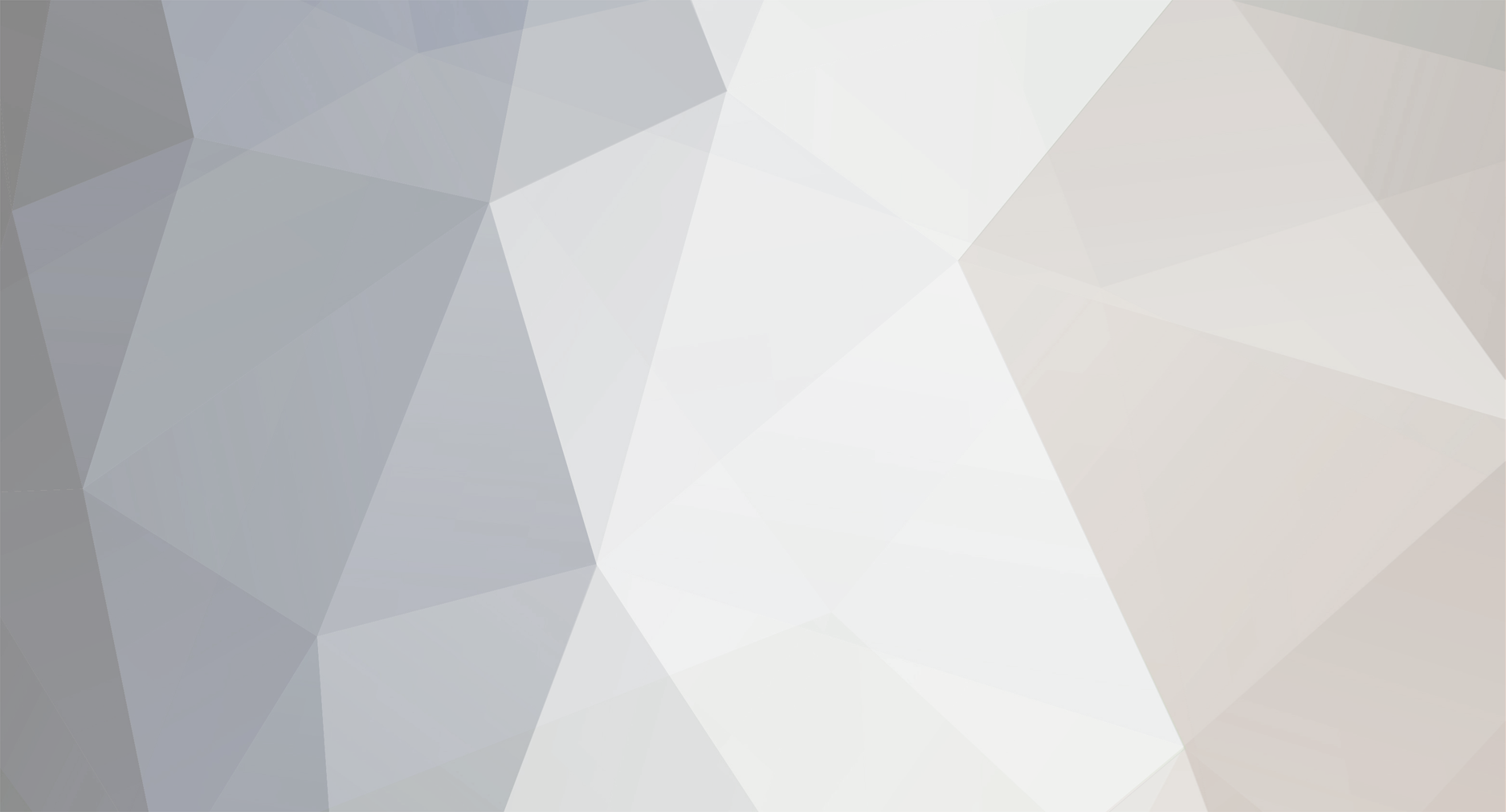
rhodesa
-
Posts
5,253 -
Joined
-
Last visited
Posts posted by rhodesa
-
-
well...the PHP code that is run when the AJAX call is made...put that in a function...like this:
function make_checkboxes ( $select_value, $checked_values = array() ) { }
In there, generate what needs to go in the DIV and either return it as a string or just echo it out directly. Assume $select_value is the value from the select box and $checked_values is an array of which which checkboxes should be pre-selected
Then, in the block of PHP code that gets run by the AJAX, use the function:
echo make_checkboxes($_GET['trade']);
since nothing is pre-checked, you only need one argument
Then, in the code to display the form, when echoing the SELECT box, make sure you use the $_POST value to determine which SELECT item should be pre-selected (you should have this). Then, in the DIV, if there is a POST, go ahead and use the function from above to show the checkboxes:
echo '<div id="extra">'; if($_POST) echo make_checkboxes($_POST['trade'],$_POST['checkboxes']); echo '</div>';
where 'checkboxes' is the name of the INPUT used for the checkboxes
-
I think you are looking for:
preg_match('/([0-9]{4})\.([0-9]{1,2})\.([0-9]{1,2})/', $data[$nn], $matches);
but it can be shortened to:
preg_match('/(\d{4})\.(\d{1,2})\.(\d{1,2})/', $data[$nn], $matches);
-
first...you need to remove the "image_small IS NOT NULL" from your WHERE clauses so the records show up at all. then, in your PHP, just set a variable at the top with the path to the default image:
$default_small = 'images/defaultimage.jpg';
then, in your loop, change this:
$image_small = $db->f("image_small");
to
$image_small = $db->f("image_small") ? $db->f("image_small") : $default_small;
edit: you could skip the extra variable declaration and just put the path of the image in the loop, i just like having it at the top so it's easy to change in the future
-
can you clarify?
do you mean, when the page is posted and there is a validation error or something...it needs to show what it did before?
or do you mean, i fill out the form, submit it successfully, and navigate somewhere else. i then navigate to the form again...at this point, the select box and div should be filled in the same as before.
-
if you have the original image, and the list of commands run on that image to reach the current image, you can always go back in time by just reverting to the original image and re-applying the actions up to the point where you want to revert to
-
the problem with the in_marray() is it can be a waste of resources. as long as your arrays stay small it's fine...you may want to modify it a bit for your case though:
