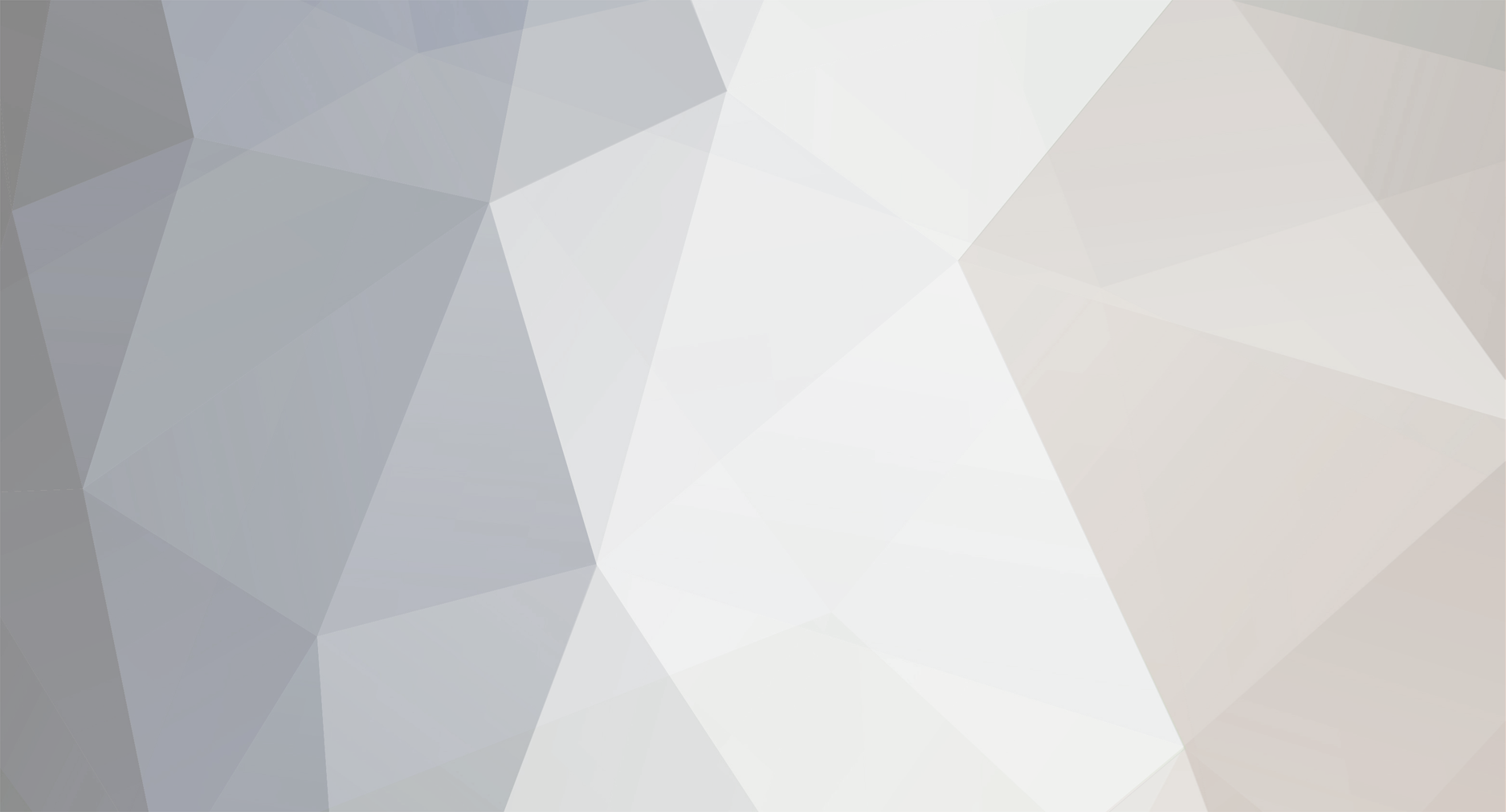
awpti
-
Posts
459 -
Joined
-
Last visited
Never
Posts posted by awpti
-
-
I can only tell you that ZF makes my eyes melt and brain explode. On a very random basis.
I can't even determine from your code why it would throw the errors your seeing, let alone why it was previously kicking back an empty array. That's weird.
It LOOKS fine..
-
This is generally done using regular expressions to look at the content and match valid URLs.
This tends to be slow compared to using tags since ereg/preg functions are, by their very nature, slow. Better to keep with the tagging system and start making use of, say, FCKEditor or TinyMCE to make posting easier.
-
Absolutely. I'd recommend going to the vBulletin Support Forums for information on how to do this.
-
FTP is a protocal. Doesn't matter if you connect via IP or some random hostname as long as that hostname reverses to the same IP that the actual FTP Service lives on.
ex:
geeklan.com
www.geeklan.com
ftp.geeklan.com
All reverse to: 208.109.240.109
I can connect via FTP to any of those addresses.
As far as other programs timing out.. sounds like an issue with the application.
By the way.. wrong forum.
-
I had to do something like this a long time ago (years):
<?php $quotes = file('quotes.txt'); $rand = rand(0, count($quotes) -1); echo $quotes[$rand];
quotes.txt:
This is a quote! -Cite This is another quote! -Cite This is yet another quote! -Cite Wow! More quotes! -Cite
Obviously, the less you have as far as number of quotes, the less random it's going to be.
If you are belching out a number of quotes and they must be unique, you'll need to keep track of keys that have been echo'd and make sure they aren't pushed out again.
-
So, because he found the issue within the 2 hours between the last response, he's a bad person for it?
Ouch.
