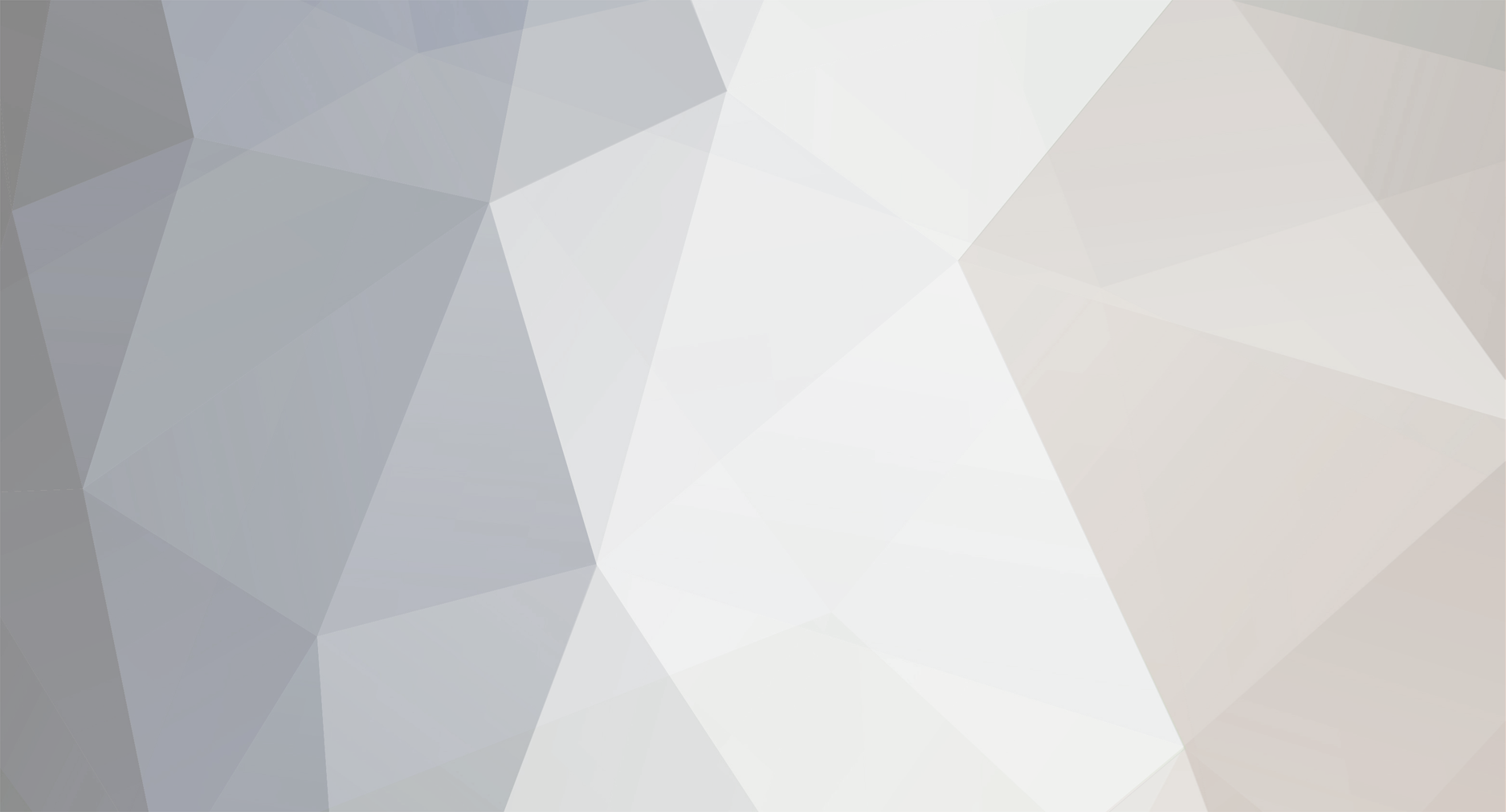
laffin
Members-
Posts
1,200 -
Joined
-
Last visited
Everything posted by laffin
-
Form Page (Submit Button) -> Processing Page (use header redirection) -> Display Page To Do this all in one page, u will check $_POST statements of $_SERVER['REQUEST_METHOD'] <?php if($_SERVER['REQUEST_METHOD']=='POST') { $username=$_POST['name']; // process your variables here } ?> <FORM METHOD='POST'> Username: <INPUT TYPE="TEXT" name='name' <?= (!empty($username)?"value=\"$username\"":'')?>><BR> <INPUT TYPE='SUBMIT'> </FORM> Very simple example of an all in one script
-
how to differentiate which submit button user clicks???