function my_in_array($key, $needle, $haystack) { foreach($haystack as $item) { if($item[$key] == $needle) return true; } return false; } if (!my_in_array('primary_domain', $variable, $array)) { }
-
um...it's not "bad"...just be careful to clear those out
you can also pass the items to be modified in a GET variable when forwarding to modify.php:
$keys = array(1,4,6,7,9); header('Location: modify.php?keys='.implode('|',$keys)); exit;
the just explode the values on modify.php to know which ones you are modifying
-
when using $array with in_array() it looks at just the values of $array...which is 10 different arrays. it doesn't look any deeper then that. in each item of $array, you have another array. That array has an item with a key of 'primary_domain' in it...are there other keys or is this the only one?
-
ajax is really the best way to go here...if you post your whole file, i can probably show you how to work it in...and maybe show you how to clean up some of the other code
-
you can't push it to a new page, both the modify/delete code has to be handled by the decision.php script. this is easy enough though...you can either put all the code in one file, or create a function for each and just call the appropriate function
-
3 problems...fixed and commented:
<html> <head> <title>Overzicht van nieuwsberichten</title> <style> >!- p, td, {font-family:'Verdana, Arial'; font-size: 11pt} -> </style> </head> <body> <?php $link = mysql_connect('localhost', 'root', ''); if (!$link) { die('Not connected : ' . mysql_error()); } $db_selected = mysql_select_db('nieuwssysteem', $link); if (!$db_selected) { die ('Can\'t use foo : ' . mysql_error()); } $sql= "SELECT titel, nieuwsbericht, geplaatst FROM nieuwsberichten"; $resultaat= mysql_query($sql); $aantal= mysql_num_fields($resultaat); echo "<table border=\"1\">"; echo "<tr>"; for ($nr=0; $nr < $aantal; $nr++) { //Missing $ on $nr $veldnaam= strtolower(mysql_field_name($resultaat, $nr)); echo "<td class=\"kop\">$veldnaam</td>"; } echo "</tr>"; $rijen = mysql_num_rows($resultaat); if ($rijen > 0) { for ($nr=0; $nr < $rijen; $nr++) { //Missing $ on $nr echo "<tr>"; for ($velden=0; $velden < $aantal; $velden++) { $waarde = mysql_result($resultaat, $nr, $velden); if (!waarde) { $waarde= " "; } echo "<td>$waarde</td>"; }//Missing brace here } } echo"</table>"; ?> </body> </html>
-
the variables inside the function are local to that function, so you need to pass them as arguments:
write_the_results($states4us, $sectors4us, $email4us);
-
What are the lines before it...you are probably missing a semi-colon somewhere
-
no...line 477 not 777...I still don't see where write_the_results() is called...it should be on line 477 though. Is it not?
