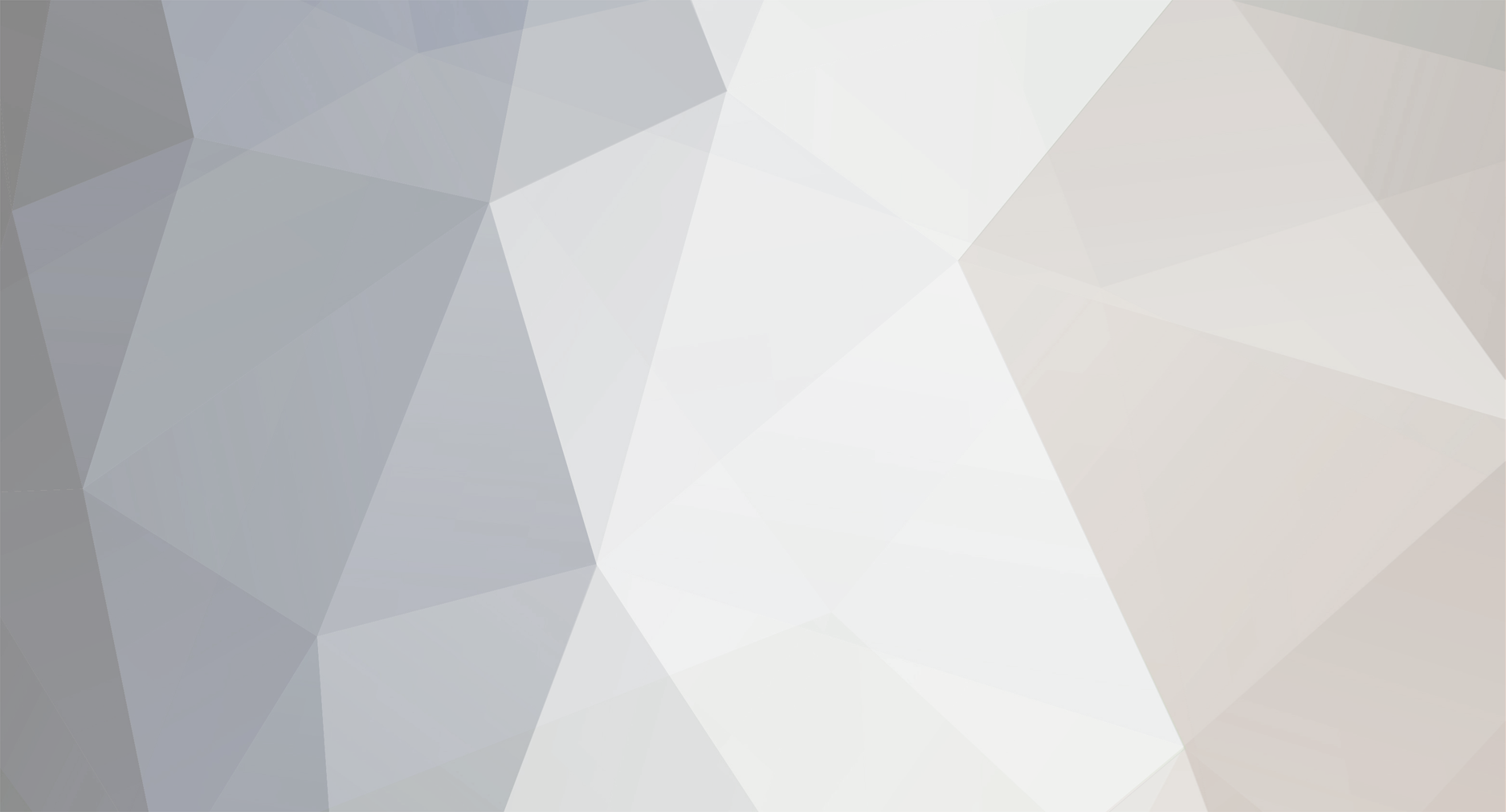
psychowolvesbane
Members-
Posts
204 -
Joined
-
Last visited
Never
Everything posted by psychowolvesbane
-
[SOLVED] Simple php query problem
psychowolvesbane replied to SocomNegotiator's topic in PHP Coding Help
Try mysql_fetch_array() instead of mysql_fetch_row. -
It looks like it's worked in JS when I tried that so I'm assuming it will work just as fine with php.
