i > 1 && $i % 26 == 0) echo '</td><td>'; else if ($i) echo ''; ++$i; $aTypes = array ("Spec Ad Campaign", "100 x 100 Logo", "100 x 35 (Featured Developer/Broker)", "100 x 40 (Featured Lender)", "120 x 180 (Home Page Auto)", "120 x 45 (Half Tile - Jobs)", "120x60 (Section Sponsor)", "125 x 40 (Profile Page logo)", "135 x 31 (Autos)", "135 x 60 (Autos)", "135 x 60 (Logotile - Jobs)", "150 x 40 (Auto Logo)", "160 x 240 (monster tile)", "160 x 400 (Skyscraper)", "160 x 600 (Tower)", "170x30 (Section Sponsor)", "240 x 180 JPG/GIF Auto Video", "240 x 180 size FLV video ", "300 x 250 (Story Ad)", "300 x 600 (Halfpage)", "468 x 60 (Banner Jpg/Gif)", "728 x 90 (Leaderboard)", "95 x 75 (Sweeps Logo) ", "Agent Profile Page", "Contest", "Creative Change", "Employer Profile", "Holiday Shop", "Home of the Week", "HP Half Banner (234 x 60 Static)", "Mobile Ads (320x53 *300x50*216x36*168x28)", "Newsletter", "Peelback", "Pencil Ad", "Print", "Real Deals", "Resize ad campaign", "Roll-Over Skyscraper", "Site Sponsor logo (170x30)", "Splash Page Production", "Travel Deals Update", "Video Ad", "Web Development", "Web Maintenance", "Web Quote (or Design)"); $dbTypes = explode(',',$row['type']); foreach ($aTypes as $type){ if(in_array($aType,$dbTypes)){ echo "<input style='font-size:10px;' name='type[]' type='checkbox' value='$type' CHECKED><span style='color:#000;'>$type</span></input><br/>"; }else { echo "<input style='font-size:10px;' name='type[]' type='checkbox' value='$type'><span style='color:#000;'>$type</span></input><br/>"; } } } echo '</td></tr></table>'; ?> </div>
-
I have a set of 26 checkboxes that are stored in an array. The below code dumps the comma separated array values into a single record column. // dumping the type of job checkboxes $type = $_POST['type']; var_dump($type); // Setting up a blank variable to be used in the coming loop. $allStyles = ""; // For every checkbox value sent to the form. foreach ($type as $style) { // Append the string with the current array element, and then add a comma and a space at the end. $allTypes .= $style . ", "; } // Delete the last two characters from the string. $allTypes = substr($allTypes, 0, -2); // end dumping the type of job checkboxes This works great! But my needs go past this. The record can be edited. So I need to get the values back out of the database and populate the correct check boxes for editing. The checkboxes are generated by this code. <div class="text">Job Type (<a class="editlist" href="javascript:editlist('listedit.php?edit=typelist',700,400);">edit list</a>):</div> <div class="field"> <?PHP $connection=mysql_connect ("localhost", "name", "pass") or die ("I cannot connect to the database."); $db=mysql_select_db ("database", $connection) or die (mysql_error()); $query = "SELECT type FROM typelist ORDER BY type ASC"; $sql_result = mysql_query($query, $connection) or die (mysql_error()); $i=1; echo '<table valign="top"><tr><td>'; while ($row = mysql_fetch_array($sql_result)) { $type = $row["type"]; if ($i > 1 && $i % 26 == 0) echo '</td><td>'; else if ($i) echo ''; ++$i; echo "<input style='font-size:10px;' name='type[]' type='checkbox' value='$type'><span style='color:#000;'>$type</span></input><br/>"; } echo '</td></tr></table>'; ?> </div> I am having a problem getting these checkboxes repopulated. I think that a way to do this is to: Get the values out of the database in a array Compare the values the list of all possible values. Check the boxes of the values confirmed to be there. I didn't build the application so I need help to work through this problem.
-
Updating a record while displaying all changes to it.
sjslovechild posted a topic in PHP Coding Help
I don't know if I'm wording this right. I have a textarea that is entered into a database. When this is edited, I want to store and display a list of all the changes that have been made to it. Like a conversation in a forum. The reason for this is that I want past changes to be locked from editing. Would a new database table storing only this text fields data need to be created? Maybe have column for the work request number and a second column for the text entered? But then wouldn't I need an third identifier column so the changes can be displayed in the right order? Looking for sage like wisdom Thanks Brad_Strickland -
The search form that passes information to this code is a drop down menu of Clients. The query has to be by clients. Mind you dates are passed back from the database. Is there a way to keep the query by client and still track the month. So that when it changes I can insert a <HR> and the total hours work on that client for that month?
