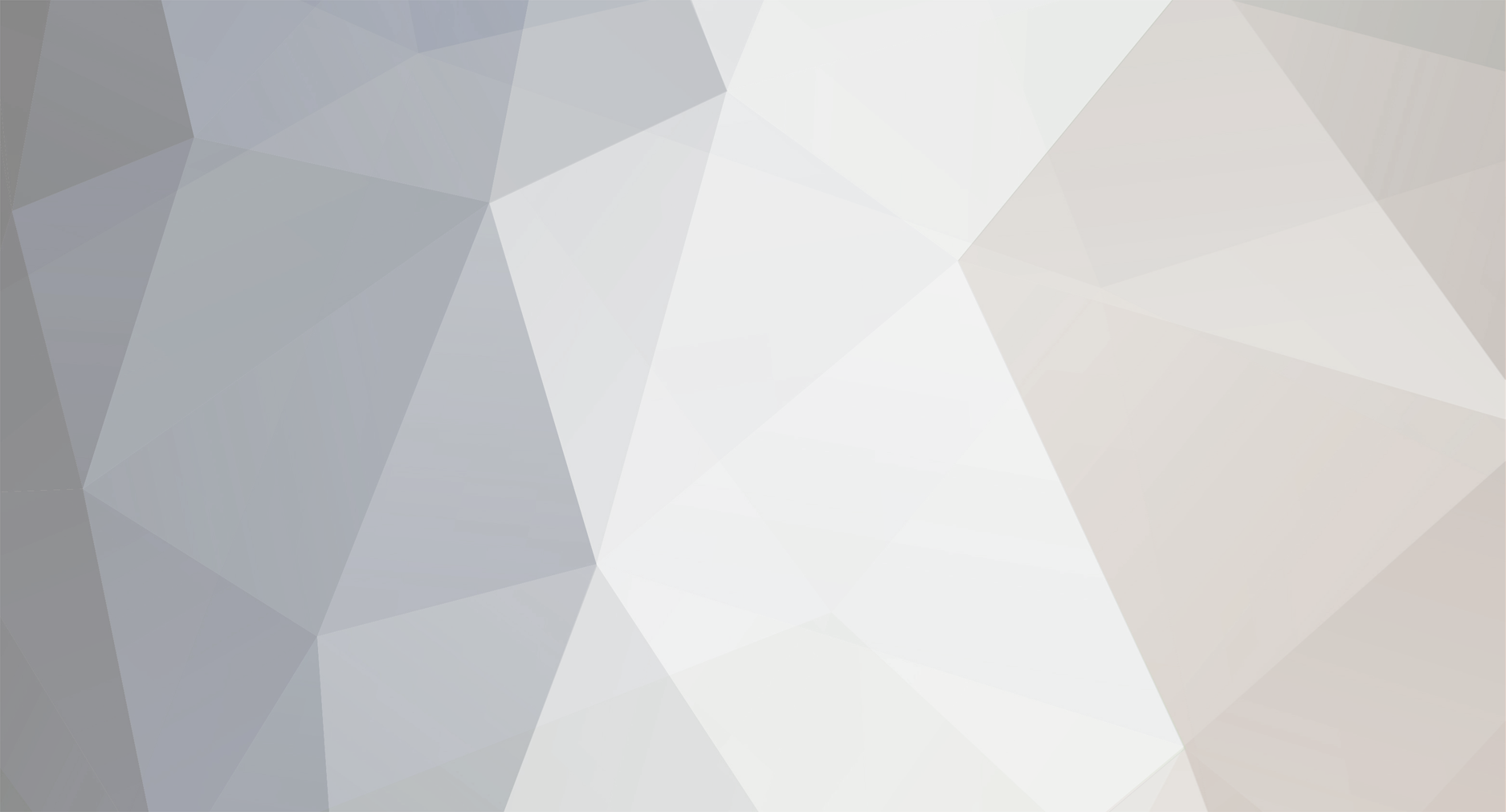
p2grace
Members-
Posts
1,024 -
Joined
-
Last visited
Never
Everything posted by p2grace
-
For best practice you'd usually put that information into a file and include it. The code itself would need to be above the insert query. Here's how to connect: define("DB_USER","username"); define("DB_PASS","password"); define("DB_DATABASE","database"); mysql_connect('localhost',DB_USER,DB_PASS); @mysql_select_db(DB_DATABASE) or die("Unable to select database!"); mysql_query("SET NAMES 'utf8'");
-
The whole insert statement would look like this: <? if(isset($_POST['name'])){ $name = $_POST['name']; $url = $_POST['url']; $description = $_POST['description']; $query = "INSERT INTO `db_table` (`id`,`name`,`url`,`description`) VALUES ('','$name','$url','$description')"; $run = mysql_query($query); } ?>
-
Yes, make a var for both. $url = $_POST['url']; $description = $_POST['description'];
-
This is an insert statement ,not a select statement. The statement should look like this: <? if(isset($_POST['name'])){ $name = $_POST['name']; $query = "INSERT INTO `db_table` (`id`,`name`,`url`,`description`) VALUES ('','$name','$url','$description')"; $run = mysql_query($query); } ?>
-
Change your select statement to the one I gave you earlier. Typically for search engines you don't want to use "WHERE `db_value` = '$submit_value'" instead use "WHERE `db_value` LIKE '%$submit_value%'"
-
Just glancing at it, it looks alright.
