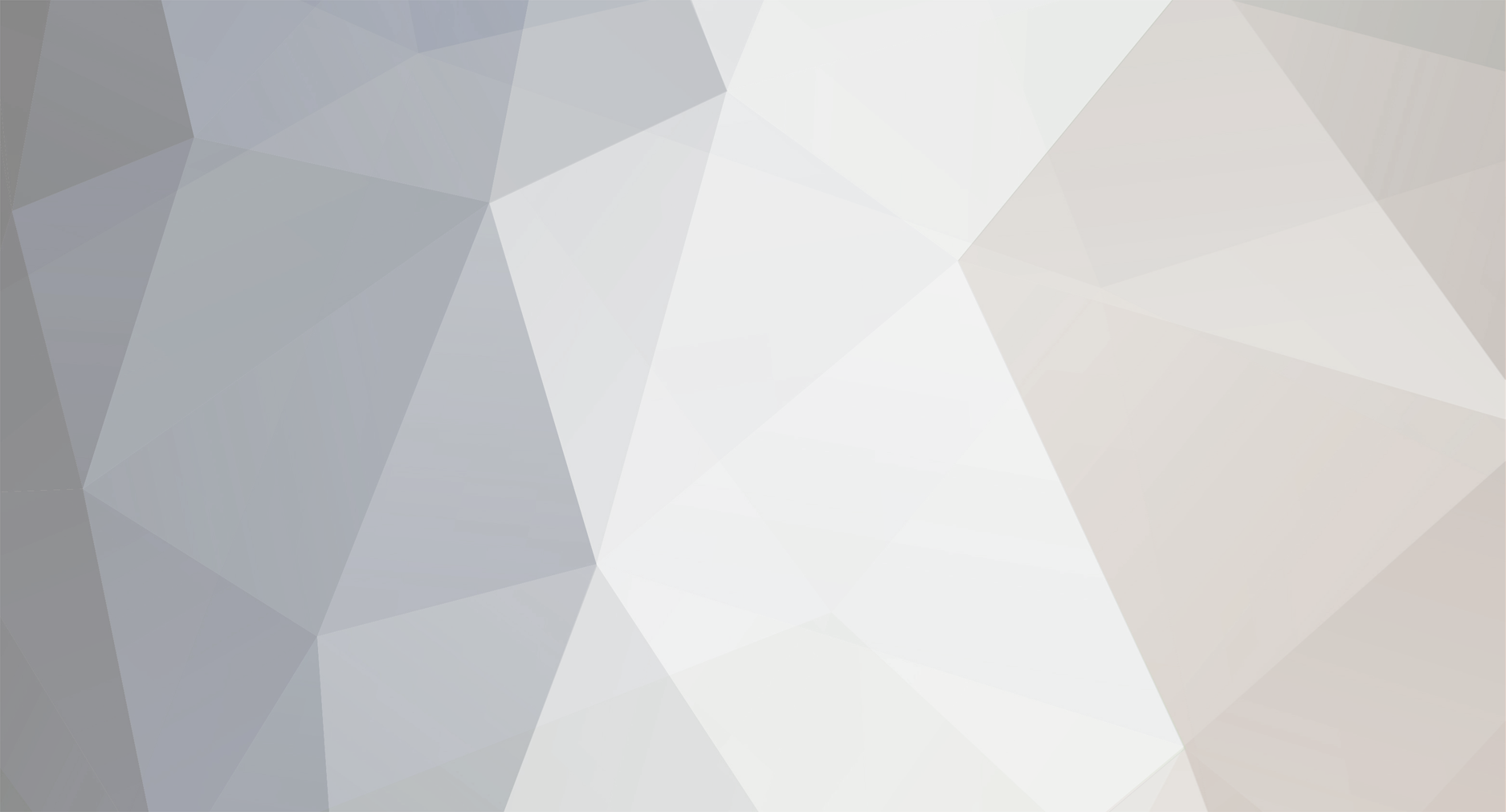
law
-
Posts
91 -
Joined
-
Last visited
Never
Posts posted by law
-
-
Ok im using ajax as a glorified iframe. I have a big registration form im loading into a div on my main page. The form has its own unique CSS from the main page (when called individually it works fine). When it is loaded through the main page div all of the main page CSS code over writes the css declaired within the head of the called document. This messes up the look of the whole registration form. I noticed two very different occurrences between browsers aswell. FireFox loads both css files but uses the main page CSS when they contain the same tag (example: <h1> tag is designed to look completely different on both pages.) IE ignores the called pages CSS all together. It doesn't load any of it on the main page.
