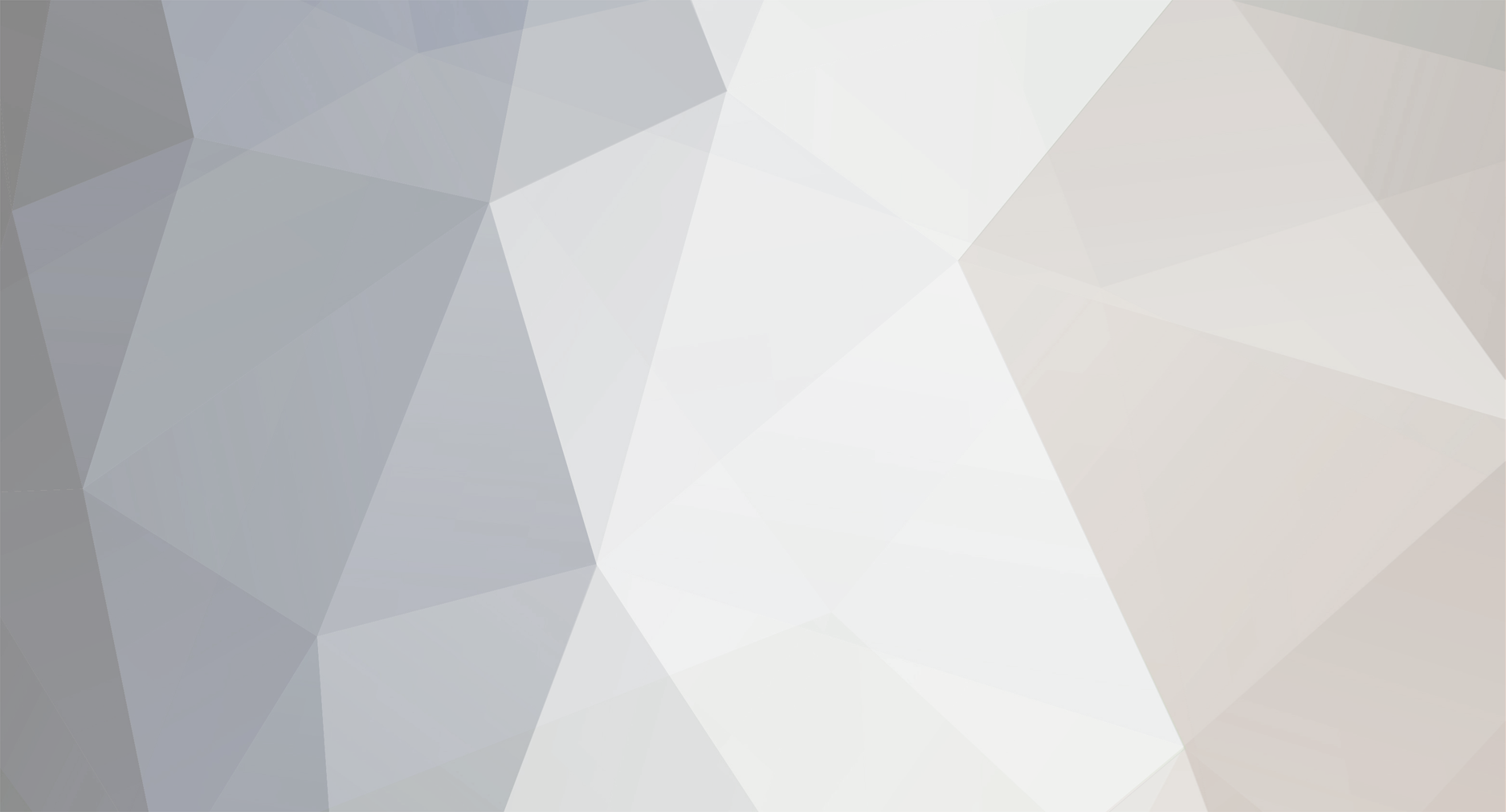
STaRDoGG
Members-
Posts
26 -
Joined
-
Last visited
Everything posted by STaRDoGG
-
Hey all, What's the most efficient way to wait until a page on your own website is done being rendered, and then parse it for something specific? The reason I'm having to scrape it rather than just generate it myself is because the part being scraped if being generated in an iframe on my site via another site, and the data inside of it is dynamic. Thanks
-
I'm writing a simple logging routine that checks if a name is already in the array, and decide how to proceed from there. So my array needs to retain it's items (names) each time the function is called. Right now, everything works fine with the exception of the array retaining it's items. The script (page) gets reloaded once every couple of minutes. Here's the entire function: function Logging($aimid, $Online) { // *Logs any changes for a user* // This array will hold only the AimID's of anyone who is currently Online static $UserLogArray = array(); //echo '<pre>' . print_r($UserLogArray, true) . $aimid . ' ' . $Online . '</pre>'; switch ($Online) { Case '1': // User is Online ... // search for the current AimID (User Nickname) in the static $UserLogArray if (($i = array_search($aimid, $UserLogArray)) !== FALSE) { // $i = contains the array key of the name found in the array. // ... AND the user was found in the array, meaning we already added them to it, so do nothing, just exit. //exit; return; } // ... user wasn't found in the array, so add them and log it ... // Add aimid to array here, and the string to add to the log file $UserLogArray[] = $aimid; $write = "Online: " . $aimid . " " . Fetch_TimeStamp() . "\n"; break; Case '0': // User is Offline ... // search for the current AimID (User Nickname) in the static $UserLogArray if (($i = array_search($aimid, $)) !== FALSE) { // $i = contains the array key of the name found in the array. // ... AND the user was found in the array, so remove them from the array, and write that they went offline to the log. array_splice($UserLogArray, $i, 1); $write = "Offline: " . $aimid . " " . Fetch_TimeStamp() . "\n"; break; } // ... user wasn't found in the array, so just exit. return; } // Finally, open the Log and append to the file $fp = fopen('aim-log.txt', 'a'); $write = fputs($fp, $write); fclose($fp); } I've