-
In phpmyadmin, your table should look as follows: table_name id (primary key, auto-increment, int) name (varchar) url (varchar) description (varchar with long length, or text)
-
Got it, When you create your database table make sure you create an id as a primary key that auto increments. When a form is submitted, the data creates a new row in the database table, where the id is unique to that row. When performing the search you'd use something like: $query = "SELECT `id`,`name`,`url`,`description` FROM `db_table` WHERE `name` LIKE '%$search_str%' OR `url` LIKE '%$search_str%' OR `description` LIKE '%$search_str%'"; $run = mysql_query($query); while($arr = mysql_fetch_assoc($run)){ extract($arr); echo "<h2><a href=\"$url\">$name</a></h2>". "<p>$description</p>"; } This is obviously a very basic example, but I believe it accomplishes what you're requesting.
-
Do you want a single input searched against the url, title, or description fields in a database table? Or are you looking for results based off a given url, title, and description? Basically, depending on what exactly you're trying to accomplish, you can either setup the query to parse those three fields for the given input, or concatenate the three fields into one and then perform a search off the concatenated string. Can you give me more details on what exactly it is you are trying to do?
-
That's probably the best way to do it.
-
Your welcome, and I think it's alright, it's a standard method of sending email.
-
I agree, take a look at the tutorial. I was just trying to give you a quick answer.
-
Remove the html from the top of the file, the headers are set there. Remove: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <title>Untitled Document</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> </head> <body> keep the php code Remove </body> </html> The only thing in the processing page should be the php code.
-
Also note, the redirect will only work if the headers haven't already been established. The processing needs to take place before the html headers are set.
-
First create a database table, then establish a database connection. After those two steps, the code above will work.
-
The redirect should be: header("Location: http://www.stmagnusbayhotel.co.uk/thanks.html"); The email from address will be what you specified in the headers, and is sent by the server. It will only send from your business email address if that's what you specify in the From Address.
-
Simple example: <? if(isset($_POST['name'])){ $name = $_POST['name']; $query = "INSERT INTO `db_table` (`id`,`name`) VALUES ('','$name')"; $run = mysql_query($query); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="content-type" content="text/html; charset=utf-8" /> <title>Sample Form</title> </head> <body> <form name="sampleForm" id="sampleForm" action="index.php" method="post" enctype="multipart/form-data"> Name: <input type="text" size="20" id="name" name="name" /> <br /> <input type="submit" /> </form> </body> </html>
-
Try this: $headers = "From: webmaster@stmagnusbayhotel.co.uk\r\n" . "X-Mailer: PHP" . phpversion() . "\r\n" . "MIME-Version: 1.0\r\n" . "Content-Type: text/html; charset=utf-8\r\n" . "Content-Transfer-Encoding: 8bit\r\n\r\n"; // this emails you the results of the form mail( "Webmaster@stmagnusbayhotel.co.uk", "New Contact Message", print_r( $_POST, true),"$headers"); // this will redriect the user somewhere away from this page header("location: http://www.stmagnusbayhotel.co.uk/thanks.html"); ?>
-
You could also try adding "`" around the mysql fields. function getRating($id, $tablename){ $sel = mysql_query("SELECT `rating_num` FROM `$tablename` WHERE `rating_id` = '$id'"); }
-
What exactly are you trying to accomplish by finding where it's stored?
-
If it isn't specified it's lifetime is set to 24 inactive minutes.
-
Hi, I believe the session max lifetimes are set in the php.ini file. I'm not sure if this is what you're asking, but in order to get IE to regenerate its session id, I usually use session_regenerate_id(); right after session_start();