As a side not my JS validation which is declared within the called page doesn't work when it is called through (even when i change the links to be relative the the main page).
-
thank you so very much i did not realize this was a possibility!
-
I am working for an organization at a large university in my area. The IT department at this university will not let us make any adjustments to their configuration settings AT All. So how can I produce a SAFE $_POST submission system for things like Image uploads and Long text files. I have heard that typically the limit is 2mb. Is there any way "hack" wise that I might could move around this? Multiple $_POST submissions that could be reassembled on the server? or heck anything... I just don't want to have to abandon this project with so many hours into it already.
** Note: I just tested out some issues on their servers and found the limit is much lower then 2mb. I attempted to $_POST several pages of text around 800kb of data, and it would not allow it through. This is a crucial feature of what the organization was wanting to perform on their site. **
-
I'm updating a field that is varchar. As a test I'm passing the string "Updated Title."
Here is my error: Truncated incorrect DOUBLE value: 'Title Updated'
Here is my code
//-==========Variables========================- $var0 = $_POST['creator']; $var1 = $_POST['date']; $var2 = $_POST['table']; $var3 = $_POST['id']; $var4 = $_POST['body']; $var5 = $_POST['title']; //-==========================================- if ($var3 !== 0){ $result = mysql_query("UPDATE $var2 SET title = '$var5' AND body = '$var4' AND creator = '$var0' AND date = '$var1' WHERE id = '$var3'")or die(mysql_error()); echo "update successful"; }else { $result = mysql_query("INSERT INTO $var2(title, body, creator, date) VALUES('$var5', '$var4', '$var0', '$var1')"); echo "<br/> insert successful";
My guess is this is some sort of syntax error. I am self taught which = I know nothing about correct syntax or structure. (or much of anything :-X)
-
nl2br(htmlentities($_POST['editor1_content']));
I apologize I am too drowsy... I was doing this
$variable= "nl2br(htmlentities($_POST['editor1_content']))";
which was giving me the whitespace error.. I noticed it after you guys had recommended that i echo it.. thank you for your time sorry.. I hate being the guy who always makes the stupid mistakes! :-X
** edit: If some one else searches this later take out the quotations just assign the variable like so
$variable= nl2br(htmlentities($_POST['editor1_content']));
while painfully obvious.. maybe someone is as dumb as i am and this will help them one day.. lol don't force yourself to code for a deadline.. it just makes you sloppy! **
-
I am using this great WYSIWYG editor from http://koivi.com/WYSIWYG-Editor/ it does all that I need. However it outputs the edited data like this
<?php echo nl2br(htmlentities($_POST['editor1_content'])); ?>
How can I take the data and produce a variable? If i try the obvious way
<?php $variable= nl2br(htmlentities($_POST['editor1_content'])); ?>
It give me a whitespace error? I have no idea what nl2br or htmlentites does to the $_POST however I am sure I need them for the data to be properly transferred to the mysql database. (That is the whole reason behind producing a variable.)
**Edit: I looked up in the php manual to see what nl2br and htmlentities were used to accomplish. It is now extremely important to me that their functionality for converting things into html be included in database entry.
Is there a way to produce their output assigned to a variable? **
-
unfortunately, it didn't die.. im really baffled as to what the issue could be.
for ($num=1;$num<=$num_rows;$num++){ $galleryqa = "SELECT * FROM gallery WHERE id = $num"; $gallerysqla = mysql_query($galleryqa) or die(mysql_error()); $galleryrowa = mysql_fetch_array($gallerysqla) or die(mysql_error());
ERROR
Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /www/includes/gallery.php on line 27
Could an older version of php on this server cause this issue?
-
If you are getting that error, then there is most probably a problem with the query. Change:
$gallerysqla = mysql_query($galleryqa);
to
$gallerysqla = mysql_query($galleryqa) or die(mysql_error());
I changed it to "or die." Yet, I get the same error, and the page does not "die" it continues to populate the rest of the page. here is the code change.
$galleryqa = "SELECT * FROM gallery WHERE id = $num"; $gallerysqla = mysql_query($galleryqa); $galleryrowa = mysql_fetch_array($gallerysqla) or die(mysql_error()) //<== line 25;
Here is the error
Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /home/failsafehomes/www/includes/gallery.php on line 25
-
i apologize here is the error
Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /www/includes/gallery.php on line 26
-
I just installed a clients website on another server as they requested. This is the error i get. This error for some reason unknown to me does not appear on my machine that I develop on. Anyone have any ideas?
This is a gallery.