-
I would use - to separate them. Anyway how would I go about using that in an if statement when comparing the $NUSNum variable? Something like this? if (!preg_match('/[0-9]{6}[-]{0,1}[0-9]{4}[-]{0,1}[0-9]{4}[-]{0,1}[0-9]{4}[-]{0,1}/', $NUSNum)) I changed one of my Email validation masks to make this based on what you gave me.
-
Well I would like both client and server side in case people don't have JS enabled, so lets concentrate on server-side upon submission.
-
I have created a form that has a input field that users can enter their 18 digit (Format: 6,4,4,4) NUS number (For those who don't know what that is its the National Union of Students in the UK), and I was hoping to try and add an input mask instead of the numeric validation I have already that doesn't find out if it's okay or not. If that isn't possible then I might have a go at using 4 separate input fields instead. What do you think, is it possible to do it the first way?
-
use the Submit Button value, so the name of the submit button is checked with the value you gave it or by using the isset() function if it has a value when submitted: if(isset($_POST['SubmitB'])) { ...
-
Try just using the sha1 or md5 function to encrypt the password outside of the database, and store the hash as is in the db without using any built in password function in sql. Then when it comes to login the password the user enters also needs to be encrypted the same way and if that one and the hash in the database match then it will be correct. P.S. You must also check to see if the character length of the field in which you store the password is big enough to store the entire hash, otherwise part of it will be missing and messes up the comparisons.
-
Okay, I've only had a quick look at both scripts and I can think of a few things that might make things easier. Bare in mind I would not be able to properly test this myself without making a dummy database which I can't atm. First a minor change: You could change $ok to increment and count how many times an error has occurred, then if there are no errors ($ok == 0) then run the last bit of script. To increment use: $ok++; As far as I'm aware (as long as $uploaded_size and uploaded_type are working) the first script is okay. Now the second one... On the line "<p>Thank you, you have registered - you may now login[/url].</p>" why do you have a closing [/url] tag without an open one? Another thing is that md5 encryption isn't as safe as the sha1() function, but that's up to you $_POST['pass'] = sha1($_POST['pass']); Then when it comes to the login form you just sha1() the password they enter against the value in the record which is already encrypted and no one knows what it is, even the Admin. Lastly is this sql script: $insert = "INSERT INTO users (username, password) VALUES ('".$_POST['username']."', '".$_POST['pass']."')"; Should look like: $insert = "INSERT INTO users (username, password) VALUES ('$_POST['username']', '$_POST['pass']')"; There is no need to use the quoting on top of the usual ' ' for values, even for variables. The rest of the script and the form looks okay, but as I said I can't use it to be 100% positive.
-
Well for ordering in sql the word is ORDER BY itemname ASC, for Ascending or DESC for descendng. So you normally place this at the very end of the script for that table (in case you have multiple table queries). So for you I would recommend placing that in the find_items2 so that will change the order of the id's in both tables. For a search box you could have a separate version of find_items2 based on your search: $find_items2 = mysql_query("SELECT * FROM safety_box WHERE owner = '$userid' AND itemnumber='$searchresult' AND game = '$game' ORDER BY item_id LIMIT $start,50"); As for a A to Z separator it's far more complicated, but if you had a menu list of letters from A to Z along the top of the page and they link to the page but with a difference. The links should each look like this: http://prod_list.php?searchcat=$letter Then on the page you use the $letter variable to do another similar search as above: $find_items2 = mysql_query("SELECT * FROM safety_box WHERE owner = '$userid' AND item_name LIKE ' "$letter."%' AND game = '$game' ORDER BY item_id LIMIT $start,50"); So this will only display those records that begin with that letter, or at least I hope it does as this is a just a quickie script. Hope this helps or at least points you in the right direction.
