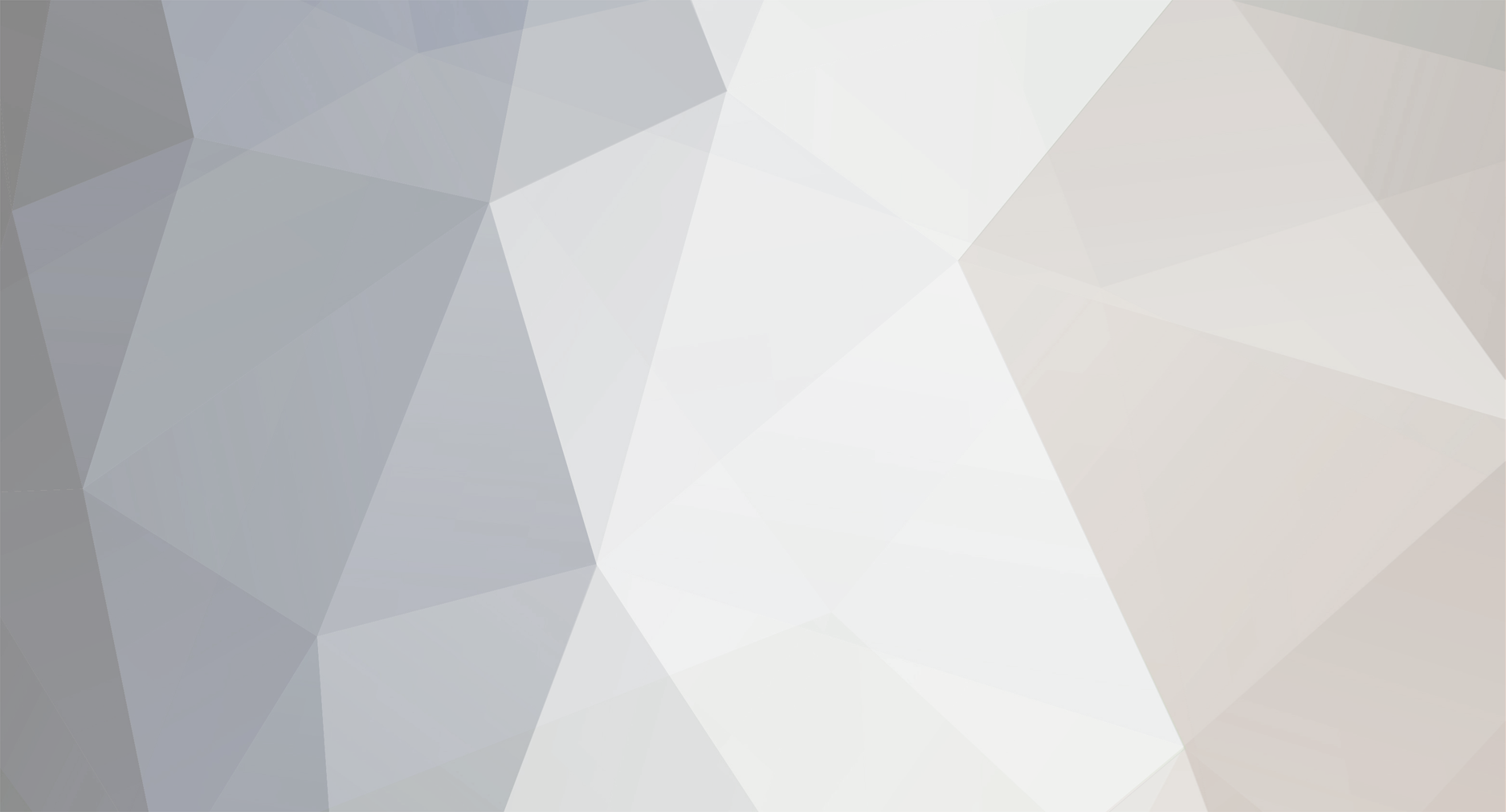
rubing
-
Posts
366 -
Joined
-
Last visited
Never
Posts posted by rubing
-
-
I am downloading large files via php with libcurl. The range option does not seem to be working:
$cp=curl_init($file); $fp=fopen ($g, "w"); curl_setopt ($cp, CURLOPT_FILE, $fp); curl_setopt ($cp, CURLOPT_HEADER ,0); curl_setopt($cp,CURLOPT_CONNECTTIMEOUT,20); curl_setopt($cp,CURLOPT_RANGE,0-3000000); curl_setopt($cp, CURLOPT_USERAGENT, $useragent); curl_setopt($cp, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($cp, CURLOPT_HTTPHEADER, $headers); curl_setopt($cp, CURLOPT_AUTOREFERER, true); curl_setopt($cp, CURLOPT_COOKIEJAR, "cookie.txt"); curl_setopt($cp, CURLOPT_COOKIEFILE, "cookie.txt"); curl_setopt($cp,CURLOPT_TIMEOUT,260); //lets download the mp3 directly for now if ($proxy!==FALSE) { curl_setopt($cp, CURLOPT_PROXY, $proxy); } curl_exec($cp);
Running this script and I receive an error like this:
Operation timed out after 260000 milliseconds with 4303905 out of 7709889 bytes received
So, obiously its downloading more than the range I specified above.
