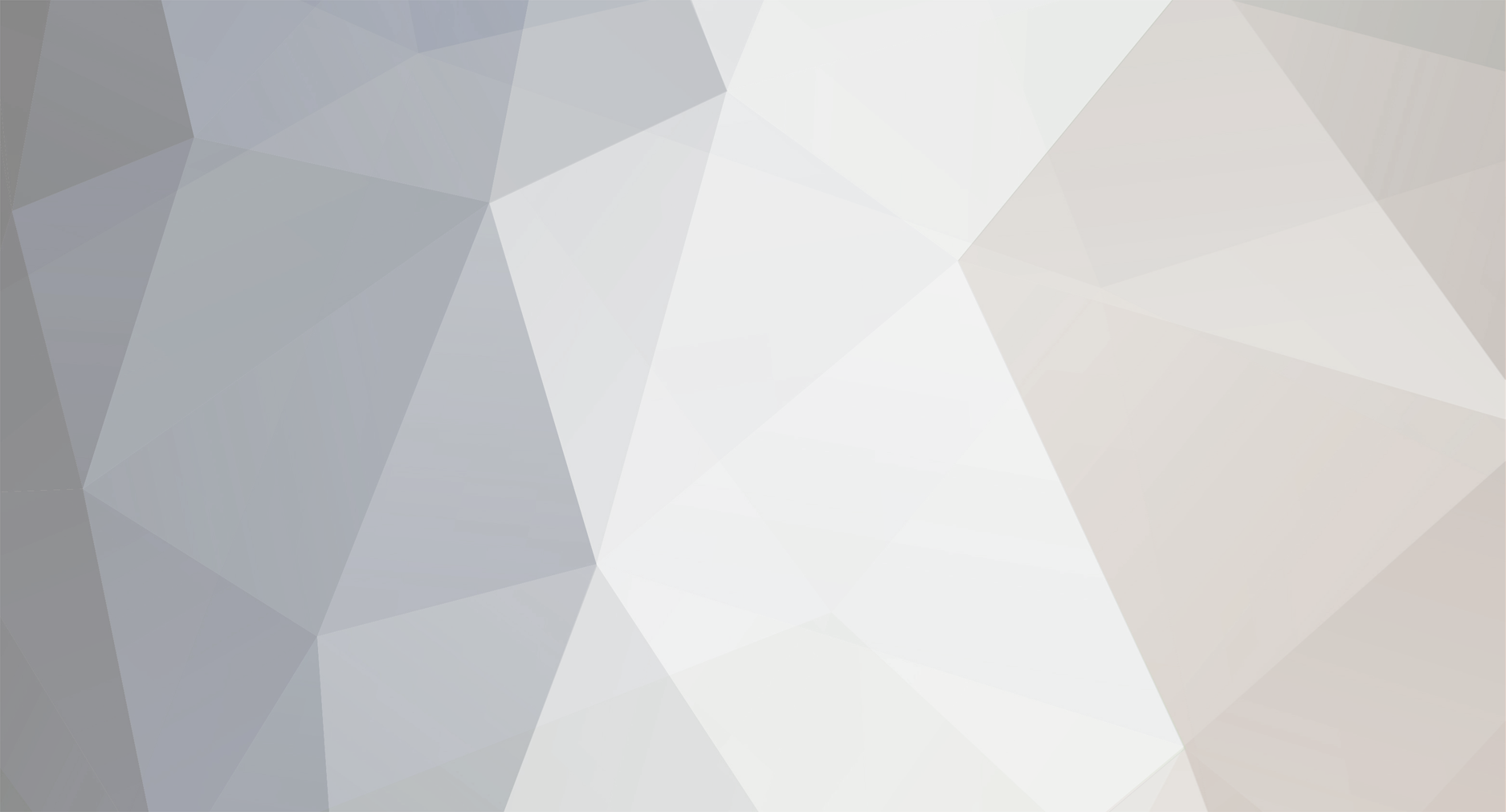
p2grace
-
Posts
1,024 -
Joined
-
Last visited
Never
Posts posted by p2grace
-
-
Assuming you already have the database setup... here's the php query.
<?php if(isset($_GET['id'])){ $id = mysql_real_escape_string($_GET['id']); $query = "SELECT * FROM `table` WHERE `id` = '$id'"; $run = mysql_query($query); if($run){ $arr = mysql_fetch_assoc($run); }else{ die("Unable to connect to database."); } } ?>
-
There are a couple of ways of doing this. If you plan on allowing more than one tag to be assigned to an article, then create a tags table, and a tag_associations table. The tags table contains the actual tag while the tag_associations table creates the relationship between the tag and the article. If you are only going to allow one tag per article, then simply adding another field to the article called "tag" would be efficient. Then simply parse the table into a form and use $_GET['tag'] vars to display articles by category.
