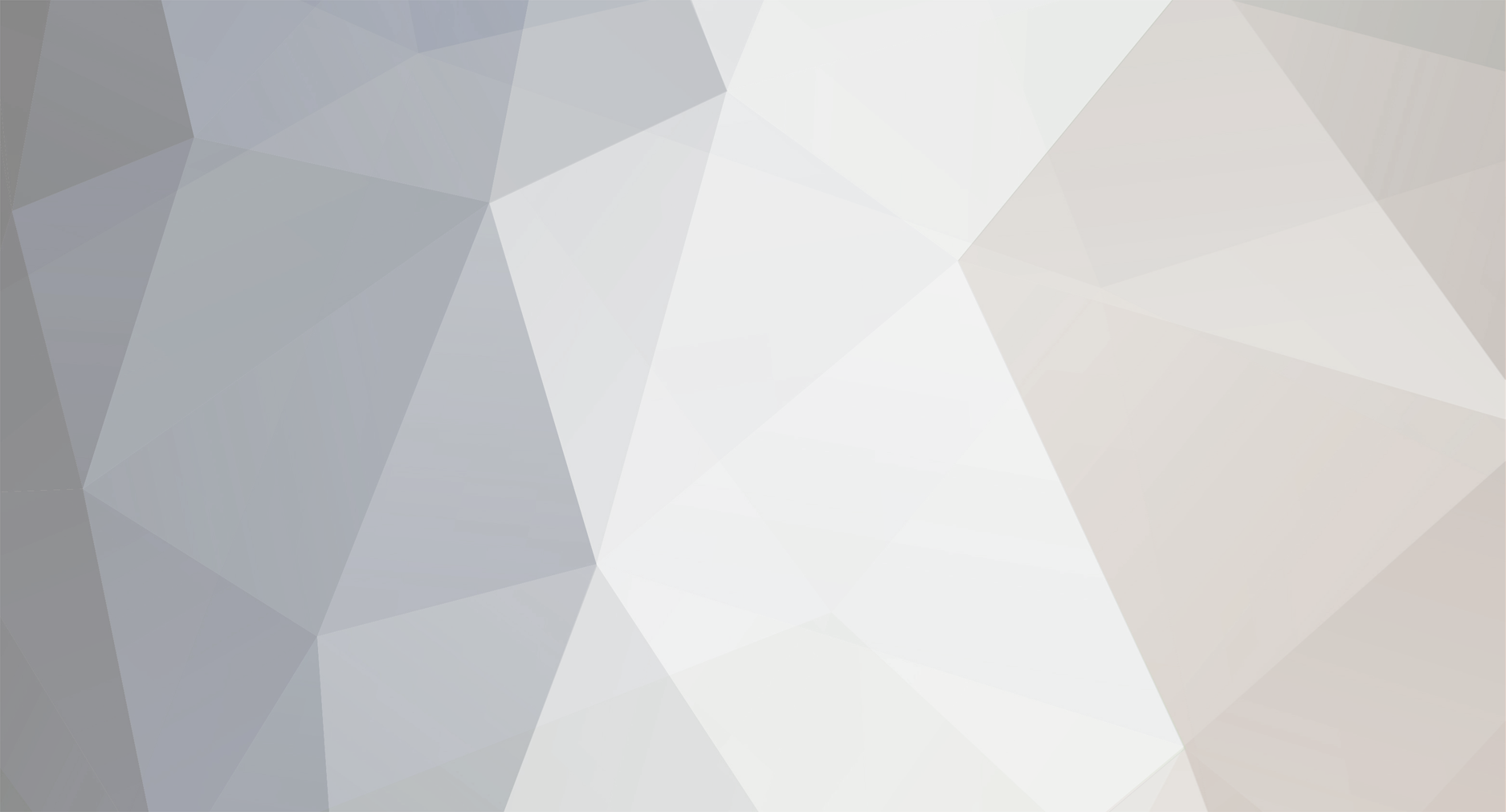
poleposters
Members-
Posts
244 -
Joined
-
Last visited
Never
Everything posted by poleposters
-
Its late and I'm tired, so this is a very lazy answer. Below is my upload/resize script. The resize script is a little different from yours but if you study the script you might be able to work out how to put yours together with an upload. I hope it helps. <?php require_once ('config.inc.php'); include ('header.html'); include "mysql_connect.php"; $couponid=$_GET['couponid']; if(!isset($_SESSION['first_name'])){ print "You must be logged in to add a coupon";} else{ if(isset($_POST['Submit'])){ $size = 100; // the thumbnail height $filedir = 'pics/'; // the directory for the original image $thumbdir = 'pics/'; // the directory for the thumbnail image $prefix = 'small_'; // the prefix to be added to the original name $maxfile = '2000000'; $mode = '0666'; $userfile_name = $_FILES['image']['name']; $userfile_tmp = $_FILES['image']['tmp_name']; $userfile_size = $_FILES['image']['size']; $userfile_type = $_FILES['image']['type']; $userfile_error=$_FILES['image']['error']; $business_id=$_SESSION['business_id']; $business_name=$_POST['business_name']; $line_one=$_POST['line_one']; $line_two=$_POST['line_two']; $address=$_POST['address']; $phone=$_POST['phone']; $cat_id=$_POST['cat_id']; $suburb=$_POST['suburb']; $postcode=$_POST['postcode']; if($userfile_name) { $prod_img = $filedir.$userfile_name; $prod_img_thumb = $thumbdir.$prefix.$userfile_name; move_uploaded_file($userfile_tmp, $prod_img); chmod ($prod_img, octdec($mode)); $sizes = getimagesize($prod_img); $aspect_ratio = 4/3; if ($sizes[1] <= $size) { $new_width = $sizes[0]; $new_height = $sizes[1]; } else { $new_height = $size; $new_width = abs($new_height*$aspect_ratio); } $destimg=ImageCreateTrueColor($new_width,$new_height) or die('Problem In Creating image'); $srcimg=ImageCreateFromJPEG($prod_img) or die('Problem In opening Source Image'); if(function_exists('imagecopyresampled')) { imagecopyresampled($destimg,$srcimg,0,0,0,0,$new_width,$new_height,ImageSX($srcimg),ImageSY($srcimg)) or die('Problem In resizing'); } else { Imagecopyresized($destimg,$srcimg,0,0,0,0,$new_width,$new_height,ImageSX($srcimg),ImageSY($srcimg)) or die('Problem In resizing'); } ImageJPEG($destimg,$prod_img_thumb,90) or die('Problem In saving'); imagedestroy($destimg); $coupon_image=$prod_img_thumb; } else { $coupon_image="one.gif"; } $query="UPDATE coupon SET cat_id=$cat_id, coupon_image=$coupon_image,business_name=$business_name,line_one=$line_one,line_two=$line_two,address=$address,suburb=$suburb,postcode=$postcode,phone=$phone,date_added=NOW() WHERE coupon_id=$couponid"; $result = mysql_query ($query) or trigger_error("Query: $query\n<br />MySQL Error: " . mysql_error()); } else{ $query="SELECT * FROM coupon WHERE coupon_id=$couponid"; $result=mysql_query($query); $selectlinks=mysql_fetch_array($result); $businessname=$selectlinks['business_name']; $lineone=$selectlinks['line_one']; $linetwo=$selectlinks['line_two']; $address=$selectlinks['address']; $phone=$selectlinks['phone']; $postcode=$selectlinks['postcode']; $suburb=$selectlinks['suburb']; $catid=$selectlinks['cat_id']; echo " <form method='POST' action='edit_coupon.php?couponid=$couponid' enctype='multipart/form-data'> <table id='quote'> <tr> <td class='label' colspan='3'><label for='business_name'>Business Name</label></td> <td class='field'colspan='2'><input type='text' name='business_name' id='business_name' tabindex='1' value='$businessname' /></td> </tr> <tr> <td class='label' colspan='3'><label for='line_one'>Line One</label></td> <td class='field' colspan='2'><input type='text' name='line_one' id='line_one' tabindex='1' value='$lineone' /></td> </tr> <tr> <td class='label' colspan='3'><label for='line_two'>Line Two</label></td> <td class='field' colspan='2'><input type='text' name='line_two' id='line_two' tabindex='1' value='$linetwo' /></td> </tr> <tr> <td class='label' colspan='3'><label for='address'>Address</label></td> <td class='field' colspan='2'><input type='text' name='address' id='address' tabindex='1' value='$address'/></td> </tr> <tr> <td class='label' colspan='3'><label for='phone'>Phone</label></td> <td class='field' colspan='2'><input type='text' name='phone' id='phone' tabindex='1' value='$phone' /></td> </tr> <tr> <td class='label' colspan='3'><label for='postcode'>Postcode</label></td> <td class='field' colspan='2'><input type='text' name='postcode' id='postcode' tabindex='1' value=' $postcode' /></td> </tr> <tr> <td class='label' colspan='3'><label for='suburb'>Suburb</label></td> <td class='field' colspan='2'><input type='text' name='suburb' id='suburb' tabindex='1' value= '$suburb' /></td> </tr> <tr> <td class='label' colspan='3'><label for='cat_id'>Category</label></td> <td class='field' colspan='2'> <select name='cat_id' id='cat_id' tabindex='8'> <option value=''>-- Select ---</option> <option value='1' >Food</option> <option value='2' >Fashion</option> <option value='3' >Entertainment</option> </select></td> </tr> <tr> <td ><input type='file' name='image'></td> </tr> <tr> <td ><input type='Submit' name='Submit' value='Submit'></td> </tr> </table> </form>"; } } ?>
-