================MYSQL EXAMPLE================== // this is NOT the database just a rough example ------------------------------------------ -GalleryNames Table - NAME OF TABLE ------------------------------------------ -id-----NameOfGal-ImageidMax-ImageidMin- COLUMN HEADERS ------------------------------------------ -#(pk)--Varchar----#(ImgID)--#(ImgID)--- TABLE DATA ------------------------------------------ -------------------------------------- -GalleryImages Table - NAME OF TABLE -------------------------------------- -(img)id--------ImgSRC-------ImgTitle- COLUMN HEADERS -------------------------------------- -#(ImgID)------Varchar--------Varchar--- TABLE DATA -------------------------------------- //***ImgSRC points to the location of the picture on the server***\\
Query that is generating the problem.
for ($num=1;$num<=$num_rows;$num++){ $galleryqa = "SELECT * FROM gallery WHERE id = $num"; $gallerysqla = mysql_query($galleryqa); // echo "$gallerysqla"; ///========================THIS ECHO RETURNS VALID RESOURCE ID #'s $galleryrowa = mysql_fetch_array($gallerysqla);//<==Problem $gallerytitle[$num] = $galleryrowa[galtitle]; $galleryinfo1[$num] = $galleryrowa[galinfo1]; $galleryinfo2[$num] = $galleryrowa[galinfo2]; $galleryinfo3[$num] = $galleryrowa[galinfo3]; $galleryidmin[$num] = $galleryrowa[imgidmin]; $galleryidmax[$num] = $galleryrowa[imgidmax];
-
if you don't have "date submitted" field or you want to grab the highest id row of some other table you can use num_row to produce the id number for your query
-
ok i tried it.. thats a no go either.. i still get "nosession --"
-
sorry should have been more specific.. the code never satisfies this statement
if ($submit == "Login"){
none of the other $_POST ['Variables'] are being picked up either... soo when i submit the form brings me to the login page and all it says is the following
"nosession -- -- "
this leads me to believe that i'm retrieving or sending the POSTs wrong.. or something is wrong with my WAMP.. i have other login systems that work.. so i don't know if its WAMP.. also should i turn GLOBALS off? i have them on currently (i'm developing on a laptop that doesn't serve the pages publicly) however i have heard that they could be a security threat.. so is it best for "good coding" to not use globals?
-
I'm sure that this is some stupid error but my login script doesn't work.. but can some one tell me why this doesn't work.. also i would love and security advice you guys may have
if($userloggedin !== 1){ /* if($_GET loggedin==1){ echo "<h5>Previous session was deleted. For security reasons, please log back in.</h5>"; } if($_GET improperlogin==1) { echo "<h5>Your Username or Password are incorrect.</h5>" } */ echo" <form action='./includes/login.php' method='POST'> <input type='text' value='Username'/> <input type='password' value='Password'/> <input type='Submit' value='Login' class='button' /> </form> </p> "; }else{ echo " <a href = './includes/logout.php'>Logout</a> "; }
<?php include_once('../dbconfig.php'); if (!isset($_SESSION['user_name'])){ // Diagnosing code only $submit = "$_POST[submit]"; $user = "$_POST[username]"; $pass = "$_POST[Password]"; echo"nosession $submit -- $user -- $pass"; //The above will not be included in the code ^ if ($submit == "Login"){ $md5pass = md5($_POST['password']); $sql = "SELECT id, nickname, privilages FROM admin WHERE username = '$user_name' AND password = '$md5pass'"; $result = mysql_query($sql) or die (mysql_error()); $num = mysql_num_rows($result); echo"submitted"; if ( $num !== 0 ) { // A matching row was found - the user is authenticated. session_start(); list($user_id,$user_name,$user_level) = mysql_fetch_row($result); // this sets variables in the session $_SESSION['user_id'] = $user_id; $_SESSION['user_name']= $user_name; $_SESSION['user_level'] = $user_level; $admin_id = $_SESSION['user_id']; $admin_name = $_SESSION['user_name']; $adminpriv = $_SESSION['user_level']; $usersession = md5($admin_name); //=======================Query's========================= //take user id and $usersession and put it into the database... delete row from user id if it exists $sql = "SELECT id FROM adsession WHERE id = $admin_id"; $result = mysql_query($sql) or die (mysql_error()); $num = mysql_num_rows($result); if ( $num == 0 ) { $sql = "INSERT INTO adsession (id,md5name) value ('$admin_id','$usersession')"; $result = mysql_query($sql) or die (mysql_error()); } else{ header("Location: ./includes/logout.php?loggedin=1"); } //======================================================= header("Location: admenu.php?session=$usersession&action=none"); //} //echo "Logged in..."; //exit() //header("Location: admenu.php?session=$usersession&action=none"); } else { header("Location: admenu.php?login=InvalidLogin"); } } } else { echo "Checking your session and verifying you"; } ?>
-
If using if statements, use elseif. Otherwise, I would suggest using switch() instead.
ok ill look up switch() i've never used it before.. and whats the difference in if/if else? is it faster? or just a preference of yours?