laffin replied to josephChiaWY's topic in PHP Coding Help
Either add a hidden input with the id for that user or change the submit name for that form instance. -
why not just use mysql_fetch_assoc? this way the array keys have the field names so a SELECT id,name,phone,zip would return an array like $row = array ('id' => '1', 'name' => 'wrave', 'phone' => '555-111-2222', 'zip' => '12345'); with a foreach($row as $key => $Val) which can give u the both key index and the value or use $fields=array_keys($row) if u need to seperate the keys from the values (Displayed as a table header)
-
Depends on the application you are gearing for. Although u can do the same thing in C/C++. The question comes in, how to implement it into a web server. DB Access, and other libraries. PHP is geared for rapid web development, under most environments. C/C++ u wud have to gather/build these libraries. As well as compile/test under different environments if you wanted to be cross platform. So Development language is dependant what the application is geared for. These arguments of This language is better than that language is left for those who limit their capabilities to one realm. So go ahead, learn some PHP, learn some C++, Basic, Python, Java, Ruby, Lua, Rebol and the hundred other languages that are available, and enjoy learning
-
mysql_fetch_array returns false when it is done executing, thus triggering the die situation. but there is no error to display while($row = mysql_fetch_array($data) or die("Error: ".mysql_error())){ print $row['name'] ."<br/>"; } to see yer code in action what shud be done is $data=mysql_query("SELECT * from users") or die("MySQL Error:". mysql_error()); while($row = mysql_fetch_array($data)){ print $row['name'] ."<br/>"; } now if u need to know if it returned any rows before your loop $data=mysql_query("SELECT * from users") or die("MySQL Error:". mysql_error()); if(!mysql_num_rows($data)) echo "No Info"; else { while($row = mysql_fetch_array($data)){ print $row['name'] ."<br/>"; } }
-
Need help pointing to a script I need>! pleaseee
laffin replied to Scabby's topic in PHP Coding Help
Now if u had a sample script, that was semi-functional, as haku stated, more ppl would be willing to help. But ya want a script from scratch, with very little information. Thus requesting someone to write it for u. Yer right, the SQL is very simple, since all u have to do is lookup a zipcode or similar info, and send info to the designation. This is where the API comes in. -
Checking for proper file extensions, if present, return true...
laffin replied to cgm225's topic in PHP Coding Help
if u use strpos, it will find the first occurance use strrpos instead which finds the last occurance. in the example i use preg_match('/^.+\.(jpg|jpeg|gif|png)$/i',$filename) smallest way of accomplishing of what is asked, without extra support functions however as Andy states, an extension does not deem it a valid image. try using the image information routines to validate a valid image. -
Checking for proper file extensions, if present, return true...