tried commenting out static $UserLogArray = array(); in the function, and placing this session code at the top of the file instead: session_start(); if (!is_array($_SESSION['UserLogArray'])) $_SESSION['UserLogArray'] = array(); But when I do that, I get errors saying there's a problem with the second parameter in array_search. I've also tried changing the array var declaration at the beginning of the function to this instead: static $UserLogArray; if (is_null($UserLogArray)){ $UserLogArray = array(); } But I get the same error again about the array_search parameter. Anyone able to shed some light on this? Thanks.
-
Oops, that last brace is just from this at the top of the code: if (variable_get('enable_stats_guests', 1)) { I forgot to paste that last night.
-
Hey all, I know this is on the easier side, but I'm kinda drunk and my brain is fried from too much coding lately, lol. Here is the code I'm running into a problem with: // Display a list of currently online guests. if ($total_guests) { $output.="<div class=\"item-list\"><h7>Anonymous</h7><ul><fine>"; $guestitems = array(); // Create array of guests we want to filter out $GSONExclude = array_map('trim', explode("\n", variable_get('stats_filter', 'googlebot'))); while ($guests-- && $account = db_fetch_object($guests_hostname)) { $guestitems[] = $account->hostname; $resolved = gethostbyaddr($account->hostname); } foreach ($GSONExclude as $value) { $pos = strpos($resolved, $value); if ($pos !== true) { $output.="<li><a title=\"Go to address\" href=\"http://www.maxmind.com/app/locate_ip?ips=$account->hostname\" target=\"_blank\">$account->hostname</a><br>" . $resolved; break; } } $output.="</fine></ul></div>"; } } To sum up, what I'm trying to do is, this code generates a list of anonymous users, (in drupal) but I want to filter OUT the search engine spiders. $resolved contains the resolved DNS of an IP address. so if it contains a string like "crawl" or "spider" (which is being taken from the $GSONExclude array) I want to filter it out, and not add it to the list. Anything else, I want added to the list. Anyone with a fresh brain able to point out whats wrong? I'd appreciate it. Thanks!
-
I have the page set to meta refresh every 2 minutes or so already. But like I said, the time keeps changing rather than staying the same, like I want it to/it should.