-
Not necessarily. Check your error logs (error_log if on an Apache server)
-
I don't know!
What's the error? A little information goes a long way.
-
<?php include 'config.php'; mysql_select_db("exembar_site")or die("Cannot find specified Database!"); $user = mysql_real_escape_string($_POST['user']); $pass = mysql_real_escape_string($_POST['pass']); $result = mysql_query("SELECT * FROM `staff` WHERE `username`='{$user}' AND `password`='{$pass}'"); $count = mysql_num_rows($result); if($count == 1): echo 'Login Successful'; else: echo 'Login failed!'; endif; //commented out below and fixed syntax/logic. /* if($count == 1): //Since you only expect one result, a while() loop is not necessary) $info = mysql_fetch_assoc($result); session_start(); $_SESSION['id'] = $info['id']; $_SESSION['user'] = $info['user']; header("location:http://team.exembarstudios.exofire.net"); else: session_start(); $_SESSION['error'] = "Invalid Username or Password"; header("location:http://team.exembarstudios.exofire.net/login.php"); endif; */ ?>
You have a lot of needless repetition in your code. I've fixed the logic a bit. If it spits out "Login Successful!", then it's a problem with your index page on that domain.
Alternative PHP Syntax For the WIN!
-
Utilize FTP functions built in to PHP.
It'll look messy, that's about all I can say.
-
Ouch, that hurts my head.
Here's one I wrote up:
<?php class awpnav { function build_links($input_menu, $css_id, $css_id_ns, $uri) { if(!is_array($input_menu)) { return FALSE; } else { foreach($input_menu as $key => $value) { $class = ''; if($uri == str_replace('/', '', $key)) { $class = 'id="'.$css_id.'"'; } elseif($css_id_ns !== '') { $class = 'id="'.$css_id_ns.'"'; } $menu_final[] = '<li '.$class.'><a href="'.$key.'">'.$value.'</a></li>'; } } //Self-explanitory class. Returns a simple array or FALSE return $menu_final; } }
Here's how you use it:
<?php $menu = array ( '/' => 'Home', '/hosting' => 'Web Hosting', '/services' => 'Services', '/about' => 'About' ); $uri = ....; // Code to figure out the KEY of the array $css_id = 'current'; $css_id_ns = ''; //unselected item has no CSS ID $nav = new awpnav; $result = $nav->build_links($menu, $css_id, $css_id_ns, $uri);
Essentially:
build_links(array $menu, string $css_id, string $css_id_ns, string $uri);
It figures out which menu option to highlight based on the array key. The value of the key is what I actually pass to the page title before rendering the view (content). This needs to be manually edited each time you add a new menu item, but you don't have to add 5-6 lines of HTML+some code.
You can see it working here: http://awpti.org/
-
I accidentally this thread.
-
I bet you have Firebug.
Turn it off.
-
you're missing a quote here:
$daysofweek = array("Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday);
Look carefully.
-
Yeah. A non-32bit OS and PHP Compiled and designed specifically to handle files greather than 2.4GB in size.
Might be smarter to try two or more files (one calls the other). I can't imagine any scenario where this type of situation would be required. I smell bad design process.
-
I'd just recommend refactoring this beast. It's frightening.
Break it up into common functions and features. Try to stay away from strictly procedural coding as this will lead to painful amounts of repetition in your code. Wasted time on your part (spend coding) and wasted time spent parsing (server side).
Sit down with a knowledgeable developer (there are plenty here that willing to offer their insight into problems) and hash this beast out into something that isn't so frightening.
Consider looking into a framework (I recommend CodeIgniter) as these sort of force you into good development habits. Or, at least, slant you towards good habits.
-
Why not just use javascript?
Kinda pointless to do it in PHP and then spend the time writing the necessary JS/AJAX Functionality to check and recheck server-time.
-
Uh, better yet.
Read this - the proper documentation for the CI Framework.
The pagination content laid out on phpfreaks.com will not help in with this framework.
All of your questions can be answered there regarding functionality.
-
Please tab out and format your code according to accepted standards.
It is unreadable in this form.
Also, we would need all the files, including database structure and filler content to know the true location of the problem. As it stands, there is nothing to look at.
Have you checked the log files?
-
Most likely someone broke your FTP Password and just uploaded the changes.
Some content can be pulled in via javascript (hence "only viewable via the web").
Use a stronger password and check for vulnerabilities in your PHP Application (I'm guessing you didn't write it, so check the developer's site for news/info).
-
CV is what the brits call Resumes.
I'm not british, but I call mine a CV (Curriculum Vitae), too.
-
What does this have to do with PHP?
Contact cPanel support.
-
Up we go.
I have a decent amount of documentation relating to GeekWAN up on the private wiki. Been working on this project pretty hard this last weekend. And I'm still looking for an interested second/third party.
Would love to find a designer and at least one more like-minded developer (doesn't mind using an existing framework, quick thinker and creative).
(I may have a designer, though that's still up in the air)
-
PHP.net does it via round-robin based upon your 'guessed' geolocation.
Most hosts (ex, digg.com, cnn.com) utilize load-balancing. One head server redirects traffic via a piece of hardware or software to numerous other servers to handle the load. The load-balancer handles the incoming and outgoing flow of traffic, from what I recall.
Just having multiple files on a single server is pointless and does nothing - server load is affected by the number of processes running and how demanding they are on the server. 200 apache processes is still 200 apache processes.
codeigniter concept
in Frameworks
Posted
Oldish internet meme. Look it up.
Specifically: I accidentally the economy (G.W. Bush actually said this in a speech due to his lack of pacing)