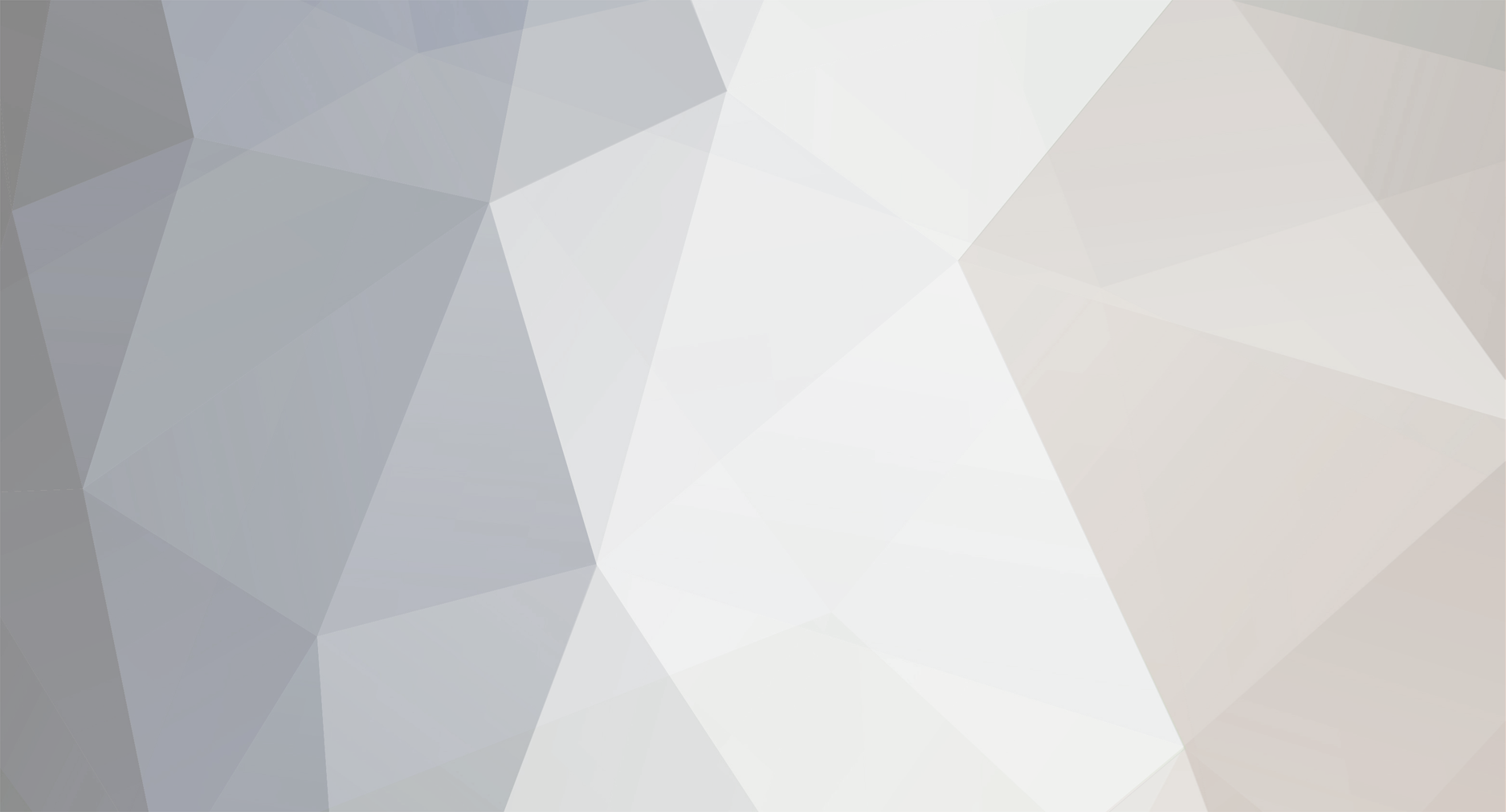
waynewex
-
Posts
2,405 -
Joined
-
Last visited
Posts posted by waynewex
-
-
I'm not getting any text error as such; its just that its not redirecting; at all. This means that its failing either on or before the first IF statement. I added the semi-colon (VERY STUPID MISTAKE) but alas, its still staying on the same page.
<?php session_start(); $user="*****"; $password="*******"; $database="********"; mysql_connect("*************" ,$user,$password); @mysql_select_db($database) or die(mysql_error); $username = $_POST[username]; $ptrying = $_POST[password]; $secured_password = sha1($ptrying); $query = ("SELECT * FROM USERS WHERE USERNAME='$username' AND PASSWORD ='$secured_password'") or die(mysql_error); $result = mysql_query($query); if (mysql_num_rows($result) > 0) { $row= mysql_fetch_array( $result ); if(($username == $row[uSERNAME]) && ($secured_password == $row[PASSWORD])) { session_register('username'); header('Location:http://*********.com/privatedata.php'); } else { header('Location:http://********.com/login.php'); } } mysql_close($conn); ?>
Thanks for trying guys.
-
Okay, my code looks like this at the moment but its still not going anywhere.
$username = $_POST[username]; $ptrying = $_POST[password]; $secured_password = sha1($ptrying); $query = ("SELECT * FROM USERS WHERE USERNAME='$username' AND PASSWORD ='$secured_password'") or die(mysql_error); $result = mysql_query($query) if (mysql_num_rows($result) > 0) { $row= mysql_fetch_array( $result ); if(($username == $row[uSERNAME]) && ($secured_password == $row[PASSWORD])) { session_register('username'); header('Location:http://******.com/privatedata.php'); echo 'its right'; } else { header('Location:http://******.com/login.php'); } }
-
Any ideas?