-
From my understanding the return value is bash's. And the 255 code means that the program generated a return value that was out of range.
Anyways, I just managed to fix by setting the permissions on these directories as 777
It seems that i need to do this with every directory php may write to, even though I added apache to the same group as my main user who owns these folders. I guess it's not really a problem since I am the only one who accesses the site, but this still bugs for the long term.
-
I am trying to execute system command, but am getting a return value of 255. (i guess from bash)
Here is my php script:
<?php echo '<pre>'; passthru('lame --mp3input --resample 44.1 -m s --alt-preset cbr 192 -q 0 /home/bob/public_html/blahblah.com/public/ads/fosters.mp3 /home/bob/public_html/blahblah.com/public/ads/fosters2.mp3',$retval); // Printing additional info echo ' </pre> <hr />output: <hr />Return value: ' . $retval; ?>
And this is the output I get:
output:
Return value: 255
When I input this command on my shell it works fine.
-
actually i overlooked the pecl uninstall command
-
I just installed the pecl extension pecl_http. while it was installing i saw a warning fly by saying i did not have the libevent libraries installed. I went ahead installed them, but now if i try to reinstall the pecl_http extension it says:
Ignoring installed package pecl/pecl_http
Nothing to install
How can I recompile or uninstall/reinstall.
-
ok, just used 'INSERT IGNORE' and its fixed!
-
This was a bad idea!
Now, I am receiving an error whenever I try to insert a duplicate:
Exception: Duplicate entry '3359686450-3128' for key 2 in /home/bob/public_html/blahalha.com/public/blahah.php on line 70
-
well i figured out that it was a curl process. in this app curl downloads a remote file through a proxy. the proxy must've been going really slowly.
i fixed it, by setting the curl option CURLOPT_TIMEOUT 200
-
great ideas, i like them all
I ended up making a unique index as per instructions here:
http://www.gsdesign.ro/blog/mysql-make-a-combination-of-2-columns-unique/
-
I have a php web application that sometimes gets hung up. This is problematic b/c when it hangs there are no errors presented. How do I figure out what went wrong in this case??? Thanks!
-
hi, i need help with a query.
I want to change the following query, so that will not insert duplicate entries for records that have: (ip AND port) of an already existing record.
INSERT INTO ProxyList (ip,port,speed) VALUES ($ip,$port,$speed);
-
Really!! What a bunch of assholes! I guess I'll have to find a proxy to use.
-
I am trying to parse an xml/rss url, however when I try to get the url i get a http 304 not modified error. For example if I try to retrieve the file using file_get_contents, I receive the following error:
Warning: file_get_contents(http://www.xroxy.com/rss) [function.file-get-contents]: failed to open stream: HTTP request failed! HTTP/1.1 304 Not Modified in /home/rubing/public_html/mysite.com/public/xml.php on line 2
I guess this is b/c apache is keeping a cached copy of this page?? How do I tell it not to cache it. Or alternatively retrieve this cached copy??
THank you very much!
-
I am afraid there is some serious bug here!!! I am seeing these errors intermittenly, sometimes it happens, sometimes it doesn't. It crops up on a number of different queries. Here is the query i have seen it come up for the most often. [error follows query]
$sessionid=session_id(); $sessionid=$conn->real_escape_string($sessionid); //echo $sessionid; //lookup ad to play $query="SELECT ad.ad_id, ad.owner_api, ad.file,ad.plays_purchased, ad.times_played, ad_limit.advert_id, ad_log.sess_id, ad_log.date_played, ad_log.ad_played, plays_per_session, COUNT( ad_log.ad_played ) AS num_times_played FROM ad LEFT JOIN ad_limit ON ad.ad_id = ad_limit.advert_id LEFT JOIN ad_log ON ( ad_log.ad_played = ad.ad_id AND ad_log.sess_id ='".$sessionid."' ) WHERE ad.owner_api =".$api." AND ad.plays_purchased > ad.times_played GROUP BY ad.ad_id HAVING num_times_played < plays_per_session ORDER BY num_times_played ASC LIMIT 1"; //Set the query up to be executed $result = $conn->query($query); //*note api is an integer assigned by a get variable: //$api= (int) $_GET['api']
Error:
mysqli_sql_exception: No index used in query/prepared statement SELECT ad.ad_id, ad.owner_api, ad.file,ad.plays_purchased, ad.times_played, ad_limit.advert_id, ad_log.sess_id, ad_log.date_played, ad_log.ad_played, plays_per_session, COUNT( ad_log.ad_played ) AS num_times_played FROM ad LEFT JOIN ad_limit ON ad.ad_id = ad_limit.advert_id LEFT JOIN ad_log ON ( ad_log.ad_played = ad.ad_id AND ad_log.sess_id ='dec2316aa286d564e349096574b44861' ) WHERE ad.owner_api =1 AND ad.plays_purchased > ad.times_played GROUP BY ad.ad_id HAVING num_times_played < plays_per_session ORDER BY num_times_played ASC LIMIT 1 in /home/rubing/public_html/citymusicnow.com/public/music_serve.php on line 118
Call Stack
# Time Memory Function Location
1 0.0725 166688 {main}( ) ../music_serve.php:0
2 0.0753 258544 mysqli->query( ) ../music_serve.php:118
-
error reporting indicated that the error was due to not having an index defined. I defined several indices and it worked. I don't understand this b/c it was working fine 2 days ago. And it works fine in phpmyadmin w/out the indices.