-
I highly doubt that it's possible to have a standalone php application as it is the topping of the huge html cake.
-
[SOLVED] str_replace() exact replacement problem
psychowolvesbane replied to psychowolvesbane's topic in PHP Coding Help
Okay, I have it working at last, thanks! //Updating Clothing Records $sql = "SELECT AvailableColours FROM Clothing"; $rs = mysql_query($sql, $conn) or die(mysql_error()); $ToFind = "?"; if(mysql_affected_rows($conn) > 0) { while($row = mysql_fetch_array($rs)) { $AvailableColoursStr = $row['AvailableColours']; echo $AvailableColoursStr." is the old record<br>"; $AvailableColour1 = explode($ToFind, $AvailableColoursStr); foreach($AvailableColour1 AS $AColour) { if($AColour !=="") { if($AColour == $EditThisColourName) { $AColour = str_replace("$EditThisColourName", "$ColourName", "$AColour"); } $AvailableColour2.=$AColour."?"; } } echo $AvailableColour2." is the new record<br>"; $sqlUpdate1 = "UPDATE Clothing SET AvailableColours = '$AvailableColour2' WHERE AvailableColours ='$AvailableColoursStr'"; $rsUpdate1 = mysql_query($sqlUpdate1,$conn) or die('Problem with query: ' . $sqlUpdate1 . '<br />' . mysql_error()); $AvailableColours1 =""; $AvailableColour2 =""; } } -
you need to have: $recordset = mysql_query($query,$conn); ($conn is your connection details and assumes you have connected to the database) $data = mysql_fetch_array($recordset);
-
[SOLVED] str_replace() exact replacement problem
psychowolvesbane replied to psychowolvesbane's topic in PHP Coding Help
So, something like this?: //Updating Clothing Records $sql = "SELECT AvailableColours FROM Clothing"; $rs = mysql_query($sql, $conn) or die(mysql_error()); $ToFind = "?"; if(mysql_affected_rows($conn) > 0) { while($row = mysql_fetch_array($rs)) { $AvailableColoursStr = $row['AvailableColours']; echo $AvailableColoursStr." is the old record<br>"; $AvailableColours1 = explode($ToFind, $AvailableColoursStr) foreach($AvailableColours1 AS $Acolour) { if($Acolour == $EditThisColourName) { $AColour = str_replace("$EditThisColourName", "$ColourName", "$AColour"); } $AvailableColour2.=$AColour."?"; } echo $AvailableColours1." is the new record<br>"; //$sqlUpdate1 = "UPDATE Clothing SET AvailableColours = '$AvailableColours1' WHERE AvailableColours ='$AvailableColoursStr'"; //$rsUpdate1 = mysql_query($sqlUpdate1,$conn) or die('Problem with query: ' . $sqlUpdate1 . '<br />' . mysql_error()); $AvailableColours1 =""; } } -
Well to answer your first question, once you extract the information from the database once you only need to assign the data to local variables that can be accessed anywhere on the same page as long as the database is accessed on the first occurrence. So you have: $Location = $row['location']; $Field2 = $row['field2']; ...and so on. Then just reference $Location anytime you want that information again afterwards. Okay, moving on... For multiple tables the query should look something like this: $sql "SELECT table1.column1, table2.column2 FROM table1, table2 WHERE table1.column1 = 'value1' AND table2.column2 ='value2' "; you don't need to include the table1. and table2. reference in the SELECT and WHERE part but it helps you when it comes to reading bigger scripts.