laffin replied to cgm225's topic in PHP Coding Help
<?php header('Content-type: text/plain'); $filename='some.jPeG'; echo preg_match('/^.+\.(jpg|jpeg|gif|png)$/i',$filename)."\n"; $filename='some.gif'; echo preg_match('/^.+\.(jpg|jpeg|gif|png)$/i',$filename)."\n"; $filename='some.PNG'; echo preg_match('/^.+\.(jpg|jpeg|gif|png)$/i',$filename)."\n"; $filename='some.php.gif'; echo preg_match('/^.+\.(jpg|jpeg|gif|png)$/i',$filename)."\n"; $filename='some.php'; echo preg_match('/^.+\.(jpg|jpeg|gif|png)$/i',$filename)."\n"; ?> -
problem strtotime had a problem with the ';' in the string so have to remove that before getting all the stats. but it's pretty simple <?php header('Content-type: text/plain'); $da="February 16, 2008; 12:06 pm"; $da=str_replace(';','',$da); $diff=time(); $dad=strtotime($da); $diff-=$dad; $weeks=intval($diff/(7*24*60*60)); $diff%=(7*24*60*60); $days=intval($diff/(24*60*60)); $diff%=(24*60*60); $hrs=intval($diff/(60*60)); $diff%=(60*60); $min=intval($diff/60); $secs=$diff%=60; $ago= (!empty($weeks)?"$weeks week".($weeks!=1?'s':'').',':''). ((!empty($days) || !empty($weeks))?"$days day".($days!=1?'s':'').',':''). ((!empty($hrs) || !empty($days) || !empty($weeks))?"$hrs hour".($hrs!=1?'s':'').',':''). ((!empty($min) || !empty($hrs) || !empty($days) || !empty($weeks))?"$min minute".($min!=1?'s':'').',':''). "$secs second".($secs!=1?'s':''); echo $ago; ?>
-
Grabbing text from a site and adding to variables?
laffin replied to sean123's topic in PHP Coding Help
preg_match('@\s*([^\s]+)\s*Level: (\d+)\s+Ratio:\s+([\d.]+)+\s+Rank:\s+(\d+).+?Kills:\s+(\d+)\s+Deaths:\s+(\d+)\s+Experience:\s+(\d+)$@',$str,$matches); \s represents white spaces (spaces tabs newlines) -
Grabbing text from a site and adding to variables?
laffin replied to sean123's topic in PHP Coding Help
use preg match <?php header('Content-type: text/plain'); $str="username Level: 25 Ratio: 1.54 Rank: 3266 th Kills: 73761 Deaths: 9977 Experience: 674534632"; preg_match('@([^\s]+)\s*Level: (\d+)\s+Ratio:\s+([\d.]+)+\s+Rank:\s+(\d+).+?Kills:\s+(\d+)\s+Deaths:\s+(\d+)\s+Experience:\s+(\d+)$@',$str,$matches); print_r($matches); ?> /* Output: Array ( [0] => username Level: 25 Ratio: 1.54 Rank: 3266 th Kills: 73761 Deaths: 9977 Experience: 674534632 [1] => username [2] => 25 [3] => 1.54 [4] => 3266 [5] => 73761 [6] => 9977 [7] => 674534632 ) */ as u can see everything is captured well, item 0 can be ignored, as that is just the matched string. -
if u looked at the html coding for the page is what your after with either strpos or preg_match ya can get the temp I prefer preg_match <?php $contents=file_get_contents('http://www.almanac.com/weatherhistory/oneday.php?number=725090&wban=14739&day=1&month=1&year=1946&searchtype=zip'); preg_match('@MEAN<br>TEMPERATURE.*?;">([\d\.]+)</b>@ms',$contents,$match); $mean=$match[1]; echo "Mean Temp: $mean<br>"; ?>
-
