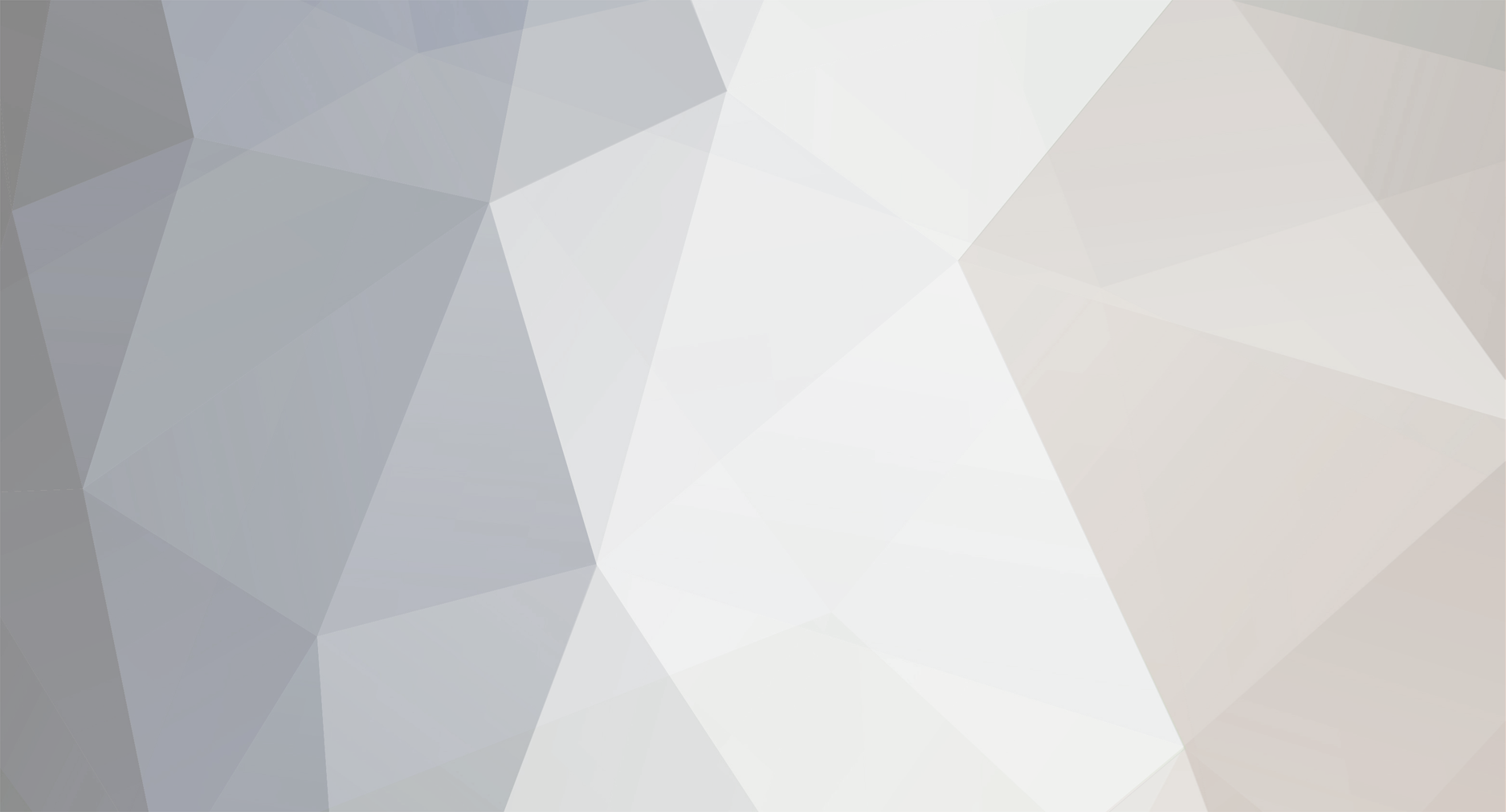
ucffool
Members-
Posts
100 -
Joined
-
Last visited
Everything posted by ucffool
-
You would have an .htaccess file in the document root with something like: Options +FollowSymLinks RewriteEngine on # Change all URLs that contain letters and numbers only to TheTarget.php RewriteRule ([0-9a-z]+)$ $1.php [QSA,L] Note, I haven't tested the above code, but it should work (or I'm sure someone else will correct me)
-
Assuming he is looking for 1 number only. I realize that's the way rand() will work, I was just getting the bigger concept across. Either way, I suppose it is good someone clarified. I would recommend mt_rand() or mt_rand(0,9) instead.