-
Hi, I'm trying to use the str_replace function to replace the name of certain colours that I have listed within the Clothing Table in my database: RecordExample1: GoldGreen?NavyRed?RoyalWhite?WhiteRed? RecordExample2: Black?BlackGrey?DeepNavy? RecordExample3: BlackGrey?NavyWhite? Each individual colour is separated by the "?". Now I have another Table called ProductColours that lists all of these individual colours, and a form that changes the values. So on that form, whenever I change the value of one of the colours I most likely will need to update the collection of colours in the Clothing Table. That is where the string replacement comes in, however I cannot get it to be completely accurate and only effect the exact original match of the previous name. Take RecordExample 2, I am changing the name of the colour "Black" to "Blue", so that should change the string to: Blue?BlackGrey?DeepNavy? however it changes all occurrences of Black so it ends up as Blue?BlueGrey?DeepNavy? ! The same will happen if I were to change White to Pink, which would effect 3 incorrect colours. How do I stop it from doing that? code: <?php //Updating Clothing Records $sql = "SELECT AvailableColours FROM Clothing"; $rs = mysql_query($sql, $conn) or die(mysql_error()); $ToFind = "?"; if(mysql_affected_rows($conn) > 0) { while($row = mysql_fetch_array($rs)) { $AvailableColoursStr = $row['AvailableColours']; echo $AvailableColoursStr." is the old record<br>"; $AvailableColours1 = str_replace("$OldColourName", "$NewColourName", "$AvailableColoursStr"); echo $AvailableColours1." is the new record<br>"; $sqlUpdate1 = "UPDATE Clothing SET AvailableColours = '$AvailableColours1' WHERE AvailableColours ='$AvailableColoursStr'"; $rsUpdate1 = mysql_query($sqlUpdate1,$conn) or die('Problem with query: ' . $sqlUpdate1 . '<br />' . mysql_error()); $AvailableColours1 =""; } } ?>
-
Hi, I have a OnMouseOver event and OnClick on a table row that acts as a link to a further page depending on what was listed in that row. On the MouseOver event it is supposed to change the mouse pointer to a hand, but for some reason Firefox (2.0.0.13) doesn't like the code whereas in IE7 it works without any problems. Here's the code I use: <span class="TextHeader1">Clothing Categories</span> <table> <?php $conn = mysql_connect($Host,$Username,$Password) or die(mysql_error()); $db = mysql_select_db($Dbname, $conn); $sql = "SELECT DISTINCT ItemType FROM Clothing ORDER BY ItemType"; $rs = mysql_query($sql,$conn) or die('Problem with query: ' . $sql . '<br />' . mysql_error()); while($row = mysql_fetch_array($rs)) { $ItemType = $row['ItemType']; echo "<tr style=\"color:#E61732; line-height:8pt; font-size:9pt\" onMouseOver=\"this.style.backgroundColor='#FF7777'; this.style.color='#CC0000'; this.style.cursor='hand'\" onMouseOut=\"this.style.backgroundColor='white'; this.style.color='#E61732'\" onClick=\"location.href='search.php?Category=$ItemType'\"> <td>>$ItemType</td> </tr>"; } MySQL_close($conn); ?> </table> The error I get in the ErrorConsole is: Error in parsing value for property 'cursor'. Declaration dropped. Any one got ideas?
-
Random String Verification help
psychowolvesbane replied to psychowolvesbane's topic in PHP Coding Help
It looks like the issue is Javascript based so I shall have to call this solved for now. -
Are you sure you have session_start() function on every page?
