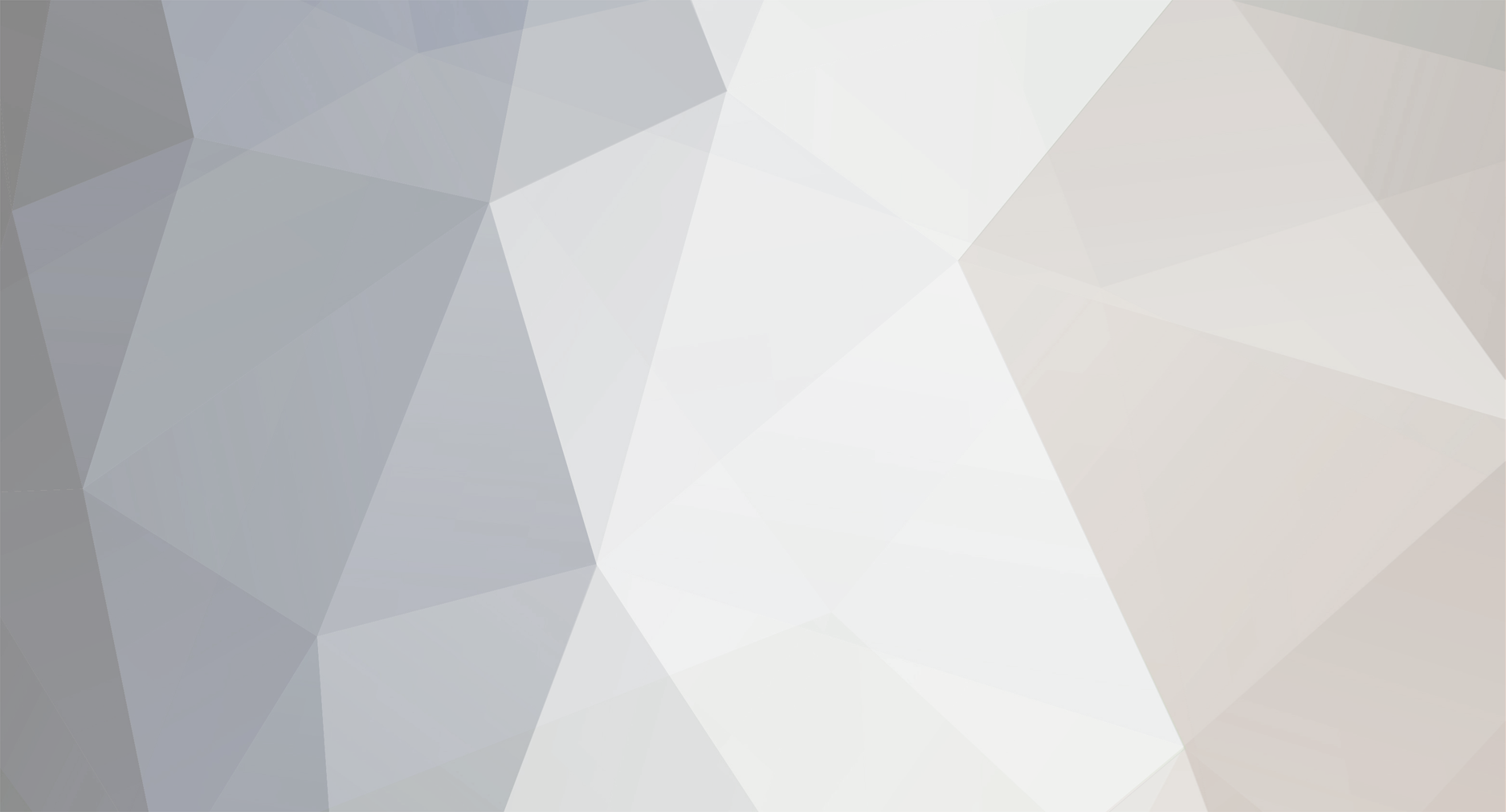
Bauer418
-
Posts
206 -
Joined
-
Last visited
Never
Posts posted by Bauer418
-
-
This should be a working example (don't currently have a testing server to put it on) which I'll post without using INSERT ON DUPLICATE KEY UPDATE so you can clear up your problems.