<?php if(isset($_GET['page'])) $page = $_GET['page']; else $page = 1; switch($page) { case 1: //Whatever here break; case 2: //Whatever here break; } ?>
By the way...I think your issue has more to do with logic. In your code, you're saying that certain variables are defined ONLY if it's on a certain page number. What if they're not on that page? What part of your code addresses that?
um no the logic is correct if they are not on page 1 through 5 then they are not on a page that shows up in my top menu.. which means the menu does not need to represent it.. this code is purely to tell the menu what button is to be depressed..
-
Sorry about the ridiculous subject. I have been trying to implement some more features on a website and I'm using some $_GET techniques. Apparently I suck at them tho.. does anyone see whats up with this?
if (isset($_GET['page'])) { $page == $_GET['page']; } else { $page == 1; } if ($page == 1){ $homeact = active; } if ($page == 2){ $calact = active; } if ($page == 3){ $aboutact = active; } if ($page == 4){ $comact = active; } if ($page == 5){ $contact = active; } <div id="menu-container"> <ul> echo " <li><a href='./index.php?page=1#Home' title='Welcome! Our home is your home!' class='$homeact'>Home</a></li> <li><a href='./index.php?page=2#Calendar' title='Upcoming Events' class='$calact'>Calendar</a></li> <li><a href='./index.php?page=3#Aboutus' title='Learn About Us' style='width: 8.7em;' class='$aboutact'>About Us</a></li> <li><a href='./index.php?page=4#Community' title='Give back to the CSA' class='$comact'>Community</a></li> <li><a href='./index.php?page=5#Contact' title='Contact Us' style='width: 7em;' class='$contact'>Contact</a></li> </ul> ";
The "active" class is supposed to make the button appear "on." Also as a side note.. I can't get $page to print ANYTHING when i try to echo it.
-
move #Community to the end of url
you nailed it! ... thanks a ton
-
Now if I could only get this simple pagination to work!! any one see what i'm doing wrong?
if (isset($_GET['galno'])) { $pageno = $_GET['galno']; } else { $pageno = 1; } $pageno = (int)$pageno; if ($pageno > $num_rows) { $pageno = $num_rows; } if ($pageno < 1) { $pageno = 1; } if ($pageno == 1) { echo " FIRST PREV "; } else { echo " <a href='./index.php#Community?galno=1'>FIRST</a> "; $prevpage = $pageno - 1; echo " <a href='./index.php#Community?galno=$prevpage'>PREV</a> "; } if ($pageno == $num_rows) { echo " NEXT LAST "; } else { $nextpage = $pageno + 1; echo " <a href='./index.php#Community?galno=$nextpage'>NEXT</a> "; echo " <a href='./index.php#Community?galno=$num_rows'>LAST</a> "; } echo " ( Page $pageno of $num_rows ) "; $num = $pageno;
$num_rows = the number of galleries I want to display in my database
$num = is in the gallery code to pick out what gallery to show
#Community = the page "container" where the galleries are located
The issue is that the $pageno always = 1.. that means the if($_GET(clause)) is never true? and i have no idea why??
-
well thats the exact reason.. im trying to make the gallery look "professional" / fancy
-
im racking my brain here.. and its not very big to begin with so im probly doing major damage to it..