-
Below is my code for displaying query results of customers it is ordered by the month. I need to get a total for each month below the month and insert a hr below that. So when the month changes I need to: 1. Total the hours for that month 2. Below the total insert a hr 3. below the hr continue to the next month. This seems simple but for some reason I'm getting stumped. <?php $searchbyclient = $_POST['client']; $host = 'BLah' ; $user = 'BLah' ; $pass = 'BLah' ; $db = 'BLah' ; $table = 'worklog' ; $show_all = "SELECT * FROM $table WHERE clientname = '$searchbyclient'"; mysql_connect ($host,$user,$pass) or die ( mysql_error ()); mysql_select_db ($db)or die ( mysql_error ()); $result = mysql_query ($show_all) or die ( mysql_error ()); echo "<h2>Customer Time Reporting Results</h2>"; echo "<div><a href='reportform.php'>Run another report</a> | <a href='submitjob.php'>Back to Submit Job</a></div>"; echo "<table border='0' cellpadding='2' cellspacing='18' align='center'>"; echo "<tr><th>Date</th><th>Clientname</th><th>Job</th><th>Designer</th><th>Ad Rep</th><th>Time Spent</th></tr>"; $currentdate =''; while($row = mysql_fetch_array($result)){ // Print out the contents of each row into a table if($row['datetime'] != $currentdate) { echo "<tr><td bgcolor='#FFFFFF'>"; $output = date('F, d, Y', strtotime($row['datetime'])); echo $output; echo "</td><td>"; } echo $row['clientname']; echo "</td><td>"; echo $row['job']; echo "</td><td>"; echo $row['designer']; echo "</td><td>"; echo $row['adrep']; echo "</td><td>"; echo $row['time']; echo "</td></tr>"; } echo "<tr><td bgcolor='#FFFFFF' colspan='6' align='right'>"; //this is the query that adds up the time entered $query1 = "SELECT SUM(time) FROM worklog WHERE clientname = '$searchbyclient'"; $result1 = mysql_query($query1) or die(mysql_error()); // Print out result while($row = mysql_fetch_array($result1)){ echo "Total = ". $row['SUM(time)']; echo "<br />"; }; echo "</td></tr>"; echo "</table>"; ?>
-
I'm using PHP Version 4.4.3 on a Windows NT WEBHOST 5.2 build 3790 I'm having a odd problem with uploading image gallery pics to the server. I can upload the files with my php script and see the files via a FTP program. But the upload files have no file permissions. The server admin tells me that it is the Anonymous User permissions are not begin assigned. The code I'm using is below. Is there anything in the code that I'm missing? thanks. <?php // define a constant for the maximum upload size define ('MAX_FILE_SIZE', 51200); if (array_key_exists('upload', $_POST)) { // define constant for upload folder define('UPLOAD_DIR', '/path/to/picture/directory/pics/'); // replace any spaces in original filename with underscores // at the same time, assign to a simpler variable $file = str_replace(' ', '_', $_FILES['image']['name']); // convert the maximum size to KB $max = number_format(MAX_FILE_SIZE/1024, 1).'KB'; // create an array of permitted MIME types $permitted = array('image/gif', 'image/jpeg', 'image/pjpeg', 'image/png'); // begin by assuming the file is unacceptable $sizeOK = false; $typeOK = false; // check that file is within the permitted size if ($_FILES['image']['size'] > 0 && $_FILES['image']['size'] <= MAX_FILE_SIZE) { $sizeOK = true; } // check that file is of an permitted MIME type foreach ($permitted as $type) { if ($type == $_FILES['image']['type']) { $typeOK = true; break; } } if ($sizeOK && $typeOK) { switch($_FILES['image']['error']) { case 0: // move the file to the upload folder and rename it $success = move_uploaded_file($_FILES['image']['tmp_name'], UPLOAD_DIR.$file); if ($success) { $result = "$file uploaded successfully"; } else { $result = "Error uploading $file. Please try again."; } break; case 3: $result = "Error uploading $file. Please try again."; default: $result = "System error uploading $file. Contact webmaster."; } } elseif ($_FILES['image']['error'] == 4) { $result = 'No file selected'; } else { $result = "$file cannot be uploaded. Maximum size: $max. Acceptable file types: gif, jpg, png."; } } ?>