-
Here's the current code. Still staying on the same page and not redirecting. DB connection is okay etc.
$usertrying = $_POST[username];
$ptrying = $_POST[password];
$secured_password = sha1($ptrying);
$user_correct = false;
$pw_correct = false;
$query = "SELECT * FROM `USERS` WHERE `USERNAME`='$usertrying' AND `PASSWORD`='$secured_password'";
$result = mysql_query($query)
if (mysql_num_rows($result) > 0) {
$user_correct = true;
$pw_correct = true;
}
if(($user_correct == true) && ($pw_correct == true))
{
session_register('username');
header('Location:http://****.com/privatedata.php');
}
else
{
header('Location:http://*****.com/login.php');
}
-
Btw, I changed the $username_correct to $user_correct and the same with $pw_correct. Still the same result.
-
Okay, I've changed it a bit; but its keeping me on the page and not responding to the redirects. (Btw, thanks for the corrections)
$usertrying = $_POST[username];
$ptrying = $_POST[password];
$secured_password = sha1($ptrying);
$user_correct = false;
$pw_correct = false;
$query = "SELECT * FROM `USERS` WHERE `USERNAME`='$usertrying' AND `PASSWORD`='$secured_password'";
$result = mysql_query($query)
if (mysql_num_rows($result) > 0) {
$user_correct = true;
$pw_correct = true;
}
if(($username_correct == true) && ($password_correct == true))
{
session_register('username');
header('Location:http://*********.com/privatedata.php');
}
else
{
header('Location:http://*******.com/login.php');
}
mysql_close($conn);
-
Here's my changed code, its still returning me to the login page which means the last if statement is falling on the IF.
<?php session_start();
$user="*******";
$password="********";
$database="*********";
mysql_connect("**************" ,$user,$password);
@mysql_select_db($database) or die( "FATAL ERROR");
$usertrying = $_POST[username];
$ptrying = $_POST[password];
$secured_password = sha1($ptrying);
$user_check = "SELECT USERNAME FROM USERS WHERE USERNAME = '$usertrying'";
$result = mysql_query ($user_check);
$username_correct = false;
$password_correct = false;
while($row = mysql_fetch_array($result) )
{
if ($row[uSERNAME] == $usertrying)
{
$username_correct = true;
break;
}
}
if ($username_correct)
{
$pw_check = "SELECT PASSWORD FROM USERS WHERE USERNAME = '$usertrying'";
$result = mysql_query ($pw_check);
if ($result == $secured_password)
{
$password_correct = true;
}
}
if(($username_correct == "true") && ($password_correct == "true"))
{
session_register('username');
header('Location:http://*********.com/privatedata.php');
}
else
{
header('Location:http://**********.com/login.php');
}
mysql_close($conn);
?>
Charlie Holder. I'll have a look at your code now. Thanks for helping.
-
Okay. I'm pretty new to Php. My course is all about Java (wont make any friends by saying that). I'm trying to create a pretty simple login for my website; nothing special at all because I am a newbie/n00b (your bread, your butter). Anyway, my registration page works well; delivers the results I want to etc. The problem is my login page. And for some reason that I'm sure I'll be banging my head off over later, its not working. At first, it was allowing anyone to log in, which was bad; now, its not allowing anyone to log in.
Here's the code. Please have a look over and let me know. I want to learn off you guys from my mistakes.
<?php session_start();
$user="************";
$password="**********";
$database="**********";
mysql_connect("*************" ,$user,$password);
@mysql_select_db($database) or die( "FATAL ERROR");
$usertrying = $_POST[username];
$ptrying = $_POST[password];
$secured_password = sha1($password);
$user_check = "SELECT USERNAME FROM USERS WHERE USERNAME = '$username'";
$result = mysql_query ($user_check);
$username_correct = false;
$password_correct = false;
while($row = mysql_fetch_array($result) )
{
if ($row[uSERNAME] == $usertrying)
{
$username_correct = true;
break;
}
}
if ($username_correct)
{
$pw_check = "SELECT PASSWORD FROM USERS WHERE USERNAME = '$username'";
$result = mysql_query ($pw_check);
if ($result == $secured_password)
{
$password_correct = true;
}
}
if($username_correct && $password_correct)
{
session_register('username');
header('Location:http://**********.com/privatedata.php');
}
else
{
header('Location:http://*********.com/login.php');
}
mysql_close($conn);
?>
-
Thank you very much!
-
Hey all, I'm new to this. The other day I decided to start with PHP. I enabled my ISS on Vista and it works etc. I installed PHP 5. Now.. I'm used to Tomcat and JSP. So... The one question that I have been looking to have answered is this:
Where is my root folder? I mean, I've been Googling all evening and haven't found one piece of information about it. In Tomcat, I just place the file in my root folder and hey presto. In my PHP folder however, there seems to be no sign of it? Anyone willing to help me with this unbelievably simple question?
Regards,
Wayne.
[SOLVED] Need help guys. Please.
in PHP Coding Help
Posted
Sorry, here's the code with the "or die" fixed. Another stupid mistake. Its just that I've been rearranging this piece of code for ages trying to figure out whats wrong. Its still not redirecting and I'm getting no error.