-
before i give you a code sample...is all the data on one page already? if not, you can't do the CSS route
-
<?php if(isset($_GET['trade'])){ //Everything in here will get echoed in the DIV echo "You selected: ".$_GET['trade']; exit; } if($_SERVER['REQUEST_METHOD'] == 'POST'){ //For debugging print_r($_POST); //Your error checking goes here //Once validated, so whatever exit; } ?> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.3/jquery.min.js"></script> <form name="x" action="" method="POST"> Some Text Box: <input type="text" name="some_text" /><br /> Our Select Box: <select name="trade" onchange="$('#extra').load('?trade='+this.value);"> <option value="1">item1</option> <option value="2">item2</option> <option value="3">item3</option> <option value="4">item4</option> </select> <div id="extra" style="color:red;"></div> Another Text Box: <input type="text" name="another_text" /><br /> <input type="submit" value="register"> </form>
-
Ok, if you want PHP to do something with the variable, you need to make another request. If you are ok with the page submitting, You can do this:
<form name="x" action="" method="GET"> <select name="trade" onchange="this.form.submit();"> <option value="1">item1</option> <option value="2">item2</option> <option value="3">item3</option> <option value="4">item4</option> </select> <div id="extra"><?php if(isset($_GET['trade'])){ echo "You selected ".$_GET['trade']; } ?></div> </form>
...I changed it to GET instead of POST, so if the user hits refresh they are presented with that annoying "Do you want to resubmit the data" prompt.
If you don't want the page refreshed, you can do it with AJAX. With this method, you can make a background request with JavaScript to the server, the server will return something, and then you can put that inside the DIV. Let me know if that is the route you want to go...
edit: ah, THAT is your problem. You could do that...you would need a hidden form field, that get's set on the onchange before the form is submitted....but I think the AJAX would be the better way to go....i'll post an example in a sec
-
Nothing jumps out at me as being wrong. But if you want to save yourself a headache, I would use this:
http://phpmailer.worxware.com/index.php?pg=phpmailer
It does all the work needed to send multipart emails including attachments
-
if($_GET['view'] == 'error'){ echo "<error>" . (defined($_GET['ecode']) ? constant($_GET['ecode']) : "Unknown Error") . "</error">; }
-
So you want a printer friendly page? You have two options, if it can be done with CSS, the best way is to create a new stylesheet that overrides anything you have on the page and hides all the useless stuff. Then add it with the media type of "print". That way, when looking at the page, they see the pretty version, but when printing, it's just a nice, basic, easy to read version.
If not all the data is on the screen (aka there is pagination), you will want to make a Printer Friendly link which opens a new window with all the data.
-
ok....we need to have a talk about client and server side code
PHP is server side code. When the client's browser calls for the page, the server takes the PHP file and runs it through the PHP parser. The PHP code does it's thing and spits out a bunch of text and sends it to the client. The contents of this can be seen by doing a view source on the page in your browser.
Then, the client receives all that text and runs it turns it into the HTML/CSS/JavaScript that you see in visual form on your browser. Now that the page is loaded, only client side actions can occur, which means no more PHP. You can pass data back to the server (so PHP can do something with it), but you will need to make another request (either via a page refresh, form post, or ajax call).
That being said, from what I understand, you don't need to pass the value back to PHP. Is there a reason PHP would need the value of that SELECT? Or does the value of the select just need to be displayed inside that DIV?
-
what is line 477 in state_entry.php? it says you aren't passing any arguments to it
-
what kind of variable? a php variable?
...just don't have anything in the DIV...i just left the "Your selection will apear here" text to show how it worked
-
the date() function can have multiple fields in one:
$pastDays = strtotime("-30 days"); $past = date('d/m/y',$pastDays);
...but if this relates to your other post, you won't be able to do a simple compare if the field in the table is a VARCHAR...you should convert it to a DATE field and then use MySQL functions instead of PHP
WEird Combination of fwrite and simplexml
in PHP Coding Help
Posted
should be