-
Random String Verification help
psychowolvesbane replied to psychowolvesbane's topic in PHP Coding Help
Does anyone have any idea what I can do? -
Random String Verification help
psychowolvesbane replied to psychowolvesbane's topic in PHP Coding Help
Okay it doesn't display anything on the page beyond the Verification Required: table column and misses out the Submit and Reset buttons. -
In a form I've made I have tried to create a random string variable that is displayed on the form and for the users to copy into another text box for comparison and validation, however it doesn't seem to work as I had hoped, take a look at the code near the bottom of the script. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <?php session_Start(); include "admin/connect_details.php"; $random_str= $_SESSION['Rand_str']; $Forename = $_POST['Forename']; $Surname = $_POST['Surname']; $Email = $_POST['Email']; $ConfirmEmail = $_POST['ConfirmEmail']; $User = $_POST['Username']; $UserPass = $_POST['Password']; $ConfirmUserPass = $_POST['ConfirmPassword']; $Verify = $_POST['Verify']; $conn = mysql_connect($Host,$Username,$Password) or die(mysql_error()); $db = mysql_select_db($Dbname, $conn); $sql = "SELECT Username FROM UserAccount WHERE Username='$User'"; $rs = mysql_query($sql,$conn) or die('Problem with query: ' . $sql . '<br />' . mysql_error()); $Row = mysql_fetch_array($rs); $ExistingUser = $Row['Username']; if (isset($_POST['SubmitB'])) { if(empty($Forename)) { $Errors++; $MsgForename = "! Please enter your Forename"; } if(empty($Surname)) { $Errors++; $MsgSurname = "! Please enter your Surname"; } if (!preg_match('/^[A-Z0-9._%-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i', $Email)) { $Errors++; $MsgEmail = "! Please Enter a valid Email Address"; } elseif($Email != $ConfirmEmail) { $Errors++; $MsgEmail = "! The Email Addresses do not match please re-confirm them"; } if(empty($User)) { $Errors++; $MsgUser = "! Please enter a Username"; } elseif($ExistingUser == $User) { $Errors++; $MsgUser = "! That Username has already been taken, please enter a new one"; } if(empty($UserPass)) { $Errors++; $MsgPassword = "! Please enter a Password"; } elseif($UserPass != $ConfirmUserPass) { $Errors++; $MsgPassword = "! Please re-enter your Password"; } if($Errors==0) { $UserPass=sha1($Userpass); $conn = mysql_connect($Host,$Username,$Password) or die(mysql_error()); $db = mysql_select_db($Dbname, $conn); $sql1 = "SELECT UserID FROM UserAccount WHERE UserID='User*'"; $rs1 = mysql_query($sql1,$conn) or die('Problem with query: ' . $sql1 . '<br />' . mysql_error()); $NoOfUsers = mysql_num_rows($rs1); $UserID = "User".($NoOfUsers+1); $X = 1; while($OkUserID == false) { $sql2 = "SELECT UserID FROM UserAccount WHERE UserID='$UserID'"; $rs2 = mysql_query($sql2,$conn) or die('Problem with query: ' . $sql2 . '<br />' . mysql_error()); if(mysql_num_rows($rs2)==1) { $OkUserID = false; $UserID = "User".$X; } else { $OkUserID = true; } $X++; } $sqlNew = "INSERT INTO UserAccount (UserID,Username,Password,Forename,Surname,Email,AccountType,Status) VALUES('$UserID','$User','$UserPass','$Forename','$Surname','$Email','Student','0')"; $rsNew = mysql_query($sqlNew,$conn)or die('Problem with query: ' . $sqlNew . '<br />' . mysql_error()); if(mysql_num_rows($rsNew)==0) { $ValidReg = false; } else { $ValidReg = true; } mysql_close($conn); } } ?> <html> <head> <title>Clothing Line</title> <link href="admin/stylesheetCL.css" rel="stylesheet"> <?php require('admin/jscript.inc'); if($ValidLogin==true) { ?> <meta HTTP-EQUIV="REFRESH" content="0; url=index.php"> <?php } ?> <script language='JavaScript' type='text/JavaScript'> <!-- function random_string($len=5, $str='') { For($i=1; $i<=$len; $i++) { $ord=rand(48, 90); if((($ord >= 48) && ($ord <= 57)) || (($ord >= 65) && ($ord<= 90))) { $str.