Heya, I needed something similar for a site I'm building. Just to make sure I understand, You want to use a default image wherever an image is not specified? I took care of this in my register script. The user would enter their info, If they did not choose to upload an image, the location of a default image would be inserted into the database instead. If you can be more specific about what you wanna achieve I might be able to help with some ideas.
-
Hi all, I have this really handy image resize and upload script. It works great,but i'd like to modify it to handle multiple uploads on the one form. Any ideas? <?php require_once ('config.inc.php'); include ('header.html'); include "mysql_connect.php"; if(!isset($_SESSION['first_name'])){ print "You must be logged in to add a slideshow";} else{ if(isset($_POST['Submit'])){ $size = 240; // the thumbnail height $filedir = 'slide/'; // the directory for the original image $thumbdir = 'slide/'; // the directory for the thumbnail image $prefix = 'slide_'; // the prefix to be added to the original name $maxfile = '2000000'; $mode = '0666'; $userfile_name = $_FILES['image']['name']; $userfile_tmp = $_FILES['image']['tmp_name']; $userfile_size = $_FILES['image']['size']; $userfile_type = $_FILES['image']['type']; $userfile_error=$_FILES['image']['error']; $business_id=$_SESSION['business_id']; if($userfile_name) { $prod_img = $filedir.$userfile_name; $prod_img_thumb = $thumbdir.$prefix.$userfile_name; move_uploaded_file($userfile_tmp, $prod_img); chmod ($prod_img, octdec($mode)); $sizes = getimagesize($prod_img); $aspect_ratio = 4/3; if ($sizes[1] <= $size) { $new_width = $sizes[0]; $new_height = $sizes[1]; } else { $new_height = $size; $new_width = abs($new_height*$aspect_ratio); } $destimg=ImageCreateTrueColor($new_width,$new_height) or die('Problem In Creating image'); $srcimg=ImageCreateFromJPEG($prod_img) or die('Problem In opening Source Image'); if(function_exists('imagecopyresampled')) { imagecopyresampled($destimg,$srcimg,0,0,0,0,$new_width,$new_height,ImageSX($srcimg),ImageSY($srcimg)) or die('Problem In resizing'); } else { Imagecopyresized($destimg,$srcimg,0,0,0,0,$new_width,$new_height,ImageSX($srcimg),ImageSY($srcimg)) or die('Problem In resizing'); } ImageJPEG($destimg,$prod_img_thumb,90) or die('Problem In saving'); imagedestroy($destimg); $slide=$prod_img_thumb; } else { $coupon_image="one.gif"; } $query="INSERT INTO slideshow(slide_id,business_id,slide) VALUES ('','$business_id','$slide')"; $result = mysql_query ($query) or trigger_error("Query: $query\n<br />MySQL Error: " . mysql_error()); } else{ echo ' <form method="POST" action="'.$_SERVER['PHP_SELF'].'" enctype="multipart/form-data"> <table id="quote"> <td ><input type="file" name="image"></td> </tr> <tr> <td ><input type="Submit" name="Submit" value="Submit"></td> </tr> </table> </form>'; } } ?>
-
Information only viewable if criteria from database meet.