Heh, i like working with timestamps, and standard dates strings, and arrays. so wrote this code up. note: i use an array to break up the dates into a multidimensional array, by year,month,day. once that is accomplished, it's much easier to build a logic to create date ranges. Since all is seperated it's just the day logic that needs work on <?php function GetDateRange($da) { if(empty($da)) return NULL; foreach($da as $day) { $ds = gmdate('Y-m-d',$day); $dp = explode('-',$ds); $dma[$dp[0]][$dp[1]][]=$dp[2]; } $out=''; foreach($dma as $ky => $years) { foreach($years as $km => $months) { $dr=0; $days=array(); $dc=count($months); for($i=1;$i<$dc;$i++) { $yd=$months[$i-1]; $td=$months[$i]; if(!$dr) { if($yd==($td-1)) $dr=$yd; else $days[]=$yd; } else { if ($dr && $yd!=($td-1)) { $days[]="$dr-$yd"; $dr=0; } } } if($dr) $days[]="$dr-$td"; else $days[]="$td"; $out.=(!empty($out)?' ':'').implode(',',$days); $out.=' '.gmdate('F',strtotime("$ky-$km")); } $out.=' '. $ky; } return $out; } header('Content-type: text/plain'); define('SECS_PER_DAY',(24*60*60)); $start_date="2007-12-15"; $da[]=$lt=strtotime($start_date); mt_srand(time()); for($i=1;$i<15;$i++) { $days=mt_rand(1,5)*SECS_PER_DAY; $da[]=($lt+=$days); } foreach ($da as $key => $day) { echo sprintf("%02d",$key) .") ". gmdate("Y-m-d",$day) ."\n"; } echo GetDateRange($da); echo "\n\n"; $da=array(); $start_date="12/15/2007"; $da[]=$lt=strtotime($start_date); mt_srand(time()); for($i=1;$i<15;$i++) { $days=mt_rand(1,2)*SECS_PER_DAY; $da[]=$lt+=$days; } foreach ($da as $key => $day) { echo sprintf("%02d",$key) .") ". gmdate("Y-m-d",$day) ."\n"; } echo GetDateRange($da); echo "\n"; ?> I got these results
-
thank you page to redirect to different urls
laffin replied to andycharrington's topic in PHP Coding Help
What I'm saying, is everything was standardized, what ya ask wudda been possible. but not everyone follows thru on the specs HTTP_REFERRER wudda been an easy way to detect where a user came from $_SERVER['HTTP_REFERRER'] However this header variable is sent by the browser, which some may not send it, and if ppl know about this, some will try faking it. So your best bet is to use SESSIONS. now if u have a standard include that u include everywhere you can set a session, of the last page the user was on and exclude it from setting this session if it's in yer thankyou.php standard.php which is included in all php pages (or just add it to another global php file that is included to all yer scripts) [code] <?php session_start(); if(!PROCESSING) $_SESSION['lastpage']=$_SERVER['REQUEST_URI']; ?> thankyou.php <?php define('PROCESSING',1); include('standard.php') if(empty($_SESSION['lastpage'])) die('Can not access this page directly"); $baseurl=basedir($_SESSION['lastpage']); ?> <html> <head> <title>Thanks</title> <META http-equiv="refresh" content="5;URL=<?=$baseurl?>"></head> <body bgcolor="#ffffff"> <center>Thanks for using our system, you will now be sent from whence ya came</center> </body> </html> [/code] -
Its not hard as u think. just have to keep track of previous Y-m-d and the one currently being operated on
-
thank you page to redirect to different urls
laffin replied to andycharrington's topic in PHP Coding Help
U have to modify your forms. there is a method of doing what u are asking however, it relies on the browser to send the information which may be faked or a browser may not send. your best bet is to use sessions. and store the last page that a user is on. except on the thank you page, which can use this variable to return the user to the last page -