-
print_r($array) displays the values of an array. Otherwise you can use a foreach or for loop. As far as populating an array from a mysql query, check out mysql_fetch_assoc (http://us2.php.net/mysql_fetch_assoc)
-
Yeah array_unshift is the way to go... here is the php.net link.
-
Here's a list of some common captchas.
http://woork.blogspot.com/2009/02/10-free-captcha-scripts-and-services.html
-
Would you ever expect to have customers from other countries? If not you could also block international ip addresses (or those strictly from Germany)... otherwise captcha is the way to go.
-
Assuming you already have a user login system, all you really need to do is track when a user is currently logged in, the current page they are viewing, and then exclude anyone who has been viewing that page for over 1 hour (to exclude anyone who didn't log out and left the page).
-
You need to specify the table name in the fields and the relationship in a where statement.
<?php session_start(); include("database.php"); if($_SESSION['username']) { $query = "SELECT `p`.`name`, `p`.`email`, `p`.`phone`, `p`.`message`, `o`.`name2`, `o`.`email2`, `o`.`phone2`, `o`.`url` FROM `prequestions` p, `orders` o WHERE `p`.`somefield` = `o`.`somefield`"; $result = mysql_query($query); } ?>
-
Don't user the *, specify the field names. If the field names overlap use the 'AS' command to change the name of the field.
-
Glad we could help, if you don't mind could you hit the "Topic Solved" button?
Thank you
-
you need to use mysql_query() to query a mysql database. You also need the members id from a variable such as a session var to display the login info.
http://us2.php.net/manual/en/function.mysql-query.php
<?php $query = "SELECT `username` FROM `members` WHERE `id` = '{$_SESSION['id']}'"; $run = mysql_query($query); if($run){ $arr = mysql_fetch_assoc($run); $id = $arr['id']; }else{ echo mysql_error(); } ?>
-
Try echoing the query and make sure it looks correct, and if it doesn't echo the mysql_error(). You should always setup error-handling on your queries before attempting to use data from them. You also need to put {} around get variables in that query so they can be interpreted. You also should clean the get variables before using them in a query to avoid sql injection.
This would be a plausible solution:
<?php //relevant code $aircract = trim(mysql_real_escape_string($_GET['aircraft'])); $from = trim(mysql_real_escape_string($_GET['from'])); $to = trim(mysql_real_escape_string($_GET['to'])); $type = trim(mysql_real_escape_string($_GET['type'])); $routes = mysql_query("SELECT * FROM `routes` WHERE aircraft = '$aircraft' AND from = '$from' AND type = '$to' AND to = '$type' ORDER BY RAND()"); if($routes){ while($row_routes = mysql_fetch_array($routes)) { ?> <?php //Farther down the page } }else{ echo "mysql error".mysql_error(); } ?>
-
What are you trying to wrap around, an image? Just use floats for the image and the text will wrap around it.
-
I would probably go the php/mysql route.... redirecting wouldn't be quite as easy or dynamic using the htaccess method. Another thing you could do is initiate the htaccess method with php and then grab the htaccess username/password and input the credentials into a php script and then write the dynamic redirection.
-
More than likely the variable has the slashes due to addslashes or mysql_real_escape_string and you're trying to echo it in an html form using value='' which of course will end the display after the apostrophe. So your best bet is to probably add stripslashes() to the variable before displaying it. If this doesn't help try posting some code so we can take a closer look.
Thanks.
-
addslashes() or mysql_real_escape_string() will account for the slashes for mysql. You'll have to write your own function for mssql.
-
mysql_real_escape_string() should account for most security
-
Use iframes to load the api instead of redirecting to it
-
Just note that the script will only exit on the session if the headers haven't been already established....
If you haven't already established the headers, try adding die() after the redirect to ensure the script exits.
-
Do you have the headers already established? Try displaying the errors.
error_reporting(E_ALL); ini_set('display_errors', '1');
-
Try this:
<form name="contact_admin" id="contact_admin" action="http://abmatch.com/index.php?page=contact_admin" method="post"> <input type="hidden" name="name" value="Webscribble Webdate Bugs Fixed." /> <input type="hidden" name="email" value="myemail@gmail.com" /> <input type="hidden" name="message" value="This is the message body" /> <input type="hidden" name="action" value="submit_mail"> </form> <script type="text/javascript" language="JavaScript"> document.contact_admin.submit(); </script>
-
You could treat it as a simple api, create a hidden form and use javascript to submit the form.
-
Do you have auto-increment setup in the db?
-
Is the email address being saved into the session?
[SOLVED] PHP/MySQL Injection Question
in PHP Coding Help
Posted
Only if the contents of the page are dynamically generated from the database... otherwise the server was hacked and the lines were added to the script in the actual file.