poleposters replied to KurveMedia's topic in PHP Coding Help
Hey Mike, Not sure if you've posted the right bit of code.I'm only quite new to PHP.I can see that you've selected the listing data and then put it into an array.But I don't think you show the code that actually prints the listing. What you need to do is make sure that the query also pulls out the data from the paid_listing column, so that when you print the listings you can print the extra info for the paid listing. e.g print "yourarrayname[business_name];" print "yourarrayname[phone_number];" print "yourarrayname[address];" if(yourarrayname[paid listing]==1) print"yourarrayname[linktogooglemap];" This way the premium listing link to googlemaps only appears if the paid_listing field for that business is ==1. -
It could be because you've left out the curly braces on your if conditional. if ($b == 0) { $message = "You have entered the wrong confirmation code."; }
-
comparing checked values to record fields
poleposters replied to glennn.php's topic in PHP Coding Help
Hey I have a hunch that it might be your WHERE syntax. Can you clarify your question.Its a little hard to understand -
Are you sure you have the right number of divs? Try adding a </div> after the while loop.
-
[SOLVED] how do I redirect a page back to a dynamic url?
poleposters replied to poleposters's topic in PHP Coding Help
Fantastic. Thanks heaps! -
Hi Stuart. Just to make sure. Do you mean the text breaks out of the text area box when your user enters the decription? Or do you mean it breaks out of the page when you view the preview? If the problem is when you view the preview, then itsa problem with your HTML/CSS. Try changing your code to post the description within the div class="des". That way the description is bounded by the width/height you specify in your CSS file. <code> this is the preview page <div class="des">description . . . <?=stripslashes($_POST['des'])?></div> I hope it works. </code>
-
Information only viewable if criteria from database meet.
poleposters replied to KurveMedia's topic in PHP Coding Help
Hi Mike My approach is to add a column in your database called paid_listing and assign it a value either '0' or '1'. '0' for unpaid listings and '1' for paid listings. Then all you need to do is run a query that tests the value of this field. ie if paid listing==1 print "link to google maps" I could be more specific if you posted some of your code. Hope it helps. Matt -
Hi, Can anyone let me know if this is possible? My site displays several pages of listings. But on the right hand side of the page I have a newsletter signup form. If someone decides to signup while theyre browsing, I want them to return automatically to the page they were viewing. For example after the newsletter script runs I'd like them to return to the URL http://localhost/searchcat.php?catid=1&searchterm=2000&start=10 which is the second page of results with the category id '1' and the search term '2000'. Any clues? Cheers
-
I'm creating a business directory. I have two tables I need to use for a query. One is called coupon and contains all the info for the listing. ie, business name, phone, blurb etc. The other is the user info which includes the registrants details and a column called paid_listing. This is set as zero by default however changes to "1" when the listing is paid. At the moment I have a query which displays the listing aswell as a button to view the business profile.I want to make the business profile as part of the paid listing. Basically I just need to run a condition where if paid_listing>0 the button is displayed.My problem is that the paid_listing column is in the user table not the coupon table. I know I need to perform a join. The code below is the script I have now without the conditional for the view profile. $selectlinks="SELECT * from coupon WHERE postcode like '%$searchterm' and cat_id=$catid or suburb like '%$searchterm' and cat_id=$catid order by business_name DESC limit $start,$numentries"; $selectlinks2=mysql_query($selectlinks) or