great, other optimizations ya may want to try and improve is the main for loop which goes character by character until it finds a control chracter. ya can prolly use strpos, to find the next control character, than process a chunk of the text before. than adjust the pointer. once ya start getting it slimmed down it shud be a lot more manageable
-
what's the purpose of the % in strtotime? this wud result as '%01' which when converted to integer will take the '%' and return a 0
-
When I went over the code, I do get a lot of what's going on. and preg_match, may just overcomplicate the code u have already. but we can still change some things around <?php // attribute flags... define('AT_ITALICS',1); define('AT_BOLD',2); define('AT_UNDERLINE',4); define('AT_THROUGH',; define('AT_OVERLINE',16); define('AT_BLINK',32); define('AT_SUPERS',64); define('AT_SUBS',128); define('AT_FONTCHANGE',256); define('AT_FCOLORCHANGE',512); define('AT_BCOLORCHANGE',1024); define('AT_FONTSIZECHANGE',2048); define('AT_PARAGRAGH',4096); define('AT_CHANGE',8192); function at_reset(&$at) { $at = array( 'flags' => 0, 'font' => 0, 'fcolor' => 'black', 'bcolor' => 'white', 'fontsize' => 3, 'changecnt' => 0, ); } function process_flags(&$at) { $fonts = array( "", "font-family: serif; white-space: normal; ", "font-family: monospace; white-space: normal; ", "font-family: monospace; white-space: pre; ", "font-family: fantasy; white-space: normal; ", "font-family: cursive; white-space: normal; " ); // font sizes 0-6 $fontsizes = array( 'xx-small','x-small','small','medium','large','x-large','xx-large' ); $out=''; if ($at['flags']&AT_CHANGE) { $out = "<span style='". (($at['flags']&AT_ITALICS)?"font-style: italic; ":''). (($at['flags']&AT_BOLD)?"font-weight: bold; ":''). (($at['flags']&AT_SUPERS)?"vertical-align: top; font-size: 50%; ":''). (($at['flags']&AT_SUBS)?"vertical-align: bottom; font-size: 50%; ":''). (($at['flags']& (AT_UNDERLINE | AT_THROUGH | AT_OVERLINE | AT_BLINK))?("text-decoration: ". (($at['flags'] & AT_UNDERLINE)?"underline ":''). (($at['flags'] & AT_THROUGH)?"line-through ":''). (($at['flags'] & AT_OVERLINE)?"overline ":''). (($at['flags'] & AT_BLINK)?"blink ":''). "; "):''). (($at['flags']&AT_FONTCHANGE)?$fonts[$at['font']]:''). (($at['flags']&AT_FONTSIZECHANGE)?("font-size: ".$fontsizes[$at['fontsize']]."; "):''). ((($at['flags']&AT_FCOLORCHANGE)&&(!empty($at['fcolor'])))?("color: $at[fcolor]; "):''). ((($at['flags']&AT_BCOLORCHANGE)&&(!empty($at['bcolor'])))?("background-color: $at[bcolor]; "):''). "'>"; $at['flags'] ^= (AT_CHANGE | AT_FCOLORCHANGE | AT_BCOLORCHANGE | AT_FONTSIZECHANGE | AT_FONTCHANGE); $at['changecnt']++; } return $out; } function str_textcodes($strin) { // dos colors to html colors $d2h_colors = array( '#000','#a00','#0a0','#a50','#00a','#a0a','#0aa','#aaa', '#555','#f55','#5f5','#ff5','#55f','#f5f','#5ff','#fff' ); $justification = array( "left", "right", "justify", "center", "left; text-indent: 32px", "right; text-indent: 32px", "justify; text-indent: 32px", "center; text-indent: 32px", "left; padding-left: 32px"); $specialchars= array('2591','2592','2593','2588','2580','2584','258c','2590','250c','252c','2510', '251c','253c','2524','2514','2534','2518','2502','2500',); at_reset($at); $out=''; $slen=strlen($strin); for($cpos=0;$cpos<$slen;$cpos++) { if($strin[$cpos]=='^') { switch($strin[++$cpos]) { case '0': while($at['changecnt']--) $out .