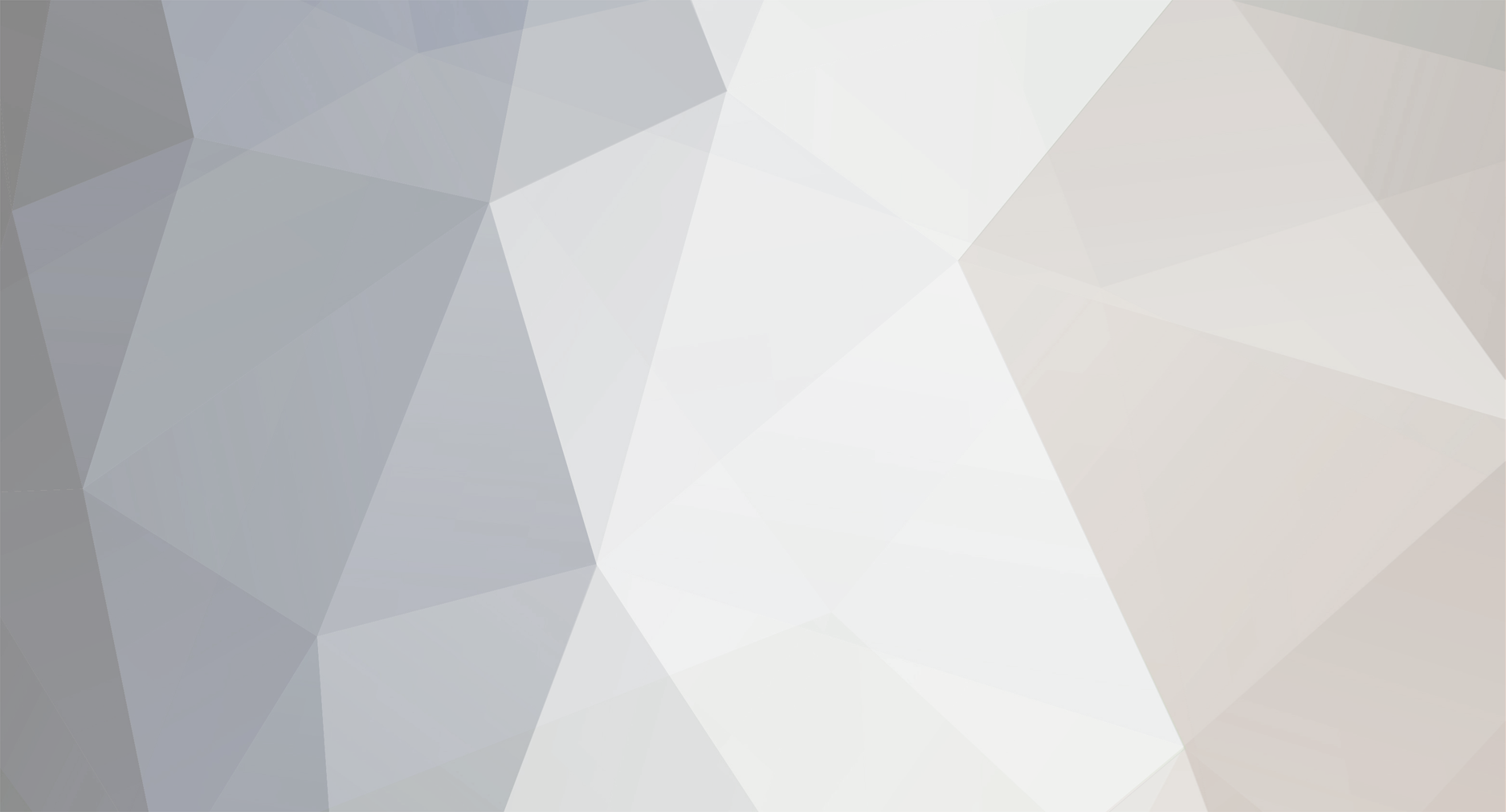
Zhadus
-
Posts
376 -
Joined
-
Last visited
Never
Posts posted by Zhadus
-
-
Is it still executing? The code should work fine as long as you are aware that the code you want executed IF the submit button has been pressed, is inside those { & } brackets.
-
Not sure how your server is configured, but have you tried changing your file from index2.html to index2.php and seeing if it'll run correctly?
-
Now that I figured out the problem. Fully agreed. Without internet browser settings set to update page each visit, it doesn't work. Friend was set on "automatic" and only worked when closing browser.
Anyway, a conversion to database from this should be easy and a bit more efficient.
-
I'm trying to develop a very small scale and simple chatroom for 2-3 people to use at a time. It will be used for admins only on a website.
To start with, I'm just having all the chat text stored in a text file and there is no login or verification etc. it just posts using IP.
It seems to work properly on IE6, but it wipes the 'chat' window whenever it refreshes on IE7.