die(mysql_error()); $numlinks=mysql_num_rows($selectlinks2); if($numlinks>0) { while($selectlinks3=mysql_fetch_array($selectlinks2)) { $selectlinks3['line_one']=htmlspecialchars($selectlinks3['line_one']); $selectlinks3['line_two']=htmlspecialchars($selectlinks3['line_two']); $selectlinks3['business_name']=htmlspecialchars($selectlinks3['business_name']); $selectlinks3['phone']=htmlspecialchars($selectlinks3['phone']); print "<div id='row'>"; print "<div id='coupon'>"; print "<div id='img'><img src='$selectlinks3[coupon_image]'></div>"; print "<div id='main'>"; print "<h2>$selectlinks3[line_one]</h2>"; print "<h2>$selectlinks3[line_two]</h2>"; print "<h3>$selectlinks3[business_name]</h3>"; print "<h4>$selectlinks3[phone]</h4>"; print "</div>"; print "</div>"; print "<div id='profile'>"; print "<a href='profile.php?businessid=$selectlinks3[business_id]'><img src='view.gif'></a><br/>"; print "<a href='#'><img src='print.gif'></a><br/>"; print "<a href='#'><img src='send.gif'></a><br/>"; print "</div>"; print "</div>"; } This script works great.But to retrieve the paid_listing data I need to join the users table with the coupon table. This is what I've tried $selectlinks="SELECT * from coupon,users WHERE coupon.business_id=users.business_id AND postcode like '%$searchterm' and cat_id=$catid or suburb like '%$searchterm' and cat_id=$catid order by business_name DESC limit $start,$numentries"; $selectlinks2=mysql_query($selectlinks) or die(mysql_error()); $numlinks=mysql_num_rows($selectlinks2); if($numlinks>0) { while($selectlinks3=mysql_fetch_array($selectlinks2)) { $selectlinks3['line_one']=htmlspecialchars($selectlinks3['line_one']); $selectlinks3['line_two']=htmlspecialchars($selectlinks3['line_two']); $selectlinks3['business_name']=htmlspecialchars($selectlinks3['business_name']); $selectlinks3['phone']=htmlspecialchars($selectlinks3['phone']); print "<div id='row'>"; print "<div id='coupon'>"; print "<div id='img'><img src='$selectlinks3[coupon_image]'></div>"; print "<div id='main'>"; print "<h2>$selectlinks3[line_one]</h2>"; print "<h2>$selectlinks3[line_two]</h2>"; print "<h3>$selectlinks3[business_name]</h3>"; print "<h4>$selectlinks3[phone]</h4>"; print "</div>"; print "</div>"; print "<div id='profile'>"; if ($selectlinks3['phone']>0) { print "<a href='profile.php?businessid=$selectlinks3[business_id]'><img src='view.gif'></a><br/>"; } print "<a href='#'><img src='print.gif'></a><br/>"; print "<a href='#'><img src='send.gif'></a><br/>"; print "</div>"; print "</div>"; } The problem is when I run the script, I get the same listing appearing mulitple times if I search by "suburb". However if I type in the postcode(zip), the listings appear fine. Which leads me to believe that the problem is with the conditionals attached to my query. $selectlinks="SELECT * from coupon,users WHERE coupon.business_id=users.business_id AND postcode like '%$searchterm' and cat_id=$catid or suburb like '%$searchterm' and cat_id=$catid order by business_name DESC limit $start,$numentries"; Can anyone help with the syntax? Cheers,
-
Hey dutchboy. I've got the same problem.I know this is an old post, so if you work it out can you please post the solution. I'm going to keep looking. If i find anything I'll post back. Cheers.
-
Thanks cooldude. That sorted the problem, Cheers.
-
Hi, I have s php slideshow script which I'm trying to modify so that all the slide URLs are pulled from a database instead of manually coded. Here is the original script. <?php //include slideshow.php in your script include "slideshow.php"; //add 3 slides $slideshow[ 'slide' ][ 0 ] = array ( 'url' => "images/plant0.jpg" ); $slideshow[ 'slide' ][ 1 ] = array ( 'url' => "images/plant1.jpg" ); $slideshow[ 'slide' ][ 2 ] = array ( 'url' => "images/plant2.jpg" ); //send the slideshow data Send_Slideshow_Data ( $slideshow ); ?> My approach has been to grab the data from the database and store it into an array then run a loop. As below. $query = 'SELECT slide FROM slideshow WHERE business_id=2 '; $result = mysql_query ($query); $numlinks=mysql_num_rows($result); while($row = mysql_fetch_array ($result)){ $slideshow[ 'slide' ][ 0 ] = array ( 'url' => "$row[0]" ); When I run this query I only get one result? What am I doing wrong?