= "</span>"; at_reset($at); break; case 'i': $at['flags'] |= AT_ITALICS | AT_CHANGE; break; case 'e': $at['flags'] |= AT_BOLD | AT_CHANGE; break; case 'u': $at['flags'] |= AT_UNDERLINE | AT_CHANGE; break; case 't': $at['flags'] |= AT_THROUGH | AT_CHANGE; break; case 'o': $at['flags'] |= AT_OVERLINE | AT_CHANGE; break; case 'x': $at['flags'] |= AT_BLINK | AT_CHANGE; break; case 'f': (($fn=strpos('pmcfs',$strin[++$cpos]))!==FALSE) && $at['font']=$fn+1; ($fn!==FALSE) && $at['flags'] |= AT_FONTCHANGE | AT_CHANGE; break; case '~': $at['flags'] |= AT_SUPERS | AT_CHANGE; break; case '_': $at['flags'] |= AT_SUBS | AT_CHANGE; break; case 'r': $at['fcolor']^=$at['bcolor']; $at['bcolor']^=$at['fcolor']; $at['fcolor']^=$at['bcolor']; $at['flags'] |= AT_FCOLORCHANGE | AT_BCOLORCHANGE | AT_CHANGE; break; case 'c': $at['fcolor'] = $d2h_colors[hexdec($strin[++$cpos])]; $at['flags'] |= AT_FCOLORCHANGE | AT_CHANGE; break; case 'b': $at['bcolor'] = $d2h_colors[hexdec($strin[++$cpos])]; $at['flags'] |= AT_BCOLORCHANGE | AT_CHANGE; break; case 'C': $at['fcolor']='#'.strtoupper(substr($strin, ++$cpos, 6)); $cpos+=5; $at['flags'] |= AT_FCOLORCHANGE | AT_CHANGE; break; case 'B': $at['bcolor']='#'.strtoupper(substr($strin, ++$cpos, 6)); $cpos+=5; $at['flags'] |= AT_BCOLORCHANGE | AT_CHANGE; break; case 's': $s = $strin[++$cpos]; $at['fontsize'] = (($s >= '0') && ($s <= '6'))?intval($s):3; $at['flags'] |= AT_FONTSIZECHANGE | AT_CHANGE; break; case 'p': $out .= (($at['flags'] & AT_PARAGRAGH)?'</P>':'') . '<P>'; $at['flags'] |= AT_PARAGRAGH; break; case 'P': $out .= (($at['flags'] & AT_PARAGRAGH)?'</P>':''); $at['flags'] |= AT_PARAGRAGH; $out .='<P'. ((($fn=strpos('lrjcLRJCi',$strin[$cpos+1]))!==FALSE)?(" style='text-align: $justification[$fn];'"):'') .'>'; ($fn) && $cpos++; break; case 'n': $out .= "<br>"; break; case 'g': $out .= process_flags($at); $out.=(($fn=strpos('0123tblrqweasdzxcvh',$strin[$cpos+1]))!==FALSE)?" &#x$specialchars[$fn];":' '; if($fn!==FALSE) $cpos++; break; case '^': $out.='^'; break; } } else { $out .= process_flags($at) . $strin[$cpos]; } } while($at['chnagecnt']--) $out .= "</span>"; if ($at['flags']&AT_PARAGRAGH) $out .= '</P>'; return $out; } $str = <<<EOS ^e^c1^s5TEXT CODES:^0 can be used in text messages, articles, and bulletins posted by users on the BBS. ^p^i^uRESET^0^n ^fm^^0^0 = Reset all attribute changes; clean slate; everything reset to defaults... ^p^i^uATTRIBUTES^0^n ^fm^^e^0 = ^eBold^0^n ^fm^^i^0 = ^iItalics^0^n ^fm^^u^0 = ^uUnderline^0^n ^fm^^t^0 = ^tStrike through^0^n ^fm^^o^0 = ^oOver-line^0^n ^fm^^x^0 = ^xBlink^0 (not supported on all browsers) ^p^i^uSCIENTIFIC^0^n ^fm^^~^0 = ^~Superscript^0 (These two are mutually exclusive)^n ^fm^^_^0 = ^_Subscript^0 ^p^i^uTYPE-FACES^0^n ^fm^^f^in^0 = Type-face font (dependent on browser) where ^i^fmn^0 = ^n ^Pi^fmp^0 ^fpproportional^0^n ^fmm^0 ^fmmonospaced^0^n ^fcc^0 ^fccode^0^n ^fcf^0 ^fffantasy^0^n ^fcs^0 ^fsscript^0^n ^p^i^uTYPE-FACE SIZING^0^n ^fm^^s#^0 = Change font size. (0=^s0smallest^0, 3=^s3normal^0 6=^s6largest^0) ^p^i^uCOLORING^0^n ^fm^^r^0 = ^rreverse^0. (swap foreground and background colors)^n ^fm^^C^i######^0 = change foreground color with hex (rrggbb) color.