=chr($ord); } else { $str.=random_string(1); } } return $str; } function Validate(f){ return (ValidateValues(f)==0 ? true : false ); } function ValidateValues(f) { var Errors = 0 if((document.registration_form.Forename.value+'').length<1) { document.getElementById('mySpan1').innerHTML='! Please Enter your Forename!'; Errors++; } else { document.getElementById('mySpan1').innerHTML=''; } if((document.registration_form.Surname.value+'').length<1) { document.getElementById('mySpan2').innerHTML='! Please Enter your Surname!'; Errors++; } else { document.getElementById('mySpan2').innerHTML=''; } if(document.registration_form.Email.value =="") { Errors++ document.getElementById('mySpan3').innerHTML='! Please Enter an Email Address!'; } else if(!document.registration_form.Email.value.match(/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/)) { Errors++ document.getElementById('mySpan3').innerHTML='! Please Enter a Valid Email Address!'; } else if(document.registration_form.Email.value !== document.registration_form.ConfirmEmail.value) { Errors++ document.getElementById('mySpan3').innerHTML='! The Email Addresses do not match, please re-confirm them!'; } else { document.getElementById('mySpan3').innerHTML=''; } if((document.registration_form.Username.value+'').length<1) { document.getElementById('mySpan4').innerHTML='! Please Enter a Username!'; Errors++; } else { document.getElementById('mySpan4').innerHTML=''; } if((document.registration_form.Password.value+'').length<1) { document.getElementById('mySpan5').innerHTML='! Please Enter a Password!'; Errors++; } else if(document.registration_form.Password.value !== document.registration_form.ConfirmPassword.value) { Errors++ document.getElementById('mySpan5').innerHTML='! The Email Addresses do not match, please re-confirm them!'; } else { document.getElementById('mySpan5').innerHTML=''; } return Errors; } //--> </script> </head> <body> <?php require('admin/header.inc'); require('menu.inc') ?> <div class="DivMain"> <span class="head2">Registration Form</span><br> <span class="errmsg">* All Marked fields required</span><br> <form method="post" name="registration_form" action="registration_form.php" onSubmit="return Validate(this);"> <table style="width: auto; position: absolute;"> <tr><td width="194"><span id="mySpan1" class="errmsg"><?php echo $MsgForename?></span></td> </tr> <tr><td><span class="errmsg">* </span><span class="head4">Forename:</span></td> <td width="302"><label><input type="text" maxlength="15" name="Forename" value="<?php echo $Forename?>"/></label> <tr><td><span id="mySpan2" class="errmsg"><?php echo $MsgSurname?></span></td></tr> <tr><td><span class="errmsg">* </span><span class="head4">Surname:</span></td> <td><label><input type="text" maxlength="20" name="Surname" value="<?php echo $Surname?>"/></label></td></tr> <tr></tr> <tr><td><span id="mySpan3" class="errmsg"><?php echo $MsgEmail?></span></td></tr> <tr><td><span class="errmsg">* </span><span class="head4"> Email:</span></td> <td><label> <input type="text" maxlength="50" name="Email" value="<?php echo $Email?>"/> </label></td></tr> <tr><td><span class="errmsg">* </span><span class="head4">Confirm Email:</span></td> <td><label><input type="text" maxlength="50" name="ConfirmEmail" value="<?php echo $ConfirmEmail?>"/> </label></td></tr> <tr></tr> <tr><td><span id="mySpan4" class="errmsg"><?php echo $MsgUser?></span></td></tr> <tr><td><span class="errmsg">* </span><span class="head4">Username:</span></td> <td><label><input type="text" name="Username" value="<?php echo $User?>"/></label></td></tr> <tr></tr> <tr><td><span id="mySpan5" class="errmsg"><?php echo $MsgPassword?