-
Thanks cmgmyr, The script is the best example I've seen using lat/long. But the only data I can obtain cheaply for Australian zipcodes is using the table above with the search zip, number of adjoining zips and the other adjoining zips. The main problem I'm having with understanding how to set up the queries is that some zipcodes have three neighbours while other have anything up to ten neighbours, which means I'm going to have blank fields in my table. Any ideas on how to set up the tables?
-
Hi, I'm building a business directory and want to include a search function so that when a zip code is entered results are returned for the zipcode and all the neighbouring zip codes. I know its possible to use latitude and longtitude to create a radius search, however I only want to return results from zip codes which share a common border with the input zip. I have been supplied some sample zipcode data from a mapping company. The full database is quite expensive, so I'd like to make sure I can actually use the information before I shell out for it. Below are a few rows of sample data. The first field is the zip code, the second is the number of adjoining zip codes and the remainder is the neighbouring zip codes 2000,5,2007,2008,2009,2010,2011 2006,4,2008,2037,2042,2050 2007,4,2000,2008,2009,2037 2008,8,2000,2006,2007,2010,2016,2037,2042,2043 2009,3,2000,2007,2037 2010,6,2000,2008,2011,2016,2021,2027 I'm pretty lost on this one. If anyone has any ideas on how to setup the tables and perform the queries that would be appreciated. Thanks.
-
I am creating a form to add information to a business directory. The script displays the business name, a two line description, an address, phone ,category and a profile image. The script works great. Except I want the profile image upload to be optional. If i submit the form without uploading an image I get an error message. I've tried writing the form with various if-else conditionals but they keep coming up with the wrong result. Any ideas. Thanks. <?php if(isset($_POST['Submit'])) { $business_name=$_POST['business_name']; $line_one=$_POST['line_one']; $line_two=$_POST['line_two']; $address=$_POST['address']; $phone=$_POST['phone']; $cat_id=$_POST['cat_id']; $size = 150; // the thumbnail height $filedir = 'pics/'; // the directory for the original image $thumbdir = 'pics/'; // the directory for the thumbnail image $prefix = 'small_'; // the prefix to be added to the original name $maxfile = '2000000'; $mode = '0666'; $userfile_name = $_FILES['image']['name']; $userfile_tmp = $_FILES['image']['tmp_name']; $userfile_size = $_FILES['image']['size']; $userfile_type = $_FILES['image']['type']; $prod_img = $filedir.$userfile_name; $prod_img_thumb = $thumbdir.$prefix.$userfile_name; move_uploaded_file($userfile_tmp, $prod_img); chmod ($prod_img, octdec($mode)); $sizes = getimagesize($prod_img); $aspect_ratio = $sizes[1]/$sizes[0]; if ($sizes[1] <= $size) { $new_width = $sizes[0]; $new_height = $sizes[1]; }else{ $new_height = $size; $new_width = abs($new_height/$aspect_ratio); } $destimg=ImageCreateTrueColor($new_width,$new_height) or die('Problem In Creating image'); $srcimg=ImageCreateFromJPEG($prod_img) or die('Problem In opening Source Image'); if(function_exists('imagecopyresampled')) { imagecopyresampled($destimg,$srcimg,0,0,0,0,$new_width,$new_height,ImageSX($srcimg),ImageSY($srcimg)) or die('Problem In resizing'); }else{ Imagecopyresized($destimg,$srcimg,0,0,0,0,$new_width,$new_height,ImageSX($srcimg),ImageSY($srcimg)) or die('Problem In resizing'); } ImageJPEG($destimg,$prod_img_thumb,90) or die('Problem In saving'); imagedestroy($destimg); $coupon_image=$prod_img_thumb; echo ' <a href="'.$prod_img.'"> <img src="'.$prod_img_thumb.'" width="'.$new_width.'" heigt="'.$new_height.'"> </a>'; echo $business_name; echo $line_one; echo $line_two; echo $address; echo $phone; echo $coupon_image; echo $cat_id; }else{// display upload form
-
Hi all, I'm new to php and this has me stumped. I'm building a business directory and want my users to be able to enter their zip code or suburb into a text input and then select a category to retrieve rows from my database that match both the zip/suburb and the category. I know how to create a submit button for the zip/suburb and the list of category IDs but I want to be able to do it with the one click. ie the category link contains infromation for both the category ID and the submit for the zip/suburb. Any help is greatly appreciated.