^n ^fm^^B^i######^0 = change background color with hex (rrggbb) color.^n ^fm^^c#^0 = change foreground color with quick color. (below)^n ^fm^^b^i#^0 = change background color with quick color. (below)^n ^Pi^fm0^0 ^c0Black^0^n ^fm1^0 ^c1Red^0^n ^fm2^0 ^c2Green^0^n ^fm3^0 ^c3Brown^0^n ^fm4^0 ^c4Blue^0^n ^fm5^0 ^c5Magenta^0^n ^fm6^0 ^c6Cyan^0^n ^fm7^0 ^b0^c7Light Gray^0^n ^fm8^0 ^c8Dark Gray^0^n ^fm9^0 ^b0^c9Bright Red^0^n ^fmA^0 ^b0^caBright Green^0^n ^fmB^0 ^b0^cbYellow^0^n ^fmC^0 ^b0^ccBright Blue^0^n ^fmD^0 ^b0^cdBright Magenta^0^n ^fmE^0 ^b0^ceBright Cyan^0^n ^fmF^0 ^b0^cfWhite^0 ^p^i^uFORMATTING^0^n ^fm^^n^0 = new line^n ^fm^^p^0 = new paragraph^n ^fm^^P^ix^0 = new paragraph with justification/indentation. ^Pi^fml^0 left^n ^fmr^0 right^n ^fmc^0 center^n ^fmj^0 justify^n ^fmL^0 left w/indent^n ^fmR^0 right w/indent^n ^fmC^0 center w/indent^n ^fmJ^0 justify w/indent^n ^fmi^0 indent entire paragraph ^p^i^uSPECIAL CHARACTERS^0^n ^fm^^^^^0 = ^^^n ^fm^^g^in^0 = graphic character. (not supported on all browsers) Where ^fm^in^0 =^n ^Pi^fm0^0 ^g0^n ^fm1^0 ^g1^n ^fm2^0 ^g2^n ^fm3^0 ^g3^n ^fmt^0 ^gt^n ^fmb^0 ^gb^n ^fml^0 ^gl^n ^fmr^0 ^gr^n ^fmq^0 ^gq^n ^fmw^0 ^gw^n ^fme^0 ^ge^n ^fma^0 ^ga^n ^fms^0 ^gs^n ^fmd^0 ^gd^n ^fmz^0 ^gz^n ^fmx^0 ^gx^n ^fmc^0 ^gc^n ^fmv^0 ^gv^n ^fmh^0 ^gh^n ^p ^fp^i^eThe above was generated with...^0^n EOS; echo str_textcodes($str).'<br><br>'; echo '<pre><code>'.$str.'</code></pre>'; ?> i moved some 2 pieces of code into their own functions, cutting the code significantly. and opted for a strpos on the 2ndary code of some functions. using arrays for matching Which Cut out a lot of Code changed the way the flags were being done, using bits instead of different variables, which cut out even more code. Not shure on performance, ya may have to loop it 100-500 times to get it profiled.
-
there also is a class called geoip. T
-
One thing that I liked about php, moving from C to php was that much easier because the similarities. I used to work on several BBS's in the early 90's as well, I was doing a lot of mods for 4 different sites (WWIV). the code does look interersting, i'll take a look later on today.
-
Holy Cow, that looks like similar code to the old modem BBS codes. ya can do lots of optimizing, lots of switches. A lot of the switch statements can be replaced with preg_replace statements, regex may be difficult to get started with, but once ya get started, it makes things like search/replace and pattern searching a whole lot easier.
-
Online Registration Restricted to Users only NOT WORKING
laffin replied to skiingguru1611's topic in PHP Coding Help
oops shud be } else { sumtimes brain dun catch errors created by fingers