-
Hmmm, ok. I am fetching an Aol instant messenger XML file, with a list of screen names in the XML, with each one's specific info in the nodes. When any of the screen names changes their Status Message, AIM sets a unix timestamp on it, so I would assume, that even though the timestamp will grow in number (due to the passage of time since it's been set), if I do a calculation using strtotime, to figure out how far in the past it calculates to, it should always show the exact same time/date it was set, until the user changes their Status Message again. My problem so far is: I run it through 2 separate functions, the first, converts the Unix Timestamp into hours, minutes and seconds, returning a format like this: 12:35:45 After I get those numbers back, I send it through the second function with those numbers ($hours = 12, $minutes = 35, $seconds = 45) Then I;m using the strtotime to subtract that amount of time from the current timestamp, and finally, using Date to show it in a more user friendly format, like: Status Message Set on: Thu Dec 12, 2009, 12:35 (45) pm It seems to set to correct time after running through both functions the very first run-through, however, any refresh of the page will add however many seconds, minutes, etc. that have passed since I ran it through the original time, rather than staticly show the same exact timestamp no matter how many refreshes. So to sum up, basically, if I'm on AIM and change my status message, no matte rhow much time passes, my timestamp will always remain the same until I change it again. My code isn't keeping it set to the same time, it keeps increasing as I hit refresh for some reason.
-
Do you want me to send you the actual php file? Other than having the actual php file, the code in the original post is about all there is to it, as far as the problem functions go. I can email you the file itself if you want to pm me an email address.
-
I'm using Date at the bottom of the second function, but I need to calc how long ago in the past it was first. I use date to format the results. The only thing I'm thinking is possibly the conversion in the first function is causing the problem?
-
Hey all, I'm playing around with some code, and basically the idea is: Person changes their profile I fetch some XML that has a unix timestamp for the time the person changed their profile, so it'll keep increasing everytime I fetch the XML. It looks like this: <profileTime>12086</profileTime> I then run it through a function to convert the unix time to hours:mins:secs Finally I run it through another function to calc how long in the past that was, so it can be displayed in a user friendly format. The issue is, every time I run it through the second function, the final displayed time keeps increasing, rather than staying the same, which it should stay the same, because the person only changed their profile once, at that original time. Here is the first function, where I convert the unix time to h:m:s: function convert($sec, $padHours = false) { $hms = null; $hours = intval(intval($sec) / 3600); $hms .= ($padHours) ? str_pad($hours, 2, "0", STR_PAD_LEFT). ':' : $hours. ':'; $minutes = intval(($sec / 60) % 60); $hms .= str_pad($minutes, 2, "0", STR_PAD_LEFT). ':'; $seconds = intval($sec % 60); $hms .= str_pad($seconds, 2, "0", STR_PAD_LEFT); return $hms; } And here's the code in teh second function to format it: function olddate($hours, $minutes, $seconds) { /* List of working timezones here: * * http://www.php.net/manual/en/timezones.php */ date_default_timezone_set('America/Chicago'); // Calculate the exact day and time the status message was set $pf_time = strtotime("-".$hours." hours ".$minutes." minutes ".$seconds." seconds"); echo date("D F j, Y, g:i (s) a", $pf_time); //return date("D F j, Y, g:i (s) a", $pf_time); } Anyone able to offer any advice? Thanks
-
This isn't a strong point of mine, any tips/guidance?
-
Is there a way to get an sql dump to be able to import later, that only dumps from a specific table AND only when it matches a specific column header? For example, I have a table named "Messages", which has many columns/rows and many users, so I want to only export the rows that are from a specific user. The user is identifiable by the number "3" in the column named "uid". So I'd like to be able to export from the Messages table and only for user 3. Possible? TIA
-
AHA! the trimming did the trick. Thanks
-
Hi all, Running into a strange issue with an array. Anyone able to spot the problem? To sum up, if i use the manual array creation, the foreach loop works, but when I use the explode method to create the array, it doesn't work. It will return "no match", even if it is a match. I have added "##" to the comments in the code below on where the problems are arising. Thanks for any help in advance. function Member_Exclusions ($GSName) { // To ensure we don't have to worry about case sensitivity, lets do all comparisons as lowercase $GSName = strtolower($GSName); // ## Uncommenting the 2 lines below, makes the foreach loop work correctly ... //$GSExclude[0] = "Lisa"; //$GSExclude[1] = "Michelle"; // ## However, creating the array with the following line, does not work ... // (The array is created from a text box, separating names by a new line) // Using the echo's as a test, the array IS being created correctly ... $GSExclude = explode("\n", variable_get('Member_filter_exclusions_list', '')); //echo $GSExclude[0]; //test //echo $GSExclude[1]; // Loop through the array of members, looking for the current one foreach ($GSExclude as $value) { $value = strtolower($value); //echo $value; //echo $value[0]; // ## Here is where the issue is: $value is echoing both names form the array // on a single line, rather than just one name per index in the array. if ($value == $GSName) { // Found our friend $GSResult = "match"; break; }else{ // Nope $GSResult = "no match"; } } return $GSResult; }
-
I'm used to coding in .NET where I can step line by line, and see whats in each variable, set breakpoints, etc. Is there a similar way to do this (on a remote server) in PHP? I have installed Komodo which can do it, however, I'm stuck working with a server with a PHP version just slightly too old ( 4.3.8 ) to get it to work with Komodo's debugger, and I have no choice in the matter. The host won't upgrade for fear of breaking too many old scripts. Does anyone know of another way to do this, other than just adding a ton of prints? it would save me a ton of headaches doing it all in my head. Especially when working with arrays. lol TIA
-
I know this isn't particularly helpful, but just thought I'd mention that from my experience with the Mail function, Mail seems to have a bit of a mind of it's own. Sometimes I just plain don't get the mail, most of the times I do. When I don't they seem to just disappear off into the Bermuda Triangle, never to be seen again.