-
This is driving me crazy. I have a query that works fine when I test it using phpmyadmin. However, when I try to execute it in my php script I receive the following error:
Warning: mysqli_result::fetch_assoc() [function.mysqli-result-fetch-assoc]: Couldn't fetch mysqli_result in /home/public_html/music_serve1.php on line 100
Here is the query:
SELECT ad.ad_id, ad.owner_api, ad.file, ad.plays_purchased, ad.times_played, ad_limit.advert_id, ad_log.sess_id, ad_log.date_played, ad_log.ad_played, plays_per_session, COUNT( ad_log.ad_played ) AS num_times_played FROM ad LEFT JOIN ad_limit ON ad.ad_id = ad_limit.advert_id LEFT JOIN ad_log ON ( ad_log.ad_played = ad.ad_id AND ad_log.sess_id = '927fd56a2dbf9aecd4162711bc159284' ) WHERE ad.owner_api =1 AND ad.plays_purchased > ad.times_played GROUP BY ad.ad_id HAVING num_times_played < plays_per_session ORDER BY num_times_played ASC LIMIT 1
-
yeah, but it seems to me that it should work if is set permissions as
700, b/c php is running with user id = 1000, the same as my user id. Since the folder is owned by my user, there shoudn't be a problem...yet there is?
-
If i set the permissions to 777 it works of, but still gives me a permission denied message for 755. ???
-
sorry i got it a little backwards....here is my user info:
uid=1000(bob) gid=1000(bob) groups=1000(bob)
and php gives the same uid=1000, gid=1000
the directory i am trying to write to is listed as follows:
drwxr-xr-x 2 bob bob 4096 Oct 19 00:43 played
,so there really shouldn't be a problem, right??
However I get the following error:
Warning: fopen(../public/played/e_santiago.mp3) [function.fopen]: failed to open stream: Permission denied in /home/bob/public_html/citymusicnow.com/private/lib_php/merge_mp3.inc on line 481
-
sorry i had that backwards. php is running as a user with id: 1000.
root id: 4096
shouldn't php run as root?
-
I get a permission denied warning when trying to write a file to disk using fopen, however fopen is enabled. I think this comes down to permissions. My suid and guid for php is 1000, however my directories on my server are all owned by a user 4096. Do I have to create a common group for php and the user??
Alternatively making all folders readable and writeable seems insecure and dangerous.
-
that is odd (kinda dumb)
so this is a constructor method? let me see if i'm hearing you clearly obsidian.
when class fridge is instantiated a dictionary list (optionally passed in), will be created and assigned to items
-
I'm learing python from a WROX beginning Python book. I am having trouble understanding their __init__(self, items={}): function. I guess it's a constructor function that's run when the class is instantiated? Here is their code:
#!/usr/bin/python class Fridge: """This class implements a fridge where ingredients can be added and removed individually, or in groups. The fridge will retain a count of every ingredient added or removed, and will raise an error if a sufficient quantity of an ingredient isn't present. Methods: has(food_name [, quantity]) - checks if the string food_name is in the fridge. Quantity will be set to 1 if you don't specify a number. has_various(foods) - checks if enough of every food in the dictionary is in the fridge add_one(food_name) - adds a single food_name to the fridge add_many(food_dict) - adds a whole dictionary filled with food get_one(food_name) - takes out a single food_name from the fridge get_many(food_dict) - takes out a whole dictionary worth of food. get_ingredients(food) - If passed an object that has the __ingredients__ method, get_many will invoke this to get the list of ingredients. """ def __init__(self, items={}): """Optionally pass in an initial dictionary of items""" if type(items) != type({}): raise TypeError, "Fridge requires a dictionary but was given %s" % type(items) self.items = items return ...
Is 'self' a reserved word here refrencing the object? OR just a regular variable?
What is the point of this line??
self.items = items
does there really need to be a return statement?
??? ???
-
That doesn't work either. Is there a command to take away the command prompt??
copying variables from parent
in Application Design
Posted
I was RTFMing today reading up on basic classes, when i came accross this users post to the php manual:
I copied and pasted his script, but it did not work. Then I figured that he must mean to replace $father_class with the actual instance name of the father class object. So, I replaced $father_class with just $father.
Php issues the warning:
Warning: Invalid argument supplied for foreach() in /home/bob/public_html/dirtrag.com/public/test.php on line 15
Finally, I said to myself:
self: why does this dude have the ampersand (&) in his code:
so, I tried removing the ampersand. still it does not work.
??? ???