Text file (parts.txt - this file isn't tab delimited...it's actually 5 spaces, so the code won't work through copy/paste without changing the spaces to a tab):
prt-1535 My Part This part has 3 parts prt-1536 My Part 2 This part has 4 parts
Script file (parts.php - there are spaces in the fopen and fgetcsv commands because the forum won't let me post otherwise):
<?php $csv = f open('parts.txt', 'r'); while ($line = f getcsv($csv, 0, "\t")) { list($part_num, $part_name, $part_desc) = $line; $query = "SELECT * FROM parts WHERE part_num='" . $part_num . "'"; $result = mysql_query($query) or die(mysql_error()); if (mysql_num_rows($result)) { mysql_query("UPDATE parts SET part_name='" . $part_name . "', part_desc='" . $part_desc . "' WHERE part_num='" . $part_num . "'") or die(mysql_error()); print "Existing part updated in the database"; } else { mysql_query("INSERT INTO parts SET part_num='" . $part_num . "', part_name='" . $part_name . "', part_desc='" . $part_desc . "'") or die(mysql_error()); print "New part added to the database"; } } ?>
-
Did you give up on the single line INSERT ON DUPLICATE KEY UPDATE?
-
Take the space out between the word MAX and the parenthesis, it should say MAX(ordId) not MAX (ordId). Makes a huge difference in SQL.
-
You placed standard ticks. You need to use back ticks. It's the key to the left of the number 1, underneath escape.
-
It's because "order" (your MySQL table name) is also a MySQL keyword. Enclose it in back ticks, so it looks like: `order`
-
You could use SQL's:
INSERT ... ON DUPLICATE KEY UPDATE
Which will perform an update rather than a delete/reinsert
-
Before exit(), you would add:
header('Location: signupC.html');
-
New orderID should be set to 1 in that case.
-
<?php $query = "SELECT MAX (orderId) + 1 AS newOrderId FROM order"; $result = mysql_query($query) or die(mysql_error()); $row = mysql_fetch_assoc($result); $new_orderid = $row['newOrderId']; ?>
$new_orderid now contains your new order id.
-
Looks like what you were trying to achieve here:
if( $link['catparent'] == $category['ID'] ){ $selected = 'selected="selected"'; }
Your problem may lie in the fact that there's no space after the value and before the word selected. Try:
if( $link['catparent'] == $category['ID'] ){ $selected = ' selected="selected"'; }
-
Since your part numbers are set by you, not your database, it makes this a bit easier. I see that you already have the part number column in your database set to be a unique index. You can run a query such as:
REPLACE INTO table_name SET part_number='partnum', otherdata='otherval'
And continue finishing that SQL query. REPLACE INTO will search to see if a row with that part_number already exists. If it does, it'll delete the old row and replace it with the new data. If none exists, it'll just insert a new row.
-
register_globals is an old way in which global variables (url parameters, posted data, cookies, sessions, etc.) would be assigned to a variable with their name. For example, consider the url:
index.php?test=true
In the index.php script, $test would automatically be set to the value true. Eventually, PHP realized what kind of a security flaw this was. If your site relied on a variable $logged_in to determine if a user was logged in, in theory, someone could come to your site with the url
index.php?logged_in=1
And PHP would replace whatever previously defined variable of $logged_in you had, with the value 1 set from the URL. So, register_globals was removed, and now you're forced to use $_GET for parameters in the URL, $_POST for posted data, $_COOKIE for cookies, $_SESSION for sessions, etc.
That basically sums up the first line of the code (with the exception of knowing that urldecode and trim are just to clean up the value).
The rest of the code checks to see if the image exists (the purpose of the if statement) and then displays it if it does. If not, it displays the alternative text.
-
You'll can use usort (sorting based on a custom user-callback function) to sort based on a child of an array.