-
Aahh gotcha. Funny thing was I originally had both of them together, then while testing later it seems to behave the same, so I took out what just appeared ot be 'extra formatting'. I put it back again. Thanks all
-
Thanks guys, Stickynote's solution did the trick
-
Hey all, PHP isn't a particular strength of mine syntax-wise; can anyone spot what's wrong with this line? if ($GSFilterEx == false || $GSFilterEx == true && !GS_Exclusions($GSName) == "match") $GSFilterEX is a checkbox that returns either 0 or 1 (false or true). GS_Exclusions is a function that either returns "match" or "no match" depending on of a particular persons name was found in an array in that function. I've already tested the function and it works ok. What I want it to do is: There is some code to run following that line, that I only want to execute IF the checkbox is either unchecked, OR it is check AND is not a match. So essentially, the code following that line should always execute unless there is a match with the user's name. For some reason though, the code following that line is not being executed, even though I have the box checked, and it's not a match. Anyone have any ideas? Thanks in advance
-
Hi all, Not too well versed in PHP, and I am trying to create an array that stores IP addresses, and later on I do a foreach loop on the array. I am storing them at the top of the script like this: $filterIP[0] = "11.11.11.11"; $filterIP[1] = "22.22.222.2"; etc. When I comment out all elements of the array except the first [0], the script runs as intended. However, when I uncomment [1] or more, I get this error: unexpected T_IS_EQUAL on the [1] line. For kicks, I tried making the arrays into: $filterIP[0] == "11.11.11.11"; $filterIP[1] == "22.22.222.2"; (Double equals signs) as a test, but then I got an error during the foreach loop. Anyone have any advice? Thanks
-
Hi all I'm not well versed in php, and was hoping someone would write a small routine fo rme? I have a flat file named Known.txt, and it contains IP's and names, in this format (one per line): 192.168.1.1,Bob Anderson 192.168.100.1,Fred Davis in my php file, I'd like for a routine to parse the txt file for the matching IP, and when found, return the name associated with it. So 192.168.100.1 would simply return "Fred Davis". I'd really appreciate it! Huge thanks in advance SDC
-
I stumped everyone?
-
Lo all, I am doing some ip[ logging using an image. And it requires a .htaccess file to work, which is below (and works fine): AuthUserFile /dev/null AuthGroupFile /dev/null RewriteEngine On ReWriteRule .*\.(jpg|gif)$ http://www.mysite.com/mypage/visitorlog.php [R,L] I have decided now that I want to give just that folder a password to block prying eyes. So I made this alteration (I'll leave out the .htpasswd file code since I know it's ok): AuthUserFile /home/www/mysite.com/mypage/.dhjpofh AuthType Basic AuthName "Hi There" Require valid-user AuthGroupFile /dev/null RewriteEngine On ReWriteRule .*\.(jpg|gif)$ http://www.mysite.com/mypage/visitorlog.php [R,L] Now the problem I ran into, is that the gif image that originally worked with the first htaccess code, that kicks off the entire logging process, is now a broken image using the new code, so the logging no longer happens. I am no wizard with the .htaccess stuff so i figure it's probably something simple, but I need the help of the pro's. All help much appreciated. =)