-
Security concern using while(list($key,$value)=each($_REQUEST))
ucffool replied to lalomarquez's topic in PHP Coding Help
It's amazing what a fat finger will do sometimes Fixed, thanks. -
Security concern using while(list($key,$value)=each($_REQUEST))
ucffool replied to lalomarquez's topic in PHP Coding Help
I had that feeling too until I had to write a book about it (in a way someone else wouldn't take an hour to figure out how it works), so don't feel bad. Check my sig, there is a free PDF version of my book and you can check out the sprintf() function explanation (takes 3 pages) if you want. If not, that's fine too. -
That will tell him the number of rows that have at least 1 NULL column, but I think he also needs, "to count how many of these 4 columns are empty with no value?" I would use array_count_value() to get the number of NULL values in each row. $null_count = array(); $result = mysql_query("SELECT * FROM table WHERE slot_id1 = '' || slot_id2 = '' || slot_id3 = '' || slot_id4 = '' "); while ($row = mysql_fetch_assoc($result)){ $count_array = array_count_values($row); $null_count[] = $count_array['']; } The key or problem here is that array_count_values() can only count integers or strings ( because it makes the value the KEY in the array), and thus if it is an empty string ('') it can count it, but if it is NULL, it will error out.
-
I think what you want is one string that contains the output of rand() run 20 times? $string = ''; for ($x=0; $x<20; $x++){ $string .= rand(); // concatenate onto string the result of rand() } echo $string;
-
can you put the rewrite rules in an .htaccess file in the directory in question?
-
Security concern using while(list($key,$value)=each($_REQUEST))
ucffool replied to lalomarquez's topic in PHP Coding Help
You can use sprintf() or regular expressions to clean up stuff as well, the rule of thumb is if you have user input, DO NOT trust it. anyone can POST or GET to one of your pages, scrub it for what you expect. Always specify what you EXPECT, not what to exclude (a losing battle). -
If you use array_values() it will return a new array with the same values from your original array, but reindexed as you would expect: <?php $number[0] = 'zero'; $number[1] = 'one'; $number[2] = 'two'; $number[20] = 'twenty'; $number[30] = 'thirty'; $number[40] = 'fourty'; $newarray = array_values($number); print_r($newarray); ?> Array { [0] => zero; [1] => one; [2] => two; [3] => twenty; [4] => thirty; [5] => fourty; }
-
# mysqli_execute — Alias for mysqli_stmt_execute Deprecated usually means it was replaced with something else and may be removed in future versions. Another example is mysql_db_query(): 4.0.6 This function is deprecated, do not use this function. Use mysql_select_db() and mysql_query() instead.
-
how can i count number of lines in all of my php scripts
ucffool replied to jasonc's topic in PHP Coding Help
Or a combination of scandir(), file_get_contents(), and count(). -
need help answers disappear after i click submit.
ucffool replied to cyrixware's topic in PHP Coding Help
So basically you want to make sure that they have answered every question before they can click submit? Sounds like javascript. If you want to do it with PHP, you could evaluate all submitted answers on the processing page, and if they left something out, send back to the original question page through GET the values they already submitted, and on the original page, check for these values and then process the questions appropriately (say for instance, placing a SELECTED or selected='selected' in an <option>) -
Remember, for every: <tr></tr> it should have 2 <td></td>, one for each column. from glancing at your code, you have it only doing a single IF statement evaluated once for whether there should be a <td></td>.
-
How to transfer jpeg from remote server to my own?
ucffool replied to egaertner's topic in PHP Coding Help
<?php $url = 'http://some.domain.com/filename.jpg'; $file = file_get_contents(urlencode($url)); file_put_contents('filename.jpg',$file); ?> Try that. If that fails, probably because of this: php.ini setting allow_url_fopen -
How to transfer jpeg from remote server to my own?
ucffool replied to egaertner's topic in PHP Coding Help
<?php $url = 'http://some.domain.com/filename.jpg'; $file = file_get_contents($url); file_put_contents('filename.jpg',$file); ?> -
I think this is what you are looking for (pulled from my book, see sig): SELECT * FROM table1 INNER JOIN table2 on table1.id = table2.id // Returns all columns in table1 and table2 where the id matches // on both tables (any rows in table1 without an id that matches // is excluded from the resulting combined results) SELECT table1.* FROM table1 INNER JOIN table2 on table1.id = table2.id // Same as above, except only columns from table1 are included SELECT table1.id FROM table1 INNER JOIN table2 on table1.id = table2.id // Same as above, except only the id column from table1 is included SELECT table.id AS tableid FROM table // Give an alias to the column using AS (so id will result as tableid)
-
Check out the functions: chunk_split() explode()
-
Don't see anything wrong with it. If you are doing something that is more constructed (such as letters or numbers only), doing some extra regular expression checks will help (Also consider restricting the submitted content to a specific length to prevent a buffer overflow situation). Another opinion would also be good, I'm not an end-all-be-all.