-
Your problem is that you're relying on register_globals which probably isn't enabled. You can try this in display.php:
<?php $pid = trim(urldecode($_GET['pid'])); if (isset($pid) && !empty($pid) && file_exists('../photos/' . $pid . '.jpg')) { print "<img src='../photos/" . $pid . ".jpg' />"; } else { print "Image does not exist"; } ?>
-
What's wrong with something simple, such as:
SELECT MAX(order_id) + 1 FROM orders
Which will take the highest order ID available, add one, and use it.
-
Thanks So Much!
I used this:
$data = "$row_rsActAnn[dateFrom]-$row_rsActAnn[dateTo]";
list($dateFromYear, $dateFromMonth, $dateFromDay, $dateToYear, $dateToMonth, $dateToDay) = explode("-", $data);
if ($dateFromMonth =="01") {
$dateFromMonth = "January";
}
if ($dateFromMonth =="02") {
$dateFromMonth = "February";
}
[...]
Not to get picky at you, but it may be more logical to set an array of $months, such as:
$months = array(1 => 'January', 2 => 'February', 3 => 'March' ...);
And then later in the script you can check $months[$dateFromMonth]. Instead of having lines and lines of if statements (relatively inefficient).
Also, you're aware that this will only handles
-
The reason your first query failed is because you had used the variable $metode in the query which was undefined. You can use a blank search in MySQL, meaning "...WHERE column LIKE '%%'" won't trigger an error. However, when PHP sees "...WHERE $metode LIKE '%%'" and doesn't have a value for $metode, you get "...WHERE LIKE '%%'" which will trigger a MySQL error. Also, try cleaning up your code again. Why do you set $variable1-24 when you can just use the originally indexed items of the array? It would save you coding space, time, and size.
-
mysql_affected_row will tell you how many rows were updated, not which ones. Although, if you were the one editing the rows, shouldn't you be able to tell which ones you changed?
-
Right here:
$description = trim($description[4][$i]);
You are overwriting the array that the script is looping through with a string. Try my example posted above, seeing as it has already been coded as a function as well.
-
Run just this, and tell me what you get:
<?php $username = "********"; $password = "********"; $hostname = "MYSQLHOST"; $dbhandle = mysql_connect($hostname, $username, $password) or die("Unable to connect to MySQL"); $selected = mysql_select_db("*********",$dbhandle) or die("Could not select *********"); $id = $_GET['id']; $query = mysql_query("SELECT tblLodges.strLodgeName, tblLodges.intLodgeNumber, tblLodges.strDistrictName, tblLodges.strLodgeMailingCity, tblLodges.strLodgeMailingPostCode, tblLodges.strLodgeCounty, tblOfficers.strOfficerTitle, tblOfficers.strFirstName, tblOfficers.strLastName, tblOfficers.BusinessPhone, tblOfficers.PersEmail FROM tblLodges LEFT JOIN tblOfficers ON tblLodges.lngLodgeID = tblOfficers.lngLodgeID WHERE $metode LIKE '%$search%' LIMIT 0, 50") or die(mysql_error()); ?>
Also, you also use a variable $metode in both queries which is not defined (at least, not in the part of the script that you showed us)
-
Even if you could, certain HTML code could cause validation errors on your page.
Consider:
<div>This is example text, <a href='#'>link</a> more random text</div>
If you cut the string after the </a>, you would still have an open <div> which you would have to close at some point or you may risk screwing up the layout of your page. I would say it's better to either strip the tags from the shortened version, or creating a completely separate "preview" that uses a different field in your DB or whatever your source is.
-
That's what the code above should do, just replace $_POST['name_field'] with the var containing the data.
-
$names = explode(',', $_POST['name_field']); array_walk($names, create_function('$value, $key', 'return trim($value);'));
This will take a string from a posted field (couldn't quite understand if you want the user to enter them comma-separated, or if you want them that way in the DB) and then split it into an array (and trim each item in the array to remove unnecessary spaces)
-
Place quotes around $name, so it should look like
echo("<div class='leftBold'>Name:</div><div class='rightTined'><input type='text' name='name' size='30' value=\"$name\"></div>");
PHP Navigation file
in PHP Coding Help
Posted
The settings are in your httpd.conf file, look for a line such as
AddHandler application/x-httpd-php php
And change it to
AddHandler application/x-httpd-php html htm php