Does anyone see the issue? Any comments on simplifying it or a better way of doing things will be appreciated.
easyim.html
<html> <head> <script type="text/javascript"> function refreshfunc(num) { ajaxRequest("read"); setTimeout('refreshfunc(5)', num * 1000); } function callback(serverData, serverStatus, mainval) { // Called automatically when we get data back from server if (mainval == 'read') document.getElementById('output').innerHTML = serverData; // Display an alert box with the recieved data else ajaxRequest('read'); } function ajaxRequest(mainval) { var url; if (mainval == 'read') url='MYPATH/getdata.php?doc=chat.txt'; // Get File URL if (mainval == 'write') url='MYPATH/writedata.php?doc=chat.txt&message=' + mainMSG; // Write File URL var mainMSG = document.getElementById('message').value; if (mainval == 'write') document.getElementById('message').value = ''; var AJAX = null; // Initialize the AJAX variable. if (window.XMLHttpRequest) { // Does this browser have an XMLHttpRequest object? AJAX=new XMLHttpRequest(); // Yes -- initialize it. } else { // No, try to initialize it IE style AJAX=new ActiveXObject("Microsoft.XMLHTTP"); // Wheee, ActiveX, how do we format c: again? } // End setup Ajax. if (AJAX==null) { // If we couldn't initialize Ajax... alert("Your browser doesn't support AJAX."); // Sorry msg. return false // Return false, couldn't set up ajax } AJAX.onreadystatechange = function() { // When the browser has the request info.. if (AJAX.readyState==4 || AJAX.readyState=="complete") { // see if the complete flag is set. callback(AJAX.responseText, AJAX.status, mainval); // Pass the response to our processing function } // End Ajax readystate check. } AJAX.open("GET", url, true); // Open the url this object was set-up with. AJAX.send(null); // Send the request. } </script> </head> <body onload="refreshfunc(5);"> <input type="button" value="Refresh" onclick="ajaxRequest('read');"> <input type="text" id="message"> <input type="button" value="Send Message" onclick="ajaxRequest('write');"> <div id="output"></div> </body> </html>
getdata.php
<?php $myFile = $_GET['doc']; $fh = fopen($myFile, 'r'); $old = ''; while(!feof($fh)) $old .= fgets($fh); echo $old; ?>
writedata.php
<?php date_default_timezone_set('GMT'); $myFile = $_GET['doc']; $chat = stripslashes($_GET['message']); $fh = fopen($myFile, 'r'); $old = ''; while(!feof($fh)) $old .= fgets($fh); fclose($fh); $fh = fopen($myFile, 'w'); $ip = $_SERVER['REMOTE_ADDR']; $time = date('h:i:s'); $chat = '(' . $time . ') ' . $ip . ': ' . $chat . '<br />' . $old; if (fwrite($fh, $chat) === FALSE) { exit; } ?>
-
You could do:
$GLOBALS['vein']=new vein();
Outside of the class and function.
-
That definitely proves it - just checking
No idea off hand, doing some testing to try and find out though.
-
How positive are you that the site is running PHP5?
-
Not positive, but try putting:
require_once('library/vein_funcs.php'); $vein=new vein();
inside of the class?
-
You need to store the information into a database like MySQL when you change the input and then the display area needs to load it from the database.
-
<?php $birthday = split('/', $birth); $years = date('Y') - $birthday[2]; if (birthday[1] > date('m')) { $years++; } elseif ((birthday[1] == date('n')) && (birthday[0] >= date('j'))) { $years++; } ?>
Untested, and might be an easier way, but that should work.
-
Use scandir() and check the .jpg in the filename from the array it retrieves.
-
Absolutely phenomenal. Thank you very much Mchl. Make it seem so easy
-
I'm new to the more advanced MySQL queries and what I currently need done I'm not even sure is possible.
I want to display a list of results and ORDER BY the email field, however, I have another field that states whether the email is visible to the public or is hidden. I want to include the hidden ones in the result, but not sort it (they should appear at the bottom of the results).
If this is possible, what is the general syntax? I am aware of other ways I can do it without MySQL, but this is preferred if possible.
-
The function that rhodesa posted should work great, but keep in mind that not all fonts are monospace fonts, so test it with an all caps link, or force all lowercase on the link.
Edit: In response to your question, you run the function with $referred as the input and return it into $referred.
-
Welcome to the PHP world. Please be more descriptive in the future though and google is your friend. It's a generous morning though, so:
<?php $file = "testfile.txt"; $fh = fopen($file, 'w') or die("Unable to Open"); $data = "Input Goes Here"; fwrite($fh, $data); fclose($fh); ?>
That will open testfile.txt or create it if it doesn't exist and write $data to it. The "w" portion in the fopen means it will overwrite anything already in there. This will be putting it in the same directory of the page that's running it.
-
\b would be word boundary, \s would be a whitespace, which works just fine in a sentence without symbols.
-
You should not insert the blog_id.
Also, you don't have enough values to fill all the 'NOT NULL' fields.
-
$i+2 should be $i += 2 I believe.
-
Check your password, if that's fine, please post your code so we can take a look.
-
No password is defined.
That gives an undefined variable, access denied, and connection to database failed.
-
A MySQL database or a flat text file will be required. You can use PHP to automatically read a directory and list the songs, but if you want them in a specific order (What I can tell from your picture) you'll need to do the following:
Put the files in the "song" folder and have your page read all the files using PHP. Then save the files in that order in the database or in a text file.
Then on the page, modify it using HTML forms and saving the changes to the text file.
Each time you visit the page, it should check that folder and see if there is anything new or missing.
If missing, remove from list, and if new, add at the end of the list.
There is the basic process if you're willing to learn more PHP to accomplish it.
-
Link it to his account in your database. If you don't have them login, then it really can't be saved indefinitely.
-
Instead of coming up with a wildcard, use regex or strpos().
-
If you have an undefined variable, and a function is using it, it's a non-object. That would be the first thing to investigate.
adding to the end of a cell
in PHP Coding Help
Posted
There are really multiple ways of doing it. I would recommend storing the values themselves in an array. For example:
this way, you get this as the output:
Otherwise you can use explode() for the info. And in what you need, and implode.
Or you could do it the easy way like rhodesa