-
While that works for str_pad(), luckily, php.net states it is expecting an integer for STR_PAD_LEFT. The only reason I mention this is that some other functions, as I learned putting a book together (see my sig in a few days, once reviews are back), they state they take an integer and they only accept the string version of the flag, and other functions accept only an integer that represents one of the flags. On a sidebar, I always forget about range, I wonder which loop is faster, the for() or foreach() with range. Oh heck, ran a test: <?php $time = microtime(1); $month = range(1,1000); foreach($month as $months){ $months=str_pad($months,2,'0',1); } $time = number_format(microtime(1)-$time, 6); echo "$time seconds to complete FOREACH with RANGE."; $time = microtime(1); for($month=1;$month<=1000;$month++){ $months=str_pad($month,2,'0',1); } $time = number_format(microtime(1)-$time, 6); echo "$time seconds to complete FOR loop."; ?> Results: 0.002322 seconds to complete FOREACH with RANGE. 0.002290 seconds to complete FOR loop. I ran it a bunch of times, and while the values varied, FOR was 95% of the time faster, about 2%-25% faster. Of course, this is less than 1/1000th of a second, and we are doing a loop of 12 values, not 1000... so it's a moot point. Just thought I would share.
-
It's called a ternary conditional. expression ? ValueIfTRUE : ValueIfFALSE; Evaluate expression, if it is true, the value after the ? and before the : is used. If it is false, the value after the : and before the ; is used. It returns either the values defined (in your case, assigning them to $prev_page).
-
Just to get you started: <HTML> <form action="search.php" method="POST"> <select name='days'> <?php for ($x=1;$x<=31;$x++){ // basic FOR loop to easily create the html echo "<option value='$x'>$x</option>"; } ?> <select name='month'> <?php for ($x=1;$x<=12;$x++){ echo "<option value='$x'>$x</option>"; } ?> <select name='year'> <?php for ($y=2007; $y <= date('Y') ;$y++){ // date('Y') returns the current 4-digit year. This will show all years from 2007 to the current year. echo "<option value='$y'>$y</option>"; } ?> <input type='submit' name='search' value='search'> </form> </HTML> <?php // search.php $dd=$_POST[days]; $dd = str_pad($dd, 2, '0', 1); // If it is less than 2 characters long, add a 0 on the left side $mm=$_POST[month]; $mm = str_pad($mm, 2, '0', 1); // If it is less than 2 characters long, add a 0 on the left side $yy=$_POST[year]; $j=$dd . "-" . $mm . "-" . $yy . ".html"; echo $j; $month = strtolower(date('M', strtotime("$yy$mm$dd 12:00:00"))); // A lot going on here // strtolower makes the results all lowercase to match your 'mar' for march // date('M', ...) returns the 3-letter form of month // strtotime() is returning the unix timestamp for date() for the submitted date, at 12 noon. $def_dir="/var/www/html/backup/$month"; ?> I've used some simpler functions here because if we used sprintf() you'd be in a corner crying (I say that because after a year, I finally figured out how to use it, otherwise I was crying). So yeah, there you go. I didn't test it, I just wrote it, but it should get you going in the right direction.
-
Should be += as you said. Past that, I am just posting because trying to follow all your loops is really hard with the current formatting.
-
no, I won't disagree that under XHTML selected="selected" is correct. It's just a simple matter of assumptions. You assumed they were using XHTML, I assumed HTML. It's just that simple. It's the OP's fault. ;-) We are both right... maybe.
-
Here is a rough idea of what you want to do (and it is most likely not a drop-in): <?php switch (change[3]){ case '1': $query = 'SELECT name FROM level_change WHERE id = 7'; break; case '2': $query = 'SELECT name FROM group_change WHERE id = 7'; break; default: // Display an error break; } $result = mysql_query($query); $change[4] = mysql_result($result, 0); ?> I hope it at least gives you the idea.
-
include('products.php'); Wherever in the page you want the contents of the page to be evaluated and included.