Im working on a gallery.. the idea is that an administrator uploads photos & adds them to the database.. now the photos are placed into galleries and then called into one page.. the galleries look and work beautifully when only one is on a page at a time.. how can i make multiple galleries using <div>'s and <span>'s play TOGETHER and not over lap each other
<?php for ($num=1;$num<=$num_rows;$num++){ echo " <script type='text/javascript'> $(document).ready(function(){ $('.gallery_demo_unstyled').addClass('gallery_demo'); // adds new class name to maintain degradability $('ul.gallery_demo').galleria({ history : true, // activates the history object for bookmarking, back-button etc. clickNext : true, // helper for making the image clickable insert : '#main_image', // the containing selector for our main image onImage : function(image,caption,thumb) { // let's add some image effects for demonstration purposes // fade in the image & caption image.css('display','none').fadeIn(1000); caption.css('display','none').fadeIn(1000); // fetch the thumbnail container var _li = thumb.parents('li'); // fade out inactive thumbnail _li.siblings().children('img.selected').fadeTo(500,0.3); // fade in active thumbnail thumb.fadeTo('fast',1).addClass('selected'); // add a title for the clickable image image.attr('title','Next image >>'); }, onThumb : function(thumb) { // thumbnail effects goes here // fetch the thumbnail container var _li = thumb.parents('li'); // if thumbnail is active, fade all the way. var _fadeTo = _li.is('.active') ? '1' : '0.3'; // fade in the thumbnail when finnished loading thumb.css({display:'none',opacity:_fadeTo}).fadeIn(1500); // hover effects thumb.hover( function() { thumb.fadeTo('fast',1); }, function() { _li.not('.active').children('img').fadeTo('fast',0.3); } // don't fade out if the parent is active ) } }); }); </script>"; echo " <div class='demo'> <div id='main_image'></div> <ul class='gallery_demo_unstyled'>"; for ($i=$galleryidmin[$num];$i<=$galleryidmax[$num];$i++){ if($galimgsrc[$i] != null){ echo " <li><img src='$galimgsrc[$i]' alt='$galimgalt[$i]' title='$galimgtitle[$i]'></li> "; } } echo " </ul> <p class='nav'><a href='#' onclick='$.galleria.prev(); return false;'>« previous</a> | <a href='#' onclick='$.galleria.next(); return false;'>next »</a></p> </div> <div class='info'>"; if($gallerytitle[$num] != null){ echo " <h2>$gallerytitle[$num]</h2> <p>$galleryinfo1[$num]</p> <p>$galleryinfo2[$num]</p> <p>$galleryinfo3[$num]</p> "; } } ?> </div></div>
I grabbed someone else's gallery code and i'm using it for my site wish i remembered who so i could give credit.. but it was just some random internet site.. any way.. I don't know javascript.. soo how can i add some form of incrementation or some way to make each gallery unique... here is the CSS code for the gallery
<style media="screen,projection" type="text/css"> /* BEGIN DEMO STYLE */ * {margin:0;padding:0} .demo{position:relative;margin-top: 2em;} .gallery_demo{ align:top; width:800px; margin:0 auto;} .gallery_demo li{align:top; width:100px; height:50px; border:10px double #111; margin: 2px 2px; background:#000;} .gallery_demo li div{align:top; top:240px} .gallery_demo li div .caption{align:top; font:italic 1em/1.4 georgia,serif;} </style>
I'm lost.. on this one.. I was really hoping to get multiple galleries on one page.. if thats not possible then i guess ill learn how to do pagination? or would that even work?
-
SELECT * FROM Gallery, Images WHERE Images.id BETWEEN Gallery.imgminid AND Gallery.imgmaxid AND Gallery.ID='1'
i got the concept down.. now i just have to refine it.. thanks guys.. problem solved
-
ok i slept on it.. and now it makes since.. programming all night is over rated! or maby im just terrible at it.
anyway.. here is what i need to be my new sql query.. can anyone tell me how this "should" be written because its currently not working correctly
SELECT * FROM Gallery, Images WHERE Gallery.imgminid = Images.id AND Gallery.imgmaxid >= Images.id AND Gallery.ID='1'
the imgminid and the imgmaxid are intended to provide me with the range that the user wants within their gallery once i have this working ill work on expanding and improving the idea i just need an example that works for now.. so can anyone tell me how to add multiple clauses to a WHERE statement? in this code so that i can begin the process of rewriting my php code
-
interesting.. great.. ok let me explain what im doing with the above code maby you can point out something im not seeing... because i don't know how to normalize this database.. I'm making a photo gallery of sorts.. the idea is that the user can choose what pictures from the database that they want to show up on their gallery and then name their own gallery.. the idea is that the small community (just a local college group website.. nothing fancy.. they can put up all their photo's and make a gallery of events from the way they remember their night/event being) so the pictures are all indexed in the database.. but they don't really have a foreign key (as of now) the user just creates a gallery in the gallery table and then once they choose the pictures it puts the picture id's into the gallery table and that becomes my foreign key to use in the other query to pull the pictures..
soo thats my issue i need a variable that knows what the gallery it belongs to and what picture goes in said gallery soo my variable would have to look something like
$gallery[$num].$picture[num]
except that variable won't work?
-
ok im sure this is not the place for this question but can you give me an example of what a joined query would look like or do? I think i have the idea but i learn best through examples
Upside down Javascrip equalizer
in Javascript Help
Posted
OK, I found this javascript equalizer. It woks great. I cut it all up with php. I intend to make it easily editable. I am awful with javascript. I can't figure out how to flip this code. I would like the equalizer to operate upside down. The effect would look similar to filter: flipv; except be cross browser compatible.