></span><br></td></tr> <tr><td><span class="errmsg">* </span><span class="head4">Password:</span></td> <td><label><input name="Password" type="Password" value="<?php echo $UserPass?>" maxlength="15"/> </label></td></tr> <tr><td><span class="errmsg">* </span><span class="head4">Confirm Password:</span></td> <td><label><input name="ConfirmPassword" type="password" value="<?php echo $ConfirmUserPass?>" maxlength="15"/> </label></td></tr> <tr></tr> <tr><td><span id="mySpan5" class="errmsg"><?php echo $MsgVerify?></span></td></tr> <tr><td><span class="errmsg">* </span><span class="head4">Verify Registration <br />(Copy the text exactly):</span> </td><td><?php $rand_str=random_string(5);?> <span class"head2"><?php echo $rand_str?></span> <?php $_SESSION['Rand_str']= $rand_str?></td></tr> <tr><td></td><td><label><input type="text" maxlength="5" name="Verify"/></label> <?php unset($rand_str)?></td></tr> <tr><td></td><td> <input type="submit" style="overflow:visible; width:auto" class="buttonS" name="SubmitB" value="Submit" onMouseOver="OverMouse(this)"; onMouseOut="OutMouse(this)"/> <input type="reset" class="buttonS" name="ResetB" value="Reset" onmouseover="OvermOuse(this)"; onmouseout="OutMouse(this)"/></td></tr> </table> </form> </div> </body> </html> Can anyone help with this?
-
Check Button validation help
psychowolvesbane replied to psychowolvesbane's topic in Javascript Help
BTW I have got this solved via another source, the code I used without using ID's was: var valuesSelected=[]; var checks=document['AddProduct']['Sizes[]'], i=0, t='', c; while(c=checks[i++]) { c.checked?valuesSelected[valuesSelected.length]=c.value:null; } !valuesSelected.length?t='! Please choose any relevant Sizes!':null; document.getElementById('mySpan7').innerHTML=t; Topic Solved! -
Check Button validation help
psychowolvesbane replied to psychowolvesbane's topic in Javascript Help
I get what you're saying but I'm unsure how to change what I already have in my function to access the id's, because the only way I know how to access an element by it's id is document.getElementById. So in this case would it be document.getElementById(' "Sizes"+i ')? -
I got 7 checkboxes which are basically sizes for clothing, XS, S, M, L, XL, XXL, and 3XL and I am trying to validate them using javascript, all I need is for it to know if any have been checked, and if none haven't bring up the warning message I already have in place. The only problem I've been getting so far is accessing the check button group I've made, which is an array called Sizes[]. Here's the form code: <?php $CBSizes = array("XS","Small", "Medium", "Large", "XL", "XXL", "3XL"); ?> <span id="mySpan7" class="errmsg"><?php echo $Message_ASizes ?></span><br> <span class="head4">Available Sizes:</span><br> <table id="Sizes"><tr> <?php $X=0; while($X!=7) { $Size = $CBSizes[$X]; echo"<td>$Size:</td>"; echo"<td><input type='checkbox' name='Sizes[]' value='$Size'"; if($SizeA[$Size]==true){echo"Checked";}?>></td></tr><tr> <?php $X++; } ?> </tr></table> As you can see I use the array CBSizes to grab the names per value of $X, and place all the Sizes into the Sizes[] array. I have a method already in PHP for validating it but I haven't got a working one for Javascript. Here's the code that's in my main validation function: numBoxesSelected = 0; var valuesSelected = new Array; for (var i=1; i <= numBoxes; i++) { if (eval("document.AddProduct.Sizes" + i + ".checked")) { valuesSelected[numBoxesSelected] = eval("document.AddProduct.Sizes" + i + ".value"); numBoxesSelected++; } } if(numBoxesSelected ==0) { document.getElementById('mySpan7').innerHTML='! Please choose any relevant Sizes!'; } else { document.getElementById('mySpan7').innerHTML=''; } Thanks in advance for those that help.